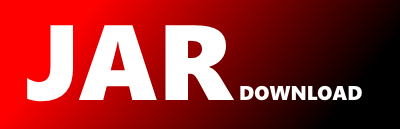
com.google.api.services.plus.Plus Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-06-10 at 05:00:13 UTC
* Modify at your own risk.
*/
package com.google.api.services.plus;
/**
* Service definition for Plus (v1).
*
*
* Builds on top of the Google+ platform.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link PlusRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Plus extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Google+ API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "plus/v1/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Plus(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Plus(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Activities collection.
*
* The typical use is:
*
* {@code Plus plus = new Plus(...);}
* {@code Plus.Activities.List request = plus.activities().list(parameters ...)}
*
*
* @return the resource collection
*/
public Activities activities() {
return new Activities();
}
/**
* The "activities" collection of methods.
*/
public class Activities {
/**
* Get an activity.
*
* Create a request for the method "activities.get".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param activityId The ID of the activity to get.
* @return the request
*/
public Get get(java.lang.String activityId) throws java.io.IOException {
Get result = new Get(activityId);
initialize(result);
return result;
}
public class Get extends PlusRequest {
private static final String REST_PATH = "activities/{activityId}";
/**
* Get an activity.
*
* Create a request for the method "activities.get".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param activityId The ID of the activity to get.
* @since 1.13
*/
protected Get(java.lang.String activityId) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.Activity.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the activity to get. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** The ID of the activity to get.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** The ID of the activity to get. */
public Get setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all of the activities in the specified collection for a particular user.
*
* Create a request for the method "activities.list".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The ID of the user to get activities for. The special value "me" can be used to indicate the
* authenticated user.
* @param collection The collection of activities to list.
* @return the request
*/
public List list(java.lang.String userId, java.lang.String collection) throws java.io.IOException {
List result = new List(userId, collection);
initialize(result);
return result;
}
public class List extends PlusRequest {
private static final String REST_PATH = "people/{userId}/activities/{collection}";
/**
* List all of the activities in the specified collection for a particular user.
*
* Create a request for the method "activities.list".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The ID of the user to get activities for. The special value "me" can be used to indicate the
* authenticated user.
* @param collection The collection of activities to list.
* @since 1.13
*/
protected List(java.lang.String userId, java.lang.String collection) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.ActivityFeed.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The ID of the user to get activities for. The special value "me" can be used to indicate
* the authenticated user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user to get activities for. The special value "me" can be used to indicate the
authenticated user.
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The ID of the user to get activities for. The special value "me" can be used to indicate
* the authenticated user.
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The collection of activities to list. */
@com.google.api.client.util.Key
private java.lang.String collection;
/** The collection of activities to list.
*/
public java.lang.String getCollection() {
return collection;
}
/** The collection of activities to list. */
public List setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/**
* The maximum number of activities to include in the response, which is used for paging. For
* any response, the actual number returned might be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of activities to include in the response, which is used for paging. For any
response, the actual number returned might be less than the specified maxResults. [default: 20]
[minimum: 1] [maximum: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of activities to include in the response, which is used for paging. For
* any response, the actual number returned might be less than the specified maxResults.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of "nextPageToken" from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Search public activities.
*
* Create a request for the method "activities.search".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param query Full-text search query string.
* @return the request
*/
public Search search(java.lang.String query) throws java.io.IOException {
Search result = new Search(query);
initialize(result);
return result;
}
public class Search extends PlusRequest {
private static final String REST_PATH = "activities";
/**
* Search public activities.
*
* Create a request for the method "activities.search".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation. {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param query Full-text search query string.
* @since 1.13
*/
protected Search(java.lang.String query) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.ActivityFeed.class);
this.query = com.google.api.client.util.Preconditions.checkNotNull(query, "Required parameter query must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUserIp(java.lang.String userIp) {
return (Search) super.setUserIp(userIp);
}
/** Full-text search query string. */
@com.google.api.client.util.Key
private java.lang.String query;
/** Full-text search query string.
*/
public java.lang.String getQuery() {
return query;
}
/** Full-text search query string. */
public Search setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* Specify the preferred language to search with. See search language codes for available
* values.
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** Specify the preferred language to search with. See search language codes for available values.
[default: en-US]
*/
public java.lang.String getLanguage() {
return language;
}
/**
* Specify the preferred language to search with. See search language codes for available
* values.
*/
public Search setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* The maximum number of activities to include in the response, which is used for paging. For
* any response, the actual number returned might be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of activities to include in the response, which is used for paging. For any
response, the actual number returned might be less than the specified maxResults. [default: 10]
[minimum: 1] [maximum: 20]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of activities to include in the response, which is used for paging. For
* any response, the actual number returned might be less than the specified maxResults.
*/
public Search setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Specifies how to order search results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Specifies how to order search results. [default: recent]
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Specifies how to order search results. */
public Search setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response. This token can be of any length.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of "nextPageToken" from the previous response. This token
can be of any length.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response. This token can be of any length.
*/
public Search setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Comments collection.
*
* The typical use is:
*
* {@code Plus plus = new Plus(...);}
* {@code Plus.Comments.List request = plus.comments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Comments comments() {
return new Comments();
}
/**
* The "comments" collection of methods.
*/
public class Comments {
/**
* Get a comment.
*
* Create a request for the method "comments.get".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param commentId The ID of the comment to get.
* @return the request
*/
public Get get(java.lang.String commentId) throws java.io.IOException {
Get result = new Get(commentId);
initialize(result);
return result;
}
public class Get extends PlusRequest {
private static final String REST_PATH = "comments/{commentId}";
/**
* Get a comment.
*
* Create a request for the method "comments.get".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param commentId The ID of the comment to get.
* @since 1.13
*/
protected Get(java.lang.String commentId) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.Comment.class);
this.commentId = com.google.api.client.util.Preconditions.checkNotNull(commentId, "Required parameter commentId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the comment to get. */
@com.google.api.client.util.Key
private java.lang.String commentId;
/** The ID of the comment to get.
*/
public java.lang.String getCommentId() {
return commentId;
}
/** The ID of the comment to get. */
public Get setCommentId(java.lang.String commentId) {
this.commentId = commentId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all of the comments for an activity.
*
* Create a request for the method "comments.list".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param activityId The ID of the activity to get comments for.
* @return the request
*/
public List list(java.lang.String activityId) throws java.io.IOException {
List result = new List(activityId);
initialize(result);
return result;
}
public class List extends PlusRequest {
private static final String REST_PATH = "activities/{activityId}/comments";
/**
* List all of the comments for an activity.
*
* Create a request for the method "comments.list".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param activityId The ID of the activity to get comments for.
* @since 1.13
*/
protected List(java.lang.String activityId) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.CommentFeed.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the activity to get comments for. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** The ID of the activity to get comments for.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** The ID of the activity to get comments for. */
public List setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
/**
* The maximum number of comments to include in the response, which is used for paging. For
* any response, the actual number returned might be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of comments to include in the response, which is used for paging. For any
response, the actual number returned might be less than the specified maxResults. [default: 20]
[minimum: 0] [maximum: 500]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of comments to include in the response, which is used for paging. For
* any response, the actual number returned might be less than the specified maxResults.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of "nextPageToken" from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The order in which to sort the list of comments. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** The order in which to sort the list of comments. [default: ascending]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** The order in which to sort the list of comments. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the People collection.
*
* The typical use is:
*
* {@code Plus plus = new Plus(...);}
* {@code Plus.People.List request = plus.people().list(parameters ...)}
*
*
* @return the resource collection
*/
public People people() {
return new People();
}
/**
* The "people" collection of methods.
*/
public class People {
/**
* Get a person's profile. If your app uses scope https://www.googleapis.com/auth/plus.login, this
* method is guaranteed to return ageRange and language.
*
* Create a request for the method "people.get".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId The ID of the person to get the profile for. The special value "me" can be used to indicate the
* authenticated user.
* @return the request
*/
public Get get(java.lang.String userId) throws java.io.IOException {
Get result = new Get(userId);
initialize(result);
return result;
}
public class Get extends PlusRequest {
private static final String REST_PATH = "people/{userId}";
/**
* Get a person's profile. If your app uses scope https://www.googleapis.com/auth/plus.login, this
* method is guaranteed to return ageRange and language.
*
* Create a request for the method "people.get".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The ID of the person to get the profile for. The special value "me" can be used to indicate the
* authenticated user.
* @since 1.13
*/
protected Get(java.lang.String userId) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.Person.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The ID of the person to get the profile for. The special value "me" can be used to indicate
* the authenticated user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the person to get the profile for. The special value "me" can be used to indicate the
authenticated user.
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The ID of the person to get the profile for. The special value "me" can be used to indicate
* the authenticated user.
*/
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all of the people in the specified collection.
*
* Create a request for the method "people.list".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId Get the collection of people for the person identified. Use "me" to indicate the authenticated user.
* @param collection The collection of people to list.
* @return the request
*/
public List list(java.lang.String userId, java.lang.String collection) throws java.io.IOException {
List result = new List(userId, collection);
initialize(result);
return result;
}
public class List extends PlusRequest {
private static final String REST_PATH = "people/{userId}/people/{collection}";
/**
* List all of the people in the specified collection.
*
* Create a request for the method "people.list".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId Get the collection of people for the person identified. Use "me" to indicate the authenticated user.
* @param collection The collection of people to list.
* @since 1.13
*/
protected List(java.lang.String userId, java.lang.String collection) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.PeopleFeed.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Get the collection of people for the person identified. Use "me" to indicate the
* authenticated user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** Get the collection of people for the person identified. Use "me" to indicate the authenticated
user.
*/
public java.lang.String getUserId() {
return userId;
}
/**
* Get the collection of people for the person identified. Use "me" to indicate the
* authenticated user.
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The collection of people to list. */
@com.google.api.client.util.Key
private java.lang.String collection;
/** The collection of people to list.
*/
public java.lang.String getCollection() {
return collection;
}
/** The collection of people to list. */
public List setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/**
* The maximum number of people to include in the response, which is used for paging. For any
* response, the actual number returned might be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of people to include in the response, which is used for paging. For any
response, the actual number returned might be less than the specified maxResults. [default: 100]
[minimum: 1] [maximum: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of people to include in the response, which is used for paging. For any
* response, the actual number returned might be less than the specified maxResults.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The order to return people in. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** The order to return people in.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** The order to return people in. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of "nextPageToken" from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* List all of the people in the specified collection for a particular activity.
*
* Create a request for the method "people.listByActivity".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link ListByActivity#execute()} method to invoke the remote operation.
*
* @param activityId The ID of the activity to get the list of people for.
* @param collection The collection of people to list.
* @return the request
*/
public ListByActivity listByActivity(java.lang.String activityId, java.lang.String collection) throws java.io.IOException {
ListByActivity result = new ListByActivity(activityId, collection);
initialize(result);
return result;
}
public class ListByActivity extends PlusRequest {
private static final String REST_PATH = "activities/{activityId}/people/{collection}";
/**
* List all of the people in the specified collection for a particular activity.
*
* Create a request for the method "people.listByActivity".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link ListByActivity#execute()} method to invoke the remote operation.
* {@link ListByActivity#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param activityId The ID of the activity to get the list of people for.
* @param collection The collection of people to list.
* @since 1.13
*/
protected ListByActivity(java.lang.String activityId, java.lang.String collection) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.PeopleFeed.class);
this.activityId = com.google.api.client.util.Preconditions.checkNotNull(activityId, "Required parameter activityId must be specified.");
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListByActivity setAlt(java.lang.String alt) {
return (ListByActivity) super.setAlt(alt);
}
@Override
public ListByActivity setFields(java.lang.String fields) {
return (ListByActivity) super.setFields(fields);
}
@Override
public ListByActivity setKey(java.lang.String key) {
return (ListByActivity) super.setKey(key);
}
@Override
public ListByActivity setOauthToken(java.lang.String oauthToken) {
return (ListByActivity) super.setOauthToken(oauthToken);
}
@Override
public ListByActivity setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListByActivity) super.setPrettyPrint(prettyPrint);
}
@Override
public ListByActivity setQuotaUser(java.lang.String quotaUser) {
return (ListByActivity) super.setQuotaUser(quotaUser);
}
@Override
public ListByActivity setUserIp(java.lang.String userIp) {
return (ListByActivity) super.setUserIp(userIp);
}
/** The ID of the activity to get the list of people for. */
@com.google.api.client.util.Key
private java.lang.String activityId;
/** The ID of the activity to get the list of people for.
*/
public java.lang.String getActivityId() {
return activityId;
}
/** The ID of the activity to get the list of people for. */
public ListByActivity setActivityId(java.lang.String activityId) {
this.activityId = activityId;
return this;
}
/** The collection of people to list. */
@com.google.api.client.util.Key
private java.lang.String collection;
/** The collection of people to list.
*/
public java.lang.String getCollection() {
return collection;
}
/** The collection of people to list. */
public ListByActivity setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/**
* The maximum number of people to include in the response, which is used for paging. For any
* response, the actual number returned might be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of people to include in the response, which is used for paging. For any
response, the actual number returned might be less than the specified maxResults. [default: 20]
[minimum: 1] [maximum: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of people to include in the response, which is used for paging. For any
* response, the actual number returned might be less than the specified maxResults.
*/
public ListByActivity setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of "nextPageToken" from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response.
*/
public ListByActivity setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListByActivity set(String parameterName, Object value) {
return (ListByActivity) super.set(parameterName, value);
}
}
/**
* Search all public profiles.
*
* Create a request for the method "people.search".
*
* This request holds the parameters needed by the plus server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param query Specify a query string for full text search of public text in all profiles.
* @return the request
*/
public Search search(java.lang.String query) throws java.io.IOException {
Search result = new Search(query);
initialize(result);
return result;
}
public class Search extends PlusRequest {
private static final String REST_PATH = "people";
/**
* Search all public profiles.
*
* Create a request for the method "people.search".
*
* This request holds the parameters needed by the the plus server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation. {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param query Specify a query string for full text search of public text in all profiles.
* @since 1.13
*/
protected Search(java.lang.String query) {
super(Plus.this, "GET", REST_PATH, null, com.google.api.services.plus.model.PeopleFeed.class);
this.query = com.google.api.client.util.Preconditions.checkNotNull(query, "Required parameter query must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUserIp(java.lang.String userIp) {
return (Search) super.setUserIp(userIp);
}
/** Specify a query string for full text search of public text in all profiles. */
@com.google.api.client.util.Key
private java.lang.String query;
/** Specify a query string for full text search of public text in all profiles.
*/
public java.lang.String getQuery() {
return query;
}
/** Specify a query string for full text search of public text in all profiles. */
public Search setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* Specify the preferred language to search with. See search language codes for available
* values.
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** Specify the preferred language to search with. See search language codes for available values.
[default: en-US]
*/
public java.lang.String getLanguage() {
return language;
}
/**
* Specify the preferred language to search with. See search language codes for available
* values.
*/
public Search setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* The maximum number of people to include in the response, which is used for paging. For any
* response, the actual number returned might be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of people to include in the response, which is used for paging. For any
response, the actual number returned might be less than the specified maxResults. [default: 25]
[minimum: 1] [maximum: 50]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of people to include in the response, which is used for paging. For any
* response, the actual number returned might be less than the specified maxResults.
*/
public Search setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response. This token can be of any length.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of "nextPageToken" from the previous response. This token
can be of any length.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of "nextPageToken" from the previous
* response. This token can be of any length.
*/
public Search setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Plus}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Plus}. */
@Override
public Plus build() {
return new Plus(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link PlusRequestInitializer}.
*
* @since 1.12
*/
public Builder setPlusRequestInitializer(
PlusRequestInitializer plusRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(plusRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}