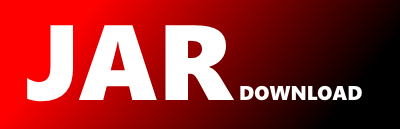
com.google.api.services.mapsengine.model.Asset Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2015-11-16 19:10:01 UTC)
* on 2015-12-05 at 02:54:01 UTC
* Modify at your own risk.
*/
package com.google.api.services.mapsengine.model;
/**
* An asset is any Google Maps Engine resource that has a globally unique ID. Assets include maps,
* layers, vector tables, raster collections, and rasters. Projects and features are not considered
* assets.
*
* More detailed information about an asset can be obtained by querying the asset's particular
* endpoint.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Maps Engine API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Asset extends com.google.api.client.json.GenericJson {
/**
* A rectangular bounding box which contains all of the data in this asset. The box is expressed
* as \"west, south, east, north\". The numbers represent latitude and longitude in decimal
* degrees.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List bbox;
/**
* The creation time of this asset. The value is an RFC 3339-formatted date-time value (for
* example, 1970-01-01T00:00:00Z).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime creationTime;
/**
* The email address of the creator of this asset. This is only returned on GET requests and not
* LIST requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/**
* The asset's description.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The ETag, used to refer to the current version of the asset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* The asset's globally unique ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The last modified time of this asset. The value is an RFC 3339-formatted date-time value (for
* example, 1970-01-01T00:00:00Z).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime lastModifiedTime;
/**
* The email address of the last modifier of this asset. This is only returned on GET requests and
* not LIST requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lastModifierEmail;
/**
* The asset's name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The ID of the project to which the asset belongs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/**
* The URL to query to retrieve the asset's complete object. The assets endpoint only returns
* high-level information about a resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/**
* An array of text strings, with each string representing a tag. More information about tags can
* be found in the Tagging data article of the Maps Engine help center.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tags;
/**
* The type of asset. One of raster, rasterCollection, table, map, or layer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* If true, WRITERs of the asset are able to edit the asset permissions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean writersCanEditPermissions;
/**
* A rectangular bounding box which contains all of the data in this asset. The box is expressed
* as \"west, south, east, north\". The numbers represent latitude and longitude in decimal
* degrees.
* @return value or {@code null} for none
*/
public java.util.List getBbox() {
return bbox;
}
/**
* A rectangular bounding box which contains all of the data in this asset. The box is expressed
* as \"west, south, east, north\". The numbers represent latitude and longitude in decimal
* degrees.
* @param bbox bbox or {@code null} for none
*/
public Asset setBbox(java.util.List bbox) {
this.bbox = bbox;
return this;
}
/**
* The creation time of this asset. The value is an RFC 3339-formatted date-time value (for
* example, 1970-01-01T00:00:00Z).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getCreationTime() {
return creationTime;
}
/**
* The creation time of this asset. The value is an RFC 3339-formatted date-time value (for
* example, 1970-01-01T00:00:00Z).
* @param creationTime creationTime or {@code null} for none
*/
public Asset setCreationTime(com.google.api.client.util.DateTime creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* The email address of the creator of this asset. This is only returned on GET requests and not
* LIST requests.
* @return value or {@code null} for none
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* The email address of the creator of this asset. This is only returned on GET requests and not
* LIST requests.
* @param creatorEmail creatorEmail or {@code null} for none
*/
public Asset setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* The asset's description.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The asset's description.
* @param description description or {@code null} for none
*/
public Asset setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The ETag, used to refer to the current version of the asset.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* The ETag, used to refer to the current version of the asset.
* @param etag etag or {@code null} for none
*/
public Asset setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* The asset's globally unique ID.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The asset's globally unique ID.
* @param id id or {@code null} for none
*/
public Asset setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The last modified time of this asset. The value is an RFC 3339-formatted date-time value (for
* example, 1970-01-01T00:00:00Z).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getLastModifiedTime() {
return lastModifiedTime;
}
/**
* The last modified time of this asset. The value is an RFC 3339-formatted date-time value (for
* example, 1970-01-01T00:00:00Z).
* @param lastModifiedTime lastModifiedTime or {@code null} for none
*/
public Asset setLastModifiedTime(com.google.api.client.util.DateTime lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
return this;
}
/**
* The email address of the last modifier of this asset. This is only returned on GET requests and
* not LIST requests.
* @return value or {@code null} for none
*/
public java.lang.String getLastModifierEmail() {
return lastModifierEmail;
}
/**
* The email address of the last modifier of this asset. This is only returned on GET requests and
* not LIST requests.
* @param lastModifierEmail lastModifierEmail or {@code null} for none
*/
public Asset setLastModifierEmail(java.lang.String lastModifierEmail) {
this.lastModifierEmail = lastModifierEmail;
return this;
}
/**
* The asset's name.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The asset's name.
* @param name name or {@code null} for none
*/
public Asset setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The ID of the project to which the asset belongs.
* @return value or {@code null} for none
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of the project to which the asset belongs.
* @param projectId projectId or {@code null} for none
*/
public Asset setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* The URL to query to retrieve the asset's complete object. The assets endpoint only returns
* high-level information about a resource.
* @return value or {@code null} for none
*/
public java.lang.String getResource() {
return resource;
}
/**
* The URL to query to retrieve the asset's complete object. The assets endpoint only returns
* high-level information about a resource.
* @param resource resource or {@code null} for none
*/
public Asset setResource(java.lang.String resource) {
this.resource = resource;
return this;
}
/**
* An array of text strings, with each string representing a tag. More information about tags can
* be found in the Tagging data article of the Maps Engine help center.
* @return value or {@code null} for none
*/
public java.util.List getTags() {
return tags;
}
/**
* An array of text strings, with each string representing a tag. More information about tags can
* be found in the Tagging data article of the Maps Engine help center.
* @param tags tags or {@code null} for none
*/
public Asset setTags(java.util.List tags) {
this.tags = tags;
return this;
}
/**
* The type of asset. One of raster, rasterCollection, table, map, or layer.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The type of asset. One of raster, rasterCollection, table, map, or layer.
* @param type type or {@code null} for none
*/
public Asset setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* If true, WRITERs of the asset are able to edit the asset permissions.
* @return value or {@code null} for none
*/
public java.lang.Boolean getWritersCanEditPermissions() {
return writersCanEditPermissions;
}
/**
* If true, WRITERs of the asset are able to edit the asset permissions.
* @param writersCanEditPermissions writersCanEditPermissions or {@code null} for none
*/
public Asset setWritersCanEditPermissions(java.lang.Boolean writersCanEditPermissions) {
this.writersCanEditPermissions = writersCanEditPermissions;
return this;
}
@Override
public Asset set(String fieldName, Object value) {
return (Asset) super.set(fieldName, value);
}
@Override
public Asset clone() {
return (Asset) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy