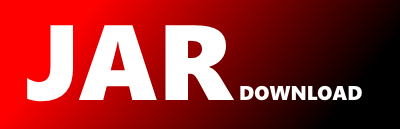
com.google.api.services.orkut.model.Activity Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2013-08-01 15:32:38 UTC)
* on 2013-08-06 at 21:02:08 UTC
* Modify at your own risk.
*/
package com.google.api.services.orkut.model;
/**
* Model definition for Activity.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Orkut API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Activity extends com.google.api.client.json.GenericJson {
/**
* Identifies who has access to see this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Acl access;
/**
* The person who performed the activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private OrkutAuthorResource actor;
/**
* The ID for the activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The kind of activity. Always orkut#activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Links to resources related to this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List links;
static {
// hack to force ProGuard to consider OrkutLinkResource used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(OrkutLinkResource.class);
}
/**
* The activity's object.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("object")
private OrkutObject orkutObject;
/**
* The time at which the activity was initially published.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime published;
/**
* Title of the activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The time at which the activity was last updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime updated;
/**
* This activity's verb, indicating what action was performed. Possible values are: - add - User
* added new content to profile or album, e.g. video, photo. - post - User publish content to the
* stream, e.g. status, scrap. - update - User commented on an activity. - make-friend - User
* added a new friend. - birthday - User has a birthday.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String verb;
/**
* Identifies who has access to see this activity.
* @return value or {@code null} for none
*/
public Acl getAccess() {
return access;
}
/**
* Identifies who has access to see this activity.
* @param access access or {@code null} for none
*/
public Activity setAccess(Acl access) {
this.access = access;
return this;
}
/**
* The person who performed the activity.
* @return value or {@code null} for none
*/
public OrkutAuthorResource getActor() {
return actor;
}
/**
* The person who performed the activity.
* @param actor actor or {@code null} for none
*/
public Activity setActor(OrkutAuthorResource actor) {
this.actor = actor;
return this;
}
/**
* The ID for the activity.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The ID for the activity.
* @param id id or {@code null} for none
*/
public Activity setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The kind of activity. Always orkut#activity.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* The kind of activity. Always orkut#activity.
* @param kind kind or {@code null} for none
*/
public Activity setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Links to resources related to this activity.
* @return value or {@code null} for none
*/
public java.util.List getLinks() {
return links;
}
/**
* Links to resources related to this activity.
* @param links links or {@code null} for none
*/
public Activity setLinks(java.util.List links) {
this.links = links;
return this;
}
/**
* The activity's object.
* @return value or {@code null} for none
*/
public OrkutObject getObject() {
return orkutObject;
}
/**
* The activity's object.
* @param orkutObject orkutObject or {@code null} for none
*/
public Activity setObject(OrkutObject orkutObject) {
this.orkutObject = orkutObject;
return this;
}
/**
* The time at which the activity was initially published.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getPublished() {
return published;
}
/**
* The time at which the activity was initially published.
* @param published published or {@code null} for none
*/
public Activity setPublished(com.google.api.client.util.DateTime published) {
this.published = published;
return this;
}
/**
* Title of the activity.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Title of the activity.
* @param title title or {@code null} for none
*/
public Activity setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* The time at which the activity was last updated.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getUpdated() {
return updated;
}
/**
* The time at which the activity was last updated.
* @param updated updated or {@code null} for none
*/
public Activity setUpdated(com.google.api.client.util.DateTime updated) {
this.updated = updated;
return this;
}
/**
* This activity's verb, indicating what action was performed. Possible values are: - add - User
* added new content to profile or album, e.g. video, photo. - post - User publish content to the
* stream, e.g. status, scrap. - update - User commented on an activity. - make-friend - User
* added a new friend. - birthday - User has a birthday.
* @return value or {@code null} for none
*/
public java.lang.String getVerb() {
return verb;
}
/**
* This activity's verb, indicating what action was performed. Possible values are: - add - User
* added new content to profile or album, e.g. video, photo. - post - User publish content to the
* stream, e.g. status, scrap. - update - User commented on an activity. - make-friend - User
* added a new friend. - birthday - User has a birthday.
* @param verb verb or {@code null} for none
*/
public Activity setVerb(java.lang.String verb) {
this.verb = verb;
return this;
}
@Override
public Activity set(String fieldName, Object value) {
return (Activity) super.set(fieldName, value);
}
@Override
public Activity clone() {
return (Activity) super.clone();
}
/**
* The activity's object.
*/
public static final class OrkutObject extends com.google.api.client.json.GenericJson {
/**
* The HTML-formatted content, suitable for display. When updating an activity's content, post the
* changes to this property, using the value of originalContent as a starting point. If the update
* is successful, the server adds HTML formatting and responds with this formatted content.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String content;
/**
* The list of additional items.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List items;
static {
// hack to force ProGuard to consider OrkutActivityobjectsResource used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(OrkutActivityobjectsResource.class);
}
/**
* The type of the object affected by the activity. Clients can use this information to style the
* rendered activity object differently depending on the content.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String objectType;
/**
* Comments in reply to this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Replies replies;
/**
* The HTML-formatted content, suitable for display. When updating an activity's content, post the
* changes to this property, using the value of originalContent as a starting point. If the update
* is successful, the server adds HTML formatting and responds with this formatted content.
* @return value or {@code null} for none
*/
public java.lang.String getContent() {
return content;
}
/**
* The HTML-formatted content, suitable for display. When updating an activity's content, post the
* changes to this property, using the value of originalContent as a starting point. If the update
* is successful, the server adds HTML formatting and responds with this formatted content.
* @param content content or {@code null} for none
*/
public OrkutObject setContent(java.lang.String content) {
this.content = content;
return this;
}
/**
* The list of additional items.
* @return value or {@code null} for none
*/
public java.util.List getItems() {
return items;
}
/**
* The list of additional items.
* @param items items or {@code null} for none
*/
public OrkutObject setItems(java.util.List items) {
this.items = items;
return this;
}
/**
* The type of the object affected by the activity. Clients can use this information to style the
* rendered activity object differently depending on the content.
* @return value or {@code null} for none
*/
public java.lang.String getObjectType() {
return objectType;
}
/**
* The type of the object affected by the activity. Clients can use this information to style the
* rendered activity object differently depending on the content.
* @param objectType objectType or {@code null} for none
*/
public OrkutObject setObjectType(java.lang.String objectType) {
this.objectType = objectType;
return this;
}
/**
* Comments in reply to this activity.
* @return value or {@code null} for none
*/
public Replies getReplies() {
return replies;
}
/**
* Comments in reply to this activity.
* @param replies replies or {@code null} for none
*/
public OrkutObject setReplies(Replies replies) {
this.replies = replies;
return this;
}
@Override
public OrkutObject set(String fieldName, Object value) {
return (OrkutObject) super.set(fieldName, value);
}
@Override
public OrkutObject clone() {
return (OrkutObject) super.clone();
}
/**
* Comments in reply to this activity.
*/
public static final class Replies extends com.google.api.client.json.GenericJson {
/**
* The list of comments.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List items;
/**
* Total number of comments.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger totalItems;
/**
* URL for the collection of comments in reply to this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The list of comments.
* @return value or {@code null} for none
*/
public java.util.List getItems() {
return items;
}
/**
* The list of comments.
* @param items items or {@code null} for none
*/
public Replies setItems(java.util.List items) {
this.items = items;
return this;
}
/**
* Total number of comments.
* @return value or {@code null} for none
*/
public java.math.BigInteger getTotalItems() {
return totalItems;
}
/**
* Total number of comments.
* @param totalItems totalItems or {@code null} for none
*/
public Replies setTotalItems(java.math.BigInteger totalItems) {
this.totalItems = totalItems;
return this;
}
/**
* URL for the collection of comments in reply to this activity.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* URL for the collection of comments in reply to this activity.
* @param url url or {@code null} for none
*/
public Replies setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Replies set(String fieldName, Object value) {
return (Replies) super.set(fieldName, value);
}
@Override
public Replies clone() {
return (Replies) super.clone();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy