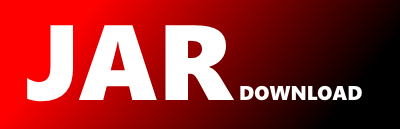
com.google.api.services.qpxExpress.model.PricingInfo Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2014-02-14 18:40:25 UTC)
* on 2014-02-22 at 02:08:00 UTC
* Modify at your own risk.
*/
package com.google.api.services.qpxExpress.model;
/**
* The price of one or more travel segments. The currency used to purchase tickets is usually
* determined by the sale/ticketing city or the sale/ticketing country, unless none are specified,
* in which case it defaults to that of the journey origin country.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the QPX Express API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PricingInfo extends com.google.api.client.json.GenericJson {
/**
* The total fare in the base fare currency (the currency of the country of origin). This element
* is only present when the sales currency and the currency of the country of commencement are
* different.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String baseFareTotal;
/**
* The fare used to price one or more segments.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List fare;
static {
// hack to force ProGuard to consider FareInfo used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(FareInfo.class);
}
/**
* The horizontal fare calculation. This is a field on a ticket that displays all of the relevant
* items that go into the calculation of the fare.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fareCalculation;
/**
* Identifies this as a pricing object, representing the price of one or more travel segments.
* Value: the fixed string qpxexpress#pricingInfo.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The latest ticketing time for this pricing assuming the reservation occurs at ticketing time
* and there is no change in fares/rules. The time is local to the point of sale (POS).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String latestTicketingTime;
/**
* The number of passengers to which this price applies.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PassengerCounts passengers;
/**
* The passenger type code for this pricing. An alphanumeric code used by a carrier to restrict
* fares to certain categories of passenger. For instance, a fare might be valid only for senior
* citizens.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ptc;
/**
* Whether the fares on this pricing are refundable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean refundable;
/**
* The total fare in the sale or equivalent currency.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String saleFareTotal;
/**
* The taxes in the sale or equivalent currency.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String saleTaxTotal;
/**
* Total per-passenger price (fare and tax) in the sale or equivalent currency.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String saleTotal;
/**
* The per-segment price and baggage information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List segmentPricing;
static {
// hack to force ProGuard to consider SegmentPricing used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(SegmentPricing.class);
}
/**
* The taxes used to calculate the tax total per ticket.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tax;
/**
* The total fare in the base fare currency (the currency of the country of origin). This element
* is only present when the sales currency and the currency of the country of commencement are
* different.
* @return value or {@code null} for none
*/
public java.lang.String getBaseFareTotal() {
return baseFareTotal;
}
/**
* The total fare in the base fare currency (the currency of the country of origin). This element
* is only present when the sales currency and the currency of the country of commencement are
* different.
* @param baseFareTotal baseFareTotal or {@code null} for none
*/
public PricingInfo setBaseFareTotal(java.lang.String baseFareTotal) {
this.baseFareTotal = baseFareTotal;
return this;
}
/**
* The fare used to price one or more segments.
* @return value or {@code null} for none
*/
public java.util.List getFare() {
return fare;
}
/**
* The fare used to price one or more segments.
* @param fare fare or {@code null} for none
*/
public PricingInfo setFare(java.util.List fare) {
this.fare = fare;
return this;
}
/**
* The horizontal fare calculation. This is a field on a ticket that displays all of the relevant
* items that go into the calculation of the fare.
* @return value or {@code null} for none
*/
public java.lang.String getFareCalculation() {
return fareCalculation;
}
/**
* The horizontal fare calculation. This is a field on a ticket that displays all of the relevant
* items that go into the calculation of the fare.
* @param fareCalculation fareCalculation or {@code null} for none
*/
public PricingInfo setFareCalculation(java.lang.String fareCalculation) {
this.fareCalculation = fareCalculation;
return this;
}
/**
* Identifies this as a pricing object, representing the price of one or more travel segments.
* Value: the fixed string qpxexpress#pricingInfo.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies this as a pricing object, representing the price of one or more travel segments.
* Value: the fixed string qpxexpress#pricingInfo.
* @param kind kind or {@code null} for none
*/
public PricingInfo setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The latest ticketing time for this pricing assuming the reservation occurs at ticketing time
* and there is no change in fares/rules. The time is local to the point of sale (POS).
* @return value or {@code null} for none
*/
public java.lang.String getLatestTicketingTime() {
return latestTicketingTime;
}
/**
* The latest ticketing time for this pricing assuming the reservation occurs at ticketing time
* and there is no change in fares/rules. The time is local to the point of sale (POS).
* @param latestTicketingTime latestTicketingTime or {@code null} for none
*/
public PricingInfo setLatestTicketingTime(java.lang.String latestTicketingTime) {
this.latestTicketingTime = latestTicketingTime;
return this;
}
/**
* The number of passengers to which this price applies.
* @return value or {@code null} for none
*/
public PassengerCounts getPassengers() {
return passengers;
}
/**
* The number of passengers to which this price applies.
* @param passengers passengers or {@code null} for none
*/
public PricingInfo setPassengers(PassengerCounts passengers) {
this.passengers = passengers;
return this;
}
/**
* The passenger type code for this pricing. An alphanumeric code used by a carrier to restrict
* fares to certain categories of passenger. For instance, a fare might be valid only for senior
* citizens.
* @return value or {@code null} for none
*/
public java.lang.String getPtc() {
return ptc;
}
/**
* The passenger type code for this pricing. An alphanumeric code used by a carrier to restrict
* fares to certain categories of passenger. For instance, a fare might be valid only for senior
* citizens.
* @param ptc ptc or {@code null} for none
*/
public PricingInfo setPtc(java.lang.String ptc) {
this.ptc = ptc;
return this;
}
/**
* Whether the fares on this pricing are refundable.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRefundable() {
return refundable;
}
/**
* Whether the fares on this pricing are refundable.
* @param refundable refundable or {@code null} for none
*/
public PricingInfo setRefundable(java.lang.Boolean refundable) {
this.refundable = refundable;
return this;
}
/**
* The total fare in the sale or equivalent currency.
* @return value or {@code null} for none
*/
public java.lang.String getSaleFareTotal() {
return saleFareTotal;
}
/**
* The total fare in the sale or equivalent currency.
* @param saleFareTotal saleFareTotal or {@code null} for none
*/
public PricingInfo setSaleFareTotal(java.lang.String saleFareTotal) {
this.saleFareTotal = saleFareTotal;
return this;
}
/**
* The taxes in the sale or equivalent currency.
* @return value or {@code null} for none
*/
public java.lang.String getSaleTaxTotal() {
return saleTaxTotal;
}
/**
* The taxes in the sale or equivalent currency.
* @param saleTaxTotal saleTaxTotal or {@code null} for none
*/
public PricingInfo setSaleTaxTotal(java.lang.String saleTaxTotal) {
this.saleTaxTotal = saleTaxTotal;
return this;
}
/**
* Total per-passenger price (fare and tax) in the sale or equivalent currency.
* @return value or {@code null} for none
*/
public java.lang.String getSaleTotal() {
return saleTotal;
}
/**
* Total per-passenger price (fare and tax) in the sale or equivalent currency.
* @param saleTotal saleTotal or {@code null} for none
*/
public PricingInfo setSaleTotal(java.lang.String saleTotal) {
this.saleTotal = saleTotal;
return this;
}
/**
* The per-segment price and baggage information.
* @return value or {@code null} for none
*/
public java.util.List getSegmentPricing() {
return segmentPricing;
}
/**
* The per-segment price and baggage information.
* @param segmentPricing segmentPricing or {@code null} for none
*/
public PricingInfo setSegmentPricing(java.util.List segmentPricing) {
this.segmentPricing = segmentPricing;
return this;
}
/**
* The taxes used to calculate the tax total per ticket.
* @return value or {@code null} for none
*/
public java.util.List getTax() {
return tax;
}
/**
* The taxes used to calculate the tax total per ticket.
* @param tax tax or {@code null} for none
*/
public PricingInfo setTax(java.util.List tax) {
this.tax = tax;
return this;
}
@Override
public PricingInfo set(String fieldName, Object value) {
return (PricingInfo) super.set(fieldName, value);
}
@Override
public PricingInfo clone() {
return (PricingInfo) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy