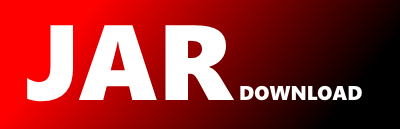
com.google.api.services.mapsengine.model.DisplayRule Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-11-16 19:10:01 UTC)
* on 2015-12-05 at 02:54:33 UTC
* Modify at your own risk.
*/
package com.google.api.services.mapsengine.model;
/**
* A display rule of the vector style.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Maps Engine API. For a detailed explanation
* see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DisplayRule extends com.google.api.client.json.GenericJson {
/**
* This display rule will only be applied to features that match all of the filters here. If
* filters is empty, then the rule applies to all features.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List filters;
/**
* Style applied to lines. Required for LineString Geometry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LineStyle lineOptions;
/**
* Display rule name. Name is not unique and cannot be used for identification purpose.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Style applied to points. Required for Point Geometry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PointStyle pointOptions;
/**
* Style applied to polygons. Required for Polygon Geometry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PolygonStyle polygonOptions;
/**
* The zoom levels that this display rule apply.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ZoomLevels zoomLevels;
/**
* This display rule will only be applied to features that match all of the filters here. If
* filters is empty, then the rule applies to all features.
* @return value or {@code null} for none
*/
public java.util.List getFilters() {
return filters;
}
/**
* This display rule will only be applied to features that match all of the filters here. If
* filters is empty, then the rule applies to all features.
* @param filters filters or {@code null} for none
*/
public DisplayRule setFilters(java.util.List filters) {
this.filters = filters;
return this;
}
/**
* Style applied to lines. Required for LineString Geometry.
* @return value or {@code null} for none
*/
public LineStyle getLineOptions() {
return lineOptions;
}
/**
* Style applied to lines. Required for LineString Geometry.
* @param lineOptions lineOptions or {@code null} for none
*/
public DisplayRule setLineOptions(LineStyle lineOptions) {
this.lineOptions = lineOptions;
return this;
}
/**
* Display rule name. Name is not unique and cannot be used for identification purpose.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Display rule name. Name is not unique and cannot be used for identification purpose.
* @param name name or {@code null} for none
*/
public DisplayRule setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Style applied to points. Required for Point Geometry.
* @return value or {@code null} for none
*/
public PointStyle getPointOptions() {
return pointOptions;
}
/**
* Style applied to points. Required for Point Geometry.
* @param pointOptions pointOptions or {@code null} for none
*/
public DisplayRule setPointOptions(PointStyle pointOptions) {
this.pointOptions = pointOptions;
return this;
}
/**
* Style applied to polygons. Required for Polygon Geometry.
* @return value or {@code null} for none
*/
public PolygonStyle getPolygonOptions() {
return polygonOptions;
}
/**
* Style applied to polygons. Required for Polygon Geometry.
* @param polygonOptions polygonOptions or {@code null} for none
*/
public DisplayRule setPolygonOptions(PolygonStyle polygonOptions) {
this.polygonOptions = polygonOptions;
return this;
}
/**
* The zoom levels that this display rule apply.
* @return value or {@code null} for none
*/
public ZoomLevels getZoomLevels() {
return zoomLevels;
}
/**
* The zoom levels that this display rule apply.
* @param zoomLevels zoomLevels or {@code null} for none
*/
public DisplayRule setZoomLevels(ZoomLevels zoomLevels) {
this.zoomLevels = zoomLevels;
return this;
}
@Override
public DisplayRule set(String fieldName, Object value) {
return (DisplayRule) super.set(fieldName, value);
}
@Override
public DisplayRule clone() {
return (DisplayRule) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy