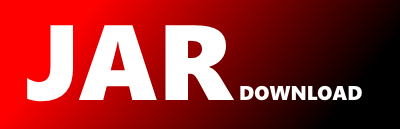
com.google.api.services.jobs.v4.CloudTalentSolution Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.jobs.v4;
/**
* Service definition for CloudTalentSolution (v4).
*
*
* Cloud Talent Solution provides the capability to create, read, update, and delete job postings, as well as search jobs based on keywords and filters.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudTalentSolutionRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudTalentSolution extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Talent Solution API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://jobs.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://jobs.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudTalentSolution(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudTalentSolution(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code CloudTalentSolution jobs = new CloudTalentSolution(...);}
* {@code CloudTalentSolution.Projects.List request = jobs.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudTalentSolution jobs = new CloudTalentSolution(...);}
* {@code CloudTalentSolution.Operations.List request = jobs.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Tenants collection.
*
* The typical use is:
*
* {@code CloudTalentSolution jobs = new CloudTalentSolution(...);}
* {@code CloudTalentSolution.Tenants.List request = jobs.tenants().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tenants tenants() {
return new Tenants();
}
/**
* The "tenants" collection of methods.
*/
public class Tenants {
/**
* Completes the specified prefix with keyword suggestions. Intended for use by a job search auto-
* complete search box.
*
* Create a request for the method "tenants.completeQuery".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link CompleteQuery#execute()} method to invoke the remote operation.
*
* @param tenant Required. Resource name of tenant the completion is performed within. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @return the request
*/
public CompleteQuery completeQuery(java.lang.String tenant) throws java.io.IOException {
CompleteQuery result = new CompleteQuery(tenant);
initialize(result);
return result;
}
public class CompleteQuery extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+tenant}:completeQuery";
private final java.util.regex.Pattern TENANT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Completes the specified prefix with keyword suggestions. Intended for use by a job search auto-
* complete search box.
*
* Create a request for the method "tenants.completeQuery".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link CompleteQuery#execute()} method to invoke the remote operation.
* {@link CompleteQuery#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param tenant Required. Resource name of tenant the completion is performed within. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @since 1.13
*/
protected CompleteQuery(java.lang.String tenant) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.CompleteQueryResponse.class);
this.tenant = com.google.api.client.util.Preconditions.checkNotNull(tenant, "Required parameter tenant must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TENANT_PATTERN.matcher(tenant).matches(),
"Parameter tenant must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public CompleteQuery set$Xgafv(java.lang.String $Xgafv) {
return (CompleteQuery) super.set$Xgafv($Xgafv);
}
@Override
public CompleteQuery setAccessToken(java.lang.String accessToken) {
return (CompleteQuery) super.setAccessToken(accessToken);
}
@Override
public CompleteQuery setAlt(java.lang.String alt) {
return (CompleteQuery) super.setAlt(alt);
}
@Override
public CompleteQuery setCallback(java.lang.String callback) {
return (CompleteQuery) super.setCallback(callback);
}
@Override
public CompleteQuery setFields(java.lang.String fields) {
return (CompleteQuery) super.setFields(fields);
}
@Override
public CompleteQuery setKey(java.lang.String key) {
return (CompleteQuery) super.setKey(key);
}
@Override
public CompleteQuery setOauthToken(java.lang.String oauthToken) {
return (CompleteQuery) super.setOauthToken(oauthToken);
}
@Override
public CompleteQuery setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CompleteQuery) super.setPrettyPrint(prettyPrint);
}
@Override
public CompleteQuery setQuotaUser(java.lang.String quotaUser) {
return (CompleteQuery) super.setQuotaUser(quotaUser);
}
@Override
public CompleteQuery setUploadType(java.lang.String uploadType) {
return (CompleteQuery) super.setUploadType(uploadType);
}
@Override
public CompleteQuery setUploadProtocol(java.lang.String uploadProtocol) {
return (CompleteQuery) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of tenant the completion is performed within. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String tenant;
/** Required. Resource name of tenant the completion is performed within. The format is
"projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public java.lang.String getTenant() {
return tenant;
}
/**
* Required. Resource name of tenant the completion is performed within. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public CompleteQuery setTenant(java.lang.String tenant) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TENANT_PATTERN.matcher(tenant).matches(),
"Parameter tenant must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.tenant = tenant;
return this;
}
/**
* If provided, restricts completion to specified company. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
*/
@com.google.api.client.util.Key
private java.lang.String company;
/** If provided, restricts completion to specified company. The format is
"projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
"projects/foo/tenants/bar/companies/baz".
*/
public java.lang.String getCompany() {
return company;
}
/**
* If provided, restricts completion to specified company. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
*/
public CompleteQuery setCompany(java.lang.String company) {
this.company = company;
return this;
}
/**
* The list of languages of the query. This is the BCP-47 language code, such as "en-US" or
* "sr-Latn". For more information, see [Tags for Identifying
* Languages](https://tools.ietf.org/html/bcp47). The maximum number of allowed characters
* is 255.
*/
@com.google.api.client.util.Key
private java.util.List languageCodes;
/** The list of languages of the query. This is the BCP-47 language code, such as "en-US" or "sr-Latn".
For more information, see [Tags for Identifying Languages](https://tools.ietf.org/html/bcp47). The
maximum number of allowed characters is 255.
*/
public java.util.List getLanguageCodes() {
return languageCodes;
}
/**
* The list of languages of the query. This is the BCP-47 language code, such as "en-US" or
* "sr-Latn". For more information, see [Tags for Identifying
* Languages](https://tools.ietf.org/html/bcp47). The maximum number of allowed characters
* is 255.
*/
public CompleteQuery setLanguageCodes(java.util.List languageCodes) {
this.languageCodes = languageCodes;
return this;
}
/** Required. Completion result count. The maximum allowed page size is 10. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Required. Completion result count. The maximum allowed page size is 10.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Required. Completion result count. The maximum allowed page size is 10. */
public CompleteQuery setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Required. The query used to generate suggestions. The maximum number of allowed
* characters is 255.
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. The query used to generate suggestions. The maximum number of allowed characters is 255.
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. The query used to generate suggestions. The maximum number of allowed
* characters is 255.
*/
public CompleteQuery setQuery(java.lang.String query) {
this.query = query;
return this;
}
/** The scope of the completion. The defaults is CompletionScope.PUBLIC. */
@com.google.api.client.util.Key
private java.lang.String scope;
/** The scope of the completion. The defaults is CompletionScope.PUBLIC.
*/
public java.lang.String getScope() {
return scope;
}
/** The scope of the completion. The defaults is CompletionScope.PUBLIC. */
public CompleteQuery setScope(java.lang.String scope) {
this.scope = scope;
return this;
}
/** The completion topic. The default is CompletionType.COMBINED. */
@com.google.api.client.util.Key
private java.lang.String type;
/** The completion topic. The default is CompletionType.COMBINED.
*/
public java.lang.String getType() {
return type;
}
/** The completion topic. The default is CompletionType.COMBINED. */
public CompleteQuery setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public CompleteQuery set(String parameterName, Object value) {
return (CompleteQuery) super.set(parameterName, value);
}
}
/**
* Creates a new tenant entity.
*
* Create a request for the method "tenants.create".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
* @param content the {@link com.google.api.services.jobs.v4.model.Tenant}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.jobs.v4.model.Tenant content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/tenants";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Creates a new tenant entity.
*
* Create a request for the method "tenants.create".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
* @param content the {@link com.google.api.services.jobs.v4.model.Tenant}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.jobs.v4.model.Tenant content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.Tenant.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the project under which the tenant is created. The format is
"projects/{project_id}", for example, "projects/foo".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes specified tenant.
*
* Create a request for the method "tenants.delete".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the tenant to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Deletes specified tenant.
*
* Create a request for the method "tenants.delete".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the tenant to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudTalentSolution.this, "DELETE", REST_PATH, null, com.google.api.services.jobs.v4.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the tenant to be deleted. The format is
"projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the tenant to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves specified tenant.
*
* Create a request for the method "tenants.get".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the tenant to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Retrieves specified tenant.
*
* Create a request for the method "tenants.get".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the tenant to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.Tenant.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the tenant to be retrieved. The format is
"projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the tenant to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all tenants associated with the project.
*
* Create a request for the method "tenants.list".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/tenants";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists all tenants associated with the project.
*
* Create a request for the method "tenants.list".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.ListTenantsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the project under which the tenant is created. The format is
"projects/{project_id}", for example, "projects/foo".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the project under which the tenant is created. The format is
* "projects/{project_id}", for example, "projects/foo".
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of tenants to be returned, at most 100. Default is 100 if a non-
* positive number is provided.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of tenants to be returned, at most 100. Default is 100 if a non-positive number
is provided.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of tenants to be returned, at most 100. Default is 100 if a non-
* positive number is provided.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The starting indicator from which to return results. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The starting indicator from which to return results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The starting indicator from which to return results. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates specified tenant.
*
* Create a request for the method "tenants.patch".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required during tenant update. The resource name for a tenant. This is generated by the service when
* a tenant is created. The format is "projects/{project_id}/tenants/{tenant_id}", for
* example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.Tenant}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.jobs.v4.model.Tenant content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Updates specified tenant.
*
* Create a request for the method "tenants.patch".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required during tenant update. The resource name for a tenant. This is generated by the service when
* a tenant is created. The format is "projects/{project_id}/tenants/{tenant_id}", for
* example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.Tenant}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.jobs.v4.model.Tenant content) {
super(CloudTalentSolution.this, "PATCH", REST_PATH, content, com.google.api.services.jobs.v4.model.Tenant.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required during tenant update. The resource name for a tenant. This is generated by the
* service when a tenant is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required during tenant update. The resource name for a tenant. This is generated by the service
when a tenant is created. The format is "projects/{project_id}/tenants/{tenant_id}", for example,
"projects/foo/tenants/bar".
*/
public java.lang.String getName() {
return name;
}
/**
* Required during tenant update. The resource name for a tenant. This is generated by the
* service when a tenant is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.name = name;
return this;
}
/**
* Strongly recommended for the best service experience. If update_mask is provided, only
* the specified fields in tenant are updated. Otherwise all the fields are updated. A field
* mask to specify the tenant fields to be updated. Only top level fields of Tenant are
* supported.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Strongly recommended for the best service experience. If update_mask is provided, only the
specified fields in tenant are updated. Otherwise all the fields are updated. A field mask to
specify the tenant fields to be updated. Only top level fields of Tenant are supported.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Strongly recommended for the best service experience. If update_mask is provided, only
* the specified fields in tenant are updated. Otherwise all the fields are updated. A field
* mask to specify the tenant fields to be updated. Only top level fields of Tenant are
* supported.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ClientEvents collection.
*
* The typical use is:
*
* {@code CloudTalentSolution jobs = new CloudTalentSolution(...);}
* {@code CloudTalentSolution.ClientEvents.List request = jobs.clientEvents().list(parameters ...)}
*
*
* @return the resource collection
*/
public ClientEvents clientEvents() {
return new ClientEvents();
}
/**
* The "clientEvents" collection of methods.
*/
public class ClientEvents {
/**
* Report events issued when end user interacts with customer's application that uses Cloud Talent
* Solution. You may inspect the created events in [self service
* tools](https://console.cloud.google.com/talent-solution/overview). [Learn
* more](https://cloud.google.com/talent-solution/docs/management-tools) about self service tools.
*
* Create a request for the method "clientEvents.create".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the tenant under which the event is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.ClientEvent}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.jobs.v4.model.ClientEvent content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/clientEvents";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Report events issued when end user interacts with customer's application that uses Cloud Talent
* Solution. You may inspect the created events in [self service
* tools](https://console.cloud.google.com/talent-solution/overview). [Learn
* more](https://cloud.google.com/talent-solution/docs/management-tools) about self service tools.
*
* Create a request for the method "clientEvents.create".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the tenant under which the event is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.ClientEvent}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.jobs.v4.model.ClientEvent content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.ClientEvent.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the tenant under which the event is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the tenant under which the event is created. The format is
"projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the tenant under which the event is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Companies collection.
*
* The typical use is:
*
* {@code CloudTalentSolution jobs = new CloudTalentSolution(...);}
* {@code CloudTalentSolution.Companies.List request = jobs.companies().list(parameters ...)}
*
*
* @return the resource collection
*/
public Companies companies() {
return new Companies();
}
/**
* The "companies" collection of methods.
*/
public class Companies {
/**
* Creates a new company entity.
*
* Create a request for the method "companies.create".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.Company}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.jobs.v4.model.Company content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/companies";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Creates a new company entity.
*
* Create a request for the method "companies.create".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.Company}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.jobs.v4.model.Company content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.Company.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the tenant under which the company is created. The format is
"projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes specified company. Prerequisite: The company has no jobs associated with it.
*
* Create a request for the method "companies.delete".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the company to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
/**
* Deletes specified company. Prerequisite: The company has no jobs associated with it.
*
* Create a request for the method "companies.delete".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the company to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudTalentSolution.this, "DELETE", REST_PATH, null, com.google.api.services.jobs.v4.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the company to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the company to be deleted. The format is
"projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
"projects/foo/tenants/bar/companies/baz".
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the company to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves specified company.
*
* Create a request for the method "companies.get".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the company to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/api-test-project/tenants/foo/companies/bar".
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
/**
* Retrieves specified company.
*
* Create a request for the method "companies.get".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the company to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/api-test-project/tenants/foo/companies/bar".
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.Company.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the company to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/api-test-project/tenants/foo/companies/bar".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the company to be retrieved. The format is
"projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example, "projects/api-
test-project/tenants/foo/companies/bar".
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the company to be retrieved. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/api-test-project/tenants/foo/companies/bar".
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all companies associated with the project.
*
* Create a request for the method "companies.list".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/companies";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Lists all companies associated with the project.
*
* Create a request for the method "companies.list".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.ListCompaniesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the tenant under which the company is created. The format is
"projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the tenant under which the company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}", for example, "projects/foo/tenants/bar".
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of companies to be returned, at most 100. Default is 100 if a non-
* positive number is provided.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of companies to be returned, at most 100. Default is 100 if a non-positive
number is provided.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of companies to be returned, at most 100. Default is 100 if a non-
* positive number is provided.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The starting indicator from which to return results. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The starting indicator from which to return results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The starting indicator from which to return results. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Set to true if the companies requested must have open jobs. Defaults to false. If true,
* at most page_size of companies are fetched, among which only those with open jobs are
* returned.
*/
@com.google.api.client.util.Key
private java.lang.Boolean requireOpenJobs;
/** Set to true if the companies requested must have open jobs. Defaults to false. If true, at most
page_size of companies are fetched, among which only those with open jobs are returned.
*/
public java.lang.Boolean getRequireOpenJobs() {
return requireOpenJobs;
}
/**
* Set to true if the companies requested must have open jobs. Defaults to false. If true,
* at most page_size of companies are fetched, among which only those with open jobs are
* returned.
*/
public List setRequireOpenJobs(java.lang.Boolean requireOpenJobs) {
this.requireOpenJobs = requireOpenJobs;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates specified company.
*
* Create a request for the method "companies.patch".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required during company update. The resource name for a company. This is generated by the service
* when a company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
* @param content the {@link com.google.api.services.jobs.v4.model.Company}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.jobs.v4.model.Company content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
/**
* Updates specified company.
*
* Create a request for the method "companies.patch".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required during company update. The resource name for a company. This is generated by the service
* when a company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
* @param content the {@link com.google.api.services.jobs.v4.model.Company}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.jobs.v4.model.Company content) {
super(CloudTalentSolution.this, "PATCH", REST_PATH, content, com.google.api.services.jobs.v4.model.Company.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required during company update. The resource name for a company. This is generated by
* the service when a company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required during company update. The resource name for a company. This is generated by the service
when a company is created. The format is
"projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
"projects/foo/tenants/bar/companies/baz".
*/
public java.lang.String getName() {
return name;
}
/**
* Required during company update. The resource name for a company. This is generated by
* the service when a company is created. The format is
* "projects/{project_id}/tenants/{tenant_id}/companies/{company_id}", for example,
* "projects/foo/tenants/bar/companies/baz".
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/companies/[^/]+$");
}
this.name = name;
return this;
}
/**
* Strongly recommended for the best service experience. If update_mask is provided, only
* the specified fields in company are updated. Otherwise all the fields are updated. A
* field mask to specify the company fields to be updated. Only top level fields of
* Company are supported.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Strongly recommended for the best service experience. If update_mask is provided, only the
specified fields in company are updated. Otherwise all the fields are updated. A field mask to
specify the company fields to be updated. Only top level fields of Company are supported.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Strongly recommended for the best service experience. If update_mask is provided, only
* the specified fields in company are updated. Otherwise all the fields are updated. A
* field mask to specify the company fields to be updated. Only top level fields of
* Company are supported.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Jobs collection.
*
* The typical use is:
*
* {@code CloudTalentSolution jobs = new CloudTalentSolution(...);}
* {@code CloudTalentSolution.Jobs.List request = jobs.jobs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Jobs jobs() {
return new Jobs();
}
/**
* The "jobs" collection of methods.
*/
public class Jobs {
/**
* Begins executing a batch create jobs operation.
*
* Create a request for the method "jobs.batchCreate".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link BatchCreate#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.BatchCreateJobsRequest}
* @return the request
*/
public BatchCreate batchCreate(java.lang.String parent, com.google.api.services.jobs.v4.model.BatchCreateJobsRequest content) throws java.io.IOException {
BatchCreate result = new BatchCreate(parent, content);
initialize(result);
return result;
}
public class BatchCreate extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/jobs:batchCreate";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Begins executing a batch create jobs operation.
*
* Create a request for the method "jobs.batchCreate".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link BatchCreate#execute()} method to invoke the remote operation.
* {@link
* BatchCreate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.BatchCreateJobsRequest}
* @since 1.13
*/
protected BatchCreate(java.lang.String parent, com.google.api.services.jobs.v4.model.BatchCreateJobsRequest content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public BatchCreate set$Xgafv(java.lang.String $Xgafv) {
return (BatchCreate) super.set$Xgafv($Xgafv);
}
@Override
public BatchCreate setAccessToken(java.lang.String accessToken) {
return (BatchCreate) super.setAccessToken(accessToken);
}
@Override
public BatchCreate setAlt(java.lang.String alt) {
return (BatchCreate) super.setAlt(alt);
}
@Override
public BatchCreate setCallback(java.lang.String callback) {
return (BatchCreate) super.setCallback(callback);
}
@Override
public BatchCreate setFields(java.lang.String fields) {
return (BatchCreate) super.setFields(fields);
}
@Override
public BatchCreate setKey(java.lang.String key) {
return (BatchCreate) super.setKey(key);
}
@Override
public BatchCreate setOauthToken(java.lang.String oauthToken) {
return (BatchCreate) super.setOauthToken(oauthToken);
}
@Override
public BatchCreate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchCreate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchCreate setQuotaUser(java.lang.String quotaUser) {
return (BatchCreate) super.setQuotaUser(quotaUser);
}
@Override
public BatchCreate setUploadType(java.lang.String uploadType) {
return (BatchCreate) super.setUploadType(uploadType);
}
@Override
public BatchCreate setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchCreate) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the tenant under which the job is created. The format is
"projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public BatchCreate setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchCreate set(String parameterName, Object value) {
return (BatchCreate) super.set(parameterName, value);
}
}
/**
* Begins executing a batch delete jobs operation.
*
* Create a request for the method "jobs.batchDelete".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar". The
* parent of all of the jobs specified in `names` must match this field.
* @param content the {@link com.google.api.services.jobs.v4.model.BatchDeleteJobsRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String parent, com.google.api.services.jobs.v4.model.BatchDeleteJobsRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(parent, content);
initialize(result);
return result;
}
public class BatchDelete extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/jobs:batchDelete";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Begins executing a batch delete jobs operation.
*
* Create a request for the method "jobs.batchDelete".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
* {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar". The
* parent of all of the jobs specified in `names` must match this field.
* @param content the {@link com.google.api.services.jobs.v4.model.BatchDeleteJobsRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String parent, com.google.api.services.jobs.v4.model.BatchDeleteJobsRequest content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public BatchDelete set$Xgafv(java.lang.String $Xgafv) {
return (BatchDelete) super.set$Xgafv($Xgafv);
}
@Override
public BatchDelete setAccessToken(java.lang.String accessToken) {
return (BatchDelete) super.setAccessToken(accessToken);
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setCallback(java.lang.String callback) {
return (BatchDelete) super.setCallback(callback);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUploadType(java.lang.String uploadType) {
return (BatchDelete) super.setUploadType(uploadType);
}
@Override
public BatchDelete setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchDelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* The parent of all of the jobs specified in `names` must match this field.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the tenant under which the job is created. The format is
"projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar". The parent of
all of the jobs specified in `names` must match this field.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* The parent of all of the jobs specified in `names` must match this field.
*/
public BatchDelete setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Begins executing a batch update jobs operation.
*
* Create a request for the method "jobs.batchUpdate".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.BatchUpdateJobsRequest}
* @return the request
*/
public BatchUpdate batchUpdate(java.lang.String parent, com.google.api.services.jobs.v4.model.BatchUpdateJobsRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(parent, content);
initialize(result);
return result;
}
public class BatchUpdate extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/jobs:batchUpdate";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Begins executing a batch update jobs operation.
*
* Create a request for the method "jobs.batchUpdate".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
* {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.BatchUpdateJobsRequest}
* @since 1.13
*/
protected BatchUpdate(java.lang.String parent, com.google.api.services.jobs.v4.model.BatchUpdateJobsRequest content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public BatchUpdate set$Xgafv(java.lang.String $Xgafv) {
return (BatchUpdate) super.set$Xgafv($Xgafv);
}
@Override
public BatchUpdate setAccessToken(java.lang.String accessToken) {
return (BatchUpdate) super.setAccessToken(accessToken);
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setCallback(java.lang.String callback) {
return (BatchUpdate) super.setCallback(callback);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUploadType(java.lang.String uploadType) {
return (BatchUpdate) super.setUploadType(uploadType);
}
@Override
public BatchUpdate setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchUpdate) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the tenant under which the job is created. The format is
"projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public BatchUpdate setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
/**
* Creates a new job. Typically, the job becomes searchable within 10 seconds, but it may take up to
* 5 minutes.
*
* Create a request for the method "jobs.create".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.Job}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.jobs.v4.model.Job content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/jobs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Creates a new job. Typically, the job becomes searchable within 10 seconds, but it may take up
* to 5 minutes.
*
* Create a request for the method "jobs.create".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.Job}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.jobs.v4.model.Job content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.Job.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the tenant under which the job is created. The format is
"projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified job. Typically, the job becomes unsearchable within 10 seconds, but it may
* take up to 5 minutes.
*
* Create a request for the method "jobs.delete".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the job to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
/**
* Deletes the specified job. Typically, the job becomes unsearchable within 10 seconds, but it
* may take up to 5 minutes.
*
* Create a request for the method "jobs.delete".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the job to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudTalentSolution.this, "DELETE", REST_PATH, null, com.google.api.services.jobs.v4.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the job to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the job to be deleted. The format is
"projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
"projects/foo/tenants/bar/jobs/baz".
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the job to be deleted. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified job, whose status is OPEN or recently EXPIRED within the last 90 days.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the job to retrieve. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
/**
* Retrieves the specified job, whose status is OPEN or recently EXPIRED within the last 90 days.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the job to retrieve. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.Job.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the job to retrieve. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the job to retrieve. The format is
"projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
"projects/foo/tenants/bar/jobs/baz".
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the job to retrieve. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz".
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists jobs by filter.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/jobs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Lists jobs by filter.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudTalentSolution.this, "GET", REST_PATH, null, com.google.api.services.jobs.v4.model.ListJobsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the tenant under which the job is created. The format is
"projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the tenant under which the job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The filter string specifies the jobs to be enumerated. Supported operator: =,
* AND The fields eligible for filtering are: * `companyName` * `requisitionId` * `status`
* Available values: OPEN, EXPIRED, ALL. Defaults to OPEN if no value is specified. At
* least one of `companyName` and `requisitionId` must present or an INVALID_ARGUMENT
* error is thrown. Sample Query: * companyName = "projects/foo/tenants/bar/companies/baz"
* * companyName = "projects/foo/tenants/bar/companies/baz" AND requisitionId = "req-1" *
* companyName = "projects/foo/tenants/bar/companies/baz" AND status = "EXPIRED" *
* requisitionId = "req-1" * requisitionId = "req-1" AND status = "EXPIRED"
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Required. The filter string specifies the jobs to be enumerated. Supported operator: =, AND The
fields eligible for filtering are: * `companyName` * `requisitionId` * `status` Available values:
OPEN, EXPIRED, ALL. Defaults to OPEN if no value is specified. At least one of `companyName` and
`requisitionId` must present or an INVALID_ARGUMENT error is thrown. Sample Query: * companyName =
"projects/foo/tenants/bar/companies/baz" * companyName = "projects/foo/tenants/bar/companies/baz"
AND requisitionId = "req-1" * companyName = "projects/foo/tenants/bar/companies/baz" AND status =
"EXPIRED" * requisitionId = "req-1" * requisitionId = "req-1" AND status = "EXPIRED"
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Required. The filter string specifies the jobs to be enumerated. Supported operator: =,
* AND The fields eligible for filtering are: * `companyName` * `requisitionId` * `status`
* Available values: OPEN, EXPIRED, ALL. Defaults to OPEN if no value is specified. At
* least one of `companyName` and `requisitionId` must present or an INVALID_ARGUMENT
* error is thrown. Sample Query: * companyName = "projects/foo/tenants/bar/companies/baz"
* * companyName = "projects/foo/tenants/bar/companies/baz" AND requisitionId = "req-1" *
* companyName = "projects/foo/tenants/bar/companies/baz" AND status = "EXPIRED" *
* requisitionId = "req-1" * requisitionId = "req-1" AND status = "EXPIRED"
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The desired job attributes returned for jobs in the search response. Defaults to
* JobView.JOB_VIEW_FULL if no value is specified.
*/
@com.google.api.client.util.Key
private java.lang.String jobView;
/** The desired job attributes returned for jobs in the search response. Defaults to
JobView.JOB_VIEW_FULL if no value is specified.
*/
public java.lang.String getJobView() {
return jobView;
}
/**
* The desired job attributes returned for jobs in the search response. Defaults to
* JobView.JOB_VIEW_FULL if no value is specified.
*/
public List setJobView(java.lang.String jobView) {
this.jobView = jobView;
return this;
}
/**
* The maximum number of jobs to be returned per page of results. If job_view is set to
* JobView.JOB_VIEW_ID_ONLY, the maximum allowed page size is 1000. Otherwise, the maximum
* allowed page size is 100. Default is 100 if empty or a number < 1 is specified.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of jobs to be returned per page of results. If job_view is set to
JobView.JOB_VIEW_ID_ONLY, the maximum allowed page size is 1000. Otherwise, the maximum allowed
page size is 100. Default is 100 if empty or a number < 1 is specified.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of jobs to be returned per page of results. If job_view is set to
* JobView.JOB_VIEW_ID_ONLY, the maximum allowed page size is 1000. Otherwise, the maximum
* allowed page size is 100. Default is 100 if empty or a number < 1 is specified.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The starting point of a query result. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The starting point of a query result.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The starting point of a query result. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates specified job. Typically, updated contents become visible in search results within 10
* seconds, but it may take up to 5 minutes.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required during job update. The resource name for the job. This is generated by the service when a
* job is created. The format is "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}".
* For example, "projects/foo/tenants/bar/jobs/baz". Use of this field in job queries and API
* calls is preferred over the use of requisition_id since this value is unique.
* @param content the {@link com.google.api.services.jobs.v4.model.Job}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.jobs.v4.model.Job content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
/**
* Updates specified job. Typically, updated contents become visible in search results within 10
* seconds, but it may take up to 5 minutes.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required during job update. The resource name for the job. This is generated by the service when a
* job is created. The format is "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}".
* For example, "projects/foo/tenants/bar/jobs/baz". Use of this field in job queries and API
* calls is preferred over the use of requisition_id since this value is unique.
* @param content the {@link com.google.api.services.jobs.v4.model.Job}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.jobs.v4.model.Job content) {
super(CloudTalentSolution.this, "PATCH", REST_PATH, content, com.google.api.services.jobs.v4.model.Job.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required during job update. The resource name for the job. This is generated by the
* service when a job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz". Use of this field in job queries and API calls is
* preferred over the use of requisition_id since this value is unique.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required during job update. The resource name for the job. This is generated by the service when a
job is created. The format is "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For
example, "projects/foo/tenants/bar/jobs/baz". Use of this field in job queries and API calls is
preferred over the use of requisition_id since this value is unique.
*/
public java.lang.String getName() {
return name;
}
/**
* Required during job update. The resource name for the job. This is generated by the
* service when a job is created. The format is
* "projects/{project_id}/tenants/{tenant_id}/jobs/{job_id}". For example,
* "projects/foo/tenants/bar/jobs/baz". Use of this field in job queries and API calls is
* preferred over the use of requisition_id since this value is unique.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+/jobs/[^/]+$");
}
this.name = name;
return this;
}
/**
* Strongly recommended for the best service experience. If update_mask is provided, only
* the specified fields in job are updated. Otherwise all the fields are updated. A field
* mask to restrict the fields that are updated. Only top level fields of Job are
* supported.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Strongly recommended for the best service experience. If update_mask is provided, only the
specified fields in job are updated. Otherwise all the fields are updated. A field mask to restrict
the fields that are updated. Only top level fields of Job are supported.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Strongly recommended for the best service experience. If update_mask is provided, only
* the specified fields in job are updated. Otherwise all the fields are updated. A field
* mask to restrict the fields that are updated. Only top level fields of Job are
* supported.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Searches for jobs using the provided SearchJobsRequest. This call constrains the visibility of
* jobs present in the database, and only returns jobs that the caller has permission to search
* against.
*
* Create a request for the method "jobs.search".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.SearchJobsRequest}
* @return the request
*/
public Search search(java.lang.String parent, com.google.api.services.jobs.v4.model.SearchJobsRequest content) throws java.io.IOException {
Search result = new Search(parent, content);
initialize(result);
return result;
}
public class Search extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/jobs:search";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Searches for jobs using the provided SearchJobsRequest. This call constrains the visibility of
* jobs present in the database, and only returns jobs that the caller has permission to search
* against.
*
* Create a request for the method "jobs.search".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation. {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.SearchJobsRequest}
* @since 1.13
*/
protected Search(java.lang.String parent, com.google.api.services.jobs.v4.model.SearchJobsRequest content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.SearchJobsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the tenant to search within. The format is
"projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public Search setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Searches for jobs using the provided SearchJobsRequest. This API call is intended for the use
* case of targeting passive job seekers (for example, job seekers who have signed up to receive
* email alerts about potential job opportunities), it has different algorithmic adjustments that
* are designed to specifically target passive job seekers. This call constrains the visibility of
* jobs present in the database, and only returns jobs the caller has permission to search against.
*
* Create a request for the method "jobs.searchForAlert".
*
* This request holds the parameters needed by the jobs server. After setting any optional
* parameters, call the {@link SearchForAlert#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.SearchJobsRequest}
* @return the request
*/
public SearchForAlert searchForAlert(java.lang.String parent, com.google.api.services.jobs.v4.model.SearchJobsRequest content) throws java.io.IOException {
SearchForAlert result = new SearchForAlert(parent, content);
initialize(result);
return result;
}
public class SearchForAlert extends CloudTalentSolutionRequest {
private static final String REST_PATH = "v4/{+parent}/jobs:searchForAlert";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/tenants/[^/]+$");
/**
* Searches for jobs using the provided SearchJobsRequest. This API call is intended for the use
* case of targeting passive job seekers (for example, job seekers who have signed up to receive
* email alerts about potential job opportunities), it has different algorithmic adjustments that
* are designed to specifically target passive job seekers. This call constrains the visibility of
* jobs present in the database, and only returns jobs the caller has permission to search
* against.
*
* Create a request for the method "jobs.searchForAlert".
*
* This request holds the parameters needed by the the jobs server. After setting any optional
* parameters, call the {@link SearchForAlert#execute()} method to invoke the remote operation.
* {@link SearchForAlert#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param parent Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
* @param content the {@link com.google.api.services.jobs.v4.model.SearchJobsRequest}
* @since 1.13
*/
protected SearchForAlert(java.lang.String parent, com.google.api.services.jobs.v4.model.SearchJobsRequest content) {
super(CloudTalentSolution.this, "POST", REST_PATH, content, com.google.api.services.jobs.v4.model.SearchJobsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
}
@Override
public SearchForAlert set$Xgafv(java.lang.String $Xgafv) {
return (SearchForAlert) super.set$Xgafv($Xgafv);
}
@Override
public SearchForAlert setAccessToken(java.lang.String accessToken) {
return (SearchForAlert) super.setAccessToken(accessToken);
}
@Override
public SearchForAlert setAlt(java.lang.String alt) {
return (SearchForAlert) super.setAlt(alt);
}
@Override
public SearchForAlert setCallback(java.lang.String callback) {
return (SearchForAlert) super.setCallback(callback);
}
@Override
public SearchForAlert setFields(java.lang.String fields) {
return (SearchForAlert) super.setFields(fields);
}
@Override
public SearchForAlert setKey(java.lang.String key) {
return (SearchForAlert) super.setKey(key);
}
@Override
public SearchForAlert setOauthToken(java.lang.String oauthToken) {
return (SearchForAlert) super.setOauthToken(oauthToken);
}
@Override
public SearchForAlert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchForAlert) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchForAlert setQuotaUser(java.lang.String quotaUser) {
return (SearchForAlert) super.setQuotaUser(quotaUser);
}
@Override
public SearchForAlert setUploadType(java.lang.String uploadType) {
return (SearchForAlert) super.setUploadType(uploadType);
}
@Override
public SearchForAlert setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchForAlert) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the tenant to search within. The format is
"projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the tenant to search within. The format is
* "projects/{project_id}/tenants/{tenant_id}". For example, "projects/foo/tenants/bar".
*/
public SearchForAlert setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/tenants/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public SearchForAlert set(String parameterName, Object value) {
return (SearchForAlert) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link CloudTalentSolution}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudTalentSolution}. */
@Override
public CloudTalentSolution build() {
return new CloudTalentSolution(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudTalentSolutionRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudTalentSolutionRequestInitializer(
CloudTalentSolutionRequestInitializer cloudtalentsolutionRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudtalentsolutionRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}