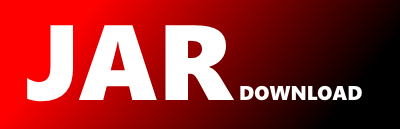
com.google.api.services.plus.model.Activity Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-06-10 at 05:00:13 UTC
* Modify at your own risk.
*/
package com.google.api.services.plus.model;
/**
* Model definition for Activity.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google+ API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Activity extends com.google.api.client.json.GenericJson {
/**
* Identifies who has access to see this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Acl access;
/**
* The person who performed this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Actor actor;
/**
* Street address where this activity occurred.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String address;
/**
* Additional content added by the person who shared this activity, applicable only when resharing
* an activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String annotation;
/**
* If this activity is a crosspost from another system, this property specifies the ID of the
* original activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String crosspostSource;
/**
* ETag of this response for caching purposes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* Latitude and longitude where this activity occurred. Format is latitude followed by longitude,
* space separated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String geocode;
/**
* The ID of this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* Identifies this resource as an activity. Value: "plus#activity".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The location where this activity occurred.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Place location;
/**
* The object of this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("object")
private PlusObject object__;
/**
* ID of the place where this activity occurred.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String placeId;
/**
* Name of the place where this activity occurred.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String placeName;
/**
* The service provider that initially published this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Provider provider;
/**
* The time at which this activity was initially published. Formatted as an RFC 3339 timestamp.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime published;
/**
* Radius, in meters, of the region where this activity occurred, centered at the latitude and
* longitude identified in geocode.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String radius;
/**
* Title of this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The time at which this activity was last updated. Formatted as an RFC 3339 timestamp.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime updated;
/**
* The link to this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* This activity's verb, which indicates the action that was performed. Possible values include,
* but are not limited to, the following values: - "post" - Publish content to the stream. -
* "share" - Reshare an activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String verb;
/**
* Identifies who has access to see this activity.
* @return value or {@code null} for none
*/
public Acl getAccess() {
return access;
}
/**
* Identifies who has access to see this activity.
* @param access access or {@code null} for none
*/
public Activity setAccess(Acl access) {
this.access = access;
return this;
}
/**
* The person who performed this activity.
* @return value or {@code null} for none
*/
public Actor getActor() {
return actor;
}
/**
* The person who performed this activity.
* @param actor actor or {@code null} for none
*/
public Activity setActor(Actor actor) {
this.actor = actor;
return this;
}
/**
* Street address where this activity occurred.
* @return value or {@code null} for none
*/
public java.lang.String getAddress() {
return address;
}
/**
* Street address where this activity occurred.
* @param address address or {@code null} for none
*/
public Activity setAddress(java.lang.String address) {
this.address = address;
return this;
}
/**
* Additional content added by the person who shared this activity, applicable only when resharing
* an activity.
* @return value or {@code null} for none
*/
public java.lang.String getAnnotation() {
return annotation;
}
/**
* Additional content added by the person who shared this activity, applicable only when resharing
* an activity.
* @param annotation annotation or {@code null} for none
*/
public Activity setAnnotation(java.lang.String annotation) {
this.annotation = annotation;
return this;
}
/**
* If this activity is a crosspost from another system, this property specifies the ID of the
* original activity.
* @return value or {@code null} for none
*/
public java.lang.String getCrosspostSource() {
return crosspostSource;
}
/**
* If this activity is a crosspost from another system, this property specifies the ID of the
* original activity.
* @param crosspostSource crosspostSource or {@code null} for none
*/
public Activity setCrosspostSource(java.lang.String crosspostSource) {
this.crosspostSource = crosspostSource;
return this;
}
/**
* ETag of this response for caching purposes.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* ETag of this response for caching purposes.
* @param etag etag or {@code null} for none
*/
public Activity setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Latitude and longitude where this activity occurred. Format is latitude followed by longitude,
* space separated.
* @return value or {@code null} for none
*/
public java.lang.String getGeocode() {
return geocode;
}
/**
* Latitude and longitude where this activity occurred. Format is latitude followed by longitude,
* space separated.
* @param geocode geocode or {@code null} for none
*/
public Activity setGeocode(java.lang.String geocode) {
this.geocode = geocode;
return this;
}
/**
* The ID of this activity.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of this activity.
* @param id id or {@code null} for none
*/
public Activity setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Identifies this resource as an activity. Value: "plus#activity".
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies this resource as an activity. Value: "plus#activity".
* @param kind kind or {@code null} for none
*/
public Activity setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The location where this activity occurred.
* @return value or {@code null} for none
*/
public Place getLocation() {
return location;
}
/**
* The location where this activity occurred.
* @param location location or {@code null} for none
*/
public Activity setLocation(Place location) {
this.location = location;
return this;
}
/**
* The object of this activity.
* @return value or {@code null} for none
*/
public PlusObject getObject() {
return object__;
}
/**
* The object of this activity.
* @param object__ object__ or {@code null} for none
*/
public Activity setObject(PlusObject object__) {
this.object__ = object__;
return this;
}
/**
* ID of the place where this activity occurred.
* @return value or {@code null} for none
*/
public java.lang.String getPlaceId() {
return placeId;
}
/**
* ID of the place where this activity occurred.
* @param placeId placeId or {@code null} for none
*/
public Activity setPlaceId(java.lang.String placeId) {
this.placeId = placeId;
return this;
}
/**
* Name of the place where this activity occurred.
* @return value or {@code null} for none
*/
public java.lang.String getPlaceName() {
return placeName;
}
/**
* Name of the place where this activity occurred.
* @param placeName placeName or {@code null} for none
*/
public Activity setPlaceName(java.lang.String placeName) {
this.placeName = placeName;
return this;
}
/**
* The service provider that initially published this activity.
* @return value or {@code null} for none
*/
public Provider getProvider() {
return provider;
}
/**
* The service provider that initially published this activity.
* @param provider provider or {@code null} for none
*/
public Activity setProvider(Provider provider) {
this.provider = provider;
return this;
}
/**
* The time at which this activity was initially published. Formatted as an RFC 3339 timestamp.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getPublished() {
return published;
}
/**
* The time at which this activity was initially published. Formatted as an RFC 3339 timestamp.
* @param published published or {@code null} for none
*/
public Activity setPublished(com.google.api.client.util.DateTime published) {
this.published = published;
return this;
}
/**
* Radius, in meters, of the region where this activity occurred, centered at the latitude and
* longitude identified in geocode.
* @return value or {@code null} for none
*/
public java.lang.String getRadius() {
return radius;
}
/**
* Radius, in meters, of the region where this activity occurred, centered at the latitude and
* longitude identified in geocode.
* @param radius radius or {@code null} for none
*/
public Activity setRadius(java.lang.String radius) {
this.radius = radius;
return this;
}
/**
* Title of this activity.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Title of this activity.
* @param title title or {@code null} for none
*/
public Activity setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* The time at which this activity was last updated. Formatted as an RFC 3339 timestamp.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getUpdated() {
return updated;
}
/**
* The time at which this activity was last updated. Formatted as an RFC 3339 timestamp.
* @param updated updated or {@code null} for none
*/
public Activity setUpdated(com.google.api.client.util.DateTime updated) {
this.updated = updated;
return this;
}
/**
* The link to this activity.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The link to this activity.
* @param url url or {@code null} for none
*/
public Activity setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* This activity's verb, which indicates the action that was performed. Possible values include,
* but are not limited to, the following values: - "post" - Publish content to the stream. -
* "share" - Reshare an activity.
* @return value or {@code null} for none
*/
public java.lang.String getVerb() {
return verb;
}
/**
* This activity's verb, which indicates the action that was performed. Possible values include,
* but are not limited to, the following values: - "post" - Publish content to the stream. -
* "share" - Reshare an activity.
* @param verb verb or {@code null} for none
*/
public Activity setVerb(java.lang.String verb) {
this.verb = verb;
return this;
}
@Override
public Activity set(String fieldName, Object value) {
return (Activity) super.set(fieldName, value);
}
@Override
public Activity clone() {
return (Activity) super.clone();
}
/**
* The person who performed this activity.
*/
public static final class Actor extends com.google.api.client.json.GenericJson {
/**
* Actor info specific to particular clients.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ClientSpecificActorInfo clientSpecificActorInfo;
/**
* The name of the actor, suitable for display.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* The ID of the actor's Person resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The image representation of the actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Image image;
/**
* An object representation of the individual components of name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Name name;
/**
* The link to the actor's Google profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* Verification status of actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Verification verification;
/**
* Actor info specific to particular clients.
* @return value or {@code null} for none
*/
public ClientSpecificActorInfo getClientSpecificActorInfo() {
return clientSpecificActorInfo;
}
/**
* Actor info specific to particular clients.
* @param clientSpecificActorInfo clientSpecificActorInfo or {@code null} for none
*/
public Actor setClientSpecificActorInfo(ClientSpecificActorInfo clientSpecificActorInfo) {
this.clientSpecificActorInfo = clientSpecificActorInfo;
return this;
}
/**
* The name of the actor, suitable for display.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The name of the actor, suitable for display.
* @param displayName displayName or {@code null} for none
*/
public Actor setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* The ID of the actor's Person resource.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the actor's Person resource.
* @param id id or {@code null} for none
*/
public Actor setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The image representation of the actor.
* @return value or {@code null} for none
*/
public Image getImage() {
return image;
}
/**
* The image representation of the actor.
* @param image image or {@code null} for none
*/
public Actor setImage(Image image) {
this.image = image;
return this;
}
/**
* An object representation of the individual components of name.
* @return value or {@code null} for none
*/
public Name getName() {
return name;
}
/**
* An object representation of the individual components of name.
* @param name name or {@code null} for none
*/
public Actor setName(Name name) {
this.name = name;
return this;
}
/**
* The link to the actor's Google profile.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The link to the actor's Google profile.
* @param url url or {@code null} for none
*/
public Actor setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* Verification status of actor.
* @return value or {@code null} for none
*/
public Verification getVerification() {
return verification;
}
/**
* Verification status of actor.
* @param verification verification or {@code null} for none
*/
public Actor setVerification(Verification verification) {
this.verification = verification;
return this;
}
@Override
public Actor set(String fieldName, Object value) {
return (Actor) super.set(fieldName, value);
}
@Override
public Actor clone() {
return (Actor) super.clone();
}
/**
* Actor info specific to particular clients.
*/
public static final class ClientSpecificActorInfo extends com.google.api.client.json.GenericJson {
/**
* Actor info specific to YouTube clients.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private YoutubeActorInfo youtubeActorInfo;
/**
* Actor info specific to YouTube clients.
* @return value or {@code null} for none
*/
public YoutubeActorInfo getYoutubeActorInfo() {
return youtubeActorInfo;
}
/**
* Actor info specific to YouTube clients.
* @param youtubeActorInfo youtubeActorInfo or {@code null} for none
*/
public ClientSpecificActorInfo setYoutubeActorInfo(YoutubeActorInfo youtubeActorInfo) {
this.youtubeActorInfo = youtubeActorInfo;
return this;
}
@Override
public ClientSpecificActorInfo set(String fieldName, Object value) {
return (ClientSpecificActorInfo) super.set(fieldName, value);
}
@Override
public ClientSpecificActorInfo clone() {
return (ClientSpecificActorInfo) super.clone();
}
/**
* Actor info specific to YouTube clients.
*/
public static final class YoutubeActorInfo extends com.google.api.client.json.GenericJson {
/**
* ID of the YouTube channel owned by the Actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String channelId;
/**
* ID of the YouTube channel owned by the Actor.
* @return value or {@code null} for none
*/
public java.lang.String getChannelId() {
return channelId;
}
/**
* ID of the YouTube channel owned by the Actor.
* @param channelId channelId or {@code null} for none
*/
public YoutubeActorInfo setChannelId(java.lang.String channelId) {
this.channelId = channelId;
return this;
}
@Override
public YoutubeActorInfo set(String fieldName, Object value) {
return (YoutubeActorInfo) super.set(fieldName, value);
}
@Override
public YoutubeActorInfo clone() {
return (YoutubeActorInfo) super.clone();
}
}
}
/**
* The image representation of the actor.
*/
public static final class Image extends com.google.api.client.json.GenericJson {
/**
* The URL of the actor's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The URL of the actor's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The URL of the actor's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* @param url url or {@code null} for none
*/
public Image setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Image set(String fieldName, Object value) {
return (Image) super.set(fieldName, value);
}
@Override
public Image clone() {
return (Image) super.clone();
}
}
/**
* An object representation of the individual components of name.
*/
public static final class Name extends com.google.api.client.json.GenericJson {
/**
* The family name ("last name") of the actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String familyName;
/**
* The given name ("first name") of the actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String givenName;
/**
* The family name ("last name") of the actor.
* @return value or {@code null} for none
*/
public java.lang.String getFamilyName() {
return familyName;
}
/**
* The family name ("last name") of the actor.
* @param familyName familyName or {@code null} for none
*/
public Name setFamilyName(java.lang.String familyName) {
this.familyName = familyName;
return this;
}
/**
* The given name ("first name") of the actor.
* @return value or {@code null} for none
*/
public java.lang.String getGivenName() {
return givenName;
}
/**
* The given name ("first name") of the actor.
* @param givenName givenName or {@code null} for none
*/
public Name setGivenName(java.lang.String givenName) {
this.givenName = givenName;
return this;
}
@Override
public Name set(String fieldName, Object value) {
return (Name) super.set(fieldName, value);
}
@Override
public Name clone() {
return (Name) super.clone();
}
}
/**
* Verification status of actor.
*/
public static final class Verification extends com.google.api.client.json.GenericJson {
/**
* Verification for one-time or manual processes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adHocVerified;
/**
* Verification for one-time or manual processes.
* @return value or {@code null} for none
*/
public java.lang.String getAdHocVerified() {
return adHocVerified;
}
/**
* Verification for one-time or manual processes.
* @param adHocVerified adHocVerified or {@code null} for none
*/
public Verification setAdHocVerified(java.lang.String adHocVerified) {
this.adHocVerified = adHocVerified;
return this;
}
@Override
public Verification set(String fieldName, Object value) {
return (Verification) super.set(fieldName, value);
}
@Override
public Verification clone() {
return (Verification) super.clone();
}
}
}
/**
* The object of this activity.
*/
public static final class PlusObject extends com.google.api.client.json.GenericJson {
/**
* If this activity's object is itself another activity, such as when a person reshares an
* activity, this property specifies the original activity's actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Actor actor;
/**
* The media objects attached to this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List attachments;
static {
// hack to force ProGuard to consider Attachments used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Attachments.class);
}
/**
* The HTML-formatted content, which is suitable for display.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String content;
/**
* The ID of the object. When resharing an activity, this is the ID of the activity that is being
* reshared.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The type of the object. Possible values include, but are not limited to, the following values:
* - "note" - Textual content. - "activity" - A Google+ activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String objectType;
/**
* The content (text) as provided by the author, which is stored without any HTML formatting. When
* creating or updating an activity, this value must be supplied as plain text in the request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String originalContent;
/**
* People who +1'd this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Plusoners plusoners;
/**
* Comments in reply to this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Replies replies;
/**
* People who reshared this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Resharers resharers;
/**
* The URL that points to the linked resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* If this activity's object is itself another activity, such as when a person reshares an
* activity, this property specifies the original activity's actor.
* @return value or {@code null} for none
*/
public Actor getActor() {
return actor;
}
/**
* If this activity's object is itself another activity, such as when a person reshares an
* activity, this property specifies the original activity's actor.
* @param actor actor or {@code null} for none
*/
public PlusObject setActor(Actor actor) {
this.actor = actor;
return this;
}
/**
* The media objects attached to this activity.
* @return value or {@code null} for none
*/
public java.util.List getAttachments() {
return attachments;
}
/**
* The media objects attached to this activity.
* @param attachments attachments or {@code null} for none
*/
public PlusObject setAttachments(java.util.List attachments) {
this.attachments = attachments;
return this;
}
/**
* The HTML-formatted content, which is suitable for display.
* @return value or {@code null} for none
*/
public java.lang.String getContent() {
return content;
}
/**
* The HTML-formatted content, which is suitable for display.
* @param content content or {@code null} for none
*/
public PlusObject setContent(java.lang.String content) {
this.content = content;
return this;
}
/**
* The ID of the object. When resharing an activity, this is the ID of the activity that is being
* reshared.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the object. When resharing an activity, this is the ID of the activity that is being
* reshared.
* @param id id or {@code null} for none
*/
public PlusObject setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The type of the object. Possible values include, but are not limited to, the following values:
* - "note" - Textual content. - "activity" - A Google+ activity.
* @return value or {@code null} for none
*/
public java.lang.String getObjectType() {
return objectType;
}
/**
* The type of the object. Possible values include, but are not limited to, the following values:
* - "note" - Textual content. - "activity" - A Google+ activity.
* @param objectType objectType or {@code null} for none
*/
public PlusObject setObjectType(java.lang.String objectType) {
this.objectType = objectType;
return this;
}
/**
* The content (text) as provided by the author, which is stored without any HTML formatting. When
* creating or updating an activity, this value must be supplied as plain text in the request.
* @return value or {@code null} for none
*/
public java.lang.String getOriginalContent() {
return originalContent;
}
/**
* The content (text) as provided by the author, which is stored without any HTML formatting. When
* creating or updating an activity, this value must be supplied as plain text in the request.
* @param originalContent originalContent or {@code null} for none
*/
public PlusObject setOriginalContent(java.lang.String originalContent) {
this.originalContent = originalContent;
return this;
}
/**
* People who +1'd this activity.
* @return value or {@code null} for none
*/
public Plusoners getPlusoners() {
return plusoners;
}
/**
* People who +1'd this activity.
* @param plusoners plusoners or {@code null} for none
*/
public PlusObject setPlusoners(Plusoners plusoners) {
this.plusoners = plusoners;
return this;
}
/**
* Comments in reply to this activity.
* @return value or {@code null} for none
*/
public Replies getReplies() {
return replies;
}
/**
* Comments in reply to this activity.
* @param replies replies or {@code null} for none
*/
public PlusObject setReplies(Replies replies) {
this.replies = replies;
return this;
}
/**
* People who reshared this activity.
* @return value or {@code null} for none
*/
public Resharers getResharers() {
return resharers;
}
/**
* People who reshared this activity.
* @param resharers resharers or {@code null} for none
*/
public PlusObject setResharers(Resharers resharers) {
this.resharers = resharers;
return this;
}
/**
* The URL that points to the linked resource.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The URL that points to the linked resource.
* @param url url or {@code null} for none
*/
public PlusObject setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public PlusObject set(String fieldName, Object value) {
return (PlusObject) super.set(fieldName, value);
}
@Override
public PlusObject clone() {
return (PlusObject) super.clone();
}
/**
* If this activity's object is itself another activity, such as when a person reshares an activity,
* this property specifies the original activity's actor.
*/
public static final class Actor extends com.google.api.client.json.GenericJson {
/**
* Actor info specific to particular clients.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ClientSpecificActorInfo clientSpecificActorInfo;
/**
* The original actor's name, which is suitable for display.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* ID of the original actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The image representation of the original actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Image image;
/**
* A link to the original actor's Google profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* Verification status of actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Verification verification;
/**
* Actor info specific to particular clients.
* @return value or {@code null} for none
*/
public ClientSpecificActorInfo getClientSpecificActorInfo() {
return clientSpecificActorInfo;
}
/**
* Actor info specific to particular clients.
* @param clientSpecificActorInfo clientSpecificActorInfo or {@code null} for none
*/
public Actor setClientSpecificActorInfo(ClientSpecificActorInfo clientSpecificActorInfo) {
this.clientSpecificActorInfo = clientSpecificActorInfo;
return this;
}
/**
* The original actor's name, which is suitable for display.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The original actor's name, which is suitable for display.
* @param displayName displayName or {@code null} for none
*/
public Actor setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* ID of the original actor.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* ID of the original actor.
* @param id id or {@code null} for none
*/
public Actor setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The image representation of the original actor.
* @return value or {@code null} for none
*/
public Image getImage() {
return image;
}
/**
* The image representation of the original actor.
* @param image image or {@code null} for none
*/
public Actor setImage(Image image) {
this.image = image;
return this;
}
/**
* A link to the original actor's Google profile.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* A link to the original actor's Google profile.
* @param url url or {@code null} for none
*/
public Actor setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* Verification status of actor.
* @return value or {@code null} for none
*/
public Verification getVerification() {
return verification;
}
/**
* Verification status of actor.
* @param verification verification or {@code null} for none
*/
public Actor setVerification(Verification verification) {
this.verification = verification;
return this;
}
@Override
public Actor set(String fieldName, Object value) {
return (Actor) super.set(fieldName, value);
}
@Override
public Actor clone() {
return (Actor) super.clone();
}
/**
* Actor info specific to particular clients.
*/
public static final class ClientSpecificActorInfo extends com.google.api.client.json.GenericJson {
/**
* Actor info specific to YouTube clients.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private YoutubeActorInfo youtubeActorInfo;
/**
* Actor info specific to YouTube clients.
* @return value or {@code null} for none
*/
public YoutubeActorInfo getYoutubeActorInfo() {
return youtubeActorInfo;
}
/**
* Actor info specific to YouTube clients.
* @param youtubeActorInfo youtubeActorInfo or {@code null} for none
*/
public ClientSpecificActorInfo setYoutubeActorInfo(YoutubeActorInfo youtubeActorInfo) {
this.youtubeActorInfo = youtubeActorInfo;
return this;
}
@Override
public ClientSpecificActorInfo set(String fieldName, Object value) {
return (ClientSpecificActorInfo) super.set(fieldName, value);
}
@Override
public ClientSpecificActorInfo clone() {
return (ClientSpecificActorInfo) super.clone();
}
/**
* Actor info specific to YouTube clients.
*/
public static final class YoutubeActorInfo extends com.google.api.client.json.GenericJson {
/**
* ID of the YouTube channel owned by the Actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String channelId;
/**
* ID of the YouTube channel owned by the Actor.
* @return value or {@code null} for none
*/
public java.lang.String getChannelId() {
return channelId;
}
/**
* ID of the YouTube channel owned by the Actor.
* @param channelId channelId or {@code null} for none
*/
public YoutubeActorInfo setChannelId(java.lang.String channelId) {
this.channelId = channelId;
return this;
}
@Override
public YoutubeActorInfo set(String fieldName, Object value) {
return (YoutubeActorInfo) super.set(fieldName, value);
}
@Override
public YoutubeActorInfo clone() {
return (YoutubeActorInfo) super.clone();
}
}
}
/**
* The image representation of the original actor.
*/
public static final class Image extends com.google.api.client.json.GenericJson {
/**
* A URL that points to a thumbnail photo of the original actor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* A URL that points to a thumbnail photo of the original actor.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* A URL that points to a thumbnail photo of the original actor.
* @param url url or {@code null} for none
*/
public Image setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Image set(String fieldName, Object value) {
return (Image) super.set(fieldName, value);
}
@Override
public Image clone() {
return (Image) super.clone();
}
}
/**
* Verification status of actor.
*/
public static final class Verification extends com.google.api.client.json.GenericJson {
/**
* Verification for one-time or manual processes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adHocVerified;
/**
* Verification for one-time or manual processes.
* @return value or {@code null} for none
*/
public java.lang.String getAdHocVerified() {
return adHocVerified;
}
/**
* Verification for one-time or manual processes.
* @param adHocVerified adHocVerified or {@code null} for none
*/
public Verification setAdHocVerified(java.lang.String adHocVerified) {
this.adHocVerified = adHocVerified;
return this;
}
@Override
public Verification set(String fieldName, Object value) {
return (Verification) super.set(fieldName, value);
}
@Override
public Verification clone() {
return (Verification) super.clone();
}
}
}
/**
* Model definition for ActivityObjectAttachments.
*/
public static final class Attachments extends com.google.api.client.json.GenericJson {
/**
* If the attachment is an article, this property contains a snippet of text from the article. It
* can also include descriptions for other types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String content;
/**
* The title of the attachment, such as a photo caption or an article title.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* If the attachment is a video, the embeddable link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Embed embed;
/**
* The full image URL for photo attachments.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FullImage fullImage;
/**
* The ID of the attachment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The preview image for photos or videos.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Image image;
/**
* The type of media object. Possible values include, but are not limited to, the following
* values: - "photo" - A photo. - "album" - A photo album. - "video" - A video. - "article" -
* An article, specified by a link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String objectType;
/**
* If the attachment is an album, this property is a list of potential additional thumbnails from
* the album.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List thumbnails;
static {
// hack to force ProGuard to consider Thumbnails used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Thumbnails.class);
}
/**
* The link to the attachment, which should be of type text/html.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* If the attachment is an article, this property contains a snippet of text from the article. It
* can also include descriptions for other types.
* @return value or {@code null} for none
*/
public java.lang.String getContent() {
return content;
}
/**
* If the attachment is an article, this property contains a snippet of text from the article. It
* can also include descriptions for other types.
* @param content content or {@code null} for none
*/
public Attachments setContent(java.lang.String content) {
this.content = content;
return this;
}
/**
* The title of the attachment, such as a photo caption or an article title.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The title of the attachment, such as a photo caption or an article title.
* @param displayName displayName or {@code null} for none
*/
public Attachments setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* If the attachment is a video, the embeddable link.
* @return value or {@code null} for none
*/
public Embed getEmbed() {
return embed;
}
/**
* If the attachment is a video, the embeddable link.
* @param embed embed or {@code null} for none
*/
public Attachments setEmbed(Embed embed) {
this.embed = embed;
return this;
}
/**
* The full image URL for photo attachments.
* @return value or {@code null} for none
*/
public FullImage getFullImage() {
return fullImage;
}
/**
* The full image URL for photo attachments.
* @param fullImage fullImage or {@code null} for none
*/
public Attachments setFullImage(FullImage fullImage) {
this.fullImage = fullImage;
return this;
}
/**
* The ID of the attachment.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of the attachment.
* @param id id or {@code null} for none
*/
public Attachments setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The preview image for photos or videos.
* @return value or {@code null} for none
*/
public Image getImage() {
return image;
}
/**
* The preview image for photos or videos.
* @param image image or {@code null} for none
*/
public Attachments setImage(Image image) {
this.image = image;
return this;
}
/**
* The type of media object. Possible values include, but are not limited to, the following
* values: - "photo" - A photo. - "album" - A photo album. - "video" - A video. - "article" -
* An article, specified by a link.
* @return value or {@code null} for none
*/
public java.lang.String getObjectType() {
return objectType;
}
/**
* The type of media object. Possible values include, but are not limited to, the following
* values: - "photo" - A photo. - "album" - A photo album. - "video" - A video. - "article" -
* An article, specified by a link.
* @param objectType objectType or {@code null} for none
*/
public Attachments setObjectType(java.lang.String objectType) {
this.objectType = objectType;
return this;
}
/**
* If the attachment is an album, this property is a list of potential additional thumbnails from
* the album.
* @return value or {@code null} for none
*/
public java.util.List getThumbnails() {
return thumbnails;
}
/**
* If the attachment is an album, this property is a list of potential additional thumbnails from
* the album.
* @param thumbnails thumbnails or {@code null} for none
*/
public Attachments setThumbnails(java.util.List thumbnails) {
this.thumbnails = thumbnails;
return this;
}
/**
* The link to the attachment, which should be of type text/html.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The link to the attachment, which should be of type text/html.
* @param url url or {@code null} for none
*/
public Attachments setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Attachments set(String fieldName, Object value) {
return (Attachments) super.set(fieldName, value);
}
@Override
public Attachments clone() {
return (Attachments) super.clone();
}
/**
* If the attachment is a video, the embeddable link.
*/
public static final class Embed extends com.google.api.client.json.GenericJson {
/**
* Media type of the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* URL of the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* Media type of the link.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Media type of the link.
* @param type type or {@code null} for none
*/
public Embed setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* URL of the link.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* URL of the link.
* @param url url or {@code null} for none
*/
public Embed setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Embed set(String fieldName, Object value) {
return (Embed) super.set(fieldName, value);
}
@Override
public Embed clone() {
return (Embed) super.clone();
}
}
/**
* The full image URL for photo attachments.
*/
public static final class FullImage extends com.google.api.client.json.GenericJson {
/**
* The height, in pixels, of the linked resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long height;
/**
* Media type of the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* URL of the image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The width, in pixels, of the linked resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long width;
/**
* The height, in pixels, of the linked resource.
* @return value or {@code null} for none
*/
public java.lang.Long getHeight() {
return height;
}
/**
* The height, in pixels, of the linked resource.
* @param height height or {@code null} for none
*/
public FullImage setHeight(java.lang.Long height) {
this.height = height;
return this;
}
/**
* Media type of the link.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Media type of the link.
* @param type type or {@code null} for none
*/
public FullImage setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* URL of the image.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* URL of the image.
* @param url url or {@code null} for none
*/
public FullImage setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* The width, in pixels, of the linked resource.
* @return value or {@code null} for none
*/
public java.lang.Long getWidth() {
return width;
}
/**
* The width, in pixels, of the linked resource.
* @param width width or {@code null} for none
*/
public FullImage setWidth(java.lang.Long width) {
this.width = width;
return this;
}
@Override
public FullImage set(String fieldName, Object value) {
return (FullImage) super.set(fieldName, value);
}
@Override
public FullImage clone() {
return (FullImage) super.clone();
}
}
/**
* The preview image for photos or videos.
*/
public static final class Image extends com.google.api.client.json.GenericJson {
/**
* The height, in pixels, of the linked resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long height;
/**
* Media type of the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* Image URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The width, in pixels, of the linked resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long width;
/**
* The height, in pixels, of the linked resource.
* @return value or {@code null} for none
*/
public java.lang.Long getHeight() {
return height;
}
/**
* The height, in pixels, of the linked resource.
* @param height height or {@code null} for none
*/
public Image setHeight(java.lang.Long height) {
this.height = height;
return this;
}
/**
* Media type of the link.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Media type of the link.
* @param type type or {@code null} for none
*/
public Image setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* Image URL.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* Image URL.
* @param url url or {@code null} for none
*/
public Image setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* The width, in pixels, of the linked resource.
* @return value or {@code null} for none
*/
public java.lang.Long getWidth() {
return width;
}
/**
* The width, in pixels, of the linked resource.
* @param width width or {@code null} for none
*/
public Image setWidth(java.lang.Long width) {
this.width = width;
return this;
}
@Override
public Image set(String fieldName, Object value) {
return (Image) super.set(fieldName, value);
}
@Override
public Image clone() {
return (Image) super.clone();
}
}
/**
* Model definition for ActivityObjectAttachmentsThumbnails.
*/
public static final class Thumbnails extends com.google.api.client.json.GenericJson {
/**
* Potential name of the thumbnail.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Image resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Image image;
/**
* URL of the webpage containing the image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* Potential name of the thumbnail.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Potential name of the thumbnail.
* @param description description or {@code null} for none
*/
public Thumbnails setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Image resource.
* @return value or {@code null} for none
*/
public Image getImage() {
return image;
}
/**
* Image resource.
* @param image image or {@code null} for none
*/
public Thumbnails setImage(Image image) {
this.image = image;
return this;
}
/**
* URL of the webpage containing the image.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* URL of the webpage containing the image.
* @param url url or {@code null} for none
*/
public Thumbnails setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Thumbnails set(String fieldName, Object value) {
return (Thumbnails) super.set(fieldName, value);
}
@Override
public Thumbnails clone() {
return (Thumbnails) super.clone();
}
/**
* Image resource.
*/
public static final class Image extends com.google.api.client.json.GenericJson {
/**
* The height, in pixels, of the linked resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long height;
/**
* Media type of the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* Image url.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The width, in pixels, of the linked resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long width;
/**
* The height, in pixels, of the linked resource.
* @return value or {@code null} for none
*/
public java.lang.Long getHeight() {
return height;
}
/**
* The height, in pixels, of the linked resource.
* @param height height or {@code null} for none
*/
public Image setHeight(java.lang.Long height) {
this.height = height;
return this;
}
/**
* Media type of the link.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Media type of the link.
* @param type type or {@code null} for none
*/
public Image setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* Image url.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* Image url.
* @param url url or {@code null} for none
*/
public Image setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* The width, in pixels, of the linked resource.
* @return value or {@code null} for none
*/
public java.lang.Long getWidth() {
return width;
}
/**
* The width, in pixels, of the linked resource.
* @param width width or {@code null} for none
*/
public Image setWidth(java.lang.Long width) {
this.width = width;
return this;
}
@Override
public Image set(String fieldName, Object value) {
return (Image) super.set(fieldName, value);
}
@Override
public Image clone() {
return (Image) super.clone();
}
}
}
}
/**
* People who +1'd this activity.
*/
public static final class Plusoners extends com.google.api.client.json.GenericJson {
/**
* The URL for the collection of people who +1'd this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Total number of people who +1'd this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long totalItems;
/**
* The URL for the collection of people who +1'd this activity.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* The URL for the collection of people who +1'd this activity.
* @param selfLink selfLink or {@code null} for none
*/
public Plusoners setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* Total number of people who +1'd this activity.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalItems() {
return totalItems;
}
/**
* Total number of people who +1'd this activity.
* @param totalItems totalItems or {@code null} for none
*/
public Plusoners setTotalItems(java.lang.Long totalItems) {
this.totalItems = totalItems;
return this;
}
@Override
public Plusoners set(String fieldName, Object value) {
return (Plusoners) super.set(fieldName, value);
}
@Override
public Plusoners clone() {
return (Plusoners) super.clone();
}
}
/**
* Comments in reply to this activity.
*/
public static final class Replies extends com.google.api.client.json.GenericJson {
/**
* The URL for the collection of comments in reply to this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Total number of comments on this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long totalItems;
/**
* The URL for the collection of comments in reply to this activity.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* The URL for the collection of comments in reply to this activity.
* @param selfLink selfLink or {@code null} for none
*/
public Replies setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* Total number of comments on this activity.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalItems() {
return totalItems;
}
/**
* Total number of comments on this activity.
* @param totalItems totalItems or {@code null} for none
*/
public Replies setTotalItems(java.lang.Long totalItems) {
this.totalItems = totalItems;
return this;
}
@Override
public Replies set(String fieldName, Object value) {
return (Replies) super.set(fieldName, value);
}
@Override
public Replies clone() {
return (Replies) super.clone();
}
}
/**
* People who reshared this activity.
*/
public static final class Resharers extends com.google.api.client.json.GenericJson {
/**
* The URL for the collection of resharers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Total number of people who reshared this activity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long totalItems;
/**
* The URL for the collection of resharers.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* The URL for the collection of resharers.
* @param selfLink selfLink or {@code null} for none
*/
public Resharers setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* Total number of people who reshared this activity.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalItems() {
return totalItems;
}
/**
* Total number of people who reshared this activity.
* @param totalItems totalItems or {@code null} for none
*/
public Resharers setTotalItems(java.lang.Long totalItems) {
this.totalItems = totalItems;
return this;
}
@Override
public Resharers set(String fieldName, Object value) {
return (Resharers) super.set(fieldName, value);
}
@Override
public Resharers clone() {
return (Resharers) super.clone();
}
}
}
/**
* The service provider that initially published this activity.
*/
public static final class Provider extends com.google.api.client.json.GenericJson {
/**
* Name of the service provider.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Name of the service provider.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Name of the service provider.
* @param title title or {@code null} for none
*/
public Provider setTitle(java.lang.String title) {
this.title = title;
return this;
}
@Override
public Provider set(String fieldName, Object value) {
return (Provider) super.set(fieldName, value);
}
@Override
public Provider clone() {
return (Provider) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy