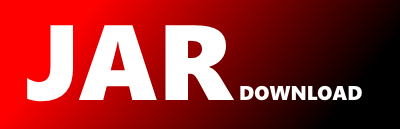
com.google.api.services.mapsengine.MapsEngine Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2015-11-16 19:10:01 UTC)
* on 2015-12-05 at 02:54:01 UTC
* Modify at your own risk.
*/
package com.google.api.services.mapsengine;
/**
* Service definition for MapsEngine (v1).
*
*
* The Google Maps Engine API allows developers to store and query geospatial vector and raster data.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link MapsEngineRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class MapsEngine extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.21.0 of the Google Maps Engine API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "mapsengine/v1/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public MapsEngine(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
MapsEngine(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Assets collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Assets.List request = mapsengine.assets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Assets assets() {
return new Assets();
}
/**
* The "assets" collection of methods.
*/
public class Assets {
/**
* Return metadata for a particular asset.
*
* Create a request for the method "assets.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset.
* @return the request
*/
public Get get(java.lang.String id) throws java.io.IOException {
Get result = new Get(id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "assets/{id}";
/**
* Return metadata for a particular asset.
*
* Create a request for the method "assets.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset.
* @since 1.13
*/
protected Get(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.Asset.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the asset. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return all assets readable by the current user.
*
* Create a request for the method "assets.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "assets";
/**
* Return all assets readable by the current user.
*
* Create a request for the method "assets.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.AssetsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or after this time.
*/
public com.google.api.client.util.DateTime getModifiedAfter() {
return modifiedAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
public List setModifiedAfter(com.google.api.client.util.DateTime modifiedAfter) {
this.modifiedAfter = modifiedAfter;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or after this time.
*/
public com.google.api.client.util.DateTime getCreatedAfter() {
return createdAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
public List setCreatedAfter(com.google.api.client.util.DateTime createdAfter) {
this.createdAfter = createdAfter;
return this;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
@com.google.api.client.util.Key
private java.lang.String tags;
/** A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public java.lang.String getTags() {
return tags;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public List setTags(java.lang.String tags) {
this.tags = tags;
return this;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
@com.google.api.client.util.Key
private java.lang.String search;
/** An unstructured search string used to filter the set of results based on asset metadata.
*/
public java.lang.String getSearch() {
return search;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
public List setSearch(java.lang.String search) {
this.search = search;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/** An email address representing a user. Returned assets that have been created by the user associated
with the provided email address.
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
public List setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String bbox;
/** A bounding box, expressed as "west,south,east,north". If set, only assets which intersect this
bounding box will be returned.
*/
public java.lang.String getBbox() {
return bbox;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
public List setBbox(java.lang.String bbox) {
this.bbox = bbox;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or before this time.
*/
public com.google.api.client.util.DateTime getModifiedBefore() {
return modifiedBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
public List setModifiedBefore(com.google.api.client.util.DateTime modifiedBefore) {
this.modifiedBefore = modifiedBefore;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or before this time.
*/
public com.google.api.client.util.DateTime getCreatedBefore() {
return createdBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
public List setCreatedBefore(com.google.api.client.util.DateTime createdBefore) {
this.createdBefore = createdBefore;
return this;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/** The role parameter indicates that the response should only contain assets where the current user
has the specified level of access.
*/
public java.lang.String getRole() {
return role;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
public List setRole(java.lang.String role) {
this.role = role;
return this;
}
/**
* A comma separated list of asset types. Returned assets will have one of the types from the
* provided list. Supported values are 'map', 'layer', 'rasterCollection' and 'table'.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/** A comma separated list of asset types. Returned assets will have one of the types from the provided
list. Supported values are 'map', 'layer', 'rasterCollection' and 'table'.
*/
public java.lang.String getType() {
return type;
}
/**
* A comma separated list of asset types. Returned assets will have one of the types from the
* provided list. Supported values are 'map', 'layer', 'rasterCollection' and 'table'.
*/
public List setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Parents collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Parents.List request = mapsengine.parents().list(parameters ...)}
*
*
* @return the resource collection
*/
public Parents parents() {
return new Parents();
}
/**
* The "parents" collection of methods.
*/
public class Parents {
/**
* Return all parent ids of the specified asset.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset whose parents will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "assets/{id}/parents";
/**
* Return all parent ids of the specified asset.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset whose parents will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.ParentsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the asset whose parents will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset whose parents will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset whose parents will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
50.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Permissions collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Permissions.List request = mapsengine.permissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Permissions permissions() {
return new Permissions();
}
/**
* The "permissions" collection of methods.
*/
public class Permissions {
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset whose permissions will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "assets/{id}/permissions";
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset whose permissions will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PermissionsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the asset whose permissions will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset whose permissions will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset whose permissions will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Layers collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Layers.List request = mapsengine.layers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Layers layers() {
return new Layers();
}
/**
* The "layers" collection of methods.
*/
public class Layers {
/**
* Cancel processing on a layer asset.
*
* Create a request for the method "layers.cancelProcessing".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link CancelProcessing#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer.
* @return the request
*/
public CancelProcessing cancelProcessing(java.lang.String id) throws java.io.IOException {
CancelProcessing result = new CancelProcessing(id);
initialize(result);
return result;
}
public class CancelProcessing extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/cancelProcessing";
/**
* Cancel processing on a layer asset.
*
* Create a request for the method "layers.cancelProcessing".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link CancelProcessing#execute()} method to invoke the remote
* operation. {@link CancelProcessing#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param id The ID of the layer.
* @since 1.13
*/
protected CancelProcessing(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.ProcessResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public CancelProcessing setAlt(java.lang.String alt) {
return (CancelProcessing) super.setAlt(alt);
}
@Override
public CancelProcessing setFields(java.lang.String fields) {
return (CancelProcessing) super.setFields(fields);
}
@Override
public CancelProcessing setKey(java.lang.String key) {
return (CancelProcessing) super.setKey(key);
}
@Override
public CancelProcessing setOauthToken(java.lang.String oauthToken) {
return (CancelProcessing) super.setOauthToken(oauthToken);
}
@Override
public CancelProcessing setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CancelProcessing) super.setPrettyPrint(prettyPrint);
}
@Override
public CancelProcessing setQuotaUser(java.lang.String quotaUser) {
return (CancelProcessing) super.setQuotaUser(quotaUser);
}
@Override
public CancelProcessing setUserIp(java.lang.String userIp) {
return (CancelProcessing) super.setUserIp(userIp);
}
/** The ID of the layer. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer. */
public CancelProcessing setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public CancelProcessing set(String parameterName, Object value) {
return (CancelProcessing) super.set(parameterName, value);
}
}
/**
* Create a layer asset.
*
* Create a request for the method "layers.create".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.mapsengine.model.Layer}
* @return the request
*/
public Create create(com.google.api.services.mapsengine.model.Layer content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends MapsEngineRequest {
private static final String REST_PATH = "layers";
/**
* Create a layer asset.
*
* Create a request for the method "layers.create".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.mapsengine.model.Layer}
* @since 1.13
*/
protected Create(com.google.api.services.mapsengine.model.Layer content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.Layer.class);
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getLayerType(), "Layer.getLayerType()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Layer.getName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getProjectId(), "Layer.getProjectId()");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/** Whether to queue the created layer for processing. */
@com.google.api.client.util.Key
private java.lang.Boolean process;
/** Whether to queue the created layer for processing.
*/
public java.lang.Boolean getProcess() {
return process;
}
/** Whether to queue the created layer for processing. */
public Create setProcess(java.lang.Boolean process) {
this.process = process;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete a layer.
*
* Create a request for the method "layers.delete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer. Only the layer creator or project owner are permitted to delete. If the layer
* is published, or included in a map, the request will fail. Unpublish the layer, and remove
* it from all maps prior to deleting.
* @return the request
*/
public Delete delete(java.lang.String id) throws java.io.IOException {
Delete result = new Delete(id);
initialize(result);
return result;
}
public class Delete extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}";
/**
* Delete a layer.
*
* Create a request for the method "layers.delete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer. Only the layer creator or project owner are permitted to delete. If the layer
* is published, or included in a map, the request will fail. Unpublish the layer, and remove
* it from all maps prior to deleting.
* @since 1.13
*/
protected Delete(java.lang.String id) {
super(MapsEngine.this, "DELETE", REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the layer. Only the layer creator or project owner are permitted to delete. If
* the layer is published, or included in a map, the request will fail. Unpublish the layer,
* and remove it from all maps prior to deleting.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer. Only the layer creator or project owner are permitted to delete. If the layer
is published, or included in a map, the request will fail. Unpublish the layer, and remove it from
all maps prior to deleting.
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the layer. Only the layer creator or project owner are permitted to delete. If
* the layer is published, or included in a map, the request will fail. Unpublish the layer,
* and remove it from all maps prior to deleting.
*/
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Return metadata for a particular layer.
*
* Create a request for the method "layers.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer.
* @return the request
*/
public Get get(java.lang.String id) throws java.io.IOException {
Get result = new Get(id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}";
/**
* Return metadata for a particular layer.
*
* Create a request for the method "layers.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer.
* @since 1.13
*/
protected Get(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.Layer.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the layer. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Deprecated: The version parameter indicates which version of the layer should be returned.
* When version is set to published, the published version of the layer will be returned.
* Please use the layers.getPublished endpoint instead.
*/
@com.google.api.client.util.Key
private java.lang.String version;
/** Deprecated: The version parameter indicates which version of the layer should be returned. When
version is set to published, the published version of the layer will be returned. Please use the
layers.getPublished endpoint instead.
*/
public java.lang.String getVersion() {
return version;
}
/**
* Deprecated: The version parameter indicates which version of the layer should be returned.
* When version is set to published, the published version of the layer will be returned.
* Please use the layers.getPublished endpoint instead.
*/
public Get setVersion(java.lang.String version) {
this.version = version;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return the published metadata for a particular layer.
*
* Create a request for the method "layers.getPublished".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link GetPublished#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer.
* @return the request
*/
public GetPublished getPublished(java.lang.String id) throws java.io.IOException {
GetPublished result = new GetPublished(id);
initialize(result);
return result;
}
public class GetPublished extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/published";
/**
* Return the published metadata for a particular layer.
*
* Create a request for the method "layers.getPublished".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link GetPublished#execute()} method to invoke the remote
* operation. {@link
* GetPublished#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer.
* @since 1.13
*/
protected GetPublished(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PublishedLayer.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetPublished setAlt(java.lang.String alt) {
return (GetPublished) super.setAlt(alt);
}
@Override
public GetPublished setFields(java.lang.String fields) {
return (GetPublished) super.setFields(fields);
}
@Override
public GetPublished setKey(java.lang.String key) {
return (GetPublished) super.setKey(key);
}
@Override
public GetPublished setOauthToken(java.lang.String oauthToken) {
return (GetPublished) super.setOauthToken(oauthToken);
}
@Override
public GetPublished setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetPublished) super.setPrettyPrint(prettyPrint);
}
@Override
public GetPublished setQuotaUser(java.lang.String quotaUser) {
return (GetPublished) super.setQuotaUser(quotaUser);
}
@Override
public GetPublished setUserIp(java.lang.String userIp) {
return (GetPublished) super.setUserIp(userIp);
}
/** The ID of the layer. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer. */
public GetPublished setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public GetPublished set(String parameterName, Object value) {
return (GetPublished) super.set(parameterName, value);
}
}
/**
* Return all layers readable by the current user.
*
* Create a request for the method "layers.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "layers";
/**
* Return all layers readable by the current user.
*
* Create a request for the method "layers.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.LayersListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or after this time.
*/
public com.google.api.client.util.DateTime getModifiedAfter() {
return modifiedAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
public List setModifiedAfter(com.google.api.client.util.DateTime modifiedAfter) {
this.modifiedAfter = modifiedAfter;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or after this time.
*/
public com.google.api.client.util.DateTime getCreatedAfter() {
return createdAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
public List setCreatedAfter(com.google.api.client.util.DateTime createdAfter) {
this.createdAfter = createdAfter;
return this;
}
@com.google.api.client.util.Key
private java.lang.String processingStatus;
/**
*/
public java.lang.String getProcessingStatus() {
return processingStatus;
}
public List setProcessingStatus(java.lang.String processingStatus) {
this.processingStatus = processingStatus;
return this;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
@com.google.api.client.util.Key
private java.lang.String tags;
/** A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public java.lang.String getTags() {
return tags;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public List setTags(java.lang.String tags) {
this.tags = tags;
return this;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
@com.google.api.client.util.Key
private java.lang.String search;
/** An unstructured search string used to filter the set of results based on asset metadata.
*/
public java.lang.String getSearch() {
return search;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
public List setSearch(java.lang.String search) {
this.search = search;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/** An email address representing a user. Returned assets that have been created by the user associated
with the provided email address.
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
public List setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String bbox;
/** A bounding box, expressed as "west,south,east,north". If set, only assets which intersect this
bounding box will be returned.
*/
public java.lang.String getBbox() {
return bbox;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
public List setBbox(java.lang.String bbox) {
this.bbox = bbox;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or before this time.
*/
public com.google.api.client.util.DateTime getModifiedBefore() {
return modifiedBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
public List setModifiedBefore(com.google.api.client.util.DateTime modifiedBefore) {
this.modifiedBefore = modifiedBefore;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or before this time.
*/
public com.google.api.client.util.DateTime getCreatedBefore() {
return createdBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
public List setCreatedBefore(com.google.api.client.util.DateTime createdBefore) {
this.createdBefore = createdBefore;
return this;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/** The role parameter indicates that the response should only contain assets where the current user
has the specified level of access.
*/
public java.lang.String getRole() {
return role;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
public List setRole(java.lang.String role) {
this.role = role;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Return all published layers readable by the current user.
*
* Create a request for the method "layers.listPublished".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link ListPublished#execute()} method to invoke the remote operation.
*
* @return the request
*/
public ListPublished listPublished() throws java.io.IOException {
ListPublished result = new ListPublished();
initialize(result);
return result;
}
public class ListPublished extends MapsEngineRequest {
private static final String REST_PATH = "layers/published";
/**
* Return all published layers readable by the current user.
*
* Create a request for the method "layers.listPublished".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link ListPublished#execute()} method to invoke the remote
* operation. {@link ListPublished#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @since 1.13
*/
protected ListPublished() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PublishedLayersListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListPublished setAlt(java.lang.String alt) {
return (ListPublished) super.setAlt(alt);
}
@Override
public ListPublished setFields(java.lang.String fields) {
return (ListPublished) super.setFields(fields);
}
@Override
public ListPublished setKey(java.lang.String key) {
return (ListPublished) super.setKey(key);
}
@Override
public ListPublished setOauthToken(java.lang.String oauthToken) {
return (ListPublished) super.setOauthToken(oauthToken);
}
@Override
public ListPublished setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListPublished) super.setPrettyPrint(prettyPrint);
}
@Override
public ListPublished setQuotaUser(java.lang.String quotaUser) {
return (ListPublished) super.setQuotaUser(quotaUser);
}
@Override
public ListPublished setUserIp(java.lang.String userIp) {
return (ListPublished) super.setUserIp(userIp);
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public ListPublished setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public ListPublished setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public ListPublished setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public ListPublished set(String parameterName, Object value) {
return (ListPublished) super.set(parameterName, value);
}
}
/**
* Mutate a layer asset.
*
* Create a request for the method "layers.patch".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer.
* @param content the {@link com.google.api.services.mapsengine.model.Layer}
* @return the request
*/
public Patch patch(java.lang.String id, com.google.api.services.mapsengine.model.Layer content) throws java.io.IOException {
Patch result = new Patch(id, content);
initialize(result);
return result;
}
public class Patch extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}";
/**
* Mutate a layer asset.
*
* Create a request for the method "layers.patch".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer.
* @param content the {@link com.google.api.services.mapsengine.model.Layer}
* @since 1.13
*/
protected Patch(java.lang.String id, com.google.api.services.mapsengine.model.Layer content) {
super(MapsEngine.this, "PATCH", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** The ID of the layer. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer. */
public Patch setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Process a layer asset.
*
* Create a request for the method "layers.process".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Process#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer.
* @return the request
*/
public Process process(java.lang.String id) throws java.io.IOException {
Process result = new Process(id);
initialize(result);
return result;
}
public class Process extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/process";
/**
* Process a layer asset.
*
* Create a request for the method "layers.process".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Process#execute()} method to invoke the remote operation.
* {@link
* Process#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer.
* @since 1.13
*/
protected Process(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.ProcessResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Process setAlt(java.lang.String alt) {
return (Process) super.setAlt(alt);
}
@Override
public Process setFields(java.lang.String fields) {
return (Process) super.setFields(fields);
}
@Override
public Process setKey(java.lang.String key) {
return (Process) super.setKey(key);
}
@Override
public Process setOauthToken(java.lang.String oauthToken) {
return (Process) super.setOauthToken(oauthToken);
}
@Override
public Process setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Process) super.setPrettyPrint(prettyPrint);
}
@Override
public Process setQuotaUser(java.lang.String quotaUser) {
return (Process) super.setQuotaUser(quotaUser);
}
@Override
public Process setUserIp(java.lang.String userIp) {
return (Process) super.setUserIp(userIp);
}
/** The ID of the layer. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer. */
public Process setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Process set(String parameterName, Object value) {
return (Process) super.set(parameterName, value);
}
}
/**
* Publish a layer asset.
*
* Create a request for the method "layers.publish".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Publish#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer.
* @return the request
*/
public Publish publish(java.lang.String id) throws java.io.IOException {
Publish result = new Publish(id);
initialize(result);
return result;
}
public class Publish extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/publish";
/**
* Publish a layer asset.
*
* Create a request for the method "layers.publish".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Publish#execute()} method to invoke the remote operation.
* {@link
* Publish#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer.
* @since 1.13
*/
protected Publish(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.PublishResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Publish setAlt(java.lang.String alt) {
return (Publish) super.setAlt(alt);
}
@Override
public Publish setFields(java.lang.String fields) {
return (Publish) super.setFields(fields);
}
@Override
public Publish setKey(java.lang.String key) {
return (Publish) super.setKey(key);
}
@Override
public Publish setOauthToken(java.lang.String oauthToken) {
return (Publish) super.setOauthToken(oauthToken);
}
@Override
public Publish setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Publish) super.setPrettyPrint(prettyPrint);
}
@Override
public Publish setQuotaUser(java.lang.String quotaUser) {
return (Publish) super.setQuotaUser(quotaUser);
}
@Override
public Publish setUserIp(java.lang.String userIp) {
return (Publish) super.setUserIp(userIp);
}
/** The ID of the layer. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer. */
public Publish setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* If set to true, the API will allow publication of the layer even if it's out of date. If
* not true, you'll need to reprocess any out-of-date layer before publishing.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** If set to true, the API will allow publication of the layer even if it's out of date. If not true,
you'll need to reprocess any out-of-date layer before publishing.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* If set to true, the API will allow publication of the layer even if it's out of date. If
* not true, you'll need to reprocess any out-of-date layer before publishing.
*/
public Publish setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Publish set(String parameterName, Object value) {
return (Publish) super.set(parameterName, value);
}
}
/**
* Unpublish a layer asset.
*
* Create a request for the method "layers.unpublish".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Unpublish#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer.
* @return the request
*/
public Unpublish unpublish(java.lang.String id) throws java.io.IOException {
Unpublish result = new Unpublish(id);
initialize(result);
return result;
}
public class Unpublish extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/unpublish";
/**
* Unpublish a layer asset.
*
* Create a request for the method "layers.unpublish".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Unpublish#execute()} method to invoke the remote
* operation. {@link
* Unpublish#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer.
* @since 1.13
*/
protected Unpublish(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.PublishResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Unpublish setAlt(java.lang.String alt) {
return (Unpublish) super.setAlt(alt);
}
@Override
public Unpublish setFields(java.lang.String fields) {
return (Unpublish) super.setFields(fields);
}
@Override
public Unpublish setKey(java.lang.String key) {
return (Unpublish) super.setKey(key);
}
@Override
public Unpublish setOauthToken(java.lang.String oauthToken) {
return (Unpublish) super.setOauthToken(oauthToken);
}
@Override
public Unpublish setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unpublish) super.setPrettyPrint(prettyPrint);
}
@Override
public Unpublish setQuotaUser(java.lang.String quotaUser) {
return (Unpublish) super.setQuotaUser(quotaUser);
}
@Override
public Unpublish setUserIp(java.lang.String userIp) {
return (Unpublish) super.setUserIp(userIp);
}
/** The ID of the layer. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer. */
public Unpublish setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Unpublish set(String parameterName, Object value) {
return (Unpublish) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Parents collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Parents.List request = mapsengine.parents().list(parameters ...)}
*
*
* @return the resource collection
*/
public Parents parents() {
return new Parents();
}
/**
* The "parents" collection of methods.
*/
public class Parents {
/**
* Return all parent ids of the specified layer.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the layer whose parents will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/parents";
/**
* Return all parent ids of the specified layer.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the layer whose parents will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.ParentsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the layer whose parents will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the layer whose parents will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the layer whose parents will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
50.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Permissions collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Permissions.List request = mapsengine.permissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Permissions permissions() {
return new Permissions();
}
/**
* The "permissions" collection of methods.
*/
public class Permissions {
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(id, content);
initialize(result);
return result;
}
public class BatchDelete extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/permissions/batchDelete";
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchDeleteResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/** The ID of the asset from which permissions will be removed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset from which permissions will be removed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset from which permissions will be removed. */
public BatchDelete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @return the request
*/
public BatchUpdate batchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(id, content);
initialize(result);
return result;
}
public class BatchUpdate extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/permissions/batchUpdate";
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchUpdate#execute()} method to invoke the remote
* operation. {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @since 1.13
*/
protected BatchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchUpdateResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUserIp(java.lang.String userIp) {
return (BatchUpdate) super.setUserIp(userIp);
}
/** The ID of the asset to which permissions will be added. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset to which permissions will be added.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset to which permissions will be added. */
public BatchUpdate setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset whose permissions will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "layers/{id}/permissions";
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset whose permissions will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PermissionsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the asset whose permissions will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset whose permissions will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset whose permissions will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Maps collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Maps.List request = mapsengine.maps().list(parameters ...)}
*
*
* @return the resource collection
*/
public Maps maps() {
return new Maps();
}
/**
* The "maps" collection of methods.
*/
public class Maps {
/**
* Create a map asset.
*
* Create a request for the method "maps.create".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.mapsengine.model.Map}
* @return the request
*/
public Create create(com.google.api.services.mapsengine.model.Map content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends MapsEngineRequest {
private static final String REST_PATH = "maps";
/**
* Create a map asset.
*
* Create a request for the method "maps.create".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.mapsengine.model.Map}
* @since 1.13
*/
protected Create(com.google.api.services.mapsengine.model.Map content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.Map.class);
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Map.getName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getProjectId(), "Map.getProjectId()");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete a map.
*
* Create a request for the method "maps.delete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param id The ID of the map. Only the map creator or project owner are permitted to delete. If the map is
* published the request will fail. Unpublish the map prior to deleting.
* @return the request
*/
public Delete delete(java.lang.String id) throws java.io.IOException {
Delete result = new Delete(id);
initialize(result);
return result;
}
public class Delete extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}";
/**
* Delete a map.
*
* Create a request for the method "maps.delete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the map. Only the map creator or project owner are permitted to delete. If the map is
* published the request will fail. Unpublish the map prior to deleting.
* @since 1.13
*/
protected Delete(java.lang.String id) {
super(MapsEngine.this, "DELETE", REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the map. Only the map creator or project owner are permitted to delete. If the
* map is published the request will fail. Unpublish the map prior to deleting.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the map. Only the map creator or project owner are permitted to delete. If the map is
published the request will fail. Unpublish the map prior to deleting.
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the map. Only the map creator or project owner are permitted to delete. If the
* map is published the request will fail. Unpublish the map prior to deleting.
*/
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Return metadata for a particular map.
*
* Create a request for the method "maps.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param id The ID of the map.
* @return the request
*/
public Get get(java.lang.String id) throws java.io.IOException {
Get result = new Get(id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}";
/**
* Return metadata for a particular map.
*
* Create a request for the method "maps.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the map.
* @since 1.13
*/
protected Get(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.Map.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the map. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the map.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the map. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Deprecated: The version parameter indicates which version of the map should be returned.
* When version is set to published, the published version of the map will be returned. Please
* use the maps.getPublished endpoint instead.
*/
@com.google.api.client.util.Key
private java.lang.String version;
/** Deprecated: The version parameter indicates which version of the map should be returned. When
version is set to published, the published version of the map will be returned. Please use the
maps.getPublished endpoint instead.
*/
public java.lang.String getVersion() {
return version;
}
/**
* Deprecated: The version parameter indicates which version of the map should be returned.
* When version is set to published, the published version of the map will be returned. Please
* use the maps.getPublished endpoint instead.
*/
public Get setVersion(java.lang.String version) {
this.version = version;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return the published metadata for a particular map.
*
* Create a request for the method "maps.getPublished".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link GetPublished#execute()} method to invoke the remote operation.
*
* @param id The ID of the map.
* @return the request
*/
public GetPublished getPublished(java.lang.String id) throws java.io.IOException {
GetPublished result = new GetPublished(id);
initialize(result);
return result;
}
public class GetPublished extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}/published";
/**
* Return the published metadata for a particular map.
*
* Create a request for the method "maps.getPublished".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link GetPublished#execute()} method to invoke the remote
* operation. {@link
* GetPublished#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the map.
* @since 1.13
*/
protected GetPublished(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PublishedMap.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetPublished setAlt(java.lang.String alt) {
return (GetPublished) super.setAlt(alt);
}
@Override
public GetPublished setFields(java.lang.String fields) {
return (GetPublished) super.setFields(fields);
}
@Override
public GetPublished setKey(java.lang.String key) {
return (GetPublished) super.setKey(key);
}
@Override
public GetPublished setOauthToken(java.lang.String oauthToken) {
return (GetPublished) super.setOauthToken(oauthToken);
}
@Override
public GetPublished setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetPublished) super.setPrettyPrint(prettyPrint);
}
@Override
public GetPublished setQuotaUser(java.lang.String quotaUser) {
return (GetPublished) super.setQuotaUser(quotaUser);
}
@Override
public GetPublished setUserIp(java.lang.String userIp) {
return (GetPublished) super.setUserIp(userIp);
}
/** The ID of the map. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the map.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the map. */
public GetPublished setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public GetPublished set(String parameterName, Object value) {
return (GetPublished) super.set(parameterName, value);
}
}
/**
* Return all maps readable by the current user.
*
* Create a request for the method "maps.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "maps";
/**
* Return all maps readable by the current user.
*
* Create a request for the method "maps.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.MapsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or after this time.
*/
public com.google.api.client.util.DateTime getModifiedAfter() {
return modifiedAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
public List setModifiedAfter(com.google.api.client.util.DateTime modifiedAfter) {
this.modifiedAfter = modifiedAfter;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or after this time.
*/
public com.google.api.client.util.DateTime getCreatedAfter() {
return createdAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
public List setCreatedAfter(com.google.api.client.util.DateTime createdAfter) {
this.createdAfter = createdAfter;
return this;
}
@com.google.api.client.util.Key
private java.lang.String processingStatus;
/**
*/
public java.lang.String getProcessingStatus() {
return processingStatus;
}
public List setProcessingStatus(java.lang.String processingStatus) {
this.processingStatus = processingStatus;
return this;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
@com.google.api.client.util.Key
private java.lang.String tags;
/** A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public java.lang.String getTags() {
return tags;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public List setTags(java.lang.String tags) {
this.tags = tags;
return this;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
@com.google.api.client.util.Key
private java.lang.String search;
/** An unstructured search string used to filter the set of results based on asset metadata.
*/
public java.lang.String getSearch() {
return search;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
public List setSearch(java.lang.String search) {
this.search = search;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/** An email address representing a user. Returned assets that have been created by the user associated
with the provided email address.
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
public List setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String bbox;
/** A bounding box, expressed as "west,south,east,north". If set, only assets which intersect this
bounding box will be returned.
*/
public java.lang.String getBbox() {
return bbox;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
public List setBbox(java.lang.String bbox) {
this.bbox = bbox;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or before this time.
*/
public com.google.api.client.util.DateTime getModifiedBefore() {
return modifiedBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
public List setModifiedBefore(com.google.api.client.util.DateTime modifiedBefore) {
this.modifiedBefore = modifiedBefore;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or before this time.
*/
public com.google.api.client.util.DateTime getCreatedBefore() {
return createdBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
public List setCreatedBefore(com.google.api.client.util.DateTime createdBefore) {
this.createdBefore = createdBefore;
return this;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/** The role parameter indicates that the response should only contain assets where the current user
has the specified level of access.
*/
public java.lang.String getRole() {
return role;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
public List setRole(java.lang.String role) {
this.role = role;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Return all published maps readable by the current user.
*
* Create a request for the method "maps.listPublished".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link ListPublished#execute()} method to invoke the remote operation.
*
* @return the request
*/
public ListPublished listPublished() throws java.io.IOException {
ListPublished result = new ListPublished();
initialize(result);
return result;
}
public class ListPublished extends MapsEngineRequest {
private static final String REST_PATH = "maps/published";
/**
* Return all published maps readable by the current user.
*
* Create a request for the method "maps.listPublished".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link ListPublished#execute()} method to invoke the remote
* operation. {@link ListPublished#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @since 1.13
*/
protected ListPublished() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PublishedMapsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListPublished setAlt(java.lang.String alt) {
return (ListPublished) super.setAlt(alt);
}
@Override
public ListPublished setFields(java.lang.String fields) {
return (ListPublished) super.setFields(fields);
}
@Override
public ListPublished setKey(java.lang.String key) {
return (ListPublished) super.setKey(key);
}
@Override
public ListPublished setOauthToken(java.lang.String oauthToken) {
return (ListPublished) super.setOauthToken(oauthToken);
}
@Override
public ListPublished setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListPublished) super.setPrettyPrint(prettyPrint);
}
@Override
public ListPublished setQuotaUser(java.lang.String quotaUser) {
return (ListPublished) super.setQuotaUser(quotaUser);
}
@Override
public ListPublished setUserIp(java.lang.String userIp) {
return (ListPublished) super.setUserIp(userIp);
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public ListPublished setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public ListPublished setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public ListPublished setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public ListPublished set(String parameterName, Object value) {
return (ListPublished) super.set(parameterName, value);
}
}
/**
* Mutate a map asset.
*
* Create a request for the method "maps.patch".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param id The ID of the map.
* @param content the {@link com.google.api.services.mapsengine.model.Map}
* @return the request
*/
public Patch patch(java.lang.String id, com.google.api.services.mapsengine.model.Map content) throws java.io.IOException {
Patch result = new Patch(id, content);
initialize(result);
return result;
}
public class Patch extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}";
/**
* Mutate a map asset.
*
* Create a request for the method "maps.patch".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the map.
* @param content the {@link com.google.api.services.mapsengine.model.Map}
* @since 1.13
*/
protected Patch(java.lang.String id, com.google.api.services.mapsengine.model.Map content) {
super(MapsEngine.this, "PATCH", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** The ID of the map. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the map.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the map. */
public Patch setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Publish a map asset.
*
* Create a request for the method "maps.publish".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Publish#execute()} method to invoke the remote operation.
*
* @param id The ID of the map.
* @return the request
*/
public Publish publish(java.lang.String id) throws java.io.IOException {
Publish result = new Publish(id);
initialize(result);
return result;
}
public class Publish extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}/publish";
/**
* Publish a map asset.
*
* Create a request for the method "maps.publish".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Publish#execute()} method to invoke the remote operation.
* {@link
* Publish#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the map.
* @since 1.13
*/
protected Publish(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.PublishResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Publish setAlt(java.lang.String alt) {
return (Publish) super.setAlt(alt);
}
@Override
public Publish setFields(java.lang.String fields) {
return (Publish) super.setFields(fields);
}
@Override
public Publish setKey(java.lang.String key) {
return (Publish) super.setKey(key);
}
@Override
public Publish setOauthToken(java.lang.String oauthToken) {
return (Publish) super.setOauthToken(oauthToken);
}
@Override
public Publish setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Publish) super.setPrettyPrint(prettyPrint);
}
@Override
public Publish setQuotaUser(java.lang.String quotaUser) {
return (Publish) super.setQuotaUser(quotaUser);
}
@Override
public Publish setUserIp(java.lang.String userIp) {
return (Publish) super.setUserIp(userIp);
}
/** The ID of the map. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the map.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the map. */
public Publish setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* If set to true, the API will allow publication of the map even if it's out of date. If
* false, the map must have a processingStatus of complete before publishing.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** If set to true, the API will allow publication of the map even if it's out of date. If false, the
map must have a processingStatus of complete before publishing.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* If set to true, the API will allow publication of the map even if it's out of date. If
* false, the map must have a processingStatus of complete before publishing.
*/
public Publish setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Publish set(String parameterName, Object value) {
return (Publish) super.set(parameterName, value);
}
}
/**
* Unpublish a map asset.
*
* Create a request for the method "maps.unpublish".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Unpublish#execute()} method to invoke the remote operation.
*
* @param id The ID of the map.
* @return the request
*/
public Unpublish unpublish(java.lang.String id) throws java.io.IOException {
Unpublish result = new Unpublish(id);
initialize(result);
return result;
}
public class Unpublish extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}/unpublish";
/**
* Unpublish a map asset.
*
* Create a request for the method "maps.unpublish".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Unpublish#execute()} method to invoke the remote
* operation. {@link
* Unpublish#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the map.
* @since 1.13
*/
protected Unpublish(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.PublishResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Unpublish setAlt(java.lang.String alt) {
return (Unpublish) super.setAlt(alt);
}
@Override
public Unpublish setFields(java.lang.String fields) {
return (Unpublish) super.setFields(fields);
}
@Override
public Unpublish setKey(java.lang.String key) {
return (Unpublish) super.setKey(key);
}
@Override
public Unpublish setOauthToken(java.lang.String oauthToken) {
return (Unpublish) super.setOauthToken(oauthToken);
}
@Override
public Unpublish setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unpublish) super.setPrettyPrint(prettyPrint);
}
@Override
public Unpublish setQuotaUser(java.lang.String quotaUser) {
return (Unpublish) super.setQuotaUser(quotaUser);
}
@Override
public Unpublish setUserIp(java.lang.String userIp) {
return (Unpublish) super.setUserIp(userIp);
}
/** The ID of the map. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the map.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the map. */
public Unpublish setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Unpublish set(String parameterName, Object value) {
return (Unpublish) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Permissions collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Permissions.List request = mapsengine.permissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Permissions permissions() {
return new Permissions();
}
/**
* The "permissions" collection of methods.
*/
public class Permissions {
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(id, content);
initialize(result);
return result;
}
public class BatchDelete extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}/permissions/batchDelete";
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchDeleteResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/** The ID of the asset from which permissions will be removed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset from which permissions will be removed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset from which permissions will be removed. */
public BatchDelete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @return the request
*/
public BatchUpdate batchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(id, content);
initialize(result);
return result;
}
public class BatchUpdate extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}/permissions/batchUpdate";
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchUpdate#execute()} method to invoke the remote
* operation. {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @since 1.13
*/
protected BatchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchUpdateResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUserIp(java.lang.String userIp) {
return (BatchUpdate) super.setUserIp(userIp);
}
/** The ID of the asset to which permissions will be added. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset to which permissions will be added.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset to which permissions will be added. */
public BatchUpdate setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset whose permissions will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "maps/{id}/permissions";
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset whose permissions will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PermissionsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the asset whose permissions will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset whose permissions will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset whose permissions will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Projects.List request = mapsengine.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* Return all projects readable by the current user.
*
* Create a request for the method "projects.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "projects";
/**
* Return all projects readable by the current user.
*
* Create a request for the method "projects.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.ProjectsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Icons collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Icons.List request = mapsengine.icons().list(parameters ...)}
*
*
* @return the resource collection
*/
public Icons icons() {
return new Icons();
}
/**
* The "icons" collection of methods.
*/
public class Icons {
/**
* Create an icon.
*
* Create a request for the method "icons.create".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param projectId The ID of the project.
* @param content the {@link com.google.api.services.mapsengine.model.Icon}
* @return the request
*/
public Create create(java.lang.String projectId, com.google.api.services.mapsengine.model.Icon content) throws java.io.IOException {
Create result = new Create(projectId, content);
initialize(result);
return result;
}
/**
* Create an icon.
*
* Create a request for the method "icons.create".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param projectId The ID of the project.
* @param content the {@link com.google.api.services.mapsengine.model.Icon} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Create create(java.lang.String projectId, com.google.api.services.mapsengine.model.Icon content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Create result = new Create(projectId, content, mediaContent);
initialize(result);
return result;
}
public class Create extends MapsEngineRequest {
private static final String REST_PATH = "projects/{projectId}/icons";
/**
* Create an icon.
*
* Create a request for the method "icons.create".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The ID of the project.
* @param content the {@link com.google.api.services.mapsengine.model.Icon}
* @since 1.13
*/
protected Create(java.lang.String projectId, com.google.api.services.mapsengine.model.Icon content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.Icon.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Icon.getName()");
}
/**
* Create an icon.
*
* Create a request for the method "icons.create".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param projectId The ID of the project.
* @param content the {@link com.google.api.services.mapsengine.model.Icon} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Create(java.lang.String projectId, com.google.api.services.mapsengine.model.Icon content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(MapsEngine.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.mapsengine.model.Icon.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/** The ID of the project. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of the project.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The ID of the project. */
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Return an icon or its associated metadata
*
* Create a request for the method "icons.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId The ID of the project.
* @param id The ID of the icon.
* @return the request
*/
public Get get(java.lang.String projectId, java.lang.String id) throws java.io.IOException {
Get result = new Get(projectId, id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "projects/{projectId}/icons/{id}";
/**
* Return an icon or its associated metadata
*
* Create a request for the method "icons.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The ID of the project.
* @param id The ID of the icon.
* @since 1.13
*/
protected Get(java.lang.String projectId, java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.Icon.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
initializeMediaDownload();
}
@Override
public void executeMediaAndDownloadTo(java.io.OutputStream outputStream) throws java.io.IOException {
super.executeMediaAndDownloadTo(outputStream);
}
@Override
public java.io.InputStream executeMediaAsInputStream() throws java.io.IOException {
return super.executeMediaAsInputStream();
}
@Override
public com.google.api.client.http.HttpResponse executeMedia() throws java.io.IOException {
return super.executeMedia();
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the project. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of the project.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The ID of the project. */
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** The ID of the icon. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the icon.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the icon. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return all icons in the current project
*
* Create a request for the method "icons.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId The ID of the project.
* @return the request
*/
public List list(java.lang.String projectId) throws java.io.IOException {
List result = new List(projectId);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "projects/{projectId}/icons";
/**
* Return all icons in the current project
*
* Create a request for the method "icons.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The ID of the project.
* @since 1.13
*/
protected List(java.lang.String projectId) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.IconsListResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the project. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of the project.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The ID of the project. */
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
50.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the RasterCollections collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.RasterCollections.List request = mapsengine.rasterCollections().list(parameters ...)}
*
*
* @return the resource collection
*/
public RasterCollections rasterCollections() {
return new RasterCollections();
}
/**
* The "rasterCollections" collection of methods.
*/
public class RasterCollections {
/**
* Cancel processing on a raster collection asset.
*
* Create a request for the method "rasterCollections.cancelProcessing".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link CancelProcessing#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection.
* @return the request
*/
public CancelProcessing cancelProcessing(java.lang.String id) throws java.io.IOException {
CancelProcessing result = new CancelProcessing(id);
initialize(result);
return result;
}
public class CancelProcessing extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/cancelProcessing";
/**
* Cancel processing on a raster collection asset.
*
* Create a request for the method "rasterCollections.cancelProcessing".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link CancelProcessing#execute()} method to invoke the remote
* operation. {@link CancelProcessing#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param id The ID of the raster collection.
* @since 1.13
*/
protected CancelProcessing(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.ProcessResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public CancelProcessing setAlt(java.lang.String alt) {
return (CancelProcessing) super.setAlt(alt);
}
@Override
public CancelProcessing setFields(java.lang.String fields) {
return (CancelProcessing) super.setFields(fields);
}
@Override
public CancelProcessing setKey(java.lang.String key) {
return (CancelProcessing) super.setKey(key);
}
@Override
public CancelProcessing setOauthToken(java.lang.String oauthToken) {
return (CancelProcessing) super.setOauthToken(oauthToken);
}
@Override
public CancelProcessing setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CancelProcessing) super.setPrettyPrint(prettyPrint);
}
@Override
public CancelProcessing setQuotaUser(java.lang.String quotaUser) {
return (CancelProcessing) super.setQuotaUser(quotaUser);
}
@Override
public CancelProcessing setUserIp(java.lang.String userIp) {
return (CancelProcessing) super.setUserIp(userIp);
}
/** The ID of the raster collection. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection. */
public CancelProcessing setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public CancelProcessing set(String parameterName, Object value) {
return (CancelProcessing) super.set(parameterName, value);
}
}
/**
* Create a raster collection asset.
*
* Create a request for the method "rasterCollections.create".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollection}
* @return the request
*/
public Create create(com.google.api.services.mapsengine.model.RasterCollection content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections";
/**
* Create a raster collection asset.
*
* Create a request for the method "rasterCollections.create".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollection}
* @since 1.13
*/
protected Create(com.google.api.services.mapsengine.model.RasterCollection content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.RasterCollection.class);
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getMosaic(), "RasterCollection.getMosaic()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "RasterCollection.getName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getProjectId(), "RasterCollection.getProjectId()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getRasterType(), "RasterCollection.getRasterType()");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete a raster collection.
*
* Create a request for the method "rasterCollections.delete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection. Only the raster collection creator or project owner are permitted
* to delete. If the rastor collection is included in a layer, the request will fail. Remove
* the raster collection from all layers prior to deleting.
* @return the request
*/
public Delete delete(java.lang.String id) throws java.io.IOException {
Delete result = new Delete(id);
initialize(result);
return result;
}
public class Delete extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}";
/**
* Delete a raster collection.
*
* Create a request for the method "rasterCollections.delete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection. Only the raster collection creator or project owner are permitted
* to delete. If the rastor collection is included in a layer, the request will fail. Remove
* the raster collection from all layers prior to deleting.
* @since 1.13
*/
protected Delete(java.lang.String id) {
super(MapsEngine.this, "DELETE", REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the raster collection. Only the raster collection creator or project owner are
* permitted to delete. If the rastor collection is included in a layer, the request will
* fail. Remove the raster collection from all layers prior to deleting.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection. Only the raster collection creator or project owner are permitted
to delete. If the rastor collection is included in a layer, the request will fail. Remove the
raster collection from all layers prior to deleting.
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the raster collection. Only the raster collection creator or project owner are
* permitted to delete. If the rastor collection is included in a layer, the request will
* fail. Remove the raster collection from all layers prior to deleting.
*/
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Return metadata for a particular raster collection.
*
* Create a request for the method "rasterCollections.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection.
* @return the request
*/
public Get get(java.lang.String id) throws java.io.IOException {
Get result = new Get(id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}";
/**
* Return metadata for a particular raster collection.
*
* Create a request for the method "rasterCollections.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection.
* @since 1.13
*/
protected Get(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.RasterCollection.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the raster collection. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return all raster collections readable by the current user.
*
* Create a request for the method "rasterCollections.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections";
/**
* Return all raster collections readable by the current user.
*
* Create a request for the method "rasterCollections.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.RasterCollectionsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or after this time.
*/
public com.google.api.client.util.DateTime getModifiedAfter() {
return modifiedAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
public List setModifiedAfter(com.google.api.client.util.DateTime modifiedAfter) {
this.modifiedAfter = modifiedAfter;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or after this time.
*/
public com.google.api.client.util.DateTime getCreatedAfter() {
return createdAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
public List setCreatedAfter(com.google.api.client.util.DateTime createdAfter) {
this.createdAfter = createdAfter;
return this;
}
@com.google.api.client.util.Key
private java.lang.String processingStatus;
/**
*/
public java.lang.String getProcessingStatus() {
return processingStatus;
}
public List setProcessingStatus(java.lang.String processingStatus) {
this.processingStatus = processingStatus;
return this;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
@com.google.api.client.util.Key
private java.lang.String tags;
/** A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public java.lang.String getTags() {
return tags;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public List setTags(java.lang.String tags) {
this.tags = tags;
return this;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
@com.google.api.client.util.Key
private java.lang.String search;
/** An unstructured search string used to filter the set of results based on asset metadata.
*/
public java.lang.String getSearch() {
return search;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
public List setSearch(java.lang.String search) {
this.search = search;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/** An email address representing a user. Returned assets that have been created by the user associated
with the provided email address.
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
public List setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String bbox;
/** A bounding box, expressed as "west,south,east,north". If set, only assets which intersect this
bounding box will be returned.
*/
public java.lang.String getBbox() {
return bbox;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
public List setBbox(java.lang.String bbox) {
this.bbox = bbox;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or before this time.
*/
public com.google.api.client.util.DateTime getModifiedBefore() {
return modifiedBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
public List setModifiedBefore(com.google.api.client.util.DateTime modifiedBefore) {
this.modifiedBefore = modifiedBefore;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or before this time.
*/
public com.google.api.client.util.DateTime getCreatedBefore() {
return createdBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
public List setCreatedBefore(com.google.api.client.util.DateTime createdBefore) {
this.createdBefore = createdBefore;
return this;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/** The role parameter indicates that the response should only contain assets where the current user
has the specified level of access.
*/
public java.lang.String getRole() {
return role;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
public List setRole(java.lang.String role) {
this.role = role;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Mutate a raster collection asset.
*
* Create a request for the method "rasterCollections.patch".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection.
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollection}
* @return the request
*/
public Patch patch(java.lang.String id, com.google.api.services.mapsengine.model.RasterCollection content) throws java.io.IOException {
Patch result = new Patch(id, content);
initialize(result);
return result;
}
public class Patch extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}";
/**
* Mutate a raster collection asset.
*
* Create a request for the method "rasterCollections.patch".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection.
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollection}
* @since 1.13
*/
protected Patch(java.lang.String id, com.google.api.services.mapsengine.model.RasterCollection content) {
super(MapsEngine.this, "PATCH", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** The ID of the raster collection. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection. */
public Patch setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Process a raster collection asset.
*
* Create a request for the method "rasterCollections.process".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Process#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection.
* @return the request
*/
public Process process(java.lang.String id) throws java.io.IOException {
Process result = new Process(id);
initialize(result);
return result;
}
public class Process extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/process";
/**
* Process a raster collection asset.
*
* Create a request for the method "rasterCollections.process".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Process#execute()} method to invoke the remote operation.
* {@link
* Process#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection.
* @since 1.13
*/
protected Process(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.ProcessResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Process setAlt(java.lang.String alt) {
return (Process) super.setAlt(alt);
}
@Override
public Process setFields(java.lang.String fields) {
return (Process) super.setFields(fields);
}
@Override
public Process setKey(java.lang.String key) {
return (Process) super.setKey(key);
}
@Override
public Process setOauthToken(java.lang.String oauthToken) {
return (Process) super.setOauthToken(oauthToken);
}
@Override
public Process setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Process) super.setPrettyPrint(prettyPrint);
}
@Override
public Process setQuotaUser(java.lang.String quotaUser) {
return (Process) super.setQuotaUser(quotaUser);
}
@Override
public Process setUserIp(java.lang.String userIp) {
return (Process) super.setUserIp(userIp);
}
/** The ID of the raster collection. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection. */
public Process setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Process set(String parameterName, Object value) {
return (Process) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Parents collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Parents.List request = mapsengine.parents().list(parameters ...)}
*
*
* @return the resource collection
*/
public Parents parents() {
return new Parents();
}
/**
* The "parents" collection of methods.
*/
public class Parents {
/**
* Return all parent ids of the specified raster collection.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection whose parents will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/parents";
/**
* Return all parent ids of the specified raster collection.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection whose parents will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.ParentsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the raster collection whose parents will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection whose parents will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection whose parents will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
50.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Permissions collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Permissions.List request = mapsengine.permissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Permissions permissions() {
return new Permissions();
}
/**
* The "permissions" collection of methods.
*/
public class Permissions {
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(id, content);
initialize(result);
return result;
}
public class BatchDelete extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/permissions/batchDelete";
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchDeleteResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/** The ID of the asset from which permissions will be removed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset from which permissions will be removed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset from which permissions will be removed. */
public BatchDelete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @return the request
*/
public BatchUpdate batchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(id, content);
initialize(result);
return result;
}
public class BatchUpdate extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/permissions/batchUpdate";
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchUpdate#execute()} method to invoke the remote
* operation. {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @since 1.13
*/
protected BatchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchUpdateResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUserIp(java.lang.String userIp) {
return (BatchUpdate) super.setUserIp(userIp);
}
/** The ID of the asset to which permissions will be added. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset to which permissions will be added.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset to which permissions will be added. */
public BatchUpdate setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset whose permissions will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/permissions";
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset whose permissions will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PermissionsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the asset whose permissions will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset whose permissions will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset whose permissions will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Rasters collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Rasters.List request = mapsengine.rasters().list(parameters ...)}
*
*
* @return the resource collection
*/
public Rasters rasters() {
return new Rasters();
}
/**
* The "rasters" collection of methods.
*/
public class Rasters {
/**
* Remove rasters from an existing raster collection.
*
* Up to 50 rasters can be included in a single batchDelete request. Each batchDelete request is
* atomic.
*
* Create a request for the method "rasters.batchDelete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection to which these rasters belong.
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollectionsRasterBatchDeleteRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String id, com.google.api.services.mapsengine.model.RasterCollectionsRasterBatchDeleteRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(id, content);
initialize(result);
return result;
}
public class BatchDelete extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/rasters/batchDelete";
/**
* Remove rasters from an existing raster collection.
*
* Up to 50 rasters can be included in a single batchDelete request. Each batchDelete request is
* atomic.
*
* Create a request for the method "rasters.batchDelete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection to which these rasters belong.
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollectionsRasterBatchDeleteRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String id, com.google.api.services.mapsengine.model.RasterCollectionsRasterBatchDeleteRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.RasterCollectionsRastersBatchDeleteResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/** The ID of the raster collection to which these rasters belong. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection to which these rasters belong.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection to which these rasters belong. */
public BatchDelete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Add rasters to an existing raster collection. Rasters must be successfully processed in order to
* be added to a raster collection.
*
* Up to 50 rasters can be included in a single batchInsert request. Each batchInsert request is
* atomic.
*
* Create a request for the method "rasters.batchInsert".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchInsert#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection to which these rasters belong.
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollectionsRastersBatchInsertRequest}
* @return the request
*/
public BatchInsert batchInsert(java.lang.String id, com.google.api.services.mapsengine.model.RasterCollectionsRastersBatchInsertRequest content) throws java.io.IOException {
BatchInsert result = new BatchInsert(id, content);
initialize(result);
return result;
}
public class BatchInsert extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/rasters/batchInsert";
/**
* Add rasters to an existing raster collection. Rasters must be successfully processed in order
* to be added to a raster collection.
*
* Up to 50 rasters can be included in a single batchInsert request. Each batchInsert request is
* atomic.
*
* Create a request for the method "rasters.batchInsert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchInsert#execute()} method to invoke the remote
* operation. {@link
* BatchInsert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection to which these rasters belong.
* @param content the {@link com.google.api.services.mapsengine.model.RasterCollectionsRastersBatchInsertRequest}
* @since 1.13
*/
protected BatchInsert(java.lang.String id, com.google.api.services.mapsengine.model.RasterCollectionsRastersBatchInsertRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.RasterCollectionsRastersBatchInsertResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchInsert setAlt(java.lang.String alt) {
return (BatchInsert) super.setAlt(alt);
}
@Override
public BatchInsert setFields(java.lang.String fields) {
return (BatchInsert) super.setFields(fields);
}
@Override
public BatchInsert setKey(java.lang.String key) {
return (BatchInsert) super.setKey(key);
}
@Override
public BatchInsert setOauthToken(java.lang.String oauthToken) {
return (BatchInsert) super.setOauthToken(oauthToken);
}
@Override
public BatchInsert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchInsert) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchInsert setQuotaUser(java.lang.String quotaUser) {
return (BatchInsert) super.setQuotaUser(quotaUser);
}
@Override
public BatchInsert setUserIp(java.lang.String userIp) {
return (BatchInsert) super.setUserIp(userIp);
}
/** The ID of the raster collection to which these rasters belong. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection to which these rasters belong.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection to which these rasters belong. */
public BatchInsert setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchInsert set(String parameterName, Object value) {
return (BatchInsert) super.set(parameterName, value);
}
}
/**
* Return all rasters within a raster collection.
*
* Create a request for the method "rasters.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster collection to which these rasters belong.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "rasterCollections/{id}/rasters";
/**
* Return all rasters within a raster collection.
*
* Create a request for the method "rasters.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster collection to which these rasters belong.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.RasterCollectionsRastersListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the raster collection to which these rasters belong. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster collection to which these rasters belong.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster collection to which these rasters belong. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or after this time.
*/
public com.google.api.client.util.DateTime getModifiedAfter() {
return modifiedAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
public List setModifiedAfter(com.google.api.client.util.DateTime modifiedAfter) {
this.modifiedAfter = modifiedAfter;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or after this time.
*/
public com.google.api.client.util.DateTime getCreatedAfter() {
return createdAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
public List setCreatedAfter(com.google.api.client.util.DateTime createdAfter) {
this.createdAfter = createdAfter;
return this;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
@com.google.api.client.util.Key
private java.lang.String tags;
/** A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public java.lang.String getTags() {
return tags;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public List setTags(java.lang.String tags) {
this.tags = tags;
return this;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
@com.google.api.client.util.Key
private java.lang.String search;
/** An unstructured search string used to filter the set of results based on asset metadata.
*/
public java.lang.String getSearch() {
return search;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
public List setSearch(java.lang.String search) {
this.search = search;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/** An email address representing a user. Returned assets that have been created by the user associated
with the provided email address.
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
public List setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String bbox;
/** A bounding box, expressed as "west,south,east,north". If set, only assets which intersect this
bounding box will be returned.
*/
public java.lang.String getBbox() {
return bbox;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
public List setBbox(java.lang.String bbox) {
this.bbox = bbox;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or before this time.
*/
public com.google.api.client.util.DateTime getModifiedBefore() {
return modifiedBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
public List setModifiedBefore(com.google.api.client.util.DateTime modifiedBefore) {
this.modifiedBefore = modifiedBefore;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or before this time.
*/
public com.google.api.client.util.DateTime getCreatedBefore() {
return createdBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
public List setCreatedBefore(com.google.api.client.util.DateTime createdBefore) {
this.createdBefore = createdBefore;
return this;
}
/**
* The role parameter indicates that the response should only contain assets where the
* current user has the specified level of access.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/** The role parameter indicates that the response should only contain assets where the current user
has the specified level of access.
*/
public java.lang.String getRole() {
return role;
}
/**
* The role parameter indicates that the response should only contain assets where the
* current user has the specified level of access.
*/
public List setRole(java.lang.String role) {
this.role = role;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Rasters collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Rasters.List request = mapsengine.rasters().list(parameters ...)}
*
*
* @return the resource collection
*/
public Rasters rasters() {
return new Rasters();
}
/**
* The "rasters" collection of methods.
*/
public class Rasters {
/**
* Delete a raster.
*
* Create a request for the method "rasters.delete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster. Only the raster creator or project owner are permitted to delete. If the
* raster is included in a layer or mosaic, the request will fail. Remove it from all parents
* prior to deleting.
* @return the request
*/
public Delete delete(java.lang.String id) throws java.io.IOException {
Delete result = new Delete(id);
initialize(result);
return result;
}
public class Delete extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}";
/**
* Delete a raster.
*
* Create a request for the method "rasters.delete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster. Only the raster creator or project owner are permitted to delete. If the
* raster is included in a layer or mosaic, the request will fail. Remove it from all parents
* prior to deleting.
* @since 1.13
*/
protected Delete(java.lang.String id) {
super(MapsEngine.this, "DELETE", REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the raster. Only the raster creator or project owner are permitted to delete. If
* the raster is included in a layer or mosaic, the request will fail. Remove it from all
* parents prior to deleting.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster. Only the raster creator or project owner are permitted to delete. If the
raster is included in a layer or mosaic, the request will fail. Remove it from all parents prior to
deleting.
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the raster. Only the raster creator or project owner are permitted to delete. If
* the raster is included in a layer or mosaic, the request will fail. Remove it from all
* parents prior to deleting.
*/
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Return metadata for a single raster.
*
* Create a request for the method "rasters.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster.
* @return the request
*/
public Get get(java.lang.String id) throws java.io.IOException {
Get result = new Get(id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}";
/**
* Return metadata for a single raster.
*
* Create a request for the method "rasters.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster.
* @since 1.13
*/
protected Get(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.Raster.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the raster. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return all rasters readable by the current user.
*
* Create a request for the method "rasters.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId The ID of a Maps Engine project, used to filter the response. To list all available projects with
* their IDs, send a Projects: list request. You can also find your project ID as the value
* of the DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
* @return the request
*/
public List list(java.lang.String projectId) throws java.io.IOException {
List result = new List(projectId);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "rasters";
/**
* Return all rasters readable by the current user.
*
* Create a request for the method "rasters.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The ID of a Maps Engine project, used to filter the response. To list all available projects with
* their IDs, send a Projects: list request. You can also find your project ID as the value
* of the DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
* @since 1.13
*/
protected List(java.lang.String projectId) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.RastersListResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or after this time.
*/
public com.google.api.client.util.DateTime getModifiedAfter() {
return modifiedAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
public List setModifiedAfter(com.google.api.client.util.DateTime modifiedAfter) {
this.modifiedAfter = modifiedAfter;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or after this time.
*/
public com.google.api.client.util.DateTime getCreatedAfter() {
return createdAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
public List setCreatedAfter(com.google.api.client.util.DateTime createdAfter) {
this.createdAfter = createdAfter;
return this;
}
@com.google.api.client.util.Key
private java.lang.String processingStatus;
/**
*/
public java.lang.String getProcessingStatus() {
return processingStatus;
}
public List setProcessingStatus(java.lang.String processingStatus) {
this.processingStatus = processingStatus;
return this;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
@com.google.api.client.util.Key
private java.lang.String tags;
/** A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public java.lang.String getTags() {
return tags;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public List setTags(java.lang.String tags) {
this.tags = tags;
return this;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
@com.google.api.client.util.Key
private java.lang.String search;
/** An unstructured search string used to filter the set of results based on asset metadata.
*/
public java.lang.String getSearch() {
return search;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
public List setSearch(java.lang.String search) {
this.search = search;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/** An email address representing a user. Returned assets that have been created by the user associated
with the provided email address.
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
public List setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String bbox;
/** A bounding box, expressed as "west,south,east,north". If set, only assets which intersect this
bounding box will be returned.
*/
public java.lang.String getBbox() {
return bbox;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
public List setBbox(java.lang.String bbox) {
this.bbox = bbox;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or before this time.
*/
public com.google.api.client.util.DateTime getModifiedBefore() {
return modifiedBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
public List setModifiedBefore(com.google.api.client.util.DateTime modifiedBefore) {
this.modifiedBefore = modifiedBefore;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or before this time.
*/
public com.google.api.client.util.DateTime getCreatedBefore() {
return createdBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
public List setCreatedBefore(com.google.api.client.util.DateTime createdBefore) {
this.createdBefore = createdBefore;
return this;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/** The role parameter indicates that the response should only contain assets where the current user
has the specified level of access.
*/
public java.lang.String getRole() {
return role;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
public List setRole(java.lang.String role) {
this.role = role;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Mutate a raster asset.
*
* Create a request for the method "rasters.patch".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster.
* @param content the {@link com.google.api.services.mapsengine.model.Raster}
* @return the request
*/
public Patch patch(java.lang.String id, com.google.api.services.mapsengine.model.Raster content) throws java.io.IOException {
Patch result = new Patch(id, content);
initialize(result);
return result;
}
public class Patch extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}";
/**
* Mutate a raster asset.
*
* Create a request for the method "rasters.patch".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster.
* @param content the {@link com.google.api.services.mapsengine.model.Raster}
* @since 1.13
*/
protected Patch(java.lang.String id, com.google.api.services.mapsengine.model.Raster content) {
super(MapsEngine.this, "PATCH", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** The ID of the raster. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster. */
public Patch setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Process a raster asset.
*
* Create a request for the method "rasters.process".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Process#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster.
* @return the request
*/
public Process process(java.lang.String id) throws java.io.IOException {
Process result = new Process(id);
initialize(result);
return result;
}
public class Process extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}/process";
/**
* Process a raster asset.
*
* Create a request for the method "rasters.process".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Process#execute()} method to invoke the remote operation.
* {@link
* Process#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster.
* @since 1.13
*/
protected Process(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.ProcessResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Process setAlt(java.lang.String alt) {
return (Process) super.setAlt(alt);
}
@Override
public Process setFields(java.lang.String fields) {
return (Process) super.setFields(fields);
}
@Override
public Process setKey(java.lang.String key) {
return (Process) super.setKey(key);
}
@Override
public Process setOauthToken(java.lang.String oauthToken) {
return (Process) super.setOauthToken(oauthToken);
}
@Override
public Process setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Process) super.setPrettyPrint(prettyPrint);
}
@Override
public Process setQuotaUser(java.lang.String quotaUser) {
return (Process) super.setQuotaUser(quotaUser);
}
@Override
public Process setUserIp(java.lang.String userIp) {
return (Process) super.setUserIp(userIp);
}
/** The ID of the raster. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster. */
public Process setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Process set(String parameterName, Object value) {
return (Process) super.set(parameterName, value);
}
}
/**
* Create a skeleton raster asset for upload.
*
* Create a request for the method "rasters.upload".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.mapsengine.model.Raster}
* @return the request
*/
public Upload upload(com.google.api.services.mapsengine.model.Raster content) throws java.io.IOException {
Upload result = new Upload(content);
initialize(result);
return result;
}
public class Upload extends MapsEngineRequest {
private static final String REST_PATH = "rasters/upload";
/**
* Create a skeleton raster asset for upload.
*
* Create a request for the method "rasters.upload".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.mapsengine.model.Raster}
* @since 1.13
*/
protected Upload(com.google.api.services.mapsengine.model.Raster content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.Raster.class);
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Raster.getName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getProjectId(), "Raster.getProjectId()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getRasterType(), "Raster.getRasterType()");
}
@Override
public Upload setAlt(java.lang.String alt) {
return (Upload) super.setAlt(alt);
}
@Override
public Upload setFields(java.lang.String fields) {
return (Upload) super.setFields(fields);
}
@Override
public Upload setKey(java.lang.String key) {
return (Upload) super.setKey(key);
}
@Override
public Upload setOauthToken(java.lang.String oauthToken) {
return (Upload) super.setOauthToken(oauthToken);
}
@Override
public Upload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Upload) super.setPrettyPrint(prettyPrint);
}
@Override
public Upload setQuotaUser(java.lang.String quotaUser) {
return (Upload) super.setQuotaUser(quotaUser);
}
@Override
public Upload setUserIp(java.lang.String userIp) {
return (Upload) super.setUserIp(userIp);
}
@Override
public Upload set(String parameterName, Object value) {
return (Upload) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Files collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Files.List request = mapsengine.files().list(parameters ...)}
*
*
* @return the resource collection
*/
public Files files() {
return new Files();
}
/**
* The "files" collection of methods.
*/
public class Files {
/**
* Upload a file to a raster asset.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param id The ID of the raster asset.
* @param filename The file name of this uploaded file.
* @return the request
*/
public Insert insert(java.lang.String id, java.lang.String filename) throws java.io.IOException {
Insert result = new Insert(id, filename);
initialize(result);
return result;
}
/**
* Upload a file to a raster asset.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param id The ID of the raster asset.@param filename The file name of this uploaded file.
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Insert insert(java.lang.String id, java.lang.String filename, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Insert result = new Insert(id, filename, mediaContent);
initialize(result);
return result;
}
public class Insert extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}/files";
/**
* Upload a file to a raster asset.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the raster asset.
* @param filename The file name of this uploaded file.
* @since 1.13
*/
protected Insert(java.lang.String id, java.lang.String filename) {
super(MapsEngine.this, "POST", REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
this.filename = com.google.api.client.util.Preconditions.checkNotNull(filename, "Required parameter filename must be specified.");
}
/**
* Upload a file to a raster asset.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param id The ID of the raster asset.@param filename The file name of this uploaded file.
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Insert(java.lang.String id, java.lang.String filename, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(MapsEngine.this, "POST", "/upload/" + getServicePath() + REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
this.filename = com.google.api.client.util.Preconditions.checkNotNull(filename, "Required parameter filename must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the raster asset. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the raster asset.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the raster asset. */
public Insert setId(java.lang.String id) {
this.id = id;
return this;
}
/** The file name of this uploaded file. */
@com.google.api.client.util.Key
private java.lang.String filename;
/** The file name of this uploaded file.
*/
public java.lang.String getFilename() {
return filename;
}
/** The file name of this uploaded file. */
public Insert setFilename(java.lang.String filename) {
this.filename = filename;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Parents collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Parents.List request = mapsengine.parents().list(parameters ...)}
*
*
* @return the resource collection
*/
public Parents parents() {
return new Parents();
}
/**
* The "parents" collection of methods.
*/
public class Parents {
/**
* Return all parent ids of the specified rasters.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the rasters whose parents will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}/parents";
/**
* Return all parent ids of the specified rasters.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the rasters whose parents will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.ParentsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the rasters whose parents will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the rasters whose parents will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the rasters whose parents will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
50.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Permissions collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Permissions.List request = mapsengine.permissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Permissions permissions() {
return new Permissions();
}
/**
* The "permissions" collection of methods.
*/
public class Permissions {
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(id, content);
initialize(result);
return result;
}
public class BatchDelete extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}/permissions/batchDelete";
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchDeleteResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/** The ID of the asset from which permissions will be removed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset from which permissions will be removed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset from which permissions will be removed. */
public BatchDelete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @return the request
*/
public BatchUpdate batchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(id, content);
initialize(result);
return result;
}
public class BatchUpdate extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}/permissions/batchUpdate";
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchUpdate#execute()} method to invoke the remote
* operation. {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @since 1.13
*/
protected BatchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchUpdateResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUserIp(java.lang.String userIp) {
return (BatchUpdate) super.setUserIp(userIp);
}
/** The ID of the asset to which permissions will be added. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset to which permissions will be added.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset to which permissions will be added. */
public BatchUpdate setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset whose permissions will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "rasters/{id}/permissions";
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset whose permissions will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PermissionsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the asset whose permissions will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset whose permissions will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset whose permissions will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Tables collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Tables.List request = mapsengine.tables().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tables tables() {
return new Tables();
}
/**
* The "tables" collection of methods.
*/
public class Tables {
/**
* Create a table asset.
*
* Create a request for the method "tables.create".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.mapsengine.model.Table}
* @return the request
*/
public Create create(com.google.api.services.mapsengine.model.Table content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends MapsEngineRequest {
private static final String REST_PATH = "tables";
/**
* Create a table asset.
*
* Create a request for the method "tables.create".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.mapsengine.model.Table}
* @since 1.13
*/
protected Create(com.google.api.services.mapsengine.model.Table content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.Table.class);
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Table.getName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getProjectId(), "Table.getProjectId()");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete a table.
*
* Create a request for the method "tables.delete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param id The ID of the table. Only the table creator or project owner are permitted to delete. If the table
* is included in a layer, the request will fail. Remove it from all layers prior to
* deleting.
* @return the request
*/
public Delete delete(java.lang.String id) throws java.io.IOException {
Delete result = new Delete(id);
initialize(result);
return result;
}
public class Delete extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}";
/**
* Delete a table.
*
* Create a request for the method "tables.delete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table. Only the table creator or project owner are permitted to delete. If the table
* is included in a layer, the request will fail. Remove it from all layers prior to
* deleting.
* @since 1.13
*/
protected Delete(java.lang.String id) {
super(MapsEngine.this, "DELETE", REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The ID of the table. Only the table creator or project owner are permitted to delete. If
* the table is included in a layer, the request will fail. Remove it from all layers prior to
* deleting.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table. Only the table creator or project owner are permitted to delete. If the table
is included in a layer, the request will fail. Remove it from all layers prior to deleting.
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the table. Only the table creator or project owner are permitted to delete. If
* the table is included in a layer, the request will fail. Remove it from all layers prior to
* deleting.
*/
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Return metadata for a particular table, including the schema.
*
* Create a request for the method "tables.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param id The ID of the table.
* @return the request
*/
public Get get(java.lang.String id) throws java.io.IOException {
Get result = new Get(id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}";
/**
* Return metadata for a particular table, including the schema.
*
* Create a request for the method "tables.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table.
* @since 1.13
*/
protected Get(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.Table.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the table. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@com.google.api.client.util.Key
private java.lang.String version;
/**
*/
public java.lang.String getVersion() {
return version;
}
public Get setVersion(java.lang.String version) {
this.version = version;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return all tables readable by the current user.
*
* Create a request for the method "tables.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "tables";
/**
* Return all tables readable by the current user.
*
* Create a request for the method "tables.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.TablesListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or after this time.
*/
public com.google.api.client.util.DateTime getModifiedAfter() {
return modifiedAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or after this time.
*/
public List setModifiedAfter(com.google.api.client.util.DateTime modifiedAfter) {
this.modifiedAfter = modifiedAfter;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdAfter;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or after this time.
*/
public com.google.api.client.util.DateTime getCreatedAfter() {
return createdAfter;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or after this time.
*/
public List setCreatedAfter(com.google.api.client.util.DateTime createdAfter) {
this.createdAfter = createdAfter;
return this;
}
@com.google.api.client.util.Key
private java.lang.String processingStatus;
/**
*/
public java.lang.String getProcessingStatus() {
return processingStatus;
}
public List setProcessingStatus(java.lang.String processingStatus) {
this.processingStatus = processingStatus;
return this;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of a Maps Engine project, used to filter the response. To list all available projects with
their IDs, send a Projects: list request. You can also find your project ID as the value of the
DashboardPlace:cid URL parameter when signed in to mapsengine.google.com.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of a Maps Engine project, used to filter the response. To list all available
* projects with their IDs, send a Projects: list request. You can also find your project ID
* as the value of the DashboardPlace:cid URL parameter when signed in to
* mapsengine.google.com.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
@com.google.api.client.util.Key
private java.lang.String tags;
/** A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public java.lang.String getTags() {
return tags;
}
/**
* A comma separated list of tags. Returned assets will contain all the tags from the list.
*/
public List setTags(java.lang.String tags) {
this.tags = tags;
return this;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
@com.google.api.client.util.Key
private java.lang.String search;
/** An unstructured search string used to filter the set of results based on asset metadata.
*/
public java.lang.String getSearch() {
return search;
}
/**
* An unstructured search string used to filter the set of results based on asset metadata.
*/
public List setSearch(java.lang.String search) {
this.search = search;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
100.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 100.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/** An email address representing a user. Returned assets that have been created by the user associated
with the provided email address.
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* An email address representing a user. Returned assets that have been created by the user
* associated with the provided email address.
*/
public List setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String bbox;
/** A bounding box, expressed as "west,south,east,north". If set, only assets which intersect this
bounding box will be returned.
*/
public java.lang.String getBbox() {
return bbox;
}
/**
* A bounding box, expressed as "west,south,east,north". If set, only assets which intersect
* this bounding box will be returned.
*/
public List setBbox(java.lang.String bbox) {
this.bbox = bbox;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifiedBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
modified at or before this time.
*/
public com.google.api.client.util.DateTime getModifiedBefore() {
return modifiedBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been modified at or before this time.
*/
public List setModifiedBefore(com.google.api.client.util.DateTime modifiedBefore) {
this.modifiedBefore = modifiedBefore;
return this;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createdBefore;
/** An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will have been
created at or before this time.
*/
public com.google.api.client.util.DateTime getCreatedBefore() {
return createdBefore;
}
/**
* An RFC 3339 formatted date-time value (e.g. 1970-01-01T00:00:00Z). Returned assets will
* have been created at or before this time.
*/
public List setCreatedBefore(com.google.api.client.util.DateTime createdBefore) {
this.createdBefore = createdBefore;
return this;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/** The role parameter indicates that the response should only contain assets where the current user
has the specified level of access.
*/
public java.lang.String getRole() {
return role;
}
/**
* The role parameter indicates that the response should only contain assets where the current
* user has the specified level of access.
*/
public List setRole(java.lang.String role) {
this.role = role;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Mutate a table asset.
*
* Create a request for the method "tables.patch".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param id The ID of the table.
* @param content the {@link com.google.api.services.mapsengine.model.Table}
* @return the request
*/
public Patch patch(java.lang.String id, com.google.api.services.mapsengine.model.Table content) throws java.io.IOException {
Patch result = new Patch(id, content);
initialize(result);
return result;
}
public class Patch extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}";
/**
* Mutate a table asset.
*
* Create a request for the method "tables.patch".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table.
* @param content the {@link com.google.api.services.mapsengine.model.Table}
* @since 1.13
*/
protected Patch(java.lang.String id, com.google.api.services.mapsengine.model.Table content) {
super(MapsEngine.this, "PATCH", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** The ID of the table. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table. */
public Patch setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Process a table asset.
*
* Create a request for the method "tables.process".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Process#execute()} method to invoke the remote operation.
*
* @param id The ID of the table.
* @return the request
*/
public Process process(java.lang.String id) throws java.io.IOException {
Process result = new Process(id);
initialize(result);
return result;
}
public class Process extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/process";
/**
* Process a table asset.
*
* Create a request for the method "tables.process".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Process#execute()} method to invoke the remote operation.
* {@link
* Process#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table.
* @since 1.13
*/
protected Process(java.lang.String id) {
super(MapsEngine.this, "POST", REST_PATH, null, com.google.api.services.mapsengine.model.ProcessResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Process setAlt(java.lang.String alt) {
return (Process) super.setAlt(alt);
}
@Override
public Process setFields(java.lang.String fields) {
return (Process) super.setFields(fields);
}
@Override
public Process setKey(java.lang.String key) {
return (Process) super.setKey(key);
}
@Override
public Process setOauthToken(java.lang.String oauthToken) {
return (Process) super.setOauthToken(oauthToken);
}
@Override
public Process setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Process) super.setPrettyPrint(prettyPrint);
}
@Override
public Process setQuotaUser(java.lang.String quotaUser) {
return (Process) super.setQuotaUser(quotaUser);
}
@Override
public Process setUserIp(java.lang.String userIp) {
return (Process) super.setUserIp(userIp);
}
/** The ID of the table. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table. */
public Process setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Process set(String parameterName, Object value) {
return (Process) super.set(parameterName, value);
}
}
/**
* Create a placeholder table asset to which table files can be uploaded. Once the placeholder has
* been created, files are uploaded to the
* https://www.googleapis.com/upload/mapsengine/v1/tables/table_id/files endpoint. See Table Upload
* in the Developer's Guide or Table.files: insert in the reference documentation for more
* information.
*
* Create a request for the method "tables.upload".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.mapsengine.model.Table}
* @return the request
*/
public Upload upload(com.google.api.services.mapsengine.model.Table content) throws java.io.IOException {
Upload result = new Upload(content);
initialize(result);
return result;
}
public class Upload extends MapsEngineRequest {
private static final String REST_PATH = "tables/upload";
/**
* Create a placeholder table asset to which table files can be uploaded. Once the placeholder has
* been created, files are uploaded to the
* https://www.googleapis.com/upload/mapsengine/v1/tables/table_id/files endpoint. See Table
* Upload in the Developer's Guide or Table.files: insert in the reference documentation for more
* information.
*
* Create a request for the method "tables.upload".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.mapsengine.model.Table}
* @since 1.13
*/
protected Upload(com.google.api.services.mapsengine.model.Table content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.Table.class);
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Table.getName()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getProjectId(), "Table.getProjectId()");
}
@Override
public Upload setAlt(java.lang.String alt) {
return (Upload) super.setAlt(alt);
}
@Override
public Upload setFields(java.lang.String fields) {
return (Upload) super.setFields(fields);
}
@Override
public Upload setKey(java.lang.String key) {
return (Upload) super.setKey(key);
}
@Override
public Upload setOauthToken(java.lang.String oauthToken) {
return (Upload) super.setOauthToken(oauthToken);
}
@Override
public Upload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Upload) super.setPrettyPrint(prettyPrint);
}
@Override
public Upload setQuotaUser(java.lang.String quotaUser) {
return (Upload) super.setQuotaUser(quotaUser);
}
@Override
public Upload setUserIp(java.lang.String userIp) {
return (Upload) super.setUserIp(userIp);
}
@Override
public Upload set(String parameterName, Object value) {
return (Upload) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Features collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Features.List request = mapsengine.features().list(parameters ...)}
*
*
* @return the resource collection
*/
public Features features() {
return new Features();
}
/**
* The "features" collection of methods.
*/
public class Features {
/**
* Delete all features matching the given IDs.
*
* Create a request for the method "features.batchDelete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param id The ID of the table that contains the features to be deleted.
* @param content the {@link com.google.api.services.mapsengine.model.FeaturesBatchDeleteRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String id, com.google.api.services.mapsengine.model.FeaturesBatchDeleteRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(id, content);
initialize(result);
return result;
}
public class BatchDelete extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/features/batchDelete";
/**
* Delete all features matching the given IDs.
*
* Create a request for the method "features.batchDelete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table that contains the features to be deleted.
* @param content the {@link com.google.api.services.mapsengine.model.FeaturesBatchDeleteRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String id, com.google.api.services.mapsengine.model.FeaturesBatchDeleteRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/** The ID of the table that contains the features to be deleted. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table that contains the features to be deleted.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table that contains the features to be deleted. */
public BatchDelete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Append features to an existing table.
*
* A single batchInsert request can create:
*
* - Up to 50 features. - A combined total of 10 000 vertices. Feature limits are documented in the
* Supported data formats and limits article of the Google Maps Engine help center. Note that free
* and paid accounts have different limits.
*
* For more information about inserting features, read Creating features in the Google Maps Engine
* developer's guide.
*
* Create a request for the method "features.batchInsert".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchInsert#execute()} method to invoke the remote operation.
*
* @param id The ID of the table to append the features to.
* @param content the {@link com.google.api.services.mapsengine.model.FeaturesBatchInsertRequest}
* @return the request
*/
public BatchInsert batchInsert(java.lang.String id, com.google.api.services.mapsengine.model.FeaturesBatchInsertRequest content) throws java.io.IOException {
BatchInsert result = new BatchInsert(id, content);
initialize(result);
return result;
}
public class BatchInsert extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/features/batchInsert";
/**
* Append features to an existing table.
*
* A single batchInsert request can create:
*
* - Up to 50 features. - A combined total of 10 000 vertices. Feature limits are documented in
* the Supported data formats and limits article of the Google Maps Engine help center. Note that
* free and paid accounts have different limits.
*
* For more information about inserting features, read Creating features in the Google Maps Engine
* developer's guide.
*
* Create a request for the method "features.batchInsert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchInsert#execute()} method to invoke the remote
* operation. {@link
* BatchInsert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table to append the features to.
* @param content the {@link com.google.api.services.mapsengine.model.FeaturesBatchInsertRequest}
* @since 1.13
*/
protected BatchInsert(java.lang.String id, com.google.api.services.mapsengine.model.FeaturesBatchInsertRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchInsert setAlt(java.lang.String alt) {
return (BatchInsert) super.setAlt(alt);
}
@Override
public BatchInsert setFields(java.lang.String fields) {
return (BatchInsert) super.setFields(fields);
}
@Override
public BatchInsert setKey(java.lang.String key) {
return (BatchInsert) super.setKey(key);
}
@Override
public BatchInsert setOauthToken(java.lang.String oauthToken) {
return (BatchInsert) super.setOauthToken(oauthToken);
}
@Override
public BatchInsert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchInsert) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchInsert setQuotaUser(java.lang.String quotaUser) {
return (BatchInsert) super.setQuotaUser(quotaUser);
}
@Override
public BatchInsert setUserIp(java.lang.String userIp) {
return (BatchInsert) super.setUserIp(userIp);
}
/** The ID of the table to append the features to. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table to append the features to.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table to append the features to. */
public BatchInsert setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchInsert set(String parameterName, Object value) {
return (BatchInsert) super.set(parameterName, value);
}
}
/**
* Update the supplied features.
*
* A single batchPatch request can update:
*
* - Up to 50 features. - A combined total of 10 000 vertices. Feature limits are documented in the
* Supported data formats and limits article of the Google Maps Engine help center. Note that free
* and paid accounts have different limits.
*
* Feature updates use HTTP PATCH semantics:
*
* - A supplied value replaces an existing value (if any) in that field. - Omitted fields remain
* unchanged. - Complex values in geometries and properties must be replaced as atomic units. For
* example, providing just the coordinates of a geometry is not allowed; the complete geometry,
* including type, must be supplied. - Setting a property's value to null deletes that property. For
* more information about updating features, read Updating features in the Google Maps Engine
* developer's guide.
*
* Create a request for the method "features.batchPatch".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchPatch#execute()} method to invoke the remote operation.
*
* @param id The ID of the table containing the features to be patched.
* @param content the {@link com.google.api.services.mapsengine.model.FeaturesBatchPatchRequest}
* @return the request
*/
public BatchPatch batchPatch(java.lang.String id, com.google.api.services.mapsengine.model.FeaturesBatchPatchRequest content) throws java.io.IOException {
BatchPatch result = new BatchPatch(id, content);
initialize(result);
return result;
}
public class BatchPatch extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/features/batchPatch";
/**
* Update the supplied features.
*
* A single batchPatch request can update:
*
* - Up to 50 features. - A combined total of 10 000 vertices. Feature limits are documented in
* the Supported data formats and limits article of the Google Maps Engine help center. Note that
* free and paid accounts have different limits.
*
* Feature updates use HTTP PATCH semantics:
*
* - A supplied value replaces an existing value (if any) in that field. - Omitted fields remain
* unchanged. - Complex values in geometries and properties must be replaced as atomic units. For
* example, providing just the coordinates of a geometry is not allowed; the complete geometry,
* including type, must be supplied. - Setting a property's value to null deletes that property.
* For more information about updating features, read Updating features in the Google Maps Engine
* developer's guide.
*
* Create a request for the method "features.batchPatch".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchPatch#execute()} method to invoke the remote
* operation. {@link
* BatchPatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table containing the features to be patched.
* @param content the {@link com.google.api.services.mapsengine.model.FeaturesBatchPatchRequest}
* @since 1.13
*/
protected BatchPatch(java.lang.String id, com.google.api.services.mapsengine.model.FeaturesBatchPatchRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchPatch setAlt(java.lang.String alt) {
return (BatchPatch) super.setAlt(alt);
}
@Override
public BatchPatch setFields(java.lang.String fields) {
return (BatchPatch) super.setFields(fields);
}
@Override
public BatchPatch setKey(java.lang.String key) {
return (BatchPatch) super.setKey(key);
}
@Override
public BatchPatch setOauthToken(java.lang.String oauthToken) {
return (BatchPatch) super.setOauthToken(oauthToken);
}
@Override
public BatchPatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchPatch) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchPatch setQuotaUser(java.lang.String quotaUser) {
return (BatchPatch) super.setQuotaUser(quotaUser);
}
@Override
public BatchPatch setUserIp(java.lang.String userIp) {
return (BatchPatch) super.setUserIp(userIp);
}
/** The ID of the table containing the features to be patched. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table containing the features to be patched.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table containing the features to be patched. */
public BatchPatch setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchPatch set(String parameterName, Object value) {
return (BatchPatch) super.set(parameterName, value);
}
}
/**
* Return a single feature, given its ID.
*
* Create a request for the method "features.get".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param tableId The ID of the table.
* @param id The ID of the feature to get.
* @return the request
*/
public Get get(java.lang.String tableId, java.lang.String id) throws java.io.IOException {
Get result = new Get(tableId, id);
initialize(result);
return result;
}
public class Get extends MapsEngineRequest {
private static final String REST_PATH = "tables/{tableId}/features/{id}";
/**
* Return a single feature, given its ID.
*
* Create a request for the method "features.get".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param tableId The ID of the table.
* @param id The ID of the feature to get.
* @since 1.13
*/
protected Get(java.lang.String tableId, java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.Feature.class);
this.tableId = com.google.api.client.util.Preconditions.checkNotNull(tableId, "Required parameter tableId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the table. */
@com.google.api.client.util.Key
private java.lang.String tableId;
/** The ID of the table.
*/
public java.lang.String getTableId() {
return tableId;
}
/** The ID of the table. */
public Get setTableId(java.lang.String tableId) {
this.tableId = tableId;
return this;
}
/** The ID of the feature to get. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the feature to get.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the feature to get. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
/** The table version to access. See Accessing Public Data for information. */
@com.google.api.client.util.Key
private java.lang.String version;
/** The table version to access. See Accessing Public Data for information.
*/
public java.lang.String getVersion() {
return version;
}
/** The table version to access. See Accessing Public Data for information. */
public Get setVersion(java.lang.String version) {
this.version = version;
return this;
}
/**
* A SQL-like projection clause used to specify returned properties. If this parameter is
* not included, all properties are returned.
*/
@com.google.api.client.util.Key
private java.lang.String select;
/** A SQL-like projection clause used to specify returned properties. If this parameter is not
included, all properties are returned.
*/
public java.lang.String getSelect() {
return select;
}
/**
* A SQL-like projection clause used to specify returned properties. If this parameter is
* not included, all properties are returned.
*/
public Get setSelect(java.lang.String select) {
this.select = select;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Return all features readable by the current user.
*
* Create a request for the method "features.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the table to which these features belong.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/features";
/**
* Return all features readable by the current user.
*
* Create a request for the method "features.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table to which these features belong.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.FeaturesListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the table to which these features belong. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table to which these features belong.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table to which these features belong. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* An SQL-like order by clause used to sort results. If this parameter is not included, the
* order of features is undefined.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** An SQL-like order by clause used to sort results. If this parameter is not included, the order of
features is undefined.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* An SQL-like order by clause used to sort results. If this parameter is not included, the
* order of features is undefined.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** A geometry literal that specifies the spatial restriction of the query. */
@com.google.api.client.util.Key
private java.lang.String intersects;
/** A geometry literal that specifies the spatial restriction of the query.
*/
public java.lang.String getIntersects() {
return intersects;
}
/** A geometry literal that specifies the spatial restriction of the query. */
public List setIntersects(java.lang.String intersects) {
this.intersects = intersects;
return this;
}
/**
* The maximum number of items to include in the response, used for paging. The maximum
* supported value is 1000.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in the response, used for paging. The maximum supported
value is 1000.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in the response, used for paging. The maximum
* supported value is 1000.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The table version to access. See Accessing Public Data for information. */
@com.google.api.client.util.Key
private java.lang.String version;
/** The table version to access. See Accessing Public Data for information.
*/
public java.lang.String getVersion() {
return version;
}
/** The table version to access. See Accessing Public Data for information. */
public List setVersion(java.lang.String version) {
this.version = version;
return this;
}
/**
* The total number of features to return from the query, irrespective of the number of
* pages.
*/
@com.google.api.client.util.Key
private java.lang.Long limit;
/** The total number of features to return from the query, irrespective of the number of pages.
*/
public java.lang.Long getLimit() {
return limit;
}
/**
* The total number of features to return from the query, irrespective of the number of
* pages.
*/
public List setLimit(java.lang.Long limit) {
this.limit = limit;
return this;
}
/** A comma separated list of optional data to include. Optional data available: schema. */
@com.google.api.client.util.Key
private java.lang.String include;
/** A comma separated list of optional data to include. Optional data available: schema.
*/
public java.lang.String getInclude() {
return include;
}
/** A comma separated list of optional data to include. Optional data available: schema. */
public List setInclude(java.lang.String include) {
this.include = include;
return this;
}
/** An SQL-like predicate used to filter results. */
@com.google.api.client.util.Key
private java.lang.String where;
/** An SQL-like predicate used to filter results.
*/
public java.lang.String getWhere() {
return where;
}
/** An SQL-like predicate used to filter results. */
public List setWhere(java.lang.String where) {
this.where = where;
return this;
}
/**
* A SQL-like projection clause used to specify returned properties. If this parameter is
* not included, all properties are returned.
*/
@com.google.api.client.util.Key
private java.lang.String select;
/** A SQL-like projection clause used to specify returned properties. If this parameter is not
included, all properties are returned.
*/
public java.lang.String getSelect() {
return select;
}
/**
* A SQL-like projection clause used to specify returned properties. If this parameter is
* not included, all properties are returned.
*/
public List setSelect(java.lang.String select) {
this.select = select;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Files collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Files.List request = mapsengine.files().list(parameters ...)}
*
*
* @return the resource collection
*/
public Files files() {
return new Files();
}
/**
* The "files" collection of methods.
*/
public class Files {
/**
* Upload a file to a placeholder table asset. See Table Upload in the Developer's Guide for more
* information. Supported file types are listed in the Supported data formats and limits article of
* the Google Maps Engine help center.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param id The ID of the table asset.
* @param filename The file name of this uploaded file.
* @return the request
*/
public Insert insert(java.lang.String id, java.lang.String filename) throws java.io.IOException {
Insert result = new Insert(id, filename);
initialize(result);
return result;
}
/**
* Upload a file to a placeholder table asset. See Table Upload in the Developer's Guide for more
* information. Supported file types are listed in the Supported data formats and limits article of
* the Google Maps Engine help center.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param id The ID of the table asset.@param filename The file name of this uploaded file.
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Insert insert(java.lang.String id, java.lang.String filename, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Insert result = new Insert(id, filename, mediaContent);
initialize(result);
return result;
}
public class Insert extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/files";
/**
* Upload a file to a placeholder table asset. See Table Upload in the Developer's Guide for more
* information. Supported file types are listed in the Supported data formats and limits article
* of the Google Maps Engine help center.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table asset.
* @param filename The file name of this uploaded file.
* @since 1.13
*/
protected Insert(java.lang.String id, java.lang.String filename) {
super(MapsEngine.this, "POST", REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
this.filename = com.google.api.client.util.Preconditions.checkNotNull(filename, "Required parameter filename must be specified.");
}
/**
* Upload a file to a placeholder table asset. See Table Upload in the Developer's Guide for more
* information. Supported file types are listed in the Supported data formats and limits article
* of the Google Maps Engine help center.
*
* Create a request for the method "files.insert".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param id The ID of the table asset.@param filename The file name of this uploaded file.
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Insert(java.lang.String id, java.lang.String filename, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(MapsEngine.this, "POST", "/upload/" + getServicePath() + REST_PATH, null, Void.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
this.filename = com.google.api.client.util.Preconditions.checkNotNull(filename, "Required parameter filename must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the table asset. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table asset.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table asset. */
public Insert setId(java.lang.String id) {
this.id = id;
return this;
}
/** The file name of this uploaded file. */
@com.google.api.client.util.Key
private java.lang.String filename;
/** The file name of this uploaded file.
*/
public java.lang.String getFilename() {
return filename;
}
/** The file name of this uploaded file. */
public Insert setFilename(java.lang.String filename) {
this.filename = filename;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Parents collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Parents.List request = mapsengine.parents().list(parameters ...)}
*
*
* @return the resource collection
*/
public Parents parents() {
return new Parents();
}
/**
* The "parents" collection of methods.
*/
public class Parents {
/**
* Return all parent ids of the specified table.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the table whose parents will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/parents";
/**
* Return all parent ids of the specified table.
*
* Create a request for the method "parents.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the table whose parents will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.ParentsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the table whose parents will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the table whose parents will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the table whose parents will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, used to page through large result sets. To get the next page of results,
set this parameter to the value of nextPageToken from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, used to page through large result sets. To get the next page of
* results, set this parameter to the value of nextPageToken from the previous response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of items to include in a single response page. The maximum supported value is
50.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to include in a single response page. The maximum supported
* value is 50.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Permissions collection.
*
* The typical use is:
*
* {@code MapsEngine mapsengine = new MapsEngine(...);}
* {@code MapsEngine.Permissions.List request = mapsengine.permissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Permissions permissions() {
return new Permissions();
}
/**
* The "permissions" collection of methods.
*/
public class Permissions {
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(id, content);
initialize(result);
return result;
}
public class BatchDelete extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/permissions/batchDelete";
/**
* Remove permission entries from an already existing asset.
*
* Create a request for the method "permissions.batchDelete".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset from which permissions will be removed.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchDeleteRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchDeleteResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/** The ID of the asset from which permissions will be removed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset from which permissions will be removed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset from which permissions will be removed. */
public BatchDelete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @return the request
*/
public BatchUpdate batchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(id, content);
initialize(result);
return result;
}
public class BatchUpdate extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/permissions/batchUpdate";
/**
* Add or update permission entries to an already existing asset.
*
* An asset can hold up to 20 different permission entries. Each batchInsert request is atomic.
*
* Create a request for the method "permissions.batchUpdate".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link BatchUpdate#execute()} method to invoke the remote
* operation. {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset to which permissions will be added.
* @param content the {@link com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest}
* @since 1.13
*/
protected BatchUpdate(java.lang.String id, com.google.api.services.mapsengine.model.PermissionsBatchUpdateRequest content) {
super(MapsEngine.this, "POST", REST_PATH, content, com.google.api.services.mapsengine.model.PermissionsBatchUpdateResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUserIp(java.lang.String userIp) {
return (BatchUpdate) super.setUserIp(userIp);
}
/** The ID of the asset to which permissions will be added. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset to which permissions will be added.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset to which permissions will be added. */
public BatchUpdate setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the mapsengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param id The ID of the asset whose permissions will be listed.
* @return the request
*/
public List list(java.lang.String id) throws java.io.IOException {
List result = new List(id);
initialize(result);
return result;
}
public class List extends MapsEngineRequest {
private static final String REST_PATH = "tables/{id}/permissions";
/**
* Return all of the permissions for the specified asset.
*
* Create a request for the method "permissions.list".
*
* This request holds the parameters needed by the the mapsengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param id The ID of the asset whose permissions will be listed.
* @since 1.13
*/
protected List(java.lang.String id) {
super(MapsEngine.this, "GET", REST_PATH, null, com.google.api.services.mapsengine.model.PermissionsListResponse.class);
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the asset whose permissions will be listed. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the asset whose permissions will be listed.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the asset whose permissions will be listed. */
public List setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link MapsEngine}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link MapsEngine}. */
@Override
public MapsEngine build() {
return new MapsEngine(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link MapsEngineRequestInitializer}.
*
* @since 1.12
*/
public Builder setMapsEngineRequestInitializer(
MapsEngineRequestInitializer mapsengineRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(mapsengineRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}