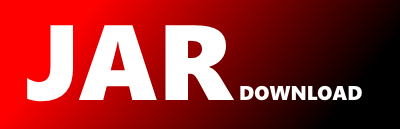
com.google.api.services.plusDomains.model.Person Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-08-12 at 04:24:37 UTC
* Modify at your own risk.
*/
package com.google.api.services.plusDomains.model;
/**
* Model definition for Person.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google+ Domains API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Person extends com.google.api.client.json.GenericJson {
/**
* A short biography for this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String aboutMe;
/**
* The person's date of birth, represented as YYYY-MM-DD.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String birthday;
/**
* The "bragging rights" line of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String braggingRights;
/**
* For followers who are visible, the number of people who have added this person or page to a
* circle.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer circledByCount;
/**
* The cover photo content.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Cover cover;
/**
* (this field is not currently used)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String currentLocation;
/**
* The name of this person, which is suitable for display.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* The hosted domain name for the user's Google Apps account. For instance, example.com. The
* plus.profile.emails.read or email scope is needed to get this domain name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String domain;
/**
* A list of email addresses that this person has, including their Google account email address,
* and the public verified email addresses on their Google+ profile. The plus.profile.emails.read
* scope is needed to retrieve these email addresses, or the email scope can be used to retrieve
* just the Google account email address.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List emails;
static {
// hack to force ProGuard to consider Emails used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Emails.class);
}
/**
* ETag of this response for caching purposes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* The person's gender. Possible values include, but are not limited to, the following values: -
* "male" - Male gender. - "female" - Female gender. - "other" - Other.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gender;
/**
* The ID of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The representation of the person's profile photo.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Image image;
/**
* Whether this user has signed up for Google+.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isPlusUser;
/**
* Identifies this resource as a person. Value: "plus#person".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* An object representation of the individual components of a person's name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Name name;
/**
* The nickname of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String nickname;
/**
* Type of person within Google+. Possible values include, but are not limited to, the following
* values: - "person" - represents an actual person. - "page" - represents a page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String objectType;
/**
* The occupation of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String occupation;
/**
* A list of current or past organizations with which this person is associated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List organizations;
static {
// hack to force ProGuard to consider Organizations used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Organizations.class);
}
/**
* A list of places where this person has lived.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List placesLived;
static {
// hack to force ProGuard to consider PlacesLived used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(PlacesLived.class);
}
/**
* If a Google+ Page, the number of people who have +1'd this page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer plusOneCount;
/**
* The person's relationship status. Possible values include, but are not limited to, the
* following values: - "single" - Person is single. - "in_a_relationship" - Person is in a
* relationship. - "engaged" - Person is engaged. - "married" - Person is married. -
* "its_complicated" - The relationship is complicated. - "open_relationship" - Person is in an
* open relationship. - "widowed" - Person is widowed. - "in_domestic_partnership" - Person is
* in a domestic partnership. - "in_civil_union" - Person is in a civil union.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String relationshipStatus;
/**
* The person's skills.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String skills;
/**
* The brief description (tagline) of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String tagline;
/**
* The URL of this person's profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* A list of URLs for this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List urls;
static {
// hack to force ProGuard to consider Urls used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Urls.class);
}
/**
* Whether the person or Google+ Page has been verified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean verified;
/**
* A short biography for this person.
* @return value or {@code null} for none
*/
public java.lang.String getAboutMe() {
return aboutMe;
}
/**
* A short biography for this person.
* @param aboutMe aboutMe or {@code null} for none
*/
public Person setAboutMe(java.lang.String aboutMe) {
this.aboutMe = aboutMe;
return this;
}
/**
* The person's date of birth, represented as YYYY-MM-DD.
* @return value or {@code null} for none
*/
public java.lang.String getBirthday() {
return birthday;
}
/**
* The person's date of birth, represented as YYYY-MM-DD.
* @param birthday birthday or {@code null} for none
*/
public Person setBirthday(java.lang.String birthday) {
this.birthday = birthday;
return this;
}
/**
* The "bragging rights" line of this person.
* @return value or {@code null} for none
*/
public java.lang.String getBraggingRights() {
return braggingRights;
}
/**
* The "bragging rights" line of this person.
* @param braggingRights braggingRights or {@code null} for none
*/
public Person setBraggingRights(java.lang.String braggingRights) {
this.braggingRights = braggingRights;
return this;
}
/**
* For followers who are visible, the number of people who have added this person or page to a
* circle.
* @return value or {@code null} for none
*/
public java.lang.Integer getCircledByCount() {
return circledByCount;
}
/**
* For followers who are visible, the number of people who have added this person or page to a
* circle.
* @param circledByCount circledByCount or {@code null} for none
*/
public Person setCircledByCount(java.lang.Integer circledByCount) {
this.circledByCount = circledByCount;
return this;
}
/**
* The cover photo content.
* @return value or {@code null} for none
*/
public Cover getCover() {
return cover;
}
/**
* The cover photo content.
* @param cover cover or {@code null} for none
*/
public Person setCover(Cover cover) {
this.cover = cover;
return this;
}
/**
* (this field is not currently used)
* @return value or {@code null} for none
*/
public java.lang.String getCurrentLocation() {
return currentLocation;
}
/**
* (this field is not currently used)
* @param currentLocation currentLocation or {@code null} for none
*/
public Person setCurrentLocation(java.lang.String currentLocation) {
this.currentLocation = currentLocation;
return this;
}
/**
* The name of this person, which is suitable for display.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The name of this person, which is suitable for display.
* @param displayName displayName or {@code null} for none
*/
public Person setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* The hosted domain name for the user's Google Apps account. For instance, example.com. The
* plus.profile.emails.read or email scope is needed to get this domain name.
* @return value or {@code null} for none
*/
public java.lang.String getDomain() {
return domain;
}
/**
* The hosted domain name for the user's Google Apps account. For instance, example.com. The
* plus.profile.emails.read or email scope is needed to get this domain name.
* @param domain domain or {@code null} for none
*/
public Person setDomain(java.lang.String domain) {
this.domain = domain;
return this;
}
/**
* A list of email addresses that this person has, including their Google account email address,
* and the public verified email addresses on their Google+ profile. The plus.profile.emails.read
* scope is needed to retrieve these email addresses, or the email scope can be used to retrieve
* just the Google account email address.
* @return value or {@code null} for none
*/
public java.util.List getEmails() {
return emails;
}
/**
* A list of email addresses that this person has, including their Google account email address,
* and the public verified email addresses on their Google+ profile. The plus.profile.emails.read
* scope is needed to retrieve these email addresses, or the email scope can be used to retrieve
* just the Google account email address.
* @param emails emails or {@code null} for none
*/
public Person setEmails(java.util.List emails) {
this.emails = emails;
return this;
}
/**
* ETag of this response for caching purposes.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* ETag of this response for caching purposes.
* @param etag etag or {@code null} for none
*/
public Person setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* The person's gender. Possible values include, but are not limited to, the following values: -
* "male" - Male gender. - "female" - Female gender. - "other" - Other.
* @return value or {@code null} for none
*/
public java.lang.String getGender() {
return gender;
}
/**
* The person's gender. Possible values include, but are not limited to, the following values: -
* "male" - Male gender. - "female" - Female gender. - "other" - Other.
* @param gender gender or {@code null} for none
*/
public Person setGender(java.lang.String gender) {
this.gender = gender;
return this;
}
/**
* The ID of this person.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The ID of this person.
* @param id id or {@code null} for none
*/
public Person setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The representation of the person's profile photo.
* @return value or {@code null} for none
*/
public Image getImage() {
return image;
}
/**
* The representation of the person's profile photo.
* @param image image or {@code null} for none
*/
public Person setImage(Image image) {
this.image = image;
return this;
}
/**
* Whether this user has signed up for Google+.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsPlusUser() {
return isPlusUser;
}
/**
* Whether this user has signed up for Google+.
* @param isPlusUser isPlusUser or {@code null} for none
*/
public Person setIsPlusUser(java.lang.Boolean isPlusUser) {
this.isPlusUser = isPlusUser;
return this;
}
/**
* Identifies this resource as a person. Value: "plus#person".
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies this resource as a person. Value: "plus#person".
* @param kind kind or {@code null} for none
*/
public Person setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* An object representation of the individual components of a person's name.
* @return value or {@code null} for none
*/
public Name getName() {
return name;
}
/**
* An object representation of the individual components of a person's name.
* @param name name or {@code null} for none
*/
public Person setName(Name name) {
this.name = name;
return this;
}
/**
* The nickname of this person.
* @return value or {@code null} for none
*/
public java.lang.String getNickname() {
return nickname;
}
/**
* The nickname of this person.
* @param nickname nickname or {@code null} for none
*/
public Person setNickname(java.lang.String nickname) {
this.nickname = nickname;
return this;
}
/**
* Type of person within Google+. Possible values include, but are not limited to, the following
* values: - "person" - represents an actual person. - "page" - represents a page.
* @return value or {@code null} for none
*/
public java.lang.String getObjectType() {
return objectType;
}
/**
* Type of person within Google+. Possible values include, but are not limited to, the following
* values: - "person" - represents an actual person. - "page" - represents a page.
* @param objectType objectType or {@code null} for none
*/
public Person setObjectType(java.lang.String objectType) {
this.objectType = objectType;
return this;
}
/**
* The occupation of this person.
* @return value or {@code null} for none
*/
public java.lang.String getOccupation() {
return occupation;
}
/**
* The occupation of this person.
* @param occupation occupation or {@code null} for none
*/
public Person setOccupation(java.lang.String occupation) {
this.occupation = occupation;
return this;
}
/**
* A list of current or past organizations with which this person is associated.
* @return value or {@code null} for none
*/
public java.util.List getOrganizations() {
return organizations;
}
/**
* A list of current or past organizations with which this person is associated.
* @param organizations organizations or {@code null} for none
*/
public Person setOrganizations(java.util.List organizations) {
this.organizations = organizations;
return this;
}
/**
* A list of places where this person has lived.
* @return value or {@code null} for none
*/
public java.util.List getPlacesLived() {
return placesLived;
}
/**
* A list of places where this person has lived.
* @param placesLived placesLived or {@code null} for none
*/
public Person setPlacesLived(java.util.List placesLived) {
this.placesLived = placesLived;
return this;
}
/**
* If a Google+ Page, the number of people who have +1'd this page.
* @return value or {@code null} for none
*/
public java.lang.Integer getPlusOneCount() {
return plusOneCount;
}
/**
* If a Google+ Page, the number of people who have +1'd this page.
* @param plusOneCount plusOneCount or {@code null} for none
*/
public Person setPlusOneCount(java.lang.Integer plusOneCount) {
this.plusOneCount = plusOneCount;
return this;
}
/**
* The person's relationship status. Possible values include, but are not limited to, the
* following values: - "single" - Person is single. - "in_a_relationship" - Person is in a
* relationship. - "engaged" - Person is engaged. - "married" - Person is married. -
* "its_complicated" - The relationship is complicated. - "open_relationship" - Person is in an
* open relationship. - "widowed" - Person is widowed. - "in_domestic_partnership" - Person is
* in a domestic partnership. - "in_civil_union" - Person is in a civil union.
* @return value or {@code null} for none
*/
public java.lang.String getRelationshipStatus() {
return relationshipStatus;
}
/**
* The person's relationship status. Possible values include, but are not limited to, the
* following values: - "single" - Person is single. - "in_a_relationship" - Person is in a
* relationship. - "engaged" - Person is engaged. - "married" - Person is married. -
* "its_complicated" - The relationship is complicated. - "open_relationship" - Person is in an
* open relationship. - "widowed" - Person is widowed. - "in_domestic_partnership" - Person is
* in a domestic partnership. - "in_civil_union" - Person is in a civil union.
* @param relationshipStatus relationshipStatus or {@code null} for none
*/
public Person setRelationshipStatus(java.lang.String relationshipStatus) {
this.relationshipStatus = relationshipStatus;
return this;
}
/**
* The person's skills.
* @return value or {@code null} for none
*/
public java.lang.String getSkills() {
return skills;
}
/**
* The person's skills.
* @param skills skills or {@code null} for none
*/
public Person setSkills(java.lang.String skills) {
this.skills = skills;
return this;
}
/**
* The brief description (tagline) of this person.
* @return value or {@code null} for none
*/
public java.lang.String getTagline() {
return tagline;
}
/**
* The brief description (tagline) of this person.
* @param tagline tagline or {@code null} for none
*/
public Person setTagline(java.lang.String tagline) {
this.tagline = tagline;
return this;
}
/**
* The URL of this person's profile.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The URL of this person's profile.
* @param url url or {@code null} for none
*/
public Person setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* A list of URLs for this person.
* @return value or {@code null} for none
*/
public java.util.List getUrls() {
return urls;
}
/**
* A list of URLs for this person.
* @param urls urls or {@code null} for none
*/
public Person setUrls(java.util.List urls) {
this.urls = urls;
return this;
}
/**
* Whether the person or Google+ Page has been verified.
* @return value or {@code null} for none
*/
public java.lang.Boolean getVerified() {
return verified;
}
/**
* Whether the person or Google+ Page has been verified.
* @param verified verified or {@code null} for none
*/
public Person setVerified(java.lang.Boolean verified) {
this.verified = verified;
return this;
}
@Override
public Person set(String fieldName, Object value) {
return (Person) super.set(fieldName, value);
}
@Override
public Person clone() {
return (Person) super.clone();
}
/**
* The cover photo content.
*/
public static final class Cover extends com.google.api.client.json.GenericJson {
/**
* Extra information about the cover photo.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CoverInfo coverInfo;
/**
* The person's primary cover image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CoverPhoto coverPhoto;
/**
* The layout of the cover art. Possible values include, but are not limited to, the following
* values: - "banner" - One large image banner.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String layout;
/**
* Extra information about the cover photo.
* @return value or {@code null} for none
*/
public CoverInfo getCoverInfo() {
return coverInfo;
}
/**
* Extra information about the cover photo.
* @param coverInfo coverInfo or {@code null} for none
*/
public Cover setCoverInfo(CoverInfo coverInfo) {
this.coverInfo = coverInfo;
return this;
}
/**
* The person's primary cover image.
* @return value or {@code null} for none
*/
public CoverPhoto getCoverPhoto() {
return coverPhoto;
}
/**
* The person's primary cover image.
* @param coverPhoto coverPhoto or {@code null} for none
*/
public Cover setCoverPhoto(CoverPhoto coverPhoto) {
this.coverPhoto = coverPhoto;
return this;
}
/**
* The layout of the cover art. Possible values include, but are not limited to, the following
* values: - "banner" - One large image banner.
* @return value or {@code null} for none
*/
public java.lang.String getLayout() {
return layout;
}
/**
* The layout of the cover art. Possible values include, but are not limited to, the following
* values: - "banner" - One large image banner.
* @param layout layout or {@code null} for none
*/
public Cover setLayout(java.lang.String layout) {
this.layout = layout;
return this;
}
@Override
public Cover set(String fieldName, Object value) {
return (Cover) super.set(fieldName, value);
}
@Override
public Cover clone() {
return (Cover) super.clone();
}
/**
* Extra information about the cover photo.
*/
public static final class CoverInfo extends com.google.api.client.json.GenericJson {
/**
* The difference between the left position of the cover image and the actual displayed cover
* image. Only valid for banner layout.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer leftImageOffset;
/**
* The difference between the top position of the cover image and the actual displayed cover
* image. Only valid for banner layout.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer topImageOffset;
/**
* The difference between the left position of the cover image and the actual displayed cover
* image. Only valid for banner layout.
* @return value or {@code null} for none
*/
public java.lang.Integer getLeftImageOffset() {
return leftImageOffset;
}
/**
* The difference between the left position of the cover image and the actual displayed cover
* image. Only valid for banner layout.
* @param leftImageOffset leftImageOffset or {@code null} for none
*/
public CoverInfo setLeftImageOffset(java.lang.Integer leftImageOffset) {
this.leftImageOffset = leftImageOffset;
return this;
}
/**
* The difference between the top position of the cover image and the actual displayed cover
* image. Only valid for banner layout.
* @return value or {@code null} for none
*/
public java.lang.Integer getTopImageOffset() {
return topImageOffset;
}
/**
* The difference between the top position of the cover image and the actual displayed cover
* image. Only valid for banner layout.
* @param topImageOffset topImageOffset or {@code null} for none
*/
public CoverInfo setTopImageOffset(java.lang.Integer topImageOffset) {
this.topImageOffset = topImageOffset;
return this;
}
@Override
public CoverInfo set(String fieldName, Object value) {
return (CoverInfo) super.set(fieldName, value);
}
@Override
public CoverInfo clone() {
return (CoverInfo) super.clone();
}
}
/**
* The person's primary cover image.
*/
public static final class CoverPhoto extends com.google.api.client.json.GenericJson {
/**
* The height of the image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer height;
/**
* The URL of the image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* The width of the image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer width;
/**
* The height of the image.
* @return value or {@code null} for none
*/
public java.lang.Integer getHeight() {
return height;
}
/**
* The height of the image.
* @param height height or {@code null} for none
*/
public CoverPhoto setHeight(java.lang.Integer height) {
this.height = height;
return this;
}
/**
* The URL of the image.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The URL of the image.
* @param url url or {@code null} for none
*/
public CoverPhoto setUrl(java.lang.String url) {
this.url = url;
return this;
}
/**
* The width of the image.
* @return value or {@code null} for none
*/
public java.lang.Integer getWidth() {
return width;
}
/**
* The width of the image.
* @param width width or {@code null} for none
*/
public CoverPhoto setWidth(java.lang.Integer width) {
this.width = width;
return this;
}
@Override
public CoverPhoto set(String fieldName, Object value) {
return (CoverPhoto) super.set(fieldName, value);
}
@Override
public CoverPhoto clone() {
return (CoverPhoto) super.clone();
}
}
}
/**
* Model definition for PersonEmails.
*/
public static final class Emails extends com.google.api.client.json.GenericJson {
/**
* The type of address. Possible values include, but are not limited to, the following values: -
* "account" - Google account email address. - "home" - Home email address. - "work" - Work
* email address. - "other" - Other.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The email address.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* The type of address. Possible values include, but are not limited to, the following values: -
* "account" - Google account email address. - "home" - Home email address. - "work" - Work
* email address. - "other" - Other.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The type of address. Possible values include, but are not limited to, the following values: -
* "account" - Google account email address. - "home" - Home email address. - "work" - Work
* email address. - "other" - Other.
* @param type type or {@code null} for none
*/
public Emails setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* The email address.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* The email address.
* @param value value or {@code null} for none
*/
public Emails setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public Emails set(String fieldName, Object value) {
return (Emails) super.set(fieldName, value);
}
@Override
public Emails clone() {
return (Emails) super.clone();
}
}
/**
* The representation of the person's profile photo.
*/
public static final class Image extends com.google.api.client.json.GenericJson {
/**
* Whether the person's profile photo is the default one
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isDefault;
/**
* The URL of the person's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* Whether the person's profile photo is the default one
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsDefault() {
return isDefault;
}
/**
* Whether the person's profile photo is the default one
* @param isDefault isDefault or {@code null} for none
*/
public Image setIsDefault(java.lang.Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
/**
* The URL of the person's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* The URL of the person's profile photo. To resize the image and crop it to a square, append the
* query string ?sz=x, where x is the dimension in pixels of each side.
* @param url url or {@code null} for none
*/
public Image setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public Image set(String fieldName, Object value) {
return (Image) super.set(fieldName, value);
}
@Override
public Image clone() {
return (Image) super.clone();
}
}
/**
* An object representation of the individual components of a person's name.
*/
public static final class Name extends com.google.api.client.json.GenericJson {
/**
* The family name (last name) of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String familyName;
/**
* The full name of this person, including middle names, suffixes, etc.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String formatted;
/**
* The given name (first name) of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String givenName;
/**
* The honorific prefixes (such as "Dr." or "Mrs.") for this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String honorificPrefix;
/**
* The honorific suffixes (such as "Jr.") for this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String honorificSuffix;
/**
* The middle name of this person.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String middleName;
/**
* The family name (last name) of this person.
* @return value or {@code null} for none
*/
public java.lang.String getFamilyName() {
return familyName;
}
/**
* The family name (last name) of this person.
* @param familyName familyName or {@code null} for none
*/
public Name setFamilyName(java.lang.String familyName) {
this.familyName = familyName;
return this;
}
/**
* The full name of this person, including middle names, suffixes, etc.
* @return value or {@code null} for none
*/
public java.lang.String getFormatted() {
return formatted;
}
/**
* The full name of this person, including middle names, suffixes, etc.
* @param formatted formatted or {@code null} for none
*/
public Name setFormatted(java.lang.String formatted) {
this.formatted = formatted;
return this;
}
/**
* The given name (first name) of this person.
* @return value or {@code null} for none
*/
public java.lang.String getGivenName() {
return givenName;
}
/**
* The given name (first name) of this person.
* @param givenName givenName or {@code null} for none
*/
public Name setGivenName(java.lang.String givenName) {
this.givenName = givenName;
return this;
}
/**
* The honorific prefixes (such as "Dr." or "Mrs.") for this person.
* @return value or {@code null} for none
*/
public java.lang.String getHonorificPrefix() {
return honorificPrefix;
}
/**
* The honorific prefixes (such as "Dr." or "Mrs.") for this person.
* @param honorificPrefix honorificPrefix or {@code null} for none
*/
public Name setHonorificPrefix(java.lang.String honorificPrefix) {
this.honorificPrefix = honorificPrefix;
return this;
}
/**
* The honorific suffixes (such as "Jr.") for this person.
* @return value or {@code null} for none
*/
public java.lang.String getHonorificSuffix() {
return honorificSuffix;
}
/**
* The honorific suffixes (such as "Jr.") for this person.
* @param honorificSuffix honorificSuffix or {@code null} for none
*/
public Name setHonorificSuffix(java.lang.String honorificSuffix) {
this.honorificSuffix = honorificSuffix;
return this;
}
/**
* The middle name of this person.
* @return value or {@code null} for none
*/
public java.lang.String getMiddleName() {
return middleName;
}
/**
* The middle name of this person.
* @param middleName middleName or {@code null} for none
*/
public Name setMiddleName(java.lang.String middleName) {
this.middleName = middleName;
return this;
}
@Override
public Name set(String fieldName, Object value) {
return (Name) super.set(fieldName, value);
}
@Override
public Name clone() {
return (Name) super.clone();
}
}
/**
* Model definition for PersonOrganizations.
*/
public static final class Organizations extends com.google.api.client.json.GenericJson {
/**
* The department within the organization. Deprecated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String department;
/**
* A short description of the person's role in this organization. Deprecated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The date that the person left this organization.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String endDate;
/**
* The location of this organization. Deprecated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String location;
/**
* The name of the organization.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* If "true", indicates this organization is the person's primary one, which is typically
* interpreted as the current one.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean primary;
/**
* The date that the person joined this organization.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String startDate;
/**
* The person's job title or role within the organization.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The type of organization. Possible values include, but are not limited to, the following
* values: - "work" - Work. - "school" - School.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The department within the organization. Deprecated.
* @return value or {@code null} for none
*/
public java.lang.String getDepartment() {
return department;
}
/**
* The department within the organization. Deprecated.
* @param department department or {@code null} for none
*/
public Organizations setDepartment(java.lang.String department) {
this.department = department;
return this;
}
/**
* A short description of the person's role in this organization. Deprecated.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* A short description of the person's role in this organization. Deprecated.
* @param description description or {@code null} for none
*/
public Organizations setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The date that the person left this organization.
* @return value or {@code null} for none
*/
public java.lang.String getEndDate() {
return endDate;
}
/**
* The date that the person left this organization.
* @param endDate endDate or {@code null} for none
*/
public Organizations setEndDate(java.lang.String endDate) {
this.endDate = endDate;
return this;
}
/**
* The location of this organization. Deprecated.
* @return value or {@code null} for none
*/
public java.lang.String getLocation() {
return location;
}
/**
* The location of this organization. Deprecated.
* @param location location or {@code null} for none
*/
public Organizations setLocation(java.lang.String location) {
this.location = location;
return this;
}
/**
* The name of the organization.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the organization.
* @param name name or {@code null} for none
*/
public Organizations setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* If "true", indicates this organization is the person's primary one, which is typically
* interpreted as the current one.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPrimary() {
return primary;
}
/**
* If "true", indicates this organization is the person's primary one, which is typically
* interpreted as the current one.
* @param primary primary or {@code null} for none
*/
public Organizations setPrimary(java.lang.Boolean primary) {
this.primary = primary;
return this;
}
/**
* The date that the person joined this organization.
* @return value or {@code null} for none
*/
public java.lang.String getStartDate() {
return startDate;
}
/**
* The date that the person joined this organization.
* @param startDate startDate or {@code null} for none
*/
public Organizations setStartDate(java.lang.String startDate) {
this.startDate = startDate;
return this;
}
/**
* The person's job title or role within the organization.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* The person's job title or role within the organization.
* @param title title or {@code null} for none
*/
public Organizations setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* The type of organization. Possible values include, but are not limited to, the following
* values: - "work" - Work. - "school" - School.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The type of organization. Possible values include, but are not limited to, the following
* values: - "work" - Work. - "school" - School.
* @param type type or {@code null} for none
*/
public Organizations setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public Organizations set(String fieldName, Object value) {
return (Organizations) super.set(fieldName, value);
}
@Override
public Organizations clone() {
return (Organizations) super.clone();
}
}
/**
* Model definition for PersonPlacesLived.
*/
public static final class PlacesLived extends com.google.api.client.json.GenericJson {
/**
* If "true", this place of residence is this person's primary residence.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean primary;
/**
* A place where this person has lived. For example: "Seattle, WA", "Near Toronto".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* If "true", this place of residence is this person's primary residence.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPrimary() {
return primary;
}
/**
* If "true", this place of residence is this person's primary residence.
* @param primary primary or {@code null} for none
*/
public PlacesLived setPrimary(java.lang.Boolean primary) {
this.primary = primary;
return this;
}
/**
* A place where this person has lived. For example: "Seattle, WA", "Near Toronto".
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* A place where this person has lived. For example: "Seattle, WA", "Near Toronto".
* @param value value or {@code null} for none
*/
public PlacesLived setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public PlacesLived set(String fieldName, Object value) {
return (PlacesLived) super.set(fieldName, value);
}
@Override
public PlacesLived clone() {
return (PlacesLived) super.clone();
}
}
/**
* Model definition for PersonUrls.
*/
public static final class Urls extends com.google.api.client.json.GenericJson {
/**
* The label of the URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String label;
/**
* The type of URL. Possible values include, but are not limited to, the following values: -
* "otherProfile" - URL for another profile. - "contributor" - URL to a site for which this
* person is a contributor. - "website" - URL for this Google+ Page's primary website. - "other"
* - Other URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The URL value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* The label of the URL.
* @return value or {@code null} for none
*/
public java.lang.String getLabel() {
return label;
}
/**
* The label of the URL.
* @param label label or {@code null} for none
*/
public Urls setLabel(java.lang.String label) {
this.label = label;
return this;
}
/**
* The type of URL. Possible values include, but are not limited to, the following values: -
* "otherProfile" - URL for another profile. - "contributor" - URL to a site for which this
* person is a contributor. - "website" - URL for this Google+ Page's primary website. - "other"
* - Other URL.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The type of URL. Possible values include, but are not limited to, the following values: -
* "otherProfile" - URL for another profile. - "contributor" - URL to a site for which this
* person is a contributor. - "website" - URL for this Google+ Page's primary website. - "other"
* - Other URL.
* @param type type or {@code null} for none
*/
public Urls setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* The URL value.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* The URL value.
* @param value value or {@code null} for none
*/
public Urls setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public Urls set(String fieldName, Object value) {
return (Urls) super.set(fieldName, value);
}
@Override
public Urls clone() {
return (Urls) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy