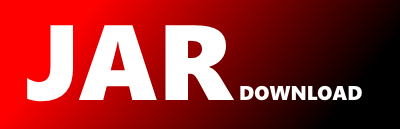
target.apidocs.com.google.api.services.pubsub.model.PubsubMessage.html Maven / Gradle / Ivy
PubsubMessage (Cloud Pub/Sub API v1-rev20241212-2.0.0)
com.google.api.services.pubsub.model
Class PubsubMessage
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.pubsub.model.PubsubMessage
-
public final class PubsubMessage
extends com.google.api.client.json.GenericJson
A message that is published by publishers and consumed by subscribers. The message must contain
either a non-empty data field or at least one attribute. Note that client libraries represent
this object differently depending on the language. See the corresponding [client library
documentation](https://cloud.google.com/pubsub/docs/reference/libraries) for more information.
See [quotas and limits] (https://cloud.google.com/pubsub/quotas) for more information about
message limits.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Cloud Pub/Sub API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
PubsubMessage()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
PubsubMessage
clone()
byte[]
decodeData()
Optional.
PubsubMessage
encodeData(byte[] data)
Optional.
Map<String,String>
getAttributes()
Optional.
String
getData()
Optional.
String
getMessageId()
ID of this message, assigned by the server when the message is published.
String
getOrderingKey()
Optional.
String
getPublishTime()
The time at which the message was published, populated by the server when it receives the
`Publish` call.
PubsubMessage
set(String fieldName,
Object value)
PubsubMessage
setAttributes(Map<String,String> attributes)
Optional.
PubsubMessage
setData(String data)
Optional.
PubsubMessage
setMessageId(String messageId)
ID of this message, assigned by the server when the message is published.
PubsubMessage
setOrderingKey(String orderingKey)
Optional.
PubsubMessage
setPublishTime(String publishTime)
The time at which the message was published, populated by the server when it receives the
`Publish` call.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAttributes
public Map<String,String> getAttributes()
Optional. Attributes for this message. If this field is empty, the message must contain non-
empty data. This can be used to filter messages on the subscription.
- Returns:
- value or
null
for none
-
setAttributes
public PubsubMessage setAttributes(Map<String,String> attributes)
Optional. Attributes for this message. If this field is empty, the message must contain non-
empty data. This can be used to filter messages on the subscription.
- Parameters:
attributes
- attributes or null
for none
-
getData
public String getData()
Optional. The message data field. If this field is empty, the message must contain at least one
attribute.
- Returns:
- value or
null
for none
- See Also:
decodeData()
-
decodeData
public byte[] decodeData()
Optional. The message data field. If this field is empty, the message must contain at least one
attribute.
- Returns:
- Base64 decoded value or
null
for none
- Since:
- 1.14
- See Also:
getData()
-
setData
public PubsubMessage setData(String data)
Optional. The message data field. If this field is empty, the message must contain at least one
attribute.
- Parameters:
data
- data or null
for none
- See Also:
#encodeData()
-
encodeData
public PubsubMessage encodeData(byte[] data)
Optional. The message data field. If this field is empty, the message must contain at least one
attribute.
- Since:
- 1.14
- See Also:
The value is encoded Base64 or {@code null} for none.
-
getMessageId
public String getMessageId()
ID of this message, assigned by the server when the message is published. Guaranteed to be
unique within the topic. This value may be read by a subscriber that receives a `PubsubMessage`
via a `Pull` call or a push delivery. It must not be populated by the publisher in a `Publish`
call.
- Returns:
- value or
null
for none
-
setMessageId
public PubsubMessage setMessageId(String messageId)
ID of this message, assigned by the server when the message is published. Guaranteed to be
unique within the topic. This value may be read by a subscriber that receives a `PubsubMessage`
via a `Pull` call or a push delivery. It must not be populated by the publisher in a `Publish`
call.
- Parameters:
messageId
- messageId or null
for none
-
getOrderingKey
public String getOrderingKey()
Optional. If non-empty, identifies related messages for which publish order should be
respected. If a `Subscription` has `enable_message_ordering` set to `true`, messages published
with the same non-empty `ordering_key` value will be delivered to subscribers in the order in
which they are received by the Pub/Sub system. All `PubsubMessage`s published in a given
`PublishRequest` must specify the same `ordering_key` value. For more information, see
[ordering messages](https://cloud.google.com/pubsub/docs/ordering).
- Returns:
- value or
null
for none
-
setOrderingKey
public PubsubMessage setOrderingKey(String orderingKey)
Optional. If non-empty, identifies related messages for which publish order should be
respected. If a `Subscription` has `enable_message_ordering` set to `true`, messages published
with the same non-empty `ordering_key` value will be delivered to subscribers in the order in
which they are received by the Pub/Sub system. All `PubsubMessage`s published in a given
`PublishRequest` must specify the same `ordering_key` value. For more information, see
[ordering messages](https://cloud.google.com/pubsub/docs/ordering).
- Parameters:
orderingKey
- orderingKey or null
for none
-
getPublishTime
public String getPublishTime()
The time at which the message was published, populated by the server when it receives the
`Publish` call. It must not be populated by the publisher in a `Publish` call.
- Returns:
- value or
null
for none
-
setPublishTime
public PubsubMessage setPublishTime(String publishTime)
The time at which the message was published, populated by the server when it receives the
`Publish` call. It must not be populated by the publisher in a `Publish` call.
- Parameters:
publishTime
- publishTime or null
for none
-
set
public PubsubMessage set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public PubsubMessage clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy