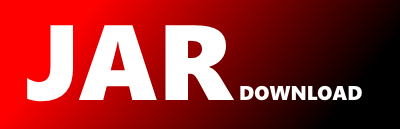
com.google.api.services.paymentsresellersubscription.v1.model.GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.paymentsresellersubscription.v1.model;
/**
* Request to find eligible promotions for the current user.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Payments Reseller Subscription API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest extends com.google.api.client.json.GenericJson {
/**
* Optional. Specifies the filters for the promotion results. The syntax is defined in
* https://google.aip.dev/160 with the following caveats: 1. Only the following features are
* supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) - Traversal
* operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields are supported:
* - `applicableProducts` - `regionCodes` - `youtubePayload.partnerEligibilityId` -
* `youtubePayload.postalCode` 3. Unless explicitly mentioned above, other features are not
* supported. Example: `applicableProducts:partners/partner1/products/product1 AND regionCodes:US
* AND youtubePayload.postalCode=94043 AND youtubePayload.partnerEligibilityId=eligibility-id`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**
* Optional. The maximum number of promotions to return. The service may return fewer than this
* value. If unspecified, at most 50 products will be returned. The maximum value is 1000; values
* above 1000 will be coerced to 1000.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/**
* Optional. A page token, received from a previous `ListPromotions` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPromotions` must match the call that provided the page token.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/**
* Optional. Specifies the filters for the promotion results. The syntax is defined in
* https://google.aip.dev/160 with the following caveats: 1. Only the following features are
* supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) - Traversal
* operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields are supported:
* - `applicableProducts` - `regionCodes` - `youtubePayload.partnerEligibilityId` -
* `youtubePayload.postalCode` 3. Unless explicitly mentioned above, other features are not
* supported. Example: `applicableProducts:partners/partner1/products/product1 AND regionCodes:US
* AND youtubePayload.postalCode=94043 AND youtubePayload.partnerEligibilityId=eligibility-id`
* @return value or {@code null} for none
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. Specifies the filters for the promotion results. The syntax is defined in
* https://google.aip.dev/160 with the following caveats: 1. Only the following features are
* supported: - Logical operator `AND` - Comparison operator `=` (no wildcards `*`) - Traversal
* operator `.` - Has operator `:` (no wildcards `*`) 2. Only the following fields are supported:
* - `applicableProducts` - `regionCodes` - `youtubePayload.partnerEligibilityId` -
* `youtubePayload.postalCode` 3. Unless explicitly mentioned above, other features are not
* supported. Example: `applicableProducts:partners/partner1/products/product1 AND regionCodes:US
* AND youtubePayload.postalCode=94043 AND youtubePayload.partnerEligibilityId=eligibility-id`
* @param filter filter or {@code null} for none
*/
public GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of promotions to return. The service may return fewer than this
* value. If unspecified, at most 50 products will be returned. The maximum value is 1000; values
* above 1000 will be coerced to 1000.
* @return value or {@code null} for none
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of promotions to return. The service may return fewer than this
* value. If unspecified, at most 50 products will be returned. The maximum value is 1000; values
* above 1000 will be coerced to 1000.
* @param pageSize pageSize or {@code null} for none
*/
public GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListPromotions` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPromotions` must match the call that provided the page token.
* @return value or {@code null} for none
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListPromotions` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPromotions` must match the call that provided the page token.
* @param pageToken pageToken or {@code null} for none
*/
public GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest set(String fieldName, Object value) {
return (GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest) super.set(fieldName, value);
}
@Override
public GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest clone() {
return (GoogleCloudPaymentsResellerSubscriptionV1FindEligiblePromotionsRequest) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy