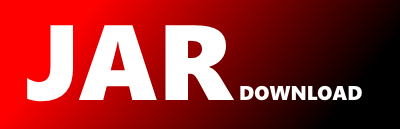
com.google.api.services.qpxExpress.model.TripOptionsRequest Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2014-02-14 18:40:25 UTC)
* on 2014-02-22 at 02:08:00 UTC
* Modify at your own risk.
*/
package com.google.api.services.qpxExpress.model;
/**
* A QPX Express search request, which will yield one or more solutions.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the QPX Express API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class TripOptionsRequest extends com.google.api.client.json.GenericJson {
/**
* Do not return solutions that cost more than this price. The alphabetical part of the price is
* in ISO 4217. The format, in regex, is [A-Z]{3}\d+(\.\d+)? Example: $102.07
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String maxPrice;
/**
* Counts for each passenger type in the request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PassengerCounts passengers;
/**
* Return only solutions with refundable fares.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean refundable;
/**
* IATA country code representing the point of sale. This determines the "equivalent amount paid"
* currency for the ticket.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String saleCountry;
/**
* The slices that make up the itinerary of this trip. A slice represents a traveler's intent, the
* portion of a low-fare search corresponding to a traveler's request to get between two points.
* One-way journeys are generally expressed using one slice, round-trips using two. An example of
* a one slice trip with three segments might be BOS-SYD, SYD-LAX, LAX-BOS if the traveler only
* stopped in SYD and LAX just long enough to change planes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List slice;
/**
* The number of solutions to return, maximum 500.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer solutions;
/**
* Do not return solutions that cost more than this price. The alphabetical part of the price is
* in ISO 4217. The format, in regex, is [A-Z]{3}\d+(\.\d+)? Example: $102.07
* @return value or {@code null} for none
*/
public java.lang.String getMaxPrice() {
return maxPrice;
}
/**
* Do not return solutions that cost more than this price. The alphabetical part of the price is
* in ISO 4217. The format, in regex, is [A-Z]{3}\d+(\.\d+)? Example: $102.07
* @param maxPrice maxPrice or {@code null} for none
*/
public TripOptionsRequest setMaxPrice(java.lang.String maxPrice) {
this.maxPrice = maxPrice;
return this;
}
/**
* Counts for each passenger type in the request.
* @return value or {@code null} for none
*/
public PassengerCounts getPassengers() {
return passengers;
}
/**
* Counts for each passenger type in the request.
* @param passengers passengers or {@code null} for none
*/
public TripOptionsRequest setPassengers(PassengerCounts passengers) {
this.passengers = passengers;
return this;
}
/**
* Return only solutions with refundable fares.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRefundable() {
return refundable;
}
/**
* Return only solutions with refundable fares.
* @param refundable refundable or {@code null} for none
*/
public TripOptionsRequest setRefundable(java.lang.Boolean refundable) {
this.refundable = refundable;
return this;
}
/**
* IATA country code representing the point of sale. This determines the "equivalent amount paid"
* currency for the ticket.
* @return value or {@code null} for none
*/
public java.lang.String getSaleCountry() {
return saleCountry;
}
/**
* IATA country code representing the point of sale. This determines the "equivalent amount paid"
* currency for the ticket.
* @param saleCountry saleCountry or {@code null} for none
*/
public TripOptionsRequest setSaleCountry(java.lang.String saleCountry) {
this.saleCountry = saleCountry;
return this;
}
/**
* The slices that make up the itinerary of this trip. A slice represents a traveler's intent, the
* portion of a low-fare search corresponding to a traveler's request to get between two points.
* One-way journeys are generally expressed using one slice, round-trips using two. An example of
* a one slice trip with three segments might be BOS-SYD, SYD-LAX, LAX-BOS if the traveler only
* stopped in SYD and LAX just long enough to change planes.
* @return value or {@code null} for none
*/
public java.util.List getSlice() {
return slice;
}
/**
* The slices that make up the itinerary of this trip. A slice represents a traveler's intent, the
* portion of a low-fare search corresponding to a traveler's request to get between two points.
* One-way journeys are generally expressed using one slice, round-trips using two. An example of
* a one slice trip with three segments might be BOS-SYD, SYD-LAX, LAX-BOS if the traveler only
* stopped in SYD and LAX just long enough to change planes.
* @param slice slice or {@code null} for none
*/
public TripOptionsRequest setSlice(java.util.List slice) {
this.slice = slice;
return this;
}
/**
* The number of solutions to return, maximum 500.
* @return value or {@code null} for none
*/
public java.lang.Integer getSolutions() {
return solutions;
}
/**
* The number of solutions to return, maximum 500.
* @param solutions solutions or {@code null} for none
*/
public TripOptionsRequest setSolutions(java.lang.Integer solutions) {
this.solutions = solutions;
return this;
}
@Override
public TripOptionsRequest set(String fieldName, Object value) {
return (TripOptionsRequest) super.set(fieldName, value);
}
@Override
public TripOptionsRequest clone() {
return (TripOptionsRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy