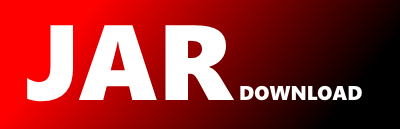
com.google.api.services.prediction.model.Training Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2013-12-19 23:55:21 UTC)
* on 2014-01-07 at 21:42:56 UTC
* Modify at your own risk.
*/
package com.google.api.services.prediction.model;
/**
* Model definition for Training.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Prediction API. For a detailed explanation see:
* http://code.google.com/p/google-api-java-client/wiki/Json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Training extends com.google.api.client.json.GenericJson {
/**
* Insert time of the model (as a RFC 3339 timestamp).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime created;
/**
* The unique name for the predictive model.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* What kind of resource this is.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Model metadata.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ModelInfo modelInfo;
/**
* Type of predictive model (classification or regression)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String modelType;
/**
* A URL to re-request this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Google storage location of the training data file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String storageDataLocation;
/**
* Google storage location of the preprocessing pmml file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String storagePMMLLocation;
/**
* Google storage location of the pmml model file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String storagePMMLModelLocation;
/**
* Training completion time (as a RFC 3339 timestamp).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime trainingComplete;
/**
* Instances to train model on.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List trainingInstances;
static {
// hack to force ProGuard to consider TrainingInstances used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(TrainingInstances.class);
}
/**
* The current status of the training job. This can be one of following: RUNNING; DONE; ERROR;
* ERROR: TRAINING JOB NOT FOUND
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String trainingStatus;
/**
* A class weighting function, which allows the importance weights for class labels to be
* specified [Categorical models only].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List> utility;
/**
* Insert time of the model (as a RFC 3339 timestamp).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getCreated() {
return created;
}
/**
* Insert time of the model (as a RFC 3339 timestamp).
* @param created created or {@code null} for none
*/
public Training setCreated(com.google.api.client.util.DateTime created) {
this.created = created;
return this;
}
/**
* The unique name for the predictive model.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The unique name for the predictive model.
* @param id id or {@code null} for none
*/
public Training setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* What kind of resource this is.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* What kind of resource this is.
* @param kind kind or {@code null} for none
*/
public Training setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Model metadata.
* @return value or {@code null} for none
*/
public ModelInfo getModelInfo() {
return modelInfo;
}
/**
* Model metadata.
* @param modelInfo modelInfo or {@code null} for none
*/
public Training setModelInfo(ModelInfo modelInfo) {
this.modelInfo = modelInfo;
return this;
}
/**
* Type of predictive model (classification or regression)
* @return value or {@code null} for none
*/
public java.lang.String getModelType() {
return modelType;
}
/**
* Type of predictive model (classification or regression)
* @param modelType modelType or {@code null} for none
*/
public Training setModelType(java.lang.String modelType) {
this.modelType = modelType;
return this;
}
/**
* A URL to re-request this resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* A URL to re-request this resource.
* @param selfLink selfLink or {@code null} for none
*/
public Training setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* Google storage location of the training data file.
* @return value or {@code null} for none
*/
public java.lang.String getStorageDataLocation() {
return storageDataLocation;
}
/**
* Google storage location of the training data file.
* @param storageDataLocation storageDataLocation or {@code null} for none
*/
public Training setStorageDataLocation(java.lang.String storageDataLocation) {
this.storageDataLocation = storageDataLocation;
return this;
}
/**
* Google storage location of the preprocessing pmml file.
* @return value or {@code null} for none
*/
public java.lang.String getStoragePMMLLocation() {
return storagePMMLLocation;
}
/**
* Google storage location of the preprocessing pmml file.
* @param storagePMMLLocation storagePMMLLocation or {@code null} for none
*/
public Training setStoragePMMLLocation(java.lang.String storagePMMLLocation) {
this.storagePMMLLocation = storagePMMLLocation;
return this;
}
/**
* Google storage location of the pmml model file.
* @return value or {@code null} for none
*/
public java.lang.String getStoragePMMLModelLocation() {
return storagePMMLModelLocation;
}
/**
* Google storage location of the pmml model file.
* @param storagePMMLModelLocation storagePMMLModelLocation or {@code null} for none
*/
public Training setStoragePMMLModelLocation(java.lang.String storagePMMLModelLocation) {
this.storagePMMLModelLocation = storagePMMLModelLocation;
return this;
}
/**
* Training completion time (as a RFC 3339 timestamp).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getTrainingComplete() {
return trainingComplete;
}
/**
* Training completion time (as a RFC 3339 timestamp).
* @param trainingComplete trainingComplete or {@code null} for none
*/
public Training setTrainingComplete(com.google.api.client.util.DateTime trainingComplete) {
this.trainingComplete = trainingComplete;
return this;
}
/**
* Instances to train model on.
* @return value or {@code null} for none
*/
public java.util.List getTrainingInstances() {
return trainingInstances;
}
/**
* Instances to train model on.
* @param trainingInstances trainingInstances or {@code null} for none
*/
public Training setTrainingInstances(java.util.List trainingInstances) {
this.trainingInstances = trainingInstances;
return this;
}
/**
* The current status of the training job. This can be one of following: RUNNING; DONE; ERROR;
* ERROR: TRAINING JOB NOT FOUND
* @return value or {@code null} for none
*/
public java.lang.String getTrainingStatus() {
return trainingStatus;
}
/**
* The current status of the training job. This can be one of following: RUNNING; DONE; ERROR;
* ERROR: TRAINING JOB NOT FOUND
* @param trainingStatus trainingStatus or {@code null} for none
*/
public Training setTrainingStatus(java.lang.String trainingStatus) {
this.trainingStatus = trainingStatus;
return this;
}
/**
* A class weighting function, which allows the importance weights for class labels to be
* specified [Categorical models only].
* @return value or {@code null} for none
*/
public java.util.List> getUtility() {
return utility;
}
/**
* A class weighting function, which allows the importance weights for class labels to be
* specified [Categorical models only].
* @param utility utility or {@code null} for none
*/
public Training setUtility(java.util.List> utility) {
this.utility = utility;
return this;
}
@Override
public Training set(String fieldName, Object value) {
return (Training) super.set(fieldName, value);
}
@Override
public Training clone() {
return (Training) super.clone();
}
/**
* Model metadata.
*/
public static final class ModelInfo extends com.google.api.client.json.GenericJson {
/**
* Estimated accuracy of model taking utility weights into account [Categorical models only].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double classWeightedAccuracy;
/**
* A number between 0.0 and 1.0, where 1.0 is 100% accurate. This is an estimate, based on the
* amount and quality of the training data, of the estimated prediction accuracy. You can use this
* is a guide to decide whether the results are accurate enough for your needs. This estimate will
* be more reliable if your real input data is similar to your training data [Categorical models
* only].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double classificationAccuracy;
/**
* An estimated mean squared error. The can be used to measure the quality of the predicted model
* [Regression models only].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double meanSquaredError;
/**
* Type of predictive model (CLASSIFICATION or REGRESSION)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String modelType;
/**
* Number of valid data instances used in the trained model.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numberInstances;
/**
* Number of class labels in the trained model [Categorical models only].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numberLabels;
/**
* Estimated accuracy of model taking utility weights into account [Categorical models only].
* @return value or {@code null} for none
*/
public java.lang.Double getClassWeightedAccuracy() {
return classWeightedAccuracy;
}
/**
* Estimated accuracy of model taking utility weights into account [Categorical models only].
* @param classWeightedAccuracy classWeightedAccuracy or {@code null} for none
*/
public ModelInfo setClassWeightedAccuracy(java.lang.Double classWeightedAccuracy) {
this.classWeightedAccuracy = classWeightedAccuracy;
return this;
}
/**
* A number between 0.0 and 1.0, where 1.0 is 100% accurate. This is an estimate, based on the
* amount and quality of the training data, of the estimated prediction accuracy. You can use this
* is a guide to decide whether the results are accurate enough for your needs. This estimate will
* be more reliable if your real input data is similar to your training data [Categorical models
* only].
* @return value or {@code null} for none
*/
public java.lang.Double getClassificationAccuracy() {
return classificationAccuracy;
}
/**
* A number between 0.0 and 1.0, where 1.0 is 100% accurate. This is an estimate, based on the
* amount and quality of the training data, of the estimated prediction accuracy. You can use this
* is a guide to decide whether the results are accurate enough for your needs. This estimate will
* be more reliable if your real input data is similar to your training data [Categorical models
* only].
* @param classificationAccuracy classificationAccuracy or {@code null} for none
*/
public ModelInfo setClassificationAccuracy(java.lang.Double classificationAccuracy) {
this.classificationAccuracy = classificationAccuracy;
return this;
}
/**
* An estimated mean squared error. The can be used to measure the quality of the predicted model
* [Regression models only].
* @return value or {@code null} for none
*/
public java.lang.Double getMeanSquaredError() {
return meanSquaredError;
}
/**
* An estimated mean squared error. The can be used to measure the quality of the predicted model
* [Regression models only].
* @param meanSquaredError meanSquaredError or {@code null} for none
*/
public ModelInfo setMeanSquaredError(java.lang.Double meanSquaredError) {
this.meanSquaredError = meanSquaredError;
return this;
}
/**
* Type of predictive model (CLASSIFICATION or REGRESSION)
* @return value or {@code null} for none
*/
public java.lang.String getModelType() {
return modelType;
}
/**
* Type of predictive model (CLASSIFICATION or REGRESSION)
* @param modelType modelType or {@code null} for none
*/
public ModelInfo setModelType(java.lang.String modelType) {
this.modelType = modelType;
return this;
}
/**
* Number of valid data instances used in the trained model.
* @return value or {@code null} for none
*/
public java.lang.Long getNumberInstances() {
return numberInstances;
}
/**
* Number of valid data instances used in the trained model.
* @param numberInstances numberInstances or {@code null} for none
*/
public ModelInfo setNumberInstances(java.lang.Long numberInstances) {
this.numberInstances = numberInstances;
return this;
}
/**
* Number of class labels in the trained model [Categorical models only].
* @return value or {@code null} for none
*/
public java.lang.Long getNumberLabels() {
return numberLabels;
}
/**
* Number of class labels in the trained model [Categorical models only].
* @param numberLabels numberLabels or {@code null} for none
*/
public ModelInfo setNumberLabels(java.lang.Long numberLabels) {
this.numberLabels = numberLabels;
return this;
}
@Override
public ModelInfo set(String fieldName, Object value) {
return (ModelInfo) super.set(fieldName, value);
}
@Override
public ModelInfo clone() {
return (ModelInfo) super.clone();
}
}
/**
* Model definition for TrainingTrainingInstances.
*/
public static final class TrainingInstances extends com.google.api.client.json.GenericJson {
/**
* The input features for this instance
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List csvInstance;
/**
* The generic output value - could be regression or class label
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String output;
/**
* The input features for this instance
* @return value or {@code null} for none
*/
public java.util.List getCsvInstance() {
return csvInstance;
}
/**
* The input features for this instance
* @param csvInstance csvInstance or {@code null} for none
*/
public TrainingInstances setCsvInstance(java.util.List csvInstance) {
this.csvInstance = csvInstance;
return this;
}
/**
* The generic output value - could be regression or class label
* @return value or {@code null} for none
*/
public java.lang.String getOutput() {
return output;
}
/**
* The generic output value - could be regression or class label
* @param output output or {@code null} for none
*/
public TrainingInstances setOutput(java.lang.String output) {
this.output = output;
return this;
}
@Override
public TrainingInstances set(String fieldName, Object value) {
return (TrainingInstances) super.set(fieldName, value);
}
@Override
public TrainingInstances clone() {
return (TrainingInstances) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy