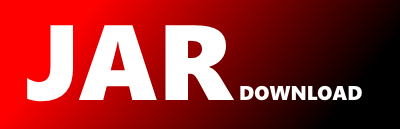
com.google.api.services.logging.v2beta1.model.LogEntry Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-10-11 at 14:55:20 UTC
* Modify at your own risk.
*/
package com.google.api.services.logging.v2beta1.model;
/**
* An individual entry in a log.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Stackdriver Logging API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class LogEntry extends com.google.api.client.json.GenericJson {
/**
* Optional. Information about the HTTP request associated with this log entry, if applicable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private HttpRequest httpRequest;
/**
* Optional. A unique ID for the log entry. If you provide this field, the logging service
* considers other log entries in the same project with the same ID as duplicates which can be
* removed. If omitted, Stackdriver Logging will generate a unique ID for this log entry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String insertId;
/**
* The log entry payload, represented as a structure that is expressed as a JSON object.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map jsonPayload;
/**
* Optional. A set of user-defined (key, value) data that provides additional information about
* the log entry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Required. The resource name of the log to which this log entry belongs. The format of the name
* is `"projects//logs/"`. Examples: `"projects/my-projectid/logs/syslog"`, `"projects/my-
* projectid/logs/library.googleapis.com%2Fbook_log"`.
*
* The log ID part of resource name must be less than 512 characters long and can only include the
* following characters: upper and lower case alphanumeric characters: [A-Za-z0-9]; and
* punctuation characters: forward-slash, underscore, hyphen, and period. Forward-slash (`/`)
* characters in the log ID must be URL-encoded.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String logName;
/**
* Optional. Information about an operation associated with the log entry, if applicable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LogEntryOperation operation;
/**
* The log entry payload, represented as a protocol buffer. Some Google Cloud Platform services
* use this field for their log entry payloads.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map protoPayload;
/**
* Required. The monitored resource associated with this log entry. Example: a log entry that
* reports a database error would be associated with the monitored resource designating the
* particular database that reported the error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MonitoredResource resource;
/**
* Optional. The severity of the log entry. The default value is `LogSeverity.DEFAULT`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String severity;
/**
* The log entry payload, represented as a Unicode string (UTF-8).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String textPayload;
/**
* Optional. The time the event described by the log entry occurred. If omitted, Stackdriver
* Logging will use the time the log entry is received.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String timestamp;
/**
* Optional. Information about the HTTP request associated with this log entry, if applicable.
* @return value or {@code null} for none
*/
public HttpRequest getHttpRequest() {
return httpRequest;
}
/**
* Optional. Information about the HTTP request associated with this log entry, if applicable.
* @param httpRequest httpRequest or {@code null} for none
*/
public LogEntry setHttpRequest(HttpRequest httpRequest) {
this.httpRequest = httpRequest;
return this;
}
/**
* Optional. A unique ID for the log entry. If you provide this field, the logging service
* considers other log entries in the same project with the same ID as duplicates which can be
* removed. If omitted, Stackdriver Logging will generate a unique ID for this log entry.
* @return value or {@code null} for none
*/
public java.lang.String getInsertId() {
return insertId;
}
/**
* Optional. A unique ID for the log entry. If you provide this field, the logging service
* considers other log entries in the same project with the same ID as duplicates which can be
* removed. If omitted, Stackdriver Logging will generate a unique ID for this log entry.
* @param insertId insertId or {@code null} for none
*/
public LogEntry setInsertId(java.lang.String insertId) {
this.insertId = insertId;
return this;
}
/**
* The log entry payload, represented as a structure that is expressed as a JSON object.
* @return value or {@code null} for none
*/
public java.util.Map getJsonPayload() {
return jsonPayload;
}
/**
* The log entry payload, represented as a structure that is expressed as a JSON object.
* @param jsonPayload jsonPayload or {@code null} for none
*/
public LogEntry setJsonPayload(java.util.Map jsonPayload) {
this.jsonPayload = jsonPayload;
return this;
}
/**
* Optional. A set of user-defined (key, value) data that provides additional information about
* the log entry.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Optional. A set of user-defined (key, value) data that provides additional information about
* the log entry.
* @param labels labels or {@code null} for none
*/
public LogEntry setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Required. The resource name of the log to which this log entry belongs. The format of the name
* is `"projects//logs/"`. Examples: `"projects/my-projectid/logs/syslog"`, `"projects/my-
* projectid/logs/library.googleapis.com%2Fbook_log"`.
*
* The log ID part of resource name must be less than 512 characters long and can only include the
* following characters: upper and lower case alphanumeric characters: [A-Za-z0-9]; and
* punctuation characters: forward-slash, underscore, hyphen, and period. Forward-slash (`/`)
* characters in the log ID must be URL-encoded.
* @return value or {@code null} for none
*/
public java.lang.String getLogName() {
return logName;
}
/**
* Required. The resource name of the log to which this log entry belongs. The format of the name
* is `"projects//logs/"`. Examples: `"projects/my-projectid/logs/syslog"`, `"projects/my-
* projectid/logs/library.googleapis.com%2Fbook_log"`.
*
* The log ID part of resource name must be less than 512 characters long and can only include the
* following characters: upper and lower case alphanumeric characters: [A-Za-z0-9]; and
* punctuation characters: forward-slash, underscore, hyphen, and period. Forward-slash (`/`)
* characters in the log ID must be URL-encoded.
* @param logName logName or {@code null} for none
*/
public LogEntry setLogName(java.lang.String logName) {
this.logName = logName;
return this;
}
/**
* Optional. Information about an operation associated with the log entry, if applicable.
* @return value or {@code null} for none
*/
public LogEntryOperation getOperation() {
return operation;
}
/**
* Optional. Information about an operation associated with the log entry, if applicable.
* @param operation operation or {@code null} for none
*/
public LogEntry setOperation(LogEntryOperation operation) {
this.operation = operation;
return this;
}
/**
* The log entry payload, represented as a protocol buffer. Some Google Cloud Platform services
* use this field for their log entry payloads.
* @return value or {@code null} for none
*/
public java.util.Map getProtoPayload() {
return protoPayload;
}
/**
* The log entry payload, represented as a protocol buffer. Some Google Cloud Platform services
* use this field for their log entry payloads.
* @param protoPayload protoPayload or {@code null} for none
*/
public LogEntry setProtoPayload(java.util.Map protoPayload) {
this.protoPayload = protoPayload;
return this;
}
/**
* Required. The monitored resource associated with this log entry. Example: a log entry that
* reports a database error would be associated with the monitored resource designating the
* particular database that reported the error.
* @return value or {@code null} for none
*/
public MonitoredResource getResource() {
return resource;
}
/**
* Required. The monitored resource associated with this log entry. Example: a log entry that
* reports a database error would be associated with the monitored resource designating the
* particular database that reported the error.
* @param resource resource or {@code null} for none
*/
public LogEntry setResource(MonitoredResource resource) {
this.resource = resource;
return this;
}
/**
* Optional. The severity of the log entry. The default value is `LogSeverity.DEFAULT`.
* @return value or {@code null} for none
*/
public java.lang.String getSeverity() {
return severity;
}
/**
* Optional. The severity of the log entry. The default value is `LogSeverity.DEFAULT`.
* @param severity severity or {@code null} for none
*/
public LogEntry setSeverity(java.lang.String severity) {
this.severity = severity;
return this;
}
/**
* The log entry payload, represented as a Unicode string (UTF-8).
* @return value or {@code null} for none
*/
public java.lang.String getTextPayload() {
return textPayload;
}
/**
* The log entry payload, represented as a Unicode string (UTF-8).
* @param textPayload textPayload or {@code null} for none
*/
public LogEntry setTextPayload(java.lang.String textPayload) {
this.textPayload = textPayload;
return this;
}
/**
* Optional. The time the event described by the log entry occurred. If omitted, Stackdriver
* Logging will use the time the log entry is received.
* @return value or {@code null} for none
*/
public String getTimestamp() {
return timestamp;
}
/**
* Optional. The time the event described by the log entry occurred. If omitted, Stackdriver
* Logging will use the time the log entry is received.
* @param timestamp timestamp or {@code null} for none
*/
public LogEntry setTimestamp(String timestamp) {
this.timestamp = timestamp;
return this;
}
@Override
public LogEntry set(String fieldName, Object value) {
return (LogEntry) super.set(fieldName, value);
}
@Override
public LogEntry clone() {
return (LogEntry) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy