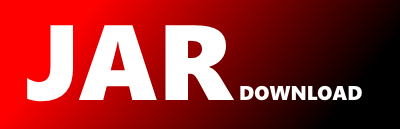
com.google.api.services.people.v1.PeopleService Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-05-04 17:28:03 UTC)
* on 2018-09-05 at 04:11:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.people.v1;
/**
* Service definition for PeopleService (v1).
*
*
* Provides access to information about profiles and contacts.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link PeopleServiceRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class PeopleService extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.25.0 of the People API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://people.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public PeopleService(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
PeopleService(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the ContactGroups collection.
*
* The typical use is:
*
* {@code PeopleService people = new PeopleService(...);}
* {@code PeopleService.ContactGroups.List request = people.contactGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public ContactGroups contactGroups() {
return new ContactGroups();
}
/**
* The "contactGroups" collection of methods.
*/
public class ContactGroups {
/**
* Get a list of contact groups owned by the authenticated user by specifying a list of contact
* group resource names.
*
* Create a request for the method "contactGroups.batchGet".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link BatchGet#execute()} method to invoke the remote operation.
*
* @return the request
*/
public BatchGet batchGet() throws java.io.IOException {
BatchGet result = new BatchGet();
initialize(result);
return result;
}
public class BatchGet extends PeopleServiceRequest {
private static final String REST_PATH = "v1/contactGroups:batchGet";
/**
* Get a list of contact groups owned by the authenticated user by specifying a list of contact
* group resource names.
*
* Create a request for the method "contactGroups.batchGet".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link BatchGet#execute()} method to invoke the remote operation.
* {@link
* BatchGet#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected BatchGet() {
super(PeopleService.this, "GET", REST_PATH, null, com.google.api.services.people.v1.model.BatchGetContactGroupsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public BatchGet set$Xgafv(java.lang.String $Xgafv) {
return (BatchGet) super.set$Xgafv($Xgafv);
}
@Override
public BatchGet setAccessToken(java.lang.String accessToken) {
return (BatchGet) super.setAccessToken(accessToken);
}
@Override
public BatchGet setAlt(java.lang.String alt) {
return (BatchGet) super.setAlt(alt);
}
@Override
public BatchGet setCallback(java.lang.String callback) {
return (BatchGet) super.setCallback(callback);
}
@Override
public BatchGet setFields(java.lang.String fields) {
return (BatchGet) super.setFields(fields);
}
@Override
public BatchGet setKey(java.lang.String key) {
return (BatchGet) super.setKey(key);
}
@Override
public BatchGet setOauthToken(java.lang.String oauthToken) {
return (BatchGet) super.setOauthToken(oauthToken);
}
@Override
public BatchGet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchGet) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchGet setQuotaUser(java.lang.String quotaUser) {
return (BatchGet) super.setQuotaUser(quotaUser);
}
@Override
public BatchGet setUploadType(java.lang.String uploadType) {
return (BatchGet) super.setUploadType(uploadType);
}
@Override
public BatchGet setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchGet) super.setUploadProtocol(uploadProtocol);
}
/** Specifies the maximum number of members to return for each group. */
@com.google.api.client.util.Key
private java.lang.Integer maxMembers;
/** Specifies the maximum number of members to return for each group.
*/
public java.lang.Integer getMaxMembers() {
return maxMembers;
}
/** Specifies the maximum number of members to return for each group. */
public BatchGet setMaxMembers(java.lang.Integer maxMembers) {
this.maxMembers = maxMembers;
return this;
}
/** The resource names of the contact groups to get. */
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** The resource names of the contact groups to get.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/** The resource names of the contact groups to get. */
public BatchGet setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
@Override
public BatchGet set(String parameterName, Object value) {
return (BatchGet) super.set(parameterName, value);
}
}
/**
* Create a new contact group owned by the authenticated user.
*
* Create a request for the method "contactGroups.create".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.people.v1.model.CreateContactGroupRequest}
* @return the request
*/
public Create create(com.google.api.services.people.v1.model.CreateContactGroupRequest content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends PeopleServiceRequest {
private static final String REST_PATH = "v1/contactGroups";
/**
* Create a new contact group owned by the authenticated user.
*
* Create a request for the method "contactGroups.create".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.people.v1.model.CreateContactGroupRequest}
* @since 1.13
*/
protected Create(com.google.api.services.people.v1.model.CreateContactGroupRequest content) {
super(PeopleService.this, "POST", REST_PATH, content, com.google.api.services.people.v1.model.ContactGroup.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete an existing contact group owned by the authenticated user by specifying a contact group
* resource name.
*
* Create a request for the method "contactGroups.delete".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name of the contact group to delete.
* @return the request
*/
public Delete delete(java.lang.String resourceName) throws java.io.IOException {
Delete result = new Delete(resourceName);
initialize(result);
return result;
}
public class Delete extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^contactGroups/[^/]+$");
/**
* Delete an existing contact group owned by the authenticated user by specifying a contact group
* resource name.
*
* Create a request for the method "contactGroups.delete".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param resourceName The resource name of the contact group to delete.
* @since 1.13
*/
protected Delete(java.lang.String resourceName) {
super(PeopleService.this, "DELETE", REST_PATH, null, com.google.api.services.people.v1.model.Empty.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The resource name of the contact group to delete. */
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name of the contact group to delete.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/** The resource name of the contact group to delete. */
public Delete setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
/** Set to true to also delete the contacts in the specified group. */
@com.google.api.client.util.Key
private java.lang.Boolean deleteContacts;
/** Set to true to also delete the contacts in the specified group.
*/
public java.lang.Boolean getDeleteContacts() {
return deleteContacts;
}
/** Set to true to also delete the contacts in the specified group. */
public Delete setDeleteContacts(java.lang.Boolean deleteContacts) {
this.deleteContacts = deleteContacts;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Get a specific contact group owned by the authenticated user by specifying a contact group
* resource name.
*
* Create a request for the method "contactGroups.get".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name of the contact group to get.
* @return the request
*/
public Get get(java.lang.String resourceName) throws java.io.IOException {
Get result = new Get(resourceName);
initialize(result);
return result;
}
public class Get extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^contactGroups/[^/]+$");
/**
* Get a specific contact group owned by the authenticated user by specifying a contact group
* resource name.
*
* Create a request for the method "contactGroups.get".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param resourceName The resource name of the contact group to get.
* @since 1.13
*/
protected Get(java.lang.String resourceName) {
super(PeopleService.this, "GET", REST_PATH, null, com.google.api.services.people.v1.model.ContactGroup.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The resource name of the contact group to get. */
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name of the contact group to get.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/** The resource name of the contact group to get. */
public Get setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
/** Specifies the maximum number of members to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxMembers;
/** Specifies the maximum number of members to return.
*/
public java.lang.Integer getMaxMembers() {
return maxMembers;
}
/** Specifies the maximum number of members to return. */
public Get setMaxMembers(java.lang.Integer maxMembers) {
this.maxMembers = maxMembers;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all contact groups owned by the authenticated user. Members of the contact groups are not
* populated.
*
* Create a request for the method "contactGroups.list".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends PeopleServiceRequest {
private static final String REST_PATH = "v1/contactGroups";
/**
* List all contact groups owned by the authenticated user. Members of the contact groups are not
* populated.
*
* Create a request for the method "contactGroups.list".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(PeopleService.this, "GET", REST_PATH, null, com.google.api.services.people.v1.model.ListContactGroupsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The next_page_token value returned from a previous call to
* [ListContactGroups](/people/api/rest/v1/contactgroups/list). Requests the next page of
* resources.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous call to
[ListContactGroups](/people/api/rest/v1/contactgroups/list). Requests the next page of resources.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The next_page_token value returned from a previous call to
* [ListContactGroups](/people/api/rest/v1/contactgroups/list). Requests the next page of
* resources.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The maximum number of resources to return. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of resources to return.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of resources to return. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A sync token, returned by a previous call to `contactgroups.list`. Only resources changed
* since the sync token was created will be returned.
*/
@com.google.api.client.util.Key
private java.lang.String syncToken;
/** A sync token, returned by a previous call to `contactgroups.list`. Only resources changed since the
sync token was created will be returned.
*/
public java.lang.String getSyncToken() {
return syncToken;
}
/**
* A sync token, returned by a previous call to `contactgroups.list`. Only resources changed
* since the sync token was created will be returned.
*/
public List setSyncToken(java.lang.String syncToken) {
this.syncToken = syncToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Update the name of an existing contact group owned by the authenticated user.
*
* Create a request for the method "contactGroups.update".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name for the contact group, assigned by the server. An ASCII
string, in the form of
* `contactGroups/`contact_group_id.
* @param content the {@link com.google.api.services.people.v1.model.UpdateContactGroupRequest}
* @return the request
*/
public Update update(java.lang.String resourceName, com.google.api.services.people.v1.model.UpdateContactGroupRequest content) throws java.io.IOException {
Update result = new Update(resourceName, content);
initialize(result);
return result;
}
public class Update extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^contactGroups/[^/]+$");
/**
* Update the name of an existing contact group owned by the authenticated user.
*
* Create a request for the method "contactGroups.update".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param resourceName The resource name for the contact group, assigned by the server. An ASCII
string, in the form of
* `contactGroups/`contact_group_id.
* @param content the {@link com.google.api.services.people.v1.model.UpdateContactGroupRequest}
* @since 1.13
*/
protected Update(java.lang.String resourceName, com.google.api.services.people.v1.model.UpdateContactGroupRequest content) {
super(PeopleService.this, "PUT", REST_PATH, content, com.google.api.services.people.v1.model.ContactGroup.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name for the contact group, assigned by the server. An ASCII string, in the
* form of `contactGroups/`contact_group_id.
*/
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name for the contact group, assigned by the server. An ASCII string, in the form of
`contactGroups/`contact_group_id.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/**
* The resource name for the contact group, assigned by the server. An ASCII string, in the
* form of `contactGroups/`contact_group_id.
*/
public Update setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Members collection.
*
* The typical use is:
*
* {@code PeopleService people = new PeopleService(...);}
* {@code PeopleService.Members.List request = people.members().list(parameters ...)}
*
*
* @return the resource collection
*/
public Members members() {
return new Members();
}
/**
* The "members" collection of methods.
*/
public class Members {
/**
* Modify the members of a contact group owned by the authenticated user.
*
* Create a request for the method "members.modify".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link Modify#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name of the contact group to modify.
* @param content the {@link com.google.api.services.people.v1.model.ModifyContactGroupMembersRequest}
* @return the request
*/
public Modify modify(java.lang.String resourceName, com.google.api.services.people.v1.model.ModifyContactGroupMembersRequest content) throws java.io.IOException {
Modify result = new Modify(resourceName, content);
initialize(result);
return result;
}
public class Modify extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}/members:modify";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^contactGroups/[^/]+$");
/**
* Modify the members of a contact group owned by the authenticated user.
*
* Create a request for the method "members.modify".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link Modify#execute()} method to invoke the remote operation. {@link
* Modify#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param resourceName The resource name of the contact group to modify.
* @param content the {@link com.google.api.services.people.v1.model.ModifyContactGroupMembersRequest}
* @since 1.13
*/
protected Modify(java.lang.String resourceName, com.google.api.services.people.v1.model.ModifyContactGroupMembersRequest content) {
super(PeopleService.this, "POST", REST_PATH, content, com.google.api.services.people.v1.model.ModifyContactGroupMembersResponse.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
}
@Override
public Modify set$Xgafv(java.lang.String $Xgafv) {
return (Modify) super.set$Xgafv($Xgafv);
}
@Override
public Modify setAccessToken(java.lang.String accessToken) {
return (Modify) super.setAccessToken(accessToken);
}
@Override
public Modify setAlt(java.lang.String alt) {
return (Modify) super.setAlt(alt);
}
@Override
public Modify setCallback(java.lang.String callback) {
return (Modify) super.setCallback(callback);
}
@Override
public Modify setFields(java.lang.String fields) {
return (Modify) super.setFields(fields);
}
@Override
public Modify setKey(java.lang.String key) {
return (Modify) super.setKey(key);
}
@Override
public Modify setOauthToken(java.lang.String oauthToken) {
return (Modify) super.setOauthToken(oauthToken);
}
@Override
public Modify setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Modify) super.setPrettyPrint(prettyPrint);
}
@Override
public Modify setQuotaUser(java.lang.String quotaUser) {
return (Modify) super.setQuotaUser(quotaUser);
}
@Override
public Modify setUploadType(java.lang.String uploadType) {
return (Modify) super.setUploadType(uploadType);
}
@Override
public Modify setUploadProtocol(java.lang.String uploadProtocol) {
return (Modify) super.setUploadProtocol(uploadProtocol);
}
/** The resource name of the contact group to modify. */
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name of the contact group to modify.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/** The resource name of the contact group to modify. */
public Modify setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^contactGroups/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
@Override
public Modify set(String parameterName, Object value) {
return (Modify) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the People collection.
*
* The typical use is:
*
* {@code PeopleService people = new PeopleService(...);}
* {@code PeopleService.People.List request = people.people().list(parameters ...)}
*
*
* @return the resource collection
*/
public People people() {
return new People();
}
/**
* The "people" collection of methods.
*/
public class People {
/**
* Create a new contact and return the person resource for that contact.
*
* Create a request for the method "people.createContact".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link CreateContact#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.people.v1.model.Person}
* @return the request
*/
public CreateContact createContact(com.google.api.services.people.v1.model.Person content) throws java.io.IOException {
CreateContact result = new CreateContact(content);
initialize(result);
return result;
}
public class CreateContact extends PeopleServiceRequest {
private static final String REST_PATH = "v1/people:createContact";
/**
* Create a new contact and return the person resource for that contact.
*
* Create a request for the method "people.createContact".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link CreateContact#execute()} method to invoke the remote operation.
* {@link CreateContact#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param content the {@link com.google.api.services.people.v1.model.Person}
* @since 1.13
*/
protected CreateContact(com.google.api.services.people.v1.model.Person content) {
super(PeopleService.this, "POST", REST_PATH, content, com.google.api.services.people.v1.model.Person.class);
}
@Override
public CreateContact set$Xgafv(java.lang.String $Xgafv) {
return (CreateContact) super.set$Xgafv($Xgafv);
}
@Override
public CreateContact setAccessToken(java.lang.String accessToken) {
return (CreateContact) super.setAccessToken(accessToken);
}
@Override
public CreateContact setAlt(java.lang.String alt) {
return (CreateContact) super.setAlt(alt);
}
@Override
public CreateContact setCallback(java.lang.String callback) {
return (CreateContact) super.setCallback(callback);
}
@Override
public CreateContact setFields(java.lang.String fields) {
return (CreateContact) super.setFields(fields);
}
@Override
public CreateContact setKey(java.lang.String key) {
return (CreateContact) super.setKey(key);
}
@Override
public CreateContact setOauthToken(java.lang.String oauthToken) {
return (CreateContact) super.setOauthToken(oauthToken);
}
@Override
public CreateContact setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateContact) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateContact setQuotaUser(java.lang.String quotaUser) {
return (CreateContact) super.setQuotaUser(quotaUser);
}
@Override
public CreateContact setUploadType(java.lang.String uploadType) {
return (CreateContact) super.setUploadType(uploadType);
}
@Override
public CreateContact setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateContact) super.setUploadProtocol(uploadProtocol);
}
/** The resource name of the owning person resource. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The resource name of the owning person resource.
*/
public java.lang.String getParent() {
return parent;
}
/** The resource name of the owning person resource. */
public CreateContact setParent(java.lang.String parent) {
this.parent = parent;
return this;
}
@Override
public CreateContact set(String parameterName, Object value) {
return (CreateContact) super.set(parameterName, value);
}
}
/**
* Delete a contact person. Any non-contact data will not be deleted.
*
* Create a request for the method "people.deleteContact".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link DeleteContact#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name of the contact to delete.
* @return the request
*/
public DeleteContact deleteContact(java.lang.String resourceName) throws java.io.IOException {
DeleteContact result = new DeleteContact(resourceName);
initialize(result);
return result;
}
public class DeleteContact extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}:deleteContact";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^people/[^/]+$");
/**
* Delete a contact person. Any non-contact data will not be deleted.
*
* Create a request for the method "people.deleteContact".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link DeleteContact#execute()} method to invoke the remote operation.
* {@link DeleteContact#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param resourceName The resource name of the contact to delete.
* @since 1.13
*/
protected DeleteContact(java.lang.String resourceName) {
super(PeopleService.this, "DELETE", REST_PATH, null, com.google.api.services.people.v1.model.Empty.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
}
@Override
public DeleteContact set$Xgafv(java.lang.String $Xgafv) {
return (DeleteContact) super.set$Xgafv($Xgafv);
}
@Override
public DeleteContact setAccessToken(java.lang.String accessToken) {
return (DeleteContact) super.setAccessToken(accessToken);
}
@Override
public DeleteContact setAlt(java.lang.String alt) {
return (DeleteContact) super.setAlt(alt);
}
@Override
public DeleteContact setCallback(java.lang.String callback) {
return (DeleteContact) super.setCallback(callback);
}
@Override
public DeleteContact setFields(java.lang.String fields) {
return (DeleteContact) super.setFields(fields);
}
@Override
public DeleteContact setKey(java.lang.String key) {
return (DeleteContact) super.setKey(key);
}
@Override
public DeleteContact setOauthToken(java.lang.String oauthToken) {
return (DeleteContact) super.setOauthToken(oauthToken);
}
@Override
public DeleteContact setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DeleteContact) super.setPrettyPrint(prettyPrint);
}
@Override
public DeleteContact setQuotaUser(java.lang.String quotaUser) {
return (DeleteContact) super.setQuotaUser(quotaUser);
}
@Override
public DeleteContact setUploadType(java.lang.String uploadType) {
return (DeleteContact) super.setUploadType(uploadType);
}
@Override
public DeleteContact setUploadProtocol(java.lang.String uploadProtocol) {
return (DeleteContact) super.setUploadProtocol(uploadProtocol);
}
/** The resource name of the contact to delete. */
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name of the contact to delete.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/** The resource name of the contact to delete. */
public DeleteContact setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
@Override
public DeleteContact set(String parameterName, Object value) {
return (DeleteContact) super.set(parameterName, value);
}
}
/**
* Provides information about a person by specifying a resource name. Use `people/me` to indicate
* the authenticated user.
*
* The request throws a 400 error if 'personFields' is not specified.
*
* Create a request for the method "people.get".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name of the person to provide information about.
- To get information about the
* authenticated user, specify `people/me`.
- To get information about a google account,
* specify
`people/`account_id.
- To get information about a contact, specify the resource
* name that
identifies the contact as returned by
* [`people.connections.list`](/people/api/rest/v1/people.connections/list).
* @return the request
*/
public Get get(java.lang.String resourceName) throws java.io.IOException {
Get result = new Get(resourceName);
initialize(result);
return result;
}
public class Get extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^people/[^/]+$");
/**
* Provides information about a person by specifying a resource name. Use `people/me` to indicate
* the authenticated user.
*
* The request throws a 400 error if 'personFields' is not specified.
*
* Create a request for the method "people.get".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param resourceName The resource name of the person to provide information about.
- To get information about the
* authenticated user, specify `people/me`.
- To get information about a google account,
* specify
`people/`account_id.
- To get information about a contact, specify the resource
* name that
identifies the contact as returned by
* [`people.connections.list`](/people/api/rest/v1/people.connections/list).
* @since 1.13
*/
protected Get(java.lang.String resourceName) {
super(PeopleService.this, "GET", REST_PATH, null, com.google.api.services.people.v1.model.Person.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the person to provide information about.
*
* - To get information about the authenticated user, specify `people/me`. - To get
* information about a google account, specify `people/`account_id. - To get information about
* a contact, specify the resource name that identifies the contact as returned by
* [`people.connections.list`](/people/api/rest/v1/people.connections/list).
*/
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name of the person to provide information about.
- To get information about the authenticated user, specify `people/me`. - To get information about
a google account, specify `people/`account_id. - To get information about a contact, specify the
resource name that identifies the contact as returned by
[`people.connections.list`](/people/api/rest/v1/people.connections/list).
*/
public java.lang.String getResourceName() {
return resourceName;
}
/**
* The resource name of the person to provide information about.
*
* - To get information about the authenticated user, specify `people/me`. - To get
* information about a google account, specify `people/`account_id. - To get information about
* a contact, specify the resource name that identifies the contact as returned by
* [`people.connections.list`](/people/api/rest/v1/people.connections/list).
*/
public Get setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
/**
* **Required.** A field mask to restrict which fields on the person are returned. Multiple
* fields can be specified by separating them with commas. Valid values are:
*
* * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos *
* emailAddresses * events * genders * imClients * interests * locales * memberships *
* metadata * names * nicknames * occupations * organizations * phoneNumbers * photos *
* relations * relationshipInterests * relationshipStatuses * residences * sipAddresses *
* skills * taglines * urls * userDefined
*/
@com.google.api.client.util.Key
private String personFields;
/**** Required.** A field mask to restrict which fields on the person are returned. Multiple fields can
** be specified by separating them with commas. Valid values are:
**
** * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos * emailAddresses
** * events * genders * imClients * interests * locales * memberships * metadata * names * nicknames
** * occupations * organizations * phoneNumbers * photos * relations * relationshipInterests *
** relationshipStatuses * residences * sipAddresses * skills * taglines * urls * userDefined
**
*/
public String getPersonFields() {
return personFields;
}
/**
* **Required.** A field mask to restrict which fields on the person are returned. Multiple
* fields can be specified by separating them with commas. Valid values are:
*
* * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos *
* emailAddresses * events * genders * imClients * interests * locales * memberships *
* metadata * names * nicknames * occupations * organizations * phoneNumbers * photos *
* relations * relationshipInterests * relationshipStatuses * residences * sipAddresses *
* skills * taglines * urls * userDefined
*/
public Get setPersonFields(String personFields) {
this.personFields = personFields;
return this;
}
/**
* **Required.** Comma-separated list of person fields to be included in the response. Each
* path should start with `person.`: for example, `person.names` or `person.photos`.
*/
@com.google.api.client.util.Key("requestMask.includeField")
private String requestMaskIncludeField;
/**** Required.** Comma-separated list of person fields to be included in the response. Each path
** should start with `person.`: for example, `person.names` or `person.photos`.
**
*/
public String getRequestMaskIncludeField() {
return requestMaskIncludeField;
}
/**
* **Required.** Comma-separated list of person fields to be included in the response. Each
* path should start with `person.`: for example, `person.names` or `person.photos`.
*/
public Get setRequestMaskIncludeField(String requestMaskIncludeField) {
this.requestMaskIncludeField = requestMaskIncludeField;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Provides information about a list of specific people by specifying a list of requested resource
* names. Use `people/me` to indicate the authenticated user.
*
* The request throws a 400 error if 'personFields' is not specified.
*
* Create a request for the method "people.getBatchGet".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link GetBatchGet#execute()} method to invoke the remote operation.
*
* @return the request
*/
public GetBatchGet getBatchGet() throws java.io.IOException {
GetBatchGet result = new GetBatchGet();
initialize(result);
return result;
}
public class GetBatchGet extends PeopleServiceRequest {
private static final String REST_PATH = "v1/people:batchGet";
/**
* Provides information about a list of specific people by specifying a list of requested resource
* names. Use `people/me` to indicate the authenticated user.
*
* The request throws a 400 error if 'personFields' is not specified.
*
* Create a request for the method "people.getBatchGet".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link GetBatchGet#execute()} method to invoke the remote operation.
* {@link
* GetBatchGet#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected GetBatchGet() {
super(PeopleService.this, "GET", REST_PATH, null, com.google.api.services.people.v1.model.GetPeopleResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetBatchGet set$Xgafv(java.lang.String $Xgafv) {
return (GetBatchGet) super.set$Xgafv($Xgafv);
}
@Override
public GetBatchGet setAccessToken(java.lang.String accessToken) {
return (GetBatchGet) super.setAccessToken(accessToken);
}
@Override
public GetBatchGet setAlt(java.lang.String alt) {
return (GetBatchGet) super.setAlt(alt);
}
@Override
public GetBatchGet setCallback(java.lang.String callback) {
return (GetBatchGet) super.setCallback(callback);
}
@Override
public GetBatchGet setFields(java.lang.String fields) {
return (GetBatchGet) super.setFields(fields);
}
@Override
public GetBatchGet setKey(java.lang.String key) {
return (GetBatchGet) super.setKey(key);
}
@Override
public GetBatchGet setOauthToken(java.lang.String oauthToken) {
return (GetBatchGet) super.setOauthToken(oauthToken);
}
@Override
public GetBatchGet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetBatchGet) super.setPrettyPrint(prettyPrint);
}
@Override
public GetBatchGet setQuotaUser(java.lang.String quotaUser) {
return (GetBatchGet) super.setQuotaUser(quotaUser);
}
@Override
public GetBatchGet setUploadType(java.lang.String uploadType) {
return (GetBatchGet) super.setUploadType(uploadType);
}
@Override
public GetBatchGet setUploadProtocol(java.lang.String uploadProtocol) {
return (GetBatchGet) super.setUploadProtocol(uploadProtocol);
}
/**
* **Required.** Comma-separated list of person fields to be included in the response. Each
* path should start with `person.`: for example, `person.names` or `person.photos`.
*/
@com.google.api.client.util.Key("requestMask.includeField")
private String requestMaskIncludeField;
/**** Required.** Comma-separated list of person fields to be included in the response. Each path
** should start with `person.`: for example, `person.names` or `person.photos`.
**
*/
public String getRequestMaskIncludeField() {
return requestMaskIncludeField;
}
/**
* **Required.** Comma-separated list of person fields to be included in the response. Each
* path should start with `person.`: for example, `person.names` or `person.photos`.
*/
public GetBatchGet setRequestMaskIncludeField(String requestMaskIncludeField) {
this.requestMaskIncludeField = requestMaskIncludeField;
return this;
}
/**
* The resource names of the people to provide information about.
*
* - To get information about the authenticated user, specify `people/me`. - To get
* information about a google account, specify `people/`account_id. - To get information about
* a contact, specify the resource name that identifies the contact as returned by
* [`people.connections.list`](/people/api/rest/v1/people.connections/list).
*
* You can include up to 50 resource names in one request.
*/
@com.google.api.client.util.Key
private java.util.List resourceNames;
/** The resource names of the people to provide information about.
- To get information about the authenticated user, specify `people/me`. - To get information about
a google account, specify `people/`account_id. - To get information about a contact, specify the
resource name that identifies the contact as returned by
[`people.connections.list`](/people/api/rest/v1/people.connections/list).
You can include up to 50 resource names in one request.
*/
public java.util.List getResourceNames() {
return resourceNames;
}
/**
* The resource names of the people to provide information about.
*
* - To get information about the authenticated user, specify `people/me`. - To get
* information about a google account, specify `people/`account_id. - To get information about
* a contact, specify the resource name that identifies the contact as returned by
* [`people.connections.list`](/people/api/rest/v1/people.connections/list).
*
* You can include up to 50 resource names in one request.
*/
public GetBatchGet setResourceNames(java.util.List resourceNames) {
this.resourceNames = resourceNames;
return this;
}
/**
* **Required.** A field mask to restrict which fields on each person are returned. Multiple
* fields can be specified by separating them with commas. Valid values are:
*
* * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos *
* emailAddresses * events * genders * imClients * interests * locales * memberships *
* metadata * names * nicknames * occupations * organizations * phoneNumbers * photos *
* relations * relationshipInterests * relationshipStatuses * residences * sipAddresses *
* skills * taglines * urls * userDefined
*/
@com.google.api.client.util.Key
private String personFields;
/**** Required.** A field mask to restrict which fields on each person are returned. Multiple fields
** can be specified by separating them with commas. Valid values are:
**
** * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos * emailAddresses
** * events * genders * imClients * interests * locales * memberships * metadata * names * nicknames
** * occupations * organizations * phoneNumbers * photos * relations * relationshipInterests *
** relationshipStatuses * residences * sipAddresses * skills * taglines * urls * userDefined
**
*/
public String getPersonFields() {
return personFields;
}
/**
* **Required.** A field mask to restrict which fields on each person are returned. Multiple
* fields can be specified by separating them with commas. Valid values are:
*
* * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos *
* emailAddresses * events * genders * imClients * interests * locales * memberships *
* metadata * names * nicknames * occupations * organizations * phoneNumbers * photos *
* relations * relationshipInterests * relationshipStatuses * residences * sipAddresses *
* skills * taglines * urls * userDefined
*/
public GetBatchGet setPersonFields(String personFields) {
this.personFields = personFields;
return this;
}
@Override
public GetBatchGet set(String parameterName, Object value) {
return (GetBatchGet) super.set(parameterName, value);
}
}
/**
* Update contact data for an existing contact person. Any non-contact data will not be modified.
*
* The request throws a 400 error if `updatePersonFields` is not specified.
*
* The request throws a 400 error if `person.metadata.sources` is not specified for the contact to
* be updated.
*
* The request throws a 412 error if `person.metadata.sources.etag` is different than the contact's
* etag, which indicates the contact has changed since its data was read. Clients should get the
* latest person and re-apply their updates to the latest person.
*
* Create a request for the method "people.updateContact".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link UpdateContact#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name for the person, assigned by the server. An ASCII string
with a max length of 27
* characters, in the form of
`people/`person_id.
* @param content the {@link com.google.api.services.people.v1.model.Person}
* @return the request
*/
public UpdateContact updateContact(java.lang.String resourceName, com.google.api.services.people.v1.model.Person content) throws java.io.IOException {
UpdateContact result = new UpdateContact(resourceName, content);
initialize(result);
return result;
}
public class UpdateContact extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}:updateContact";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^people/[^/]+$");
/**
* Update contact data for an existing contact person. Any non-contact data will not be modified.
*
* The request throws a 400 error if `updatePersonFields` is not specified.
*
* The request throws a 400 error if `person.metadata.sources` is not specified for the contact to
* be updated.
*
* The request throws a 412 error if `person.metadata.sources.etag` is different than the
* contact's etag, which indicates the contact has changed since its data was read. Clients should
* get the latest person and re-apply their updates to the latest person.
*
* Create a request for the method "people.updateContact".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link UpdateContact#execute()} method to invoke the remote operation.
* {@link UpdateContact#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param resourceName The resource name for the person, assigned by the server. An ASCII string
with a max length of 27
* characters, in the form of
`people/`person_id.
* @param content the {@link com.google.api.services.people.v1.model.Person}
* @since 1.13
*/
protected UpdateContact(java.lang.String resourceName, com.google.api.services.people.v1.model.Person content) {
super(PeopleService.this, "PATCH", REST_PATH, content, com.google.api.services.people.v1.model.Person.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
}
@Override
public UpdateContact set$Xgafv(java.lang.String $Xgafv) {
return (UpdateContact) super.set$Xgafv($Xgafv);
}
@Override
public UpdateContact setAccessToken(java.lang.String accessToken) {
return (UpdateContact) super.setAccessToken(accessToken);
}
@Override
public UpdateContact setAlt(java.lang.String alt) {
return (UpdateContact) super.setAlt(alt);
}
@Override
public UpdateContact setCallback(java.lang.String callback) {
return (UpdateContact) super.setCallback(callback);
}
@Override
public UpdateContact setFields(java.lang.String fields) {
return (UpdateContact) super.setFields(fields);
}
@Override
public UpdateContact setKey(java.lang.String key) {
return (UpdateContact) super.setKey(key);
}
@Override
public UpdateContact setOauthToken(java.lang.String oauthToken) {
return (UpdateContact) super.setOauthToken(oauthToken);
}
@Override
public UpdateContact setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateContact) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateContact setQuotaUser(java.lang.String quotaUser) {
return (UpdateContact) super.setQuotaUser(quotaUser);
}
@Override
public UpdateContact setUploadType(java.lang.String uploadType) {
return (UpdateContact) super.setUploadType(uploadType);
}
@Override
public UpdateContact setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateContact) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name for the person, assigned by the server. An ASCII string with a max length
* of 27 characters, in the form of `people/`person_id.
*/
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name for the person, assigned by the server. An ASCII string with a max length of 27
characters, in the form of `people/`person_id.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/**
* The resource name for the person, assigned by the server. An ASCII string with a max length
* of 27 characters, in the form of `people/`person_id.
*/
public UpdateContact setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
/**
* **Required.** A field mask to restrict which fields on the person are updated. Multiple
* fields can be specified by separating them with commas. All updated fields will be
* replaced. Valid values are:
*
* * addresses * biographies * birthdays * emailAddresses * events * genders * imClients *
* interests * locales * names * nicknames * occupations * organizations * phoneNumbers *
* relations * residences * sipAddresses * urls * userDefined
*/
@com.google.api.client.util.Key
private String updatePersonFields;
/**** Required.** A field mask to restrict which fields on the person are updated. Multiple fields can
** be specified by separating them with commas. All updated fields will be replaced. Valid values
** are:
**
** * addresses * biographies * birthdays * emailAddresses * events * genders * imClients * interests
** * locales * names * nicknames * occupations * organizations * phoneNumbers * relations *
** residences * sipAddresses * urls * userDefined
**
*/
public String getUpdatePersonFields() {
return updatePersonFields;
}
/**
* **Required.** A field mask to restrict which fields on the person are updated. Multiple
* fields can be specified by separating them with commas. All updated fields will be
* replaced. Valid values are:
*
* * addresses * biographies * birthdays * emailAddresses * events * genders * imClients *
* interests * locales * names * nicknames * occupations * organizations * phoneNumbers *
* relations * residences * sipAddresses * urls * userDefined
*/
public UpdateContact setUpdatePersonFields(String updatePersonFields) {
this.updatePersonFields = updatePersonFields;
return this;
}
@Override
public UpdateContact set(String parameterName, Object value) {
return (UpdateContact) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Connections collection.
*
* The typical use is:
*
* {@code PeopleService people = new PeopleService(...);}
* {@code PeopleService.Connections.List request = people.connections().list(parameters ...)}
*
*
* @return the resource collection
*/
public Connections connections() {
return new Connections();
}
/**
* The "connections" collection of methods.
*/
public class Connections {
/**
* Provides a list of the authenticated user's contacts merged with any connected profiles.
*
* The request throws a 400 error if 'personFields' is not specified.
*
* Create a request for the method "connections.list".
*
* This request holds the parameters needed by the people server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param resourceName The resource name to return connections for. Only `people/me` is valid.
* @return the request
*/
public List list(java.lang.String resourceName) throws java.io.IOException {
List result = new List(resourceName);
initialize(result);
return result;
}
public class List extends PeopleServiceRequest {
private static final String REST_PATH = "v1/{+resourceName}/connections";
private final java.util.regex.Pattern RESOURCE_NAME_PATTERN =
java.util.regex.Pattern.compile("^people/[^/]+$");
/**
* Provides a list of the authenticated user's contacts merged with any connected profiles.
*
* The request throws a 400 error if 'personFields' is not specified.
*
* Create a request for the method "connections.list".
*
* This request holds the parameters needed by the the people server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param resourceName The resource name to return connections for. Only `people/me` is valid.
* @since 1.13
*/
protected List(java.lang.String resourceName) {
super(PeopleService.this, "GET", REST_PATH, null, com.google.api.services.people.v1.model.ListConnectionsResponse.class);
this.resourceName = com.google.api.client.util.Preconditions.checkNotNull(resourceName, "Required parameter resourceName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource name to return connections for. Only `people/me` is valid. */
@com.google.api.client.util.Key
private java.lang.String resourceName;
/** The resource name to return connections for. Only `people/me` is valid.
*/
public java.lang.String getResourceName() {
return resourceName;
}
/** The resource name to return connections for. Only `people/me` is valid. */
public List setResourceName(java.lang.String resourceName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_NAME_PATTERN.matcher(resourceName).matches(),
"Parameter resourceName must conform to the pattern " +
"^people/[^/]+$");
}
this.resourceName = resourceName;
return this;
}
/**
* Whether the response should include a sync token, which can be used to get all changes
* since the last request. For subsequent sync requests use the `sync_token` param instead.
* Initial sync requests that specify `request_sync_token` have an additional rate limit.
*/
@com.google.api.client.util.Key
private java.lang.Boolean requestSyncToken;
/** Whether the response should include a sync token, which can be used to get all changes since the
last request. For subsequent sync requests use the `sync_token` param instead. Initial sync
requests that specify `request_sync_token` have an additional rate limit.
*/
public java.lang.Boolean getRequestSyncToken() {
return requestSyncToken;
}
/**
* Whether the response should include a sync token, which can be used to get all changes
* since the last request. For subsequent sync requests use the `sync_token` param instead.
* Initial sync requests that specify `request_sync_token` have an additional rate limit.
*/
public List setRequestSyncToken(java.lang.Boolean requestSyncToken) {
this.requestSyncToken = requestSyncToken;
return this;
}
/** The token of the page to be returned. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token of the page to be returned.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token of the page to be returned. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The number of connections to include in the response. Valid values are between 1 and
* 2000, inclusive. Defaults to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The number of connections to include in the response. Valid values are between 1 and 2000,
inclusive. Defaults to 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The number of connections to include in the response. Valid values are between 1 and
* 2000, inclusive. Defaults to 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* **Required.** Comma-separated list of person fields to be included in the response. Each
* path should start with `person.`: for example, `person.names` or `person.photos`.
*/
@com.google.api.client.util.Key("requestMask.includeField")
private String requestMaskIncludeField;
/**** Required.** Comma-separated list of person fields to be included in the response. Each path
** should start with `person.`: for example, `person.names` or `person.photos`.
**
*/
public String getRequestMaskIncludeField() {
return requestMaskIncludeField;
}
/**
* **Required.** Comma-separated list of person fields to be included in the response. Each
* path should start with `person.`: for example, `person.names` or `person.photos`.
*/
public List setRequestMaskIncludeField(String requestMaskIncludeField) {
this.requestMaskIncludeField = requestMaskIncludeField;
return this;
}
/**
* A sync token returned by a previous call to `people.connections.list`. Only resources
* changed since the sync token was created will be returned. Sync requests that specify
* `sync_token` have an additional rate limit.
*/
@com.google.api.client.util.Key
private java.lang.String syncToken;
/** A sync token returned by a previous call to `people.connections.list`. Only resources changed since
the sync token was created will be returned. Sync requests that specify `sync_token` have an
additional rate limit.
*/
public java.lang.String getSyncToken() {
return syncToken;
}
/**
* A sync token returned by a previous call to `people.connections.list`. Only resources
* changed since the sync token was created will be returned. Sync requests that specify
* `sync_token` have an additional rate limit.
*/
public List setSyncToken(java.lang.String syncToken) {
this.syncToken = syncToken;
return this;
}
/**
* **Required.** A field mask to restrict which fields on each person are returned. Multiple
* fields can be specified by separating them with commas. Valid values are:
*
* * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos *
* emailAddresses * events * genders * imClients * interests * locales * memberships *
* metadata * names * nicknames * occupations * organizations * phoneNumbers * photos *
* relations * relationshipInterests * relationshipStatuses * residences * sipAddresses *
* skills * taglines * urls * userDefined
*/
@com.google.api.client.util.Key
private String personFields;
/**** Required.** A field mask to restrict which fields on each person are returned. Multiple fields
** can be specified by separating them with commas. Valid values are:
**
** * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos * emailAddresses
** * events * genders * imClients * interests * locales * memberships * metadata * names * nicknames
** * occupations * organizations * phoneNumbers * photos * relations * relationshipInterests *
** relationshipStatuses * residences * sipAddresses * skills * taglines * urls * userDefined
**
*/
public String getPersonFields() {
return personFields;
}
/**
* **Required.** A field mask to restrict which fields on each person are returned. Multiple
* fields can be specified by separating them with commas. Valid values are:
*
* * addresses * ageRanges * biographies * birthdays * braggingRights * coverPhotos *
* emailAddresses * events * genders * imClients * interests * locales * memberships *
* metadata * names * nicknames * occupations * organizations * phoneNumbers * photos *
* relations * relationshipInterests * relationshipStatuses * residences * sipAddresses *
* skills * taglines * urls * userDefined
*/
public List setPersonFields(String personFields) {
this.personFields = personFields;
return this;
}
/**
* The order in which the connections should be sorted. Defaults to
* `LAST_MODIFIED_ASCENDING`.
*/
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** The order in which the connections should be sorted. Defaults to `LAST_MODIFIED_ASCENDING`.
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/**
* The order in which the connections should be sorted. Defaults to
* `LAST_MODIFIED_ASCENDING`.
*/
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link PeopleService}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link PeopleService}. */
@Override
public PeopleService build() {
return new PeopleService(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link PeopleServiceRequestInitializer}.
*
* @since 1.12
*/
public Builder setPeopleServiceRequestInitializer(
PeopleServiceRequestInitializer peopleserviceRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(peopleserviceRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}