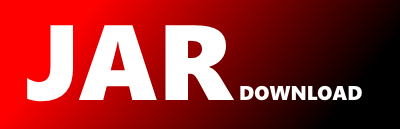
com.google.api.services.migrationcenter.v1.model.GuestConfigDetails Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.migrationcenter.v1.model;
/**
* Guest OS config information.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Migration Center API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GuestConfigDetails extends com.google.api.client.json.GenericJson {
/**
* Mount list (Linux fstab).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FstabEntryList fstab;
/**
* Hosts file (/etc/hosts).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private HostsEntryList hosts;
/**
* OS issue (typically /etc/issue in Linux).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String issue;
/**
* NFS exports.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NfsExportList nfsExports;
/**
* Security-Enhanced Linux (SELinux) mode.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selinuxMode;
/**
* Mount list (Linux fstab).
* @return value or {@code null} for none
*/
public FstabEntryList getFstab() {
return fstab;
}
/**
* Mount list (Linux fstab).
* @param fstab fstab or {@code null} for none
*/
public GuestConfigDetails setFstab(FstabEntryList fstab) {
this.fstab = fstab;
return this;
}
/**
* Hosts file (/etc/hosts).
* @return value or {@code null} for none
*/
public HostsEntryList getHosts() {
return hosts;
}
/**
* Hosts file (/etc/hosts).
* @param hosts hosts or {@code null} for none
*/
public GuestConfigDetails setHosts(HostsEntryList hosts) {
this.hosts = hosts;
return this;
}
/**
* OS issue (typically /etc/issue in Linux).
* @return value or {@code null} for none
*/
public java.lang.String getIssue() {
return issue;
}
/**
* OS issue (typically /etc/issue in Linux).
* @param issue issue or {@code null} for none
*/
public GuestConfigDetails setIssue(java.lang.String issue) {
this.issue = issue;
return this;
}
/**
* NFS exports.
* @return value or {@code null} for none
*/
public NfsExportList getNfsExports() {
return nfsExports;
}
/**
* NFS exports.
* @param nfsExports nfsExports or {@code null} for none
*/
public GuestConfigDetails setNfsExports(NfsExportList nfsExports) {
this.nfsExports = nfsExports;
return this;
}
/**
* Security-Enhanced Linux (SELinux) mode.
* @return value or {@code null} for none
*/
public java.lang.String getSelinuxMode() {
return selinuxMode;
}
/**
* Security-Enhanced Linux (SELinux) mode.
* @param selinuxMode selinuxMode or {@code null} for none
*/
public GuestConfigDetails setSelinuxMode(java.lang.String selinuxMode) {
this.selinuxMode = selinuxMode;
return this;
}
@Override
public GuestConfigDetails set(String fieldName, Object value) {
return (GuestConfigDetails) super.set(fieldName, value);
}
@Override
public GuestConfigDetails clone() {
return (GuestConfigDetails) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy