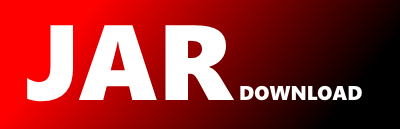
com.google.api.services.paymentsresellersubscription.v1.PaymentsResellerSubscription Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.paymentsresellersubscription.v1;
/**
* Service definition for PaymentsResellerSubscription (v1).
*
*
*
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link PaymentsResellerSubscriptionRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class PaymentsResellerSubscription extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Payments Reseller Subscription API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://paymentsresellersubscription.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://paymentsresellersubscription.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public PaymentsResellerSubscription(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
PaymentsResellerSubscription(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Partners collection.
*
* The typical use is:
*
* {@code PaymentsResellerSubscription paymentsresellersubscription = new PaymentsResellerSubscription(...);}
* {@code PaymentsResellerSubscription.Partners.List request = paymentsresellersubscription.partners().list(parameters ...)}
*
*
* @return the resource collection
*/
public Partners partners() {
return new Partners();
}
/**
* The "partners" collection of methods.
*/
public class Partners {
/**
* An accessor for creating requests from the Products collection.
*
* The typical use is:
*
* {@code PaymentsResellerSubscription paymentsresellersubscription = new PaymentsResellerSubscription(...);}
* {@code PaymentsResellerSubscription.Products.List request = paymentsresellersubscription.products().list(parameters ...)}
*
*
* @return the resource collection
*/
public Products products() {
return new Products();
}
/**
* The "products" collection of methods.
*/
public class Products {
/**
* To retrieve the products that can be resold by the partner. It should be autenticated with a
* service account. - This API doesn't apply to YouTube products currently.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+parent}/products";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* To retrieve the products that can be resold by the partner. It should be autenticated with a
* service account. - This API doesn't apply to YouTube products currently.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(PaymentsResellerSubscription.this, "GET", REST_PATH, null, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1ListProductsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent, the partner that can resell. Format: partners/{partner}
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Specifies the filters for the product results. The syntax is defined in
* https://google.aip.dev/160 with the following caveats: 1. Only the following features are
* supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) -
* Traversal operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields
* are supported: - `regionCodes` - `youtubePayload.partnerEligibilityId` -
* `youtubePayload.postalCode` 3. Unless explicitly mentioned above, other features are not
* supported. Example: `regionCodes:US AND youtubePayload.postalCode=94043 AND
* youtubePayload.partnerEligibilityId=eligibility-id`
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Specifies the filters for the product results. The syntax is defined in
https://google.aip.dev/160 with the following caveats: 1. Only the following features are
supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) - Traversal
operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields are supported: -
`regionCodes` - `youtubePayload.partnerEligibilityId` - `youtubePayload.postalCode` 3. Unless
explicitly mentioned above, other features are not supported. Example: `regionCodes:US AND
youtubePayload.postalCode=94043 AND youtubePayload.partnerEligibilityId=eligibility-id`
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Specifies the filters for the product results. The syntax is defined in
* https://google.aip.dev/160 with the following caveats: 1. Only the following features are
* supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) -
* Traversal operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields
* are supported: - `regionCodes` - `youtubePayload.partnerEligibilityId` -
* `youtubePayload.postalCode` 3. Unless explicitly mentioned above, other features are not
* supported. Example: `regionCodes:US AND youtubePayload.postalCode=94043 AND
* youtubePayload.partnerEligibilityId=eligibility-id`
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of products to return. The service may return fewer than
* this value. If unspecified, at most 50 products will be returned. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of products to return. The service may return fewer than this value.
If unspecified, at most 50 products will be returned. The maximum value is 1000; values above 1000
will be coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of products to return. The service may return fewer than
* this value. If unspecified, at most 50 products will be returned. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListProducts` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListProducts` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListProducts` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListProducts` must match the
call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListProducts` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListProducts` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Promotions collection.
*
* The typical use is:
*
* {@code PaymentsResellerSubscription paymentsresellersubscription = new PaymentsResellerSubscription(...);}
* {@code PaymentsResellerSubscription.Promotions.List request = paymentsresellersubscription.promotions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Promotions promotions() {
return new Promotions();
}
/**
* The "promotions" collection of methods.
*/
public class Promotions {
/**
* To find eligible promotions for the current user. The API requires user authorization via OAuth.
* The bare minimum oauth scope `openid` is sufficient, which will skip the consent screen.
*
* Create a request for the method "promotions.findEligible".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link FindEligible#execute()} method to invoke the
* remote operation.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest}
* @return the request
*/
public FindEligible findEligible(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest content) throws java.io.IOException {
FindEligible result = new FindEligible(parent, content);
initialize(result);
return result;
}
public class FindEligible extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+parent}/promotions:findEligible";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* To find eligible promotions for the current user. The API requires user authorization via
* OAuth. The bare minimum oauth scope `openid` is sufficient, which will skip the consent screen.
*
* Create a request for the method "promotions.findEligible".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link FindEligible#execute()} method to invoke the
* remote operation. {@link
* FindEligible#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest}
* @since 1.13
*/
protected FindEligible(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public FindEligible set$Xgafv(java.lang.String $Xgafv) {
return (FindEligible) super.set$Xgafv($Xgafv);
}
@Override
public FindEligible setAccessToken(java.lang.String accessToken) {
return (FindEligible) super.setAccessToken(accessToken);
}
@Override
public FindEligible setAlt(java.lang.String alt) {
return (FindEligible) super.setAlt(alt);
}
@Override
public FindEligible setCallback(java.lang.String callback) {
return (FindEligible) super.setCallback(callback);
}
@Override
public FindEligible setFields(java.lang.String fields) {
return (FindEligible) super.setFields(fields);
}
@Override
public FindEligible setKey(java.lang.String key) {
return (FindEligible) super.setKey(key);
}
@Override
public FindEligible setOauthToken(java.lang.String oauthToken) {
return (FindEligible) super.setOauthToken(oauthToken);
}
@Override
public FindEligible setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FindEligible) super.setPrettyPrint(prettyPrint);
}
@Override
public FindEligible setQuotaUser(java.lang.String quotaUser) {
return (FindEligible) super.setQuotaUser(quotaUser);
}
@Override
public FindEligible setUploadType(java.lang.String uploadType) {
return (FindEligible) super.setUploadType(uploadType);
}
@Override
public FindEligible setUploadProtocol(java.lang.String uploadProtocol) {
return (FindEligible) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent, the partner that can resell. Format: partners/{partner}
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
public FindEligible setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public FindEligible set(String parameterName, Object value) {
return (FindEligible) super.set(parameterName, value);
}
}
/**
* Retrieves the promotions, such as free trial, that can be used by the partner. - This API doesn't
* apply to YouTube promotions currently. It should be autenticated with a service account.
*
* Create a request for the method "promotions.list".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+parent}/promotions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* Retrieves the promotions, such as free trial, that can be used by the partner. - This API
* doesn't apply to YouTube promotions currently. It should be autenticated with a service
* account.
*
* Create a request for the method "promotions.list".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(PaymentsResellerSubscription.this, "GET", REST_PATH, null, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1ListPromotionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent, the partner that can resell. Format: partners/{partner}
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Specifies the filters for the promotion results. The syntax is defined in
* https://google.aip.dev/160 with the following caveats: 1. Only the following features are
* supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) -
* Traversal operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields
* are supported: - `applicableProducts` - `regionCodes` -
* `youtubePayload.partnerEligibilityId` - `youtubePayload.postalCode` 3. Unless explicitly
* mentioned above, other features are not supported. Example:
* `applicableProducts:partners/partner1/products/product1 AND regionCodes:US AND
* youtubePayload.postalCode=94043 AND youtubePayload.partnerEligibilityId=eligibility-id`
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Specifies the filters for the promotion results. The syntax is defined in
https://google.aip.dev/160 with the following caveats: 1. Only the following features are
supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) - Traversal
operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields are supported: -
`applicableProducts` - `regionCodes` - `youtubePayload.partnerEligibilityId` -
`youtubePayload.postalCode` 3. Unless explicitly mentioned above, other features are not supported.
Example: `applicableProducts:partners/partner1/products/product1 AND regionCodes:US AND
youtubePayload.postalCode=94043 AND youtubePayload.partnerEligibilityId=eligibility-id`
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Specifies the filters for the promotion results. The syntax is defined in
* https://google.aip.dev/160 with the following caveats: 1. Only the following features are
* supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) -
* Traversal operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields
* are supported: - `applicableProducts` - `regionCodes` -
* `youtubePayload.partnerEligibilityId` - `youtubePayload.postalCode` 3. Unless explicitly
* mentioned above, other features are not supported. Example:
* `applicableProducts:partners/partner1/products/product1 AND regionCodes:US AND
* youtubePayload.postalCode=94043 AND youtubePayload.partnerEligibilityId=eligibility-id`
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of promotions to return. The service may return fewer than
* this value. If unspecified, at most 50 products will be returned. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of promotions to return. The service may return fewer than this value.
If unspecified, at most 50 products will be returned. The maximum value is 1000; values above 1000
will be coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of promotions to return. The service may return fewer than
* this value. If unspecified, at most 50 products will be returned. The maximum value is
* 1000; values above 1000 will be coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListPromotions` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPromotions` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListPromotions` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListPromotions` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListPromotions` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPromotions` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Subscriptions collection.
*
* The typical use is:
*
* {@code PaymentsResellerSubscription paymentsresellersubscription = new PaymentsResellerSubscription(...);}
* {@code PaymentsResellerSubscription.Subscriptions.List request = paymentsresellersubscription.subscriptions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Subscriptions subscriptions() {
return new Subscriptions();
}
/**
* The "subscriptions" collection of methods.
*/
public class Subscriptions {
/**
* Used by partners to cancel a subscription service either immediately or by the end of the current
* billing cycle for their customers. It should be called directly by the partner using service
* accounts.
*
* Create a request for the method "subscriptions.cancel".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Cancel#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the subscription resource to be cancelled. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1CancelSubscriptionRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1CancelSubscriptionRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+/subscriptions/[^/]+$");
/**
* Used by partners to cancel a subscription service either immediately or by the end of the
* current billing cycle for their customers. It should be called directly by the partner using
* service accounts.
*
* Create a request for the method "subscriptions.cancel".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Cancel#execute()} method to invoke the remote
* operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the subscription resource to be cancelled. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1CancelSubscriptionRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1CancelSubscriptionRequest content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1CancelSubscriptionResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the subscription resource to be cancelled. It will have the format
* of "partners/{partner_id}/subscriptions/{subscription_id}"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the subscription resource to be cancelled. It will have the format of
"partners/{partner_id}/subscriptions/{subscription_id}"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the subscription resource to be cancelled. It will have the format
* of "partners/{partner_id}/subscriptions/{subscription_id}"
*/
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Used by partners to create a subscription for their customers. The created subscription is
* associated with the end user inferred from the end user credentials. This API must be authorized
* by the end user using OAuth.
*
* Create a request for the method "subscriptions.create".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The parent resource name, which is the identifier of the partner. It will have the format
* of "partners/{partner_id}".
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+parent}/subscriptions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* Used by partners to create a subscription for their customers. The created subscription is
* associated with the end user inferred from the end user credentials. This API must be
* authorized by the end user using OAuth.
*
* Create a request for the method "subscriptions.create".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource name, which is the identifier of the partner. It will have the format
* of "partners/{partner_id}".
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource name, which is the identifier of the partner. It will have
* the format of "partners/{partner_id}".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource name, which is the identifier of the partner. It will have the format
of "partners/{partner_id}".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource name, which is the identifier of the partner. It will have
* the format of "partners/{partner_id}".
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. Identifies the subscription resource on the Partner side. The value is
* restricted to 63 ASCII characters at the maximum. If a subscription was previously
* created with the same subscription_id, we will directly return that one.
*/
@com.google.api.client.util.Key
private java.lang.String subscriptionId;
/** Required. Identifies the subscription resource on the Partner side. The value is restricted to 63
ASCII characters at the maximum. If a subscription was previously created with the same
subscription_id, we will directly return that one.
*/
public java.lang.String getSubscriptionId() {
return subscriptionId;
}
/**
* Required. Identifies the subscription resource on the Partner side. The value is
* restricted to 63 ASCII characters at the maximum. If a subscription was previously
* created with the same subscription_id, we will directly return that one.
*/
public Create setSubscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Used by partners to entitle a previously provisioned subscription to the current end user. The
* end user identity is inferred from the authorized credential of the request. This API must be
* authorized by the end user using OAuth.
*
* Create a request for the method "subscriptions.entitle".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Entitle#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the subscription resource that is entitled to the current end user. It will
* have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1EntitleSubscriptionRequest}
* @return the request
*/
public Entitle entitle(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1EntitleSubscriptionRequest content) throws java.io.IOException {
Entitle result = new Entitle(name, content);
initialize(result);
return result;
}
public class Entitle extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+name}:entitle";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+/subscriptions/[^/]+$");
/**
* Used by partners to entitle a previously provisioned subscription to the current end user. The
* end user identity is inferred from the authorized credential of the request. This API must be
* authorized by the end user using OAuth.
*
* Create a request for the method "subscriptions.entitle".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Entitle#execute()} method to invoke the remote
* operation. {@link
* Entitle#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the subscription resource that is entitled to the current end user. It will
* have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1EntitleSubscriptionRequest}
* @since 1.13
*/
protected Entitle(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1EntitleSubscriptionRequest content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1EntitleSubscriptionResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
}
@Override
public Entitle set$Xgafv(java.lang.String $Xgafv) {
return (Entitle) super.set$Xgafv($Xgafv);
}
@Override
public Entitle setAccessToken(java.lang.String accessToken) {
return (Entitle) super.setAccessToken(accessToken);
}
@Override
public Entitle setAlt(java.lang.String alt) {
return (Entitle) super.setAlt(alt);
}
@Override
public Entitle setCallback(java.lang.String callback) {
return (Entitle) super.setCallback(callback);
}
@Override
public Entitle setFields(java.lang.String fields) {
return (Entitle) super.setFields(fields);
}
@Override
public Entitle setKey(java.lang.String key) {
return (Entitle) super.setKey(key);
}
@Override
public Entitle setOauthToken(java.lang.String oauthToken) {
return (Entitle) super.setOauthToken(oauthToken);
}
@Override
public Entitle setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Entitle) super.setPrettyPrint(prettyPrint);
}
@Override
public Entitle setQuotaUser(java.lang.String quotaUser) {
return (Entitle) super.setQuotaUser(quotaUser);
}
@Override
public Entitle setUploadType(java.lang.String uploadType) {
return (Entitle) super.setUploadType(uploadType);
}
@Override
public Entitle setUploadProtocol(java.lang.String uploadProtocol) {
return (Entitle) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the subscription resource that is entitled to the current end user.
* It will have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the subscription resource that is entitled to the current end user. It will
have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the subscription resource that is entitled to the current end user.
* It will have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
*/
public Entitle setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Entitle set(String parameterName, Object value) {
return (Entitle) super.set(parameterName, value);
}
}
/**
* [Opt-in only] Most partners should be on auto-extend by default. Used by partners to extend a
* subscription service for their customers on an ongoing basis for the subscription to remain
* active and renewable. It should be called directly by the partner using service accounts.
*
* Create a request for the method "subscriptions.extend".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Extend#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the subscription resource to be extended. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}".
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1ExtendSubscriptionRequest}
* @return the request
*/
public Extend extend(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1ExtendSubscriptionRequest content) throws java.io.IOException {
Extend result = new Extend(name, content);
initialize(result);
return result;
}
public class Extend extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+name}:extend";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+/subscriptions/[^/]+$");
/**
* [Opt-in only] Most partners should be on auto-extend by default. Used by partners to extend a
* subscription service for their customers on an ongoing basis for the subscription to remain
* active and renewable. It should be called directly by the partner using service accounts.
*
* Create a request for the method "subscriptions.extend".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Extend#execute()} method to invoke the remote
* operation. {@link
* Extend#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the subscription resource to be extended. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}".
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1ExtendSubscriptionRequest}
* @since 1.13
*/
protected Extend(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1ExtendSubscriptionRequest content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1ExtendSubscriptionResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
}
@Override
public Extend set$Xgafv(java.lang.String $Xgafv) {
return (Extend) super.set$Xgafv($Xgafv);
}
@Override
public Extend setAccessToken(java.lang.String accessToken) {
return (Extend) super.setAccessToken(accessToken);
}
@Override
public Extend setAlt(java.lang.String alt) {
return (Extend) super.setAlt(alt);
}
@Override
public Extend setCallback(java.lang.String callback) {
return (Extend) super.setCallback(callback);
}
@Override
public Extend setFields(java.lang.String fields) {
return (Extend) super.setFields(fields);
}
@Override
public Extend setKey(java.lang.String key) {
return (Extend) super.setKey(key);
}
@Override
public Extend setOauthToken(java.lang.String oauthToken) {
return (Extend) super.setOauthToken(oauthToken);
}
@Override
public Extend setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Extend) super.setPrettyPrint(prettyPrint);
}
@Override
public Extend setQuotaUser(java.lang.String quotaUser) {
return (Extend) super.setQuotaUser(quotaUser);
}
@Override
public Extend setUploadType(java.lang.String uploadType) {
return (Extend) super.setUploadType(uploadType);
}
@Override
public Extend setUploadProtocol(java.lang.String uploadProtocol) {
return (Extend) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the subscription resource to be extended. It will have the format
* of "partners/{partner_id}/subscriptions/{subscription_id}".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the subscription resource to be extended. It will have the format of
"partners/{partner_id}/subscriptions/{subscription_id}".
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the subscription resource to be extended. It will have the format
* of "partners/{partner_id}/subscriptions/{subscription_id}".
*/
public Extend setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Extend set(String parameterName, Object value) {
return (Extend) super.set(parameterName, value);
}
}
/**
* Used by partners to get a subscription by id. It should be called directly by the partner using
* service accounts.
*
* Create a request for the method "subscriptions.get".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the subscription resource to retrieve. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+/subscriptions/[^/]+$");
/**
* Used by partners to get a subscription by id. It should be called directly by the partner using
* service accounts.
*
* Create a request for the method "subscriptions.get".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the subscription resource to retrieve. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(PaymentsResellerSubscription.this, "GET", REST_PATH, null, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the subscription resource to retrieve. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the subscription resource to retrieve. It will have the format of
"partners/{partner_id}/subscriptions/{subscription_id}"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the subscription resource to retrieve. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Used by partners to provision a subscription for their customers. This creates a subscription
* without associating it with the end user account. EntitleSubscription must be called separately
* using OAuth in order for the end user account to be associated with the subscription. It should
* be called directly by the partner using service accounts.
*
* Create a request for the method "subscriptions.provision".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Provision#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The parent resource name, which is the identifier of the partner. It will have the format
* of "partners/{partner_id}".
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription}
* @return the request
*/
public Provision provision(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription content) throws java.io.IOException {
Provision result = new Provision(parent, content);
initialize(result);
return result;
}
public class Provision extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+parent}/subscriptions:provision";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* Used by partners to provision a subscription for their customers. This creates a subscription
* without associating it with the end user account. EntitleSubscription must be called separately
* using OAuth in order for the end user account to be associated with the subscription. It should
* be called directly by the partner using service accounts.
*
* Create a request for the method "subscriptions.provision".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Provision#execute()} method to invoke the
* remote operation. {@link
* Provision#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource name, which is the identifier of the partner. It will have the format
* of "partners/{partner_id}".
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription}
* @since 1.13
*/
protected Provision(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1Subscription.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public Provision set$Xgafv(java.lang.String $Xgafv) {
return (Provision) super.set$Xgafv($Xgafv);
}
@Override
public Provision setAccessToken(java.lang.String accessToken) {
return (Provision) super.setAccessToken(accessToken);
}
@Override
public Provision setAlt(java.lang.String alt) {
return (Provision) super.setAlt(alt);
}
@Override
public Provision setCallback(java.lang.String callback) {
return (Provision) super.setCallback(callback);
}
@Override
public Provision setFields(java.lang.String fields) {
return (Provision) super.setFields(fields);
}
@Override
public Provision setKey(java.lang.String key) {
return (Provision) super.setKey(key);
}
@Override
public Provision setOauthToken(java.lang.String oauthToken) {
return (Provision) super.setOauthToken(oauthToken);
}
@Override
public Provision setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Provision) super.setPrettyPrint(prettyPrint);
}
@Override
public Provision setQuotaUser(java.lang.String quotaUser) {
return (Provision) super.setQuotaUser(quotaUser);
}
@Override
public Provision setUploadType(java.lang.String uploadType) {
return (Provision) super.setUploadType(uploadType);
}
@Override
public Provision setUploadProtocol(java.lang.String uploadProtocol) {
return (Provision) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource name, which is the identifier of the partner. It will have
* the format of "partners/{partner_id}".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource name, which is the identifier of the partner. It will have the format
of "partners/{partner_id}".
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource name, which is the identifier of the partner. It will have
* the format of "partners/{partner_id}".
*/
public Provision setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. Identifies the subscription resource on the Partner side. The value is
* restricted to 63 ASCII characters at the maximum. If a subscription was previously
* created with the same subscription_id, we will directly return that one.
*/
@com.google.api.client.util.Key
private java.lang.String subscriptionId;
/** Required. Identifies the subscription resource on the Partner side. The value is restricted to 63
ASCII characters at the maximum. If a subscription was previously created with the same
subscription_id, we will directly return that one.
*/
public java.lang.String getSubscriptionId() {
return subscriptionId;
}
/**
* Required. Identifies the subscription resource on the Partner side. The value is
* restricted to 63 ASCII characters at the maximum. If a subscription was previously
* created with the same subscription_id, we will directly return that one.
*/
public Provision setSubscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
@Override
public Provision set(String parameterName, Object value) {
return (Provision) super.set(parameterName, value);
}
}
/**
* Revokes the pending cancellation of a subscription, which is currently in
* `STATE_CANCEL_AT_END_OF_CYCLE` state. If the subscription is already cancelled, the request will
* fail. - **This API doesn't apply to YouTube subscriptions.** It should be called directly by the
* partner using service accounts.
*
* Create a request for the method "subscriptions.undoCancel".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link UndoCancel#execute()} method to invoke the
* remote operation.
*
* @param name Required. The name of the subscription resource whose pending cancellation needs to be undone. It
* will have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1UndoCancelSubscriptionRequest}
* @return the request
*/
public UndoCancel undoCancel(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1UndoCancelSubscriptionRequest content) throws java.io.IOException {
UndoCancel result = new UndoCancel(name, content);
initialize(result);
return result;
}
public class UndoCancel extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+name}:undoCancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+/subscriptions/[^/]+$");
/**
* Revokes the pending cancellation of a subscription, which is currently in
* `STATE_CANCEL_AT_END_OF_CYCLE` state. If the subscription is already cancelled, the request
* will fail. - **This API doesn't apply to YouTube subscriptions.** It should be called directly
* by the partner using service accounts.
*
* Create a request for the method "subscriptions.undoCancel".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link UndoCancel#execute()} method to invoke the
* remote operation. {@link
* UndoCancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the subscription resource whose pending cancellation needs to be undone. It
* will have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1UndoCancelSubscriptionRequest}
* @since 1.13
*/
protected UndoCancel(java.lang.String name, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1UndoCancelSubscriptionRequest content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1UndoCancelSubscriptionResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
}
@Override
public UndoCancel set$Xgafv(java.lang.String $Xgafv) {
return (UndoCancel) super.set$Xgafv($Xgafv);
}
@Override
public UndoCancel setAccessToken(java.lang.String accessToken) {
return (UndoCancel) super.setAccessToken(accessToken);
}
@Override
public UndoCancel setAlt(java.lang.String alt) {
return (UndoCancel) super.setAlt(alt);
}
@Override
public UndoCancel setCallback(java.lang.String callback) {
return (UndoCancel) super.setCallback(callback);
}
@Override
public UndoCancel setFields(java.lang.String fields) {
return (UndoCancel) super.setFields(fields);
}
@Override
public UndoCancel setKey(java.lang.String key) {
return (UndoCancel) super.setKey(key);
}
@Override
public UndoCancel setOauthToken(java.lang.String oauthToken) {
return (UndoCancel) super.setOauthToken(oauthToken);
}
@Override
public UndoCancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UndoCancel) super.setPrettyPrint(prettyPrint);
}
@Override
public UndoCancel setQuotaUser(java.lang.String quotaUser) {
return (UndoCancel) super.setQuotaUser(quotaUser);
}
@Override
public UndoCancel setUploadType(java.lang.String uploadType) {
return (UndoCancel) super.setUploadType(uploadType);
}
@Override
public UndoCancel setUploadProtocol(java.lang.String uploadProtocol) {
return (UndoCancel) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the subscription resource whose pending cancellation needs to be
* undone. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the subscription resource whose pending cancellation needs to be undone. It
will have the format of "partners/{partner_id}/subscriptions/{subscription_id}"
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the subscription resource whose pending cancellation needs to be
* undone. It will have the format of
* "partners/{partner_id}/subscriptions/{subscription_id}"
*/
public UndoCancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/subscriptions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public UndoCancel set(String parameterName, Object value) {
return (UndoCancel) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the UserSessions collection.
*
* The typical use is:
*
* {@code PaymentsResellerSubscription paymentsresellersubscription = new PaymentsResellerSubscription(...);}
* {@code PaymentsResellerSubscription.UserSessions.List request = paymentsresellersubscription.userSessions().list(parameters ...)}
*
*
* @return the resource collection
*/
public UserSessions userSessions() {
return new UserSessions();
}
/**
* The "userSessions" collection of methods.
*/
public class UserSessions {
/**
* This API replaces user authorized OAuth consnet based APIs (Create, Entitle). Generates a short-
* lived token for a user session based on the user intent. You can use the session token to
* redirect the user to Google to finish the signup flow. You can re-generate new session token
* repeatedly for same request if necessary, regardless of the previous tokens being expired or not.
*
* Create a request for the method "userSessions.generate".
*
* This request holds the parameters needed by the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Generate#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1GenerateUserSessionRequest}
* @return the request
*/
public Generate generate(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1GenerateUserSessionRequest content) throws java.io.IOException {
Generate result = new Generate(parent, content);
initialize(result);
return result;
}
public class Generate extends PaymentsResellerSubscriptionRequest {
private static final String REST_PATH = "v1/{+parent}/userSessions:generate";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* This API replaces user authorized OAuth consnet based APIs (Create, Entitle). Generates a
* short-lived token for a user session based on the user intent. You can use the session token to
* redirect the user to Google to finish the signup flow. You can re-generate new session token
* repeatedly for same request if necessary, regardless of the previous tokens being expired or
* not.
*
* Create a request for the method "userSessions.generate".
*
* This request holds the parameters needed by the the paymentsresellersubscription server. After
* setting any optional parameters, call the {@link Generate#execute()} method to invoke the
* remote operation. {@link
* Generate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent, the partner that can resell. Format: partners/{partner}
* @param content the {@link com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1GenerateUserSessionRequest}
* @since 1.13
*/
protected Generate(java.lang.String parent, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1GenerateUserSessionRequest content) {
super(PaymentsResellerSubscription.this, "POST", REST_PATH, content, com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1GenerateUserSessionResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public Generate set$Xgafv(java.lang.String $Xgafv) {
return (Generate) super.set$Xgafv($Xgafv);
}
@Override
public Generate setAccessToken(java.lang.String accessToken) {
return (Generate) super.setAccessToken(accessToken);
}
@Override
public Generate setAlt(java.lang.String alt) {
return (Generate) super.setAlt(alt);
}
@Override
public Generate setCallback(java.lang.String callback) {
return (Generate) super.setCallback(callback);
}
@Override
public Generate setFields(java.lang.String fields) {
return (Generate) super.setFields(fields);
}
@Override
public Generate setKey(java.lang.String key) {
return (Generate) super.setKey(key);
}
@Override
public Generate setOauthToken(java.lang.String oauthToken) {
return (Generate) super.setOauthToken(oauthToken);
}
@Override
public Generate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Generate) super.setPrettyPrint(prettyPrint);
}
@Override
public Generate setQuotaUser(java.lang.String quotaUser) {
return (Generate) super.setQuotaUser(quotaUser);
}
@Override
public Generate setUploadType(java.lang.String uploadType) {
return (Generate) super.setUploadType(uploadType);
}
@Override
public Generate setUploadProtocol(java.lang.String uploadProtocol) {
return (Generate) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent, the partner that can resell. Format: partners/{partner}
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent, the partner that can resell. Format: partners/{partner} */
public Generate setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Generate set(String parameterName, Object value) {
return (Generate) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link PaymentsResellerSubscription}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link PaymentsResellerSubscription}. */
@Override
public PaymentsResellerSubscription build() {
return new PaymentsResellerSubscription(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link PaymentsResellerSubscriptionRequestInitializer}.
*
* @since 1.12
*/
public Builder setPaymentsResellerSubscriptionRequestInitializer(
PaymentsResellerSubscriptionRequestInitializer paymentsresellersubscriptionRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(paymentsresellersubscriptionRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}