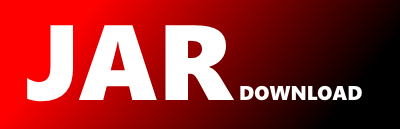
com.google.api.services.prediction.model.Analyze Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2013-12-19 23:55:21 UTC)
* on 2014-01-07 at 21:42:56 UTC
* Modify at your own risk.
*/
package com.google.api.services.prediction.model;
/**
* Model definition for Analyze.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Prediction API. For a detailed explanation see:
* http://code.google.com/p/google-api-java-client/wiki/Json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Analyze extends com.google.api.client.json.GenericJson {
/**
* Description of the data the model was trained on.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DataDescription dataDescription;
/**
* List of errors with the data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List> errors;
/**
* The unique name for the predictive model.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* What kind of resource this is.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Description of the model.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ModelDescription modelDescription;
/**
* A URL to re-request this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Description of the data the model was trained on.
* @return value or {@code null} for none
*/
public DataDescription getDataDescription() {
return dataDescription;
}
/**
* Description of the data the model was trained on.
* @param dataDescription dataDescription or {@code null} for none
*/
public Analyze setDataDescription(DataDescription dataDescription) {
this.dataDescription = dataDescription;
return this;
}
/**
* List of errors with the data.
* @return value or {@code null} for none
*/
public java.util.List> getErrors() {
return errors;
}
/**
* List of errors with the data.
* @param errors errors or {@code null} for none
*/
public Analyze setErrors(java.util.List> errors) {
this.errors = errors;
return this;
}
/**
* The unique name for the predictive model.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The unique name for the predictive model.
* @param id id or {@code null} for none
*/
public Analyze setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* What kind of resource this is.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* What kind of resource this is.
* @param kind kind or {@code null} for none
*/
public Analyze setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Description of the model.
* @return value or {@code null} for none
*/
public ModelDescription getModelDescription() {
return modelDescription;
}
/**
* Description of the model.
* @param modelDescription modelDescription or {@code null} for none
*/
public Analyze setModelDescription(ModelDescription modelDescription) {
this.modelDescription = modelDescription;
return this;
}
/**
* A URL to re-request this resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* A URL to re-request this resource.
* @param selfLink selfLink or {@code null} for none
*/
public Analyze setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
@Override
public Analyze set(String fieldName, Object value) {
return (Analyze) super.set(fieldName, value);
}
@Override
public Analyze clone() {
return (Analyze) super.clone();
}
/**
* Description of the data the model was trained on.
*/
public static final class DataDescription extends com.google.api.client.json.GenericJson {
/**
* Description of the input features in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List features;
static {
// hack to force ProGuard to consider Features used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Features.class);
}
/**
* Description of the output value or label.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private OutputFeature outputFeature;
/**
* Description of the input features in the data set.
* @return value or {@code null} for none
*/
public java.util.List getFeatures() {
return features;
}
/**
* Description of the input features in the data set.
* @param features features or {@code null} for none
*/
public DataDescription setFeatures(java.util.List features) {
this.features = features;
return this;
}
/**
* Description of the output value or label.
* @return value or {@code null} for none
*/
public OutputFeature getOutputFeature() {
return outputFeature;
}
/**
* Description of the output value or label.
* @param outputFeature outputFeature or {@code null} for none
*/
public DataDescription setOutputFeature(OutputFeature outputFeature) {
this.outputFeature = outputFeature;
return this;
}
@Override
public DataDescription set(String fieldName, Object value) {
return (DataDescription) super.set(fieldName, value);
}
@Override
public DataDescription clone() {
return (DataDescription) super.clone();
}
/**
* Model definition for AnalyzeDataDescriptionFeatures.
*/
public static final class Features extends com.google.api.client.json.GenericJson {
/**
* Description of the categorical values of this feature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Categorical categorical;
/**
* The feature index.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long index;
/**
* Description of the numeric values of this feature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Numeric numeric;
/**
* Description of multiple-word text values of this feature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Text text;
/**
* Description of the categorical values of this feature.
* @return value or {@code null} for none
*/
public Categorical getCategorical() {
return categorical;
}
/**
* Description of the categorical values of this feature.
* @param categorical categorical or {@code null} for none
*/
public Features setCategorical(Categorical categorical) {
this.categorical = categorical;
return this;
}
/**
* The feature index.
* @return value or {@code null} for none
*/
public java.lang.Long getIndex() {
return index;
}
/**
* The feature index.
* @param index index or {@code null} for none
*/
public Features setIndex(java.lang.Long index) {
this.index = index;
return this;
}
/**
* Description of the numeric values of this feature.
* @return value or {@code null} for none
*/
public Numeric getNumeric() {
return numeric;
}
/**
* Description of the numeric values of this feature.
* @param numeric numeric or {@code null} for none
*/
public Features setNumeric(Numeric numeric) {
this.numeric = numeric;
return this;
}
/**
* Description of multiple-word text values of this feature.
* @return value or {@code null} for none
*/
public Text getText() {
return text;
}
/**
* Description of multiple-word text values of this feature.
* @param text text or {@code null} for none
*/
public Features setText(Text text) {
this.text = text;
return this;
}
@Override
public Features set(String fieldName, Object value) {
return (Features) super.set(fieldName, value);
}
@Override
public Features clone() {
return (Features) super.clone();
}
/**
* Description of the categorical values of this feature.
*/
public static final class Categorical extends com.google.api.client.json.GenericJson {
/**
* Number of categorical values for this feature in the data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long count;
/**
* List of all the categories for this feature in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List values;
static {
// hack to force ProGuard to consider Values used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Values.class);
}
/**
* Number of categorical values for this feature in the data.
* @return value or {@code null} for none
*/
public java.lang.Long getCount() {
return count;
}
/**
* Number of categorical values for this feature in the data.
* @param count count or {@code null} for none
*/
public Categorical setCount(java.lang.Long count) {
this.count = count;
return this;
}
/**
* List of all the categories for this feature in the data set.
* @return value or {@code null} for none
*/
public java.util.List getValues() {
return values;
}
/**
* List of all the categories for this feature in the data set.
* @param values values or {@code null} for none
*/
public Categorical setValues(java.util.List values) {
this.values = values;
return this;
}
@Override
public Categorical set(String fieldName, Object value) {
return (Categorical) super.set(fieldName, value);
}
@Override
public Categorical clone() {
return (Categorical) super.clone();
}
/**
* Model definition for AnalyzeDataDescriptionFeaturesCategoricalValues.
*/
public static final class Values extends com.google.api.client.json.GenericJson {
/**
* Number of times this feature had this value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long count;
/**
* The category name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* Number of times this feature had this value.
* @return value or {@code null} for none
*/
public java.lang.Long getCount() {
return count;
}
/**
* Number of times this feature had this value.
* @param count count or {@code null} for none
*/
public Values setCount(java.lang.Long count) {
this.count = count;
return this;
}
/**
* The category name.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* The category name.
* @param value value or {@code null} for none
*/
public Values setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public Values set(String fieldName, Object value) {
return (Values) super.set(fieldName, value);
}
@Override
public Values clone() {
return (Values) super.clone();
}
}
}
/**
* Description of the numeric values of this feature.
*/
public static final class Numeric extends com.google.api.client.json.GenericJson {
/**
* Number of numeric values for this feature in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long count;
/**
* Mean of the numeric values of this feature in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double mean;
/**
* Variance of the numeric values of this feature in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double variance;
/**
* Number of numeric values for this feature in the data set.
* @return value or {@code null} for none
*/
public java.lang.Long getCount() {
return count;
}
/**
* Number of numeric values for this feature in the data set.
* @param count count or {@code null} for none
*/
public Numeric setCount(java.lang.Long count) {
this.count = count;
return this;
}
/**
* Mean of the numeric values of this feature in the data set.
* @return value or {@code null} for none
*/
public java.lang.Double getMean() {
return mean;
}
/**
* Mean of the numeric values of this feature in the data set.
* @param mean mean or {@code null} for none
*/
public Numeric setMean(java.lang.Double mean) {
this.mean = mean;
return this;
}
/**
* Variance of the numeric values of this feature in the data set.
* @return value or {@code null} for none
*/
public java.lang.Double getVariance() {
return variance;
}
/**
* Variance of the numeric values of this feature in the data set.
* @param variance variance or {@code null} for none
*/
public Numeric setVariance(java.lang.Double variance) {
this.variance = variance;
return this;
}
@Override
public Numeric set(String fieldName, Object value) {
return (Numeric) super.set(fieldName, value);
}
@Override
public Numeric clone() {
return (Numeric) super.clone();
}
}
/**
* Description of multiple-word text values of this feature.
*/
public static final class Text extends com.google.api.client.json.GenericJson {
/**
* Number of multiple-word text values for this feature.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long count;
/**
* Number of multiple-word text values for this feature.
* @return value or {@code null} for none
*/
public java.lang.Long getCount() {
return count;
}
/**
* Number of multiple-word text values for this feature.
* @param count count or {@code null} for none
*/
public Text setCount(java.lang.Long count) {
this.count = count;
return this;
}
@Override
public Text set(String fieldName, Object value) {
return (Text) super.set(fieldName, value);
}
@Override
public Text clone() {
return (Text) super.clone();
}
}
}
/**
* Description of the output value or label.
*/
public static final class OutputFeature extends com.google.api.client.json.GenericJson {
/**
* Description of the output values in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Numeric numeric;
/**
* Description of the output labels in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List text;
static {
// hack to force ProGuard to consider Text used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Text.class);
}
/**
* Description of the output values in the data set.
* @return value or {@code null} for none
*/
public Numeric getNumeric() {
return numeric;
}
/**
* Description of the output values in the data set.
* @param numeric numeric or {@code null} for none
*/
public OutputFeature setNumeric(Numeric numeric) {
this.numeric = numeric;
return this;
}
/**
* Description of the output labels in the data set.
* @return value or {@code null} for none
*/
public java.util.List getText() {
return text;
}
/**
* Description of the output labels in the data set.
* @param text text or {@code null} for none
*/
public OutputFeature setText(java.util.List text) {
this.text = text;
return this;
}
@Override
public OutputFeature set(String fieldName, Object value) {
return (OutputFeature) super.set(fieldName, value);
}
@Override
public OutputFeature clone() {
return (OutputFeature) super.clone();
}
/**
* Description of the output values in the data set.
*/
public static final class Numeric extends com.google.api.client.json.GenericJson {
/**
* Number of numeric output values in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long count;
/**
* Mean of the output values in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double mean;
/**
* Variance of the output values in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double variance;
/**
* Number of numeric output values in the data set.
* @return value or {@code null} for none
*/
public java.lang.Long getCount() {
return count;
}
/**
* Number of numeric output values in the data set.
* @param count count or {@code null} for none
*/
public Numeric setCount(java.lang.Long count) {
this.count = count;
return this;
}
/**
* Mean of the output values in the data set.
* @return value or {@code null} for none
*/
public java.lang.Double getMean() {
return mean;
}
/**
* Mean of the output values in the data set.
* @param mean mean or {@code null} for none
*/
public Numeric setMean(java.lang.Double mean) {
this.mean = mean;
return this;
}
/**
* Variance of the output values in the data set.
* @return value or {@code null} for none
*/
public java.lang.Double getVariance() {
return variance;
}
/**
* Variance of the output values in the data set.
* @param variance variance or {@code null} for none
*/
public Numeric setVariance(java.lang.Double variance) {
this.variance = variance;
return this;
}
@Override
public Numeric set(String fieldName, Object value) {
return (Numeric) super.set(fieldName, value);
}
@Override
public Numeric clone() {
return (Numeric) super.clone();
}
}
/**
* Model definition for AnalyzeDataDescriptionOutputFeatureText.
*/
public static final class Text extends com.google.api.client.json.GenericJson {
/**
* Number of times the output label occurred in the data set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long count;
/**
* The output label.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* Number of times the output label occurred in the data set.
* @return value or {@code null} for none
*/
public java.lang.Long getCount() {
return count;
}
/**
* Number of times the output label occurred in the data set.
* @param count count or {@code null} for none
*/
public Text setCount(java.lang.Long count) {
this.count = count;
return this;
}
/**
* The output label.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* The output label.
* @param value value or {@code null} for none
*/
public Text setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public Text set(String fieldName, Object value) {
return (Text) super.set(fieldName, value);
}
@Override
public Text clone() {
return (Text) super.clone();
}
}
}
}
/**
* Description of the model.
*/
public static final class ModelDescription extends com.google.api.client.json.GenericJson {
/**
* An output confusion matrix. This shows an estimate for how this model will do in predictions.
* This is first indexed by the true class label. For each true class label, this provides a pair
* {predicted_label, count}, where count is the estimated number of times the model will predict
* the predicted label given the true label. Will not output if more then 100 classes [Categorical
* models only].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map> confusionMatrix;
/**
* A list of the confusion matrix row totals
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map confusionMatrixRowTotals;
/**
* Basic information about the model.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Training modelinfo;
/**
* An output confusion matrix. This shows an estimate for how this model will do in predictions.
* This is first indexed by the true class label. For each true class label, this provides a pair
* {predicted_label, count}, where count is the estimated number of times the model will predict
* the predicted label given the true label. Will not output if more then 100 classes [Categorical
* models only].
* @return value or {@code null} for none
*/
public java.util.Map> getConfusionMatrix() {
return confusionMatrix;
}
/**
* An output confusion matrix. This shows an estimate for how this model will do in predictions.
* This is first indexed by the true class label. For each true class label, this provides a pair
* {predicted_label, count}, where count is the estimated number of times the model will predict
* the predicted label given the true label. Will not output if more then 100 classes [Categorical
* models only].
* @param confusionMatrix confusionMatrix or {@code null} for none
*/
public ModelDescription setConfusionMatrix(java.util.Map> confusionMatrix) {
this.confusionMatrix = confusionMatrix;
return this;
}
/**
* A list of the confusion matrix row totals
* @return value or {@code null} for none
*/
public java.util.Map getConfusionMatrixRowTotals() {
return confusionMatrixRowTotals;
}
/**
* A list of the confusion matrix row totals
* @param confusionMatrixRowTotals confusionMatrixRowTotals or {@code null} for none
*/
public ModelDescription setConfusionMatrixRowTotals(java.util.Map confusionMatrixRowTotals) {
this.confusionMatrixRowTotals = confusionMatrixRowTotals;
return this;
}
/**
* Basic information about the model.
* @return value or {@code null} for none
*/
public Training getModelinfo() {
return modelinfo;
}
/**
* Basic information about the model.
* @param modelinfo modelinfo or {@code null} for none
*/
public ModelDescription setModelinfo(Training modelinfo) {
this.modelinfo = modelinfo;
return this;
}
@Override
public ModelDescription set(String fieldName, Object value) {
return (ModelDescription) super.set(fieldName, value);
}
@Override
public ModelDescription clone() {
return (ModelDescription) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy