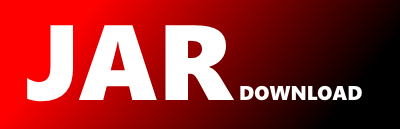
com.google.api.services.logging.v2.Logging Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.logging.v2;
/**
* Service definition for Logging (v2).
*
*
* Writes log entries and manages your Cloud Logging configuration.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link LoggingRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Logging extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Logging API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://logging.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://logging.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Logging(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Logging(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the BillingAccounts collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.BillingAccounts.List request = logging.billingAccounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public BillingAccounts billingAccounts() {
return new BillingAccounts();
}
/**
* The "billingAccounts" collection of methods.
*/
public class BillingAccounts {
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "billingAccounts.getCmekSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @return the request
*/
public GetCmekSettings getCmekSettings(java.lang.String name) throws java.io.IOException {
GetCmekSettings result = new GetCmekSettings(name);
initialize(result);
return result;
}
public class GetCmekSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/cmekSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "billingAccounts.getCmekSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
* {@link GetCmekSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @since 1.13
*/
protected GetCmekSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.CmekSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetCmekSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetCmekSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetCmekSettings setAccessToken(java.lang.String accessToken) {
return (GetCmekSettings) super.setAccessToken(accessToken);
}
@Override
public GetCmekSettings setAlt(java.lang.String alt) {
return (GetCmekSettings) super.setAlt(alt);
}
@Override
public GetCmekSettings setCallback(java.lang.String callback) {
return (GetCmekSettings) super.setCallback(callback);
}
@Override
public GetCmekSettings setFields(java.lang.String fields) {
return (GetCmekSettings) super.setFields(fields);
}
@Override
public GetCmekSettings setKey(java.lang.String key) {
return (GetCmekSettings) super.setKey(key);
}
@Override
public GetCmekSettings setOauthToken(java.lang.String oauthToken) {
return (GetCmekSettings) super.setOauthToken(oauthToken);
}
@Override
public GetCmekSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetCmekSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetCmekSettings setQuotaUser(java.lang.String quotaUser) {
return (GetCmekSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetCmekSettings setUploadType(java.lang.String uploadType) {
return (GetCmekSettings) super.setUploadType(uploadType);
}
@Override
public GetCmekSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetCmekSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
"organizations/[ORGANIZATION_ID]/cmekSettings" "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings"
"folders/[FOLDER_ID]/cmekSettings" For example:"organizations/12345/cmekSettings"Note: CMEK for the
Log Router can be configured for Google Cloud projects, folders, organizations, and billing
accounts. Once configured for an organization, it applies to all projects and folders in the Google
Cloud organization.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
public GetCmekSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetCmekSettings set(String parameterName, Object value) {
return (GetCmekSettings) super.set(parameterName, value);
}
}
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "billingAccounts.getSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @return the request
*/
public GetSettings getSettings(java.lang.String name) throws java.io.IOException {
GetSettings result = new GetSettings(name);
initialize(result);
return result;
}
public class GetSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/settings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "billingAccounts.getSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
* {@link
* GetSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @since 1.13
*/
protected GetSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Settings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetSettings setAccessToken(java.lang.String accessToken) {
return (GetSettings) super.setAccessToken(accessToken);
}
@Override
public GetSettings setAlt(java.lang.String alt) {
return (GetSettings) super.setAlt(alt);
}
@Override
public GetSettings setCallback(java.lang.String callback) {
return (GetSettings) super.setCallback(callback);
}
@Override
public GetSettings setFields(java.lang.String fields) {
return (GetSettings) super.setFields(fields);
}
@Override
public GetSettings setKey(java.lang.String key) {
return (GetSettings) super.setKey(key);
}
@Override
public GetSettings setOauthToken(java.lang.String oauthToken) {
return (GetSettings) super.setOauthToken(oauthToken);
}
@Override
public GetSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSettings setQuotaUser(java.lang.String quotaUser) {
return (GetSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetSettings setUploadType(java.lang.String uploadType) {
return (GetSettings) super.setUploadType(uploadType);
}
@Override
public GetSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
"organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
"folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can be
retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public GetSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetSettings set(String parameterName, Object value) {
return (GetSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Exclusions collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Exclusions.List request = logging.exclusions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Exclusions exclusions() {
return new Exclusions();
}
/**
* The "exclusions" collection of methods.
*/
public class Exclusions {
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-logging-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/exclusions/[^/]+$");
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion to delete:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/exclusions/[^/]+$");
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/exclusions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListExclusionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/exclusions/[^/]+$");
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the exclusion to update:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. A non-empty list of fields to change in the existing exclusion. New values for the fields
are taken from the corresponding fields in the LogExclusion included in this request. Fields not
mentioned in update_mask are not changed and are ignored in the request.For example, to change the
filter and description of an exclusion, specify an update_mask of "filter,description".
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Locations.List request = logging.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
"displayName=tokyo", and is documented in more detail in AIP-160 (https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the next_page_token field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Buckets collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Buckets.List request = logging.buckets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Buckets buckets() {
return new Buckets();
}
/**
* The "buckets" collection of methods.
*/
public class Buckets {
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created, the
* bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created,
* the bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public Create setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has been
* created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public CreateAsync createAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
CreateAsync result = new CreateAsync(parent, content);
initialize(result);
return result;
}
public class CreateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets:createAsync";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has
* been created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
* {@link
* CreateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected CreateAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public CreateAsync set$Xgafv(java.lang.String $Xgafv) {
return (CreateAsync) super.set$Xgafv($Xgafv);
}
@Override
public CreateAsync setAccessToken(java.lang.String accessToken) {
return (CreateAsync) super.setAccessToken(accessToken);
}
@Override
public CreateAsync setAlt(java.lang.String alt) {
return (CreateAsync) super.setAlt(alt);
}
@Override
public CreateAsync setCallback(java.lang.String callback) {
return (CreateAsync) super.setCallback(callback);
}
@Override
public CreateAsync setFields(java.lang.String fields) {
return (CreateAsync) super.setFields(fields);
}
@Override
public CreateAsync setKey(java.lang.String key) {
return (CreateAsync) super.setKey(key);
}
@Override
public CreateAsync setOauthToken(java.lang.String oauthToken) {
return (CreateAsync) super.setOauthToken(oauthToken);
}
@Override
public CreateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateAsync setQuotaUser(java.lang.String quotaUser) {
return (CreateAsync) super.setQuotaUser(quotaUser);
}
@Override
public CreateAsync setUploadType(java.lang.String uploadType) {
return (CreateAsync) super.setUploadType(uploadType);
}
@Override
public CreateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public CreateAsync setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public CreateAsync setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public CreateAsync set(String parameterName, Object value) {
return (CreateAsync) super.set(parameterName, value);
}
}
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After 7
* days, the bucket will be purged and all log entries in the bucket will be permanently deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After
* 7 days, the bucket will be purged and all log entries in the bucket will be permanently
* deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListBucketsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose buckets are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource must be
specified, but supplying the character - in place of LOCATION_ID will return all buckets.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @return the request
*/
public Undelete undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) throws java.io.IOException {
Undelete result = new Undelete(name, content);
initialize(result);
return result;
}
public class Undelete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:undelete";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
* {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @since 1.13
*/
protected Undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAccessToken(java.lang.String accessToken) {
return (Undelete) super.setAccessToken(accessToken);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setOauthToken(java.lang.String oauthToken) {
return (Undelete) super.setOauthToken(oauthToken);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to undelete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Undelete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public UpdateAsync updateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
UpdateAsync result = new UpdateAsync(name, content);
initialize(result);
return result;
}
public class UpdateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:updateAsync";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED,
* then FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's
* location cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
* {@link
* UpdateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected UpdateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public UpdateAsync set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAsync) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAsync setAccessToken(java.lang.String accessToken) {
return (UpdateAsync) super.setAccessToken(accessToken);
}
@Override
public UpdateAsync setAlt(java.lang.String alt) {
return (UpdateAsync) super.setAlt(alt);
}
@Override
public UpdateAsync setCallback(java.lang.String callback) {
return (UpdateAsync) super.setCallback(callback);
}
@Override
public UpdateAsync setFields(java.lang.String fields) {
return (UpdateAsync) super.setFields(fields);
}
@Override
public UpdateAsync setKey(java.lang.String key) {
return (UpdateAsync) super.setKey(key);
}
@Override
public UpdateAsync setOauthToken(java.lang.String oauthToken) {
return (UpdateAsync) super.setOauthToken(oauthToken);
}
@Override
public UpdateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAsync setQuotaUser(java.lang.String quotaUser) {
return (UpdateAsync) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAsync setUploadType(java.lang.String uploadType) {
return (UpdateAsync) super.setUploadType(uploadType);
}
@Override
public UpdateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public UpdateAsync setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public UpdateAsync setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAsync set(String parameterName, Object value) {
return (UpdateAsync) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Links collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Links.List request = logging.links().list(parameters ...)}
*
*
* @return the resource collection
*/
public Links links() {
return new Links();
}
/**
* The "links" collection of methods.
*/
public class Links {
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The full resource name of the bucket to create a link for.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
@com.google.api.client.util.Key
private java.lang.String linkId;
/** Required. The ID to use for the link. The link_id can have up to 100 characters. A valid link_id
must only have alphanumeric characters and underscores within it.
*/
public java.lang.String getLinkId() {
return linkId;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
public Create setLinkId(java.lang.String linkId) {
this.linkId = linkId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the link to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Link.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the link:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose links are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. The maximum number of results to return from this request. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The maximum number of results to return from this request. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Views collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Views.List request = logging.views().list(parameters ...)}
*
*
* @return the resource collection
*/
public Views views() {
return new Views();
}
/**
* The "views" collection of methods.
*/
public class Views {
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket in which to create the view
`"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
@com.google.api.client.util.Key
private java.lang.String viewId;
/** Required. A client-assigned identifier such as "my-view". Identifiers are limited to 100 characters
and can include only letters, digits, underscores, hyphens, and periods.
*/
public java.lang.String getViewId() {
return viewId;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
public Create setViewId(java.lang.String viewId) {
this.viewId = viewId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to delete:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the policy:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListViewsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket whose views are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to update
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in view that need an update. A field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Operations.List request = logging.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the RecentQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.RecentQueries.List request = logging.recentQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public RecentQueries recentQueries() {
return new RecentQueries();
}
/**
* The "recentQueries" collection of methods.
*/
public class RecentQueries {
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/recentQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListRecentQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations/us-
central1Note: The location portion of the resource must be specified, but supplying the character -
in place of LOCATION_ID will return all recent queries.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SavedQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.SavedQueries.List request = logging.savedQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public SavedQueries savedQueries() {
return new SavedQueries();
}
/**
* The "savedQueries" collection of methods.
*/
public class SavedQueries {
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.SavedQuery.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the saved query:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/global"
"organizations/123456789/locations/us-central1"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
@com.google.api.client.util.Key
private java.lang.String savedQueryId;
/** Optional. The ID to use for the saved query, which will become the final component of the saved
query's resource name.If the saved_query_id is not provided, the system will generate an
alphanumeric ID.The saved_query_id is limited to 100 characters and can include only the following
characters: upper and lower-case alphanumeric characters, underscores, hyphens, periods.First
character has to be alphanumeric.
*/
public java.lang.String getSavedQueryId() {
return savedQueryId;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
public Create setSavedQueryId(java.lang.String savedQueryId) {
this.savedQueryId = savedQueryId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the saved query to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example: "projects/my-
project/locations/global/savedQueries/my-saved-query"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/locations/[^/]+$");
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSavedQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/us-
central1" Note: The locations portion of the resource must be specified. To get a list of all saved
queries, a wildcard character - can be used for LOCATION_ID, for example: "projects/my-
project/locations/-"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @return the request
*/
public Delete delete(java.lang.String logName) throws java.io.IOException {
Delete result = new Delete(logName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+logName}";
private final java.util.regex.Pattern LOG_NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/logs/[^/]+$");
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @since 1.13
*/
protected Delete(java.lang.String logName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.logName = com.google.api.client.util.Preconditions.checkNotNull(logName, "Required parameter logName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^billingAccounts/[^/]+/logs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
@com.google.api.client.util.Key
private java.lang.String logName;
/** Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
organizations/[ORGANIZATION_ID]/logs/[LOG_ID] billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-project-
id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information
about log names, see LogEntry.
*/
public java.lang.String getLogName() {
return logName;
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
public Delete setLogName(java.lang.String logName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^billingAccounts/[^/]+/logs/[^/]+$");
}
this.logName = logName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Sinks collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Sinks.List request = logging.sinks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sinks sinks() {
return new Sinks();
}
/**
* The "sinks" collection of methods.
*/
public class Sinks {
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Create setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. Determines the kind of IAM identity returned as writer_identity in the new sink. If this
value is omitted or set to false, and if the sink's parent is a project, then the value returned as
writer_identity is the same group or service account used by Cloud Logging before the addition of
writer identities to this API. The sink's destination must be in the same project as the sink
itself.If this field is set to true, or if the sink is owned by a non-project resource such as an
organization, then the value of writer_identity will be a service agent
(https://cloud.google.com/iam/docs/service-account-types#service-agents) used by the sinks with the
same parent. For more information, see writer_identity in LogSink.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
public Create setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Delete delete(java.lang.String sinkName) throws java.io.IOException {
Delete result = new Delete(sinkName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/sinks/[^/]+$");
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Delete(java.lang.String sinkName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to delete, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Delete setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Get get(java.lang.String sinkName) throws java.io.IOException {
Get result = new Get(sinkName);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/sinks/[^/]+$");
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Get(java.lang.String sinkName) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Get setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+$");
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^billingAccounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter expression to constrain the sinks returned. Today, this only supports the
following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")', 'in_scope("DEFAULT")'.Description
of scopes below. ALL: Includes all of the sinks which can be returned in any other scope. ANCESTOR:
Includes intercepting sinks owned by ancestor resources. DEFAULT: Includes sinks owned by
parent.When the empty string is provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Patch patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Patch result = new Patch(sinkName, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Patch setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Patch setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Patch setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Update update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Update result = new Update(sinkName, content);
initialize(result);
return result;
}
public class Update extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^billingAccounts/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PUT", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Update setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^billingAccounts/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Update setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Update setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Update setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Entries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Entries.List request = logging.entries().list(parameters ...)}
*
*
* @return the resource collection
*/
public Entries entries() {
return new Entries();
}
/**
* The "entries" collection of methods.
*/
public class Entries {
/**
* Copies a set of log entries from a log bucket to a Cloud Storage bucket.
*
* Create a request for the method "entries.copy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Copy#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.logging.v2.model.CopyLogEntriesRequest}
* @return the request
*/
public Copy copy(com.google.api.services.logging.v2.model.CopyLogEntriesRequest content) throws java.io.IOException {
Copy result = new Copy(content);
initialize(result);
return result;
}
public class Copy extends LoggingRequest {
private static final String REST_PATH = "v2/entries:copy";
/**
* Copies a set of log entries from a log bucket to a Cloud Storage bucket.
*
* Create a request for the method "entries.copy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Copy#execute()} method to invoke the remote operation. {@link
* Copy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.logging.v2.model.CopyLogEntriesRequest}
* @since 1.13
*/
protected Copy(com.google.api.services.logging.v2.model.CopyLogEntriesRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
}
@Override
public Copy set$Xgafv(java.lang.String $Xgafv) {
return (Copy) super.set$Xgafv($Xgafv);
}
@Override
public Copy setAccessToken(java.lang.String accessToken) {
return (Copy) super.setAccessToken(accessToken);
}
@Override
public Copy setAlt(java.lang.String alt) {
return (Copy) super.setAlt(alt);
}
@Override
public Copy setCallback(java.lang.String callback) {
return (Copy) super.setCallback(callback);
}
@Override
public Copy setFields(java.lang.String fields) {
return (Copy) super.setFields(fields);
}
@Override
public Copy setKey(java.lang.String key) {
return (Copy) super.setKey(key);
}
@Override
public Copy setOauthToken(java.lang.String oauthToken) {
return (Copy) super.setOauthToken(oauthToken);
}
@Override
public Copy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Copy) super.setPrettyPrint(prettyPrint);
}
@Override
public Copy setQuotaUser(java.lang.String quotaUser) {
return (Copy) super.setQuotaUser(quotaUser);
}
@Override
public Copy setUploadType(java.lang.String uploadType) {
return (Copy) super.setUploadType(uploadType);
}
@Override
public Copy setUploadProtocol(java.lang.String uploadProtocol) {
return (Copy) super.setUploadProtocol(uploadProtocol);
}
@Override
public Copy set(String parameterName, Object value) {
return (Copy) super.set(parameterName, value);
}
}
/**
* Lists log entries. Use this method to retrieve log entries that originated from a
* project/folder/organization/billing account. For ways to export log entries, see Exporting Logs
* (https://cloud.google.com/logging/docs/export).
*
* Create a request for the method "entries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.logging.v2.model.ListLogEntriesRequest}
* @return the request
*/
public List list(com.google.api.services.logging.v2.model.ListLogEntriesRequest content) throws java.io.IOException {
List result = new List(content);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/entries:list";
/**
* Lists log entries. Use this method to retrieve log entries that originated from a
* project/folder/organization/billing account. For ways to export log entries, see Exporting Logs
* (https://cloud.google.com/logging/docs/export).
*
* Create a request for the method "entries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.logging.v2.model.ListLogEntriesRequest}
* @since 1.13
*/
protected List(com.google.api.services.logging.v2.model.ListLogEntriesRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.ListLogEntriesResponse.class);
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Streaming read of log entries as they are received. Until the stream is terminated, it will
* continue reading logs.
*
* Create a request for the method "entries.tail".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Tail#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.logging.v2.model.TailLogEntriesRequest}
* @return the request
*/
public Tail tail(com.google.api.services.logging.v2.model.TailLogEntriesRequest content) throws java.io.IOException {
Tail result = new Tail(content);
initialize(result);
return result;
}
public class Tail extends LoggingRequest {
private static final String REST_PATH = "v2/entries:tail";
/**
* Streaming read of log entries as they are received. Until the stream is terminated, it will
* continue reading logs.
*
* Create a request for the method "entries.tail".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Tail#execute()} method to invoke the remote operation. {@link
* Tail#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.logging.v2.model.TailLogEntriesRequest}
* @since 1.13
*/
protected Tail(com.google.api.services.logging.v2.model.TailLogEntriesRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.TailLogEntriesResponse.class);
}
@Override
public Tail set$Xgafv(java.lang.String $Xgafv) {
return (Tail) super.set$Xgafv($Xgafv);
}
@Override
public Tail setAccessToken(java.lang.String accessToken) {
return (Tail) super.setAccessToken(accessToken);
}
@Override
public Tail setAlt(java.lang.String alt) {
return (Tail) super.setAlt(alt);
}
@Override
public Tail setCallback(java.lang.String callback) {
return (Tail) super.setCallback(callback);
}
@Override
public Tail setFields(java.lang.String fields) {
return (Tail) super.setFields(fields);
}
@Override
public Tail setKey(java.lang.String key) {
return (Tail) super.setKey(key);
}
@Override
public Tail setOauthToken(java.lang.String oauthToken) {
return (Tail) super.setOauthToken(oauthToken);
}
@Override
public Tail setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Tail) super.setPrettyPrint(prettyPrint);
}
@Override
public Tail setQuotaUser(java.lang.String quotaUser) {
return (Tail) super.setQuotaUser(quotaUser);
}
@Override
public Tail setUploadType(java.lang.String uploadType) {
return (Tail) super.setUploadType(uploadType);
}
@Override
public Tail setUploadProtocol(java.lang.String uploadProtocol) {
return (Tail) super.setUploadProtocol(uploadProtocol);
}
@Override
public Tail set(String parameterName, Object value) {
return (Tail) super.set(parameterName, value);
}
}
/**
* Writes log entries to Logging. This API method is the only way to send log entries to Logging.
* This method is used, directly or indirectly, by the Logging agent (fluentd) and all logging
* libraries configured to use Logging. A single request may contain log entries for a maximum of
* 1000 different resource names (projects, organizations, billing accounts or folders), where the
* resource name for a log entry is determined from its logName field.
*
* Create a request for the method "entries.write".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Write#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.logging.v2.model.WriteLogEntriesRequest}
* @return the request
*/
public Write write(com.google.api.services.logging.v2.model.WriteLogEntriesRequest content) throws java.io.IOException {
Write result = new Write(content);
initialize(result);
return result;
}
public class Write extends LoggingRequest {
private static final String REST_PATH = "v2/entries:write";
/**
* Writes log entries to Logging. This API method is the only way to send log entries to Logging.
* This method is used, directly or indirectly, by the Logging agent (fluentd) and all logging
* libraries configured to use Logging. A single request may contain log entries for a maximum of
* 1000 different resource names (projects, organizations, billing accounts or folders), where the
* resource name for a log entry is determined from its logName field.
*
* Create a request for the method "entries.write".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Write#execute()} method to invoke the remote operation. {@link
* Write#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.logging.v2.model.WriteLogEntriesRequest}
* @since 1.13
*/
protected Write(com.google.api.services.logging.v2.model.WriteLogEntriesRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.WriteLogEntriesResponse.class);
}
@Override
public Write set$Xgafv(java.lang.String $Xgafv) {
return (Write) super.set$Xgafv($Xgafv);
}
@Override
public Write setAccessToken(java.lang.String accessToken) {
return (Write) super.setAccessToken(accessToken);
}
@Override
public Write setAlt(java.lang.String alt) {
return (Write) super.setAlt(alt);
}
@Override
public Write setCallback(java.lang.String callback) {
return (Write) super.setCallback(callback);
}
@Override
public Write setFields(java.lang.String fields) {
return (Write) super.setFields(fields);
}
@Override
public Write setKey(java.lang.String key) {
return (Write) super.setKey(key);
}
@Override
public Write setOauthToken(java.lang.String oauthToken) {
return (Write) super.setOauthToken(oauthToken);
}
@Override
public Write setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Write) super.setPrettyPrint(prettyPrint);
}
@Override
public Write setQuotaUser(java.lang.String quotaUser) {
return (Write) super.setQuotaUser(quotaUser);
}
@Override
public Write setUploadType(java.lang.String uploadType) {
return (Write) super.setUploadType(uploadType);
}
@Override
public Write setUploadProtocol(java.lang.String uploadProtocol) {
return (Write) super.setUploadProtocol(uploadProtocol);
}
@Override
public Write set(String parameterName, Object value) {
return (Write) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Exclusions collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Exclusions.List request = logging.exclusions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Exclusions exclusions() {
return new Exclusions();
}
/**
* The "exclusions" collection of methods.
*/
public class Exclusions {
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-logging-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/exclusions/[^/]+$");
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions
* /my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion to delete:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions
* /my-exclusion"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/exclusions/[^/]+$");
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/exclusions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions
* /my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions
* /my-exclusion"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListExclusionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/exclusions/[^/]+$");
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions
* /my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the exclusion to update:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions
* /my-exclusion"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. A non-empty list of fields to change in the existing exclusion. New values for the fields
are taken from the corresponding fields in the LogExclusion included in this request. Fields not
mentioned in update_mask are not changed and are ignored in the request.For example, to change the
filter and description of an exclusion, specify an update_mask of "filter,description".
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Folders collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Folders.List request = logging.folders().list(parameters ...)}
*
*
* @return the resource collection
*/
public Folders folders() {
return new Folders();
}
/**
* The "folders" collection of methods.
*/
public class Folders {
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "folders.getCmekSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @return the request
*/
public GetCmekSettings getCmekSettings(java.lang.String name) throws java.io.IOException {
GetCmekSettings result = new GetCmekSettings(name);
initialize(result);
return result;
}
public class GetCmekSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/cmekSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "folders.getCmekSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
* {@link GetCmekSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @since 1.13
*/
protected GetCmekSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.CmekSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetCmekSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetCmekSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetCmekSettings setAccessToken(java.lang.String accessToken) {
return (GetCmekSettings) super.setAccessToken(accessToken);
}
@Override
public GetCmekSettings setAlt(java.lang.String alt) {
return (GetCmekSettings) super.setAlt(alt);
}
@Override
public GetCmekSettings setCallback(java.lang.String callback) {
return (GetCmekSettings) super.setCallback(callback);
}
@Override
public GetCmekSettings setFields(java.lang.String fields) {
return (GetCmekSettings) super.setFields(fields);
}
@Override
public GetCmekSettings setKey(java.lang.String key) {
return (GetCmekSettings) super.setKey(key);
}
@Override
public GetCmekSettings setOauthToken(java.lang.String oauthToken) {
return (GetCmekSettings) super.setOauthToken(oauthToken);
}
@Override
public GetCmekSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetCmekSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetCmekSettings setQuotaUser(java.lang.String quotaUser) {
return (GetCmekSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetCmekSettings setUploadType(java.lang.String uploadType) {
return (GetCmekSettings) super.setUploadType(uploadType);
}
@Override
public GetCmekSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetCmekSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
"organizations/[ORGANIZATION_ID]/cmekSettings" "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings"
"folders/[FOLDER_ID]/cmekSettings" For example:"organizations/12345/cmekSettings"Note: CMEK for the
Log Router can be configured for Google Cloud projects, folders, organizations, and billing
accounts. Once configured for an organization, it applies to all projects and folders in the Google
Cloud organization.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
public GetCmekSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetCmekSettings set(String parameterName, Object value) {
return (GetCmekSettings) super.set(parameterName, value);
}
}
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "folders.getSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @return the request
*/
public GetSettings getSettings(java.lang.String name) throws java.io.IOException {
GetSettings result = new GetSettings(name);
initialize(result);
return result;
}
public class GetSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/settings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "folders.getSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
* {@link
* GetSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @since 1.13
*/
protected GetSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Settings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetSettings setAccessToken(java.lang.String accessToken) {
return (GetSettings) super.setAccessToken(accessToken);
}
@Override
public GetSettings setAlt(java.lang.String alt) {
return (GetSettings) super.setAlt(alt);
}
@Override
public GetSettings setCallback(java.lang.String callback) {
return (GetSettings) super.setCallback(callback);
}
@Override
public GetSettings setFields(java.lang.String fields) {
return (GetSettings) super.setFields(fields);
}
@Override
public GetSettings setKey(java.lang.String key) {
return (GetSettings) super.setKey(key);
}
@Override
public GetSettings setOauthToken(java.lang.String oauthToken) {
return (GetSettings) super.setOauthToken(oauthToken);
}
@Override
public GetSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSettings setQuotaUser(java.lang.String quotaUser) {
return (GetSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetSettings setUploadType(java.lang.String uploadType) {
return (GetSettings) super.setUploadType(uploadType);
}
@Override
public GetSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
"organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
"folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can be
retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public GetSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetSettings set(String parameterName, Object value) {
return (GetSettings) super.set(parameterName, value);
}
}
/**
* Updates the settings for the given resource. This method applies to all feature configurations
* for organization and folders.UpdateSettings fails when any of the following are true: The value
* of storage_location either isn't supported by Logging or violates the location OrgPolicy. The
* default_sink_config field is set, but it has an unspecified filter write mode. The value of
* kms_key_name is invalid. The associated service account doesn't have the required
* roles/cloudkms.cryptoKeyEncrypterDecrypter role assigned for the key. Access to the key is
* disabled.See Configure default settings for organizations and folders
* (https://cloud.google.com/logging/docs/default-settings) for more information.
*
* Create a request for the method "folders.updateSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name for the settings to update. "organizations/[ORGANIZATION_ID]/settings"
* For example:"organizations/12345/settings"
* @param content the {@link com.google.api.services.logging.v2.model.Settings}
* @return the request
*/
public UpdateSettings updateSettings(java.lang.String name, com.google.api.services.logging.v2.model.Settings content) throws java.io.IOException {
UpdateSettings result = new UpdateSettings(name, content);
initialize(result);
return result;
}
public class UpdateSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/settings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Updates the settings for the given resource. This method applies to all feature configurations
* for organization and folders.UpdateSettings fails when any of the following are true: The value
* of storage_location either isn't supported by Logging or violates the location OrgPolicy. The
* default_sink_config field is set, but it has an unspecified filter write mode. The value of
* kms_key_name is invalid. The associated service account doesn't have the required
* roles/cloudkms.cryptoKeyEncrypterDecrypter role assigned for the key. Access to the key is
* disabled.See Configure default settings for organizations and folders
* (https://cloud.google.com/logging/docs/default-settings) for more information.
*
* Create a request for the method "folders.updateSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateSettings#execute()} method to invoke the remote operation.
* {@link UpdateSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource name for the settings to update. "organizations/[ORGANIZATION_ID]/settings"
* For example:"organizations/12345/settings"
* @param content the {@link com.google.api.services.logging.v2.model.Settings}
* @since 1.13
*/
protected UpdateSettings(java.lang.String name, com.google.api.services.logging.v2.model.Settings content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.Settings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public UpdateSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateSettings setAccessToken(java.lang.String accessToken) {
return (UpdateSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateSettings setAlt(java.lang.String alt) {
return (UpdateSettings) super.setAlt(alt);
}
@Override
public UpdateSettings setCallback(java.lang.String callback) {
return (UpdateSettings) super.setCallback(callback);
}
@Override
public UpdateSettings setFields(java.lang.String fields) {
return (UpdateSettings) super.setFields(fields);
}
@Override
public UpdateSettings setKey(java.lang.String key) {
return (UpdateSettings) super.setKey(key);
}
@Override
public UpdateSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateSettings setUploadType(java.lang.String uploadType) {
return (UpdateSettings) super.setUploadType(uploadType);
}
@Override
public UpdateSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name for the settings to update.
* "organizations/[ORGANIZATION_ID]/settings" For example:"organizations/12345/settings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name for the settings to update. "organizations/[ORGANIZATION_ID]/settings"
For example:"organizations/12345/settings"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name for the settings to update.
* "organizations/[ORGANIZATION_ID]/settings" For example:"organizations/12345/settings"
*/
public UpdateSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask identifying which fields from settings should be updated. A field will
* be overwritten if and only if it is in the update mask. Output only fields cannot be
* updated.See FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask identifying which fields from settings should be updated. A field will be
overwritten if and only if it is in the update mask. Output only fields cannot be updated.See
FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask identifying which fields from settings should be updated. A field will
* be overwritten if and only if it is in the update mask. Output only fields cannot be
* updated.See FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
public UpdateSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateSettings set(String parameterName, Object value) {
return (UpdateSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Exclusions collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Exclusions.List request = logging.exclusions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Exclusions exclusions() {
return new Exclusions();
}
/**
* The "exclusions" collection of methods.
*/
public class Exclusions {
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-logging-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/exclusions/[^/]+$");
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion to delete:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/exclusions/[^/]+$");
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/exclusions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListExclusionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/exclusions/[^/]+$");
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the exclusion to update:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. A non-empty list of fields to change in the existing exclusion. New values for the fields
are taken from the corresponding fields in the LogExclusion included in this request. Fields not
mentioned in update_mask are not changed and are ignored in the request.For example, to change the
filter and description of an exclusion, specify an update_mask of "filter,description".
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Locations.List request = logging.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
"displayName=tokyo", and is documented in more detail in AIP-160 (https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the next_page_token field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Buckets collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Buckets.List request = logging.buckets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Buckets buckets() {
return new Buckets();
}
/**
* The "buckets" collection of methods.
*/
public class Buckets {
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created, the
* bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created,
* the bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public Create setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has been
* created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public CreateAsync createAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
CreateAsync result = new CreateAsync(parent, content);
initialize(result);
return result;
}
public class CreateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets:createAsync";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has
* been created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
* {@link
* CreateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected CreateAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public CreateAsync set$Xgafv(java.lang.String $Xgafv) {
return (CreateAsync) super.set$Xgafv($Xgafv);
}
@Override
public CreateAsync setAccessToken(java.lang.String accessToken) {
return (CreateAsync) super.setAccessToken(accessToken);
}
@Override
public CreateAsync setAlt(java.lang.String alt) {
return (CreateAsync) super.setAlt(alt);
}
@Override
public CreateAsync setCallback(java.lang.String callback) {
return (CreateAsync) super.setCallback(callback);
}
@Override
public CreateAsync setFields(java.lang.String fields) {
return (CreateAsync) super.setFields(fields);
}
@Override
public CreateAsync setKey(java.lang.String key) {
return (CreateAsync) super.setKey(key);
}
@Override
public CreateAsync setOauthToken(java.lang.String oauthToken) {
return (CreateAsync) super.setOauthToken(oauthToken);
}
@Override
public CreateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateAsync setQuotaUser(java.lang.String quotaUser) {
return (CreateAsync) super.setQuotaUser(quotaUser);
}
@Override
public CreateAsync setUploadType(java.lang.String uploadType) {
return (CreateAsync) super.setUploadType(uploadType);
}
@Override
public CreateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public CreateAsync setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public CreateAsync setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public CreateAsync set(String parameterName, Object value) {
return (CreateAsync) super.set(parameterName, value);
}
}
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After 7
* days, the bucket will be purged and all log entries in the bucket will be permanently deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After
* 7 days, the bucket will be purged and all log entries in the bucket will be permanently
* deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListBucketsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose buckets are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource must be
specified, but supplying the character - in place of LOCATION_ID will return all buckets.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @return the request
*/
public Undelete undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) throws java.io.IOException {
Undelete result = new Undelete(name, content);
initialize(result);
return result;
}
public class Undelete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:undelete";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
* {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @since 1.13
*/
protected Undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAccessToken(java.lang.String accessToken) {
return (Undelete) super.setAccessToken(accessToken);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setOauthToken(java.lang.String oauthToken) {
return (Undelete) super.setOauthToken(oauthToken);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to undelete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Undelete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public UpdateAsync updateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
UpdateAsync result = new UpdateAsync(name, content);
initialize(result);
return result;
}
public class UpdateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:updateAsync";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED,
* then FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's
* location cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
* {@link
* UpdateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected UpdateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public UpdateAsync set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAsync) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAsync setAccessToken(java.lang.String accessToken) {
return (UpdateAsync) super.setAccessToken(accessToken);
}
@Override
public UpdateAsync setAlt(java.lang.String alt) {
return (UpdateAsync) super.setAlt(alt);
}
@Override
public UpdateAsync setCallback(java.lang.String callback) {
return (UpdateAsync) super.setCallback(callback);
}
@Override
public UpdateAsync setFields(java.lang.String fields) {
return (UpdateAsync) super.setFields(fields);
}
@Override
public UpdateAsync setKey(java.lang.String key) {
return (UpdateAsync) super.setKey(key);
}
@Override
public UpdateAsync setOauthToken(java.lang.String oauthToken) {
return (UpdateAsync) super.setOauthToken(oauthToken);
}
@Override
public UpdateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAsync setQuotaUser(java.lang.String quotaUser) {
return (UpdateAsync) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAsync setUploadType(java.lang.String uploadType) {
return (UpdateAsync) super.setUploadType(uploadType);
}
@Override
public UpdateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public UpdateAsync setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public UpdateAsync setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAsync set(String parameterName, Object value) {
return (UpdateAsync) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Links collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Links.List request = logging.links().list(parameters ...)}
*
*
* @return the resource collection
*/
public Links links() {
return new Links();
}
/**
* The "links" collection of methods.
*/
public class Links {
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The full resource name of the bucket to create a link for.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
@com.google.api.client.util.Key
private java.lang.String linkId;
/** Required. The ID to use for the link. The link_id can have up to 100 characters. A valid link_id
must only have alphanumeric characters and underscores within it.
*/
public java.lang.String getLinkId() {
return linkId;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
public Create setLinkId(java.lang.String linkId) {
this.linkId = linkId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the link to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Link.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the link:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose links are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. The maximum number of results to return from this request. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The maximum number of results to return from this request. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Views collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Views.List request = logging.views().list(parameters ...)}
*
*
* @return the resource collection
*/
public Views views() {
return new Views();
}
/**
* The "views" collection of methods.
*/
public class Views {
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket in which to create the view
`"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
@com.google.api.client.util.Key
private java.lang.String viewId;
/** Required. A client-assigned identifier such as "my-view". Identifiers are limited to 100 characters
and can include only letters, digits, underscores, hyphens, and periods.
*/
public java.lang.String getViewId() {
return viewId;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
public Create setViewId(java.lang.String viewId) {
this.viewId = viewId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to delete:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the policy:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListViewsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket whose views are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to update
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in view that need an update. A field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can return
* NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can
* return NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Operations.List request = logging.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the RecentQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.RecentQueries.List request = logging.recentQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public RecentQueries recentQueries() {
return new RecentQueries();
}
/**
* The "recentQueries" collection of methods.
*/
public class RecentQueries {
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/recentQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListRecentQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations/us-
central1Note: The location portion of the resource must be specified, but supplying the character -
in place of LOCATION_ID will return all recent queries.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SavedQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.SavedQueries.List request = logging.savedQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public SavedQueries savedQueries() {
return new SavedQueries();
}
/**
* The "savedQueries" collection of methods.
*/
public class SavedQueries {
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.SavedQuery.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the saved query:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/global"
"organizations/123456789/locations/us-central1"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
@com.google.api.client.util.Key
private java.lang.String savedQueryId;
/** Optional. The ID to use for the saved query, which will become the final component of the saved
query's resource name.If the saved_query_id is not provided, the system will generate an
alphanumeric ID.The saved_query_id is limited to 100 characters and can include only the following
characters: upper and lower-case alphanumeric characters, underscores, hyphens, periods.First
character has to be alphanumeric.
*/
public java.lang.String getSavedQueryId() {
return savedQueryId;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
public Create setSavedQueryId(java.lang.String savedQueryId) {
this.savedQueryId = savedQueryId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the saved query to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example: "projects/my-
project/locations/global/savedQueries/my-saved-query"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/locations/[^/]+$");
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSavedQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/us-
central1" Note: The locations portion of the resource must be specified. To get a list of all saved
queries, a wildcard character - can be used for LOCATION_ID, for example: "projects/my-
project/locations/-"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @return the request
*/
public Delete delete(java.lang.String logName) throws java.io.IOException {
Delete result = new Delete(logName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+logName}";
private final java.util.regex.Pattern LOG_NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/logs/[^/]+$");
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @since 1.13
*/
protected Delete(java.lang.String logName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.logName = com.google.api.client.util.Preconditions.checkNotNull(logName, "Required parameter logName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^folders/[^/]+/logs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
@com.google.api.client.util.Key
private java.lang.String logName;
/** Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
organizations/[ORGANIZATION_ID]/logs/[LOG_ID] billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-project-
id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information
about log names, see LogEntry.
*/
public java.lang.String getLogName() {
return logName;
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
public Delete setLogName(java.lang.String logName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^folders/[^/]+/logs/[^/]+$");
}
this.logName = logName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Sinks collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Sinks.List request = logging.sinks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sinks sinks() {
return new Sinks();
}
/**
* The "sinks" collection of methods.
*/
public class Sinks {
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Create setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. Determines the kind of IAM identity returned as writer_identity in the new sink. If this
value is omitted or set to false, and if the sink's parent is a project, then the value returned as
writer_identity is the same group or service account used by Cloud Logging before the addition of
writer identities to this API. The sink's destination must be in the same project as the sink
itself.If this field is set to true, or if the sink is owned by a non-project resource such as an
organization, then the value of writer_identity will be a service agent
(https://cloud.google.com/iam/docs/service-account-types#service-agents) used by the sinks with the
same parent. For more information, see writer_identity in LogSink.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
public Create setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Delete delete(java.lang.String sinkName) throws java.io.IOException {
Delete result = new Delete(sinkName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/sinks/[^/]+$");
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Delete(java.lang.String sinkName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to delete, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Delete setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Get get(java.lang.String sinkName) throws java.io.IOException {
Get result = new Get(sinkName);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/sinks/[^/]+$");
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Get(java.lang.String sinkName) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Get setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter expression to constrain the sinks returned. Today, this only supports the
following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")', 'in_scope("DEFAULT")'.Description
of scopes below. ALL: Includes all of the sinks which can be returned in any other scope. ANCESTOR:
Includes intercepting sinks owned by ancestor resources. DEFAULT: Includes sinks owned by
parent.When the empty string is provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Patch patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Patch result = new Patch(sinkName, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Patch setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Patch setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Patch setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Update update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Update result = new Update(sinkName, content);
initialize(result);
return result;
}
public class Update extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PUT", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Update setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^folders/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Update setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Update setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Update setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Locations.List request = logging.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
"displayName=tokyo", and is documented in more detail in AIP-160 (https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the next_page_token field in the response. Send that page token
* to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the next_page_token field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the next_page_token field in the response. Send that page token
* to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Buckets collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Buckets.List request = logging.buckets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Buckets buckets() {
return new Buckets();
}
/**
* The "buckets" collection of methods.
*/
public class Buckets {
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created, the
* bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created,
* the bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public Create setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has been
* created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public CreateAsync createAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
CreateAsync result = new CreateAsync(parent, content);
initialize(result);
return result;
}
public class CreateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets:createAsync";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has
* been created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
* {@link
* CreateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected CreateAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
}
@Override
public CreateAsync set$Xgafv(java.lang.String $Xgafv) {
return (CreateAsync) super.set$Xgafv($Xgafv);
}
@Override
public CreateAsync setAccessToken(java.lang.String accessToken) {
return (CreateAsync) super.setAccessToken(accessToken);
}
@Override
public CreateAsync setAlt(java.lang.String alt) {
return (CreateAsync) super.setAlt(alt);
}
@Override
public CreateAsync setCallback(java.lang.String callback) {
return (CreateAsync) super.setCallback(callback);
}
@Override
public CreateAsync setFields(java.lang.String fields) {
return (CreateAsync) super.setFields(fields);
}
@Override
public CreateAsync setKey(java.lang.String key) {
return (CreateAsync) super.setKey(key);
}
@Override
public CreateAsync setOauthToken(java.lang.String oauthToken) {
return (CreateAsync) super.setOauthToken(oauthToken);
}
@Override
public CreateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateAsync setQuotaUser(java.lang.String quotaUser) {
return (CreateAsync) super.setQuotaUser(quotaUser);
}
@Override
public CreateAsync setUploadType(java.lang.String uploadType) {
return (CreateAsync) super.setUploadType(uploadType);
}
@Override
public CreateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public CreateAsync setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public CreateAsync setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public CreateAsync set(String parameterName, Object value) {
return (CreateAsync) super.set(parameterName, value);
}
}
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After 7
* days, the bucket will be purged and all log entries in the bucket will be permanently deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After
* 7 days, the bucket will be purged and all log entries in the bucket will be permanently
* deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+$");
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListBucketsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose buckets are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource must be
specified, but supplying the character - in place of LOCATION_ID will return all buckets.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @return the request
*/
public Undelete undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) throws java.io.IOException {
Undelete result = new Undelete(name, content);
initialize(result);
return result;
}
public class Undelete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:undelete";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
* {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @since 1.13
*/
protected Undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAccessToken(java.lang.String accessToken) {
return (Undelete) super.setAccessToken(accessToken);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setOauthToken(java.lang.String oauthToken) {
return (Undelete) super.setOauthToken(oauthToken);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to undelete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Undelete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public UpdateAsync updateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
UpdateAsync result = new UpdateAsync(name, content);
initialize(result);
return result;
}
public class UpdateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:updateAsync";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED,
* then FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's
* location cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
* {@link
* UpdateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected UpdateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public UpdateAsync set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAsync) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAsync setAccessToken(java.lang.String accessToken) {
return (UpdateAsync) super.setAccessToken(accessToken);
}
@Override
public UpdateAsync setAlt(java.lang.String alt) {
return (UpdateAsync) super.setAlt(alt);
}
@Override
public UpdateAsync setCallback(java.lang.String callback) {
return (UpdateAsync) super.setCallback(callback);
}
@Override
public UpdateAsync setFields(java.lang.String fields) {
return (UpdateAsync) super.setFields(fields);
}
@Override
public UpdateAsync setKey(java.lang.String key) {
return (UpdateAsync) super.setKey(key);
}
@Override
public UpdateAsync setOauthToken(java.lang.String oauthToken) {
return (UpdateAsync) super.setOauthToken(oauthToken);
}
@Override
public UpdateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAsync setQuotaUser(java.lang.String quotaUser) {
return (UpdateAsync) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAsync setUploadType(java.lang.String uploadType) {
return (UpdateAsync) super.setUploadType(uploadType);
}
@Override
public UpdateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public UpdateAsync setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public UpdateAsync setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAsync set(String parameterName, Object value) {
return (UpdateAsync) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Links collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Links.List request = logging.links().list(parameters ...)}
*
*
* @return the resource collection
*/
public Links links() {
return new Links();
}
/**
* The "links" collection of methods.
*/
public class Links {
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The full resource name of the bucket to create a link for.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
@com.google.api.client.util.Key
private java.lang.String linkId;
/** Required. The ID to use for the link. The link_id can have up to 100 characters. A valid link_id
must only have alphanumeric characters and underscores within it.
*/
public java.lang.String getLinkId() {
return linkId;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
public Create setLinkId(java.lang.String linkId) {
this.linkId = linkId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "or
* ganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_I
* D]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/l
* inks/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the link to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "or
* ganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_I
* D]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/l
* inks/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Link.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "or
* ganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_I
* D]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/l
* inks/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the link:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "or
* ganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_I
* D]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/l
* inks/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose links are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. The maximum number of results to return from this request. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The maximum number of results to return from this request. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Views collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Views.List request = logging.views().list(parameters ...)}
*
*
* @return the resource collection
*/
public Views views() {
return new Views();
}
/**
* The "views" collection of methods.
*/
public class Views {
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket in which to create the view
`"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
*/
@com.google.api.client.util.Key
private java.lang.String viewId;
/** Required. A client-assigned identifier such as "my-view". Identifiers are limited to 100 characters
and can include only letters, digits, underscores, hyphens, and periods.
*/
public java.lang.String getViewId() {
return viewId;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
*/
public Create setViewId(java.lang.String viewId) {
this.viewId = viewId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to delete:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the policy:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListViewsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket whose views are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to update
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in view that need an update. A field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can return
* NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can
* return NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Operations.List request = logging.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^[^/]+/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @return the request
*/
public Delete delete(java.lang.String logName) throws java.io.IOException {
Delete result = new Delete(logName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+logName}";
private final java.util.regex.Pattern LOG_NAME_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+/logs/[^/]+$");
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @since 1.13
*/
protected Delete(java.lang.String logName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.logName = com.google.api.client.util.Preconditions.checkNotNull(logName, "Required parameter logName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^[^/]+/[^/]+/logs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
*/
@com.google.api.client.util.Key
private java.lang.String logName;
/** Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
organizations/[ORGANIZATION_ID]/logs/[LOG_ID] billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-project-
id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information
about log names, see LogEntry.
*/
public java.lang.String getLogName() {
return logName;
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
*/
public Delete setLogName(java.lang.String logName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^[^/]+/[^/]+/logs/[^/]+$");
}
this.logName = logName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIE
* W_ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The
* resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIE
* W_ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The
* resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the MonitoredResourceDescriptors collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.MonitoredResourceDescriptors.List request = logging.monitoredResourceDescriptors().list(parameters ...)}
*
*
* @return the resource collection
*/
public MonitoredResourceDescriptors monitoredResourceDescriptors() {
return new MonitoredResourceDescriptors();
}
/**
* The "monitoredResourceDescriptors" collection of methods.
*/
public class MonitoredResourceDescriptors {
/**
* Lists the descriptors for monitored resource types used by Logging.
*
* Create a request for the method "monitoredResourceDescriptors.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/monitoredResourceDescriptors";
/**
* Lists the descriptors for monitored resource types used by Logging.
*
* Create a request for the method "monitoredResourceDescriptors.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListMonitoredResourceDescriptorsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Organizations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Organizations.List request = logging.organizations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Organizations organizations() {
return new Organizations();
}
/**
* The "organizations" collection of methods.
*/
public class Organizations {
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "organizations.getCmekSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @return the request
*/
public GetCmekSettings getCmekSettings(java.lang.String name) throws java.io.IOException {
GetCmekSettings result = new GetCmekSettings(name);
initialize(result);
return result;
}
public class GetCmekSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/cmekSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "organizations.getCmekSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
* {@link GetCmekSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @since 1.13
*/
protected GetCmekSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.CmekSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetCmekSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetCmekSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetCmekSettings setAccessToken(java.lang.String accessToken) {
return (GetCmekSettings) super.setAccessToken(accessToken);
}
@Override
public GetCmekSettings setAlt(java.lang.String alt) {
return (GetCmekSettings) super.setAlt(alt);
}
@Override
public GetCmekSettings setCallback(java.lang.String callback) {
return (GetCmekSettings) super.setCallback(callback);
}
@Override
public GetCmekSettings setFields(java.lang.String fields) {
return (GetCmekSettings) super.setFields(fields);
}
@Override
public GetCmekSettings setKey(java.lang.String key) {
return (GetCmekSettings) super.setKey(key);
}
@Override
public GetCmekSettings setOauthToken(java.lang.String oauthToken) {
return (GetCmekSettings) super.setOauthToken(oauthToken);
}
@Override
public GetCmekSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetCmekSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetCmekSettings setQuotaUser(java.lang.String quotaUser) {
return (GetCmekSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetCmekSettings setUploadType(java.lang.String uploadType) {
return (GetCmekSettings) super.setUploadType(uploadType);
}
@Override
public GetCmekSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetCmekSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
"organizations/[ORGANIZATION_ID]/cmekSettings" "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings"
"folders/[FOLDER_ID]/cmekSettings" For example:"organizations/12345/cmekSettings"Note: CMEK for the
Log Router can be configured for Google Cloud projects, folders, organizations, and billing
accounts. Once configured for an organization, it applies to all projects and folders in the Google
Cloud organization.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
public GetCmekSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetCmekSettings set(String parameterName, Object value) {
return (GetCmekSettings) super.set(parameterName, value);
}
}
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "organizations.getSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @return the request
*/
public GetSettings getSettings(java.lang.String name) throws java.io.IOException {
GetSettings result = new GetSettings(name);
initialize(result);
return result;
}
public class GetSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/settings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "organizations.getSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
* {@link
* GetSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @since 1.13
*/
protected GetSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Settings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetSettings setAccessToken(java.lang.String accessToken) {
return (GetSettings) super.setAccessToken(accessToken);
}
@Override
public GetSettings setAlt(java.lang.String alt) {
return (GetSettings) super.setAlt(alt);
}
@Override
public GetSettings setCallback(java.lang.String callback) {
return (GetSettings) super.setCallback(callback);
}
@Override
public GetSettings setFields(java.lang.String fields) {
return (GetSettings) super.setFields(fields);
}
@Override
public GetSettings setKey(java.lang.String key) {
return (GetSettings) super.setKey(key);
}
@Override
public GetSettings setOauthToken(java.lang.String oauthToken) {
return (GetSettings) super.setOauthToken(oauthToken);
}
@Override
public GetSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSettings setQuotaUser(java.lang.String quotaUser) {
return (GetSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetSettings setUploadType(java.lang.String uploadType) {
return (GetSettings) super.setUploadType(uploadType);
}
@Override
public GetSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
"organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
"folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can be
retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public GetSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetSettings set(String parameterName, Object value) {
return (GetSettings) super.set(parameterName, value);
}
}
/**
* Updates the Log Router CMEK settings for the given resource.Note: CMEK for the Log Router can
* currently only be configured for Google Cloud organizations. Once configured, it applies to all
* projects and folders in the Google Cloud organization.UpdateCmekSettings fails when any of the
* following are true: The value of kms_key_name is invalid. The associated service account doesn't
* have the required roles/cloudkms.cryptoKeyEncrypterDecrypter role assigned for the key. Access to
* the key is disabled.See Enabling CMEK for Log Router
* (https://cloud.google.com/logging/docs/routing/managed-encryption) for more information.
*
* Create a request for the method "organizations.updateCmekSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateCmekSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name for the CMEK settings to update. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can currently only
* be configured for Google Cloud organizations. Once configured, it applies to all projects
* and folders in the Google Cloud organization.
* @param content the {@link com.google.api.services.logging.v2.model.CmekSettings}
* @return the request
*/
public UpdateCmekSettings updateCmekSettings(java.lang.String name, com.google.api.services.logging.v2.model.CmekSettings content) throws java.io.IOException {
UpdateCmekSettings result = new UpdateCmekSettings(name, content);
initialize(result);
return result;
}
public class UpdateCmekSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/cmekSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Updates the Log Router CMEK settings for the given resource.Note: CMEK for the Log Router can
* currently only be configured for Google Cloud organizations. Once configured, it applies to all
* projects and folders in the Google Cloud organization.UpdateCmekSettings fails when any of the
* following are true: The value of kms_key_name is invalid. The associated service account
* doesn't have the required roles/cloudkms.cryptoKeyEncrypterDecrypter role assigned for the key.
* Access to the key is disabled.See Enabling CMEK for Log Router
* (https://cloud.google.com/logging/docs/routing/managed-encryption) for more information.
*
* Create a request for the method "organizations.updateCmekSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateCmekSettings#execute()} method to invoke the remote
* operation. {@link UpdateCmekSettings#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The resource name for the CMEK settings to update. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can currently only
* be configured for Google Cloud organizations. Once configured, it applies to all projects
* and folders in the Google Cloud organization.
* @param content the {@link com.google.api.services.logging.v2.model.CmekSettings}
* @since 1.13
*/
protected UpdateCmekSettings(java.lang.String name, com.google.api.services.logging.v2.model.CmekSettings content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.CmekSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public UpdateCmekSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateCmekSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateCmekSettings setAccessToken(java.lang.String accessToken) {
return (UpdateCmekSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateCmekSettings setAlt(java.lang.String alt) {
return (UpdateCmekSettings) super.setAlt(alt);
}
@Override
public UpdateCmekSettings setCallback(java.lang.String callback) {
return (UpdateCmekSettings) super.setCallback(callback);
}
@Override
public UpdateCmekSettings setFields(java.lang.String fields) {
return (UpdateCmekSettings) super.setFields(fields);
}
@Override
public UpdateCmekSettings setKey(java.lang.String key) {
return (UpdateCmekSettings) super.setKey(key);
}
@Override
public UpdateCmekSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateCmekSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateCmekSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateCmekSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateCmekSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateCmekSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateCmekSettings setUploadType(java.lang.String uploadType) {
return (UpdateCmekSettings) super.setUploadType(uploadType);
}
@Override
public UpdateCmekSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateCmekSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name for the CMEK settings to update.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can currently only
* be configured for Google Cloud organizations. Once configured, it applies to all projects
* and folders in the Google Cloud organization.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name for the CMEK settings to update. "projects/[PROJECT_ID]/cmekSettings"
"organizations/[ORGANIZATION_ID]/cmekSettings" "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings"
"folders/[FOLDER_ID]/cmekSettings" For example:"organizations/12345/cmekSettings"Note: CMEK for the
Log Router can currently only be configured for Google Cloud organizations. Once configured, it
applies to all projects and folders in the Google Cloud organization.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name for the CMEK settings to update.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can currently only
* be configured for Google Cloud organizations. Once configured, it applies to all projects
* and folders in the Google Cloud organization.
*/
public UpdateCmekSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask identifying which fields from cmek_settings should be updated. A field
* will be overwritten if and only if it is in the update mask. Output only fields cannot be
* updated.See FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask identifying which fields from cmek_settings should be updated. A field will be
overwritten if and only if it is in the update mask. Output only fields cannot be updated.See
FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask identifying which fields from cmek_settings should be updated. A field
* will be overwritten if and only if it is in the update mask. Output only fields cannot be
* updated.See FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
public UpdateCmekSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateCmekSettings set(String parameterName, Object value) {
return (UpdateCmekSettings) super.set(parameterName, value);
}
}
/**
* Updates the settings for the given resource. This method applies to all feature configurations
* for organization and folders.UpdateSettings fails when any of the following are true: The value
* of storage_location either isn't supported by Logging or violates the location OrgPolicy. The
* default_sink_config field is set, but it has an unspecified filter write mode. The value of
* kms_key_name is invalid. The associated service account doesn't have the required
* roles/cloudkms.cryptoKeyEncrypterDecrypter role assigned for the key. Access to the key is
* disabled.See Configure default settings for organizations and folders
* (https://cloud.google.com/logging/docs/default-settings) for more information.
*
* Create a request for the method "organizations.updateSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name for the settings to update. "organizations/[ORGANIZATION_ID]/settings"
* For example:"organizations/12345/settings"
* @param content the {@link com.google.api.services.logging.v2.model.Settings}
* @return the request
*/
public UpdateSettings updateSettings(java.lang.String name, com.google.api.services.logging.v2.model.Settings content) throws java.io.IOException {
UpdateSettings result = new UpdateSettings(name, content);
initialize(result);
return result;
}
public class UpdateSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/settings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Updates the settings for the given resource. This method applies to all feature configurations
* for organization and folders.UpdateSettings fails when any of the following are true: The value
* of storage_location either isn't supported by Logging or violates the location OrgPolicy. The
* default_sink_config field is set, but it has an unspecified filter write mode. The value of
* kms_key_name is invalid. The associated service account doesn't have the required
* roles/cloudkms.cryptoKeyEncrypterDecrypter role assigned for the key. Access to the key is
* disabled.See Configure default settings for organizations and folders
* (https://cloud.google.com/logging/docs/default-settings) for more information.
*
* Create a request for the method "organizations.updateSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateSettings#execute()} method to invoke the remote operation.
* {@link UpdateSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource name for the settings to update. "organizations/[ORGANIZATION_ID]/settings"
* For example:"organizations/12345/settings"
* @param content the {@link com.google.api.services.logging.v2.model.Settings}
* @since 1.13
*/
protected UpdateSettings(java.lang.String name, com.google.api.services.logging.v2.model.Settings content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.Settings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public UpdateSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateSettings setAccessToken(java.lang.String accessToken) {
return (UpdateSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateSettings setAlt(java.lang.String alt) {
return (UpdateSettings) super.setAlt(alt);
}
@Override
public UpdateSettings setCallback(java.lang.String callback) {
return (UpdateSettings) super.setCallback(callback);
}
@Override
public UpdateSettings setFields(java.lang.String fields) {
return (UpdateSettings) super.setFields(fields);
}
@Override
public UpdateSettings setKey(java.lang.String key) {
return (UpdateSettings) super.setKey(key);
}
@Override
public UpdateSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateSettings setUploadType(java.lang.String uploadType) {
return (UpdateSettings) super.setUploadType(uploadType);
}
@Override
public UpdateSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name for the settings to update.
* "organizations/[ORGANIZATION_ID]/settings" For example:"organizations/12345/settings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name for the settings to update. "organizations/[ORGANIZATION_ID]/settings"
For example:"organizations/12345/settings"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name for the settings to update.
* "organizations/[ORGANIZATION_ID]/settings" For example:"organizations/12345/settings"
*/
public UpdateSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask identifying which fields from settings should be updated. A field will
* be overwritten if and only if it is in the update mask. Output only fields cannot be
* updated.See FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask identifying which fields from settings should be updated. A field will be
overwritten if and only if it is in the update mask. Output only fields cannot be updated.See
FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask identifying which fields from settings should be updated. A field will
* be overwritten if and only if it is in the update mask. Output only fields cannot be
* updated.See FieldMask for more information.For example: "updateMask=kmsKeyName"
*/
public UpdateSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateSettings set(String parameterName, Object value) {
return (UpdateSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Exclusions collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Exclusions.List request = logging.exclusions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Exclusions exclusions() {
return new Exclusions();
}
/**
* The "exclusions" collection of methods.
*/
public class Exclusions {
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-logging-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/exclusions/[^/]+$");
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion to delete:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/exclusions/[^/]+$");
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/exclusions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListExclusionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/exclusions/[^/]+$");
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the exclusion to update:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. A non-empty list of fields to change in the existing exclusion. New values for the fields
are taken from the corresponding fields in the LogExclusion included in this request. Fields not
mentioned in update_mask are not changed and are ignored in the request.For example, to change the
filter and description of an exclusion, specify an update_mask of "filter,description".
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Locations.List request = logging.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
"displayName=tokyo", and is documented in more detail in AIP-160 (https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the next_page_token field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Buckets collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Buckets.List request = logging.buckets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Buckets buckets() {
return new Buckets();
}
/**
* The "buckets" collection of methods.
*/
public class Buckets {
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created, the
* bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created,
* the bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public Create setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has been
* created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public CreateAsync createAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
CreateAsync result = new CreateAsync(parent, content);
initialize(result);
return result;
}
public class CreateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets:createAsync";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has
* been created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
* {@link
* CreateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected CreateAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public CreateAsync set$Xgafv(java.lang.String $Xgafv) {
return (CreateAsync) super.set$Xgafv($Xgafv);
}
@Override
public CreateAsync setAccessToken(java.lang.String accessToken) {
return (CreateAsync) super.setAccessToken(accessToken);
}
@Override
public CreateAsync setAlt(java.lang.String alt) {
return (CreateAsync) super.setAlt(alt);
}
@Override
public CreateAsync setCallback(java.lang.String callback) {
return (CreateAsync) super.setCallback(callback);
}
@Override
public CreateAsync setFields(java.lang.String fields) {
return (CreateAsync) super.setFields(fields);
}
@Override
public CreateAsync setKey(java.lang.String key) {
return (CreateAsync) super.setKey(key);
}
@Override
public CreateAsync setOauthToken(java.lang.String oauthToken) {
return (CreateAsync) super.setOauthToken(oauthToken);
}
@Override
public CreateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateAsync setQuotaUser(java.lang.String quotaUser) {
return (CreateAsync) super.setQuotaUser(quotaUser);
}
@Override
public CreateAsync setUploadType(java.lang.String uploadType) {
return (CreateAsync) super.setUploadType(uploadType);
}
@Override
public CreateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public CreateAsync setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public CreateAsync setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public CreateAsync set(String parameterName, Object value) {
return (CreateAsync) super.set(parameterName, value);
}
}
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After 7
* days, the bucket will be purged and all log entries in the bucket will be permanently deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After
* 7 days, the bucket will be purged and all log entries in the bucket will be permanently
* deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListBucketsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose buckets are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource must be
specified, but supplying the character - in place of LOCATION_ID will return all buckets.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @return the request
*/
public Undelete undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) throws java.io.IOException {
Undelete result = new Undelete(name, content);
initialize(result);
return result;
}
public class Undelete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:undelete";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
* {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @since 1.13
*/
protected Undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAccessToken(java.lang.String accessToken) {
return (Undelete) super.setAccessToken(accessToken);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setOauthToken(java.lang.String oauthToken) {
return (Undelete) super.setOauthToken(oauthToken);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to undelete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Undelete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public UpdateAsync updateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
UpdateAsync result = new UpdateAsync(name, content);
initialize(result);
return result;
}
public class UpdateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:updateAsync";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED,
* then FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's
* location cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
* {@link
* UpdateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected UpdateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public UpdateAsync set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAsync) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAsync setAccessToken(java.lang.String accessToken) {
return (UpdateAsync) super.setAccessToken(accessToken);
}
@Override
public UpdateAsync setAlt(java.lang.String alt) {
return (UpdateAsync) super.setAlt(alt);
}
@Override
public UpdateAsync setCallback(java.lang.String callback) {
return (UpdateAsync) super.setCallback(callback);
}
@Override
public UpdateAsync setFields(java.lang.String fields) {
return (UpdateAsync) super.setFields(fields);
}
@Override
public UpdateAsync setKey(java.lang.String key) {
return (UpdateAsync) super.setKey(key);
}
@Override
public UpdateAsync setOauthToken(java.lang.String oauthToken) {
return (UpdateAsync) super.setOauthToken(oauthToken);
}
@Override
public UpdateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAsync setQuotaUser(java.lang.String quotaUser) {
return (UpdateAsync) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAsync setUploadType(java.lang.String uploadType) {
return (UpdateAsync) super.setUploadType(uploadType);
}
@Override
public UpdateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public UpdateAsync setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public UpdateAsync setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAsync set(String parameterName, Object value) {
return (UpdateAsync) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Links collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Links.List request = logging.links().list(parameters ...)}
*
*
* @return the resource collection
*/
public Links links() {
return new Links();
}
/**
* The "links" collection of methods.
*/
public class Links {
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The full resource name of the bucket to create a link for.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
@com.google.api.client.util.Key
private java.lang.String linkId;
/** Required. The ID to use for the link. The link_id can have up to 100 characters. A valid link_id
must only have alphanumeric characters and underscores within it.
*/
public java.lang.String getLinkId() {
return linkId;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
public Create setLinkId(java.lang.String linkId) {
this.linkId = linkId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the link to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Link.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the link:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose links are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. The maximum number of results to return from this request. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The maximum number of results to return from this request. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Views collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Views.List request = logging.views().list(parameters ...)}
*
*
* @return the resource collection
*/
public Views views() {
return new Views();
}
/**
* The "views" collection of methods.
*/
public class Views {
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket in which to create the view
`"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
@com.google.api.client.util.Key
private java.lang.String viewId;
/** Required. A client-assigned identifier such as "my-view". Identifiers are limited to 100 characters
and can include only letters, digits, underscores, hyphens, and periods.
*/
public java.lang.String getViewId() {
return viewId;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
public Create setViewId(java.lang.String viewId) {
this.viewId = viewId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to delete:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the policy:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListViewsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket whose views are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to update
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in view that need an update. A field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can return
* NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can
* return NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Operations.List request = logging.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the RecentQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.RecentQueries.List request = logging.recentQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public RecentQueries recentQueries() {
return new RecentQueries();
}
/**
* The "recentQueries" collection of methods.
*/
public class RecentQueries {
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/recentQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListRecentQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations/us-
central1Note: The location portion of the resource must be specified, but supplying the character -
in place of LOCATION_ID will return all recent queries.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SavedQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.SavedQueries.List request = logging.savedQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public SavedQueries savedQueries() {
return new SavedQueries();
}
/**
* The "savedQueries" collection of methods.
*/
public class SavedQueries {
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.SavedQuery.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the saved query:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/global"
"organizations/123456789/locations/us-central1"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
@com.google.api.client.util.Key
private java.lang.String savedQueryId;
/** Optional. The ID to use for the saved query, which will become the final component of the saved
query's resource name.If the saved_query_id is not provided, the system will generate an
alphanumeric ID.The saved_query_id is limited to 100 characters and can include only the following
characters: upper and lower-case alphanumeric characters, underscores, hyphens, periods.First
character has to be alphanumeric.
*/
public java.lang.String getSavedQueryId() {
return savedQueryId;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
public Create setSavedQueryId(java.lang.String savedQueryId) {
this.savedQueryId = savedQueryId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the saved query to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example: "projects/my-
project/locations/global/savedQueries/my-saved-query"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSavedQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/us-
central1" Note: The locations portion of the resource must be specified. To get a list of all saved
queries, a wildcard character - can be used for LOCATION_ID, for example: "projects/my-
project/locations/-"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @return the request
*/
public Delete delete(java.lang.String logName) throws java.io.IOException {
Delete result = new Delete(logName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+logName}";
private final java.util.regex.Pattern LOG_NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/logs/[^/]+$");
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @since 1.13
*/
protected Delete(java.lang.String logName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.logName = com.google.api.client.util.Preconditions.checkNotNull(logName, "Required parameter logName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^organizations/[^/]+/logs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
@com.google.api.client.util.Key
private java.lang.String logName;
/** Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
organizations/[ORGANIZATION_ID]/logs/[LOG_ID] billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-project-
id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information
about log names, see LogEntry.
*/
public java.lang.String getLogName() {
return logName;
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
public Delete setLogName(java.lang.String logName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^organizations/[^/]+/logs/[^/]+$");
}
this.logName = logName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Sinks collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Sinks.List request = logging.sinks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sinks sinks() {
return new Sinks();
}
/**
* The "sinks" collection of methods.
*/
public class Sinks {
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Create setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. Determines the kind of IAM identity returned as writer_identity in the new sink. If this
value is omitted or set to false, and if the sink's parent is a project, then the value returned as
writer_identity is the same group or service account used by Cloud Logging before the addition of
writer identities to this API. The sink's destination must be in the same project as the sink
itself.If this field is set to true, or if the sink is owned by a non-project resource such as an
organization, then the value of writer_identity will be a service agent
(https://cloud.google.com/iam/docs/service-account-types#service-agents) used by the sinks with the
same parent. For more information, see writer_identity in LogSink.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
public Create setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Delete delete(java.lang.String sinkName) throws java.io.IOException {
Delete result = new Delete(sinkName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/sinks/[^/]+$");
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Delete(java.lang.String sinkName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to delete, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Delete setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Get get(java.lang.String sinkName) throws java.io.IOException {
Get result = new Get(sinkName);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/sinks/[^/]+$");
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Get(java.lang.String sinkName) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Get setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter expression to constrain the sinks returned. Today, this only supports the
following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")', 'in_scope("DEFAULT")'.Description
of scopes below. ALL: Includes all of the sinks which can be returned in any other scope. ANCESTOR:
Includes intercepting sinks owned by ancestor resources. DEFAULT: Includes sinks owned by
parent.When the empty string is provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Patch patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Patch result = new Patch(sinkName, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Patch setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Patch setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Patch setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Update update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Update result = new Update(sinkName, content);
initialize(result);
return result;
}
public class Update extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PUT", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Update setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^organizations/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Update setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Update setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Update setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Projects.List request = logging.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "projects.getCmekSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @return the request
*/
public GetCmekSettings getCmekSettings(java.lang.String name) throws java.io.IOException {
GetCmekSettings result = new GetCmekSettings(name);
initialize(result);
return result;
}
public class GetCmekSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/cmekSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Gets the Logging CMEK settings for the given resource.Note: CMEK for the Log Router can be
* configured for Google Cloud projects, folders, organizations, and billing accounts. Once
* configured for an organization, it applies to all projects and folders in the Google Cloud
* organization.See Enabling CMEK for Log Router (https://cloud.google.com/logging/docs/routing
* /managed-encryption) for more information.
*
* Create a request for the method "projects.getCmekSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetCmekSettings#execute()} method to invoke the remote operation.
* {@link GetCmekSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
* "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
* @since 1.13
*/
protected GetCmekSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.CmekSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetCmekSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetCmekSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetCmekSettings setAccessToken(java.lang.String accessToken) {
return (GetCmekSettings) super.setAccessToken(accessToken);
}
@Override
public GetCmekSettings setAlt(java.lang.String alt) {
return (GetCmekSettings) super.setAlt(alt);
}
@Override
public GetCmekSettings setCallback(java.lang.String callback) {
return (GetCmekSettings) super.setCallback(callback);
}
@Override
public GetCmekSettings setFields(java.lang.String fields) {
return (GetCmekSettings) super.setFields(fields);
}
@Override
public GetCmekSettings setKey(java.lang.String key) {
return (GetCmekSettings) super.setKey(key);
}
@Override
public GetCmekSettings setOauthToken(java.lang.String oauthToken) {
return (GetCmekSettings) super.setOauthToken(oauthToken);
}
@Override
public GetCmekSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetCmekSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetCmekSettings setQuotaUser(java.lang.String quotaUser) {
return (GetCmekSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetCmekSettings setUploadType(java.lang.String uploadType) {
return (GetCmekSettings) super.setUploadType(uploadType);
}
@Override
public GetCmekSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetCmekSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve CMEK settings. "projects/[PROJECT_ID]/cmekSettings"
"organizations/[ORGANIZATION_ID]/cmekSettings" "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings"
"folders/[FOLDER_ID]/cmekSettings" For example:"organizations/12345/cmekSettings"Note: CMEK for the
Log Router can be configured for Google Cloud projects, folders, organizations, and billing
accounts. Once configured for an organization, it applies to all projects and folders in the Google
Cloud organization.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve CMEK settings.
* "projects/[PROJECT_ID]/cmekSettings" "organizations/[ORGANIZATION_ID]/cmekSettings"
* "billingAccounts/[BILLING_ACCOUNT_ID]/cmekSettings" "folders/[FOLDER_ID]/cmekSettings" For
* example:"organizations/12345/cmekSettings"Note: CMEK for the Log Router can be configured
* for Google Cloud projects, folders, organizations, and billing accounts. Once configured
* for an organization, it applies to all projects and folders in the Google Cloud
* organization.
*/
public GetCmekSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetCmekSettings set(String parameterName, Object value) {
return (GetCmekSettings) super.set(parameterName, value);
}
}
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "projects.getSettings".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @return the request
*/
public GetSettings getSettings(java.lang.String name) throws java.io.IOException {
GetSettings result = new GetSettings(name);
initialize(result);
return result;
}
public class GetSettings extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/settings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Gets the settings for the given resource.Note: Settings can be retrieved for Google Cloud
* projects, folders, organizations, and billing accounts.See View default resource settings for
* Logging (https://cloud.google.com/logging/docs/default-settings#view-org-settings) for more
* information.
*
* Create a request for the method "projects.getSettings".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
* {@link
* GetSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings
* can be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
* @since 1.13
*/
protected GetSettings(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Settings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetSettings setAccessToken(java.lang.String accessToken) {
return (GetSettings) super.setAccessToken(accessToken);
}
@Override
public GetSettings setAlt(java.lang.String alt) {
return (GetSettings) super.setAlt(alt);
}
@Override
public GetSettings setCallback(java.lang.String callback) {
return (GetSettings) super.setCallback(callback);
}
@Override
public GetSettings setFields(java.lang.String fields) {
return (GetSettings) super.setFields(fields);
}
@Override
public GetSettings setKey(java.lang.String key) {
return (GetSettings) super.setKey(key);
}
@Override
public GetSettings setOauthToken(java.lang.String oauthToken) {
return (GetSettings) super.setOauthToken(oauthToken);
}
@Override
public GetSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSettings setQuotaUser(java.lang.String quotaUser) {
return (GetSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetSettings setUploadType(java.lang.String uploadType) {
return (GetSettings) super.setUploadType(uploadType);
}
@Override
public GetSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
"organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
"folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can be
retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource for which to retrieve settings. "projects/[PROJECT_ID]/settings"
* "organizations/[ORGANIZATION_ID]/settings" "billingAccounts/[BILLING_ACCOUNT_ID]/settings"
* "folders/[FOLDER_ID]/settings" For example:"organizations/12345/settings"Note: Settings can
* be retrieved for Google Cloud projects, folders, organizations, and billing accounts.
*/
public GetSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetSettings set(String parameterName, Object value) {
return (GetSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Exclusions collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Exclusions.List request = logging.exclusions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Exclusions exclusions() {
return new Exclusions();
}
/**
* The "exclusions" collection of methods.
*/
public class Exclusions {
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Creates a new exclusion in the _Default sink in a specified parent resource. Only log entries
* belonging to that resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Create a request for the method "exclusions.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-logging-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the exclusion: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-logging-project"
* "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/exclusions/[^/]+$");
/**
* Deletes an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion to delete:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion to delete:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/exclusions/[^/]+$");
/**
* Gets the description of an exclusion in the _Default sink.
*
* Create a request for the method "exclusions.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/exclusions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of an existing exclusion:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of an existing exclusion:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/exclusions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists all the exclusions on the _Default sink in a parent resource.
*
* Create a request for the method "exclusions.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListExclusionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose exclusions are to be listed. "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/exclusions/[^/]+$");
/**
* Changes one or more properties of an existing exclusion in the _Default sink.
*
* Create a request for the method "exclusions.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
* @param content the {@link com.google.api.services.logging.v2.model.LogExclusion}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogExclusion content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogExclusion.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/exclusions/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the exclusion to update:
"projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
"organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
"folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-project/exclusions/my-
exclusion"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the exclusion to update:
* "projects/[PROJECT_ID]/exclusions/[EXCLUSION_ID]"
* "organizations/[ORGANIZATION_ID]/exclusions/[EXCLUSION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/exclusions/[EXCLUSION_ID]"
* "folders/[FOLDER_ID]/exclusions/[EXCLUSION_ID]" For example:"projects/my-
* project/exclusions/my-exclusion"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/exclusions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. A non-empty list of fields to change in the existing exclusion. New values for the fields
are taken from the corresponding fields in the LogExclusion included in this request. Fields not
mentioned in update_mask are not changed and are ignored in the request.For example, to change the
filter and description of an exclusion, specify an update_mask of "filter,description".
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. A non-empty list of fields to change in the existing exclusion. New values for
* the fields are taken from the corresponding fields in the LogExclusion included in this
* request. Fields not mentioned in update_mask are not changed and are ignored in the
* request.For example, to change the filter and description of an exclusion, specify an
* update_mask of "filter,description".
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Locations.List request = logging.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
"displayName=tokyo", and is documented in more detail in AIP-160 (https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like "displayName=tokyo", and is documented in more detail in AIP-160
* (https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the next_page_token field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the next_page_token field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Buckets collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Buckets.List request = logging.buckets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Buckets buckets() {
return new Buckets();
}
/**
* The "buckets" collection of methods.
*/
public class Buckets {
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created, the
* bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket that can be used to store log entries. After a bucket has been created,
* the bucket's location cannot be changed.
*
* Create a request for the method "buckets.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public Create setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has been
* created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public CreateAsync createAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
CreateAsync result = new CreateAsync(parent, content);
initialize(result);
return result;
}
public class CreateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets:createAsync";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a log bucket asynchronously that can be used to store log entries.After a bucket has
* been created, the bucket's location cannot be changed.
*
* Create a request for the method "buckets.createAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link CreateAsync#execute()} method to invoke the remote operation.
* {@link
* CreateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected CreateAsync(java.lang.String parent, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public CreateAsync set$Xgafv(java.lang.String $Xgafv) {
return (CreateAsync) super.set$Xgafv($Xgafv);
}
@Override
public CreateAsync setAccessToken(java.lang.String accessToken) {
return (CreateAsync) super.setAccessToken(accessToken);
}
@Override
public CreateAsync setAlt(java.lang.String alt) {
return (CreateAsync) super.setAlt(alt);
}
@Override
public CreateAsync setCallback(java.lang.String callback) {
return (CreateAsync) super.setCallback(callback);
}
@Override
public CreateAsync setFields(java.lang.String fields) {
return (CreateAsync) super.setFields(fields);
}
@Override
public CreateAsync setKey(java.lang.String key) {
return (CreateAsync) super.setKey(key);
}
@Override
public CreateAsync setOauthToken(java.lang.String oauthToken) {
return (CreateAsync) super.setOauthToken(oauthToken);
}
@Override
public CreateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateAsync setQuotaUser(java.lang.String quotaUser) {
return (CreateAsync) super.setQuotaUser(quotaUser);
}
@Override
public CreateAsync setUploadType(java.lang.String uploadType) {
return (CreateAsync) super.setUploadType(uploadType);
}
@Override
public CreateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the log bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-project/locations/global"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the log bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]" For example:"projects/my-
* project/locations/global"
*/
public CreateAsync setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
@com.google.api.client.util.Key
private java.lang.String bucketId;
/** Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to 100
characters and can include only letters, digits, underscores, hyphens, and periods. Bucket
identifiers must start with an alphanumeric character.
*/
public java.lang.String getBucketId() {
return bucketId;
}
/**
* Required. A client-assigned identifier such as "my-bucket". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and periods.
* Bucket identifiers must start with an alphanumeric character.
*/
public CreateAsync setBucketId(java.lang.String bucketId) {
this.bucketId = bucketId;
return this;
}
@Override
public CreateAsync set(String parameterName, Object value) {
return (CreateAsync) super.set(parameterName, value);
}
}
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After 7
* days, the bucket will be purged and all log entries in the bucket will be permanently deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Deletes a log bucket.Changes the bucket's lifecycle_state to the DELETE_REQUESTED state. After
* 7 days, the bucket will be purged and all log entries in the bucket will be permanently
* deleted.
*
* Create a request for the method "buckets.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Gets a log bucket.
*
* Create a request for the method "buckets.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the bucket:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the bucket:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/buckets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists log buckets.
*
* Create a request for the method "buckets.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource
* must be specified, but supplying the character - in place of LOCATION_ID will return all
* buckets.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListBucketsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose buckets are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the resource must be
specified, but supplying the character - in place of LOCATION_ID will return all buckets.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose buckets are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" Note: The locations portion of the
* resource must be specified, but supplying the character - in place of LOCATION_ID will
* return all buckets.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogBucket.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @return the request
*/
public Undelete undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) throws java.io.IOException {
Undelete result = new Undelete(name, content);
initialize(result);
return result;
}
public class Undelete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:undelete";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Undeletes a log bucket. A bucket that has been deleted can be undeleted within the grace period
* of 7 days.
*
* Create a request for the method "buckets.undelete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
* {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.UndeleteBucketRequest}
* @since 1.13
*/
protected Undelete(java.lang.String name, com.google.api.services.logging.v2.model.UndeleteBucketRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAccessToken(java.lang.String accessToken) {
return (Undelete) super.setAccessToken(accessToken);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setOauthToken(java.lang.String oauthToken) {
return (Undelete) super.setOauthToken(oauthToken);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to undelete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to undelete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public Undelete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED, then
* FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's location
* cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @return the request
*/
public UpdateAsync updateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) throws java.io.IOException {
UpdateAsync result = new UpdateAsync(name, content);
initialize(result);
return result;
}
public class UpdateAsync extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:updateAsync";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Updates a log bucket asynchronously.If the bucket has a lifecycle_state of DELETE_REQUESTED,
* then FAILED_PRECONDITION will be returned.After a bucket has been created, the bucket's
* location cannot be changed.
*
* Create a request for the method "buckets.updateAsync".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link UpdateAsync#execute()} method to invoke the remote operation.
* {@link
* UpdateAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogBucket}
* @since 1.13
*/
protected UpdateAsync(java.lang.String name, com.google.api.services.logging.v2.model.LogBucket content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public UpdateAsync set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAsync) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAsync setAccessToken(java.lang.String accessToken) {
return (UpdateAsync) super.setAccessToken(accessToken);
}
@Override
public UpdateAsync setAlt(java.lang.String alt) {
return (UpdateAsync) super.setAlt(alt);
}
@Override
public UpdateAsync setCallback(java.lang.String callback) {
return (UpdateAsync) super.setCallback(callback);
}
@Override
public UpdateAsync setFields(java.lang.String fields) {
return (UpdateAsync) super.setFields(fields);
}
@Override
public UpdateAsync setKey(java.lang.String key) {
return (UpdateAsync) super.setKey(key);
}
@Override
public UpdateAsync setOauthToken(java.lang.String oauthToken) {
return (UpdateAsync) super.setOauthToken(oauthToken);
}
@Override
public UpdateAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAsync setQuotaUser(java.lang.String quotaUser) {
return (UpdateAsync) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAsync setUploadType(java.lang.String uploadType) {
return (UpdateAsync) super.setUploadType(uploadType);
}
@Override
public UpdateAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAsync) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the bucket to update.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the bucket to update.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" For example:"projects
* /my-project/locations/global/buckets/my-bucket"
*/
public UpdateAsync setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask that specifies the fields in bucket that need an update. A bucket field will
be overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see: https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
updateMask=retention_days
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask that specifies the fields in bucket that need an update. A bucket
* field will be overwritten if, and only if, it is in the update mask. name and output
* only fields cannot be updated.For a detailed FieldMask definition, see:
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=retention_days
*/
public UpdateAsync setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAsync set(String parameterName, Object value) {
return (UpdateAsync) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Links collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Links.List request = logging.links().list(parameters ...)}
*
*
* @return the resource collection
*/
public Links links() {
return new Links();
}
/**
* The "links" collection of methods.
*/
public class Links {
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Asynchronously creates a linked dataset in BigQuery which makes it possible to use BigQuery to
* read the logs stored in the log bucket. A log bucket may currently only contain one link.
*
* Create a request for the method "links.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @param content the {@link com.google.api.services.logging.v2.model.Link}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.Link content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The full resource name of the bucket to create a link for.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The full resource name of the bucket to create a link for.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
@com.google.api.client.util.Key
private java.lang.String linkId;
/** Required. The ID to use for the link. The link_id can have up to 100 characters. A valid link_id
must only have alphanumeric characters and underscores within it.
*/
public java.lang.String getLinkId() {
return linkId;
}
/**
* Required. The ID to use for the link. The link_id can have up to 100 characters. A
* valid link_id must only have alphanumeric characters and underscores within it.
*/
public Create setLinkId(java.lang.String linkId) {
this.linkId = linkId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Deletes a link. This will also delete the corresponding BigQuery linked dataset.
*
* Create a request for the method "links.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the link to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the link to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
/**
* Gets a link.
*
* Create a request for the method "links.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "organ
* izations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "b
* illingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LIN
* K_ID]" "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Link.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the link:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the link:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]" "
* organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LI
* NK_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET
* _ID]/links/[LINK_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/links/[LINK_ID]"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/links/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/links";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists links.
*
* Create a request for the method "links.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose links are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose links are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. The maximum number of results to return from this request. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The maximum number of results to return from this request. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Views collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Views.List request = logging.views().list(parameters ...)}
*
*
* @return the resource collection
*/
public Views views() {
return new Views();
}
/**
* The "views" collection of methods.
*/
public class Views {
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Creates a view over log entries in a log bucket. A bucket may contain a maximum of 30 views.
*
* Create a request for the method "views.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket in which to create the view
`"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For example:"projects/my-
project/locations/global/buckets/my-bucket"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket in which to create the view
* `"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"` For
* example:"projects/my-project/locations/global/buckets/my-bucket"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
@com.google.api.client.util.Key
private java.lang.String viewId;
/** Required. A client-assigned identifier such as "my-view". Identifiers are limited to 100 characters
and can include only letters, digits, underscores, hyphens, and periods.
*/
public java.lang.String getViewId() {
return viewId;
}
/**
* Required. A client-assigned identifier such as "my-view". Identifiers are limited to
* 100 characters and can include only letters, digits, underscores, hyphens, and
* periods.
*/
public Create setViewId(java.lang.String viewId) {
this.viewId = viewId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Deletes a view on a log bucket. If an UNAVAILABLE error is returned, this indicates that system
* is not in a state where it can delete the view. If this occurs, please try again in a few
* minutes.
*
* Create a request for the method "views.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to delete:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to delete:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets a view on a log bucket.
*
* Create a request for the method "views.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the policy:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the policy:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "views.getIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.GetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/views";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
/**
* Lists views on a log bucket.
*
* Create a request for the method "views.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListViewsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bucket whose views are to be listed:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bucket whose views are to be listed:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Updates a view on a log bucket. This method replaces the value of the filter field from the
* existing view with the corresponding value from the new view. If an UNAVAILABLE error is
* returned, this indicates that system is not in a state where it can update the view. If this
* occurs, please try again in a few minutes.
*
* Create a request for the method "views.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
* example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
* @param content the {@link com.google.api.services.logging.v2.model.LogView}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.logging.v2.model.LogView content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogView.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the view to update
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]" For
example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the view to update
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]"
* For example:"projects/my-project/locations/global/buckets/my-bucket/views/my-view"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in view that need an update. A field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.For a detailed FieldMask definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in view that need an update. A field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.For a detailed FieldMask definition, see
* https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can return
* NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.Can
* return NOT_FOUND, INVALID_ARGUMENT, and PERMISSION_DENIED errors.
*
* Create a request for the method "views.setIamPolicy".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.logging.v2.model.SetIamPolicyRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends LoggingRequest {
private static final String REST_PATH = "v2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "views.testIamPermissions".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See Resource names
* (https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
* field.
* @param content the {@link com.google.api.services.logging.v2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.logging.v2.model.TestIamPermissionsRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See Resource names
(https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See Resource
* names (https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/buckets/[^/]+/views/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that
* more results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding
* call to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] o
* rganizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[V
* IEW_ID] billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKE
* T_ID]/views/[VIEW_ID]
* folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Operations.List request = logging.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.logging.v2.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.logging.v2.model.CancelOperationRequest content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the RecentQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.RecentQueries.List request = logging.recentQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public RecentQueries recentQueries() {
return new RecentQueries();
}
/**
* The "recentQueries" collection of methods.
*/
public class RecentQueries {
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/recentQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists the RecentQueries that were created by the user making the request.
*
* Create a request for the method "recentQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListRecentQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations/us-
central1Note: The location portion of the resource must be specified, but supplying the character -
in place of LOCATION_ID will return all recent queries.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example:projects/my-project/locations
* /us-central1Note: The location portion of the resource must be specified, but supplying
* the character - in place of LOCATION_ID will return all recent queries.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive
* values are ignored. The presence of nextPageToken in the response indicates that more
* results might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SavedQueries collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.SavedQueries.List request = logging.savedQueries().list(parameters ...)}
*
*
* @return the resource collection
*/
public SavedQueries savedQueries() {
return new SavedQueries();
}
/**
* The "savedQueries" collection of methods.
*/
public class SavedQueries {
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new SavedQuery for the user making the request.
*
* Create a request for the method "savedQueries.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
* @param content the {@link com.google.api.services.logging.v2.model.SavedQuery}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.SavedQuery content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.SavedQuery.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource in which to create the saved query:
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/global"
"organizations/123456789/locations/us-central1"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource in which to create the saved query:
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/global" "organizations/123456789/locations/us-central1"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
@com.google.api.client.util.Key
private java.lang.String savedQueryId;
/** Optional. The ID to use for the saved query, which will become the final component of the saved
query's resource name.If the saved_query_id is not provided, the system will generate an
alphanumeric ID.The saved_query_id is limited to 100 characters and can include only the following
characters: upper and lower-case alphanumeric characters, underscores, hyphens, periods.First
character has to be alphanumeric.
*/
public java.lang.String getSavedQueryId() {
return savedQueryId;
}
/**
* Optional. The ID to use for the saved query, which will become the final component of
* the saved query's resource name.If the saved_query_id is not provided, the system will
* generate an alphanumeric ID.The saved_query_id is limited to 100 characters and can
* include only the following characters: upper and lower-case alphanumeric characters,
* underscores, hyphens, periods.First character has to be alphanumeric.
*/
public Create setSavedQueryId(java.lang.String savedQueryId) {
this.savedQueryId = savedQueryId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
/**
* Deletes an existing SavedQuery that was created by the user making the request.
*
* Create a request for the method "savedQueries.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the saved query to delete.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example: "projects/my-
project/locations/global/savedQueries/my-saved-query"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full resource name of the saved query to delete.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]/savedQueries/[QUERY_ID]" For example:
* "projects/my-project/locations/global/savedQueries/my-saved-query"
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/savedQueries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/savedQueries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists the SavedQueries that were created by the user making the request.
*
* Create a request for the method "savedQueries.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations
* /us-central1" Note: The locations portion of the resource must be specified. To get a list
* of all saved queries, a wildcard character - can be used for LOCATION_ID, for example:
* "projects/my-project/locations/-"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSavedQueriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource to which the listed queries belong.
"projects/[PROJECT_ID]/locations/[LOCATION_ID]"
"organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
"folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-project/locations/us-
central1" Note: The locations portion of the resource must be specified. To get a list of all saved
queries, a wildcard character - can be used for LOCATION_ID, for example: "projects/my-
project/locations/-"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource to which the listed queries belong.
* "projects/[PROJECT_ID]/locations/[LOCATION_ID]"
* "organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]"
* "folders/[FOLDER_ID]/locations/[LOCATION_ID]" For example: "projects/my-
* project/locations/us-central1" Note: The locations portion of the resource must be
* specified. To get a list of all saved queries, a wildcard character - can be used for
* LOCATION_ID, for example: "projects/my-project/locations/-"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request.Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request.Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call
* to this method. pageToken must be the value of nextPageToken from the previous
* response. The values of other method parameters should be identical to those in the
* previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Logs collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Logs.List request = logging.logs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Logs logs() {
return new Logs();
}
/**
* The "logs" collection of methods.
*/
public class Logs {
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @return the request
*/
public Delete delete(java.lang.String logName) throws java.io.IOException {
Delete result = new Delete(logName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+logName}";
private final java.util.regex.Pattern LOG_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/logs/[^/]+$");
/**
* Deletes all the log entries in a log for the _Default Log Bucket. The log reappears if it
* receives new entries. Log entries written shortly before the delete operation might not be
* deleted. Entries received after the delete operation with a timestamp before the operation will
* be deleted.
*
* Create a request for the method "logs.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param logName Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For
* more information about log names, see LogEntry.
* @since 1.13
*/
protected Delete(java.lang.String logName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.logName = com.google.api.client.util.Preconditions.checkNotNull(logName, "Required parameter logName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^projects/[^/]+/logs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
@com.google.api.client.util.Key
private java.lang.String logName;
/** Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
organizations/[ORGANIZATION_ID]/logs/[LOG_ID] billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-project-
id/logs/syslog", "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information
about log names, see LogEntry.
*/
public java.lang.String getLogName() {
return logName;
}
/**
* Required. The resource name of the log to delete: projects/[PROJECT_ID]/logs/[LOG_ID]
* organizations/[ORGANIZATION_ID]/logs/[LOG_ID]
* billingAccounts/[BILLING_ACCOUNT_ID]/logs/[LOG_ID]
* folders/[FOLDER_ID]/logs/[LOG_ID][LOG_ID] must be URL-encoded. For example, "projects/my-
* project-id/logs/syslog",
* "organizations/123/logs/cloudaudit.googleapis.com%2Factivity".For more information about
* log names, see LogEntry.
*/
public Delete setLogName(java.lang.String logName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(LOG_NAME_PATTERN.matcher(logName).matches(),
"Parameter logName must conform to the pattern " +
"^projects/[^/]+/logs/[^/]+$");
}
this.logName = logName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/logs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists the logs in projects, organizations, folders, or billing accounts. Only logs that have
* entries are listed.
*
* Create a request for the method "logs.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
* billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name to list logs for: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name to list logs for: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** Optional. List of resource names to list logs for:
projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
organizations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
billingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]
folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To support legacy
queries, it could also be: projects/[PROJECT_ID] organizations/[ORGANIZATION_ID]
billingAccounts/[BILLING_ACCOUNT_ID] folders/[FOLDER_ID]The resource name in the parent field is
added to this list.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* Optional. List of resource names to list logs for:
* projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] organiz
* ations/[ORGANIZATION_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID] bill
* ingAccounts/[BILLING_ACCOUNT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_
* ID] folders/[FOLDER_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]/views/[VIEW_ID]To
* support legacy queries, it could also be: projects/[PROJECT_ID]
* organizations/[ORGANIZATION_ID] billingAccounts/[BILLING_ACCOUNT_ID]
* folders/[FOLDER_ID]The resource name in the parent field is added to this list.
*/
public List setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Metrics collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Metrics.List request = logging.metrics().list(parameters ...)}
*
*
* @return the resource collection
*/
public Metrics metrics() {
return new Metrics();
}
/**
* The "metrics" collection of methods.
*/
public class Metrics {
/**
* Creates a logs-based metric.
*
* Create a request for the method "metrics.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the project in which to create the metric: "projects/[PROJECT_ID]"
* The new metric must be provided in the request.
* @param content the {@link com.google.api.services.logging.v2.model.LogMetric}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogMetric content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/metrics";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Creates a logs-based metric.
*
* Create a request for the method "metrics.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the project in which to create the metric: "projects/[PROJECT_ID]"
* The new metric must be provided in the request.
* @param content the {@link com.google.api.services.logging.v2.model.LogMetric}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogMetric content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogMetric.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the project in which to create the metric:
* "projects/[PROJECT_ID]" The new metric must be provided in the request.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the project in which to create the metric: "projects/[PROJECT_ID]"
The new metric must be provided in the request.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the project in which to create the metric:
* "projects/[PROJECT_ID]" The new metric must be provided in the request.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a logs-based metric.
*
* Create a request for the method "metrics.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param metricName Required. The resource name of the metric to delete: "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
* @return the request
*/
public Delete delete(java.lang.String metricName) throws java.io.IOException {
Delete result = new Delete(metricName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+metricName}";
private final java.util.regex.Pattern METRIC_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/metrics/[^/]+$");
/**
* Deletes a logs-based metric.
*
* Create a request for the method "metrics.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param metricName Required. The resource name of the metric to delete: "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
* @since 1.13
*/
protected Delete(java.lang.String metricName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.metricName = com.google.api.client.util.Preconditions.checkNotNull(metricName, "Required parameter metricName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(METRIC_NAME_PATTERN.matcher(metricName).matches(),
"Parameter metricName must conform to the pattern " +
"^projects/[^/]+/metrics/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the metric to delete:
* "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String metricName;
/** Required. The resource name of the metric to delete: "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
*/
public java.lang.String getMetricName() {
return metricName;
}
/**
* Required. The resource name of the metric to delete:
* "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
*/
public Delete setMetricName(java.lang.String metricName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(METRIC_NAME_PATTERN.matcher(metricName).matches(),
"Parameter metricName must conform to the pattern " +
"^projects/[^/]+/metrics/[^/]+$");
}
this.metricName = metricName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a logs-based metric.
*
* Create a request for the method "metrics.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param metricName Required. The resource name of the desired metric: "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
* @return the request
*/
public Get get(java.lang.String metricName) throws java.io.IOException {
Get result = new Get(metricName);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+metricName}";
private final java.util.regex.Pattern METRIC_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/metrics/[^/]+$");
/**
* Gets a logs-based metric.
*
* Create a request for the method "metrics.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param metricName Required. The resource name of the desired metric: "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
* @since 1.13
*/
protected Get(java.lang.String metricName) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogMetric.class);
this.metricName = com.google.api.client.util.Preconditions.checkNotNull(metricName, "Required parameter metricName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(METRIC_NAME_PATTERN.matcher(metricName).matches(),
"Parameter metricName must conform to the pattern " +
"^projects/[^/]+/metrics/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the desired metric:
* "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String metricName;
/** Required. The resource name of the desired metric: "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
*/
public java.lang.String getMetricName() {
return metricName;
}
/**
* Required. The resource name of the desired metric:
* "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
*/
public Get setMetricName(java.lang.String metricName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(METRIC_NAME_PATTERN.matcher(metricName).matches(),
"Parameter metricName must conform to the pattern " +
"^projects/[^/]+/metrics/[^/]+$");
}
this.metricName = metricName;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists logs-based metrics.
*
* Create a request for the method "metrics.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project containing the metrics: "projects/[PROJECT_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/metrics";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists logs-based metrics.
*
* Create a request for the method "metrics.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project containing the metrics: "projects/[PROJECT_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListLogMetricsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the project containing the metrics: "projects/[PROJECT_ID]" */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project containing the metrics: "projects/[PROJECT_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The name of the project containing the metrics: "projects/[PROJECT_ID]" */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Creates or updates a logs-based metric.
*
* Create a request for the method "metrics.update".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param metricName Required. The resource name of the metric to update: "projects/[PROJECT_ID]/metrics/[METRIC_ID]" The
* updated metric must be provided in the request and it's name field must be the same as
* [METRIC_ID] If the metric does not exist in [PROJECT_ID], then a new metric is created.
* @param content the {@link com.google.api.services.logging.v2.model.LogMetric}
* @return the request
*/
public Update update(java.lang.String metricName, com.google.api.services.logging.v2.model.LogMetric content) throws java.io.IOException {
Update result = new Update(metricName, content);
initialize(result);
return result;
}
public class Update extends LoggingRequest {
private static final String REST_PATH = "v2/{+metricName}";
private final java.util.regex.Pattern METRIC_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/metrics/[^/]+$");
/**
* Creates or updates a logs-based metric.
*
* Create a request for the method "metrics.update".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param metricName Required. The resource name of the metric to update: "projects/[PROJECT_ID]/metrics/[METRIC_ID]" The
* updated metric must be provided in the request and it's name field must be the same as
* [METRIC_ID] If the metric does not exist in [PROJECT_ID], then a new metric is created.
* @param content the {@link com.google.api.services.logging.v2.model.LogMetric}
* @since 1.13
*/
protected Update(java.lang.String metricName, com.google.api.services.logging.v2.model.LogMetric content) {
super(Logging.this, "PUT", REST_PATH, content, com.google.api.services.logging.v2.model.LogMetric.class);
this.metricName = com.google.api.client.util.Preconditions.checkNotNull(metricName, "Required parameter metricName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(METRIC_NAME_PATTERN.matcher(metricName).matches(),
"Parameter metricName must conform to the pattern " +
"^projects/[^/]+/metrics/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the metric to update:
* "projects/[PROJECT_ID]/metrics/[METRIC_ID]" The updated metric must be provided in the
* request and it's name field must be the same as [METRIC_ID] If the metric does not exist
* in [PROJECT_ID], then a new metric is created.
*/
@com.google.api.client.util.Key
private java.lang.String metricName;
/** Required. The resource name of the metric to update: "projects/[PROJECT_ID]/metrics/[METRIC_ID]"
The updated metric must be provided in the request and it's name field must be the same as
[METRIC_ID] If the metric does not exist in [PROJECT_ID], then a new metric is created.
*/
public java.lang.String getMetricName() {
return metricName;
}
/**
* Required. The resource name of the metric to update:
* "projects/[PROJECT_ID]/metrics/[METRIC_ID]" The updated metric must be provided in the
* request and it's name field must be the same as [METRIC_ID] If the metric does not exist
* in [PROJECT_ID], then a new metric is created.
*/
public Update setMetricName(java.lang.String metricName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(METRIC_NAME_PATTERN.matcher(metricName).matches(),
"Parameter metricName must conform to the pattern " +
"^projects/[^/]+/metrics/[^/]+$");
}
this.metricName = metricName;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Sinks collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Sinks.List request = logging.sinks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sinks sinks() {
return new Sinks();
}
/**
* The "sinks" collection of methods.
*/
public class Sinks {
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "POST", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]" For
examples:"projects/my-project" "organizations/123456789"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Create setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. Determines the kind of IAM identity returned as writer_identity in the new sink. If this
value is omitted or set to false, and if the sink's parent is a project, then the value returned as
writer_identity is the same group or service account used by Cloud Logging before the addition of
writer identities to this API. The sink's destination must be in the same project as the sink
itself.If this field is set to true, or if the sink is owned by a non-project resource such as an
organization, then the value of writer_identity will be a service agent
(https://cloud.google.com/iam/docs/service-account-types#service-agents) used by the sinks with the
same parent. For more information, see writer_identity in LogSink.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. Determines the kind of IAM identity returned as writer_identity in the new
* sink. If this value is omitted or set to false, and if the sink's parent is a project,
* then the value returned as writer_identity is the same group or service account used by
* Cloud Logging before the addition of writer identities to this API. The sink's
* destination must be in the same project as the sink itself.If this field is set to true,
* or if the sink is owned by a non-project resource such as an organization, then the value
* of writer_identity will be a service agent (https://cloud.google.com/iam/docs/service-
* account-types#service-agents) used by the sinks with the same parent. For more
* information, see writer_identity in LogSink.
*/
public Create setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Delete delete(java.lang.String sinkName) throws java.io.IOException {
Delete result = new Delete(sinkName);
initialize(result);
return result;
}
public class Delete extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/sinks/[^/]+$");
/**
* Deletes a sink. If the sink has a unique writer_identity, then that service account is also
* deleted.
*
* Create a request for the method "sinks.delete".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to delete, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Delete(java.lang.String sinkName) {
super(Logging.this, "DELETE", REST_PATH, null, com.google.api.services.logging.v2.model.Empty.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to delete, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to delete, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Delete setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @return the request
*/
public Get get(java.lang.String sinkName) throws java.io.IOException {
Get result = new Get(sinkName);
initialize(result);
return result;
}
public class Get extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/sinks/[^/]+$");
/**
* Gets a sink.
*
* Create a request for the method "sinks.get".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @since 1.13
*/
protected Get(java.lang.String sinkName) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The resource name of the sink: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Get setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists sinks.
*
* Create a request for the method "sinks.list".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Logging.this, "GET", REST_PATH, null, com.google.api.services.logging.v2.model.ListSinksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
"organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]" "folders/[FOLDER_ID]"
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource whose sinks are to be listed: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]"
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter expression to constrain the sinks returned. Today, this only supports the
following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")', 'in_scope("DEFAULT")'.Description
of scopes below. ALL: Includes all of the sinks which can be returned in any other scope. ANCESTOR:
Includes intercepting sinks owned by ancestor resources. DEFAULT: Includes sinks owned by
parent.When the empty string is provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter expression to constrain the sinks returned. Today, this only supports
* the following strings: '' 'in_scope("ALL")', 'in_scope("ANCESTOR")',
* 'in_scope("DEFAULT")'.Description of scopes below. ALL: Includes all of the sinks which
* can be returned in any other scope. ANCESTOR: Includes intercepting sinks owned by
* ancestor resources. DEFAULT: Includes sinks owned by parent.When the empty string is
* provided, then the filter 'in_scope("DEFAULT")' is applied.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return from this request. Non-positive values are
ignored. The presence of nextPageToken in the response indicates that more results might be
available.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of results to return from this request. Non-positive values
* are ignored. The presence of nextPageToken in the response indicates that more results
* might be available.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If present, then retrieve the next batch of results from the preceding call to this
method. pageToken must be the value of nextPageToken from the previous response. The values of
other method parameters should be identical to those in the previous call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If present, then retrieve the next batch of results from the preceding call to
* this method. pageToken must be the value of nextPageToken from the previous response. The
* values of other method parameters should be identical to those in the previous call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Patch patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Patch result = new Patch(sinkName, content);
initialize(result);
return result;
}
public class Patch extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.patch".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Patch(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PATCH", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Patch setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Patch setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Patch setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have a
* new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Update update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Update result = new Update(sinkName, content);
initialize(result);
return result;
}
public class Update extends LoggingRequest {
private static final String REST_PATH = "v2/{+sinkName}";
private final java.util.regex.Pattern SINK_NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/sinks/[^/]+$");
/**
* Updates a sink. This method replaces the values of the destination and filter fields of the
* existing sink with the corresponding values from the new sink.The updated sink might also have
* a new writer_identity; see the unique_writer_identity field.
*
* Create a request for the method "sinks.update".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param sinkName Required. The full resource name of the sink to update, including the parent resource and the sink
* identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @since 1.13
*/
protected Update(java.lang.String sinkName, com.google.api.services.logging.v2.model.LogSink content) {
super(Logging.this, "PUT", REST_PATH, content, com.google.api.services.logging.v2.model.LogSink.class);
this.sinkName = com.google.api.client.util.Preconditions.checkNotNull(sinkName, "Required parameter sinkName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
@com.google.api.client.util.Key
private java.lang.String sinkName;
/** Required. The full resource name of the sink to update, including the parent resource and the sink
identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
"organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
"billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]" "folders/[FOLDER_ID]/sinks/[SINK_ID]" For
example:"projects/my-project/sinks/my-sink"
*/
public java.lang.String getSinkName() {
return sinkName;
}
/**
* Required. The full resource name of the sink to update, including the parent resource and
* the sink identifier: "projects/[PROJECT_ID]/sinks/[SINK_ID]"
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_ID]"
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_ID]"
* "folders/[FOLDER_ID]/sinks/[SINK_ID]" For example:"projects/my-project/sinks/my-sink"
*/
public Update setSinkName(java.lang.String sinkName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SINK_NAME_PATTERN.matcher(sinkName).matches(),
"Parameter sinkName must conform to the pattern " +
"^projects/[^/]+/sinks/[^/]+$");
}
this.sinkName = sinkName;
return this;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
@com.google.api.client.util.Key
private java.lang.String customWriterIdentity;
/** Optional. A service account provided by the caller that will be used to write the log entries. The
format must be serviceAccount:some@email. This field can only be specified if you are routing logs
to a destination outside this sink's project. If not specified, a Logging service account will
automatically be generated.
*/
public java.lang.String getCustomWriterIdentity() {
return customWriterIdentity;
}
/**
* Optional. A service account provided by the caller that will be used to write the log
* entries. The format must be serviceAccount:some@email. This field can only be specified
* if you are routing logs to a destination outside this sink's project. If not specified, a
* Logging service account will automatically be generated.
*/
public Update setCustomWriterIdentity(java.lang.String customWriterIdentity) {
this.customWriterIdentity = customWriterIdentity;
return this;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uniqueWriterIdentity;
/** Optional. See sinks.create for a description of this field. When updating a sink, the effect of
this field on the value of writer_identity in the updated sink depends on both the old and new
values of this field: If the old and new values of this field are both false or both true, then
there is no change to the sink's writer_identity. If the old value is false and the new value is
true, then writer_identity is changed to a service agent (https://cloud.google.com/iam/docs
/service-account-types#service-agents) owned by Cloud Logging. It is an error if the old value is
true and the new value is set to false or defaulted to false.
*/
public java.lang.Boolean getUniqueWriterIdentity() {
return uniqueWriterIdentity;
}
/**
* Optional. See sinks.create for a description of this field. When updating a sink, the
* effect of this field on the value of writer_identity in the updated sink depends on both
* the old and new values of this field: If the old and new values of this field are both
* false or both true, then there is no change to the sink's writer_identity. If the old
* value is false and the new value is true, then writer_identity is changed to a service
* agent (https://cloud.google.com/iam/docs/service-account-types#service-agents) owned by
* Cloud Logging. It is an error if the old value is true and the new value is set to false
* or defaulted to false.
*/
public Update setUniqueWriterIdentity(java.lang.Boolean uniqueWriterIdentity) {
this.uniqueWriterIdentity = uniqueWriterIdentity;
return this;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Field mask that specifies the fields in sink that need an update. A sink field will be
overwritten if, and only if, it is in the update mask. name and output only fields cannot be
updated.An empty updateMask is temporarily treated as using the following mask for backwards
compatibility purposes:destination,filter,includeChildrenAt some point in the future, behavior will
be removed and specifying an empty updateMask will be an error.For a detailed FieldMask definition,
see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example: updateMask=filter
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Field mask that specifies the fields in sink that need an update. A sink field
* will be overwritten if, and only if, it is in the update mask. name and output only
* fields cannot be updated.An empty updateMask is temporarily treated as using the
* following mask for backwards compatibility purposes:destination,filter,includeChildrenAt
* some point in the future, behavior will be removed and specifying an empty updateMask
* will be an error.For a detailed FieldMask definition, see https://developers.google.com
* /protocol-buffers/docs/reference/google.protobuf#google.protobuf.FieldMaskFor example:
* updateMask=filter
*/
public Update setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Sinks collection.
*
* The typical use is:
*
* {@code Logging logging = new Logging(...);}
* {@code Logging.Sinks.List request = logging.sinks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sinks sinks() {
return new Sinks();
}
/**
* The "sinks" collection of methods.
*/
public class Sinks {
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource in which to create the sink: "projects/[PROJECT_ID]"
* "organizations/[ORGANIZATION_ID]" "billingAccounts/[BILLING_ACCOUNT_ID]"
* "folders/[FOLDER_ID]" For examples:"projects/my-project" "organizations/123456789"
* @param content the {@link com.google.api.services.logging.v2.model.LogSink}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.logging.v2.model.LogSink content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends LoggingRequest {
private static final String REST_PATH = "v2/{+parent}/sinks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^[^/]+/[^/]+$");
/**
* Creates a sink that exports specified log entries to a destination. The export begins upon
* ingress, unless the sink's writer_identity is not permitted to write to the destination. A sink
* can export log entries only from the resource owning the sink.
*
* Create a request for the method "sinks.create".
*
* This request holds the parameters needed by the the logging server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.