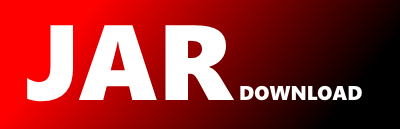
com.google.api.services.people.v1.model.ContactGroup Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-05-04 17:28:03 UTC)
* on 2018-09-05 at 04:11:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.people.v1.model;
/**
* A contact group.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the People API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ContactGroup extends com.google.api.client.json.GenericJson {
/**
* The [HTTP entity tag](https://en.wikipedia.org/wiki/HTTP_ETag) of the resource. Used for web
* cache validation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* The read-only name translated and formatted in the viewer's account locale or the `Accept-
* Language` HTTP header locale for system groups names. Group names set by the owner are the same
* as name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String formattedName;
/**
* The read-only contact group type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String groupType;
/**
* The total number of contacts in the group irrespective of max members in specified in the
* request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer memberCount;
/**
* The list of contact person resource names that are members of the contact group. The field is
* not populated for LIST requests and can only be updated through the
* [ModifyContactGroupMembers](/people/api/rest/v1/contactgroups/members/modify).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List memberResourceNames;
/**
* Metadata about the contact group.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ContactGroupMetadata metadata;
/**
* The contact group name set by the group owner or a system provided name for system groups.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The resource name for the contact group, assigned by the server. An ASCII string, in the form
* of `contactGroups/`contact_group_id.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceName;
/**
* The [HTTP entity tag](https://en.wikipedia.org/wiki/HTTP_ETag) of the resource. Used for web
* cache validation.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* The [HTTP entity tag](https://en.wikipedia.org/wiki/HTTP_ETag) of the resource. Used for web
* cache validation.
* @param etag etag or {@code null} for none
*/
public ContactGroup setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* The read-only name translated and formatted in the viewer's account locale or the `Accept-
* Language` HTTP header locale for system groups names. Group names set by the owner are the same
* as name.
* @return value or {@code null} for none
*/
public java.lang.String getFormattedName() {
return formattedName;
}
/**
* The read-only name translated and formatted in the viewer's account locale or the `Accept-
* Language` HTTP header locale for system groups names. Group names set by the owner are the same
* as name.
* @param formattedName formattedName or {@code null} for none
*/
public ContactGroup setFormattedName(java.lang.String formattedName) {
this.formattedName = formattedName;
return this;
}
/**
* The read-only contact group type.
* @return value or {@code null} for none
*/
public java.lang.String getGroupType() {
return groupType;
}
/**
* The read-only contact group type.
* @param groupType groupType or {@code null} for none
*/
public ContactGroup setGroupType(java.lang.String groupType) {
this.groupType = groupType;
return this;
}
/**
* The total number of contacts in the group irrespective of max members in specified in the
* request.
* @return value or {@code null} for none
*/
public java.lang.Integer getMemberCount() {
return memberCount;
}
/**
* The total number of contacts in the group irrespective of max members in specified in the
* request.
* @param memberCount memberCount or {@code null} for none
*/
public ContactGroup setMemberCount(java.lang.Integer memberCount) {
this.memberCount = memberCount;
return this;
}
/**
* The list of contact person resource names that are members of the contact group. The field is
* not populated for LIST requests and can only be updated through the
* [ModifyContactGroupMembers](/people/api/rest/v1/contactgroups/members/modify).
* @return value or {@code null} for none
*/
public java.util.List getMemberResourceNames() {
return memberResourceNames;
}
/**
* The list of contact person resource names that are members of the contact group. The field is
* not populated for LIST requests and can only be updated through the
* [ModifyContactGroupMembers](/people/api/rest/v1/contactgroups/members/modify).
* @param memberResourceNames memberResourceNames or {@code null} for none
*/
public ContactGroup setMemberResourceNames(java.util.List memberResourceNames) {
this.memberResourceNames = memberResourceNames;
return this;
}
/**
* Metadata about the contact group.
* @return value or {@code null} for none
*/
public ContactGroupMetadata getMetadata() {
return metadata;
}
/**
* Metadata about the contact group.
* @param metadata metadata or {@code null} for none
*/
public ContactGroup setMetadata(ContactGroupMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* The contact group name set by the group owner or a system provided name for system groups.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The contact group name set by the group owner or a system provided name for system groups.
* @param name name or {@code null} for none
*/
public ContactGroup setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The resource name for the contact group, assigned by the server. An ASCII string, in the form
* of `contactGroups/`contact_group_id.
* @return value or {@code null} for none
*/
public java.lang.String getResourceName() {
return resourceName;
}
/**
* The resource name for the contact group, assigned by the server. An ASCII string, in the form
* of `contactGroups/`contact_group_id.
* @param resourceName resourceName or {@code null} for none
*/
public ContactGroup setResourceName(java.lang.String resourceName) {
this.resourceName = resourceName;
return this;
}
@Override
public ContactGroup set(String fieldName, Object value) {
return (ContactGroup) super.set(fieldName, value);
}
@Override
public ContactGroup clone() {
return (ContactGroup) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy