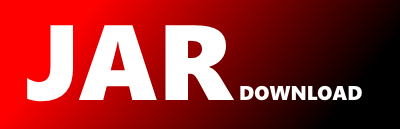
com.google.api.services.realtimebidding.v1.model.PretargetingConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.realtimebidding.v1.model;
/**
* Pretargeting configuration: a set of targeting dimensions applied at the pretargeting stage of
* the RTB funnel. These control which inventory a bidder will receive bid requests for.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Real-time Bidding API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PretargetingConfig extends com.google.api.client.json.GenericJson {
/**
* Targeting modes included by this configuration. A bid request must allow all the specified
* targeting modes. An unset value allows all bid requests to be sent, regardless of which
* targeting modes they allow.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List allowedUserTargetingModes;
/**
* Targeting on a subset of app inventory. If APP is listed in targeted_environments, the
* specified targeting is applied. A maximum of 30,000 app IDs can be targeted. An unset value for
* targeting allows all app-based bid requests to be sent. Apps can either be targeting positively
* (bid requests will be sent only if the destination app is listed in the targeting dimension) or
* negatively (bid requests will be sent only if the destination app is not listed in the
* targeting dimension).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AppTargeting appTargeting;
/**
* Output only. The identifier that corresponds to this pretargeting configuration that helps
* buyers track and attribute their spend across their own arbitrary divisions. If a bid request
* matches more than one configuration, the buyer chooses which billing_id to attribute each of
* their bids.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long billingId;
/**
* The diplay name associated with this configuration. This name must be unique among all the
* pretargeting configurations a bidder has.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* The sensitive content category label IDs excluded in this configuration. Bid requests for
* inventory with any of the specified content label IDs will not be sent. Refer to this file
* https://storage.googleapis.com/adx-rtb-dictionaries/content-labels.txt for category IDs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.List excludedContentLabelIds;
/**
* The geos included or excluded in this configuration defined in https://storage.googleapis.com
* /adx-rtb-dictionaries/geo-table.csv
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NumericTargetingDimension geoTargeting;
/**
* Creative dimensions included by this configuration. Only bid requests eligible for at least one
* of the specified creative dimensions will be sent. An unset value allows all bid requests to be
* sent, regardless of creative dimension.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includedCreativeDimensions;
static {
// hack to force ProGuard to consider CreativeDimensions used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(CreativeDimensions.class);
}
/**
* Environments that are being included. Bid requests will not be sent for a given environment if
* it is not included. Further restrictions can be applied to included environments to target only
* a subset of its inventory. An unset value includes all environments.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includedEnvironments;
/**
* Creative formats included by this configuration. Only bid requests eligible for at least one of
* the specified creative formats will be sent. An unset value will allow all bid requests to be
* sent, regardless of format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includedFormats;
/**
* The languages included in this configuration, represented by their language code. See
* https://developers.google.com/adwords/api/docs/appendix/languagecodes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includedLanguages;
/**
* The mobile operating systems included in this configuration as defined in
* https://storage.googleapis.com/adx-rtb-dictionaries/mobile-os.csv
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.List includedMobileOperatingSystemIds;
/**
* The platforms included by this configration. Bid requests for devices with the specified
* platform types will be sent. An unset value allows all bid requests to be sent, regardless of
* platform.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includedPlatforms;
/**
* User identifier types included in this configuration. At least one of the user identifier types
* specified in this list must be available for the bid request to be sent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includedUserIdTypes;
/**
* The interstitial targeting specified for this configuration. The unset value will allow bid
* requests to be sent regardless of whether they are for interstitials or not.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String interstitialTargeting;
/**
* Output only. Existing included or excluded geos that are invalid. Previously targeted geos may
* become invalid due to privacy restrictions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.List invalidGeoIds;
/**
* The maximum QPS threshold for this configuration. The bidder should receive no more than this
* number of bid requests matching this configuration per second across all their bidding
* endpoints among all trading locations. Further information available at
* https://developers.google.com/authorized-buyers/rtb/peer-guide
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long maximumQps;
/**
* The targeted minimum viewability decile, ranging in values [0, 10]. A value of 5 means that the
* configuration will only match adslots for which we predict at least 50% viewability. Values >
* 10 will be rounded down to 10. An unset value or a value of 0 indicates that bid requests will
* be sent regardless of viewability.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer minimumViewabilityDecile;
/**
* Output only. Name of the pretargeting configuration that must follow the pattern
* `bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Targeting on a subset of publisher inventory. Publishers can either be targeted positively (bid
* requests will be sent only if the publisher is listed in the targeting dimension) or negatively
* (bid requests will be sent only if the publisher is not listed in the targeting dimension). A
* maximum of 10,000 publisher IDs can be targeted. Publisher IDs are found in
* [ads.txt](https://iabtechlab.com/ads-txt/) / [app-ads.txt](https://iabtechlab.com/app-ads-txt/)
* and in bid requests in the `BidRequest.publisher_id` field on the [Google RTB
* protocol](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-proto)
* or the `BidRequest.site.publisher.id` / `BidRequest.app.publisher.id` field on the [OpenRTB
* protocol](https://developers.google.com/authorized-buyers/rtb/downloads/openrtb-adx-proto).
* Publisher IDs will be returned in the order that they were entered.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StringTargetingDimension publisherTargeting;
/**
* Output only. The state of this pretargeting configuration.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* The remarketing lists included or excluded in this configuration as defined in UserList.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NumericTargetingDimension userListTargeting;
/**
* The verticals included or excluded in this configuration as defined in
* https://developers.google.com/authorized-buyers/rtb/downloads/publisher-verticals
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NumericTargetingDimension verticalTargeting;
/**
* Targeting on a subset of site inventory. If WEB is listed in included_environments, the
* specified targeting is applied. A maximum of 50,000 site URLs can be targeted. An unset value
* for targeting allows all web-based bid requests to be sent. Sites can either be targeting
* positively (bid requests will be sent only if the destination site is listed in the targeting
* dimension) or negatively (bid requests will be sent only if the destination site is not listed
* in the pretargeting configuration).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StringTargetingDimension webTargeting;
/**
* Targeting modes included by this configuration. A bid request must allow all the specified
* targeting modes. An unset value allows all bid requests to be sent, regardless of which
* targeting modes they allow.
* @return value or {@code null} for none
*/
public java.util.List getAllowedUserTargetingModes() {
return allowedUserTargetingModes;
}
/**
* Targeting modes included by this configuration. A bid request must allow all the specified
* targeting modes. An unset value allows all bid requests to be sent, regardless of which
* targeting modes they allow.
* @param allowedUserTargetingModes allowedUserTargetingModes or {@code null} for none
*/
public PretargetingConfig setAllowedUserTargetingModes(java.util.List allowedUserTargetingModes) {
this.allowedUserTargetingModes = allowedUserTargetingModes;
return this;
}
/**
* Targeting on a subset of app inventory. If APP is listed in targeted_environments, the
* specified targeting is applied. A maximum of 30,000 app IDs can be targeted. An unset value for
* targeting allows all app-based bid requests to be sent. Apps can either be targeting positively
* (bid requests will be sent only if the destination app is listed in the targeting dimension) or
* negatively (bid requests will be sent only if the destination app is not listed in the
* targeting dimension).
* @return value or {@code null} for none
*/
public AppTargeting getAppTargeting() {
return appTargeting;
}
/**
* Targeting on a subset of app inventory. If APP is listed in targeted_environments, the
* specified targeting is applied. A maximum of 30,000 app IDs can be targeted. An unset value for
* targeting allows all app-based bid requests to be sent. Apps can either be targeting positively
* (bid requests will be sent only if the destination app is listed in the targeting dimension) or
* negatively (bid requests will be sent only if the destination app is not listed in the
* targeting dimension).
* @param appTargeting appTargeting or {@code null} for none
*/
public PretargetingConfig setAppTargeting(AppTargeting appTargeting) {
this.appTargeting = appTargeting;
return this;
}
/**
* Output only. The identifier that corresponds to this pretargeting configuration that helps
* buyers track and attribute their spend across their own arbitrary divisions. If a bid request
* matches more than one configuration, the buyer chooses which billing_id to attribute each of
* their bids.
* @return value or {@code null} for none
*/
public java.lang.Long getBillingId() {
return billingId;
}
/**
* Output only. The identifier that corresponds to this pretargeting configuration that helps
* buyers track and attribute their spend across their own arbitrary divisions. If a bid request
* matches more than one configuration, the buyer chooses which billing_id to attribute each of
* their bids.
* @param billingId billingId or {@code null} for none
*/
public PretargetingConfig setBillingId(java.lang.Long billingId) {
this.billingId = billingId;
return this;
}
/**
* The diplay name associated with this configuration. This name must be unique among all the
* pretargeting configurations a bidder has.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The diplay name associated with this configuration. This name must be unique among all the
* pretargeting configurations a bidder has.
* @param displayName displayName or {@code null} for none
*/
public PretargetingConfig setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* The sensitive content category label IDs excluded in this configuration. Bid requests for
* inventory with any of the specified content label IDs will not be sent. Refer to this file
* https://storage.googleapis.com/adx-rtb-dictionaries/content-labels.txt for category IDs.
* @return value or {@code null} for none
*/
public java.util.List getExcludedContentLabelIds() {
return excludedContentLabelIds;
}
/**
* The sensitive content category label IDs excluded in this configuration. Bid requests for
* inventory with any of the specified content label IDs will not be sent. Refer to this file
* https://storage.googleapis.com/adx-rtb-dictionaries/content-labels.txt for category IDs.
* @param excludedContentLabelIds excludedContentLabelIds or {@code null} for none
*/
public PretargetingConfig setExcludedContentLabelIds(java.util.List excludedContentLabelIds) {
this.excludedContentLabelIds = excludedContentLabelIds;
return this;
}
/**
* The geos included or excluded in this configuration defined in https://storage.googleapis.com
* /adx-rtb-dictionaries/geo-table.csv
* @return value or {@code null} for none
*/
public NumericTargetingDimension getGeoTargeting() {
return geoTargeting;
}
/**
* The geos included or excluded in this configuration defined in https://storage.googleapis.com
* /adx-rtb-dictionaries/geo-table.csv
* @param geoTargeting geoTargeting or {@code null} for none
*/
public PretargetingConfig setGeoTargeting(NumericTargetingDimension geoTargeting) {
this.geoTargeting = geoTargeting;
return this;
}
/**
* Creative dimensions included by this configuration. Only bid requests eligible for at least one
* of the specified creative dimensions will be sent. An unset value allows all bid requests to be
* sent, regardless of creative dimension.
* @return value or {@code null} for none
*/
public java.util.List getIncludedCreativeDimensions() {
return includedCreativeDimensions;
}
/**
* Creative dimensions included by this configuration. Only bid requests eligible for at least one
* of the specified creative dimensions will be sent. An unset value allows all bid requests to be
* sent, regardless of creative dimension.
* @param includedCreativeDimensions includedCreativeDimensions or {@code null} for none
*/
public PretargetingConfig setIncludedCreativeDimensions(java.util.List includedCreativeDimensions) {
this.includedCreativeDimensions = includedCreativeDimensions;
return this;
}
/**
* Environments that are being included. Bid requests will not be sent for a given environment if
* it is not included. Further restrictions can be applied to included environments to target only
* a subset of its inventory. An unset value includes all environments.
* @return value or {@code null} for none
*/
public java.util.List getIncludedEnvironments() {
return includedEnvironments;
}
/**
* Environments that are being included. Bid requests will not be sent for a given environment if
* it is not included. Further restrictions can be applied to included environments to target only
* a subset of its inventory. An unset value includes all environments.
* @param includedEnvironments includedEnvironments or {@code null} for none
*/
public PretargetingConfig setIncludedEnvironments(java.util.List includedEnvironments) {
this.includedEnvironments = includedEnvironments;
return this;
}
/**
* Creative formats included by this configuration. Only bid requests eligible for at least one of
* the specified creative formats will be sent. An unset value will allow all bid requests to be
* sent, regardless of format.
* @return value or {@code null} for none
*/
public java.util.List getIncludedFormats() {
return includedFormats;
}
/**
* Creative formats included by this configuration. Only bid requests eligible for at least one of
* the specified creative formats will be sent. An unset value will allow all bid requests to be
* sent, regardless of format.
* @param includedFormats includedFormats or {@code null} for none
*/
public PretargetingConfig setIncludedFormats(java.util.List includedFormats) {
this.includedFormats = includedFormats;
return this;
}
/**
* The languages included in this configuration, represented by their language code. See
* https://developers.google.com/adwords/api/docs/appendix/languagecodes.
* @return value or {@code null} for none
*/
public java.util.List getIncludedLanguages() {
return includedLanguages;
}
/**
* The languages included in this configuration, represented by their language code. See
* https://developers.google.com/adwords/api/docs/appendix/languagecodes.
* @param includedLanguages includedLanguages or {@code null} for none
*/
public PretargetingConfig setIncludedLanguages(java.util.List includedLanguages) {
this.includedLanguages = includedLanguages;
return this;
}
/**
* The mobile operating systems included in this configuration as defined in
* https://storage.googleapis.com/adx-rtb-dictionaries/mobile-os.csv
* @return value or {@code null} for none
*/
public java.util.List getIncludedMobileOperatingSystemIds() {
return includedMobileOperatingSystemIds;
}
/**
* The mobile operating systems included in this configuration as defined in
* https://storage.googleapis.com/adx-rtb-dictionaries/mobile-os.csv
* @param includedMobileOperatingSystemIds includedMobileOperatingSystemIds or {@code null} for none
*/
public PretargetingConfig setIncludedMobileOperatingSystemIds(java.util.List includedMobileOperatingSystemIds) {
this.includedMobileOperatingSystemIds = includedMobileOperatingSystemIds;
return this;
}
/**
* The platforms included by this configration. Bid requests for devices with the specified
* platform types will be sent. An unset value allows all bid requests to be sent, regardless of
* platform.
* @return value or {@code null} for none
*/
public java.util.List getIncludedPlatforms() {
return includedPlatforms;
}
/**
* The platforms included by this configration. Bid requests for devices with the specified
* platform types will be sent. An unset value allows all bid requests to be sent, regardless of
* platform.
* @param includedPlatforms includedPlatforms or {@code null} for none
*/
public PretargetingConfig setIncludedPlatforms(java.util.List includedPlatforms) {
this.includedPlatforms = includedPlatforms;
return this;
}
/**
* User identifier types included in this configuration. At least one of the user identifier types
* specified in this list must be available for the bid request to be sent.
* @return value or {@code null} for none
*/
public java.util.List getIncludedUserIdTypes() {
return includedUserIdTypes;
}
/**
* User identifier types included in this configuration. At least one of the user identifier types
* specified in this list must be available for the bid request to be sent.
* @param includedUserIdTypes includedUserIdTypes or {@code null} for none
*/
public PretargetingConfig setIncludedUserIdTypes(java.util.List includedUserIdTypes) {
this.includedUserIdTypes = includedUserIdTypes;
return this;
}
/**
* The interstitial targeting specified for this configuration. The unset value will allow bid
* requests to be sent regardless of whether they are for interstitials or not.
* @return value or {@code null} for none
*/
public java.lang.String getInterstitialTargeting() {
return interstitialTargeting;
}
/**
* The interstitial targeting specified for this configuration. The unset value will allow bid
* requests to be sent regardless of whether they are for interstitials or not.
* @param interstitialTargeting interstitialTargeting or {@code null} for none
*/
public PretargetingConfig setInterstitialTargeting(java.lang.String interstitialTargeting) {
this.interstitialTargeting = interstitialTargeting;
return this;
}
/**
* Output only. Existing included or excluded geos that are invalid. Previously targeted geos may
* become invalid due to privacy restrictions.
* @return value or {@code null} for none
*/
public java.util.List getInvalidGeoIds() {
return invalidGeoIds;
}
/**
* Output only. Existing included or excluded geos that are invalid. Previously targeted geos may
* become invalid due to privacy restrictions.
* @param invalidGeoIds invalidGeoIds or {@code null} for none
*/
public PretargetingConfig setInvalidGeoIds(java.util.List invalidGeoIds) {
this.invalidGeoIds = invalidGeoIds;
return this;
}
/**
* The maximum QPS threshold for this configuration. The bidder should receive no more than this
* number of bid requests matching this configuration per second across all their bidding
* endpoints among all trading locations. Further information available at
* https://developers.google.com/authorized-buyers/rtb/peer-guide
* @return value or {@code null} for none
*/
public java.lang.Long getMaximumQps() {
return maximumQps;
}
/**
* The maximum QPS threshold for this configuration. The bidder should receive no more than this
* number of bid requests matching this configuration per second across all their bidding
* endpoints among all trading locations. Further information available at
* https://developers.google.com/authorized-buyers/rtb/peer-guide
* @param maximumQps maximumQps or {@code null} for none
*/
public PretargetingConfig setMaximumQps(java.lang.Long maximumQps) {
this.maximumQps = maximumQps;
return this;
}
/**
* The targeted minimum viewability decile, ranging in values [0, 10]. A value of 5 means that the
* configuration will only match adslots for which we predict at least 50% viewability. Values >
* 10 will be rounded down to 10. An unset value or a value of 0 indicates that bid requests will
* be sent regardless of viewability.
* @return value or {@code null} for none
*/
public java.lang.Integer getMinimumViewabilityDecile() {
return minimumViewabilityDecile;
}
/**
* The targeted minimum viewability decile, ranging in values [0, 10]. A value of 5 means that the
* configuration will only match adslots for which we predict at least 50% viewability. Values >
* 10 will be rounded down to 10. An unset value or a value of 0 indicates that bid requests will
* be sent regardless of viewability.
* @param minimumViewabilityDecile minimumViewabilityDecile or {@code null} for none
*/
public PretargetingConfig setMinimumViewabilityDecile(java.lang.Integer minimumViewabilityDecile) {
this.minimumViewabilityDecile = minimumViewabilityDecile;
return this;
}
/**
* Output only. Name of the pretargeting configuration that must follow the pattern
* `bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Name of the pretargeting configuration that must follow the pattern
* `bidders/{bidder_account_id}/pretargetingConfigs/{config_id}`
* @param name name or {@code null} for none
*/
public PretargetingConfig setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Targeting on a subset of publisher inventory. Publishers can either be targeted positively (bid
* requests will be sent only if the publisher is listed in the targeting dimension) or negatively
* (bid requests will be sent only if the publisher is not listed in the targeting dimension). A
* maximum of 10,000 publisher IDs can be targeted. Publisher IDs are found in
* [ads.txt](https://iabtechlab.com/ads-txt/) / [app-ads.txt](https://iabtechlab.com/app-ads-txt/)
* and in bid requests in the `BidRequest.publisher_id` field on the [Google RTB
* protocol](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-proto)
* or the `BidRequest.site.publisher.id` / `BidRequest.app.publisher.id` field on the [OpenRTB
* protocol](https://developers.google.com/authorized-buyers/rtb/downloads/openrtb-adx-proto).
* Publisher IDs will be returned in the order that they were entered.
* @return value or {@code null} for none
*/
public StringTargetingDimension getPublisherTargeting() {
return publisherTargeting;
}
/**
* Targeting on a subset of publisher inventory. Publishers can either be targeted positively (bid
* requests will be sent only if the publisher is listed in the targeting dimension) or negatively
* (bid requests will be sent only if the publisher is not listed in the targeting dimension). A
* maximum of 10,000 publisher IDs can be targeted. Publisher IDs are found in
* [ads.txt](https://iabtechlab.com/ads-txt/) / [app-ads.txt](https://iabtechlab.com/app-ads-txt/)
* and in bid requests in the `BidRequest.publisher_id` field on the [Google RTB
* protocol](https://developers.google.com/authorized-buyers/rtb/downloads/realtime-bidding-proto)
* or the `BidRequest.site.publisher.id` / `BidRequest.app.publisher.id` field on the [OpenRTB
* protocol](https://developers.google.com/authorized-buyers/rtb/downloads/openrtb-adx-proto).
* Publisher IDs will be returned in the order that they were entered.
* @param publisherTargeting publisherTargeting or {@code null} for none
*/
public PretargetingConfig setPublisherTargeting(StringTargetingDimension publisherTargeting) {
this.publisherTargeting = publisherTargeting;
return this;
}
/**
* Output only. The state of this pretargeting configuration.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. The state of this pretargeting configuration.
* @param state state or {@code null} for none
*/
public PretargetingConfig setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* The remarketing lists included or excluded in this configuration as defined in UserList.
* @return value or {@code null} for none
*/
public NumericTargetingDimension getUserListTargeting() {
return userListTargeting;
}
/**
* The remarketing lists included or excluded in this configuration as defined in UserList.
* @param userListTargeting userListTargeting or {@code null} for none
*/
public PretargetingConfig setUserListTargeting(NumericTargetingDimension userListTargeting) {
this.userListTargeting = userListTargeting;
return this;
}
/**
* The verticals included or excluded in this configuration as defined in
* https://developers.google.com/authorized-buyers/rtb/downloads/publisher-verticals
* @return value or {@code null} for none
*/
public NumericTargetingDimension getVerticalTargeting() {
return verticalTargeting;
}
/**
* The verticals included or excluded in this configuration as defined in
* https://developers.google.com/authorized-buyers/rtb/downloads/publisher-verticals
* @param verticalTargeting verticalTargeting or {@code null} for none
*/
public PretargetingConfig setVerticalTargeting(NumericTargetingDimension verticalTargeting) {
this.verticalTargeting = verticalTargeting;
return this;
}
/**
* Targeting on a subset of site inventory. If WEB is listed in included_environments, the
* specified targeting is applied. A maximum of 50,000 site URLs can be targeted. An unset value
* for targeting allows all web-based bid requests to be sent. Sites can either be targeting
* positively (bid requests will be sent only if the destination site is listed in the targeting
* dimension) or negatively (bid requests will be sent only if the destination site is not listed
* in the pretargeting configuration).
* @return value or {@code null} for none
*/
public StringTargetingDimension getWebTargeting() {
return webTargeting;
}
/**
* Targeting on a subset of site inventory. If WEB is listed in included_environments, the
* specified targeting is applied. A maximum of 50,000 site URLs can be targeted. An unset value
* for targeting allows all web-based bid requests to be sent. Sites can either be targeting
* positively (bid requests will be sent only if the destination site is listed in the targeting
* dimension) or negatively (bid requests will be sent only if the destination site is not listed
* in the pretargeting configuration).
* @param webTargeting webTargeting or {@code null} for none
*/
public PretargetingConfig setWebTargeting(StringTargetingDimension webTargeting) {
this.webTargeting = webTargeting;
return this;
}
@Override
public PretargetingConfig set(String fieldName, Object value) {
return (PretargetingConfig) super.set(fieldName, value);
}
@Override
public PretargetingConfig clone() {
return (PretargetingConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy