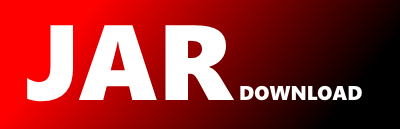
com.google.api.services.plusDomains.model.Media Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-08-12 at 04:24:37 UTC
* Modify at your own risk.
*/
package com.google.api.services.plusDomains.model;
/**
* Model definition for Media.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google+ Domains API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Media extends com.google.api.client.json.GenericJson {
/**
* The person who uploaded this media.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Author author;
/**
* The display name for this media.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* ETag of this response for caching purposes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* Exif information of the media item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Exif exif;
/**
* The height in pixels of the original image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long height;
/**
* ID of this media, which is generated by the API.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The type of resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The time at which this media was originally created in UTC. Formatted as an RFC 3339 timestamp
* that matches this example: 2010-11-25T14:30:27.655Z
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime mediaCreatedTime;
/**
* The URL of this photo or video's still image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mediaUrl;
/**
* The time at which this media was uploaded. Formatted as an RFC 3339 timestamp.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime published;
/**
* The size in bytes of this video.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long sizeBytes;
/**
* The list of video streams for this video. There might be several different streams available
* for a single video, either Flash or MPEG, of various sizes
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List streams;
/**
* A description, or caption, for this media.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String summary;
/**
* The time at which this media was last updated. This includes changes to media metadata.
* Formatted as an RFC 3339 timestamp.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime updated;
/**
* The URL for the page that hosts this media.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The duration in milliseconds of this video.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long videoDuration;
/**
* The encoding status of this video. Possible values are: - "UPLOADING" - Not all the video bytes
* have been received. - "PENDING" - Video not yet processed. - "FAILED" - Video processing
* failed. - "READY" - A single video stream is playable. - "FINAL" - All video streams are
* playable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String videoStatus;
/**
* The width in pixels of the original image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long width;
/**
* The person who uploaded this media.
* @return value or {@code null} for none
*/
public Author getAuthor() {
return author;
}
/**
* The person who uploaded this media.
* @param author author or {@code null} for none
*/
public Media setAuthor(Author author) {
this.author = author;
return this;
}
/**
* The display name for this media.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The display name for this media.
* @param displayName displayName or {@code null} for none
*/
public Media setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* ETag of this response for caching purposes.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* ETag of this response for caching purposes.
* @param etag etag or {@code null} for none
*/
public Media setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Exif information of the media item.
* @return value or {@code null} for none
*/
public Exif getExif() {
return exif;
}
/**
* Exif information of the media item.
* @param exif exif or {@code null} for none
*/
public Media setExif(Exif exif) {
this.exif = exif;
return this;
}
/**
* The height in pixels of the original image.
* @return value or {@code null} for none
*/
public java.lang.Long getHeight() {
return height;
}
/**
* The height in pixels of the original image.
* @param height height or {@code null} for none
*/
public Media setHeight(java.lang.Long height) {
this.height = height;
return this;
}
/**
* ID of this media, which is generated by the API.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* ID of this media, which is generated by the API.
* @param id id or {@code null} for none
*/
public Media setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The type of resource.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* The type of resource.
* @param kind kind or {@code null} for none
*/
public Media setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The time at which this media was originally created in UTC. Formatted as an RFC 3339 timestamp
* that matches this example: 2010-11-25T14:30:27.655Z
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getMediaCreatedTime() {
return mediaCreatedTime;
}
/**
* The time at which this media was originally created in UTC. Formatted as an RFC 3339 timestamp
* that matches this example: 2010-11-25T14:30:27.655Z
* @param mediaCreatedTime mediaCreatedTime or {@code null} for none
*/
public Media setMediaCreatedTime(com.google.api.client.util.DateTime mediaCreatedTime) {
this.mediaCreatedTime = mediaCreatedTime;
return this;
}
/**
* The URL of this photo or video's still image.
* @return value or {@code null} for none
*/
public java.lang.String getMediaUrl() {
return mediaUrl;
}
/**
* The URL of this photo or video's still image.
* @param mediaUrl mediaUrl or {@code null} for none
*/
public Media setMediaUrl(java.lang.String mediaUrl) {
this.mediaUrl = mediaUrl;
return this;
}
/**
* The time at which this media was uploaded. Formatted as an RFC 3339 timestamp.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getPublished() {
return published;
}
/**
* The time at which this media was uploaded. Formatted as an RFC 3339 timestamp.
* @param published published or {@code null} for none
*/
public Media setPublished(com.google.api.client.util.DateTime published) {
this.published = published;
return this;
}
/**
* The size in bytes of this video.
* @return value or {@code null} for none
*/
public java.lang.Long getSizeBytes() {
return sizeBytes;
}
/**
* The size in bytes of this video.
* @param sizeBytes sizeBytes or {@code null} for none
*/
public Media setSizeBytes(java.lang.Long sizeBytes) {
this.sizeBytes = sizeBytes;
return this;
}
/**
* The list of video streams for this video. There might be several different streams available
* for a single video, either Flash or MPEG, of various sizes
* @return value or {@code null} for none
*/
public java.util.List getStreams() {
return streams;
}
/**
* The list of video streams for this video. There might be several different streams available
* for a single video, either Flash or MPEG, of various sizes
* @param streams streams or {@code null} for none
*/
public Media setStreams(java.util.List streams) {
this.streams = streams;
return this;
}
/**
* A description, or caption, for this media.
* @return value or {@code null} for none
*/
public java.lang.String getSummary() {
return summary;
}
/**
* A description, or caption, for this media.
* @param summary summary or {@code null} for none
*/
public Media setSummary(java.lang.String summary) {
this.summary = summary;
return this;
}
/**
* The time at which this media was last updated. This includes changes to media metadata.
* Formatted as an RFC 3339 timestamp.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getUpdated() {
return updated;
}
/**
* The time at which this media was last updated. This includes changes to media metadata.
* Formatted as an RFC 3339 timestamp.
* @param updated updated or {@code null} for none
*/
public Media setUpdated(com.google.api.client.util.DateTime updated) {
this.updated = updated;
return this;
}
/**
* The URL for the page that hosts this media.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The URL for the page that hosts this media.
* @param url url or {@code null} for none
*/
public Media setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* The duration in milliseconds of this video.
* @return value or {@code null} for none
*/
public java.lang.Long getVideoDuration() {
return videoDuration;
}
/**
* The duration in milliseconds of this video.
* @param videoDuration videoDuration or {@code null} for none
*/
public Media setVideoDuration(java.lang.Long videoDuration) {
this.videoDuration = videoDuration;
return this;
}
/**
* The encoding status of this video. Possible values are: - "UPLOADING" - Not all the video bytes
* have been received. - "PENDING" - Video not yet processed. - "FAILED" - Video processing
* failed. - "READY" - A single video stream is playable. - "FINAL" - All video streams are
* playable.
* @return value or {@code null} for none
*/
public java.lang.String getVideoStatus() {
return videoStatus;
}
/**
* The encoding status of this video. Possible values are: - "UPLOADING" - Not all the video bytes
* have been received. - "PENDING" - Video not yet processed. - "FAILED" - Video processing
* failed. - "READY" - A single video stream is playable. - "FINAL" - All video streams are
* playable.
* @param videoStatus videoStatus or {@code null} for none
*/
public Media setVideoStatus(java.lang.String videoStatus) {
this.videoStatus = videoStatus;
return this;
}
/**
* The width in pixels of the original image.
* @return value or {@code null} for none
*/
public java.lang.Long getWidth() {
return width;
}
/**
* The width in pixels of the original image.
* @param width width or {@code null} for none
*/
public Media setWidth(java.lang.Long width) {
this.width = width;
return this;
}
@Override
public Media set(String fieldName, Object value) {
return (Media) super.set(fieldName, value);
}
@Override
public Media clone() {
return (Media) super.clone();
}
/**
* The person who uploaded this media.
*/
public static final class Author extends com.google.api.client.json.GenericJson {
/**
* The author's name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* ID of the author.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The author's Google profile image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Image image;
/**
* A link to the author's Google profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The author's name.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The author's name.
* @param displayName displayName or {@code null} for none
*/
public Author setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* ID of the author.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* ID of the author.
* @param id id or {@code null} for none
*/
public Author setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The author's Google profile image.
* @return value or {@code null} for none
*/
public Image getImage() {
return image;
}
/**
* The author's Google profile image.
* @param image image or {@code null} for none
*/
public Author setImage(Image image) {
this.image = image;
return this;
}
/**
* A link to the author's Google profile.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* A link to the author's Google profile.
* @param url url or {@code null} for none
*/
public Author setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Author set(String fieldName, Object value) {
return (Author) super.set(fieldName, value);
}
@Override
public Author clone() {
return (Author) super.clone();
}
/**
* The author's Google profile image.
*/
public static final class Image extends com.google.api.client.json.GenericJson {
/**
* The URL of the author's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The URL of the author's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The URL of the author's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* @param url url or {@code null} for none
*/
public Image setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Image set(String fieldName, Object value) {
return (Image) super.set(fieldName, value);
}
@Override
public Image clone() {
return (Image) super.clone();
}
}
}
/**
* Exif information of the media item.
*/
public static final class Exif extends com.google.api.client.json.GenericJson {
/**
* The time the media was captured. Formatted as an RFC 3339 timestamp.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime time;
/**
* The time the media was captured. Formatted as an RFC 3339 timestamp.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getTime() {
return time;
}
/**
* The time the media was captured. Formatted as an RFC 3339 timestamp.
* @param time time or {@code null} for none
*/
public Exif setTime(com.google.api.client.util.DateTime time) {
this.time = time;
return this;
}
@Override
public Exif set(String fieldName, Object value) {
return (Exif) super.set(fieldName, value);
}
@Override
public Exif clone() {
return (Exif) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy