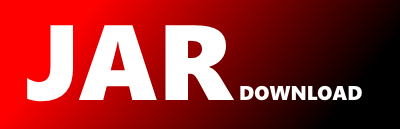
com.google.api.services.managedidentities.v1beta1.model.DomainJoinMachineRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.managedidentities.v1beta1.model;
/**
* DomainJoinMachineRequest is the request message for DomainJoinMachine method
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Managed Service for Microsoft Active Directory API.
* For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DomainJoinMachineRequest extends com.google.api.client.json.GenericJson {
/**
* Optional. force if True, forces domain join even if the computer account already exists.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/**
* Optional. OU name to which the VM needs to be domain joined. If the field is not provided, the
* VM is joined to the default OU which is created. The default OU for the domain join api is
* created as GCE Instances under the Cloud OU. Example - OU=GCE
* Instances,OU=Cloud,DC=ad,DC=test,DC=com If the field is provided, then the custom OU is
* searched for under GCE Instances OU. Example - if ou_name=test_ou then the VM is domain joined
* to the following OU: OU=test_ou,OU=GCE Instances,OU=Cloud,DC=ad,DC=test,DC=com if present. If
* OU is not present under GCE Instances, then error is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ouName;
/**
* Required. Full instance id token of compute engine VM to verify instance identity. More about
* this: https://cloud.google.com/compute/docs/instances/verifying-instance-
* identity#request_signature
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String vmIdToken;
/**
* Optional. force if True, forces domain join even if the computer account already exists.
* @return value or {@code null} for none
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Optional. force if True, forces domain join even if the computer account already exists.
* @param force force or {@code null} for none
*/
public DomainJoinMachineRequest setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* Optional. OU name to which the VM needs to be domain joined. If the field is not provided, the
* VM is joined to the default OU which is created. The default OU for the domain join api is
* created as GCE Instances under the Cloud OU. Example - OU=GCE
* Instances,OU=Cloud,DC=ad,DC=test,DC=com If the field is provided, then the custom OU is
* searched for under GCE Instances OU. Example - if ou_name=test_ou then the VM is domain joined
* to the following OU: OU=test_ou,OU=GCE Instances,OU=Cloud,DC=ad,DC=test,DC=com if present. If
* OU is not present under GCE Instances, then error is returned.
* @return value or {@code null} for none
*/
public java.lang.String getOuName() {
return ouName;
}
/**
* Optional. OU name to which the VM needs to be domain joined. If the field is not provided, the
* VM is joined to the default OU which is created. The default OU for the domain join api is
* created as GCE Instances under the Cloud OU. Example - OU=GCE
* Instances,OU=Cloud,DC=ad,DC=test,DC=com If the field is provided, then the custom OU is
* searched for under GCE Instances OU. Example - if ou_name=test_ou then the VM is domain joined
* to the following OU: OU=test_ou,OU=GCE Instances,OU=Cloud,DC=ad,DC=test,DC=com if present. If
* OU is not present under GCE Instances, then error is returned.
* @param ouName ouName or {@code null} for none
*/
public DomainJoinMachineRequest setOuName(java.lang.String ouName) {
this.ouName = ouName;
return this;
}
/**
* Required. Full instance id token of compute engine VM to verify instance identity. More about
* this: https://cloud.google.com/compute/docs/instances/verifying-instance-
* identity#request_signature
* @return value or {@code null} for none
*/
public java.lang.String getVmIdToken() {
return vmIdToken;
}
/**
* Required. Full instance id token of compute engine VM to verify instance identity. More about
* this: https://cloud.google.com/compute/docs/instances/verifying-instance-
* identity#request_signature
* @param vmIdToken vmIdToken or {@code null} for none
*/
public DomainJoinMachineRequest setVmIdToken(java.lang.String vmIdToken) {
this.vmIdToken = vmIdToken;
return this;
}
@Override
public DomainJoinMachineRequest set(String fieldName, Object value) {
return (DomainJoinMachineRequest) super.set(fieldName, value);
}
@Override
public DomainJoinMachineRequest clone() {
return (DomainJoinMachineRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy