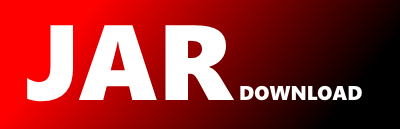
com.google.api.services.playmoviespartner.model.StoreInfo Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-08-10 at 21:12:03 UTC
* Modify at your own risk.
*/
package com.google.api.services.playmoviespartner.model;
/**
* Information about a playable sequence (video) associated with an Edit and available at the Google
* Play Store.
*
* Internally, each StoreInfo is uniquely identified by a `video_id` and `country`.
*
* Externally, Title-level EIDR or Edit-level EIDR, if provided, can also be used to identify a
* specific title or edit in a country.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play Movies Partner API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class StoreInfo extends com.google.api.client.json.GenericJson {
/**
* Audio tracks available for this Edit.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List audioTracks;
/**
* Country where Edit is available in ISO 3166-1 alpha-2 country code. Example: "US".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String country;
/**
* Edit-level EIDR ID. Example: "10.5240/1489-49A2-3956-4B2D-FE16-6".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String editLevelEidr;
/**
* The number assigned to the episode within a season. Only available on TV Edits. Example: "1".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String episodeNumber;
/**
* Whether the Edit has a 5.1 channel audio track.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasAudio51;
/**
* Whether the Edit has a EST offer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasEstOffer;
/**
* Whether the Edit has a HD offer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasHdOffer;
/**
* Whether the Edit has info cards.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasInfoCards;
/**
* Whether the Edit has a SD offer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasSdOffer;
/**
* Whether the Edit has a VOD offer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hasVodOffer;
/**
* Timestamp when the Edit went live on the Store.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String liveTime;
/**
* Knowledge Graph ID associated to this Edit, if available. This ID links the Edit to its
* knowledge entity, externally accessible at http://freebase.com. In the absense of Title EIDR or
* Edit EIDR, this ID helps link together multiple Edits across countries. Example: '/m/0ffx29'
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mid;
/**
* Default Edit name, usually in the language of the country of origin. Example: "Googlers, The".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Name of the post-production houses that manage the Edit.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List pphNames;
/**
* Google-generated ID identifying the season linked to the Edit. Only available for TV Edits.
* Example: 'ster23ex'
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String seasonId;
/**
* Default Season name, usually in the language of the country of origin. Only available for TV
* Edits Example: "Googlers, The - A Brave New World".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String seasonName;
/**
* The number assigned to the season within a show. Only available on TV Edits. Example: "1".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String seasonNumber;
/**
* Google-generated ID identifying the show linked to the Edit. Only available for TV Edits.
* Example: 'et2hsue_x'
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String showId;
/**
* Default Show name, usually in the language of the country of origin. Only available for TV
* Edits Example: "Googlers, The".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String showName;
/**
* Name of the studio that owns the Edit ordered.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String studioName;
/**
* Subtitles available for this Edit.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List subtitles;
/**
* Title-level EIDR ID. Example: "10.5240/1489-49A2-3956-4B2D-FE16-5".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String titleLevelEidr;
/**
* Google-generated ID identifying the trailer linked to the Edit. Example: 'bhd_4e_cx'
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String trailerId;
/**
* Edit type, like Movie, Episode or Season.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* Google-generated ID identifying the video linked to the Edit. Example: 'gtry456_xc'
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String videoId;
/**
* Audio tracks available for this Edit.
* @return value or {@code null} for none
*/
public java.util.List getAudioTracks() {
return audioTracks;
}
/**
* Audio tracks available for this Edit.
* @param audioTracks audioTracks or {@code null} for none
*/
public StoreInfo setAudioTracks(java.util.List audioTracks) {
this.audioTracks = audioTracks;
return this;
}
/**
* Country where Edit is available in ISO 3166-1 alpha-2 country code. Example: "US".
* @return value or {@code null} for none
*/
public java.lang.String getCountry() {
return country;
}
/**
* Country where Edit is available in ISO 3166-1 alpha-2 country code. Example: "US".
* @param country country or {@code null} for none
*/
public StoreInfo setCountry(java.lang.String country) {
this.country = country;
return this;
}
/**
* Edit-level EIDR ID. Example: "10.5240/1489-49A2-3956-4B2D-FE16-6".
* @return value or {@code null} for none
*/
public java.lang.String getEditLevelEidr() {
return editLevelEidr;
}
/**
* Edit-level EIDR ID. Example: "10.5240/1489-49A2-3956-4B2D-FE16-6".
* @param editLevelEidr editLevelEidr or {@code null} for none
*/
public StoreInfo setEditLevelEidr(java.lang.String editLevelEidr) {
this.editLevelEidr = editLevelEidr;
return this;
}
/**
* The number assigned to the episode within a season. Only available on TV Edits. Example: "1".
* @return value or {@code null} for none
*/
public java.lang.String getEpisodeNumber() {
return episodeNumber;
}
/**
* The number assigned to the episode within a season. Only available on TV Edits. Example: "1".
* @param episodeNumber episodeNumber or {@code null} for none
*/
public StoreInfo setEpisodeNumber(java.lang.String episodeNumber) {
this.episodeNumber = episodeNumber;
return this;
}
/**
* Whether the Edit has a 5.1 channel audio track.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasAudio51() {
return hasAudio51;
}
/**
* Whether the Edit has a 5.1 channel audio track.
* @param hasAudio51 hasAudio51 or {@code null} for none
*/
public StoreInfo setHasAudio51(java.lang.Boolean hasAudio51) {
this.hasAudio51 = hasAudio51;
return this;
}
/**
* Whether the Edit has a EST offer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasEstOffer() {
return hasEstOffer;
}
/**
* Whether the Edit has a EST offer.
* @param hasEstOffer hasEstOffer or {@code null} for none
*/
public StoreInfo setHasEstOffer(java.lang.Boolean hasEstOffer) {
this.hasEstOffer = hasEstOffer;
return this;
}
/**
* Whether the Edit has a HD offer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasHdOffer() {
return hasHdOffer;
}
/**
* Whether the Edit has a HD offer.
* @param hasHdOffer hasHdOffer or {@code null} for none
*/
public StoreInfo setHasHdOffer(java.lang.Boolean hasHdOffer) {
this.hasHdOffer = hasHdOffer;
return this;
}
/**
* Whether the Edit has info cards.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasInfoCards() {
return hasInfoCards;
}
/**
* Whether the Edit has info cards.
* @param hasInfoCards hasInfoCards or {@code null} for none
*/
public StoreInfo setHasInfoCards(java.lang.Boolean hasInfoCards) {
this.hasInfoCards = hasInfoCards;
return this;
}
/**
* Whether the Edit has a SD offer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasSdOffer() {
return hasSdOffer;
}
/**
* Whether the Edit has a SD offer.
* @param hasSdOffer hasSdOffer or {@code null} for none
*/
public StoreInfo setHasSdOffer(java.lang.Boolean hasSdOffer) {
this.hasSdOffer = hasSdOffer;
return this;
}
/**
* Whether the Edit has a VOD offer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHasVodOffer() {
return hasVodOffer;
}
/**
* Whether the Edit has a VOD offer.
* @param hasVodOffer hasVodOffer or {@code null} for none
*/
public StoreInfo setHasVodOffer(java.lang.Boolean hasVodOffer) {
this.hasVodOffer = hasVodOffer;
return this;
}
/**
* Timestamp when the Edit went live on the Store.
* @return value or {@code null} for none
*/
public String getLiveTime() {
return liveTime;
}
/**
* Timestamp when the Edit went live on the Store.
* @param liveTime liveTime or {@code null} for none
*/
public StoreInfo setLiveTime(String liveTime) {
this.liveTime = liveTime;
return this;
}
/**
* Knowledge Graph ID associated to this Edit, if available. This ID links the Edit to its
* knowledge entity, externally accessible at http://freebase.com. In the absense of Title EIDR or
* Edit EIDR, this ID helps link together multiple Edits across countries. Example: '/m/0ffx29'
* @return value or {@code null} for none
*/
public java.lang.String getMid() {
return mid;
}
/**
* Knowledge Graph ID associated to this Edit, if available. This ID links the Edit to its
* knowledge entity, externally accessible at http://freebase.com. In the absense of Title EIDR or
* Edit EIDR, this ID helps link together multiple Edits across countries. Example: '/m/0ffx29'
* @param mid mid or {@code null} for none
*/
public StoreInfo setMid(java.lang.String mid) {
this.mid = mid;
return this;
}
/**
* Default Edit name, usually in the language of the country of origin. Example: "Googlers, The".
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Default Edit name, usually in the language of the country of origin. Example: "Googlers, The".
* @param name name or {@code null} for none
*/
public StoreInfo setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Name of the post-production houses that manage the Edit.
* @return value or {@code null} for none
*/
public java.util.List getPphNames() {
return pphNames;
}
/**
* Name of the post-production houses that manage the Edit.
* @param pphNames pphNames or {@code null} for none
*/
public StoreInfo setPphNames(java.util.List pphNames) {
this.pphNames = pphNames;
return this;
}
/**
* Google-generated ID identifying the season linked to the Edit. Only available for TV Edits.
* Example: 'ster23ex'
* @return value or {@code null} for none
*/
public java.lang.String getSeasonId() {
return seasonId;
}
/**
* Google-generated ID identifying the season linked to the Edit. Only available for TV Edits.
* Example: 'ster23ex'
* @param seasonId seasonId or {@code null} for none
*/
public StoreInfo setSeasonId(java.lang.String seasonId) {
this.seasonId = seasonId;
return this;
}
/**
* Default Season name, usually in the language of the country of origin. Only available for TV
* Edits Example: "Googlers, The - A Brave New World".
* @return value or {@code null} for none
*/
public java.lang.String getSeasonName() {
return seasonName;
}
/**
* Default Season name, usually in the language of the country of origin. Only available for TV
* Edits Example: "Googlers, The - A Brave New World".
* @param seasonName seasonName or {@code null} for none
*/
public StoreInfo setSeasonName(java.lang.String seasonName) {
this.seasonName = seasonName;
return this;
}
/**
* The number assigned to the season within a show. Only available on TV Edits. Example: "1".
* @return value or {@code null} for none
*/
public java.lang.String getSeasonNumber() {
return seasonNumber;
}
/**
* The number assigned to the season within a show. Only available on TV Edits. Example: "1".
* @param seasonNumber seasonNumber or {@code null} for none
*/
public StoreInfo setSeasonNumber(java.lang.String seasonNumber) {
this.seasonNumber = seasonNumber;
return this;
}
/**
* Google-generated ID identifying the show linked to the Edit. Only available for TV Edits.
* Example: 'et2hsue_x'
* @return value or {@code null} for none
*/
public java.lang.String getShowId() {
return showId;
}
/**
* Google-generated ID identifying the show linked to the Edit. Only available for TV Edits.
* Example: 'et2hsue_x'
* @param showId showId or {@code null} for none
*/
public StoreInfo setShowId(java.lang.String showId) {
this.showId = showId;
return this;
}
/**
* Default Show name, usually in the language of the country of origin. Only available for TV
* Edits Example: "Googlers, The".
* @return value or {@code null} for none
*/
public java.lang.String getShowName() {
return showName;
}
/**
* Default Show name, usually in the language of the country of origin. Only available for TV
* Edits Example: "Googlers, The".
* @param showName showName or {@code null} for none
*/
public StoreInfo setShowName(java.lang.String showName) {
this.showName = showName;
return this;
}
/**
* Name of the studio that owns the Edit ordered.
* @return value or {@code null} for none
*/
public java.lang.String getStudioName() {
return studioName;
}
/**
* Name of the studio that owns the Edit ordered.
* @param studioName studioName or {@code null} for none
*/
public StoreInfo setStudioName(java.lang.String studioName) {
this.studioName = studioName;
return this;
}
/**
* Subtitles available for this Edit.
* @return value or {@code null} for none
*/
public java.util.List getSubtitles() {
return subtitles;
}
/**
* Subtitles available for this Edit.
* @param subtitles subtitles or {@code null} for none
*/
public StoreInfo setSubtitles(java.util.List subtitles) {
this.subtitles = subtitles;
return this;
}
/**
* Title-level EIDR ID. Example: "10.5240/1489-49A2-3956-4B2D-FE16-5".
* @return value or {@code null} for none
*/
public java.lang.String getTitleLevelEidr() {
return titleLevelEidr;
}
/**
* Title-level EIDR ID. Example: "10.5240/1489-49A2-3956-4B2D-FE16-5".
* @param titleLevelEidr titleLevelEidr or {@code null} for none
*/
public StoreInfo setTitleLevelEidr(java.lang.String titleLevelEidr) {
this.titleLevelEidr = titleLevelEidr;
return this;
}
/**
* Google-generated ID identifying the trailer linked to the Edit. Example: 'bhd_4e_cx'
* @return value or {@code null} for none
*/
public java.lang.String getTrailerId() {
return trailerId;
}
/**
* Google-generated ID identifying the trailer linked to the Edit. Example: 'bhd_4e_cx'
* @param trailerId trailerId or {@code null} for none
*/
public StoreInfo setTrailerId(java.lang.String trailerId) {
this.trailerId = trailerId;
return this;
}
/**
* Edit type, like Movie, Episode or Season.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Edit type, like Movie, Episode or Season.
* @param type type or {@code null} for none
*/
public StoreInfo setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* Google-generated ID identifying the video linked to the Edit. Example: 'gtry456_xc'
* @return value or {@code null} for none
*/
public java.lang.String getVideoId() {
return videoId;
}
/**
* Google-generated ID identifying the video linked to the Edit. Example: 'gtry456_xc'
* @param videoId videoId or {@code null} for none
*/
public StoreInfo setVideoId(java.lang.String videoId) {
this.videoId = videoId;
return this;
}
@Override
public StoreInfo set(String fieldName, Object value) {
return (StoreInfo) super.set(fieldName, value);
}
@Override
public StoreInfo clone() {
return (StoreInfo) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy