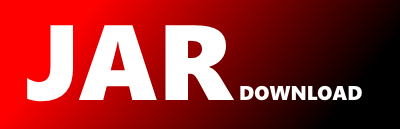
com.google.api.services.migrationcenter.v1.model.ReportSummaryGroupPreferenceSetFinding Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.migrationcenter.v1.model;
/**
* Summary Findings for a specific Group/PreferenceSet combination.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Migration Center API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ReportSummaryGroupPreferenceSetFinding extends com.google.api.client.json.GenericJson {
/**
* A set of findings that applies to Compute Engine machines in the input.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ReportSummaryComputeEngineFinding computeEngineFinding;
/**
* Description for the Preference Set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Display Name of the Preference Set
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* A set of preferences that applies to all machines in the context.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private VirtualMachinePreferences machinePreferences;
/**
* Compute monthly cost for this preference set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money monthlyCostCompute;
/**
* Network Egress monthly cost for this preference set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money monthlyCostNetworkEgress;
/**
* Licensing monthly cost for this preference set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money monthlyCostOsLicense;
/**
* Miscellaneous monthly cost for this preference set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money monthlyCostOther;
/**
* Storage monthly cost for this preference set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money monthlyCostStorage;
/**
* Total monthly cost for this preference set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money monthlyCostTotal;
/**
* A set of findings that applies to Sole-Tenant machines in the input.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ReportSummarySoleTenantFinding soleTenantFinding;
/**
* A set of findings that applies to VMWare machines in the input.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ReportSummaryVmwareEngineFinding vmwareEngineFinding;
/**
* A set of findings that applies to Compute Engine machines in the input.
* @return value or {@code null} for none
*/
public ReportSummaryComputeEngineFinding getComputeEngineFinding() {
return computeEngineFinding;
}
/**
* A set of findings that applies to Compute Engine machines in the input.
* @param computeEngineFinding computeEngineFinding or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setComputeEngineFinding(ReportSummaryComputeEngineFinding computeEngineFinding) {
this.computeEngineFinding = computeEngineFinding;
return this;
}
/**
* Description for the Preference Set.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Description for the Preference Set.
* @param description description or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Display Name of the Preference Set
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* Display Name of the Preference Set
* @param displayName displayName or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* A set of preferences that applies to all machines in the context.
* @return value or {@code null} for none
*/
public VirtualMachinePreferences getMachinePreferences() {
return machinePreferences;
}
/**
* A set of preferences that applies to all machines in the context.
* @param machinePreferences machinePreferences or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setMachinePreferences(VirtualMachinePreferences machinePreferences) {
this.machinePreferences = machinePreferences;
return this;
}
/**
* Compute monthly cost for this preference set.
* @return value or {@code null} for none
*/
public Money getMonthlyCostCompute() {
return monthlyCostCompute;
}
/**
* Compute monthly cost for this preference set.
* @param monthlyCostCompute monthlyCostCompute or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setMonthlyCostCompute(Money monthlyCostCompute) {
this.monthlyCostCompute = monthlyCostCompute;
return this;
}
/**
* Network Egress monthly cost for this preference set.
* @return value or {@code null} for none
*/
public Money getMonthlyCostNetworkEgress() {
return monthlyCostNetworkEgress;
}
/**
* Network Egress monthly cost for this preference set.
* @param monthlyCostNetworkEgress monthlyCostNetworkEgress or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setMonthlyCostNetworkEgress(Money monthlyCostNetworkEgress) {
this.monthlyCostNetworkEgress = monthlyCostNetworkEgress;
return this;
}
/**
* Licensing monthly cost for this preference set.
* @return value or {@code null} for none
*/
public Money getMonthlyCostOsLicense() {
return monthlyCostOsLicense;
}
/**
* Licensing monthly cost for this preference set.
* @param monthlyCostOsLicense monthlyCostOsLicense or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setMonthlyCostOsLicense(Money monthlyCostOsLicense) {
this.monthlyCostOsLicense = monthlyCostOsLicense;
return this;
}
/**
* Miscellaneous monthly cost for this preference set.
* @return value or {@code null} for none
*/
public Money getMonthlyCostOther() {
return monthlyCostOther;
}
/**
* Miscellaneous monthly cost for this preference set.
* @param monthlyCostOther monthlyCostOther or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setMonthlyCostOther(Money monthlyCostOther) {
this.monthlyCostOther = monthlyCostOther;
return this;
}
/**
* Storage monthly cost for this preference set.
* @return value or {@code null} for none
*/
public Money getMonthlyCostStorage() {
return monthlyCostStorage;
}
/**
* Storage monthly cost for this preference set.
* @param monthlyCostStorage monthlyCostStorage or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setMonthlyCostStorage(Money monthlyCostStorage) {
this.monthlyCostStorage = monthlyCostStorage;
return this;
}
/**
* Total monthly cost for this preference set.
* @return value or {@code null} for none
*/
public Money getMonthlyCostTotal() {
return monthlyCostTotal;
}
/**
* Total monthly cost for this preference set.
* @param monthlyCostTotal monthlyCostTotal or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setMonthlyCostTotal(Money monthlyCostTotal) {
this.monthlyCostTotal = monthlyCostTotal;
return this;
}
/**
* A set of findings that applies to Sole-Tenant machines in the input.
* @return value or {@code null} for none
*/
public ReportSummarySoleTenantFinding getSoleTenantFinding() {
return soleTenantFinding;
}
/**
* A set of findings that applies to Sole-Tenant machines in the input.
* @param soleTenantFinding soleTenantFinding or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setSoleTenantFinding(ReportSummarySoleTenantFinding soleTenantFinding) {
this.soleTenantFinding = soleTenantFinding;
return this;
}
/**
* A set of findings that applies to VMWare machines in the input.
* @return value or {@code null} for none
*/
public ReportSummaryVmwareEngineFinding getVmwareEngineFinding() {
return vmwareEngineFinding;
}
/**
* A set of findings that applies to VMWare machines in the input.
* @param vmwareEngineFinding vmwareEngineFinding or {@code null} for none
*/
public ReportSummaryGroupPreferenceSetFinding setVmwareEngineFinding(ReportSummaryVmwareEngineFinding vmwareEngineFinding) {
this.vmwareEngineFinding = vmwareEngineFinding;
return this;
}
@Override
public ReportSummaryGroupPreferenceSetFinding set(String fieldName, Object value) {
return (ReportSummaryGroupPreferenceSetFinding) super.set(fieldName, value);
}
@Override
public ReportSummaryGroupPreferenceSetFinding clone() {
return (ReportSummaryGroupPreferenceSetFinding) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy