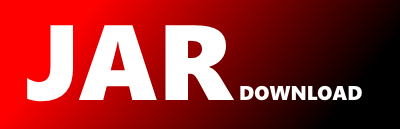
com.google.api.services.orkut.Orkut Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2013-08-01 15:32:38 UTC)
* on 2013-08-06 at 21:02:08 UTC
* Modify at your own risk.
*/
package com.google.api.services.orkut;
/**
* Service definition for Orkut (v2).
*
*
* Lets you manage activities, comments and badges in Orkut. More stuff coming in time.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link OrkutRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Orkut extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.15.0-rc of the Orkut API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "orkut/v2/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Orkut(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Orkut(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Acl collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.Acl.List request = orkut.acl().list(parameters ...)}
*
*
* @return the resource collection
*/
public Acl acl() {
return new Acl();
}
/**
* The "acl" collection of methods.
*/
public class Acl {
/**
* Excludes an element from the ACL of the activity.
*
* Create a request for the method "acl.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param activityId ID of the activity.
* @param userId ID of the user to be removed from the activity.
* @return the request
*/
public Delete delete(java.lang.String activityId, java.lang.String userId) throws java.io.IOException {
Delete result = new Delete(activityId, userId);
initialize(result);
return result;
}
public class Delete extends OrkutRequest {
private static final String REST_PATH = "activities/{activityId}/acl/{userId}";
/**
* Excludes an element from the ACL of the activity.
*
* Create a request for the method "acl.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param activityId ID of the activity.
* @param userId ID of the user to be removed from the activity.
* @since 1.13
*/
protected Delete(java.lang.String activityId, java.lang.String userId) {
super(Orkut.this, "DELETE", REST_PATH, null, Void.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** ID of the activity. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** ID of the activity.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** ID of the activity. */
public Delete setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
/** ID of the user to be removed from the activity. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of the user to be removed from the activity.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of the user to be removed from the activity. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Activities collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.Activities.List request = orkut.activities().list(parameters ...)}
*
*
* @return the resource collection
*/
public Activities activities() {
return new Activities();
}
/**
* The "activities" collection of methods.
*/
public class Activities {
/**
* Deletes an existing activity, if the access controls allow it.
*
* Create a request for the method "activities.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param activityId ID of the activity to remove.
* @return the request
*/
public Delete delete(java.lang.String activityId) throws java.io.IOException {
Delete result = new Delete(activityId);
initialize(result);
return result;
}
public class Delete extends OrkutRequest {
private static final String REST_PATH = "activities/{activityId}";
/**
* Deletes an existing activity, if the access controls allow it.
*
* Create a request for the method "activities.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param activityId ID of the activity to remove.
* @since 1.13
*/
protected Delete(java.lang.String activityId) {
super(Orkut.this, "DELETE", REST_PATH, null, Void.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** ID of the activity to remove. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** ID of the activity to remove.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** ID of the activity to remove. */
public Delete setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a list of activities.
*
* Create a request for the method "activities.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The ID of the user whose activities will be listed. Can be me to refer to the viewer (i.e. the
* authenticated user).
* @param collection The collection of activities to list.
* @return the request
*/
public List list(java.lang.String userId, java.lang.String collection) throws java.io.IOException {
List result = new List(userId, collection);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "people/{userId}/activities/{collection}";
/**
* Retrieves a list of activities.
*
* Create a request for the method "activities.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The ID of the user whose activities will be listed. Can be me to refer to the viewer (i.e. the
* authenticated user).
* @param collection The collection of activities to list.
* @since 1.13
*/
protected List(java.lang.String userId, java.lang.String collection) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.ActivityList.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The ID of the user whose activities will be listed. Can be me to refer to the viewer (i.e.
* the authenticated user).
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user whose activities will be listed. Can be me to refer to the viewer (i.e. the
authenticated user).
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The ID of the user whose activities will be listed. Can be me to refer to the viewer (i.e.
* the authenticated user).
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The collection of activities to list. */
@com.google.api.client.util.Key
private java.lang.String collection;
/** The collection of activities to list.
*/
public java.lang.String getCollection() {
return collection;
}
/** The collection of activities to list. */
public List setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/** A continuation token that allows pagination. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token that allows pagination.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A continuation token that allows pagination. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The maximum number of activities to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of activities to include in the response.
[minimum: 1] [maximum: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of activities to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ActivityVisibility collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.ActivityVisibility.List request = orkut.activityVisibility().list(parameters ...)}
*
*
* @return the resource collection
*/
public ActivityVisibility activityVisibility() {
return new ActivityVisibility();
}
/**
* The "activityVisibility" collection of methods.
*/
public class ActivityVisibility {
/**
* Gets the visibility of an existing activity.
*
* Create a request for the method "activityVisibility.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param activityId ID of the activity to get the visibility.
* @return the request
*/
public Get get(java.lang.String activityId) throws java.io.IOException {
Get result = new Get(activityId);
initialize(result);
return result;
}
public class Get extends OrkutRequest {
private static final String REST_PATH = "activities/{activityId}/visibility";
/**
* Gets the visibility of an existing activity.
*
* Create a request for the method "activityVisibility.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param activityId ID of the activity to get the visibility.
* @since 1.13
*/
protected Get(java.lang.String activityId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.Visibility.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** ID of the activity to get the visibility. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** ID of the activity to get the visibility.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** ID of the activity to get the visibility. */
public Get setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Updates the visibility of an existing activity. This method supports patch semantics.
*
* Create a request for the method "activityVisibility.patch".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param activityId ID of the activity.
* @param content the {@link com.google.api.services.orkut.model.Visibility}
* @return the request
*/
public Patch patch(java.lang.String activityId, com.google.api.services.orkut.model.Visibility content) throws java.io.IOException {
Patch result = new Patch(activityId, content);
initialize(result);
return result;
}
public class Patch extends OrkutRequest {
private static final String REST_PATH = "activities/{activityId}/visibility";
/**
* Updates the visibility of an existing activity. This method supports patch semantics.
*
* Create a request for the method "activityVisibility.patch".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param activityId ID of the activity.
* @param content the {@link com.google.api.services.orkut.model.Visibility}
* @since 1.13
*/
protected Patch(java.lang.String activityId, com.google.api.services.orkut.model.Visibility content) {
super(Orkut.this, "PATCH", REST_PATH, content, com.google.api.services.orkut.model.Visibility.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** ID of the activity. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** ID of the activity.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** ID of the activity. */
public Patch setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the visibility of an existing activity.
*
* Create a request for the method "activityVisibility.update".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param activityId ID of the activity.
* @param content the {@link com.google.api.services.orkut.model.Visibility}
* @return the request
*/
public Update update(java.lang.String activityId, com.google.api.services.orkut.model.Visibility content) throws java.io.IOException {
Update result = new Update(activityId, content);
initialize(result);
return result;
}
public class Update extends OrkutRequest {
private static final String REST_PATH = "activities/{activityId}/visibility";
/**
* Updates the visibility of an existing activity.
*
* Create a request for the method "activityVisibility.update".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param activityId ID of the activity.
* @param content the {@link com.google.api.services.orkut.model.Visibility}
* @since 1.13
*/
protected Update(java.lang.String activityId, com.google.api.services.orkut.model.Visibility content) {
super(Orkut.this, "PUT", REST_PATH, content, com.google.api.services.orkut.model.Visibility.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/** ID of the activity. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** ID of the activity.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** ID of the activity. */
public Update setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Badges collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.Badges.List request = orkut.badges().list(parameters ...)}
*
*
* @return the resource collection
*/
public Badges badges() {
return new Badges();
}
/**
* The "badges" collection of methods.
*/
public class Badges {
/**
* Retrieves a badge from a user.
*
* Create a request for the method "badges.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId The ID of the user whose badges will be listed. Can be me to refer to caller.
* @param badgeId The ID of the badge that will be retrieved.
* @return the request
*/
public Get get(java.lang.String userId, java.lang.Long badgeId) throws java.io.IOException {
Get result = new Get(userId, badgeId);
initialize(result);
return result;
}
public class Get extends OrkutRequest {
private static final String REST_PATH = "people/{userId}/badges/{badgeId}";
/**
* Retrieves a badge from a user.
*
* Create a request for the method "badges.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The ID of the user whose badges will be listed. Can be me to refer to caller.
* @param badgeId The ID of the badge that will be retrieved.
* @since 1.13
*/
protected Get(java.lang.String userId, java.lang.Long badgeId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.Badge.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.badgeId = com.google.api.client.util.Preconditions.checkNotNull(badgeId, "Required parameter badgeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the user whose badges will be listed. Can be me to refer to caller. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user whose badges will be listed. Can be me to refer to caller.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user whose badges will be listed. Can be me to refer to caller. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the badge that will be retrieved. */
@com.google.api.client.util.Key
private java.lang.Long badgeId;
/** The ID of the badge that will be retrieved.
*/
public java.lang.Long getBadgeId() {
return badgeId;
}
/** The ID of the badge that will be retrieved. */
public Get setBadgeId(java.lang.Long badgeId) {
this.badgeId = badgeId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the list of visible badges of a user.
*
* Create a request for the method "badges.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The id of the user whose badges will be listed. Can be me to refer to caller.
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "people/{userId}/badges";
/**
* Retrieves the list of visible badges of a user.
*
* Create a request for the method "badges.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The id of the user whose badges will be listed. Can be me to refer to caller.
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.BadgeList.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The id of the user whose badges will be listed. Can be me to refer to caller. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The id of the user whose badges will be listed. Can be me to refer to caller.
*/
public java.lang.String getUserId() {
return userId;
}
/** The id of the user whose badges will be listed. Can be me to refer to caller. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Comments collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.Comments.List request = orkut.comments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Comments comments() {
return new Comments();
}
/**
* The "comments" collection of methods.
*/
public class Comments {
/**
* Deletes an existing comment.
*
* Create a request for the method "comments.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param commentId ID of the comment to remove.
* @return the request
*/
public Delete delete(java.lang.String commentId) throws java.io.IOException {
Delete result = new Delete(commentId);
initialize(result);
return result;
}
public class Delete extends OrkutRequest {
private static final String REST_PATH = "comments/{commentId}";
/**
* Deletes an existing comment.
*
* Create a request for the method "comments.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param commentId ID of the comment to remove.
* @since 1.13
*/
protected Delete(java.lang.String commentId) {
super(Orkut.this, "DELETE", REST_PATH, null, Void.class);
this.commentId = com.google.api.client.util.Preconditions.checkNotNull(commentId, "Required parameter commentId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** ID of the comment to remove. */
@com.google.api.client.util.Key
private java.lang.String commentId;
/** ID of the comment to remove.
*/
public java.lang.String getCommentId() {
return commentId;
}
/** ID of the comment to remove. */
public Delete setCommentId(java.lang.String commentId) {
this.commentId = commentId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves an existing comment.
*
* Create a request for the method "comments.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param commentId ID of the comment to get.
* @return the request
*/
public Get get(java.lang.String commentId) throws java.io.IOException {
Get result = new Get(commentId);
initialize(result);
return result;
}
public class Get extends OrkutRequest {
private static final String REST_PATH = "comments/{commentId}";
/**
* Retrieves an existing comment.
*
* Create a request for the method "comments.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param commentId ID of the comment to get.
* @since 1.13
*/
protected Get(java.lang.String commentId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.Comment.class);
this.commentId = com.google.api.client.util.Preconditions.checkNotNull(commentId, "Required parameter commentId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** ID of the comment to get. */
@com.google.api.client.util.Key
private java.lang.String commentId;
/** ID of the comment to get.
*/
public java.lang.String getCommentId() {
return commentId;
}
/** ID of the comment to get. */
public Get setCommentId(java.lang.String commentId) {
this.commentId = commentId;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public Get setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new comment to an activity.
*
* Create a request for the method "comments.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param activityId The ID of the activity to contain the new comment.
* @param content the {@link com.google.api.services.orkut.model.Comment}
* @return the request
*/
public Insert insert(java.lang.String activityId, com.google.api.services.orkut.model.Comment content) throws java.io.IOException {
Insert result = new Insert(activityId, content);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "activities/{activityId}/comments";
/**
* Inserts a new comment to an activity.
*
* Create a request for the method "comments.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param activityId The ID of the activity to contain the new comment.
* @param content the {@link com.google.api.services.orkut.model.Comment}
* @since 1.13
*/
protected Insert(java.lang.String activityId, com.google.api.services.orkut.model.Comment content) {
super(Orkut.this, "POST", REST_PATH, content, com.google.api.services.orkut.model.Comment.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the activity to contain the new comment. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** The ID of the activity to contain the new comment.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** The ID of the activity to contain the new comment. */
public Insert setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of comments, possibly filtered.
*
* Create a request for the method "comments.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param activityId The ID of the activity containing the comments.
* @return the request
*/
public List list(java.lang.String activityId) throws java.io.IOException {
List result = new List(activityId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "activities/{activityId}/comments";
/**
* Retrieves a list of comments, possibly filtered.
*
* Create a request for the method "comments.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param activityId The ID of the activity containing the comments.
* @since 1.13
*/
protected List(java.lang.String activityId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommentList.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the activity containing the comments. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** The ID of the activity containing the comments.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** The ID of the activity containing the comments. */
public List setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
/** Sort search results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sort search results. [default: DESCENDING_SORT]
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Sort search results. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** A continuation token that allows pagination. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token that allows pagination.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A continuation token that allows pagination. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The maximum number of activities to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of activities to include in the response.
[minimum: 1]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of activities to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Communities collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.Communities.List request = orkut.communities().list(parameters ...)}
*
*
* @return the resource collection
*/
public Communities communities() {
return new Communities();
}
/**
* The "communities" collection of methods.
*/
public class Communities {
/**
* Retrieves the basic information (aka. profile) of a community.
*
* Create a request for the method "communities.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community to get.
* @return the request
*/
public Get get(java.lang.Integer communityId) throws java.io.IOException {
Get result = new Get(communityId);
initialize(result);
return result;
}
public class Get extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}";
/**
* Retrieves the basic information (aka. profile) of a community.
*
* Create a request for the method "communities.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community to get.
* @since 1.13
*/
protected Get(java.lang.Integer communityId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.Community.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the community to get. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community to get.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community to get. */
public Get setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public Get setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the list of communities the current user is a member of.
*
* Create a request for the method "communities.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The ID of the user whose communities will be listed. Can be me to refer to caller.
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "people/{userId}/communities";
/**
* Retrieves the list of communities the current user is a member of.
*
* Create a request for the method "communities.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The ID of the user whose communities will be listed. Can be me to refer to caller.
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityList.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the user whose communities will be listed. Can be me to refer to caller. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user whose communities will be listed. Can be me to refer to caller.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user whose communities will be listed. Can be me to refer to caller. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** How to order the communities by. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** How to order the communities by.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** How to order the communities by. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** The maximum number of communities to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of communities to include in the response.
[minimum: 1]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of communities to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityFollow collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityFollow.List request = orkut.communityFollow().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityFollow communityFollow() {
return new CommunityFollow();
}
/**
* The "communityFollow" collection of methods.
*/
public class CommunityFollow {
/**
* Removes a user from the followers of a community.
*
* Create a request for the method "communityFollow.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @return the request
*/
public Delete delete(java.lang.Integer communityId, java.lang.String userId) throws java.io.IOException {
Delete result = new Delete(communityId, userId);
initialize(result);
return result;
}
public class Delete extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/followers/{userId}";
/**
* Removes a user from the followers of a community.
*
* Create a request for the method "communityFollow.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @since 1.13
*/
protected Delete(java.lang.Integer communityId, java.lang.String userId) {
super(Orkut.this, "DELETE", REST_PATH, null, Void.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** ID of the community. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** ID of the community.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** ID of the community. */
public Delete setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of the user. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Adds a user as a follower of a community.
*
* Create a request for the method "communityFollow.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @return the request
*/
public Insert insert(java.lang.Integer communityId, java.lang.String userId) throws java.io.IOException {
Insert result = new Insert(communityId, userId);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/followers/{userId}";
/**
* Adds a user as a follower of a community.
*
* Create a request for the method "communityFollow.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @since 1.13
*/
protected Insert(java.lang.Integer communityId, java.lang.String userId) {
super(Orkut.this, "POST", REST_PATH, null, com.google.api.services.orkut.model.CommunityMembers.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** ID of the community. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** ID of the community.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** ID of the community. */
public Insert setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of the user. */
public Insert setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityMembers collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityMembers.List request = orkut.communityMembers().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityMembers communityMembers() {
return new CommunityMembers();
}
/**
* The "communityMembers" collection of methods.
*/
public class CommunityMembers {
/**
* Makes the user leave a community.
*
* Create a request for the method "communityMembers.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @return the request
*/
public Delete delete(java.lang.Integer communityId, java.lang.String userId) throws java.io.IOException {
Delete result = new Delete(communityId, userId);
initialize(result);
return result;
}
public class Delete extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/members/{userId}";
/**
* Makes the user leave a community.
*
* Create a request for the method "communityMembers.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @since 1.13
*/
protected Delete(java.lang.Integer communityId, java.lang.String userId) {
super(Orkut.this, "DELETE", REST_PATH, null, Void.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** ID of the community. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** ID of the community.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** ID of the community. */
public Delete setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of the user. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the relationship between a user and a community.
*
* Create a request for the method "communityMembers.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @return the request
*/
public Get get(java.lang.Integer communityId, java.lang.String userId) throws java.io.IOException {
Get result = new Get(communityId, userId);
initialize(result);
return result;
}
public class Get extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/members/{userId}";
/**
* Retrieves the relationship between a user and a community.
*
* Create a request for the method "communityMembers.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @since 1.13
*/
protected Get(java.lang.Integer communityId, java.lang.String userId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityMembers.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** ID of the community. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** ID of the community.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** ID of the community. */
public Get setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of the user. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public Get setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Makes the user join a community.
*
* Create a request for the method "communityMembers.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @return the request
*/
public Insert insert(java.lang.Integer communityId, java.lang.String userId) throws java.io.IOException {
Insert result = new Insert(communityId, userId);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/members/{userId}";
/**
* Makes the user join a community.
*
* Create a request for the method "communityMembers.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId ID of the community.
* @param userId ID of the user.
* @since 1.13
*/
protected Insert(java.lang.Integer communityId, java.lang.String userId) {
super(Orkut.this, "POST", REST_PATH, null, com.google.api.services.orkut.model.CommunityMembers.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** ID of the community. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** ID of the community.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** ID of the community. */
public Insert setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** ID of the user. */
public Insert setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists members of a community. Use the pagination tokens to retrieve the full list; do not rely on
* the member count available in the community profile information to know when to stop iterating,
* as that count may be approximate.
*
* Create a request for the method "communityMembers.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose members will be listed.
* @return the request
*/
public List list(java.lang.Integer communityId) throws java.io.IOException {
List result = new List(communityId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/members";
/**
* Lists members of a community. Use the pagination tokens to retrieve the full list; do not rely
* on the member count available in the community profile information to know when to stop
* iterating, as that count may be approximate.
*
* Create a request for the method "communityMembers.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose members will be listed.
* @since 1.13
*/
protected List(java.lang.Integer communityId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityMembersList.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the community whose members will be listed. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose members will be listed.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose members will be listed. */
public List setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** A continuation token that allows pagination. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token that allows pagination.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A continuation token that allows pagination. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Whether to list only community members who are friends of the user. */
@com.google.api.client.util.Key
private java.lang.Boolean friendsOnly;
/** Whether to list only community members who are friends of the user.
*/
public java.lang.Boolean getFriendsOnly() {
return friendsOnly;
}
/** Whether to list only community members who are friends of the user. */
public List setFriendsOnly(java.lang.Boolean friendsOnly) {
this.friendsOnly = friendsOnly;
return this;
}
/** The maximum number of members to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of members to include in the response.
[minimum: 1]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of members to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityMessages collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityMessages.List request = orkut.communityMessages().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityMessages communityMessages() {
return new CommunityMessages();
}
/**
* The "communityMessages" collection of methods.
*/
public class CommunityMessages {
/**
* Moves a message of the community to the trash folder.
*
* Create a request for the method "communityMessages.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose message will be moved to the trash folder.
* @param topicId The ID of the topic whose message will be moved to the trash folder.
* @param messageId The ID of the message to be moved to the trash folder.
* @return the request
*/
public Delete delete(java.lang.Integer communityId, java.lang.Long topicId, java.lang.Long messageId) throws java.io.IOException {
Delete result = new Delete(communityId, topicId, messageId);
initialize(result);
return result;
}
public class Delete extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/topics/{topicId}/messages/{messageId}";
/**
* Moves a message of the community to the trash folder.
*
* Create a request for the method "communityMessages.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose message will be moved to the trash folder.
* @param topicId The ID of the topic whose message will be moved to the trash folder.
* @param messageId The ID of the message to be moved to the trash folder.
* @since 1.13
*/
protected Delete(java.lang.Integer communityId, java.lang.Long topicId, java.lang.Long messageId) {
super(Orkut.this, "DELETE", REST_PATH, null, Void.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.topicId = com.google.api.client.util.Preconditions.checkNotNull(topicId, "Required parameter topicId must be specified.");
this.messageId = com.google.api.client.util.Preconditions.checkNotNull(messageId, "Required parameter messageId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** The ID of the community whose message will be moved to the trash folder. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose message will be moved to the trash folder.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose message will be moved to the trash folder. */
public Delete setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the topic whose message will be moved to the trash folder. */
@com.google.api.client.util.Key
private java.lang.Long topicId;
/** The ID of the topic whose message will be moved to the trash folder.
*/
public java.lang.Long getTopicId() {
return topicId;
}
/** The ID of the topic whose message will be moved to the trash folder. */
public Delete setTopicId(java.lang.Long topicId) {
this.topicId = topicId;
return this;
}
/** The ID of the message to be moved to the trash folder. */
@com.google.api.client.util.Key
private java.lang.Long messageId;
/** The ID of the message to be moved to the trash folder.
*/
public java.lang.Long getMessageId() {
return messageId;
}
/** The ID of the message to be moved to the trash folder. */
public Delete setMessageId(java.lang.Long messageId) {
this.messageId = messageId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Adds a message to a given community topic.
*
* Create a request for the method "communityMessages.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community the message should be added to.
* @param topicId The ID of the topic the message should be added to.
* @param content the {@link com.google.api.services.orkut.model.CommunityMessage}
* @return the request
*/
public Insert insert(java.lang.Integer communityId, java.lang.Long topicId, com.google.api.services.orkut.model.CommunityMessage content) throws java.io.IOException {
Insert result = new Insert(communityId, topicId, content);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/topics/{topicId}/messages";
/**
* Adds a message to a given community topic.
*
* Create a request for the method "communityMessages.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community the message should be added to.
* @param topicId The ID of the topic the message should be added to.
* @param content the {@link com.google.api.services.orkut.model.CommunityMessage}
* @since 1.13
*/
protected Insert(java.lang.Integer communityId, java.lang.Long topicId, com.google.api.services.orkut.model.CommunityMessage content) {
super(Orkut.this, "POST", REST_PATH, content, com.google.api.services.orkut.model.CommunityMessage.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.topicId = com.google.api.client.util.Preconditions.checkNotNull(topicId, "Required parameter topicId must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the community the message should be added to. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community the message should be added to.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community the message should be added to. */
public Insert setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the topic the message should be added to. */
@com.google.api.client.util.Key
private java.lang.Long topicId;
/** The ID of the topic the message should be added to.
*/
public java.lang.Long getTopicId() {
return topicId;
}
/** The ID of the topic the message should be added to. */
public Insert setTopicId(java.lang.Long topicId) {
this.topicId = topicId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the messages of a topic of a community.
*
* Create a request for the method "communityMessages.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community which messages will be listed.
* @param topicId The ID of the topic which messages will be listed.
* @return the request
*/
public List list(java.lang.Integer communityId, java.lang.Long topicId) throws java.io.IOException {
List result = new List(communityId, topicId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/topics/{topicId}/messages";
/**
* Retrieves the messages of a topic of a community.
*
* Create a request for the method "communityMessages.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community which messages will be listed.
* @param topicId The ID of the topic which messages will be listed.
* @since 1.13
*/
protected List(java.lang.Integer communityId, java.lang.Long topicId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityMessageList.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.topicId = com.google.api.client.util.Preconditions.checkNotNull(topicId, "Required parameter topicId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the community which messages will be listed. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community which messages will be listed.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community which messages will be listed. */
public List setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the topic which messages will be listed. */
@com.google.api.client.util.Key
private java.lang.Long topicId;
/** The ID of the topic which messages will be listed.
*/
public java.lang.Long getTopicId() {
return topicId;
}
/** The ID of the topic which messages will be listed. */
public List setTopicId(java.lang.Long topicId) {
this.topicId = topicId;
return this;
}
/** A continuation token that allows pagination. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token that allows pagination.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A continuation token that allows pagination. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The maximum number of messages to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of messages to include in the response.
[minimum: 1] [maximum: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of messages to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityPollComments collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityPollComments.List request = orkut.communityPollComments().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityPollComments communityPollComments() {
return new CommunityPollComments();
}
/**
* The "communityPollComments" collection of methods.
*/
public class CommunityPollComments {
/**
* Adds a comment on a community poll.
*
* Create a request for the method "communityPollComments.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose poll is being commented.
* @param pollId The ID of the poll being commented.
* @param content the {@link com.google.api.services.orkut.model.CommunityPollComment}
* @return the request
*/
public Insert insert(java.lang.Integer communityId, java.lang.String pollId, com.google.api.services.orkut.model.CommunityPollComment content) throws java.io.IOException {
Insert result = new Insert(communityId, pollId, content);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/polls/{pollId}/comments";
/**
* Adds a comment on a community poll.
*
* Create a request for the method "communityPollComments.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose poll is being commented.
* @param pollId The ID of the poll being commented.
* @param content the {@link com.google.api.services.orkut.model.CommunityPollComment}
* @since 1.13
*/
protected Insert(java.lang.Integer communityId, java.lang.String pollId, com.google.api.services.orkut.model.CommunityPollComment content) {
super(Orkut.this, "POST", REST_PATH, content, com.google.api.services.orkut.model.CommunityPollComment.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.pollId = com.google.api.client.util.Preconditions.checkNotNull(pollId, "Required parameter pollId must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the community whose poll is being commented. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose poll is being commented.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose poll is being commented. */
public Insert setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the poll being commented. */
@com.google.api.client.util.Key
private java.lang.String pollId;
/** The ID of the poll being commented.
*/
public java.lang.String getPollId() {
return pollId;
}
/** The ID of the poll being commented. */
public Insert setPollId(java.lang.String pollId) {
this.pollId = pollId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the comments of a community poll.
*
* Create a request for the method "communityPollComments.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose poll is having its comments listed.
* @param pollId The ID of the community whose polls will be listed.
* @return the request
*/
public List list(java.lang.Integer communityId, java.lang.String pollId) throws java.io.IOException {
List result = new List(communityId, pollId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/polls/{pollId}/comments";
/**
* Retrieves the comments of a community poll.
*
* Create a request for the method "communityPollComments.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose poll is having its comments listed.
* @param pollId The ID of the community whose polls will be listed.
* @since 1.13
*/
protected List(java.lang.Integer communityId, java.lang.String pollId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityPollCommentList.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.pollId = com.google.api.client.util.Preconditions.checkNotNull(pollId, "Required parameter pollId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the community whose poll is having its comments listed. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose poll is having its comments listed.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose poll is having its comments listed. */
public List setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the community whose polls will be listed. */
@com.google.api.client.util.Key
private java.lang.String pollId;
/** The ID of the community whose polls will be listed.
*/
public java.lang.String getPollId() {
return pollId;
}
/** The ID of the community whose polls will be listed. */
public List setPollId(java.lang.String pollId) {
this.pollId = pollId;
return this;
}
/** A continuation token that allows pagination. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token that allows pagination.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A continuation token that allows pagination. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The maximum number of comments to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of comments to include in the response.
[minimum: 1]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of comments to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityPollVotes collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityPollVotes.List request = orkut.communityPollVotes().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityPollVotes communityPollVotes() {
return new CommunityPollVotes();
}
/**
* The "communityPollVotes" collection of methods.
*/
public class CommunityPollVotes {
/**
* Votes on a community poll.
*
* Create a request for the method "communityPollVotes.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose poll is being voted.
* @param pollId The ID of the poll being voted.
* @param content the {@link com.google.api.services.orkut.model.CommunityPollVote}
* @return the request
*/
public Insert insert(java.lang.Integer communityId, java.lang.String pollId, com.google.api.services.orkut.model.CommunityPollVote content) throws java.io.IOException {
Insert result = new Insert(communityId, pollId, content);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/polls/{pollId}/votes";
/**
* Votes on a community poll.
*
* Create a request for the method "communityPollVotes.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose poll is being voted.
* @param pollId The ID of the poll being voted.
* @param content the {@link com.google.api.services.orkut.model.CommunityPollVote}
* @since 1.13
*/
protected Insert(java.lang.Integer communityId, java.lang.String pollId, com.google.api.services.orkut.model.CommunityPollVote content) {
super(Orkut.this, "POST", REST_PATH, content, com.google.api.services.orkut.model.CommunityPollVote.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.pollId = com.google.api.client.util.Preconditions.checkNotNull(pollId, "Required parameter pollId must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the community whose poll is being voted. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose poll is being voted.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose poll is being voted. */
public Insert setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the poll being voted. */
@com.google.api.client.util.Key
private java.lang.String pollId;
/** The ID of the poll being voted.
*/
public java.lang.String getPollId() {
return pollId;
}
/** The ID of the poll being voted. */
public Insert setPollId(java.lang.String pollId) {
this.pollId = pollId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityPolls collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityPolls.List request = orkut.communityPolls().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityPolls communityPolls() {
return new CommunityPolls();
}
/**
* The "communityPolls" collection of methods.
*/
public class CommunityPolls {
/**
* Retrieves one specific poll of a community.
*
* Create a request for the method "communityPolls.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community for whose poll will be retrieved.
* @param pollId The ID of the poll to get.
* @return the request
*/
public Get get(java.lang.Integer communityId, java.lang.String pollId) throws java.io.IOException {
Get result = new Get(communityId, pollId);
initialize(result);
return result;
}
public class Get extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/polls/{pollId}";
/**
* Retrieves one specific poll of a community.
*
* Create a request for the method "communityPolls.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community for whose poll will be retrieved.
* @param pollId The ID of the poll to get.
* @since 1.13
*/
protected Get(java.lang.Integer communityId, java.lang.String pollId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityPoll.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.pollId = com.google.api.client.util.Preconditions.checkNotNull(pollId, "Required parameter pollId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the community for whose poll will be retrieved. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community for whose poll will be retrieved.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community for whose poll will be retrieved. */
public Get setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the poll to get. */
@com.google.api.client.util.Key
private java.lang.String pollId;
/** The ID of the poll to get.
*/
public java.lang.String getPollId() {
return pollId;
}
/** The ID of the poll to get. */
public Get setPollId(java.lang.String pollId) {
this.pollId = pollId;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public Get setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the polls of a community.
*
* Create a request for the method "communityPolls.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community which polls will be listed.
* @return the request
*/
public List list(java.lang.Integer communityId) throws java.io.IOException {
List result = new List(communityId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/polls";
/**
* Retrieves the polls of a community.
*
* Create a request for the method "communityPolls.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community which polls will be listed.
* @since 1.13
*/
protected List(java.lang.Integer communityId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityPollList.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the community which polls will be listed. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community which polls will be listed.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community which polls will be listed. */
public List setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** A continuation token that allows pagination. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token that allows pagination.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A continuation token that allows pagination. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The maximum number of polls to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of polls to include in the response.
[minimum: 1]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of polls to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityRelated collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityRelated.List request = orkut.communityRelated().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityRelated communityRelated() {
return new CommunityRelated();
}
/**
* The "communityRelated" collection of methods.
*/
public class CommunityRelated {
/**
* Retrieves the communities related to another one.
*
* Create a request for the method "communityRelated.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose related communities will be listed.
* @return the request
*/
public List list(java.lang.Integer communityId) throws java.io.IOException {
List result = new List(communityId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/related";
/**
* Retrieves the communities related to another one.
*
* Create a request for the method "communityRelated.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose related communities will be listed.
* @since 1.13
*/
protected List(java.lang.Integer communityId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityList.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the community whose related communities will be listed. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose related communities will be listed.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose related communities will be listed. */
public List setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CommunityTopics collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.CommunityTopics.List request = orkut.communityTopics().list(parameters ...)}
*
*
* @return the resource collection
*/
public CommunityTopics communityTopics() {
return new CommunityTopics();
}
/**
* The "communityTopics" collection of methods.
*/
public class CommunityTopics {
/**
* Moves a topic of the community to the trash folder.
*
* Create a request for the method "communityTopics.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose topic will be moved to the trash folder.
* @param topicId The ID of the topic to be moved to the trash folder.
* @return the request
*/
public Delete delete(java.lang.Integer communityId, java.lang.Long topicId) throws java.io.IOException {
Delete result = new Delete(communityId, topicId);
initialize(result);
return result;
}
public class Delete extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/topics/{topicId}";
/**
* Moves a topic of the community to the trash folder.
*
* Create a request for the method "communityTopics.delete".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose topic will be moved to the trash folder.
* @param topicId The ID of the topic to be moved to the trash folder.
* @since 1.13
*/
protected Delete(java.lang.Integer communityId, java.lang.Long topicId) {
super(Orkut.this, "DELETE", REST_PATH, null, Void.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.topicId = com.google.api.client.util.Preconditions.checkNotNull(topicId, "Required parameter topicId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/** The ID of the community whose topic will be moved to the trash folder. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose topic will be moved to the trash folder.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose topic will be moved to the trash folder. */
public Delete setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the topic to be moved to the trash folder. */
@com.google.api.client.util.Key
private java.lang.Long topicId;
/** The ID of the topic to be moved to the trash folder.
*/
public java.lang.Long getTopicId() {
return topicId;
}
/** The ID of the topic to be moved to the trash folder. */
public Delete setTopicId(java.lang.Long topicId) {
this.topicId = topicId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a topic of a community.
*
* Create a request for the method "communityTopics.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community whose topic will be retrieved.
* @param topicId The ID of the topic to get.
* @return the request
*/
public Get get(java.lang.Integer communityId, java.lang.Long topicId) throws java.io.IOException {
Get result = new Get(communityId, topicId);
initialize(result);
return result;
}
public class Get extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/topics/{topicId}";
/**
* Retrieves a topic of a community.
*
* Create a request for the method "communityTopics.get".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community whose topic will be retrieved.
* @param topicId The ID of the topic to get.
* @since 1.13
*/
protected Get(java.lang.Integer communityId, java.lang.Long topicId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityTopic.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
this.topicId = com.google.api.client.util.Preconditions.checkNotNull(topicId, "Required parameter topicId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the community whose topic will be retrieved. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community whose topic will be retrieved.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community whose topic will be retrieved. */
public Get setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** The ID of the topic to get. */
@com.google.api.client.util.Key
private java.lang.Long topicId;
/** The ID of the topic to get.
*/
public java.lang.Long getTopicId() {
return topicId;
}
/** The ID of the topic to get. */
public Get setTopicId(java.lang.Long topicId) {
this.topicId = topicId;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public Get setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Adds a topic to a given community.
*
* Create a request for the method "communityTopics.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community the topic should be added to.
* @param content the {@link com.google.api.services.orkut.model.CommunityTopic}
* @return the request
*/
public Insert insert(java.lang.Integer communityId, com.google.api.services.orkut.model.CommunityTopic content) throws java.io.IOException {
Insert result = new Insert(communityId, content);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/topics";
/**
* Adds a topic to a given community.
*
* Create a request for the method "communityTopics.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community the topic should be added to.
* @param content the {@link com.google.api.services.orkut.model.CommunityTopic}
* @since 1.13
*/
protected Insert(java.lang.Integer communityId, com.google.api.services.orkut.model.CommunityTopic content) {
super(Orkut.this, "POST", REST_PATH, content, com.google.api.services.orkut.model.CommunityTopic.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** The ID of the community the topic should be added to. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community the topic should be added to.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community the topic should be added to. */
public Insert setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** Whether this topic is a shout. */
@com.google.api.client.util.Key
private java.lang.Boolean isShout;
/** Whether this topic is a shout.
*/
public java.lang.Boolean getIsShout() {
return isShout;
}
/** Whether this topic is a shout. */
public Insert setIsShout(java.lang.Boolean isShout) {
this.isShout = isShout;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the topics of a community.
*
* Create a request for the method "communityTopics.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param communityId The ID of the community which topics will be listed.
* @return the request
*/
public List list(java.lang.Integer communityId) throws java.io.IOException {
List result = new List(communityId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "communities/{communityId}/topics";
/**
* Retrieves the topics of a community.
*
* Create a request for the method "communityTopics.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param communityId The ID of the community which topics will be listed.
* @since 1.13
*/
protected List(java.lang.Integer communityId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.CommunityTopicList.class);
this.communityId = com.google.api.client.util.Preconditions.checkNotNull(communityId, "Required parameter communityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the community which topics will be listed. */
@com.google.api.client.util.Key
private java.lang.Integer communityId;
/** The ID of the community which topics will be listed.
*/
public java.lang.Integer getCommunityId() {
return communityId;
}
/** The ID of the community which topics will be listed. */
public List setCommunityId(java.lang.Integer communityId) {
this.communityId = communityId;
return this;
}
/** A continuation token that allows pagination. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token that allows pagination.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A continuation token that allows pagination. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The maximum number of topics to include in the response. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of topics to include in the response.
[minimum: 1] [maximum: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of topics to include in the response. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies the interface language (host language) of your user interface. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Specifies the interface language (host language) of your user interface.
*/
public java.lang.String getHl() {
return hl;
}
/** Specifies the interface language (host language) of your user interface. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Counters collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.Counters.List request = orkut.counters().list(parameters ...)}
*
*
* @return the resource collection
*/
public Counters counters() {
return new Counters();
}
/**
* The "counters" collection of methods.
*/
public class Counters {
/**
* Retrieves the counters of a user.
*
* Create a request for the method "counters.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The ID of the user whose counters will be listed. Can be me to refer to caller.
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends OrkutRequest {
private static final String REST_PATH = "people/{userId}/counters";
/**
* Retrieves the counters of a user.
*
* Create a request for the method "counters.list".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The ID of the user whose counters will be listed. Can be me to refer to caller.
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Orkut.this, "GET", REST_PATH, null, com.google.api.services.orkut.model.Counters.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the user whose counters will be listed. Can be me to refer to caller. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user whose counters will be listed. Can be me to refer to caller.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user whose counters will be listed. Can be me to refer to caller. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Scraps collection.
*
* The typical use is:
*
* {@code Orkut orkut = new Orkut(...);}
* {@code Orkut.Scraps.List request = orkut.scraps().list(parameters ...)}
*
*
* @return the resource collection
*/
public Scraps scraps() {
return new Scraps();
}
/**
* The "scraps" collection of methods.
*/
public class Scraps {
/**
* Creates a new scrap.
*
* Create a request for the method "scraps.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.orkut.model.Activity}
* @return the request
*/
public Insert insert(com.google.api.services.orkut.model.Activity content) throws java.io.IOException {
Insert result = new Insert(content);
initialize(result);
return result;
}
public class Insert extends OrkutRequest {
private static final String REST_PATH = "activities/scraps";
/**
* Creates a new scrap.
*
* Create a request for the method "scraps.insert".
*
* This request holds the parameters needed by the the orkut server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.orkut.model.Activity}
* @since 1.13
*/
protected Insert(com.google.api.services.orkut.model.Activity content) {
super(Orkut.this, "POST", REST_PATH, content, com.google.api.services.orkut.model.Activity.class);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Orkut}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Orkut}. */
@Override
public Orkut build() {
return new Orkut(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link OrkutRequestInitializer}.
*
* @since 1.12
*/
public Builder setOrkutRequestInitializer(
OrkutRequestInitializer orkutRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(orkutRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}