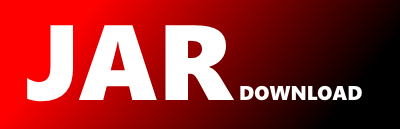
com.google.api.services.logging.v2.model.LogSink Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.logging.v2.model;
/**
* Describes a sink used to export log entries to one of the following destinations: a Cloud Logging
* log bucket, a Cloud Storage bucket, a BigQuery dataset, a Pub/Sub topic, a Cloud project.A logs
* filter controls which log entries are exported. The sink must be created within a project,
* organization, billing account, or folder.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Logging API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class LogSink extends com.google.api.client.json.GenericJson {
/**
* Optional. Options that affect sinks exporting data to BigQuery.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BigQueryOptions bigqueryOptions;
/**
* Output only. The creation timestamp of the sink.This field may not be present for older sinks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Optional. A description of this sink.The maximum length of the description is 8000 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Required. The export destination: "storage.googleapis.com/[GCS_BUCKET]"
* "bigquery.googleapis.com/projects/[PROJECT_ID]/datasets/[DATASET]"
* "pubsub.googleapis.com/projects/[PROJECT_ID]/topics/[TOPIC_ID]"
* "logging.googleapis.com/projects/[PROJECT_ID]"
* "logging.googleapis.com/projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" The
* sink's writer_identity, set when the sink is created, must have permission to write to the
* destination or else the log entries are not exported. For more information, see Exporting Logs
* with Sinks (https://cloud.google.com/logging/docs/api/tasks/exporting-logs).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String destination;
/**
* Optional. If set to true, then this sink is disabled and it does not export any log entries.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean disabled;
/**
* Optional. Log entries that match any of these exclusion filters will not be exported.If a log
* entry is matched by both filter and one of exclusion_filters it will not be exported.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List exclusions;
static {
// hack to force ProGuard to consider LogExclusion used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(LogExclusion.class);
}
/**
* Optional. An advanced logs filter (https://cloud.google.com/logging/docs/view/advanced-
* queries). The only exported log entries are those that are in the resource owning the sink and
* that match the filter.For example:logName="projects/[PROJECT_ID]/logs/[LOG_ID]" AND
* severity>=ERROR
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**
* Optional. This field applies only to sinks owned by organizations and folders. If the field is
* false, the default, only the logs owned by the sink's parent resource are available for export.
* If the field is true, then log entries from all the projects, folders, and billing accounts
* contained in the sink's parent resource are also available for export. Whether a particular log
* entry from the children is exported depends on the sink's filter expression.For example, if
* this field is true, then the filter resource.type=gce_instance would export all Compute Engine
* VM instance log entries from all projects in the sink's parent.To only export entries from
* certain child projects, filter on the project part of the log name:logName:("projects/test-
* project1/" OR "projects/test-project2/") AND resource.type=gce_instance
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeChildren;
/**
* Optional. This field applies only to sinks owned by organizations and folders.When the value of
* 'intercept_children' is true, the following restrictions apply: The sink must have the
* include_children flag set to true. The sink destination must be a Cloud project.Also, the
* following behaviors apply: Any logs matched by the sink won't be included by non-_Required
* sinks owned by child resources. The sink appears in the results of a ListSinks call from a
* child resource if the value of the filter field in its request is either 'in_scope("ALL")' or
* 'in_scope("ANCESTOR")'.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean interceptChildren;
/**
* Output only. The client-assigned sink identifier, unique within the project.For example: "my-
* syslog-errors-to-pubsub".Sink identifiers are limited to 100 characters and can include only
* the following characters: upper and lower-case alphanumeric characters, underscores, hyphens,
* periods.First character has to be alphanumeric.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Deprecated. This field is unused.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String outputVersionFormat;
/**
* Output only. The resource name of the sink. "projects/[PROJECT_ID]/sinks/[SINK_NAME]
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_NAME]
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_NAME] "folders/[FOLDER_ID]/sinks/[SINK_NAME]
* For example: projects/my_project/sinks/SINK_NAME
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceName;
/**
* Output only. The last update timestamp of the sink.This field may not be present for older
* sinks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Output only. An IAM identity—a service account or group—under which Cloud Logging writes the
* exported log entries to the sink's destination. This field is either set by specifying
* custom_writer_identity or set automatically by sinks.create and sinks.update based on the value
* of unique_writer_identity in those methods.Until you grant this identity write-access to the
* destination, log entry exports from this sink will fail. For more information, see Granting
* Access for a Resource (https://cloud.google.com/iam/docs/granting-roles-to-service-
* accounts#granting_access_to_a_service_account_for_a_resource). Consult the destination
* service's documentation to determine the appropriate IAM roles to assign to the identity.Sinks
* that have a destination that is a log bucket in the same project as the sink cannot have a
* writer_identity and no additional permissions are required.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String writerIdentity;
/**
* Optional. Options that affect sinks exporting data to BigQuery.
* @return value or {@code null} for none
*/
public BigQueryOptions getBigqueryOptions() {
return bigqueryOptions;
}
/**
* Optional. Options that affect sinks exporting data to BigQuery.
* @param bigqueryOptions bigqueryOptions or {@code null} for none
*/
public LogSink setBigqueryOptions(BigQueryOptions bigqueryOptions) {
this.bigqueryOptions = bigqueryOptions;
return this;
}
/**
* Output only. The creation timestamp of the sink.This field may not be present for older sinks.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The creation timestamp of the sink.This field may not be present for older sinks.
* @param createTime createTime or {@code null} for none
*/
public LogSink setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Optional. A description of this sink.The maximum length of the description is 8000 characters.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Optional. A description of this sink.The maximum length of the description is 8000 characters.
* @param description description or {@code null} for none
*/
public LogSink setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Required. The export destination: "storage.googleapis.com/[GCS_BUCKET]"
* "bigquery.googleapis.com/projects/[PROJECT_ID]/datasets/[DATASET]"
* "pubsub.googleapis.com/projects/[PROJECT_ID]/topics/[TOPIC_ID]"
* "logging.googleapis.com/projects/[PROJECT_ID]"
* "logging.googleapis.com/projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" The
* sink's writer_identity, set when the sink is created, must have permission to write to the
* destination or else the log entries are not exported. For more information, see Exporting Logs
* with Sinks (https://cloud.google.com/logging/docs/api/tasks/exporting-logs).
* @return value or {@code null} for none
*/
public java.lang.String getDestination() {
return destination;
}
/**
* Required. The export destination: "storage.googleapis.com/[GCS_BUCKET]"
* "bigquery.googleapis.com/projects/[PROJECT_ID]/datasets/[DATASET]"
* "pubsub.googleapis.com/projects/[PROJECT_ID]/topics/[TOPIC_ID]"
* "logging.googleapis.com/projects/[PROJECT_ID]"
* "logging.googleapis.com/projects/[PROJECT_ID]/locations/[LOCATION_ID]/buckets/[BUCKET_ID]" The
* sink's writer_identity, set when the sink is created, must have permission to write to the
* destination or else the log entries are not exported. For more information, see Exporting Logs
* with Sinks (https://cloud.google.com/logging/docs/api/tasks/exporting-logs).
* @param destination destination or {@code null} for none
*/
public LogSink setDestination(java.lang.String destination) {
this.destination = destination;
return this;
}
/**
* Optional. If set to true, then this sink is disabled and it does not export any log entries.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDisabled() {
return disabled;
}
/**
* Optional. If set to true, then this sink is disabled and it does not export any log entries.
* @param disabled disabled or {@code null} for none
*/
public LogSink setDisabled(java.lang.Boolean disabled) {
this.disabled = disabled;
return this;
}
/**
* Optional. Log entries that match any of these exclusion filters will not be exported.If a log
* entry is matched by both filter and one of exclusion_filters it will not be exported.
* @return value or {@code null} for none
*/
public java.util.List getExclusions() {
return exclusions;
}
/**
* Optional. Log entries that match any of these exclusion filters will not be exported.If a log
* entry is matched by both filter and one of exclusion_filters it will not be exported.
* @param exclusions exclusions or {@code null} for none
*/
public LogSink setExclusions(java.util.List exclusions) {
this.exclusions = exclusions;
return this;
}
/**
* Optional. An advanced logs filter (https://cloud.google.com/logging/docs/view/advanced-
* queries). The only exported log entries are those that are in the resource owning the sink and
* that match the filter.For example:logName="projects/[PROJECT_ID]/logs/[LOG_ID]" AND
* severity>=ERROR
* @return value or {@code null} for none
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. An advanced logs filter (https://cloud.google.com/logging/docs/view/advanced-
* queries). The only exported log entries are those that are in the resource owning the sink and
* that match the filter.For example:logName="projects/[PROJECT_ID]/logs/[LOG_ID]" AND
* severity>=ERROR
* @param filter filter or {@code null} for none
*/
public LogSink setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. This field applies only to sinks owned by organizations and folders. If the field is
* false, the default, only the logs owned by the sink's parent resource are available for export.
* If the field is true, then log entries from all the projects, folders, and billing accounts
* contained in the sink's parent resource are also available for export. Whether a particular log
* entry from the children is exported depends on the sink's filter expression.For example, if
* this field is true, then the filter resource.type=gce_instance would export all Compute Engine
* VM instance log entries from all projects in the sink's parent.To only export entries from
* certain child projects, filter on the project part of the log name:logName:("projects/test-
* project1/" OR "projects/test-project2/") AND resource.type=gce_instance
* @return value or {@code null} for none
*/
public java.lang.Boolean getIncludeChildren() {
return includeChildren;
}
/**
* Optional. This field applies only to sinks owned by organizations and folders. If the field is
* false, the default, only the logs owned by the sink's parent resource are available for export.
* If the field is true, then log entries from all the projects, folders, and billing accounts
* contained in the sink's parent resource are also available for export. Whether a particular log
* entry from the children is exported depends on the sink's filter expression.For example, if
* this field is true, then the filter resource.type=gce_instance would export all Compute Engine
* VM instance log entries from all projects in the sink's parent.To only export entries from
* certain child projects, filter on the project part of the log name:logName:("projects/test-
* project1/" OR "projects/test-project2/") AND resource.type=gce_instance
* @param includeChildren includeChildren or {@code null} for none
*/
public LogSink setIncludeChildren(java.lang.Boolean includeChildren) {
this.includeChildren = includeChildren;
return this;
}
/**
* Optional. This field applies only to sinks owned by organizations and folders.When the value of
* 'intercept_children' is true, the following restrictions apply: The sink must have the
* include_children flag set to true. The sink destination must be a Cloud project.Also, the
* following behaviors apply: Any logs matched by the sink won't be included by non-_Required
* sinks owned by child resources. The sink appears in the results of a ListSinks call from a
* child resource if the value of the filter field in its request is either 'in_scope("ALL")' or
* 'in_scope("ANCESTOR")'.
* @return value or {@code null} for none
*/
public java.lang.Boolean getInterceptChildren() {
return interceptChildren;
}
/**
* Optional. This field applies only to sinks owned by organizations and folders.When the value of
* 'intercept_children' is true, the following restrictions apply: The sink must have the
* include_children flag set to true. The sink destination must be a Cloud project.Also, the
* following behaviors apply: Any logs matched by the sink won't be included by non-_Required
* sinks owned by child resources. The sink appears in the results of a ListSinks call from a
* child resource if the value of the filter field in its request is either 'in_scope("ALL")' or
* 'in_scope("ANCESTOR")'.
* @param interceptChildren interceptChildren or {@code null} for none
*/
public LogSink setInterceptChildren(java.lang.Boolean interceptChildren) {
this.interceptChildren = interceptChildren;
return this;
}
/**
* Output only. The client-assigned sink identifier, unique within the project.For example: "my-
* syslog-errors-to-pubsub".Sink identifiers are limited to 100 characters and can include only
* the following characters: upper and lower-case alphanumeric characters, underscores, hyphens,
* periods.First character has to be alphanumeric.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The client-assigned sink identifier, unique within the project.For example: "my-
* syslog-errors-to-pubsub".Sink identifiers are limited to 100 characters and can include only
* the following characters: upper and lower-case alphanumeric characters, underscores, hyphens,
* periods.First character has to be alphanumeric.
* @param name name or {@code null} for none
*/
public LogSink setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Deprecated. This field is unused.
* @return value or {@code null} for none
*/
public java.lang.String getOutputVersionFormat() {
return outputVersionFormat;
}
/**
* Deprecated. This field is unused.
* @param outputVersionFormat outputVersionFormat or {@code null} for none
*/
public LogSink setOutputVersionFormat(java.lang.String outputVersionFormat) {
this.outputVersionFormat = outputVersionFormat;
return this;
}
/**
* Output only. The resource name of the sink. "projects/[PROJECT_ID]/sinks/[SINK_NAME]
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_NAME]
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_NAME] "folders/[FOLDER_ID]/sinks/[SINK_NAME]
* For example: projects/my_project/sinks/SINK_NAME
* @return value or {@code null} for none
*/
public java.lang.String getResourceName() {
return resourceName;
}
/**
* Output only. The resource name of the sink. "projects/[PROJECT_ID]/sinks/[SINK_NAME]
* "organizations/[ORGANIZATION_ID]/sinks/[SINK_NAME]
* "billingAccounts/[BILLING_ACCOUNT_ID]/sinks/[SINK_NAME] "folders/[FOLDER_ID]/sinks/[SINK_NAME]
* For example: projects/my_project/sinks/SINK_NAME
* @param resourceName resourceName or {@code null} for none
*/
public LogSink setResourceName(java.lang.String resourceName) {
this.resourceName = resourceName;
return this;
}
/**
* Output only. The last update timestamp of the sink.This field may not be present for older
* sinks.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The last update timestamp of the sink.This field may not be present for older
* sinks.
* @param updateTime updateTime or {@code null} for none
*/
public LogSink setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* Output only. An IAM identity—a service account or group—under which Cloud Logging writes the
* exported log entries to the sink's destination. This field is either set by specifying
* custom_writer_identity or set automatically by sinks.create and sinks.update based on the value
* of unique_writer_identity in those methods.Until you grant this identity write-access to the
* destination, log entry exports from this sink will fail. For more information, see Granting
* Access for a Resource (https://cloud.google.com/iam/docs/granting-roles-to-service-
* accounts#granting_access_to_a_service_account_for_a_resource). Consult the destination
* service's documentation to determine the appropriate IAM roles to assign to the identity.Sinks
* that have a destination that is a log bucket in the same project as the sink cannot have a
* writer_identity and no additional permissions are required.
* @return value or {@code null} for none
*/
public java.lang.String getWriterIdentity() {
return writerIdentity;
}
/**
* Output only. An IAM identity—a service account or group—under which Cloud Logging writes the
* exported log entries to the sink's destination. This field is either set by specifying
* custom_writer_identity or set automatically by sinks.create and sinks.update based on the value
* of unique_writer_identity in those methods.Until you grant this identity write-access to the
* destination, log entry exports from this sink will fail. For more information, see Granting
* Access for a Resource (https://cloud.google.com/iam/docs/granting-roles-to-service-
* accounts#granting_access_to_a_service_account_for_a_resource). Consult the destination
* service's documentation to determine the appropriate IAM roles to assign to the identity.Sinks
* that have a destination that is a log bucket in the same project as the sink cannot have a
* writer_identity and no additional permissions are required.
* @param writerIdentity writerIdentity or {@code null} for none
*/
public LogSink setWriterIdentity(java.lang.String writerIdentity) {
this.writerIdentity = writerIdentity;
return this;
}
@Override
public LogSink set(String fieldName, Object value) {
return (LogSink) super.set(fieldName, value);
}
@Override
public LogSink clone() {
return (LogSink) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy