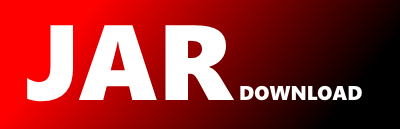
com.google.api.services.managedidentities.v1beta1.ManagedServiceforMicrosoftActiveDirectoryConsumerAPI Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.managedidentities.v1beta1;
/**
* Service definition for ManagedServiceforMicrosoftActiveDirectoryConsumerAPI (v1beta1).
*
*
* The Managed Service for Microsoft Active Directory API is used for managing a highly available, hardened service running Microsoft Active Directory (AD).
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class ManagedServiceforMicrosoftActiveDirectoryConsumerAPI extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Managed Service for Microsoft Active Directory API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://managedidentities.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://managedidentities.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.Projects.List request = managedidentities.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.Locations.List request = managedidentities.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
`"displayName=tokyo"`, and is documented in more detail in [AIP-160](https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the `next_page_token` field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Global collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.Global.List request = managedidentities.global().list(parameters ...)}
*
*
* @return the resource collection
*/
public Global global() {
return new Global();
}
/**
* The "global" collection of methods.
*/
public class Global {
/**
* An accessor for creating requests from the Domains collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.Domains.List request = managedidentities.domains().list(parameters ...)}
*
*
* @return the resource collection
*/
public Domains domains() {
return new Domains();
}
/**
* The "domains" collection of methods.
*/
public class Domains {
/**
* Adds an AD trust to a domain.
*
* Create a request for the method "domains.attachTrust".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link AttachTrust#execute()} method to invoke the remote
* operation.
*
* @param name Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.AttachTrustRequest}
* @return the request
*/
public AttachTrust attachTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.AttachTrustRequest content) throws java.io.IOException {
AttachTrust result = new AttachTrust(name, content);
initialize(result);
return result;
}
public class AttachTrust extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}:attachTrust";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Adds an AD trust to a domain.
*
* Create a request for the method "domains.attachTrust".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link AttachTrust#execute()} method to invoke the remote
* operation. {@link
* AttachTrust#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.AttachTrustRequest}
* @since 1.13
*/
protected AttachTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.AttachTrustRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public AttachTrust set$Xgafv(java.lang.String $Xgafv) {
return (AttachTrust) super.set$Xgafv($Xgafv);
}
@Override
public AttachTrust setAccessToken(java.lang.String accessToken) {
return (AttachTrust) super.setAccessToken(accessToken);
}
@Override
public AttachTrust setAlt(java.lang.String alt) {
return (AttachTrust) super.setAlt(alt);
}
@Override
public AttachTrust setCallback(java.lang.String callback) {
return (AttachTrust) super.setCallback(callback);
}
@Override
public AttachTrust setFields(java.lang.String fields) {
return (AttachTrust) super.setFields(fields);
}
@Override
public AttachTrust setKey(java.lang.String key) {
return (AttachTrust) super.setKey(key);
}
@Override
public AttachTrust setOauthToken(java.lang.String oauthToken) {
return (AttachTrust) super.setOauthToken(oauthToken);
}
@Override
public AttachTrust setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AttachTrust) super.setPrettyPrint(prettyPrint);
}
@Override
public AttachTrust setQuotaUser(java.lang.String quotaUser) {
return (AttachTrust) super.setQuotaUser(quotaUser);
}
@Override
public AttachTrust setUploadType(java.lang.String uploadType) {
return (AttachTrust) super.setUploadType(uploadType);
}
@Override
public AttachTrust setUploadProtocol(java.lang.String uploadProtocol) {
return (AttachTrust) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource domain name, project name and location using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public AttachTrust setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public AttachTrust set(String parameterName, Object value) {
return (AttachTrust) super.set(parameterName, value);
}
}
/**
* CheckMigrationPermission API gets the current state of DomainMigration
*
* Create a request for the method "domains.checkMigrationPermission".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link CheckMigrationPermission#execute()} method to invoke the
* remote operation.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.CheckMigrationPermissionRequest}
* @return the request
*/
public CheckMigrationPermission checkMigrationPermission(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.CheckMigrationPermissionRequest content) throws java.io.IOException {
CheckMigrationPermission result = new CheckMigrationPermission(domain, content);
initialize(result);
return result;
}
public class CheckMigrationPermission extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+domain}:checkMigrationPermission";
private final java.util.regex.Pattern DOMAIN_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* CheckMigrationPermission API gets the current state of DomainMigration
*
* Create a request for the method "domains.checkMigrationPermission".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link CheckMigrationPermission#execute()} method to invoke
* the remote operation. {@link CheckMigrationPermission#initialize(com.google.api.client.goog
* leapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.CheckMigrationPermissionRequest}
* @since 1.13
*/
protected CheckMigrationPermission(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.CheckMigrationPermissionRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.CheckMigrationPermissionResponse.class);
this.domain = com.google.api.client.util.Preconditions.checkNotNull(domain, "Required parameter domain must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public CheckMigrationPermission set$Xgafv(java.lang.String $Xgafv) {
return (CheckMigrationPermission) super.set$Xgafv($Xgafv);
}
@Override
public CheckMigrationPermission setAccessToken(java.lang.String accessToken) {
return (CheckMigrationPermission) super.setAccessToken(accessToken);
}
@Override
public CheckMigrationPermission setAlt(java.lang.String alt) {
return (CheckMigrationPermission) super.setAlt(alt);
}
@Override
public CheckMigrationPermission setCallback(java.lang.String callback) {
return (CheckMigrationPermission) super.setCallback(callback);
}
@Override
public CheckMigrationPermission setFields(java.lang.String fields) {
return (CheckMigrationPermission) super.setFields(fields);
}
@Override
public CheckMigrationPermission setKey(java.lang.String key) {
return (CheckMigrationPermission) super.setKey(key);
}
@Override
public CheckMigrationPermission setOauthToken(java.lang.String oauthToken) {
return (CheckMigrationPermission) super.setOauthToken(oauthToken);
}
@Override
public CheckMigrationPermission setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CheckMigrationPermission) super.setPrettyPrint(prettyPrint);
}
@Override
public CheckMigrationPermission setQuotaUser(java.lang.String quotaUser) {
return (CheckMigrationPermission) super.setQuotaUser(quotaUser);
}
@Override
public CheckMigrationPermission setUploadType(java.lang.String uploadType) {
return (CheckMigrationPermission) super.setUploadType(uploadType);
}
@Override
public CheckMigrationPermission setUploadProtocol(java.lang.String uploadProtocol) {
return (CheckMigrationPermission) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String domain;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getDomain() {
return domain;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public CheckMigrationPermission setDomain(java.lang.String domain) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.domain = domain;
return this;
}
@Override
public CheckMigrationPermission set(String parameterName, Object value) {
return (CheckMigrationPermission) super.set(parameterName, value);
}
}
/**
* Creates a Microsoft AD domain.
*
* Create a request for the method "domains.create".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource project name and location using the form:
* `projects/{project_id}/locations/global`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Domain}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.managedidentities.v1beta1.model.Domain content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+parent}/domains";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global$");
/**
* Creates a Microsoft AD domain.
*
* Create a request for the method "domains.create".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource project name and location using the form:
* `projects/{project_id}/locations/global`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Domain}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.managedidentities.v1beta1.model.Domain content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource project name and location using the form:
* `projects/{project_id}/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource project name and location using the form:
`projects/{project_id}/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource project name and location using the form:
* `projects/{project_id}/locations/global`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
this.parent = parent;
return this;
}
/**
* Required. A domain name, e.g. mydomain.myorg.com, with the following restrictions: *
* Must contain only lowercase letters, numbers, periods and hyphens. * Must start with
* a letter. * Must contain between 2-64 characters. * Must end with a number or a
* letter. * Must not start with period. * First segment length (mydomain form example
* above) shouldn't exceed 15 chars. * The last segment cannot be fully numeric. * Must
* be unique within the customer project.
*/
@com.google.api.client.util.Key
private java.lang.String domainName;
/** Required. A domain name, e.g. mydomain.myorg.com, with the following restrictions: * Must contain
only lowercase letters, numbers, periods and hyphens. * Must start with a letter. * Must contain
between 2-64 characters. * Must end with a number or a letter. * Must not start with period. *
First segment length (mydomain form example above) shouldn't exceed 15 chars. * The last segment
cannot be fully numeric. * Must be unique within the customer project.
*/
public java.lang.String getDomainName() {
return domainName;
}
/**
* Required. A domain name, e.g. mydomain.myorg.com, with the following restrictions: *
* Must contain only lowercase letters, numbers, periods and hyphens. * Must start with
* a letter. * Must contain between 2-64 characters. * Must end with a number or a
* letter. * Must not start with period. * First segment length (mydomain form example
* above) shouldn't exceed 15 chars. * The last segment cannot be fully numeric. * Must
* be unique within the customer project.
*/
public Create setDomainName(java.lang.String domainName) {
this.domainName = domainName;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a domain.
*
* Create a request for the method "domains.delete".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Deletes a domain.
*
* Create a request for the method "domains.delete".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "DELETE", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Removes an AD trust.
*
* Create a request for the method "domains.detachTrust".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link DetachTrust#execute()} method to invoke the remote
* operation.
*
* @param name Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.DetachTrustRequest}
* @return the request
*/
public DetachTrust detachTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.DetachTrustRequest content) throws java.io.IOException {
DetachTrust result = new DetachTrust(name, content);
initialize(result);
return result;
}
public class DetachTrust extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}:detachTrust";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Removes an AD trust.
*
* Create a request for the method "domains.detachTrust".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link DetachTrust#execute()} method to invoke the remote
* operation. {@link
* DetachTrust#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.DetachTrustRequest}
* @since 1.13
*/
protected DetachTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.DetachTrustRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public DetachTrust set$Xgafv(java.lang.String $Xgafv) {
return (DetachTrust) super.set$Xgafv($Xgafv);
}
@Override
public DetachTrust setAccessToken(java.lang.String accessToken) {
return (DetachTrust) super.setAccessToken(accessToken);
}
@Override
public DetachTrust setAlt(java.lang.String alt) {
return (DetachTrust) super.setAlt(alt);
}
@Override
public DetachTrust setCallback(java.lang.String callback) {
return (DetachTrust) super.setCallback(callback);
}
@Override
public DetachTrust setFields(java.lang.String fields) {
return (DetachTrust) super.setFields(fields);
}
@Override
public DetachTrust setKey(java.lang.String key) {
return (DetachTrust) super.setKey(key);
}
@Override
public DetachTrust setOauthToken(java.lang.String oauthToken) {
return (DetachTrust) super.setOauthToken(oauthToken);
}
@Override
public DetachTrust setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DetachTrust) super.setPrettyPrint(prettyPrint);
}
@Override
public DetachTrust setQuotaUser(java.lang.String quotaUser) {
return (DetachTrust) super.setQuotaUser(quotaUser);
}
@Override
public DetachTrust setUploadType(java.lang.String uploadType) {
return (DetachTrust) super.setUploadType(uploadType);
}
@Override
public DetachTrust setUploadProtocol(java.lang.String uploadProtocol) {
return (DetachTrust) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource domain name, project name, and location using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public DetachTrust setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public DetachTrust set(String parameterName, Object value) {
return (DetachTrust) super.set(parameterName, value);
}
}
/**
* Disable Domain Migration
*
* Create a request for the method "domains.disableMigration".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link DisableMigration#execute()} method to invoke the remote
* operation.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.DisableMigrationRequest}
* @return the request
*/
public DisableMigration disableMigration(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.DisableMigrationRequest content) throws java.io.IOException {
DisableMigration result = new DisableMigration(domain, content);
initialize(result);
return result;
}
public class DisableMigration extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+domain}:disableMigration";
private final java.util.regex.Pattern DOMAIN_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Disable Domain Migration
*
* Create a request for the method "domains.disableMigration".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link DisableMigration#execute()} method to invoke the
* remote operation. {@link DisableMigration#initialize(com.google.api.client.googleapis.servi
* ces.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.DisableMigrationRequest}
* @since 1.13
*/
protected DisableMigration(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.DisableMigrationRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.domain = com.google.api.client.util.Preconditions.checkNotNull(domain, "Required parameter domain must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public DisableMigration set$Xgafv(java.lang.String $Xgafv) {
return (DisableMigration) super.set$Xgafv($Xgafv);
}
@Override
public DisableMigration setAccessToken(java.lang.String accessToken) {
return (DisableMigration) super.setAccessToken(accessToken);
}
@Override
public DisableMigration setAlt(java.lang.String alt) {
return (DisableMigration) super.setAlt(alt);
}
@Override
public DisableMigration setCallback(java.lang.String callback) {
return (DisableMigration) super.setCallback(callback);
}
@Override
public DisableMigration setFields(java.lang.String fields) {
return (DisableMigration) super.setFields(fields);
}
@Override
public DisableMigration setKey(java.lang.String key) {
return (DisableMigration) super.setKey(key);
}
@Override
public DisableMigration setOauthToken(java.lang.String oauthToken) {
return (DisableMigration) super.setOauthToken(oauthToken);
}
@Override
public DisableMigration setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DisableMigration) super.setPrettyPrint(prettyPrint);
}
@Override
public DisableMigration setQuotaUser(java.lang.String quotaUser) {
return (DisableMigration) super.setQuotaUser(quotaUser);
}
@Override
public DisableMigration setUploadType(java.lang.String uploadType) {
return (DisableMigration) super.setUploadType(uploadType);
}
@Override
public DisableMigration setUploadProtocol(java.lang.String uploadProtocol) {
return (DisableMigration) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String domain;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getDomain() {
return domain;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public DisableMigration setDomain(java.lang.String domain) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.domain = domain;
return this;
}
@Override
public DisableMigration set(String parameterName, Object value) {
return (DisableMigration) super.set(parameterName, value);
}
}
/**
* DomainJoinMachine API joins a Compute Engine VM to the domain
*
* Create a request for the method "domains.domainJoinMachine".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link DomainJoinMachine#execute()} method to invoke the remote
* operation.
*
* @param domain Required. The domain resource name using the form:
* projects/{project_id}/locations/global/domains/{domain_name}
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.DomainJoinMachineRequest}
* @return the request
*/
public DomainJoinMachine domainJoinMachine(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.DomainJoinMachineRequest content) throws java.io.IOException {
DomainJoinMachine result = new DomainJoinMachine(domain, content);
initialize(result);
return result;
}
public class DomainJoinMachine extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+domain}:domainJoinMachine";
private final java.util.regex.Pattern DOMAIN_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* DomainJoinMachine API joins a Compute Engine VM to the domain
*
* Create a request for the method "domains.domainJoinMachine".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link DomainJoinMachine#execute()} method to invoke the
* remote operation. {@link DomainJoinMachine#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param domain Required. The domain resource name using the form:
* projects/{project_id}/locations/global/domains/{domain_name}
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.DomainJoinMachineRequest}
* @since 1.13
*/
protected DomainJoinMachine(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.DomainJoinMachineRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.DomainJoinMachineResponse.class);
this.domain = com.google.api.client.util.Preconditions.checkNotNull(domain, "Required parameter domain must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public DomainJoinMachine set$Xgafv(java.lang.String $Xgafv) {
return (DomainJoinMachine) super.set$Xgafv($Xgafv);
}
@Override
public DomainJoinMachine setAccessToken(java.lang.String accessToken) {
return (DomainJoinMachine) super.setAccessToken(accessToken);
}
@Override
public DomainJoinMachine setAlt(java.lang.String alt) {
return (DomainJoinMachine) super.setAlt(alt);
}
@Override
public DomainJoinMachine setCallback(java.lang.String callback) {
return (DomainJoinMachine) super.setCallback(callback);
}
@Override
public DomainJoinMachine setFields(java.lang.String fields) {
return (DomainJoinMachine) super.setFields(fields);
}
@Override
public DomainJoinMachine setKey(java.lang.String key) {
return (DomainJoinMachine) super.setKey(key);
}
@Override
public DomainJoinMachine setOauthToken(java.lang.String oauthToken) {
return (DomainJoinMachine) super.setOauthToken(oauthToken);
}
@Override
public DomainJoinMachine setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DomainJoinMachine) super.setPrettyPrint(prettyPrint);
}
@Override
public DomainJoinMachine setQuotaUser(java.lang.String quotaUser) {
return (DomainJoinMachine) super.setQuotaUser(quotaUser);
}
@Override
public DomainJoinMachine setUploadType(java.lang.String uploadType) {
return (DomainJoinMachine) super.setUploadType(uploadType);
}
@Override
public DomainJoinMachine setUploadProtocol(java.lang.String uploadProtocol) {
return (DomainJoinMachine) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* projects/{project_id}/locations/global/domains/{domain_name}
*/
@com.google.api.client.util.Key
private java.lang.String domain;
/** Required. The domain resource name using the form:
projects/{project_id}/locations/global/domains/{domain_name}
*/
public java.lang.String getDomain() {
return domain;
}
/**
* Required. The domain resource name using the form:
* projects/{project_id}/locations/global/domains/{domain_name}
*/
public DomainJoinMachine setDomain(java.lang.String domain) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.domain = domain;
return this;
}
@Override
public DomainJoinMachine set(String parameterName, Object value) {
return (DomainJoinMachine) super.set(parameterName, value);
}
}
/**
* Enable Domain Migration
*
* Create a request for the method "domains.enableMigration".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link EnableMigration#execute()} method to invoke the remote
* operation.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.EnableMigrationRequest}
* @return the request
*/
public EnableMigration enableMigration(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.EnableMigrationRequest content) throws java.io.IOException {
EnableMigration result = new EnableMigration(domain, content);
initialize(result);
return result;
}
public class EnableMigration extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+domain}:enableMigration";
private final java.util.regex.Pattern DOMAIN_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Enable Domain Migration
*
* Create a request for the method "domains.enableMigration".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link EnableMigration#execute()} method to invoke the remote
* operation. {@link EnableMigration#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.EnableMigrationRequest}
* @since 1.13
*/
protected EnableMigration(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.EnableMigrationRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.domain = com.google.api.client.util.Preconditions.checkNotNull(domain, "Required parameter domain must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public EnableMigration set$Xgafv(java.lang.String $Xgafv) {
return (EnableMigration) super.set$Xgafv($Xgafv);
}
@Override
public EnableMigration setAccessToken(java.lang.String accessToken) {
return (EnableMigration) super.setAccessToken(accessToken);
}
@Override
public EnableMigration setAlt(java.lang.String alt) {
return (EnableMigration) super.setAlt(alt);
}
@Override
public EnableMigration setCallback(java.lang.String callback) {
return (EnableMigration) super.setCallback(callback);
}
@Override
public EnableMigration setFields(java.lang.String fields) {
return (EnableMigration) super.setFields(fields);
}
@Override
public EnableMigration setKey(java.lang.String key) {
return (EnableMigration) super.setKey(key);
}
@Override
public EnableMigration setOauthToken(java.lang.String oauthToken) {
return (EnableMigration) super.setOauthToken(oauthToken);
}
@Override
public EnableMigration setPrettyPrint(java.lang.Boolean prettyPrint) {
return (EnableMigration) super.setPrettyPrint(prettyPrint);
}
@Override
public EnableMigration setQuotaUser(java.lang.String quotaUser) {
return (EnableMigration) super.setQuotaUser(quotaUser);
}
@Override
public EnableMigration setUploadType(java.lang.String uploadType) {
return (EnableMigration) super.setUploadType(uploadType);
}
@Override
public EnableMigration setUploadProtocol(java.lang.String uploadProtocol) {
return (EnableMigration) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String domain;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getDomain() {
return domain;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public EnableMigration setDomain(java.lang.String domain) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.domain = domain;
return this;
}
@Override
public EnableMigration set(String parameterName, Object value) {
return (EnableMigration) super.set(parameterName, value);
}
}
/**
* Extend Schema for Domain
*
* Create a request for the method "domains.extendSchema".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link ExtendSchema#execute()} method to invoke the remote
* operation.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ExtendSchemaRequest}
* @return the request
*/
public ExtendSchema extendSchema(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.ExtendSchemaRequest content) throws java.io.IOException {
ExtendSchema result = new ExtendSchema(domain, content);
initialize(result);
return result;
}
public class ExtendSchema extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+domain}:extendSchema";
private final java.util.regex.Pattern DOMAIN_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Extend Schema for Domain
*
* Create a request for the method "domains.extendSchema".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link ExtendSchema#execute()} method to invoke the remote
* operation. {@link
* ExtendSchema#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param domain Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ExtendSchemaRequest}
* @since 1.13
*/
protected ExtendSchema(java.lang.String domain, com.google.api.services.managedidentities.v1beta1.model.ExtendSchemaRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.domain = com.google.api.client.util.Preconditions.checkNotNull(domain, "Required parameter domain must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public ExtendSchema set$Xgafv(java.lang.String $Xgafv) {
return (ExtendSchema) super.set$Xgafv($Xgafv);
}
@Override
public ExtendSchema setAccessToken(java.lang.String accessToken) {
return (ExtendSchema) super.setAccessToken(accessToken);
}
@Override
public ExtendSchema setAlt(java.lang.String alt) {
return (ExtendSchema) super.setAlt(alt);
}
@Override
public ExtendSchema setCallback(java.lang.String callback) {
return (ExtendSchema) super.setCallback(callback);
}
@Override
public ExtendSchema setFields(java.lang.String fields) {
return (ExtendSchema) super.setFields(fields);
}
@Override
public ExtendSchema setKey(java.lang.String key) {
return (ExtendSchema) super.setKey(key);
}
@Override
public ExtendSchema setOauthToken(java.lang.String oauthToken) {
return (ExtendSchema) super.setOauthToken(oauthToken);
}
@Override
public ExtendSchema setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExtendSchema) super.setPrettyPrint(prettyPrint);
}
@Override
public ExtendSchema setQuotaUser(java.lang.String quotaUser) {
return (ExtendSchema) super.setQuotaUser(quotaUser);
}
@Override
public ExtendSchema setUploadType(java.lang.String uploadType) {
return (ExtendSchema) super.setUploadType(uploadType);
}
@Override
public ExtendSchema setUploadProtocol(java.lang.String uploadProtocol) {
return (ExtendSchema) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String domain;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getDomain() {
return domain;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public ExtendSchema setDomain(java.lang.String domain) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DOMAIN_PATTERN.matcher(domain).matches(),
"Parameter domain must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.domain = domain;
return this;
}
@Override
public ExtendSchema set(String parameterName, Object value) {
return (ExtendSchema) super.set(parameterName, value);
}
}
/**
* Gets information about a domain.
*
* Create a request for the method "domains.get".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Gets information about a domain.
*
* Create a request for the method "domains.get".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Domain.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "domains.getIamPolicy".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "domains.getIamPolicy".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Gets the domain ldaps settings.
*
* Create a request for the method "domains.getLdapssettings".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link GetLdapssettings#execute()} method to invoke the remote
* operation.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @return the request
*/
public GetLdapssettings getLdapssettings(java.lang.String name) throws java.io.IOException {
GetLdapssettings result = new GetLdapssettings(name);
initialize(result);
return result;
}
public class GetLdapssettings extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}/ldapssettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Gets the domain ldaps settings.
*
* Create a request for the method "domains.getLdapssettings".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link GetLdapssettings#execute()} method to invoke the
* remote operation. {@link GetLdapssettings#initialize(com.google.api.client.googleapis.servi
* ces.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @since 1.13
*/
protected GetLdapssettings(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.LDAPSSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetLdapssettings set$Xgafv(java.lang.String $Xgafv) {
return (GetLdapssettings) super.set$Xgafv($Xgafv);
}
@Override
public GetLdapssettings setAccessToken(java.lang.String accessToken) {
return (GetLdapssettings) super.setAccessToken(accessToken);
}
@Override
public GetLdapssettings setAlt(java.lang.String alt) {
return (GetLdapssettings) super.setAlt(alt);
}
@Override
public GetLdapssettings setCallback(java.lang.String callback) {
return (GetLdapssettings) super.setCallback(callback);
}
@Override
public GetLdapssettings setFields(java.lang.String fields) {
return (GetLdapssettings) super.setFields(fields);
}
@Override
public GetLdapssettings setKey(java.lang.String key) {
return (GetLdapssettings) super.setKey(key);
}
@Override
public GetLdapssettings setOauthToken(java.lang.String oauthToken) {
return (GetLdapssettings) super.setOauthToken(oauthToken);
}
@Override
public GetLdapssettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetLdapssettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetLdapssettings setQuotaUser(java.lang.String quotaUser) {
return (GetLdapssettings) super.setQuotaUser(quotaUser);
}
@Override
public GetLdapssettings setUploadType(java.lang.String uploadType) {
return (GetLdapssettings) super.setUploadType(uploadType);
}
@Override
public GetLdapssettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetLdapssettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public GetLdapssettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetLdapssettings set(String parameterName, Object value) {
return (GetLdapssettings) super.set(parameterName, value);
}
}
/**
* Lists domains in a project.
*
* Create a request for the method "domains.list".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+parent}/domains";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global$");
/**
* Lists domains in a project.
*
* Create a request for the method "domains.list".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.ListDomainsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the domain location using the form:
`projects/{project_id}/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter specifying constraints of a list operation. For example,
* `Domain.fqdn="mydomain.myorginization"`.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter specifying constraints of a list operation. For example,
`Domain.fqdn="mydomain.myorginization"`.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter specifying constraints of a list operation. For example,
* `Domain.fqdn="mydomain.myorginization"`.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Specifies the ordering of results. See [Sorting
* order](https://cloud.google.com/apis/design/design_patterns#sorting_order) for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Specifies the ordering of results. See [Sorting
order](https://cloud.google.com/apis/design/design_patterns#sorting_order) for more information.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. Specifies the ordering of results. See [Sorting
* order](https://cloud.google.com/apis/design/design_patterns#sorting_order) for more
* information.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value of
* 1000 will be used. Regardless of the page_size value, the response may include a
* partial list. Callers should rely on a response's next_page_token to determine if
* there are additional results to list.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of items to return. If not specified, a default value of 1000 will be
used. Regardless of the page_size value, the response may include a partial list. Callers should
rely on a response's next_page_token to determine if there are additional results to list.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value of
* 1000 will be used. Regardless of the page_size value, the response may include a
* partial list. Callers should rely on a response's next_page_token to determine if
* there are additional results to list.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The `next_page_token` value returned from a previous ListDomainsRequest request, if
* any.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The `next_page_token` value returned from a previous ListDomainsRequest request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The `next_page_token` value returned from a previous ListDomainsRequest request, if
* any.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the metadata and configuration of a domain.
*
* Create a request for the method "domains.patch".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The unique name of the domain using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Domain}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.Domain content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Updates the metadata and configuration of a domain.
*
* Create a request for the method "domains.patch".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The unique name of the domain using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Domain}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.Domain content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "PATCH", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The unique name of the domain using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The unique name of the domain using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The unique name of the domain using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this field.
* The elements of the repeated paths field may only include fields from Domain: *
* `labels` * `locations` * `authorized_networks` * `audit_logs_enabled`
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask of fields to update. At least one path must be supplied in this field. The elements
of the repeated paths field may only include fields from Domain: * `labels` * `locations` *
`authorized_networks` * `audit_logs_enabled`
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this field.
* The elements of the repeated paths field may only include fields from Domain: *
* `labels` * `locations` * `authorized_networks` * `audit_logs_enabled`
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the DNS conditional forwarder.
*
* Create a request for the method "domains.reconfigureTrust".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link ReconfigureTrust#execute()} method to invoke the remote
* operation.
*
* @param name Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ReconfigureTrustRequest}
* @return the request
*/
public ReconfigureTrust reconfigureTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.ReconfigureTrustRequest content) throws java.io.IOException {
ReconfigureTrust result = new ReconfigureTrust(name, content);
initialize(result);
return result;
}
public class ReconfigureTrust extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}:reconfigureTrust";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Updates the DNS conditional forwarder.
*
* Create a request for the method "domains.reconfigureTrust".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link ReconfigureTrust#execute()} method to invoke the
* remote operation. {@link ReconfigureTrust#initialize(com.google.api.client.googleapis.servi
* ces.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ReconfigureTrustRequest}
* @since 1.13
*/
protected ReconfigureTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.ReconfigureTrustRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public ReconfigureTrust set$Xgafv(java.lang.String $Xgafv) {
return (ReconfigureTrust) super.set$Xgafv($Xgafv);
}
@Override
public ReconfigureTrust setAccessToken(java.lang.String accessToken) {
return (ReconfigureTrust) super.setAccessToken(accessToken);
}
@Override
public ReconfigureTrust setAlt(java.lang.String alt) {
return (ReconfigureTrust) super.setAlt(alt);
}
@Override
public ReconfigureTrust setCallback(java.lang.String callback) {
return (ReconfigureTrust) super.setCallback(callback);
}
@Override
public ReconfigureTrust setFields(java.lang.String fields) {
return (ReconfigureTrust) super.setFields(fields);
}
@Override
public ReconfigureTrust setKey(java.lang.String key) {
return (ReconfigureTrust) super.setKey(key);
}
@Override
public ReconfigureTrust setOauthToken(java.lang.String oauthToken) {
return (ReconfigureTrust) super.setOauthToken(oauthToken);
}
@Override
public ReconfigureTrust setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReconfigureTrust) super.setPrettyPrint(prettyPrint);
}
@Override
public ReconfigureTrust setQuotaUser(java.lang.String quotaUser) {
return (ReconfigureTrust) super.setQuotaUser(quotaUser);
}
@Override
public ReconfigureTrust setUploadType(java.lang.String uploadType) {
return (ReconfigureTrust) super.setUploadType(uploadType);
}
@Override
public ReconfigureTrust setUploadProtocol(java.lang.String uploadProtocol) {
return (ReconfigureTrust) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource domain name, project name and location using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource domain name, project name and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public ReconfigureTrust setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ReconfigureTrust set(String parameterName, Object value) {
return (ReconfigureTrust) super.set(parameterName, value);
}
}
/**
* Resets a domain's administrator password.
*
* Create a request for the method "domains.resetAdminPassword".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link ResetAdminPassword#execute()} method to invoke the remote
* operation.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ResetAdminPasswordRequest}
* @return the request
*/
public ResetAdminPassword resetAdminPassword(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.ResetAdminPasswordRequest content) throws java.io.IOException {
ResetAdminPassword result = new ResetAdminPassword(name, content);
initialize(result);
return result;
}
public class ResetAdminPassword extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}:resetAdminPassword";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Resets a domain's administrator password.
*
* Create a request for the method "domains.resetAdminPassword".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link ResetAdminPassword#execute()} method to invoke the
* remote operation. {@link ResetAdminPassword#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ResetAdminPasswordRequest}
* @since 1.13
*/
protected ResetAdminPassword(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.ResetAdminPasswordRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.ResetAdminPasswordResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public ResetAdminPassword set$Xgafv(java.lang.String $Xgafv) {
return (ResetAdminPassword) super.set$Xgafv($Xgafv);
}
@Override
public ResetAdminPassword setAccessToken(java.lang.String accessToken) {
return (ResetAdminPassword) super.setAccessToken(accessToken);
}
@Override
public ResetAdminPassword setAlt(java.lang.String alt) {
return (ResetAdminPassword) super.setAlt(alt);
}
@Override
public ResetAdminPassword setCallback(java.lang.String callback) {
return (ResetAdminPassword) super.setCallback(callback);
}
@Override
public ResetAdminPassword setFields(java.lang.String fields) {
return (ResetAdminPassword) super.setFields(fields);
}
@Override
public ResetAdminPassword setKey(java.lang.String key) {
return (ResetAdminPassword) super.setKey(key);
}
@Override
public ResetAdminPassword setOauthToken(java.lang.String oauthToken) {
return (ResetAdminPassword) super.setOauthToken(oauthToken);
}
@Override
public ResetAdminPassword setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ResetAdminPassword) super.setPrettyPrint(prettyPrint);
}
@Override
public ResetAdminPassword setQuotaUser(java.lang.String quotaUser) {
return (ResetAdminPassword) super.setQuotaUser(quotaUser);
}
@Override
public ResetAdminPassword setUploadType(java.lang.String uploadType) {
return (ResetAdminPassword) super.setUploadType(uploadType);
}
@Override
public ResetAdminPassword setUploadProtocol(java.lang.String uploadProtocol) {
return (ResetAdminPassword) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public ResetAdminPassword setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ResetAdminPassword set(String parameterName, Object value) {
return (ResetAdminPassword) super.set(parameterName, value);
}
}
/**
* RestoreBackup restores domain mentioned in the RestoreBackupRequest
*
* Create a request for the method "domains.restore".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Restore#execute()} method to invoke the remote operation.
*
* @param name Required. resource name for the domain to which the backup belongs
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.RestoreDomainRequest}
* @return the request
*/
public Restore restore(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.RestoreDomainRequest content) throws java.io.IOException {
Restore result = new Restore(name, content);
initialize(result);
return result;
}
public class Restore extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}:restore";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* RestoreBackup restores domain mentioned in the RestoreBackupRequest
*
* Create a request for the method "domains.restore".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Restore#execute()} method to invoke the remote
* operation. {@link
* Restore#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. resource name for the domain to which the backup belongs
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.RestoreDomainRequest}
* @since 1.13
*/
protected Restore(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.RestoreDomainRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public Restore set$Xgafv(java.lang.String $Xgafv) {
return (Restore) super.set$Xgafv($Xgafv);
}
@Override
public Restore setAccessToken(java.lang.String accessToken) {
return (Restore) super.setAccessToken(accessToken);
}
@Override
public Restore setAlt(java.lang.String alt) {
return (Restore) super.setAlt(alt);
}
@Override
public Restore setCallback(java.lang.String callback) {
return (Restore) super.setCallback(callback);
}
@Override
public Restore setFields(java.lang.String fields) {
return (Restore) super.setFields(fields);
}
@Override
public Restore setKey(java.lang.String key) {
return (Restore) super.setKey(key);
}
@Override
public Restore setOauthToken(java.lang.String oauthToken) {
return (Restore) super.setOauthToken(oauthToken);
}
@Override
public Restore setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Restore) super.setPrettyPrint(prettyPrint);
}
@Override
public Restore setQuotaUser(java.lang.String quotaUser) {
return (Restore) super.setQuotaUser(quotaUser);
}
@Override
public Restore setUploadType(java.lang.String uploadType) {
return (Restore) super.setUploadType(uploadType);
}
@Override
public Restore setUploadProtocol(java.lang.String uploadProtocol) {
return (Restore) super.setUploadProtocol(uploadProtocol);
}
/** Required. resource name for the domain to which the backup belongs */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. resource name for the domain to which the backup belongs
*/
public java.lang.String getName() {
return name;
}
/** Required. resource name for the domain to which the backup belongs */
public Restore setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Restore set(String parameterName, Object value) {
return (Restore) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "domains.setIamPolicy".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "domains.setIamPolicy".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "domains.testIamPermissions".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "domains.testIamPermissions".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Patches a single ldaps settings.
*
* Create a request for the method "domains.updateLdapssettings".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link UpdateLdapssettings#execute()} method to invoke the remote
* operation.
*
* @param name The resource name of the LDAPS settings. Uses the form:
* `projects/{project}/locations/{location}/domains/{domain}`.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.LDAPSSettings}
* @return the request
*/
public UpdateLdapssettings updateLdapssettings(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.LDAPSSettings content) throws java.io.IOException {
UpdateLdapssettings result = new UpdateLdapssettings(name, content);
initialize(result);
return result;
}
public class UpdateLdapssettings extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}/ldapssettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Patches a single ldaps settings.
*
* Create a request for the method "domains.updateLdapssettings".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link UpdateLdapssettings#execute()} method to invoke the
* remote operation. {@link UpdateLdapssettings#initialize(com.google.api.client.googleapis.se
* rvices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name The resource name of the LDAPS settings. Uses the form:
* `projects/{project}/locations/{location}/domains/{domain}`.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.LDAPSSettings}
* @since 1.13
*/
protected UpdateLdapssettings(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.LDAPSSettings content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "PATCH", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public UpdateLdapssettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateLdapssettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateLdapssettings setAccessToken(java.lang.String accessToken) {
return (UpdateLdapssettings) super.setAccessToken(accessToken);
}
@Override
public UpdateLdapssettings setAlt(java.lang.String alt) {
return (UpdateLdapssettings) super.setAlt(alt);
}
@Override
public UpdateLdapssettings setCallback(java.lang.String callback) {
return (UpdateLdapssettings) super.setCallback(callback);
}
@Override
public UpdateLdapssettings setFields(java.lang.String fields) {
return (UpdateLdapssettings) super.setFields(fields);
}
@Override
public UpdateLdapssettings setKey(java.lang.String key) {
return (UpdateLdapssettings) super.setKey(key);
}
@Override
public UpdateLdapssettings setOauthToken(java.lang.String oauthToken) {
return (UpdateLdapssettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateLdapssettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateLdapssettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateLdapssettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateLdapssettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateLdapssettings setUploadType(java.lang.String uploadType) {
return (UpdateLdapssettings) super.setUploadType(uploadType);
}
@Override
public UpdateLdapssettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateLdapssettings) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the LDAPS settings. Uses the form:
* `projects/{project}/locations/{location}/domains/{domain}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the LDAPS settings. Uses the form:
`projects/{project}/locations/{location}/domains/{domain}`.
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the LDAPS settings. Uses the form:
* `projects/{project}/locations/{location}/domains/{domain}`.
*/
public UpdateLdapssettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this field.
* For the `FieldMask` definition, see https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask of fields to update. At least one path must be supplied in this field. For the
`FieldMask` definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this field.
* For the `FieldMask` definition, see https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask
*/
public UpdateLdapssettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateLdapssettings set(String parameterName, Object value) {
return (UpdateLdapssettings) super.set(parameterName, value);
}
}
/**
* Validates a trust state, that the target domain is reachable, and that the target domain is able
* to accept incoming trust requests.
*
* Create a request for the method "domains.validateTrust".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link ValidateTrust#execute()} method to invoke the remote
* operation.
*
* @param name Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ValidateTrustRequest}
* @return the request
*/
public ValidateTrust validateTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.ValidateTrustRequest content) throws java.io.IOException {
ValidateTrust result = new ValidateTrust(name, content);
initialize(result);
return result;
}
public class ValidateTrust extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}:validateTrust";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Validates a trust state, that the target domain is reachable, and that the target domain is
* able to accept incoming trust requests.
*
* Create a request for the method "domains.validateTrust".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link ValidateTrust#execute()} method to invoke the remote
* operation. {@link ValidateTrust#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param name Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.ValidateTrustRequest}
* @since 1.13
*/
protected ValidateTrust(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.ValidateTrustRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public ValidateTrust set$Xgafv(java.lang.String $Xgafv) {
return (ValidateTrust) super.set$Xgafv($Xgafv);
}
@Override
public ValidateTrust setAccessToken(java.lang.String accessToken) {
return (ValidateTrust) super.setAccessToken(accessToken);
}
@Override
public ValidateTrust setAlt(java.lang.String alt) {
return (ValidateTrust) super.setAlt(alt);
}
@Override
public ValidateTrust setCallback(java.lang.String callback) {
return (ValidateTrust) super.setCallback(callback);
}
@Override
public ValidateTrust setFields(java.lang.String fields) {
return (ValidateTrust) super.setFields(fields);
}
@Override
public ValidateTrust setKey(java.lang.String key) {
return (ValidateTrust) super.setKey(key);
}
@Override
public ValidateTrust setOauthToken(java.lang.String oauthToken) {
return (ValidateTrust) super.setOauthToken(oauthToken);
}
@Override
public ValidateTrust setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ValidateTrust) super.setPrettyPrint(prettyPrint);
}
@Override
public ValidateTrust setQuotaUser(java.lang.String quotaUser) {
return (ValidateTrust) super.setQuotaUser(quotaUser);
}
@Override
public ValidateTrust setUploadType(java.lang.String uploadType) {
return (ValidateTrust) super.setUploadType(uploadType);
}
@Override
public ValidateTrust setUploadProtocol(java.lang.String uploadProtocol) {
return (ValidateTrust) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource domain name, project name, and location using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource domain name, project name, and location using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public ValidateTrust setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ValidateTrust set(String parameterName, Object value) {
return (ValidateTrust) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Backups collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.Backups.List request = managedidentities.backups().list(parameters ...)}
*
*
* @return the resource collection
*/
public Backups backups() {
return new Backups();
}
/**
* The "backups" collection of methods.
*/
public class Backups {
/**
* Creates a Backup for a domain.
*
* Create a request for the method "backups.create".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Backup}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.managedidentities.v1beta1.model.Backup content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+parent}/backups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Creates a Backup for a domain.
*
* Create a request for the method "backups.create".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Backup}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.managedidentities.v1beta1.model.Backup content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. Backup Id, unique name to identify the backups with the following
* restrictions: * Must be lowercase letters, numbers, and hyphens * Must start with a
* letter. * Must contain between 1-63 characters. * Must end with a number or a
* letter. * Must be unique within the domain.
*/
@com.google.api.client.util.Key
private java.lang.String backupId;
/** Required. Backup Id, unique name to identify the backups with the following restrictions: * Must be
lowercase letters, numbers, and hyphens * Must start with a letter. * Must contain between 1-63
characters. * Must end with a number or a letter. * Must be unique within the domain.
*/
public java.lang.String getBackupId() {
return backupId;
}
/**
* Required. Backup Id, unique name to identify the backups with the following
* restrictions: * Must be lowercase letters, numbers, and hyphens * Must start with a
* letter. * Must contain between 1-63 characters. * Must end with a number or a
* letter. * Must be unique within the domain.
*/
public Create setBackupId(java.lang.String backupId) {
this.backupId = backupId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes identified Backup.
*
* Create a request for the method "backups.delete".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
/**
* Deletes identified Backup.
*
* Create a request for the method "backups.delete".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "DELETE", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The backup resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single Backup.
*
* Create a request for the method "backups.get".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
/**
* Gets details of a single Backup.
*
* Create a request for the method "backups.get".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Backup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The backup resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The backup resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}/backups/{backup_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "backups.getIamPolicy".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "backups.getIamPolicy".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate
* value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate
* value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses
* version 1. To learn which resources support conditions in their IAM policies, see
* the [IAM documentation](https://cloud.google.com/iam/help/conditions/resource-
* policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses
* version 1. To learn which resources support conditions in their IAM policies, see
* the [IAM documentation](https://cloud.google.com/iam/help/conditions/resource-
* policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists Backup in a given project.
*
* Create a request for the method "backups.list".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+parent}/backups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Lists Backup in a given project.
*
* Create a request for the method "backups.list".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.ListBackupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The domain resource name using the form:
`projects/{project_id}/locations/global/domains/{domain_name}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The domain resource name using the form:
* `projects/{project_id}/locations/global/domains/{domain_name}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filter specifying constraints of a list operation. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filter specifying constraints of a list operation.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filter specifying constraints of a list operation. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Specifies the ordering of results following syntax at
* https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Specifies the ordering of results following syntax at
https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. Specifies the ordering of results following syntax at
* https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value
* of 1000 will be used by the service. Regardless of the page_size value, the
* response may include a partial list and a caller should only rely on response's
* next_page_token to determine if there are more instances left to be queried.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of items to return. If not specified, a default value of 1000 will be
used by the service. Regardless of the page_size value, the response may include a partial list and
a caller should only rely on response's next_page_token to determine if there are more instances
left to be queried.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value
* of 1000 will be used by the service. Regardless of the page_size value, the
* response may include a partial list and a caller should only rely on response's
* next_page_token to determine if there are more instances left to be queried.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. The next_page_token value returned from a previous List request, if any.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The next_page_token value returned from a previous List request, if any.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the labels for specified Backup.
*
* Create a request for the method "backups.patch".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The unique name of the Backup in the form of
* projects/{project_id}/locations/global/domains/{domain_name}/backups/{name}
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Backup}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.Backup content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
/**
* Updates the labels for specified Backup.
*
* Create a request for the method "backups.patch".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The unique name of the Backup in the form of
* projects/{project_id}/locations/global/domains/{domain_name}/backups/{name}
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Backup}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.Backup content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "PATCH", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The unique name of the Backup in the form of
* projects/{project_id}/locations/global/domains/{domain_name}/backups/{name}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The unique name of the Backup in the form of
projects/{project_id}/locations/global/domains/{domain_name}/backups/{name}
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The unique name of the Backup in the form of
* projects/{project_id}/locations/global/domains/{domain_name}/backups/{name}
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this
* field. The elements of the repeated paths field may only include these fields from
* Backup: * `labels`
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask of fields to update. At least one path must be supplied in this field. The elements
of the repeated paths field may only include these fields from Backup: * `labels`
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this
* field. The elements of the repeated paths field may only include these fields from
* Backup: * `labels`
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "backups.setIamPolicy".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "backups.setIamPolicy".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate
* value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate
* value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "backups.testIamPermissions".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "backups.testIamPermissions".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See
* [Resource names](https://cloud.google.com/apis/design/resource_names) for the
* appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See
* [Resource names](https://cloud.google.com/apis/design/resource_names) for the
* appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/backups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SqlIntegrations collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.SqlIntegrations.List request = managedidentities.sqlIntegrations().list(parameters ...)}
*
*
* @return the resource collection
*/
public SqlIntegrations sqlIntegrations() {
return new SqlIntegrations();
}
/**
* The "sqlIntegrations" collection of methods.
*/
public class SqlIntegrations {
/**
* Gets details of a single sqlIntegration.
*
* Create a request for the method "sqlIntegrations.get".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. SqlIntegration resource name using the form:
* `projects/{project_id}/locations/global/domains/sqlIntegrations/{name}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+/sqlIntegrations/[^/]+$");
/**
* Gets details of a single sqlIntegration.
*
* Create a request for the method "sqlIntegrations.get".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. SqlIntegration resource name using the form:
* `projects/{project_id}/locations/global/domains/sqlIntegrations/{name}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.SqlIntegration.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/sqlIntegrations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. SqlIntegration resource name using the form:
* `projects/{project_id}/locations/global/domains/sqlIntegrations/{name}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. SqlIntegration resource name using the form:
`projects/{project_id}/locations/global/domains/sqlIntegrations/{name}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. SqlIntegration resource name using the form:
* `projects/{project_id}/locations/global/domains/sqlIntegrations/{name}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+/sqlIntegrations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists SqlIntegrations in a given domain.
*
* Create a request for the method "sqlIntegrations.list".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the SqlIntegrations using the form:
* `projects/{project_id}/locations/global/domains`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+parent}/sqlIntegrations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/domains/[^/]+$");
/**
* Lists SqlIntegrations in a given domain.
*
* Create a request for the method "sqlIntegrations.list".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the SqlIntegrations using the form:
* `projects/{project_id}/locations/global/domains`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.ListSqlIntegrationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the SqlIntegrations using the form:
* `projects/{project_id}/locations/global/domains`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the SqlIntegrations using the form:
`projects/{project_id}/locations/global/domains`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the SqlIntegrations using the form:
* `projects/{project_id}/locations/global/domains`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global/domains/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Filter specifying constraints of a list operation. For example,
* `SqlIntegration.name="sql"`.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filter specifying constraints of a list operation. For example,
`SqlIntegration.name="sql"`.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Filter specifying constraints of a list operation. For example,
* `SqlIntegration.name="sql"`.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Specifies the ordering of results following syntax at
* https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Specifies the ordering of results following syntax at
https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. Specifies the ordering of results following syntax at
* https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value
* of 1000 will be used by the service. Regardless of the page_size value, the
* response may include a partial list and a caller should only rely on
* response'ANIZATIONs next_page_token to determine if there are more instances left
* to be queried.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of items to return. If not specified, a default value of 1000 will be
used by the service. Regardless of the page_size value, the response may include a partial list and
a caller should only rely on response'ANIZATIONs next_page_token to determine if there are more
instances left to be queried.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value
* of 1000 will be used by the service. Regardless of the page_size value, the
* response may include a partial list and a caller should only rely on
* response'ANIZATIONs next_page_token to determine if there are more instances left
* to be queried.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. The next_page_token value returned from a previous List request, if any.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The next_page_token value returned from a previous List request, if any.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.Operations.List request = managedidentities.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Cancel#execute()} method to invoke the remote
* operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.CancelOperationRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "DELETE", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Peerings collection.
*
* The typical use is:
*
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI managedidentities = new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(...);}
* {@code ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.Peerings.List request = managedidentities.peerings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Peerings peerings() {
return new Peerings();
}
/**
* The "peerings" collection of methods.
*/
public class Peerings {
/**
* Creates a Peering for Managed AD instance.
*
* Create a request for the method "peerings.create".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource project name and location using the form:
* `projects/{project_id}/locations/global`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Peering}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.managedidentities.v1beta1.model.Peering content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+parent}/peerings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global$");
/**
* Creates a Peering for Managed AD instance.
*
* Create a request for the method "peerings.create".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource project name and location using the form:
* `projects/{project_id}/locations/global`
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Peering}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.managedidentities.v1beta1.model.Peering content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource project name and location using the form:
* `projects/{project_id}/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource project name and location using the form:
`projects/{project_id}/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource project name and location using the form:
* `projects/{project_id}/locations/global`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
this.parent = parent;
return this;
}
/** Required. Peering Id, unique name to identify peering. */
@com.google.api.client.util.Key
private java.lang.String peeringId;
/** Required. Peering Id, unique name to identify peering.
*/
public java.lang.String getPeeringId() {
return peeringId;
}
/** Required. Peering Id, unique name to identify peering. */
public Create setPeeringId(java.lang.String peeringId) {
this.peeringId = peeringId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes identified Peering.
*
* Create a request for the method "peerings.delete".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/peerings/[^/]+$");
/**
* Deletes identified Peering.
*
* Create a request for the method "peerings.delete".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "DELETE", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Peering resource name using the form:
`projects/{project_id}/locations/global/peerings/{peering_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single Peering.
*
* Create a request for the method "peerings.get".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/peerings/[^/]+$");
/**
* Gets details of a single Peering.
*
* Create a request for the method "peerings.get".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Peering.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Peering resource name using the form:
`projects/{project_id}/locations/global/peerings/{peering_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Peering resource name using the form:
* `projects/{project_id}/locations/global/peerings/{peering_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "peerings.getIamPolicy".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/peerings/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "peerings.getIamPolicy".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists Peerings in a given project.
*
* Create a request for the method "peerings.list".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+parent}/peerings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global$");
/**
* Lists Peerings in a given project.
*
* Create a request for the method "peerings.list".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "GET", REST_PATH, null, com.google.api.services.managedidentities.v1beta1.model.ListPeeringsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the domain location using the form:
`projects/{project_id}/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the domain location using the form:
* `projects/{project_id}/locations/global`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/global$");
}
this.parent = parent;
return this;
}
/**
* Optional. Filter specifying constraints of a list operation. For example,
* `peering.authoized_network ="/projects/myprojectid"`.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filter specifying constraints of a list operation. For example,
`peering.authoized_network ="/projects/myprojectid"`.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Filter specifying constraints of a list operation. For example,
* `peering.authoized_network ="/projects/myprojectid"`.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Specifies the ordering of results following syntax at
* https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Specifies the ordering of results following syntax at
https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Optional. Specifies the ordering of results following syntax at
* https://cloud.google.com/apis/design/design_patterns#sorting_order.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value of
* 1000 will be used by the service. Regardless of the page_size value, the response may
* include a partial list and a caller should only rely on response's next_page_token to
* determine if there are more instances left to be queried.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of items to return. If not specified, a default value of 1000 will be
used by the service. Regardless of the page_size value, the response may include a partial list and
a caller should only rely on response's next_page_token to determine if there are more instances
left to be queried.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of items to return. If not specified, a default value of
* 1000 will be used by the service. Regardless of the page_size value, the response may
* include a partial list and a caller should only rely on response's next_page_token to
* determine if there are more instances left to be queried.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. The next_page_token value returned from a previous List request, if any.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The next_page_token value returned from a previous List request, if any.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The next_page_token value returned from a previous List request, if any.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the labels for specified Peering.
*
* Create a request for the method "peerings.patch".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Unique name of the peering in this scope including projects and location using the
* form: `projects/{project_id}/locations/global/peerings/{peering_id}`.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Peering}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.Peering content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/peerings/[^/]+$");
/**
* Updates the labels for specified Peering.
*
* Create a request for the method "peerings.patch".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Unique name of the peering in this scope including projects and location using the
* form: `projects/{project_id}/locations/global/peerings/{peering_id}`.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.Peering}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.managedidentities.v1beta1.model.Peering content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "PATCH", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Unique name of the peering in this scope including projects and location
* using the form: `projects/{project_id}/locations/global/peerings/{peering_id}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Unique name of the peering in this scope including projects and location using the
form: `projects/{project_id}/locations/global/peerings/{peering_id}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Unique name of the peering in this scope including projects and location
* using the form: `projects/{project_id}/locations/global/peerings/{peering_id}`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this field.
* The elements of the repeated paths field may only include these fields from Peering:
* * `labels`
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask of fields to update. At least one path must be supplied in this field. The elements
of the repeated paths field may only include these fields from Peering: * `labels`
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Mask of fields to update. At least one path must be supplied in this field.
* The elements of the repeated paths field may only include these fields from Peering:
* * `labels`
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "peerings.setIamPolicy".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/peerings/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "peerings.setIamPolicy".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.SetIamPolicyRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "peerings.testIamPermissions".
*
* This request holds the parameters needed by the managedidentities server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequest {
private static final String REST_PATH = "v1beta1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/global/peerings/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "peerings.testIamPermissions".
*
* This request holds the parameters needed by the the managedidentities server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsRequest content) {
super(ManagedServiceforMicrosoftActiveDirectoryConsumerAPI.this, "POST", REST_PATH, content, com.google.api.services.managedidentities.v1beta1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/global/peerings/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
}
}
}
/**
* Builder for {@link ManagedServiceforMicrosoftActiveDirectoryConsumerAPI}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link ManagedServiceforMicrosoftActiveDirectoryConsumerAPI}. */
@Override
public ManagedServiceforMicrosoftActiveDirectoryConsumerAPI build() {
return new ManagedServiceforMicrosoftActiveDirectoryConsumerAPI(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequestInitializer}.
*
* @since 1.12
*/
public Builder setManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequestInitializer(
ManagedServiceforMicrosoftActiveDirectoryConsumerAPIRequestInitializer managedserviceformicrosoftactivedirectoryconsumerapiRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(managedserviceformicrosoftactivedirectoryconsumerapiRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}