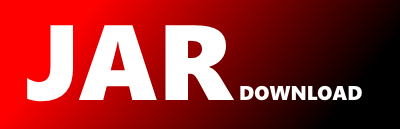
com.google.api.services.mapsengine.model.Map Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2015-11-16 19:10:01 UTC)
* on 2015-12-05 at 02:54:01 UTC
* Modify at your own risk.
*/
package com.google.api.services.mapsengine.model;
/**
* A Map is a collection of Layers, optionally contained within folders.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Maps Engine API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Map extends com.google.api.client.json.GenericJson {
/**
* A rectangular bounding box which contains all of the data in this Map. The box is expressed as
* \"west, south, east, north\". The numbers represent latitude and longitude in decimal degrees.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List bbox;
/**
* The contents of this Map.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List contents;
/**
* The creation time of this map. The value is an RFC 3339 formatted date-time value (e.g.
* 1970-01-01T00:00:00Z).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime creationTime;
/**
* The email address of the creator of this map. This is only returned on GET requests and not
* LIST requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creatorEmail;
/**
* An array of four numbers (west, south, east, north) which defines the rectangular bounding box
* of the default viewport. The numbers represent latitude and longitude in decimal degrees.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List defaultViewport;
/**
* The description of this Map, supplied by the author.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Deprecated: The name of an access list of the Map Editor type. The user on whose behalf the
* request is being sent must be an editor on that access list. Note: Google Maps Engine no longer
* uses access lists. Instead, each asset has its own list of permissions. For backward
* compatibility, the API still accepts access lists for projects that are already using access
* lists. If you created a GME account/project after July 14th, 2014, you will not be able to send
* API requests that include access lists. Note: This is an input field only. It is not returned
* in response to a list or get request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String draftAccessList;
/**
* The ETag, used to refer to the current version of the asset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* A globally unique ID, used to refer to this Map.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The last modified time of this map. The value is an RFC 3339 formatted date-time value (e.g.
* 1970-01-01T00:00:00Z).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime lastModifiedTime;
/**
* The email address of the last modifier of this map. This is only returned on GET requests and
* not LIST requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lastModifierEmail;
/**
* The name of this Map, supplied by the author.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The processing status of this map. Map processing is automatically started once a map becomes
* ready for processing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String processingStatus;
/**
* The ID of the project that this Map is in.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/**
* Deprecated: The access list to whom view permissions are granted. The value must be the name of
* a Maps Engine access list of the Map Viewer type, and the user must be a viewer on that list.
* Note: Google Maps Engine no longer uses access lists. Instead, each asset has its own list of
* permissions. For backward compatibility, the API still accepts access lists for projects that
* are already using access lists. If you created a GME account/project after July 14th, 2014, you
* will not be able to send API requests that include access lists. This is an input field only.
* It is not returned in response to a list or get request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String publishedAccessList;
/**
* The publishing status of this map.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String publishingStatus;
/**
* Tags of this Map.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tags;
/**
* Deprecated: An array containing the available versions of this Map. Currently may only contain
* "published". The publishingStatus field should be used instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List versions;
/**
* If true, WRITERs of the asset are able to edit the asset permissions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean writersCanEditPermissions;
/**
* A rectangular bounding box which contains all of the data in this Map. The box is expressed as
* \"west, south, east, north\". The numbers represent latitude and longitude in decimal degrees.
* @return value or {@code null} for none
*/
public java.util.List getBbox() {
return bbox;
}
/**
* A rectangular bounding box which contains all of the data in this Map. The box is expressed as
* \"west, south, east, north\". The numbers represent latitude and longitude in decimal degrees.
* @param bbox bbox or {@code null} for none
*/
public Map setBbox(java.util.List bbox) {
this.bbox = bbox;
return this;
}
/**
* The contents of this Map.
* @return value or {@code null} for none
*/
public java.util.List getContents() {
return contents;
}
/**
* The contents of this Map.
* @param contents contents or {@code null} for none
*/
public Map setContents(java.util.List contents) {
this.contents = contents;
return this;
}
/**
* The creation time of this map. The value is an RFC 3339 formatted date-time value (e.g.
* 1970-01-01T00:00:00Z).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getCreationTime() {
return creationTime;
}
/**
* The creation time of this map. The value is an RFC 3339 formatted date-time value (e.g.
* 1970-01-01T00:00:00Z).
* @param creationTime creationTime or {@code null} for none
*/
public Map setCreationTime(com.google.api.client.util.DateTime creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* The email address of the creator of this map. This is only returned on GET requests and not
* LIST requests.
* @return value or {@code null} for none
*/
public java.lang.String getCreatorEmail() {
return creatorEmail;
}
/**
* The email address of the creator of this map. This is only returned on GET requests and not
* LIST requests.
* @param creatorEmail creatorEmail or {@code null} for none
*/
public Map setCreatorEmail(java.lang.String creatorEmail) {
this.creatorEmail = creatorEmail;
return this;
}
/**
* An array of four numbers (west, south, east, north) which defines the rectangular bounding box
* of the default viewport. The numbers represent latitude and longitude in decimal degrees.
* @return value or {@code null} for none
*/
public java.util.List getDefaultViewport() {
return defaultViewport;
}
/**
* An array of four numbers (west, south, east, north) which defines the rectangular bounding box
* of the default viewport. The numbers represent latitude and longitude in decimal degrees.
* @param defaultViewport defaultViewport or {@code null} for none
*/
public Map setDefaultViewport(java.util.List defaultViewport) {
this.defaultViewport = defaultViewport;
return this;
}
/**
* The description of this Map, supplied by the author.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The description of this Map, supplied by the author.
* @param description description or {@code null} for none
*/
public Map setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Deprecated: The name of an access list of the Map Editor type. The user on whose behalf the
* request is being sent must be an editor on that access list. Note: Google Maps Engine no longer
* uses access lists. Instead, each asset has its own list of permissions. For backward
* compatibility, the API still accepts access lists for projects that are already using access
* lists. If you created a GME account/project after July 14th, 2014, you will not be able to send
* API requests that include access lists. Note: This is an input field only. It is not returned
* in response to a list or get request.
* @return value or {@code null} for none
*/
public java.lang.String getDraftAccessList() {
return draftAccessList;
}
/**
* Deprecated: The name of an access list of the Map Editor type. The user on whose behalf the
* request is being sent must be an editor on that access list. Note: Google Maps Engine no longer
* uses access lists. Instead, each asset has its own list of permissions. For backward
* compatibility, the API still accepts access lists for projects that are already using access
* lists. If you created a GME account/project after July 14th, 2014, you will not be able to send
* API requests that include access lists. Note: This is an input field only. It is not returned
* in response to a list or get request.
* @param draftAccessList draftAccessList or {@code null} for none
*/
public Map setDraftAccessList(java.lang.String draftAccessList) {
this.draftAccessList = draftAccessList;
return this;
}
/**
* The ETag, used to refer to the current version of the asset.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* The ETag, used to refer to the current version of the asset.
* @param etag etag or {@code null} for none
*/
public Map setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* A globally unique ID, used to refer to this Map.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* A globally unique ID, used to refer to this Map.
* @param id id or {@code null} for none
*/
public Map setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The last modified time of this map. The value is an RFC 3339 formatted date-time value (e.g.
* 1970-01-01T00:00:00Z).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getLastModifiedTime() {
return lastModifiedTime;
}
/**
* The last modified time of this map. The value is an RFC 3339 formatted date-time value (e.g.
* 1970-01-01T00:00:00Z).
* @param lastModifiedTime lastModifiedTime or {@code null} for none
*/
public Map setLastModifiedTime(com.google.api.client.util.DateTime lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
return this;
}
/**
* The email address of the last modifier of this map. This is only returned on GET requests and
* not LIST requests.
* @return value or {@code null} for none
*/
public java.lang.String getLastModifierEmail() {
return lastModifierEmail;
}
/**
* The email address of the last modifier of this map. This is only returned on GET requests and
* not LIST requests.
* @param lastModifierEmail lastModifierEmail or {@code null} for none
*/
public Map setLastModifierEmail(java.lang.String lastModifierEmail) {
this.lastModifierEmail = lastModifierEmail;
return this;
}
/**
* The name of this Map, supplied by the author.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name of this Map, supplied by the author.
* @param name name or {@code null} for none
*/
public Map setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The processing status of this map. Map processing is automatically started once a map becomes
* ready for processing.
* @return value or {@code null} for none
*/
public java.lang.String getProcessingStatus() {
return processingStatus;
}
/**
* The processing status of this map. Map processing is automatically started once a map becomes
* ready for processing.
* @param processingStatus processingStatus or {@code null} for none
*/
public Map setProcessingStatus(java.lang.String processingStatus) {
this.processingStatus = processingStatus;
return this;
}
/**
* The ID of the project that this Map is in.
* @return value or {@code null} for none
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The ID of the project that this Map is in.
* @param projectId projectId or {@code null} for none
*/
public Map setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Deprecated: The access list to whom view permissions are granted. The value must be the name of
* a Maps Engine access list of the Map Viewer type, and the user must be a viewer on that list.
* Note: Google Maps Engine no longer uses access lists. Instead, each asset has its own list of
* permissions. For backward compatibility, the API still accepts access lists for projects that
* are already using access lists. If you created a GME account/project after July 14th, 2014, you
* will not be able to send API requests that include access lists. This is an input field only.
* It is not returned in response to a list or get request.
* @return value or {@code null} for none
*/
public java.lang.String getPublishedAccessList() {
return publishedAccessList;
}
/**
* Deprecated: The access list to whom view permissions are granted. The value must be the name of
* a Maps Engine access list of the Map Viewer type, and the user must be a viewer on that list.
* Note: Google Maps Engine no longer uses access lists. Instead, each asset has its own list of
* permissions. For backward compatibility, the API still accepts access lists for projects that
* are already using access lists. If you created a GME account/project after July 14th, 2014, you
* will not be able to send API requests that include access lists. This is an input field only.
* It is not returned in response to a list or get request.
* @param publishedAccessList publishedAccessList or {@code null} for none
*/
public Map setPublishedAccessList(java.lang.String publishedAccessList) {
this.publishedAccessList = publishedAccessList;
return this;
}
/**
* The publishing status of this map.
* @return value or {@code null} for none
*/
public java.lang.String getPublishingStatus() {
return publishingStatus;
}
/**
* The publishing status of this map.
* @param publishingStatus publishingStatus or {@code null} for none
*/
public Map setPublishingStatus(java.lang.String publishingStatus) {
this.publishingStatus = publishingStatus;
return this;
}
/**
* Tags of this Map.
* @return value or {@code null} for none
*/
public java.util.List getTags() {
return tags;
}
/**
* Tags of this Map.
* @param tags tags or {@code null} for none
*/
public Map setTags(java.util.List tags) {
this.tags = tags;
return this;
}
/**
* Deprecated: An array containing the available versions of this Map. Currently may only contain
* "published". The publishingStatus field should be used instead.
* @return value or {@code null} for none
*/
public java.util.List getVersions() {
return versions;
}
/**
* Deprecated: An array containing the available versions of this Map. Currently may only contain
* "published". The publishingStatus field should be used instead.
* @param versions versions or {@code null} for none
*/
public Map setVersions(java.util.List versions) {
this.versions = versions;
return this;
}
/**
* If true, WRITERs of the asset are able to edit the asset permissions.
* @return value or {@code null} for none
*/
public java.lang.Boolean getWritersCanEditPermissions() {
return writersCanEditPermissions;
}
/**
* If true, WRITERs of the asset are able to edit the asset permissions.
* @param writersCanEditPermissions writersCanEditPermissions or {@code null} for none
*/
public Map setWritersCanEditPermissions(java.lang.Boolean writersCanEditPermissions) {
this.writersCanEditPermissions = writersCanEditPermissions;
return this;
}
@Override
public Map set(String fieldName, Object value) {
return (Map) super.set(fieldName, value);
}
@Override
public Map clone() {
return (Map) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy