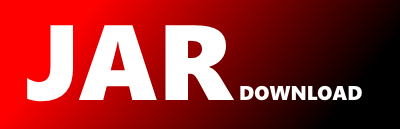
com.google.api.services.realtimebidding.v1.model.DestinationNotWorkingEvidence Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.realtimebidding.v1.model;
/**
* Evidence of the creative's destination URL not functioning properly or having been incorrectly
* set up.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Real-time Bidding API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DestinationNotWorkingEvidence extends com.google.api.client.json.GenericJson {
/**
* DNS lookup errors.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dnsError;
/**
* The full non-working URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String expandedUrl;
/**
* HTTP error code (for example, 404 or 5xx)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer httpError;
/**
* Page was crawled successfully, but was detected as either a page with no content or an error
* page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String invalidPage;
/**
* Approximate time when the ad destination was last checked.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String lastCheckTime;
/**
* Platform of the non-working URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String platform;
/**
* HTTP redirect chain error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String redirectionError;
/**
* Rejected because of malformed URLs or invalid requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String urlRejected;
/**
* DNS lookup errors.
* @return value or {@code null} for none
*/
public java.lang.String getDnsError() {
return dnsError;
}
/**
* DNS lookup errors.
* @param dnsError dnsError or {@code null} for none
*/
public DestinationNotWorkingEvidence setDnsError(java.lang.String dnsError) {
this.dnsError = dnsError;
return this;
}
/**
* The full non-working URL.
* @return value or {@code null} for none
*/
public java.lang.String getExpandedUrl() {
return expandedUrl;
}
/**
* The full non-working URL.
* @param expandedUrl expandedUrl or {@code null} for none
*/
public DestinationNotWorkingEvidence setExpandedUrl(java.lang.String expandedUrl) {
this.expandedUrl = expandedUrl;
return this;
}
/**
* HTTP error code (for example, 404 or 5xx)
* @return value or {@code null} for none
*/
public java.lang.Integer getHttpError() {
return httpError;
}
/**
* HTTP error code (for example, 404 or 5xx)
* @param httpError httpError or {@code null} for none
*/
public DestinationNotWorkingEvidence setHttpError(java.lang.Integer httpError) {
this.httpError = httpError;
return this;
}
/**
* Page was crawled successfully, but was detected as either a page with no content or an error
* page.
* @return value or {@code null} for none
*/
public java.lang.String getInvalidPage() {
return invalidPage;
}
/**
* Page was crawled successfully, but was detected as either a page with no content or an error
* page.
* @param invalidPage invalidPage or {@code null} for none
*/
public DestinationNotWorkingEvidence setInvalidPage(java.lang.String invalidPage) {
this.invalidPage = invalidPage;
return this;
}
/**
* Approximate time when the ad destination was last checked.
* @return value or {@code null} for none
*/
public String getLastCheckTime() {
return lastCheckTime;
}
/**
* Approximate time when the ad destination was last checked.
* @param lastCheckTime lastCheckTime or {@code null} for none
*/
public DestinationNotWorkingEvidence setLastCheckTime(String lastCheckTime) {
this.lastCheckTime = lastCheckTime;
return this;
}
/**
* Platform of the non-working URL.
* @return value or {@code null} for none
*/
public java.lang.String getPlatform() {
return platform;
}
/**
* Platform of the non-working URL.
* @param platform platform or {@code null} for none
*/
public DestinationNotWorkingEvidence setPlatform(java.lang.String platform) {
this.platform = platform;
return this;
}
/**
* HTTP redirect chain error.
* @return value or {@code null} for none
*/
public java.lang.String getRedirectionError() {
return redirectionError;
}
/**
* HTTP redirect chain error.
* @param redirectionError redirectionError or {@code null} for none
*/
public DestinationNotWorkingEvidence setRedirectionError(java.lang.String redirectionError) {
this.redirectionError = redirectionError;
return this;
}
/**
* Rejected because of malformed URLs or invalid requests.
* @return value or {@code null} for none
*/
public java.lang.String getUrlRejected() {
return urlRejected;
}
/**
* Rejected because of malformed URLs or invalid requests.
* @param urlRejected urlRejected or {@code null} for none
*/
public DestinationNotWorkingEvidence setUrlRejected(java.lang.String urlRejected) {
this.urlRejected = urlRejected;
return this;
}
@Override
public DestinationNotWorkingEvidence set(String fieldName, Object value) {
return (DestinationNotWorkingEvidence) super.set(fieldName, value);
}
@Override
public DestinationNotWorkingEvidence clone() {
return (DestinationNotWorkingEvidence) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy