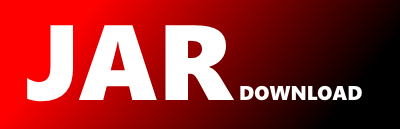
com.google.api.services.realtimebidding.v1.model.CreativeServingDecision Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.realtimebidding.v1.model;
/**
* Top level status and detected attributes of a creative.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Real-time Bidding API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CreativeServingDecision extends com.google.api.client.json.GenericJson {
/**
* The detected ad technology providers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AdTechnologyProviders adTechnologyProviders;
/**
* The policy compliance of this creative in China. When approved or disapproved, this applies to
* both deals and open auction in China. When pending review, this creative is allowed to serve
* for deals but not for open auction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PolicyCompliance chinaPolicyCompliance;
/**
* Policy compliance of this creative when bidding on Programmatic Guaranteed and Preferred Deals
* (outside of Russia and China).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PolicyCompliance dealsPolicyCompliance;
/**
* Detected advertisers and brands.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedAdvertisers;
static {
// hack to force ProGuard to consider AdvertiserAndBrand used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AdvertiserAndBrand.class);
}
/**
* Publisher-excludable attributes that were detected for this creative. Can be used to filter the
* response of the creatives.list method. If the `excluded_attribute` field of a [bid
* request](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-proto)
* contains one of the attributes that were declared or detected for a given creative, and a bid
* is submitted with that creative, the bid will be filtered before the auction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedAttributes;
/**
* The set of detected destination URLs for the creative. Can be used to filter the response of
* the creatives.list method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedClickThroughUrls;
/**
* The detected domains for this creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedDomains;
/**
* The detected languages for this creative. The order is arbitrary. The codes are 2 or 5
* characters and are documented at
* https://developers.google.com/adwords/api/docs/appendix/languagecodes. Can be used to filter
* the response of the creatives.list method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedLanguages;
/**
* Detected product categories, if any. See the ad-product-categories.txt file in the technical
* documentation for a list of IDs. Can be used to filter the response of the creatives.list
* method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedProductCategories;
/**
* Detected sensitive categories, if any. Can be used to filter the response of the creatives.list
* method. See the ad-sensitive-categories.txt file in the technical documentation for a list of
* IDs. You should use these IDs along with the excluded-sensitive-category field in the bid
* request to filter your bids.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedSensitiveCategories;
/**
* IDs of the ad technology vendors that were detected to be used by this creative. See
* https://storage.googleapis.com/adx-rtb-dictionaries/vendors.txt for possible values. Can be
* used to filter the response of the creatives.list method. If the `allowed_vendor_type` field of
* a [bid request](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-
* proto) does not contain one of the vendor type IDs that were declared or detected for a given
* creative, and a bid is submitted with that creative, the bid will be filtered before the
* auction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedVendorIds;
/**
* The last time the creative status was updated. Can be used to filter the response of the
* creatives.list method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String lastStatusUpdate;
/**
* Policy compliance of this creative when bidding in open auction, private auction, or auction
* packages (outside of Russia and China).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PolicyCompliance networkPolicyCompliance;
/**
* Policy compliance of this creative when bidding in Open Bidding (outside of Russia and China).
* For the list of platform policies, see:
* https://support.google.com/platformspolicy/answer/3013851.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PolicyCompliance platformPolicyCompliance;
/**
* The policy compliance of this creative in Russia. When approved or disapproved, this applies to
* both deals and open auction in Russia. When pending review, this creative is allowed to serve
* for deals but not for open auction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PolicyCompliance russiaPolicyCompliance;
/**
* The detected ad technology providers.
* @return value or {@code null} for none
*/
public AdTechnologyProviders getAdTechnologyProviders() {
return adTechnologyProviders;
}
/**
* The detected ad technology providers.
* @param adTechnologyProviders adTechnologyProviders or {@code null} for none
*/
public CreativeServingDecision setAdTechnologyProviders(AdTechnologyProviders adTechnologyProviders) {
this.adTechnologyProviders = adTechnologyProviders;
return this;
}
/**
* The policy compliance of this creative in China. When approved or disapproved, this applies to
* both deals and open auction in China. When pending review, this creative is allowed to serve
* for deals but not for open auction.
* @return value or {@code null} for none
*/
public PolicyCompliance getChinaPolicyCompliance() {
return chinaPolicyCompliance;
}
/**
* The policy compliance of this creative in China. When approved or disapproved, this applies to
* both deals and open auction in China. When pending review, this creative is allowed to serve
* for deals but not for open auction.
* @param chinaPolicyCompliance chinaPolicyCompliance or {@code null} for none
*/
public CreativeServingDecision setChinaPolicyCompliance(PolicyCompliance chinaPolicyCompliance) {
this.chinaPolicyCompliance = chinaPolicyCompliance;
return this;
}
/**
* Policy compliance of this creative when bidding on Programmatic Guaranteed and Preferred Deals
* (outside of Russia and China).
* @return value or {@code null} for none
*/
public PolicyCompliance getDealsPolicyCompliance() {
return dealsPolicyCompliance;
}
/**
* Policy compliance of this creative when bidding on Programmatic Guaranteed and Preferred Deals
* (outside of Russia and China).
* @param dealsPolicyCompliance dealsPolicyCompliance or {@code null} for none
*/
public CreativeServingDecision setDealsPolicyCompliance(PolicyCompliance dealsPolicyCompliance) {
this.dealsPolicyCompliance = dealsPolicyCompliance;
return this;
}
/**
* Detected advertisers and brands.
* @return value or {@code null} for none
*/
public java.util.List getDetectedAdvertisers() {
return detectedAdvertisers;
}
/**
* Detected advertisers and brands.
* @param detectedAdvertisers detectedAdvertisers or {@code null} for none
*/
public CreativeServingDecision setDetectedAdvertisers(java.util.List detectedAdvertisers) {
this.detectedAdvertisers = detectedAdvertisers;
return this;
}
/**
* Publisher-excludable attributes that were detected for this creative. Can be used to filter the
* response of the creatives.list method. If the `excluded_attribute` field of a [bid
* request](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-proto)
* contains one of the attributes that were declared or detected for a given creative, and a bid
* is submitted with that creative, the bid will be filtered before the auction.
* @return value or {@code null} for none
*/
public java.util.List getDetectedAttributes() {
return detectedAttributes;
}
/**
* Publisher-excludable attributes that were detected for this creative. Can be used to filter the
* response of the creatives.list method. If the `excluded_attribute` field of a [bid
* request](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-proto)
* contains one of the attributes that were declared or detected for a given creative, and a bid
* is submitted with that creative, the bid will be filtered before the auction.
* @param detectedAttributes detectedAttributes or {@code null} for none
*/
public CreativeServingDecision setDetectedAttributes(java.util.List detectedAttributes) {
this.detectedAttributes = detectedAttributes;
return this;
}
/**
* The set of detected destination URLs for the creative. Can be used to filter the response of
* the creatives.list method.
* @return value or {@code null} for none
*/
public java.util.List getDetectedClickThroughUrls() {
return detectedClickThroughUrls;
}
/**
* The set of detected destination URLs for the creative. Can be used to filter the response of
* the creatives.list method.
* @param detectedClickThroughUrls detectedClickThroughUrls or {@code null} for none
*/
public CreativeServingDecision setDetectedClickThroughUrls(java.util.List detectedClickThroughUrls) {
this.detectedClickThroughUrls = detectedClickThroughUrls;
return this;
}
/**
* The detected domains for this creative.
* @return value or {@code null} for none
*/
public java.util.List getDetectedDomains() {
return detectedDomains;
}
/**
* The detected domains for this creative.
* @param detectedDomains detectedDomains or {@code null} for none
*/
public CreativeServingDecision setDetectedDomains(java.util.List detectedDomains) {
this.detectedDomains = detectedDomains;
return this;
}
/**
* The detected languages for this creative. The order is arbitrary. The codes are 2 or 5
* characters and are documented at
* https://developers.google.com/adwords/api/docs/appendix/languagecodes. Can be used to filter
* the response of the creatives.list method.
* @return value or {@code null} for none
*/
public java.util.List getDetectedLanguages() {
return detectedLanguages;
}
/**
* The detected languages for this creative. The order is arbitrary. The codes are 2 or 5
* characters and are documented at
* https://developers.google.com/adwords/api/docs/appendix/languagecodes. Can be used to filter
* the response of the creatives.list method.
* @param detectedLanguages detectedLanguages or {@code null} for none
*/
public CreativeServingDecision setDetectedLanguages(java.util.List detectedLanguages) {
this.detectedLanguages = detectedLanguages;
return this;
}
/**
* Detected product categories, if any. See the ad-product-categories.txt file in the technical
* documentation for a list of IDs. Can be used to filter the response of the creatives.list
* method.
* @return value or {@code null} for none
*/
public java.util.List getDetectedProductCategories() {
return detectedProductCategories;
}
/**
* Detected product categories, if any. See the ad-product-categories.txt file in the technical
* documentation for a list of IDs. Can be used to filter the response of the creatives.list
* method.
* @param detectedProductCategories detectedProductCategories or {@code null} for none
*/
public CreativeServingDecision setDetectedProductCategories(java.util.List detectedProductCategories) {
this.detectedProductCategories = detectedProductCategories;
return this;
}
/**
* Detected sensitive categories, if any. Can be used to filter the response of the creatives.list
* method. See the ad-sensitive-categories.txt file in the technical documentation for a list of
* IDs. You should use these IDs along with the excluded-sensitive-category field in the bid
* request to filter your bids.
* @return value or {@code null} for none
*/
public java.util.List getDetectedSensitiveCategories() {
return detectedSensitiveCategories;
}
/**
* Detected sensitive categories, if any. Can be used to filter the response of the creatives.list
* method. See the ad-sensitive-categories.txt file in the technical documentation for a list of
* IDs. You should use these IDs along with the excluded-sensitive-category field in the bid
* request to filter your bids.
* @param detectedSensitiveCategories detectedSensitiveCategories or {@code null} for none
*/
public CreativeServingDecision setDetectedSensitiveCategories(java.util.List detectedSensitiveCategories) {
this.detectedSensitiveCategories = detectedSensitiveCategories;
return this;
}
/**
* IDs of the ad technology vendors that were detected to be used by this creative. See
* https://storage.googleapis.com/adx-rtb-dictionaries/vendors.txt for possible values. Can be
* used to filter the response of the creatives.list method. If the `allowed_vendor_type` field of
* a [bid request](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-
* proto) does not contain one of the vendor type IDs that were declared or detected for a given
* creative, and a bid is submitted with that creative, the bid will be filtered before the
* auction.
* @return value or {@code null} for none
*/
public java.util.List getDetectedVendorIds() {
return detectedVendorIds;
}
/**
* IDs of the ad technology vendors that were detected to be used by this creative. See
* https://storage.googleapis.com/adx-rtb-dictionaries/vendors.txt for possible values. Can be
* used to filter the response of the creatives.list method. If the `allowed_vendor_type` field of
* a [bid request](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-
* proto) does not contain one of the vendor type IDs that were declared or detected for a given
* creative, and a bid is submitted with that creative, the bid will be filtered before the
* auction.
* @param detectedVendorIds detectedVendorIds or {@code null} for none
*/
public CreativeServingDecision setDetectedVendorIds(java.util.List detectedVendorIds) {
this.detectedVendorIds = detectedVendorIds;
return this;
}
/**
* The last time the creative status was updated. Can be used to filter the response of the
* creatives.list method.
* @return value or {@code null} for none
*/
public String getLastStatusUpdate() {
return lastStatusUpdate;
}
/**
* The last time the creative status was updated. Can be used to filter the response of the
* creatives.list method.
* @param lastStatusUpdate lastStatusUpdate or {@code null} for none
*/
public CreativeServingDecision setLastStatusUpdate(String lastStatusUpdate) {
this.lastStatusUpdate = lastStatusUpdate;
return this;
}
/**
* Policy compliance of this creative when bidding in open auction, private auction, or auction
* packages (outside of Russia and China).
* @return value or {@code null} for none
*/
public PolicyCompliance getNetworkPolicyCompliance() {
return networkPolicyCompliance;
}
/**
* Policy compliance of this creative when bidding in open auction, private auction, or auction
* packages (outside of Russia and China).
* @param networkPolicyCompliance networkPolicyCompliance or {@code null} for none
*/
public CreativeServingDecision setNetworkPolicyCompliance(PolicyCompliance networkPolicyCompliance) {
this.networkPolicyCompliance = networkPolicyCompliance;
return this;
}
/**
* Policy compliance of this creative when bidding in Open Bidding (outside of Russia and China).
* For the list of platform policies, see:
* https://support.google.com/platformspolicy/answer/3013851.
* @return value or {@code null} for none
*/
public PolicyCompliance getPlatformPolicyCompliance() {
return platformPolicyCompliance;
}
/**
* Policy compliance of this creative when bidding in Open Bidding (outside of Russia and China).
* For the list of platform policies, see:
* https://support.google.com/platformspolicy/answer/3013851.
* @param platformPolicyCompliance platformPolicyCompliance or {@code null} for none
*/
public CreativeServingDecision setPlatformPolicyCompliance(PolicyCompliance platformPolicyCompliance) {
this.platformPolicyCompliance = platformPolicyCompliance;
return this;
}
/**
* The policy compliance of this creative in Russia. When approved or disapproved, this applies to
* both deals and open auction in Russia. When pending review, this creative is allowed to serve
* for deals but not for open auction.
* @return value or {@code null} for none
*/
public PolicyCompliance getRussiaPolicyCompliance() {
return russiaPolicyCompliance;
}
/**
* The policy compliance of this creative in Russia. When approved or disapproved, this applies to
* both deals and open auction in Russia. When pending review, this creative is allowed to serve
* for deals but not for open auction.
* @param russiaPolicyCompliance russiaPolicyCompliance or {@code null} for none
*/
public CreativeServingDecision setRussiaPolicyCompliance(PolicyCompliance russiaPolicyCompliance) {
this.russiaPolicyCompliance = russiaPolicyCompliance;
return this;
}
@Override
public CreativeServingDecision set(String fieldName, Object value) {
return (CreativeServingDecision) super.set(fieldName, value);
}
@Override
public CreativeServingDecision clone() {
return (CreativeServingDecision) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy