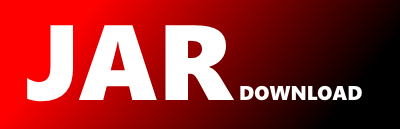
com.google.api.services.realtimebidding.v1.RealTimeBidding Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.realtimebidding.v1;
/**
* Service definition for RealTimeBidding (v1).
*
*
* Allows external bidders to manage their RTB integration with Google. This includes managing bidder endpoints, QPS quotas, configuring what ad inventory to receive via pretargeting, submitting creatives for verification, and accessing creative metadata such as approval status.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link RealTimeBiddingRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class RealTimeBidding extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Real-time Bidding API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://realtimebidding.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://realtimebidding.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public RealTimeBidding(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
RealTimeBidding(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Bidders collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.Bidders.List request = realtimebidding.bidders().list(parameters ...)}
*
*
* @return the resource collection
*/
public Bidders bidders() {
return new Bidders();
}
/**
* The "bidders" collection of methods.
*/
public class Bidders {
/**
* Gets a bidder account by its name.
*
* Create a request for the method "bidders.get".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the bidder to get. Format: `bidders/{bidderAccountId}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Gets a bidder account by its name.
*
* Create a request for the method "bidders.get".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the bidder to get. Format: `bidders/{bidderAccountId}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.Bidder.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the bidder to get. Format: `bidders/{bidderAccountId}` */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the bidder to get. Format: `bidders/{bidderAccountId}`
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the bidder to get. Format: `bidders/{bidderAccountId}` */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the bidder accounts that belong to the caller.
*
* Create a request for the method "bidders.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/bidders";
/**
* Lists all the bidder accounts that belong to the caller.
*
* Create a request for the method "bidders.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListBiddersResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of bidders to return. If unspecified, at most 100 bidders will be
* returned. The maximum value is 500; values above 500 will be coerced to 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of bidders to return. If unspecified, at most 100 bidders will be returned. The
maximum value is 500; values above 500 will be coerced to 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of bidders to return. If unspecified, at most 100 bidders will be
* returned. The maximum value is 500; values above 500 will be coerced to 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. This value is received from
* a previous `ListBidders` call in ListBiddersResponse.nextPageToken.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. This value is received from a
previous `ListBidders` call in ListBiddersResponse.nextPageToken.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. This value is received from
* a previous `ListBidders` call in ListBiddersResponse.nextPageToken.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Creatives collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.Creatives.List request = realtimebidding.creatives().list(parameters ...)}
*
*
* @return the resource collection
*/
public Creatives creatives() {
return new Creatives();
}
/**
* The "creatives" collection of methods.
*/
public class Creatives {
/**
* Lists creatives as they are at the time of the initial request. This call may take multiple hours
* to complete. For large, paginated requests, this method returns a snapshot of creatives at the
* time of request for the first page. `lastStatusUpdate` and `creativeServingDecision` may be
* outdated for creatives on sequential pages. We recommend [Google Cloud
* Pub/Sub](//cloud.google.com/pubsub/docs/overview) to view the latest status.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the parent buyer that owns the creatives. The pattern for this resource is either
* `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For `buyers/{buyerAccountId}`,
* the `buyerAccountId` can be one of the following: 1. The ID of the buyer that is accessing
* their own creatives. 2. The ID of the child seat buyer under a bidder account. So for
* listing creatives pertaining to the child seat buyer (`456`) under bidder account (`123`),
* you would use the pattern: `buyers/456`. 3. The ID of the bidder itself. So for listing
* creatives pertaining to bidder (`123`), you would use `buyers/123`. If you want to access
* all creatives pertaining to both the bidder and all of its child seat accounts, you would
* use `bidders/{bidderAccountId}`, for example, for all creatives pertaining to bidder
* (`123`), use `bidders/123`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/creatives";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Lists creatives as they are at the time of the initial request. This call may take multiple
* hours to complete. For large, paginated requests, this method returns a snapshot of creatives
* at the time of request for the first page. `lastStatusUpdate` and `creativeServingDecision` may
* be outdated for creatives on sequential pages. We recommend [Google Cloud
* Pub/Sub](//cloud.google.com/pubsub/docs/overview) to view the latest status.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the parent buyer that owns the creatives. The pattern for this resource is either
* `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For `buyers/{buyerAccountId}`,
* the `buyerAccountId` can be one of the following: 1. The ID of the buyer that is accessing
* their own creatives. 2. The ID of the child seat buyer under a bidder account. So for
* listing creatives pertaining to the child seat buyer (`456`) under bidder account (`123`),
* you would use the pattern: `buyers/456`. 3. The ID of the bidder itself. So for listing
* creatives pertaining to bidder (`123`), you would use `buyers/123`. If you want to access
* all creatives pertaining to both the bidder and all of its child seat accounts, you would
* use `bidders/{bidderAccountId}`, for example, for all creatives pertaining to bidder
* (`123`), use `bidders/123`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListCreativesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the parent buyer that owns the creatives. The pattern for this resource
* is either `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For
* `buyers/{buyerAccountId}`, the `buyerAccountId` can be one of the following: 1. The ID of
* the buyer that is accessing their own creatives. 2. The ID of the child seat buyer under
* a bidder account. So for listing creatives pertaining to the child seat buyer (`456`)
* under bidder account (`123`), you would use the pattern: `buyers/456`. 3. The ID of the
* bidder itself. So for listing creatives pertaining to bidder (`123`), you would use
* `buyers/123`. If you want to access all creatives pertaining to both the bidder and all
* of its child seat accounts, you would use `bidders/{bidderAccountId}`, for example, for
* all creatives pertaining to bidder (`123`), use `bidders/123`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the parent buyer that owns the creatives. The pattern for this resource is either
`buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For `buyers/{buyerAccountId}`, the
`buyerAccountId` can be one of the following: 1. The ID of the buyer that is accessing their own
creatives. 2. The ID of the child seat buyer under a bidder account. So for listing creatives
pertaining to the child seat buyer (`456`) under bidder account (`123`), you would use the pattern:
`buyers/456`. 3. The ID of the bidder itself. So for listing creatives pertaining to bidder
(`123`), you would use `buyers/123`. If you want to access all creatives pertaining to both the
bidder and all of its child seat accounts, you would use `bidders/{bidderAccountId}`, for example,
for all creatives pertaining to bidder (`123`), use `bidders/123`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the parent buyer that owns the creatives. The pattern for this resource
* is either `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For
* `buyers/{buyerAccountId}`, the `buyerAccountId` can be one of the following: 1. The ID of
* the buyer that is accessing their own creatives. 2. The ID of the child seat buyer under
* a bidder account. So for listing creatives pertaining to the child seat buyer (`456`)
* under bidder account (`123`), you would use the pattern: `buyers/456`. 3. The ID of the
* bidder itself. So for listing creatives pertaining to bidder (`123`), you would use
* `buyers/123`. If you want to access all creatives pertaining to both the bidder and all
* of its child seat accounts, you would use `bidders/{bidderAccountId}`, for example, for
* all creatives pertaining to bidder (`123`), use `bidders/123`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Query string to filter creatives. If no filter is specified, all active creatives will be
* returned. Example: 'accountId=12345 AND (dealsStatus:DISAPPROVED AND
* disapprovalReason:UNACCEPTABLE_CONTENT) OR declaredAttributes:IS_COOKIE_TARGETED'
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Query string to filter creatives. If no filter is specified, all active creatives will be returned.
Example: 'accountId=12345 AND (dealsStatus:DISAPPROVED AND disapprovalReason:UNACCEPTABLE_CONTENT)
OR declaredAttributes:IS_COOKIE_TARGETED'
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Query string to filter creatives. If no filter is specified, all active creatives will be
* returned. Example: 'accountId=12345 AND (dealsStatus:DISAPPROVED AND
* disapprovalReason:UNACCEPTABLE_CONTENT) OR declaredAttributes:IS_COOKIE_TARGETED'
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Requested page size. The server may return fewer creatives than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, server will
* pick an appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer creatives than requested (due to timeout
constraint) even if more are available through another call. If unspecified, server will pick an
appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer creatives than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, server will
* pick an appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListCreativesResponse.nextPageToken returned from the previous call to the
* 'ListCreatives' method. Page tokens for continued pages are valid for up to five hours,
* counting from the call to 'ListCreatives' for the first page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListCreativesResponse.nextPageToken returned from the previous call to the 'ListCreatives' method.
Page tokens for continued pages are valid for up to five hours, counting from the call to
'ListCreatives' for the first page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListCreativesResponse.nextPageToken returned from the previous call to the
* 'ListCreatives' method. Page tokens for continued pages are valid for up to five hours,
* counting from the call to 'ListCreatives' for the first page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Controls the amount of information included in the response. By default only
* creativeServingDecision is included. To retrieve the entire creative resource (including
* the declared fields and the creative content) specify the view as "FULL".
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Controls the amount of information included in the response. By default only
creativeServingDecision is included. To retrieve the entire creative resource (including the
declared fields and the creative content) specify the view as "FULL".
*/
public java.lang.String getView() {
return view;
}
/**
* Controls the amount of information included in the response. By default only
* creativeServingDecision is included. To retrieve the entire creative resource (including
* the declared fields and the creative content) specify the view as "FULL".
*/
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Watches all creatives pertaining to a bidder. It is sufficient to invoke this endpoint once per
* bidder. A Pub/Sub topic will be created and notifications will be pushed to the topic when any of
* the bidder's creatives change status. All of the bidder's service accounts will have access to
* read from the topic. Subsequent invocations of this method will return the existing Pub/Sub
* configuration.
*
* Create a request for the method "creatives.watch".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Watch#execute()} method to invoke the remote operation.
*
* @param parent Required. To watch all creatives pertaining to the bidder and all its child seat accounts, the
* bidder must follow the pattern `bidders/{bidderAccountId}`.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.WatchCreativesRequest}
* @return the request
*/
public Watch watch(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.WatchCreativesRequest content) throws java.io.IOException {
Watch result = new Watch(parent, content);
initialize(result);
return result;
}
public class Watch extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/creatives:watch";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Watches all creatives pertaining to a bidder. It is sufficient to invoke this endpoint once per
* bidder. A Pub/Sub topic will be created and notifications will be pushed to the topic when any
* of the bidder's creatives change status. All of the bidder's service accounts will have access
* to read from the topic. Subsequent invocations of this method will return the existing Pub/Sub
* configuration.
*
* Create a request for the method "creatives.watch".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Watch#execute()} method to invoke the remote operation.
* {@link
* Watch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. To watch all creatives pertaining to the bidder and all its child seat accounts, the
* bidder must follow the pattern `bidders/{bidderAccountId}`.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.WatchCreativesRequest}
* @since 1.13
*/
protected Watch(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.WatchCreativesRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.WatchCreativesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public Watch set$Xgafv(java.lang.String $Xgafv) {
return (Watch) super.set$Xgafv($Xgafv);
}
@Override
public Watch setAccessToken(java.lang.String accessToken) {
return (Watch) super.setAccessToken(accessToken);
}
@Override
public Watch setAlt(java.lang.String alt) {
return (Watch) super.setAlt(alt);
}
@Override
public Watch setCallback(java.lang.String callback) {
return (Watch) super.setCallback(callback);
}
@Override
public Watch setFields(java.lang.String fields) {
return (Watch) super.setFields(fields);
}
@Override
public Watch setKey(java.lang.String key) {
return (Watch) super.setKey(key);
}
@Override
public Watch setOauthToken(java.lang.String oauthToken) {
return (Watch) super.setOauthToken(oauthToken);
}
@Override
public Watch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Watch) super.setPrettyPrint(prettyPrint);
}
@Override
public Watch setQuotaUser(java.lang.String quotaUser) {
return (Watch) super.setQuotaUser(quotaUser);
}
@Override
public Watch setUploadType(java.lang.String uploadType) {
return (Watch) super.setUploadType(uploadType);
}
@Override
public Watch setUploadProtocol(java.lang.String uploadProtocol) {
return (Watch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. To watch all creatives pertaining to the bidder and all its child seat
* accounts, the bidder must follow the pattern `bidders/{bidderAccountId}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. To watch all creatives pertaining to the bidder and all its child seat accounts, the
bidder must follow the pattern `bidders/{bidderAccountId}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. To watch all creatives pertaining to the bidder and all its child seat
* accounts, the bidder must follow the pattern `bidders/{bidderAccountId}`.
*/
public Watch setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Watch set(String parameterName, Object value) {
return (Watch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Endpoints collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.Endpoints.List request = realtimebidding.endpoints().list(parameters ...)}
*
*
* @return the resource collection
*/
public Endpoints endpoints() {
return new Endpoints();
}
/**
* The "endpoints" collection of methods.
*/
public class Endpoints {
/**
* Gets a bidder endpoint by its name.
*
* Create a request for the method "endpoints.get".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the bidder endpoint to get. Format:
* `bidders/{bidderAccountId}/endpoints/{endpointId}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/endpoints/[^/]+$");
/**
* Gets a bidder endpoint by its name.
*
* Create a request for the method "endpoints.get".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the bidder endpoint to get. Format:
* `bidders/{bidderAccountId}/endpoints/{endpointId}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.Endpoint.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/endpoints/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the bidder endpoint to get. Format:
* `bidders/{bidderAccountId}/endpoints/{endpointId}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the bidder endpoint to get. Format:
`bidders/{bidderAccountId}/endpoints/{endpointId}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the bidder endpoint to get. Format:
* `bidders/{bidderAccountId}/endpoints/{endpointId}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/endpoints/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the bidder's endpoints.
*
* Create a request for the method "endpoints.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the bidder whose endpoints will be listed. Format: `bidders/{bidderAccountId}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/endpoints";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Lists all the bidder's endpoints.
*
* Create a request for the method "endpoints.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the bidder whose endpoints will be listed. Format: `bidders/{bidderAccountId}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListEndpointsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the bidder whose endpoints will be listed. Format:
* `bidders/{bidderAccountId}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the bidder whose endpoints will be listed. Format: `bidders/{bidderAccountId}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the bidder whose endpoints will be listed. Format:
* `bidders/{bidderAccountId}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of endpoints to return. If unspecified, at most 100 endpoints will be
* returned. The maximum value is 500; values above 500 will be coerced to 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of endpoints to return. If unspecified, at most 100 endpoints will be returned.
The maximum value is 500; values above 500 will be coerced to 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of endpoints to return. If unspecified, at most 100 endpoints will be
* returned. The maximum value is 500; values above 500 will be coerced to 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. This value is received
* from a previous `ListEndpoints` call in ListEndpointsResponse.nextPageToken.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. This value is received from a
previous `ListEndpoints` call in ListEndpointsResponse.nextPageToken.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. This value is received
* from a previous `ListEndpoints` call in ListEndpointsResponse.nextPageToken.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a bidder's endpoint.
*
* Create a request for the method "endpoints.patch".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Name of the endpoint resource that must follow the pattern
* `bidders/{bidderAccountId}/endpoints/{endpointId}`, where {bidderAccountId} is the account
* ID of the bidder who operates this endpoint, and {endpointId} is a unique ID assigned by
* the server.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.Endpoint}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.realtimebidding.v1.model.Endpoint content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/endpoints/[^/]+$");
/**
* Updates a bidder's endpoint.
*
* Create a request for the method "endpoints.patch".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Name of the endpoint resource that must follow the pattern
* `bidders/{bidderAccountId}/endpoints/{endpointId}`, where {bidderAccountId} is the account
* ID of the bidder who operates this endpoint, and {endpointId} is a unique ID assigned by
* the server.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.Endpoint}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.realtimebidding.v1.model.Endpoint content) {
super(RealTimeBidding.this, "PATCH", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.Endpoint.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/endpoints/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Name of the endpoint resource that must follow the pattern
* `bidders/{bidderAccountId}/endpoints/{endpointId}`, where {bidderAccountId} is the
* account ID of the bidder who operates this endpoint, and {endpointId} is a unique ID
* assigned by the server.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Name of the endpoint resource that must follow the pattern
`bidders/{bidderAccountId}/endpoints/{endpointId}`, where {bidderAccountId} is the account ID of
the bidder who operates this endpoint, and {endpointId} is a unique ID assigned by the server.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Name of the endpoint resource that must follow the pattern
* `bidders/{bidderAccountId}/endpoints/{endpointId}`, where {bidderAccountId} is the
* account ID of the bidder who operates this endpoint, and {endpointId} is a unique ID
* assigned by the server.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/endpoints/[^/]+$");
}
this.name = name;
return this;
}
/** Field mask to use for partial in-place updates. */
@com.google.api.client.util.Key
private String updateMask;
/** Field mask to use for partial in-place updates.
*/
public String getUpdateMask() {
return updateMask;
}
/** Field mask to use for partial in-place updates. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the PretargetingConfigs collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.PretargetingConfigs.List request = realtimebidding.pretargetingConfigs().list(parameters ...)}
*
*
* @return the resource collection
*/
public PretargetingConfigs pretargetingConfigs() {
return new PretargetingConfigs();
}
/**
* The "pretargetingConfigs" collection of methods.
*/
public class PretargetingConfigs {
/**
* Activates a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.activate".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Activate#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.ActivatePretargetingConfigRequest}
* @return the request
*/
public Activate activate(java.lang.String name, com.google.api.services.realtimebidding.v1.model.ActivatePretargetingConfigRequest content) throws java.io.IOException {
Activate result = new Activate(name, content);
initialize(result);
return result;
}
public class Activate extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}:activate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Activates a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.activate".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Activate#execute()} method to invoke the remote operation.
* {@link
* Activate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.ActivatePretargetingConfigRequest}
* @since 1.13
*/
protected Activate(java.lang.String name, com.google.api.services.realtimebidding.v1.model.ActivatePretargetingConfigRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public Activate set$Xgafv(java.lang.String $Xgafv) {
return (Activate) super.set$Xgafv($Xgafv);
}
@Override
public Activate setAccessToken(java.lang.String accessToken) {
return (Activate) super.setAccessToken(accessToken);
}
@Override
public Activate setAlt(java.lang.String alt) {
return (Activate) super.setAlt(alt);
}
@Override
public Activate setCallback(java.lang.String callback) {
return (Activate) super.setCallback(callback);
}
@Override
public Activate setFields(java.lang.String fields) {
return (Activate) super.setFields(fields);
}
@Override
public Activate setKey(java.lang.String key) {
return (Activate) super.setKey(key);
}
@Override
public Activate setOauthToken(java.lang.String oauthToken) {
return (Activate) super.setOauthToken(oauthToken);
}
@Override
public Activate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Activate) super.setPrettyPrint(prettyPrint);
}
@Override
public Activate setQuotaUser(java.lang.String quotaUser) {
return (Activate) super.setQuotaUser(quotaUser);
}
@Override
public Activate setUploadType(java.lang.String uploadType) {
return (Activate) super.setUploadType(uploadType);
}
@Override
public Activate setUploadProtocol(java.lang.String uploadProtocol) {
return (Activate) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public Activate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Activate set(String parameterName, Object value) {
return (Activate) super.set(parameterName, value);
}
}
/**
* Adds targeted apps to the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.addTargetedApps".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link AddTargetedApps#execute()} method to invoke the remote
* operation.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.AddTargetedAppsRequest}
* @return the request
*/
public AddTargetedApps addTargetedApps(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.AddTargetedAppsRequest content) throws java.io.IOException {
AddTargetedApps result = new AddTargetedApps(pretargetingConfig, content);
initialize(result);
return result;
}
public class AddTargetedApps extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+pretargetingConfig}:addTargetedApps";
private final java.util.regex.Pattern PRETARGETING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Adds targeted apps to the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.addTargetedApps".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link AddTargetedApps#execute()} method to invoke the remote
* operation. {@link AddTargetedApps#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.AddTargetedAppsRequest}
* @since 1.13
*/
protected AddTargetedApps(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.AddTargetedAppsRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.pretargetingConfig = com.google.api.client.util.Preconditions.checkNotNull(pretargetingConfig, "Required parameter pretargetingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public AddTargetedApps set$Xgafv(java.lang.String $Xgafv) {
return (AddTargetedApps) super.set$Xgafv($Xgafv);
}
@Override
public AddTargetedApps setAccessToken(java.lang.String accessToken) {
return (AddTargetedApps) super.setAccessToken(accessToken);
}
@Override
public AddTargetedApps setAlt(java.lang.String alt) {
return (AddTargetedApps) super.setAlt(alt);
}
@Override
public AddTargetedApps setCallback(java.lang.String callback) {
return (AddTargetedApps) super.setCallback(callback);
}
@Override
public AddTargetedApps setFields(java.lang.String fields) {
return (AddTargetedApps) super.setFields(fields);
}
@Override
public AddTargetedApps setKey(java.lang.String key) {
return (AddTargetedApps) super.setKey(key);
}
@Override
public AddTargetedApps setOauthToken(java.lang.String oauthToken) {
return (AddTargetedApps) super.setOauthToken(oauthToken);
}
@Override
public AddTargetedApps setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddTargetedApps) super.setPrettyPrint(prettyPrint);
}
@Override
public AddTargetedApps setQuotaUser(java.lang.String quotaUser) {
return (AddTargetedApps) super.setQuotaUser(quotaUser);
}
@Override
public AddTargetedApps setUploadType(java.lang.String uploadType) {
return (AddTargetedApps) super.setUploadType(uploadType);
}
@Override
public AddTargetedApps setUploadProtocol(java.lang.String uploadProtocol) {
return (AddTargetedApps) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String pretargetingConfig;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getPretargetingConfig() {
return pretargetingConfig;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public AddTargetedApps setPretargetingConfig(java.lang.String pretargetingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.pretargetingConfig = pretargetingConfig;
return this;
}
@Override
public AddTargetedApps set(String parameterName, Object value) {
return (AddTargetedApps) super.set(parameterName, value);
}
}
/**
* Adds targeted publishers to the pretargeting config.
*
* Create a request for the method "pretargetingConfigs.addTargetedPublishers".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link AddTargetedPublishers#execute()} method to invoke the remote
* operation.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.AddTargetedPublishersRequest}
* @return the request
*/
public AddTargetedPublishers addTargetedPublishers(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.AddTargetedPublishersRequest content) throws java.io.IOException {
AddTargetedPublishers result = new AddTargetedPublishers(pretargetingConfig, content);
initialize(result);
return result;
}
public class AddTargetedPublishers extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+pretargetingConfig}:addTargetedPublishers";
private final java.util.regex.Pattern PRETARGETING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Adds targeted publishers to the pretargeting config.
*
* Create a request for the method "pretargetingConfigs.addTargetedPublishers".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link AddTargetedPublishers#execute()} method to invoke the
* remote operation. {@link AddTargetedPublishers#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.AddTargetedPublishersRequest}
* @since 1.13
*/
protected AddTargetedPublishers(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.AddTargetedPublishersRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.pretargetingConfig = com.google.api.client.util.Preconditions.checkNotNull(pretargetingConfig, "Required parameter pretargetingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public AddTargetedPublishers set$Xgafv(java.lang.String $Xgafv) {
return (AddTargetedPublishers) super.set$Xgafv($Xgafv);
}
@Override
public AddTargetedPublishers setAccessToken(java.lang.String accessToken) {
return (AddTargetedPublishers) super.setAccessToken(accessToken);
}
@Override
public AddTargetedPublishers setAlt(java.lang.String alt) {
return (AddTargetedPublishers) super.setAlt(alt);
}
@Override
public AddTargetedPublishers setCallback(java.lang.String callback) {
return (AddTargetedPublishers) super.setCallback(callback);
}
@Override
public AddTargetedPublishers setFields(java.lang.String fields) {
return (AddTargetedPublishers) super.setFields(fields);
}
@Override
public AddTargetedPublishers setKey(java.lang.String key) {
return (AddTargetedPublishers) super.setKey(key);
}
@Override
public AddTargetedPublishers setOauthToken(java.lang.String oauthToken) {
return (AddTargetedPublishers) super.setOauthToken(oauthToken);
}
@Override
public AddTargetedPublishers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddTargetedPublishers) super.setPrettyPrint(prettyPrint);
}
@Override
public AddTargetedPublishers setQuotaUser(java.lang.String quotaUser) {
return (AddTargetedPublishers) super.setQuotaUser(quotaUser);
}
@Override
public AddTargetedPublishers setUploadType(java.lang.String uploadType) {
return (AddTargetedPublishers) super.setUploadType(uploadType);
}
@Override
public AddTargetedPublishers setUploadProtocol(java.lang.String uploadProtocol) {
return (AddTargetedPublishers) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String pretargetingConfig;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getPretargetingConfig() {
return pretargetingConfig;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public AddTargetedPublishers setPretargetingConfig(java.lang.String pretargetingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.pretargetingConfig = pretargetingConfig;
return this;
}
@Override
public AddTargetedPublishers set(String parameterName, Object value) {
return (AddTargetedPublishers) super.set(parameterName, value);
}
}
/**
* Adds targeted sites to the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.addTargetedSites".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link AddTargetedSites#execute()} method to invoke the remote
* operation.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.AddTargetedSitesRequest}
* @return the request
*/
public AddTargetedSites addTargetedSites(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.AddTargetedSitesRequest content) throws java.io.IOException {
AddTargetedSites result = new AddTargetedSites(pretargetingConfig, content);
initialize(result);
return result;
}
public class AddTargetedSites extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+pretargetingConfig}:addTargetedSites";
private final java.util.regex.Pattern PRETARGETING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Adds targeted sites to the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.addTargetedSites".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link AddTargetedSites#execute()} method to invoke the remote
* operation. {@link AddTargetedSites#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.AddTargetedSitesRequest}
* @since 1.13
*/
protected AddTargetedSites(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.AddTargetedSitesRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.pretargetingConfig = com.google.api.client.util.Preconditions.checkNotNull(pretargetingConfig, "Required parameter pretargetingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public AddTargetedSites set$Xgafv(java.lang.String $Xgafv) {
return (AddTargetedSites) super.set$Xgafv($Xgafv);
}
@Override
public AddTargetedSites setAccessToken(java.lang.String accessToken) {
return (AddTargetedSites) super.setAccessToken(accessToken);
}
@Override
public AddTargetedSites setAlt(java.lang.String alt) {
return (AddTargetedSites) super.setAlt(alt);
}
@Override
public AddTargetedSites setCallback(java.lang.String callback) {
return (AddTargetedSites) super.setCallback(callback);
}
@Override
public AddTargetedSites setFields(java.lang.String fields) {
return (AddTargetedSites) super.setFields(fields);
}
@Override
public AddTargetedSites setKey(java.lang.String key) {
return (AddTargetedSites) super.setKey(key);
}
@Override
public AddTargetedSites setOauthToken(java.lang.String oauthToken) {
return (AddTargetedSites) super.setOauthToken(oauthToken);
}
@Override
public AddTargetedSites setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddTargetedSites) super.setPrettyPrint(prettyPrint);
}
@Override
public AddTargetedSites setQuotaUser(java.lang.String quotaUser) {
return (AddTargetedSites) super.setQuotaUser(quotaUser);
}
@Override
public AddTargetedSites setUploadType(java.lang.String uploadType) {
return (AddTargetedSites) super.setUploadType(uploadType);
}
@Override
public AddTargetedSites setUploadProtocol(java.lang.String uploadProtocol) {
return (AddTargetedSites) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String pretargetingConfig;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getPretargetingConfig() {
return pretargetingConfig;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public AddTargetedSites setPretargetingConfig(java.lang.String pretargetingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.pretargetingConfig = pretargetingConfig;
return this;
}
@Override
public AddTargetedSites set(String parameterName, Object value) {
return (AddTargetedSites) super.set(parameterName, value);
}
}
/**
* Creates a pretargeting configuration. A pretargeting configuration's state
* (PretargetingConfig.state) is active upon creation, and it will start to affect traffic shortly
* after. A bidder may create a maximum of 10 pretargeting configurations. Attempts to exceed this
* maximum results in a 400 bad request error.
*
* Create a request for the method "pretargetingConfigs.create".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the bidder to create the pretargeting configuration for. Format:
* bidders/{bidderAccountId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.PretargetingConfig}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.PretargetingConfig content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/pretargetingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Creates a pretargeting configuration. A pretargeting configuration's state
* (PretargetingConfig.state) is active upon creation, and it will start to affect traffic shortly
* after. A bidder may create a maximum of 10 pretargeting configurations. Attempts to exceed this
* maximum results in a 400 bad request error.
*
* Create a request for the method "pretargetingConfigs.create".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the bidder to create the pretargeting configuration for. Format:
* bidders/{bidderAccountId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.PretargetingConfig}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.PretargetingConfig content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the bidder to create the pretargeting configuration for. Format:
* bidders/{bidderAccountId}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the bidder to create the pretargeting configuration for. Format:
bidders/{bidderAccountId}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the bidder to create the pretargeting configuration for. Format:
* bidders/{bidderAccountId}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.delete".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the pretargeting configuration to delete. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Deletes a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.delete".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the pretargeting configuration to delete. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(RealTimeBidding.this, "DELETE", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration to delete. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the pretargeting configuration to delete. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the pretargeting configuration to delete. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.get".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the pretargeting configuration to get. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Gets a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.get".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the pretargeting configuration to get. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the pretargeting configuration to get. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the pretargeting configuration to get. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the pretargeting configuration to get. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all pretargeting configurations for a single bidder.
*
* Create a request for the method "pretargetingConfigs.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the bidder whose pretargeting configurations will be listed. Format:
* bidders/{bidderAccountId}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/pretargetingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Lists all pretargeting configurations for a single bidder.
*
* Create a request for the method "pretargetingConfigs.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the bidder whose pretargeting configurations will be listed. Format:
* bidders/{bidderAccountId}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListPretargetingConfigsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the bidder whose pretargeting configurations will be listed. Format:
* bidders/{bidderAccountId}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the bidder whose pretargeting configurations will be listed. Format:
bidders/{bidderAccountId}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the bidder whose pretargeting configurations will be listed. Format:
* bidders/{bidderAccountId}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of pretargeting configurations to return. If unspecified, at most 10
* pretargeting configurations will be returned. The maximum value is 100; values above 100
* will be coerced to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of pretargeting configurations to return. If unspecified, at most 10
pretargeting configurations will be returned. The maximum value is 100; values above 100 will be
coerced to 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of pretargeting configurations to return. If unspecified, at most 10
* pretargeting configurations will be returned. The maximum value is 100; values above 100
* will be coerced to 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. This value is received
* from a previous `ListPretargetingConfigs` call in
* ListPretargetingConfigsResponse.nextPageToken.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. This value is received from a
previous `ListPretargetingConfigs` call in ListPretargetingConfigsResponse.nextPageToken.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. This value is received
* from a previous `ListPretargetingConfigs` call in
* ListPretargetingConfigsResponse.nextPageToken.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.patch".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Name of the pretargeting configuration that must follow the pattern
* `bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
* @param content the {@link com.google.api.services.realtimebidding.v1.model.PretargetingConfig}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.realtimebidding.v1.model.PretargetingConfig content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Updates a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.patch".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Name of the pretargeting configuration that must follow the pattern
* `bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
* @param content the {@link com.google.api.services.realtimebidding.v1.model.PretargetingConfig}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.realtimebidding.v1.model.PretargetingConfig content) {
super(RealTimeBidding.this, "PATCH", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Name of the pretargeting configuration that must follow the pattern
* `bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Name of the pretargeting configuration that must follow the pattern
`bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Name of the pretargeting configuration that must follow the pattern
* `bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.name = name;
return this;
}
/** Field mask to use for partial in-place updates. */
@com.google.api.client.util.Key
private String updateMask;
/** Field mask to use for partial in-place updates.
*/
public String getUpdateMask() {
return updateMask;
}
/** Field mask to use for partial in-place updates. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Removes targeted apps from the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.removeTargetedApps".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link RemoveTargetedApps#execute()} method to invoke the remote
* operation.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.RemoveTargetedAppsRequest}
* @return the request
*/
public RemoveTargetedApps removeTargetedApps(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.RemoveTargetedAppsRequest content) throws java.io.IOException {
RemoveTargetedApps result = new RemoveTargetedApps(pretargetingConfig, content);
initialize(result);
return result;
}
public class RemoveTargetedApps extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+pretargetingConfig}:removeTargetedApps";
private final java.util.regex.Pattern PRETARGETING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Removes targeted apps from the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.removeTargetedApps".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link RemoveTargetedApps#execute()} method to invoke the remote
* operation. {@link RemoveTargetedApps#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.RemoveTargetedAppsRequest}
* @since 1.13
*/
protected RemoveTargetedApps(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.RemoveTargetedAppsRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.pretargetingConfig = com.google.api.client.util.Preconditions.checkNotNull(pretargetingConfig, "Required parameter pretargetingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public RemoveTargetedApps set$Xgafv(java.lang.String $Xgafv) {
return (RemoveTargetedApps) super.set$Xgafv($Xgafv);
}
@Override
public RemoveTargetedApps setAccessToken(java.lang.String accessToken) {
return (RemoveTargetedApps) super.setAccessToken(accessToken);
}
@Override
public RemoveTargetedApps setAlt(java.lang.String alt) {
return (RemoveTargetedApps) super.setAlt(alt);
}
@Override
public RemoveTargetedApps setCallback(java.lang.String callback) {
return (RemoveTargetedApps) super.setCallback(callback);
}
@Override
public RemoveTargetedApps setFields(java.lang.String fields) {
return (RemoveTargetedApps) super.setFields(fields);
}
@Override
public RemoveTargetedApps setKey(java.lang.String key) {
return (RemoveTargetedApps) super.setKey(key);
}
@Override
public RemoveTargetedApps setOauthToken(java.lang.String oauthToken) {
return (RemoveTargetedApps) super.setOauthToken(oauthToken);
}
@Override
public RemoveTargetedApps setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveTargetedApps) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveTargetedApps setQuotaUser(java.lang.String quotaUser) {
return (RemoveTargetedApps) super.setQuotaUser(quotaUser);
}
@Override
public RemoveTargetedApps setUploadType(java.lang.String uploadType) {
return (RemoveTargetedApps) super.setUploadType(uploadType);
}
@Override
public RemoveTargetedApps setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveTargetedApps) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String pretargetingConfig;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getPretargetingConfig() {
return pretargetingConfig;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public RemoveTargetedApps setPretargetingConfig(java.lang.String pretargetingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.pretargetingConfig = pretargetingConfig;
return this;
}
@Override
public RemoveTargetedApps set(String parameterName, Object value) {
return (RemoveTargetedApps) super.set(parameterName, value);
}
}
/**
* Removes targeted publishers from the pretargeting config.
*
* Create a request for the method "pretargetingConfigs.removeTargetedPublishers".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link RemoveTargetedPublishers#execute()} method to invoke the
* remote operation.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.RemoveTargetedPublishersRequest}
* @return the request
*/
public RemoveTargetedPublishers removeTargetedPublishers(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.RemoveTargetedPublishersRequest content) throws java.io.IOException {
RemoveTargetedPublishers result = new RemoveTargetedPublishers(pretargetingConfig, content);
initialize(result);
return result;
}
public class RemoveTargetedPublishers extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+pretargetingConfig}:removeTargetedPublishers";
private final java.util.regex.Pattern PRETARGETING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Removes targeted publishers from the pretargeting config.
*
* Create a request for the method "pretargetingConfigs.removeTargetedPublishers".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link RemoveTargetedPublishers#execute()} method to invoke the
* remote operation. {@link RemoveTargetedPublishers#initialize(com.google.api.client.googleap
* is.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.RemoveTargetedPublishersRequest}
* @since 1.13
*/
protected RemoveTargetedPublishers(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.RemoveTargetedPublishersRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.pretargetingConfig = com.google.api.client.util.Preconditions.checkNotNull(pretargetingConfig, "Required parameter pretargetingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public RemoveTargetedPublishers set$Xgafv(java.lang.String $Xgafv) {
return (RemoveTargetedPublishers) super.set$Xgafv($Xgafv);
}
@Override
public RemoveTargetedPublishers setAccessToken(java.lang.String accessToken) {
return (RemoveTargetedPublishers) super.setAccessToken(accessToken);
}
@Override
public RemoveTargetedPublishers setAlt(java.lang.String alt) {
return (RemoveTargetedPublishers) super.setAlt(alt);
}
@Override
public RemoveTargetedPublishers setCallback(java.lang.String callback) {
return (RemoveTargetedPublishers) super.setCallback(callback);
}
@Override
public RemoveTargetedPublishers setFields(java.lang.String fields) {
return (RemoveTargetedPublishers) super.setFields(fields);
}
@Override
public RemoveTargetedPublishers setKey(java.lang.String key) {
return (RemoveTargetedPublishers) super.setKey(key);
}
@Override
public RemoveTargetedPublishers setOauthToken(java.lang.String oauthToken) {
return (RemoveTargetedPublishers) super.setOauthToken(oauthToken);
}
@Override
public RemoveTargetedPublishers setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveTargetedPublishers) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveTargetedPublishers setQuotaUser(java.lang.String quotaUser) {
return (RemoveTargetedPublishers) super.setQuotaUser(quotaUser);
}
@Override
public RemoveTargetedPublishers setUploadType(java.lang.String uploadType) {
return (RemoveTargetedPublishers) super.setUploadType(uploadType);
}
@Override
public RemoveTargetedPublishers setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveTargetedPublishers) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String pretargetingConfig;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getPretargetingConfig() {
return pretargetingConfig;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public RemoveTargetedPublishers setPretargetingConfig(java.lang.String pretargetingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.pretargetingConfig = pretargetingConfig;
return this;
}
@Override
public RemoveTargetedPublishers set(String parameterName, Object value) {
return (RemoveTargetedPublishers) super.set(parameterName, value);
}
}
/**
* Removes targeted sites from the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.removeTargetedSites".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link RemoveTargetedSites#execute()} method to invoke the remote
* operation.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.RemoveTargetedSitesRequest}
* @return the request
*/
public RemoveTargetedSites removeTargetedSites(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.RemoveTargetedSitesRequest content) throws java.io.IOException {
RemoveTargetedSites result = new RemoveTargetedSites(pretargetingConfig, content);
initialize(result);
return result;
}
public class RemoveTargetedSites extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+pretargetingConfig}:removeTargetedSites";
private final java.util.regex.Pattern PRETARGETING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Removes targeted sites from the pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.removeTargetedSites".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link RemoveTargetedSites#execute()} method to invoke the remote
* operation. {@link RemoveTargetedSites#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param pretargetingConfig Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.RemoveTargetedSitesRequest}
* @since 1.13
*/
protected RemoveTargetedSites(java.lang.String pretargetingConfig, com.google.api.services.realtimebidding.v1.model.RemoveTargetedSitesRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.pretargetingConfig = com.google.api.client.util.Preconditions.checkNotNull(pretargetingConfig, "Required parameter pretargetingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public RemoveTargetedSites set$Xgafv(java.lang.String $Xgafv) {
return (RemoveTargetedSites) super.set$Xgafv($Xgafv);
}
@Override
public RemoveTargetedSites setAccessToken(java.lang.String accessToken) {
return (RemoveTargetedSites) super.setAccessToken(accessToken);
}
@Override
public RemoveTargetedSites setAlt(java.lang.String alt) {
return (RemoveTargetedSites) super.setAlt(alt);
}
@Override
public RemoveTargetedSites setCallback(java.lang.String callback) {
return (RemoveTargetedSites) super.setCallback(callback);
}
@Override
public RemoveTargetedSites setFields(java.lang.String fields) {
return (RemoveTargetedSites) super.setFields(fields);
}
@Override
public RemoveTargetedSites setKey(java.lang.String key) {
return (RemoveTargetedSites) super.setKey(key);
}
@Override
public RemoveTargetedSites setOauthToken(java.lang.String oauthToken) {
return (RemoveTargetedSites) super.setOauthToken(oauthToken);
}
@Override
public RemoveTargetedSites setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveTargetedSites) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveTargetedSites setQuotaUser(java.lang.String quotaUser) {
return (RemoveTargetedSites) super.setQuotaUser(quotaUser);
}
@Override
public RemoveTargetedSites setUploadType(java.lang.String uploadType) {
return (RemoveTargetedSites) super.setUploadType(uploadType);
}
@Override
public RemoveTargetedSites setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveTargetedSites) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String pretargetingConfig;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getPretargetingConfig() {
return pretargetingConfig;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public RemoveTargetedSites setPretargetingConfig(java.lang.String pretargetingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRETARGETING_CONFIG_PATTERN.matcher(pretargetingConfig).matches(),
"Parameter pretargetingConfig must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.pretargetingConfig = pretargetingConfig;
return this;
}
@Override
public RemoveTargetedSites set(String parameterName, Object value) {
return (RemoveTargetedSites) super.set(parameterName, value);
}
}
/**
* Suspends a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.suspend".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Suspend#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.SuspendPretargetingConfigRequest}
* @return the request
*/
public Suspend suspend(java.lang.String name, com.google.api.services.realtimebidding.v1.model.SuspendPretargetingConfigRequest content) throws java.io.IOException {
Suspend result = new Suspend(name, content);
initialize(result);
return result;
}
public class Suspend extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}:suspend";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/pretargetingConfigs/[^/]+$");
/**
* Suspends a pretargeting configuration.
*
* Create a request for the method "pretargetingConfigs.suspend".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Suspend#execute()} method to invoke the remote operation.
* {@link
* Suspend#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
* @param content the {@link com.google.api.services.realtimebidding.v1.model.SuspendPretargetingConfigRequest}
* @since 1.13
*/
protected Suspend(java.lang.String name, com.google.api.services.realtimebidding.v1.model.SuspendPretargetingConfigRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.PretargetingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
}
@Override
public Suspend set$Xgafv(java.lang.String $Xgafv) {
return (Suspend) super.set$Xgafv($Xgafv);
}
@Override
public Suspend setAccessToken(java.lang.String accessToken) {
return (Suspend) super.setAccessToken(accessToken);
}
@Override
public Suspend setAlt(java.lang.String alt) {
return (Suspend) super.setAlt(alt);
}
@Override
public Suspend setCallback(java.lang.String callback) {
return (Suspend) super.setCallback(callback);
}
@Override
public Suspend setFields(java.lang.String fields) {
return (Suspend) super.setFields(fields);
}
@Override
public Suspend setKey(java.lang.String key) {
return (Suspend) super.setKey(key);
}
@Override
public Suspend setOauthToken(java.lang.String oauthToken) {
return (Suspend) super.setOauthToken(oauthToken);
}
@Override
public Suspend setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Suspend) super.setPrettyPrint(prettyPrint);
}
@Override
public Suspend setQuotaUser(java.lang.String quotaUser) {
return (Suspend) super.setQuotaUser(quotaUser);
}
@Override
public Suspend setUploadType(java.lang.String uploadType) {
return (Suspend) super.setUploadType(uploadType);
}
@Override
public Suspend setUploadProtocol(java.lang.String uploadProtocol) {
return (Suspend) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the pretargeting configuration. Format:
bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the pretargeting configuration. Format:
* bidders/{bidderAccountId}/pretargetingConfig/{configId}
*/
public Suspend setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/pretargetingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Suspend set(String parameterName, Object value) {
return (Suspend) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the PublisherConnections collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.PublisherConnections.List request = realtimebidding.publisherConnections().list(parameters ...)}
*
*
* @return the resource collection
*/
public PublisherConnections publisherConnections() {
return new PublisherConnections();
}
/**
* The "publisherConnections" collection of methods.
*/
public class PublisherConnections {
/**
* Batch approves multiple publisher connections.
*
* Create a request for the method "publisherConnections.batchApprove".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link BatchApprove#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The bidder for whom publisher connections will be approved. Format: `bidders/{bidder}`
* where `{bidder}` is the account ID of the bidder.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.BatchApprovePublisherConnectionsRequest}
* @return the request
*/
public BatchApprove batchApprove(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.BatchApprovePublisherConnectionsRequest content) throws java.io.IOException {
BatchApprove result = new BatchApprove(parent, content);
initialize(result);
return result;
}
public class BatchApprove extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/publisherConnections:batchApprove";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Batch approves multiple publisher connections.
*
* Create a request for the method "publisherConnections.batchApprove".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link BatchApprove#execute()} method to invoke the remote
* operation. {@link
* BatchApprove#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bidder for whom publisher connections will be approved. Format: `bidders/{bidder}`
* where `{bidder}` is the account ID of the bidder.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.BatchApprovePublisherConnectionsRequest}
* @since 1.13
*/
protected BatchApprove(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.BatchApprovePublisherConnectionsRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.BatchApprovePublisherConnectionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public BatchApprove set$Xgafv(java.lang.String $Xgafv) {
return (BatchApprove) super.set$Xgafv($Xgafv);
}
@Override
public BatchApprove setAccessToken(java.lang.String accessToken) {
return (BatchApprove) super.setAccessToken(accessToken);
}
@Override
public BatchApprove setAlt(java.lang.String alt) {
return (BatchApprove) super.setAlt(alt);
}
@Override
public BatchApprove setCallback(java.lang.String callback) {
return (BatchApprove) super.setCallback(callback);
}
@Override
public BatchApprove setFields(java.lang.String fields) {
return (BatchApprove) super.setFields(fields);
}
@Override
public BatchApprove setKey(java.lang.String key) {
return (BatchApprove) super.setKey(key);
}
@Override
public BatchApprove setOauthToken(java.lang.String oauthToken) {
return (BatchApprove) super.setOauthToken(oauthToken);
}
@Override
public BatchApprove setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchApprove) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchApprove setQuotaUser(java.lang.String quotaUser) {
return (BatchApprove) super.setQuotaUser(quotaUser);
}
@Override
public BatchApprove setUploadType(java.lang.String uploadType) {
return (BatchApprove) super.setUploadType(uploadType);
}
@Override
public BatchApprove setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchApprove) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bidder for whom publisher connections will be approved. Format:
* `bidders/{bidder}` where `{bidder}` is the account ID of the bidder.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bidder for whom publisher connections will be approved. Format: `bidders/{bidder}`
where `{bidder}` is the account ID of the bidder.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bidder for whom publisher connections will be approved. Format:
* `bidders/{bidder}` where `{bidder}` is the account ID of the bidder.
*/
public BatchApprove setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchApprove set(String parameterName, Object value) {
return (BatchApprove) super.set(parameterName, value);
}
}
/**
* Batch rejects multiple publisher connections.
*
* Create a request for the method "publisherConnections.batchReject".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link BatchReject#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The bidder for whom publisher connections will be rejected. Format: `bidders/{bidder}`
* where `{bidder}` is the account ID of the bidder.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.BatchRejectPublisherConnectionsRequest}
* @return the request
*/
public BatchReject batchReject(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.BatchRejectPublisherConnectionsRequest content) throws java.io.IOException {
BatchReject result = new BatchReject(parent, content);
initialize(result);
return result;
}
public class BatchReject extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/publisherConnections:batchReject";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Batch rejects multiple publisher connections.
*
* Create a request for the method "publisherConnections.batchReject".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link BatchReject#execute()} method to invoke the remote
* operation. {@link
* BatchReject#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The bidder for whom publisher connections will be rejected. Format: `bidders/{bidder}`
* where `{bidder}` is the account ID of the bidder.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.BatchRejectPublisherConnectionsRequest}
* @since 1.13
*/
protected BatchReject(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.BatchRejectPublisherConnectionsRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.BatchRejectPublisherConnectionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public BatchReject set$Xgafv(java.lang.String $Xgafv) {
return (BatchReject) super.set$Xgafv($Xgafv);
}
@Override
public BatchReject setAccessToken(java.lang.String accessToken) {
return (BatchReject) super.setAccessToken(accessToken);
}
@Override
public BatchReject setAlt(java.lang.String alt) {
return (BatchReject) super.setAlt(alt);
}
@Override
public BatchReject setCallback(java.lang.String callback) {
return (BatchReject) super.setCallback(callback);
}
@Override
public BatchReject setFields(java.lang.String fields) {
return (BatchReject) super.setFields(fields);
}
@Override
public BatchReject setKey(java.lang.String key) {
return (BatchReject) super.setKey(key);
}
@Override
public BatchReject setOauthToken(java.lang.String oauthToken) {
return (BatchReject) super.setOauthToken(oauthToken);
}
@Override
public BatchReject setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchReject) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchReject setQuotaUser(java.lang.String quotaUser) {
return (BatchReject) super.setQuotaUser(quotaUser);
}
@Override
public BatchReject setUploadType(java.lang.String uploadType) {
return (BatchReject) super.setUploadType(uploadType);
}
@Override
public BatchReject setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchReject) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The bidder for whom publisher connections will be rejected. Format:
* `bidders/{bidder}` where `{bidder}` is the account ID of the bidder.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The bidder for whom publisher connections will be rejected. Format: `bidders/{bidder}`
where `{bidder}` is the account ID of the bidder.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The bidder for whom publisher connections will be rejected. Format:
* `bidders/{bidder}` where `{bidder}` is the account ID of the bidder.
*/
public BatchReject setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchReject set(String parameterName, Object value) {
return (BatchReject) super.set(parameterName, value);
}
}
/**
* Gets a publisher connection.
*
* Create a request for the method "publisherConnections.get".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the publisher whose connection information is to be retrieved. In the pattern
* `bidders/{bidder}/publisherConnections/{publisher}` where `{bidder}` is the account ID of
* the bidder, and `{publisher}` is the ads.txt/app-ads.txt publisher ID. See
* publisherConnection.name.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/publisherConnections/[^/]+$");
/**
* Gets a publisher connection.
*
* Create a request for the method "publisherConnections.get".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the publisher whose connection information is to be retrieved. In the pattern
* `bidders/{bidder}/publisherConnections/{publisher}` where `{bidder}` is the account ID of
* the bidder, and `{publisher}` is the ads.txt/app-ads.txt publisher ID. See
* publisherConnection.name.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.PublisherConnection.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/publisherConnections/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the publisher whose connection information is to be retrieved. In the
* pattern `bidders/{bidder}/publisherConnections/{publisher}` where `{bidder}` is the
* account ID of the bidder, and `{publisher}` is the ads.txt/app-ads.txt publisher ID. See
* publisherConnection.name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the publisher whose connection information is to be retrieved. In the pattern
`bidders/{bidder}/publisherConnections/{publisher}` where `{bidder}` is the account ID of the
bidder, and `{publisher}` is the ads.txt/app-ads.txt publisher ID. See publisherConnection.name.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the publisher whose connection information is to be retrieved. In the
* pattern `bidders/{bidder}/publisherConnections/{publisher}` where `{bidder}` is the
* account ID of the bidder, and `{publisher}` is the ads.txt/app-ads.txt publisher ID. See
* publisherConnection.name.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/publisherConnections/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists publisher connections for a given bidder.
*
* Create a request for the method "publisherConnections.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the bidder for which publishers have initiated connections. The pattern for this
* resource is `bidders/{bidder}` where `{bidder}` represents the account ID of the bidder.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/publisherConnections";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Lists publisher connections for a given bidder.
*
* Create a request for the method "publisherConnections.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the bidder for which publishers have initiated connections. The pattern for this
* resource is `bidders/{bidder}` where `{bidder}` represents the account ID of the bidder.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListPublisherConnectionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the bidder for which publishers have initiated connections. The pattern
* for this resource is `bidders/{bidder}` where `{bidder}` represents the account ID of the
* bidder.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the bidder for which publishers have initiated connections. The pattern for this
resource is `bidders/{bidder}` where `{bidder}` represents the account ID of the bidder.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the bidder for which publishers have initiated connections. The pattern
* for this resource is `bidders/{bidder}` where `{bidder}` represents the account ID of the
* bidder.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^bidders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Query string to filter publisher connections. Connections can be filtered by
* `displayName`, `publisherPlatform`, and `biddingState`. If no filter is specified, all
* publisher connections will be returned. Example: 'displayName="Great Publisher*" AND
* publisherPlatform=ADMOB AND biddingState != PENDING' See https://google.aip.dev/160 for
* more information about filtering syntax.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Query string to filter publisher connections. Connections can be filtered by `displayName`,
`publisherPlatform`, and `biddingState`. If no filter is specified, all publisher connections will
be returned. Example: 'displayName="Great Publisher*" AND publisherPlatform=ADMOB AND biddingState
!= PENDING' See https://google.aip.dev/160 for more information about filtering syntax.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Query string to filter publisher connections. Connections can be filtered by
* `displayName`, `publisherPlatform`, and `biddingState`. If no filter is specified, all
* publisher connections will be returned. Example: 'displayName="Great Publisher*" AND
* publisherPlatform=ADMOB AND biddingState != PENDING' See https://google.aip.dev/160 for
* more information about filtering syntax.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Order specification by which results should be sorted. If no sort order is specified, the
* results will be returned in alphabetic order based on the publisher's publisher code.
* Results can be sorted by `createTime`. Example: 'createTime DESC'.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Order specification by which results should be sorted. If no sort order is specified, the results
will be returned in alphabetic order based on the publisher's publisher code. Results can be sorted
by `createTime`. Example: 'createTime DESC'.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Order specification by which results should be sorted. If no sort order is specified, the
* results will be returned in alphabetic order based on the publisher's publisher code.
* Results can be sorted by `createTime`. Example: 'createTime DESC'.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Requested page size. The server may return fewer results than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, the server
* will pick an appropriate default. Acceptable values are 1 to 5000, inclusive.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested (due to timeout constraint)
even if more are available through another call. If unspecified, the server will pick an
appropriate default. Acceptable values are 1 to 5000, inclusive.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, the server
* will pick an appropriate default. Acceptable values are 1 to 5000, inclusive.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListPublisherConnectionsResponse.nextPageToken returned from the previous call
* to the 'ListPublisherConnections' method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListPublisherConnectionsResponse.nextPageToken returned from the previous call to the
'ListPublisherConnections' method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListPublisherConnectionsResponse.nextPageToken returned from the previous call
* to the 'ListPublisherConnections' method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Buyers collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.Buyers.List request = realtimebidding.buyers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Buyers buyers() {
return new Buyers();
}
/**
* The "buyers" collection of methods.
*/
public class Buyers {
/**
* Gets a buyer account by its name.
*
* Create a request for the method "buyers.get".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the buyer to get. Format: `buyers/{buyerId}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+$");
/**
* Gets a buyer account by its name.
*
* Create a request for the method "buyers.get".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the buyer to get. Format: `buyers/{buyerId}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.Buyer.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the buyer to get. Format: `buyers/{buyerId}` */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the buyer to get. Format: `buyers/{buyerId}`
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the buyer to get. Format: `buyers/{buyerId}` */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Deprecated. This will be removed in October 2023. For more information, see the release notes:
* https://developers.google.com/authorized-buyers/apis/relnotes#real-time-bidding-api Gets
* remarketing tag for a buyer. A remarketing tag is a piece of JavaScript code that can be placed
* on a web page. When a user visits a page containing a remarketing tag, Google adds the user to a
* user list.
*
* Create a request for the method "buyers.getRemarketingTag".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link GetRemarketingTag#execute()} method to invoke the remote
* operation.
*
* @param name Required. To fetch remarketing tag for an account, name must follow the pattern `buyers/{accountId}`
* where `{accountId}` represents ID of a buyer that owns the remarketing tag. For a bidder
* accessing remarketing tag on behalf of a child seat buyer, `{accountId}` should represent
* the ID of the child seat buyer. To fetch remarketing tag for a specific user list, name
* must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
* @return the request
*/
public GetRemarketingTag getRemarketingTag(java.lang.String name) throws java.io.IOException {
GetRemarketingTag result = new GetRemarketingTag(name);
initialize(result);
return result;
}
public class GetRemarketingTag extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}:getRemarketingTag";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+$");
/**
* Deprecated. This will be removed in October 2023. For more information, see the release notes:
* https://developers.google.com/authorized-buyers/apis/relnotes#real-time-bidding-api Gets
* remarketing tag for a buyer. A remarketing tag is a piece of JavaScript code that can be placed
* on a web page. When a user visits a page containing a remarketing tag, Google adds the user to
* a user list.
*
* Create a request for the method "buyers.getRemarketingTag".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link GetRemarketingTag#execute()} method to invoke the remote
* operation. {@link GetRemarketingTag#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. To fetch remarketing tag for an account, name must follow the pattern `buyers/{accountId}`
* where `{accountId}` represents ID of a buyer that owns the remarketing tag. For a bidder
* accessing remarketing tag on behalf of a child seat buyer, `{accountId}` should represent
* the ID of the child seat buyer. To fetch remarketing tag for a specific user list, name
* must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
* @since 1.13
*/
protected GetRemarketingTag(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.GetRemarketingTagResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetRemarketingTag set$Xgafv(java.lang.String $Xgafv) {
return (GetRemarketingTag) super.set$Xgafv($Xgafv);
}
@Override
public GetRemarketingTag setAccessToken(java.lang.String accessToken) {
return (GetRemarketingTag) super.setAccessToken(accessToken);
}
@Override
public GetRemarketingTag setAlt(java.lang.String alt) {
return (GetRemarketingTag) super.setAlt(alt);
}
@Override
public GetRemarketingTag setCallback(java.lang.String callback) {
return (GetRemarketingTag) super.setCallback(callback);
}
@Override
public GetRemarketingTag setFields(java.lang.String fields) {
return (GetRemarketingTag) super.setFields(fields);
}
@Override
public GetRemarketingTag setKey(java.lang.String key) {
return (GetRemarketingTag) super.setKey(key);
}
@Override
public GetRemarketingTag setOauthToken(java.lang.String oauthToken) {
return (GetRemarketingTag) super.setOauthToken(oauthToken);
}
@Override
public GetRemarketingTag setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetRemarketingTag) super.setPrettyPrint(prettyPrint);
}
@Override
public GetRemarketingTag setQuotaUser(java.lang.String quotaUser) {
return (GetRemarketingTag) super.setQuotaUser(quotaUser);
}
@Override
public GetRemarketingTag setUploadType(java.lang.String uploadType) {
return (GetRemarketingTag) super.setUploadType(uploadType);
}
@Override
public GetRemarketingTag setUploadProtocol(java.lang.String uploadProtocol) {
return (GetRemarketingTag) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. To fetch remarketing tag for an account, name must follow the pattern
* `buyers/{accountId}` where `{accountId}` represents ID of a buyer that owns the remarketing
* tag. For a bidder accessing remarketing tag on behalf of a child seat buyer, `{accountId}`
* should represent the ID of the child seat buyer. To fetch remarketing tag for a specific
* user list, name must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See
* UserList.name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. To fetch remarketing tag for an account, name must follow the pattern
`buyers/{accountId}` where `{accountId}` represents ID of a buyer that owns the remarketing tag.
For a bidder accessing remarketing tag on behalf of a child seat buyer, `{accountId}` should
represent the ID of the child seat buyer. To fetch remarketing tag for a specific user list, name
must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. To fetch remarketing tag for an account, name must follow the pattern
* `buyers/{accountId}` where `{accountId}` represents ID of a buyer that owns the remarketing
* tag. For a bidder accessing remarketing tag on behalf of a child seat buyer, `{accountId}`
* should represent the ID of the child seat buyer. To fetch remarketing tag for a specific
* user list, name must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See
* UserList.name.
*/
public GetRemarketingTag setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetRemarketingTag set(String parameterName, Object value) {
return (GetRemarketingTag) super.set(parameterName, value);
}
}
/**
* Lists all buyer account information the calling buyer user or service account is permissioned to
* manage.
*
* Create a request for the method "buyers.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/buyers";
/**
* Lists all buyer account information the calling buyer user or service account is permissioned
* to manage.
*
* Create a request for the method "buyers.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListBuyersResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of buyers to return. If unspecified, at most 100 buyers will be
* returned. The maximum value is 500; values above 500 will be coerced to 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of buyers to return. If unspecified, at most 100 buyers will be returned. The
maximum value is 500; values above 500 will be coerced to 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of buyers to return. If unspecified, at most 100 buyers will be
* returned. The maximum value is 500; values above 500 will be coerced to 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. This value is received from
* a previous `ListBuyers` call in ListBuyersResponse.nextPageToken.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. This value is received from a
previous `ListBuyers` call in ListBuyersResponse.nextPageToken.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. This value is received from
* a previous `ListBuyers` call in ListBuyersResponse.nextPageToken.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Creatives collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.Creatives.List request = realtimebidding.creatives().list(parameters ...)}
*
*
* @return the resource collection
*/
public Creatives creatives() {
return new Creatives();
}
/**
* The "creatives" collection of methods.
*/
public class Creatives {
/**
* Creates a creative.
*
* Create a request for the method "creatives.create".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the parent buyer that the new creative belongs to that must follow the pattern
* `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of the buyer
* who owns a creative. For a bidder accessing creatives on behalf of a child seat buyer,
* `{buyerAccountId}` should represent the account ID of the child seat buyer.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.Creative}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.Creative content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/creatives";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+$");
/**
* Creates a creative.
*
* Create a request for the method "creatives.create".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the parent buyer that the new creative belongs to that must follow the pattern
* `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of the buyer
* who owns a creative. For a bidder accessing creatives on behalf of a child seat buyer,
* `{buyerAccountId}` should represent the account ID of the child seat buyer.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.Creative}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.Creative content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.Creative.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the parent buyer that the new creative belongs to that must follow
* the pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID
* of the buyer who owns a creative. For a bidder accessing creatives on behalf of a child
* seat buyer, `{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the parent buyer that the new creative belongs to that must follow the
pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of the buyer
who owns a creative. For a bidder accessing creatives on behalf of a child seat buyer,
`{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the parent buyer that the new creative belongs to that must follow
* the pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID
* of the buyer who owns a creative. For a bidder accessing creatives on behalf of a child
* seat buyer, `{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a creative.
*
* Create a request for the method "creatives.get".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the creative to retrieve. See creative.name.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+/creatives/[^/]+$");
/**
* Gets a creative.
*
* Create a request for the method "creatives.get".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the creative to retrieve. See creative.name.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.Creative.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/creatives/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the creative to retrieve. See creative.name. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the creative to retrieve. See creative.name.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the creative to retrieve. See creative.name. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/creatives/[^/]+$");
}
this.name = name;
return this;
}
/**
* Controls the amount of information included in the response. By default only
* creativeServingDecision is included. To retrieve the entire creative resource (including
* the declared fields and the creative content) specify the view as "FULL".
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Controls the amount of information included in the response. By default only
creativeServingDecision is included. To retrieve the entire creative resource (including the
declared fields and the creative content) specify the view as "FULL".
*/
public java.lang.String getView() {
return view;
}
/**
* Controls the amount of information included in the response. By default only
* creativeServingDecision is included. To retrieve the entire creative resource (including
* the declared fields and the creative content) specify the view as "FULL".
*/
public Get setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists creatives as they are at the time of the initial request. This call may take multiple hours
* to complete. For large, paginated requests, this method returns a snapshot of creatives at the
* time of request for the first page. `lastStatusUpdate` and `creativeServingDecision` may be
* outdated for creatives on sequential pages. We recommend [Google Cloud
* Pub/Sub](//cloud.google.com/pubsub/docs/overview) to view the latest status.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the parent buyer that owns the creatives. The pattern for this resource is either
* `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For `buyers/{buyerAccountId}`,
* the `buyerAccountId` can be one of the following: 1. The ID of the buyer that is accessing
* their own creatives. 2. The ID of the child seat buyer under a bidder account. So for
* listing creatives pertaining to the child seat buyer (`456`) under bidder account (`123`),
* you would use the pattern: `buyers/456`. 3. The ID of the bidder itself. So for listing
* creatives pertaining to bidder (`123`), you would use `buyers/123`. If you want to access
* all creatives pertaining to both the bidder and all of its child seat accounts, you would
* use `bidders/{bidderAccountId}`, for example, for all creatives pertaining to bidder
* (`123`), use `bidders/123`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/creatives";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+$");
/**
* Lists creatives as they are at the time of the initial request. This call may take multiple
* hours to complete. For large, paginated requests, this method returns a snapshot of creatives
* at the time of request for the first page. `lastStatusUpdate` and `creativeServingDecision` may
* be outdated for creatives on sequential pages. We recommend [Google Cloud
* Pub/Sub](//cloud.google.com/pubsub/docs/overview) to view the latest status.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the parent buyer that owns the creatives. The pattern for this resource is either
* `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For `buyers/{buyerAccountId}`,
* the `buyerAccountId` can be one of the following: 1. The ID of the buyer that is accessing
* their own creatives. 2. The ID of the child seat buyer under a bidder account. So for
* listing creatives pertaining to the child seat buyer (`456`) under bidder account (`123`),
* you would use the pattern: `buyers/456`. 3. The ID of the bidder itself. So for listing
* creatives pertaining to bidder (`123`), you would use `buyers/123`. If you want to access
* all creatives pertaining to both the bidder and all of its child seat accounts, you would
* use `bidders/{bidderAccountId}`, for example, for all creatives pertaining to bidder
* (`123`), use `bidders/123`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListCreativesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the parent buyer that owns the creatives. The pattern for this resource
* is either `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For
* `buyers/{buyerAccountId}`, the `buyerAccountId` can be one of the following: 1. The ID of
* the buyer that is accessing their own creatives. 2. The ID of the child seat buyer under
* a bidder account. So for listing creatives pertaining to the child seat buyer (`456`)
* under bidder account (`123`), you would use the pattern: `buyers/456`. 3. The ID of the
* bidder itself. So for listing creatives pertaining to bidder (`123`), you would use
* `buyers/123`. If you want to access all creatives pertaining to both the bidder and all
* of its child seat accounts, you would use `bidders/{bidderAccountId}`, for example, for
* all creatives pertaining to bidder (`123`), use `bidders/123`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the parent buyer that owns the creatives. The pattern for this resource is either
`buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For `buyers/{buyerAccountId}`, the
`buyerAccountId` can be one of the following: 1. The ID of the buyer that is accessing their own
creatives. 2. The ID of the child seat buyer under a bidder account. So for listing creatives
pertaining to the child seat buyer (`456`) under bidder account (`123`), you would use the pattern:
`buyers/456`. 3. The ID of the bidder itself. So for listing creatives pertaining to bidder
(`123`), you would use `buyers/123`. If you want to access all creatives pertaining to both the
bidder and all of its child seat accounts, you would use `bidders/{bidderAccountId}`, for example,
for all creatives pertaining to bidder (`123`), use `bidders/123`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the parent buyer that owns the creatives. The pattern for this resource
* is either `buyers/{buyerAccountId}` or `bidders/{bidderAccountId}`. For
* `buyers/{buyerAccountId}`, the `buyerAccountId` can be one of the following: 1. The ID of
* the buyer that is accessing their own creatives. 2. The ID of the child seat buyer under
* a bidder account. So for listing creatives pertaining to the child seat buyer (`456`)
* under bidder account (`123`), you would use the pattern: `buyers/456`. 3. The ID of the
* bidder itself. So for listing creatives pertaining to bidder (`123`), you would use
* `buyers/123`. If you want to access all creatives pertaining to both the bidder and all
* of its child seat accounts, you would use `bidders/{bidderAccountId}`, for example, for
* all creatives pertaining to bidder (`123`), use `bidders/123`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Query string to filter creatives. If no filter is specified, all active creatives will be
* returned. Example: 'accountId=12345 AND (dealsStatus:DISAPPROVED AND
* disapprovalReason:UNACCEPTABLE_CONTENT) OR declaredAttributes:IS_COOKIE_TARGETED'
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Query string to filter creatives. If no filter is specified, all active creatives will be returned.
Example: 'accountId=12345 AND (dealsStatus:DISAPPROVED AND disapprovalReason:UNACCEPTABLE_CONTENT)
OR declaredAttributes:IS_COOKIE_TARGETED'
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Query string to filter creatives. If no filter is specified, all active creatives will be
* returned. Example: 'accountId=12345 AND (dealsStatus:DISAPPROVED AND
* disapprovalReason:UNACCEPTABLE_CONTENT) OR declaredAttributes:IS_COOKIE_TARGETED'
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Requested page size. The server may return fewer creatives than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, server will
* pick an appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer creatives than requested (due to timeout
constraint) even if more are available through another call. If unspecified, server will pick an
appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer creatives than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, server will
* pick an appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListCreativesResponse.nextPageToken returned from the previous call to the
* 'ListCreatives' method. Page tokens for continued pages are valid for up to five hours,
* counting from the call to 'ListCreatives' for the first page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListCreativesResponse.nextPageToken returned from the previous call to the 'ListCreatives' method.
Page tokens for continued pages are valid for up to five hours, counting from the call to
'ListCreatives' for the first page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListCreativesResponse.nextPageToken returned from the previous call to the
* 'ListCreatives' method. Page tokens for continued pages are valid for up to five hours,
* counting from the call to 'ListCreatives' for the first page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Controls the amount of information included in the response. By default only
* creativeServingDecision is included. To retrieve the entire creative resource (including
* the declared fields and the creative content) specify the view as "FULL".
*/
@com.google.api.client.util.Key
private java.lang.String view;
/** Controls the amount of information included in the response. By default only
creativeServingDecision is included. To retrieve the entire creative resource (including the
declared fields and the creative content) specify the view as "FULL".
*/
public java.lang.String getView() {
return view;
}
/**
* Controls the amount of information included in the response. By default only
* creativeServingDecision is included. To retrieve the entire creative resource (including
* the declared fields and the creative content) specify the view as "FULL".
*/
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a creative.
*
* Create a request for the method "creatives.patch".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Name of the creative. Follows the pattern `buyers/{buyer}/creatives/{creative}`, where
* `{buyer}` represents the account ID of the buyer who owns the creative, and `{creative}`
* is the buyer-specific creative ID that references this creative in the bid response.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.Creative}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.realtimebidding.v1.model.Creative content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+/creatives/[^/]+$");
/**
* Updates a creative.
*
* Create a request for the method "creatives.patch".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Name of the creative. Follows the pattern `buyers/{buyer}/creatives/{creative}`, where
* `{buyer}` represents the account ID of the buyer who owns the creative, and `{creative}`
* is the buyer-specific creative ID that references this creative in the bid response.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.Creative}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.realtimebidding.v1.model.Creative content) {
super(RealTimeBidding.this, "PATCH", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.Creative.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/creatives/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Name of the creative. Follows the pattern
* `buyers/{buyer}/creatives/{creative}`, where `{buyer}` represents the account ID of the
* buyer who owns the creative, and `{creative}` is the buyer-specific creative ID that
* references this creative in the bid response.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Name of the creative. Follows the pattern `buyers/{buyer}/creatives/{creative}`, where
`{buyer}` represents the account ID of the buyer who owns the creative, and `{creative}` is the
buyer-specific creative ID that references this creative in the bid response.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Name of the creative. Follows the pattern
* `buyers/{buyer}/creatives/{creative}`, where `{buyer}` represents the account ID of the
* buyer who owns the creative, and `{creative}` is the buyer-specific creative ID that
* references this creative in the bid response.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/creatives/[^/]+$");
}
this.name = name;
return this;
}
/** Field mask to use for partial in-place updates. */
@com.google.api.client.util.Key
private String updateMask;
/** Field mask to use for partial in-place updates.
*/
public String getUpdateMask() {
return updateMask;
}
/** Field mask to use for partial in-place updates. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the UserLists collection.
*
* The typical use is:
*
* {@code RealTimeBidding realtimebidding = new RealTimeBidding(...);}
* {@code RealTimeBidding.UserLists.List request = realtimebidding.userLists().list(parameters ...)}
*
*
* @return the resource collection
*/
public UserLists userLists() {
return new UserLists();
}
/**
* The "userLists" collection of methods.
*/
public class UserLists {
/**
* Change the status of a user list to CLOSED. This prevents new users from being added to the user
* list.
*
* Create a request for the method "userLists.close".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Close#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the user list to close. See UserList.name
* @param content the {@link com.google.api.services.realtimebidding.v1.model.CloseUserListRequest}
* @return the request
*/
public Close close(java.lang.String name, com.google.api.services.realtimebidding.v1.model.CloseUserListRequest content) throws java.io.IOException {
Close result = new Close(name, content);
initialize(result);
return result;
}
public class Close extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}:close";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+/userLists/[^/]+$");
/**
* Change the status of a user list to CLOSED. This prevents new users from being added to the
* user list.
*
* Create a request for the method "userLists.close".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Close#execute()} method to invoke the remote operation.
* {@link
* Close#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the user list to close. See UserList.name
* @param content the {@link com.google.api.services.realtimebidding.v1.model.CloseUserListRequest}
* @since 1.13
*/
protected Close(java.lang.String name, com.google.api.services.realtimebidding.v1.model.CloseUserListRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.UserList.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
}
@Override
public Close set$Xgafv(java.lang.String $Xgafv) {
return (Close) super.set$Xgafv($Xgafv);
}
@Override
public Close setAccessToken(java.lang.String accessToken) {
return (Close) super.setAccessToken(accessToken);
}
@Override
public Close setAlt(java.lang.String alt) {
return (Close) super.setAlt(alt);
}
@Override
public Close setCallback(java.lang.String callback) {
return (Close) super.setCallback(callback);
}
@Override
public Close setFields(java.lang.String fields) {
return (Close) super.setFields(fields);
}
@Override
public Close setKey(java.lang.String key) {
return (Close) super.setKey(key);
}
@Override
public Close setOauthToken(java.lang.String oauthToken) {
return (Close) super.setOauthToken(oauthToken);
}
@Override
public Close setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Close) super.setPrettyPrint(prettyPrint);
}
@Override
public Close setQuotaUser(java.lang.String quotaUser) {
return (Close) super.setQuotaUser(quotaUser);
}
@Override
public Close setUploadType(java.lang.String uploadType) {
return (Close) super.setUploadType(uploadType);
}
@Override
public Close setUploadProtocol(java.lang.String uploadProtocol) {
return (Close) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the user list to close. See UserList.name */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the user list to close. See UserList.name
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the user list to close. See UserList.name */
public Close setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Close set(String parameterName, Object value) {
return (Close) super.set(parameterName, value);
}
}
/**
* Create a new user list.
*
* Create a request for the method "userLists.create".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the parent buyer of the user list to be retrieved that must follow the pattern
* `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of the buyer
* who owns user lists. For a bidder accessing user lists on behalf of a child seat buyer ,
* `{buyerAccountId}` should represent the account ID of the child seat buyer.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.UserList}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.UserList content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/userLists";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+$");
/**
* Create a new user list.
*
* Create a request for the method "userLists.create".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the parent buyer of the user list to be retrieved that must follow the pattern
* `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of the buyer
* who owns user lists. For a bidder accessing user lists on behalf of a child seat buyer ,
* `{buyerAccountId}` should represent the account ID of the child seat buyer.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.UserList}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.realtimebidding.v1.model.UserList content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.UserList.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the parent buyer of the user list to be retrieved that must follow
* the pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID
* of the buyer who owns user lists. For a bidder accessing user lists on behalf of a child
* seat buyer , `{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the parent buyer of the user list to be retrieved that must follow the
pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of the buyer
who owns user lists. For a bidder accessing user lists on behalf of a child seat buyer ,
`{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the parent buyer of the user list to be retrieved that must follow
* the pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID
* of the buyer who owns user lists. For a bidder accessing user lists on behalf of a child
* seat buyer , `{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a user list by its name.
*
* Create a request for the method "userLists.get".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the user list to be retrieved. See UserList.name.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+/userLists/[^/]+$");
/**
* Gets a user list by its name.
*
* Create a request for the method "userLists.get".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the user list to be retrieved. See UserList.name.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.UserList.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the user list to be retrieved. See UserList.name. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the user list to be retrieved. See UserList.name.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the user list to be retrieved. See UserList.name. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Deprecated. This will be removed in October 2023. For more information, see the release notes:
* https://developers.google.com/authorized-buyers/apis/relnotes#real-time-bidding-api Gets
* remarketing tag for a buyer. A remarketing tag is a piece of JavaScript code that can be placed
* on a web page. When a user visits a page containing a remarketing tag, Google adds the user to a
* user list.
*
* Create a request for the method "userLists.getRemarketingTag".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link GetRemarketingTag#execute()} method to invoke the remote
* operation.
*
* @param name Required. To fetch remarketing tag for an account, name must follow the pattern `buyers/{accountId}`
* where `{accountId}` represents ID of a buyer that owns the remarketing tag. For a bidder
* accessing remarketing tag on behalf of a child seat buyer, `{accountId}` should represent
* the ID of the child seat buyer. To fetch remarketing tag for a specific user list, name
* must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
* @return the request
*/
public GetRemarketingTag getRemarketingTag(java.lang.String name) throws java.io.IOException {
GetRemarketingTag result = new GetRemarketingTag(name);
initialize(result);
return result;
}
public class GetRemarketingTag extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}:getRemarketingTag";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+/userLists/[^/]+$");
/**
* Deprecated. This will be removed in October 2023. For more information, see the release notes:
* https://developers.google.com/authorized-buyers/apis/relnotes#real-time-bidding-api Gets
* remarketing tag for a buyer. A remarketing tag is a piece of JavaScript code that can be placed
* on a web page. When a user visits a page containing a remarketing tag, Google adds the user to
* a user list.
*
* Create a request for the method "userLists.getRemarketingTag".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link GetRemarketingTag#execute()} method to invoke the remote
* operation. {@link GetRemarketingTag#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. To fetch remarketing tag for an account, name must follow the pattern `buyers/{accountId}`
* where `{accountId}` represents ID of a buyer that owns the remarketing tag. For a bidder
* accessing remarketing tag on behalf of a child seat buyer, `{accountId}` should represent
* the ID of the child seat buyer. To fetch remarketing tag for a specific user list, name
* must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
* @since 1.13
*/
protected GetRemarketingTag(java.lang.String name) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.GetRemarketingTagResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetRemarketingTag set$Xgafv(java.lang.String $Xgafv) {
return (GetRemarketingTag) super.set$Xgafv($Xgafv);
}
@Override
public GetRemarketingTag setAccessToken(java.lang.String accessToken) {
return (GetRemarketingTag) super.setAccessToken(accessToken);
}
@Override
public GetRemarketingTag setAlt(java.lang.String alt) {
return (GetRemarketingTag) super.setAlt(alt);
}
@Override
public GetRemarketingTag setCallback(java.lang.String callback) {
return (GetRemarketingTag) super.setCallback(callback);
}
@Override
public GetRemarketingTag setFields(java.lang.String fields) {
return (GetRemarketingTag) super.setFields(fields);
}
@Override
public GetRemarketingTag setKey(java.lang.String key) {
return (GetRemarketingTag) super.setKey(key);
}
@Override
public GetRemarketingTag setOauthToken(java.lang.String oauthToken) {
return (GetRemarketingTag) super.setOauthToken(oauthToken);
}
@Override
public GetRemarketingTag setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetRemarketingTag) super.setPrettyPrint(prettyPrint);
}
@Override
public GetRemarketingTag setQuotaUser(java.lang.String quotaUser) {
return (GetRemarketingTag) super.setQuotaUser(quotaUser);
}
@Override
public GetRemarketingTag setUploadType(java.lang.String uploadType) {
return (GetRemarketingTag) super.setUploadType(uploadType);
}
@Override
public GetRemarketingTag setUploadProtocol(java.lang.String uploadProtocol) {
return (GetRemarketingTag) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. To fetch remarketing tag for an account, name must follow the pattern
* `buyers/{accountId}` where `{accountId}` represents ID of a buyer that owns the
* remarketing tag. For a bidder accessing remarketing tag on behalf of a child seat buyer,
* `{accountId}` should represent the ID of the child seat buyer. To fetch remarketing tag
* for a specific user list, name must follow the pattern
* `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. To fetch remarketing tag for an account, name must follow the pattern
`buyers/{accountId}` where `{accountId}` represents ID of a buyer that owns the remarketing tag.
For a bidder accessing remarketing tag on behalf of a child seat buyer, `{accountId}` should
represent the ID of the child seat buyer. To fetch remarketing tag for a specific user list, name
must follow the pattern `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. To fetch remarketing tag for an account, name must follow the pattern
* `buyers/{accountId}` where `{accountId}` represents ID of a buyer that owns the
* remarketing tag. For a bidder accessing remarketing tag on behalf of a child seat buyer,
* `{accountId}` should represent the ID of the child seat buyer. To fetch remarketing tag
* for a specific user list, name must follow the pattern
* `buyers/{accountId}/userLists/{userListId}`. See UserList.name.
*/
public GetRemarketingTag setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetRemarketingTag set(String parameterName, Object value) {
return (GetRemarketingTag) super.set(parameterName, value);
}
}
/**
* Lists the user lists visible to the current user.
*
* Create a request for the method "userLists.list".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the parent buyer for the user lists to be returned that must follow the
* pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of
* the buyer who owns user lists. For a bidder accessing user lists on behalf of a child seat
* buyer , `{buyerAccountId}` should represent the account ID of the child seat buyer.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+parent}/userLists";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+$");
/**
* Lists the user lists visible to the current user.
*
* Create a request for the method "userLists.list".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the parent buyer for the user lists to be returned that must follow the
* pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of
* the buyer who owns user lists. For a bidder accessing user lists on behalf of a child seat
* buyer , `{buyerAccountId}` should represent the account ID of the child seat buyer.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(RealTimeBidding.this, "GET", REST_PATH, null, com.google.api.services.realtimebidding.v1.model.ListUserListsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the parent buyer for the user lists to be returned that must follow
* the pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID
* of the buyer who owns user lists. For a bidder accessing user lists on behalf of a child
* seat buyer , `{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the parent buyer for the user lists to be returned that must follow the
pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID of the buyer
who owns user lists. For a bidder accessing user lists on behalf of a child seat buyer ,
`{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the parent buyer for the user lists to be returned that must follow
* the pattern `buyers/{buyerAccountId}`, where `{buyerAccountId}` represents the account ID
* of the buyer who owns user lists. For a bidder accessing user lists on behalf of a child
* seat buyer , `{buyerAccountId}` should represent the account ID of the child seat buyer.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^buyers/[^/]+$");
}
this.parent = parent;
return this;
}
/** The number of results to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The number of results to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The number of results to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Continuation page token (as received from a previous response). */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation page token (as received from a previous response).
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation page token (as received from a previous response). */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Change the status of a user list to OPEN. This allows new users to be added to the user list.
*
* Create a request for the method "userLists.open".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Open#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the user list to open. See UserList.name
* @param content the {@link com.google.api.services.realtimebidding.v1.model.OpenUserListRequest}
* @return the request
*/
public Open open(java.lang.String name, com.google.api.services.realtimebidding.v1.model.OpenUserListRequest content) throws java.io.IOException {
Open result = new Open(name, content);
initialize(result);
return result;
}
public class Open extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}:open";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+/userLists/[^/]+$");
/**
* Change the status of a user list to OPEN. This allows new users to be added to the user list.
*
* Create a request for the method "userLists.open".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Open#execute()} method to invoke the remote operation.
* {@link Open#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the user list to open. See UserList.name
* @param content the {@link com.google.api.services.realtimebidding.v1.model.OpenUserListRequest}
* @since 1.13
*/
protected Open(java.lang.String name, com.google.api.services.realtimebidding.v1.model.OpenUserListRequest content) {
super(RealTimeBidding.this, "POST", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.UserList.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
}
@Override
public Open set$Xgafv(java.lang.String $Xgafv) {
return (Open) super.set$Xgafv($Xgafv);
}
@Override
public Open setAccessToken(java.lang.String accessToken) {
return (Open) super.setAccessToken(accessToken);
}
@Override
public Open setAlt(java.lang.String alt) {
return (Open) super.setAlt(alt);
}
@Override
public Open setCallback(java.lang.String callback) {
return (Open) super.setCallback(callback);
}
@Override
public Open setFields(java.lang.String fields) {
return (Open) super.setFields(fields);
}
@Override
public Open setKey(java.lang.String key) {
return (Open) super.setKey(key);
}
@Override
public Open setOauthToken(java.lang.String oauthToken) {
return (Open) super.setOauthToken(oauthToken);
}
@Override
public Open setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Open) super.setPrettyPrint(prettyPrint);
}
@Override
public Open setQuotaUser(java.lang.String quotaUser) {
return (Open) super.setQuotaUser(quotaUser);
}
@Override
public Open setUploadType(java.lang.String uploadType) {
return (Open) super.setUploadType(uploadType);
}
@Override
public Open setUploadProtocol(java.lang.String uploadProtocol) {
return (Open) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the user list to open. See UserList.name */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the user list to open. See UserList.name
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the user list to open. See UserList.name */
public Open setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Open set(String parameterName, Object value) {
return (Open) super.set(parameterName, value);
}
}
/**
* Update the given user list. Only user lists with URLRestrictions can be updated.
*
* Create a request for the method "userLists.update".
*
* This request holds the parameters needed by the realtimebidding server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param name Output only. Name of the user list that must follow the pattern
* `buyers/{buyer}/userLists/{user_list}`, where `{buyer}` represents the account ID of the
* buyer who owns the user list. For a bidder accessing user lists on behalf of a child seat
* buyer, `{buyer}` represents the account ID of the child seat buyer. `{user_list}` is an
* int64 identifier assigned by Google to uniquely identify a user list.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.UserList}
* @return the request
*/
public Update update(java.lang.String name, com.google.api.services.realtimebidding.v1.model.UserList content) throws java.io.IOException {
Update result = new Update(name, content);
initialize(result);
return result;
}
public class Update extends RealTimeBiddingRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^buyers/[^/]+/userLists/[^/]+$");
/**
* Update the given user list. Only user lists with URLRestrictions can be updated.
*
* Create a request for the method "userLists.update".
*
* This request holds the parameters needed by the the realtimebidding server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Name of the user list that must follow the pattern
* `buyers/{buyer}/userLists/{user_list}`, where `{buyer}` represents the account ID of the
* buyer who owns the user list. For a bidder accessing user lists on behalf of a child seat
* buyer, `{buyer}` represents the account ID of the child seat buyer. `{user_list}` is an
* int64 identifier assigned by Google to uniquely identify a user list.
* @param content the {@link com.google.api.services.realtimebidding.v1.model.UserList}
* @since 1.13
*/
protected Update(java.lang.String name, com.google.api.services.realtimebidding.v1.model.UserList content) {
super(RealTimeBidding.this, "PUT", REST_PATH, content, com.google.api.services.realtimebidding.v1.model.UserList.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Name of the user list that must follow the pattern
* `buyers/{buyer}/userLists/{user_list}`, where `{buyer}` represents the account ID of the
* buyer who owns the user list. For a bidder accessing user lists on behalf of a child seat
* buyer, `{buyer}` represents the account ID of the child seat buyer. `{user_list}` is an
* int64 identifier assigned by Google to uniquely identify a user list.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Name of the user list that must follow the pattern
`buyers/{buyer}/userLists/{user_list}`, where `{buyer}` represents the account ID of the buyer who
owns the user list. For a bidder accessing user lists on behalf of a child seat buyer, `{buyer}`
represents the account ID of the child seat buyer. `{user_list}` is an int64 identifier assigned by
Google to uniquely identify a user list.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Name of the user list that must follow the pattern
* `buyers/{buyer}/userLists/{user_list}`, where `{buyer}` represents the account ID of the
* buyer who owns the user list. For a bidder accessing user lists on behalf of a child seat
* buyer, `{buyer}` represents the account ID of the child seat buyer. `{user_list}` is an
* int64 identifier assigned by Google to uniquely identify a user list.
*/
public Update setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^buyers/[^/]+/userLists/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link RealTimeBidding}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link RealTimeBidding}. */
@Override
public RealTimeBidding build() {
return new RealTimeBidding(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link RealTimeBiddingRequestInitializer}.
*
* @since 1.12
*/
public Builder setRealTimeBiddingRequestInitializer(
RealTimeBiddingRequestInitializer realtimebiddingRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(realtimebiddingRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}