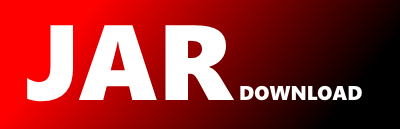
com.google.api.services.notebooks.v2.model.GceSetup Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.notebooks.v2.model;
/**
* The definition of how to configure a VM instance outside of Resources and Identity.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Notebooks API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GceSetup extends com.google.api.client.json.GenericJson {
/**
* Optional. The hardware accelerators used on this instance. If you use accelerators, make sure
* that your configuration has [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list). Currently supports only one
* accelerator configuration.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List acceleratorConfigs;
static {
// hack to force ProGuard to consider AcceleratorConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AcceleratorConfig.class);
}
/**
* Optional. The boot disk for the VM.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BootDisk bootDisk;
/**
* Optional. Use a container image to start the notebook instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ContainerImage containerImage;
/**
* Optional. Data disks attached to the VM instance. Currently supports only one data disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List dataDisks;
static {
// hack to force ProGuard to consider DataDisk used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(DataDisk.class);
}
/**
* Optional. If true, no external IP will be assigned to this VM instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean disablePublicIp;
/**
* Optional. Flag to enable ip forwarding or not, default false/off.
* https://cloud.google.com/vpc/docs/using-routes#canipforward
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableIpForwarding;
/**
* Optional. Configuration for GPU drivers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GPUDriverConfig gpuDriverConfig;
/**
* Optional. The machine type of the VM instance. https://cloud.google.com/compute/docs/machine-
* resource
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String machineType;
/**
* Optional. Custom metadata to apply to this instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map metadata;
/**
* Optional. The minimum CPU platform to use for this instance. The list of valid values can be
* found in https://cloud.google.com/compute/docs/instances/specify-min-cpu-
* platform#availablezones
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String minCpuPlatform;
/**
* Optional. The network interfaces for the VM. Supports only one interface.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List networkInterfaces;
/**
* Optional. The service account that serves as an identity for the VM instance. Currently
* supports only one service account.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List serviceAccounts;
/**
* Optional. Shielded VM configuration. [Images using supported Shielded VM
* features](https://cloud.google.com/compute/docs/instances/modifying-shielded-vm).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ShieldedInstanceConfig shieldedInstanceConfig;
/**
* Optional. The Compute Engine network tags to add to runtime (see [Add network
* tags](https://cloud.google.com/vpc/docs/add-remove-network-tags)).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tags;
/**
* Optional. Use a Compute Engine VM image to start the notebook instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private VmImage vmImage;
/**
* Optional. The hardware accelerators used on this instance. If you use accelerators, make sure
* that your configuration has [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list). Currently supports only one
* accelerator configuration.
* @return value or {@code null} for none
*/
public java.util.List getAcceleratorConfigs() {
return acceleratorConfigs;
}
/**
* Optional. The hardware accelerators used on this instance. If you use accelerators, make sure
* that your configuration has [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list). Currently supports only one
* accelerator configuration.
* @param acceleratorConfigs acceleratorConfigs or {@code null} for none
*/
public GceSetup setAcceleratorConfigs(java.util.List acceleratorConfigs) {
this.acceleratorConfigs = acceleratorConfigs;
return this;
}
/**
* Optional. The boot disk for the VM.
* @return value or {@code null} for none
*/
public BootDisk getBootDisk() {
return bootDisk;
}
/**
* Optional. The boot disk for the VM.
* @param bootDisk bootDisk or {@code null} for none
*/
public GceSetup setBootDisk(BootDisk bootDisk) {
this.bootDisk = bootDisk;
return this;
}
/**
* Optional. Use a container image to start the notebook instance.
* @return value or {@code null} for none
*/
public ContainerImage getContainerImage() {
return containerImage;
}
/**
* Optional. Use a container image to start the notebook instance.
* @param containerImage containerImage or {@code null} for none
*/
public GceSetup setContainerImage(ContainerImage containerImage) {
this.containerImage = containerImage;
return this;
}
/**
* Optional. Data disks attached to the VM instance. Currently supports only one data disk.
* @return value or {@code null} for none
*/
public java.util.List getDataDisks() {
return dataDisks;
}
/**
* Optional. Data disks attached to the VM instance. Currently supports only one data disk.
* @param dataDisks dataDisks or {@code null} for none
*/
public GceSetup setDataDisks(java.util.List dataDisks) {
this.dataDisks = dataDisks;
return this;
}
/**
* Optional. If true, no external IP will be assigned to this VM instance.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDisablePublicIp() {
return disablePublicIp;
}
/**
* Optional. If true, no external IP will be assigned to this VM instance.
* @param disablePublicIp disablePublicIp or {@code null} for none
*/
public GceSetup setDisablePublicIp(java.lang.Boolean disablePublicIp) {
this.disablePublicIp = disablePublicIp;
return this;
}
/**
* Optional. Flag to enable ip forwarding or not, default false/off.
* https://cloud.google.com/vpc/docs/using-routes#canipforward
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableIpForwarding() {
return enableIpForwarding;
}
/**
* Optional. Flag to enable ip forwarding or not, default false/off.
* https://cloud.google.com/vpc/docs/using-routes#canipforward
* @param enableIpForwarding enableIpForwarding or {@code null} for none
*/
public GceSetup setEnableIpForwarding(java.lang.Boolean enableIpForwarding) {
this.enableIpForwarding = enableIpForwarding;
return this;
}
/**
* Optional. Configuration for GPU drivers.
* @return value or {@code null} for none
*/
public GPUDriverConfig getGpuDriverConfig() {
return gpuDriverConfig;
}
/**
* Optional. Configuration for GPU drivers.
* @param gpuDriverConfig gpuDriverConfig or {@code null} for none
*/
public GceSetup setGpuDriverConfig(GPUDriverConfig gpuDriverConfig) {
this.gpuDriverConfig = gpuDriverConfig;
return this;
}
/**
* Optional. The machine type of the VM instance. https://cloud.google.com/compute/docs/machine-
* resource
* @return value or {@code null} for none
*/
public java.lang.String getMachineType() {
return machineType;
}
/**
* Optional. The machine type of the VM instance. https://cloud.google.com/compute/docs/machine-
* resource
* @param machineType machineType or {@code null} for none
*/
public GceSetup setMachineType(java.lang.String machineType) {
this.machineType = machineType;
return this;
}
/**
* Optional. Custom metadata to apply to this instance.
* @return value or {@code null} for none
*/
public java.util.Map getMetadata() {
return metadata;
}
/**
* Optional. Custom metadata to apply to this instance.
* @param metadata metadata or {@code null} for none
*/
public GceSetup setMetadata(java.util.Map metadata) {
this.metadata = metadata;
return this;
}
/**
* Optional. The minimum CPU platform to use for this instance. The list of valid values can be
* found in https://cloud.google.com/compute/docs/instances/specify-min-cpu-
* platform#availablezones
* @return value or {@code null} for none
*/
public java.lang.String getMinCpuPlatform() {
return minCpuPlatform;
}
/**
* Optional. The minimum CPU platform to use for this instance. The list of valid values can be
* found in https://cloud.google.com/compute/docs/instances/specify-min-cpu-
* platform#availablezones
* @param minCpuPlatform minCpuPlatform or {@code null} for none
*/
public GceSetup setMinCpuPlatform(java.lang.String minCpuPlatform) {
this.minCpuPlatform = minCpuPlatform;
return this;
}
/**
* Optional. The network interfaces for the VM. Supports only one interface.
* @return value or {@code null} for none
*/
public java.util.List getNetworkInterfaces() {
return networkInterfaces;
}
/**
* Optional. The network interfaces for the VM. Supports only one interface.
* @param networkInterfaces networkInterfaces or {@code null} for none
*/
public GceSetup setNetworkInterfaces(java.util.List networkInterfaces) {
this.networkInterfaces = networkInterfaces;
return this;
}
/**
* Optional. The service account that serves as an identity for the VM instance. Currently
* supports only one service account.
* @return value or {@code null} for none
*/
public java.util.List getServiceAccounts() {
return serviceAccounts;
}
/**
* Optional. The service account that serves as an identity for the VM instance. Currently
* supports only one service account.
* @param serviceAccounts serviceAccounts or {@code null} for none
*/
public GceSetup setServiceAccounts(java.util.List serviceAccounts) {
this.serviceAccounts = serviceAccounts;
return this;
}
/**
* Optional. Shielded VM configuration. [Images using supported Shielded VM
* features](https://cloud.google.com/compute/docs/instances/modifying-shielded-vm).
* @return value or {@code null} for none
*/
public ShieldedInstanceConfig getShieldedInstanceConfig() {
return shieldedInstanceConfig;
}
/**
* Optional. Shielded VM configuration. [Images using supported Shielded VM
* features](https://cloud.google.com/compute/docs/instances/modifying-shielded-vm).
* @param shieldedInstanceConfig shieldedInstanceConfig or {@code null} for none
*/
public GceSetup setShieldedInstanceConfig(ShieldedInstanceConfig shieldedInstanceConfig) {
this.shieldedInstanceConfig = shieldedInstanceConfig;
return this;
}
/**
* Optional. The Compute Engine network tags to add to runtime (see [Add network
* tags](https://cloud.google.com/vpc/docs/add-remove-network-tags)).
* @return value or {@code null} for none
*/
public java.util.List getTags() {
return tags;
}
/**
* Optional. The Compute Engine network tags to add to runtime (see [Add network
* tags](https://cloud.google.com/vpc/docs/add-remove-network-tags)).
* @param tags tags or {@code null} for none
*/
public GceSetup setTags(java.util.List tags) {
this.tags = tags;
return this;
}
/**
* Optional. Use a Compute Engine VM image to start the notebook instance.
* @return value or {@code null} for none
*/
public VmImage getVmImage() {
return vmImage;
}
/**
* Optional. Use a Compute Engine VM image to start the notebook instance.
* @param vmImage vmImage or {@code null} for none
*/
public GceSetup setVmImage(VmImage vmImage) {
this.vmImage = vmImage;
return this;
}
@Override
public GceSetup set(String fieldName, Object value) {
return (GceSetup) super.set(fieldName, value);
}
@Override
public GceSetup clone() {
return (GceSetup) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy