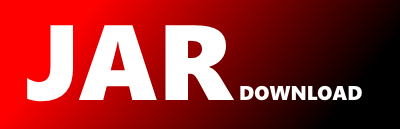
com.google.api.services.retail.v2.CloudRetail Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.retail.v2;
/**
* Service definition for CloudRetail (v2).
*
*
* Vertex AI Search for Retail API is made up of Retail Search, Browse and Recommendations. These discovery AI solutions help you implement personalized search, browse and recommendations, based on machine learning models, across your websites and mobile applications.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudRetailRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudRetail extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Vertex AI Search for Retail API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://retail.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://retail.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudRetail(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudRetail(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Projects.List request = retail.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Locations.List request = retail.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* An accessor for creating requests from the Catalogs collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Catalogs.List request = retail.catalogs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Catalogs catalogs() {
return new Catalogs();
}
/**
* The "catalogs" collection of methods.
*/
public class Catalogs {
/**
* Completes the specified prefix with keyword suggestions. This feature is only available for users
* who have Retail Search enabled. Enable Retail Search on Cloud Console before using this feature.
*
* Create a request for the method "catalogs.completeQuery".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link CompleteQuery#execute()} method to invoke the remote operation.
*
* @param catalog Required. Catalog for which the completion is performed. Full resource name of catalog, such as
* `projects/locations/global/catalogs/default_catalog`.
* @return the request
*/
public CompleteQuery completeQuery(java.lang.String catalog) throws java.io.IOException {
CompleteQuery result = new CompleteQuery(catalog);
initialize(result);
return result;
}
public class CompleteQuery extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+catalog}:completeQuery";
private final java.util.regex.Pattern CATALOG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Completes the specified prefix with keyword suggestions. This feature is only available for
* users who have Retail Search enabled. Enable Retail Search on Cloud Console before using this
* feature.
*
* Create a request for the method "catalogs.completeQuery".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link CompleteQuery#execute()} method to invoke the remote operation.
* {@link CompleteQuery#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param catalog Required. Catalog for which the completion is performed. Full resource name of catalog, such as
* `projects/locations/global/catalogs/default_catalog`.
* @since 1.13
*/
protected CompleteQuery(java.lang.String catalog) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2CompleteQueryResponse.class);
this.catalog = com.google.api.client.util.Preconditions.checkNotNull(catalog, "Required parameter catalog must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public CompleteQuery set$Xgafv(java.lang.String $Xgafv) {
return (CompleteQuery) super.set$Xgafv($Xgafv);
}
@Override
public CompleteQuery setAccessToken(java.lang.String accessToken) {
return (CompleteQuery) super.setAccessToken(accessToken);
}
@Override
public CompleteQuery setAlt(java.lang.String alt) {
return (CompleteQuery) super.setAlt(alt);
}
@Override
public CompleteQuery setCallback(java.lang.String callback) {
return (CompleteQuery) super.setCallback(callback);
}
@Override
public CompleteQuery setFields(java.lang.String fields) {
return (CompleteQuery) super.setFields(fields);
}
@Override
public CompleteQuery setKey(java.lang.String key) {
return (CompleteQuery) super.setKey(key);
}
@Override
public CompleteQuery setOauthToken(java.lang.String oauthToken) {
return (CompleteQuery) super.setOauthToken(oauthToken);
}
@Override
public CompleteQuery setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CompleteQuery) super.setPrettyPrint(prettyPrint);
}
@Override
public CompleteQuery setQuotaUser(java.lang.String quotaUser) {
return (CompleteQuery) super.setQuotaUser(quotaUser);
}
@Override
public CompleteQuery setUploadType(java.lang.String uploadType) {
return (CompleteQuery) super.setUploadType(uploadType);
}
@Override
public CompleteQuery setUploadProtocol(java.lang.String uploadProtocol) {
return (CompleteQuery) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Catalog for which the completion is performed. Full resource name of catalog,
* such as `projects/locations/global/catalogs/default_catalog`.
*/
@com.google.api.client.util.Key
private java.lang.String catalog;
/** Required. Catalog for which the completion is performed. Full resource name of catalog, such as
`projects/locations/global/catalogs/default_catalog`.
*/
public java.lang.String getCatalog() {
return catalog;
}
/**
* Required. Catalog for which the completion is performed. Full resource name of catalog,
* such as `projects/locations/global/catalogs/default_catalog`.
*/
public CompleteQuery setCatalog(java.lang.String catalog) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.catalog = catalog;
return this;
}
/**
* Determines which dataset to use for fetching completion. "user-data" will use the
* imported dataset through CompletionService.ImportCompletionData. "cloud-retail" will
* use the dataset generated by cloud retail based on user events. If leave empty, it will
* use the "user-data". Current supported values: * user-data * cloud-retail: This option
* requires enabling auto-learning function first. See
* [guidelines](https://cloud.google.com/retail/docs/completion-overview#generated-
* completion-dataset).
*/
@com.google.api.client.util.Key
private java.lang.String dataset;
/** Determines which dataset to use for fetching completion. "user-data" will use the imported dataset
through CompletionService.ImportCompletionData. "cloud-retail" will use the dataset generated by
cloud retail based on user events. If leave empty, it will use the "user-data". Current supported
values: * user-data * cloud-retail: This option requires enabling auto-learning function first. See
[guidelines](https://cloud.google.com/retail/docs/completion-overview#generated-completion-
dataset).
*/
public java.lang.String getDataset() {
return dataset;
}
/**
* Determines which dataset to use for fetching completion. "user-data" will use the
* imported dataset through CompletionService.ImportCompletionData. "cloud-retail" will
* use the dataset generated by cloud retail based on user events. If leave empty, it will
* use the "user-data". Current supported values: * user-data * cloud-retail: This option
* requires enabling auto-learning function first. See
* [guidelines](https://cloud.google.com/retail/docs/completion-overview#generated-
* completion-dataset).
*/
public CompleteQuery setDataset(java.lang.String dataset) {
this.dataset = dataset;
return this;
}
/**
* The device type context for completion suggestions. We recommend that you leave this
* field empty. It can apply different suggestions on different device types, e.g.
* `DESKTOP`, `MOBILE`. If it is empty, the suggestions are across all device types.
* Supported formats: * `UNKNOWN_DEVICE_TYPE` * `DESKTOP` * `MOBILE` * A customized string
* starts with `OTHER_`, e.g. `OTHER_IPHONE`.
*/
@com.google.api.client.util.Key
private java.lang.String deviceType;
/** The device type context for completion suggestions. We recommend that you leave this field empty.
It can apply different suggestions on different device types, e.g. `DESKTOP`, `MOBILE`. If it is
empty, the suggestions are across all device types. Supported formats: * `UNKNOWN_DEVICE_TYPE` *
`DESKTOP` * `MOBILE` * A customized string starts with `OTHER_`, e.g. `OTHER_IPHONE`.
*/
public java.lang.String getDeviceType() {
return deviceType;
}
/**
* The device type context for completion suggestions. We recommend that you leave this
* field empty. It can apply different suggestions on different device types, e.g.
* `DESKTOP`, `MOBILE`. If it is empty, the suggestions are across all device types.
* Supported formats: * `UNKNOWN_DEVICE_TYPE` * `DESKTOP` * `MOBILE` * A customized string
* starts with `OTHER_`, e.g. `OTHER_IPHONE`.
*/
public CompleteQuery setDeviceType(java.lang.String deviceType) {
this.deviceType = deviceType;
return this;
}
/**
* If true, attribute suggestions are enabled and provided in the response. This field is
* only available for the "cloud-retail" dataset.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableAttributeSuggestions;
/** If true, attribute suggestions are enabled and provided in the response. This field is only
available for the "cloud-retail" dataset.
*/
public java.lang.Boolean getEnableAttributeSuggestions() {
return enableAttributeSuggestions;
}
/**
* If true, attribute suggestions are enabled and provided in the response. This field is
* only available for the "cloud-retail" dataset.
*/
public CompleteQuery setEnableAttributeSuggestions(java.lang.Boolean enableAttributeSuggestions) {
this.enableAttributeSuggestions = enableAttributeSuggestions;
return this;
}
/**
* The entity for customers who run multiple entities, domains, sites, or regions, for
* example, `Google US`, `Google Ads`, `Waymo`, `google.com`, `youtube.com`, etc. If this
* is set, it must be an exact match with UserEvent.entity to get per-entity autocomplete
* results.
*/
@com.google.api.client.util.Key
private java.lang.String entity;
/** The entity for customers who run multiple entities, domains, sites, or regions, for example,
`Google US`, `Google Ads`, `Waymo`, `google.com`, `youtube.com`, etc. If this is set, it must be an
exact match with UserEvent.entity to get per-entity autocomplete results.
*/
public java.lang.String getEntity() {
return entity;
}
/**
* The entity for customers who run multiple entities, domains, sites, or regions, for
* example, `Google US`, `Google Ads`, `Waymo`, `google.com`, `youtube.com`, etc. If this
* is set, it must be an exact match with UserEvent.entity to get per-entity autocomplete
* results.
*/
public CompleteQuery setEntity(java.lang.String entity) {
this.entity = entity;
return this;
}
/**
* Note that this field applies for `user-data` dataset only. For requests with `cloud-
* retail` dataset, setting this field has no effect. The language filters applied to the
* output suggestions. If set, it should contain the language of the query. If not set,
* suggestions are returned without considering language restrictions. This is the BCP-47
* language code, such as "en-US" or "sr-Latn". For more information, see [Tags for
* Identifying Languages](https://tools.ietf.org/html/bcp47). The maximum number of
* language codes is 3.
*/
@com.google.api.client.util.Key
private java.util.List languageCodes;
/** Note that this field applies for `user-data` dataset only. For requests with `cloud-retail`
dataset, setting this field has no effect. The language filters applied to the output suggestions.
If set, it should contain the language of the query. If not set, suggestions are returned without
considering language restrictions. This is the BCP-47 language code, such as "en-US" or "sr-Latn".
For more information, see [Tags for Identifying Languages](https://tools.ietf.org/html/bcp47). The
maximum number of language codes is 3.
*/
public java.util.List getLanguageCodes() {
return languageCodes;
}
/**
* Note that this field applies for `user-data` dataset only. For requests with `cloud-
* retail` dataset, setting this field has no effect. The language filters applied to the
* output suggestions. If set, it should contain the language of the query. If not set,
* suggestions are returned without considering language restrictions. This is the BCP-47
* language code, such as "en-US" or "sr-Latn". For more information, see [Tags for
* Identifying Languages](https://tools.ietf.org/html/bcp47). The maximum number of
* language codes is 3.
*/
public CompleteQuery setLanguageCodes(java.util.List languageCodes) {
this.languageCodes = languageCodes;
return this;
}
/**
* Completion max suggestions. If left unset or set to 0, then will fallback to the
* configured value CompletionConfig.max_suggestions. The maximum allowed max suggestions
* is 20. If it is set higher, it will be capped by 20.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxSuggestions;
/** Completion max suggestions. If left unset or set to 0, then will fallback to the configured value
CompletionConfig.max_suggestions. The maximum allowed max suggestions is 20. If it is set higher,
it will be capped by 20.
*/
public java.lang.Integer getMaxSuggestions() {
return maxSuggestions;
}
/**
* Completion max suggestions. If left unset or set to 0, then will fallback to the
* configured value CompletionConfig.max_suggestions. The maximum allowed max suggestions
* is 20. If it is set higher, it will be capped by 20.
*/
public CompleteQuery setMaxSuggestions(java.lang.Integer maxSuggestions) {
this.maxSuggestions = maxSuggestions;
return this;
}
/**
* Required. The query used to generate suggestions. The maximum number of allowed
* characters is 255.
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** Required. The query used to generate suggestions. The maximum number of allowed characters is 255.
*/
public java.lang.String getQuery() {
return query;
}
/**
* Required. The query used to generate suggestions. The maximum number of allowed
* characters is 255.
*/
public CompleteQuery setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* Required field. A unique identifier for tracking visitors. For example, this could be
* implemented with an HTTP cookie, which should be able to uniquely identify a visitor on
* a single device. This unique identifier should not change if the visitor logs in or out
* of the website. The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String visitorId;
/** Required field. A unique identifier for tracking visitors. For example, this could be implemented
with an HTTP cookie, which should be able to uniquely identify a visitor on a single device. This
unique identifier should not change if the visitor logs in or out of the website. The field must be
a UTF-8 encoded string with a length limit of 128 characters. Otherwise, an INVALID_ARGUMENT error
is returned.
*/
public java.lang.String getVisitorId() {
return visitorId;
}
/**
* Required field. A unique identifier for tracking visitors. For example, this could be
* implemented with an HTTP cookie, which should be able to uniquely identify a visitor on
* a single device. This unique identifier should not change if the visitor logs in or out
* of the website. The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*/
public CompleteQuery setVisitorId(java.lang.String visitorId) {
this.visitorId = visitorId;
return this;
}
@Override
public CompleteQuery set(String parameterName, Object value) {
return (CompleteQuery) super.set(parameterName, value);
}
}
/**
* Exports analytics metrics. `Operation.response` is of type `ExportAnalyticsMetricsResponse`.
* `Operation.metadata` is of type `ExportMetadata`.
*
* Create a request for the method "catalogs.exportAnalyticsMetrics".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link ExportAnalyticsMetrics#execute()} method to invoke the remote
* operation.
*
* @param catalog Required. Full resource name of the parent catalog. Expected format: `projects/locations/catalogs`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ExportAnalyticsMetricsRequest}
* @return the request
*/
public ExportAnalyticsMetrics exportAnalyticsMetrics(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ExportAnalyticsMetricsRequest content) throws java.io.IOException {
ExportAnalyticsMetrics result = new ExportAnalyticsMetrics(catalog, content);
initialize(result);
return result;
}
public class ExportAnalyticsMetrics extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+catalog}:exportAnalyticsMetrics";
private final java.util.regex.Pattern CATALOG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Exports analytics metrics. `Operation.response` is of type `ExportAnalyticsMetricsResponse`.
* `Operation.metadata` is of type `ExportMetadata`.
*
* Create a request for the method "catalogs.exportAnalyticsMetrics".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link ExportAnalyticsMetrics#execute()} method to invoke the remote
* operation. {@link ExportAnalyticsMetrics#initialize(com.google.api.client.googleapis.servic
* es.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param catalog Required. Full resource name of the parent catalog. Expected format: `projects/locations/catalogs`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ExportAnalyticsMetricsRequest}
* @since 1.13
*/
protected ExportAnalyticsMetrics(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ExportAnalyticsMetricsRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.catalog = com.google.api.client.util.Preconditions.checkNotNull(catalog, "Required parameter catalog must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public ExportAnalyticsMetrics set$Xgafv(java.lang.String $Xgafv) {
return (ExportAnalyticsMetrics) super.set$Xgafv($Xgafv);
}
@Override
public ExportAnalyticsMetrics setAccessToken(java.lang.String accessToken) {
return (ExportAnalyticsMetrics) super.setAccessToken(accessToken);
}
@Override
public ExportAnalyticsMetrics setAlt(java.lang.String alt) {
return (ExportAnalyticsMetrics) super.setAlt(alt);
}
@Override
public ExportAnalyticsMetrics setCallback(java.lang.String callback) {
return (ExportAnalyticsMetrics) super.setCallback(callback);
}
@Override
public ExportAnalyticsMetrics setFields(java.lang.String fields) {
return (ExportAnalyticsMetrics) super.setFields(fields);
}
@Override
public ExportAnalyticsMetrics setKey(java.lang.String key) {
return (ExportAnalyticsMetrics) super.setKey(key);
}
@Override
public ExportAnalyticsMetrics setOauthToken(java.lang.String oauthToken) {
return (ExportAnalyticsMetrics) super.setOauthToken(oauthToken);
}
@Override
public ExportAnalyticsMetrics setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportAnalyticsMetrics) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportAnalyticsMetrics setQuotaUser(java.lang.String quotaUser) {
return (ExportAnalyticsMetrics) super.setQuotaUser(quotaUser);
}
@Override
public ExportAnalyticsMetrics setUploadType(java.lang.String uploadType) {
return (ExportAnalyticsMetrics) super.setUploadType(uploadType);
}
@Override
public ExportAnalyticsMetrics setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportAnalyticsMetrics) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of the parent catalog. Expected format:
* `projects/locations/catalogs`
*/
@com.google.api.client.util.Key
private java.lang.String catalog;
/** Required. Full resource name of the parent catalog. Expected format: `projects/locations/catalogs`
*/
public java.lang.String getCatalog() {
return catalog;
}
/**
* Required. Full resource name of the parent catalog. Expected format:
* `projects/locations/catalogs`
*/
public ExportAnalyticsMetrics setCatalog(java.lang.String catalog) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.catalog = catalog;
return this;
}
@Override
public ExportAnalyticsMetrics set(String parameterName, Object value) {
return (ExportAnalyticsMetrics) super.set(parameterName, value);
}
}
/**
* Gets an AttributesConfig.
*
* Create a request for the method "catalogs.getAttributesConfig".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link GetAttributesConfig#execute()} method to invoke the remote operation.
*
* @param name Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @return the request
*/
public GetAttributesConfig getAttributesConfig(java.lang.String name) throws java.io.IOException {
GetAttributesConfig result = new GetAttributesConfig(name);
initialize(result);
return result;
}
public class GetAttributesConfig extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
/**
* Gets an AttributesConfig.
*
* Create a request for the method "catalogs.getAttributesConfig".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link GetAttributesConfig#execute()} method to invoke the remote
* operation. {@link GetAttributesConfig#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @since 1.13
*/
protected GetAttributesConfig(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAttributesConfig set$Xgafv(java.lang.String $Xgafv) {
return (GetAttributesConfig) super.set$Xgafv($Xgafv);
}
@Override
public GetAttributesConfig setAccessToken(java.lang.String accessToken) {
return (GetAttributesConfig) super.setAccessToken(accessToken);
}
@Override
public GetAttributesConfig setAlt(java.lang.String alt) {
return (GetAttributesConfig) super.setAlt(alt);
}
@Override
public GetAttributesConfig setCallback(java.lang.String callback) {
return (GetAttributesConfig) super.setCallback(callback);
}
@Override
public GetAttributesConfig setFields(java.lang.String fields) {
return (GetAttributesConfig) super.setFields(fields);
}
@Override
public GetAttributesConfig setKey(java.lang.String key) {
return (GetAttributesConfig) super.setKey(key);
}
@Override
public GetAttributesConfig setOauthToken(java.lang.String oauthToken) {
return (GetAttributesConfig) super.setOauthToken(oauthToken);
}
@Override
public GetAttributesConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAttributesConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAttributesConfig setQuotaUser(java.lang.String quotaUser) {
return (GetAttributesConfig) super.setQuotaUser(quotaUser);
}
@Override
public GetAttributesConfig setUploadType(java.lang.String uploadType) {
return (GetAttributesConfig) super.setUploadType(uploadType);
}
@Override
public GetAttributesConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAttributesConfig) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/locat
* ions/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Full AttributesConfig resource name. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/locat
* ions/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public GetAttributesConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
this.name = name;
return this;
}
@Override
public GetAttributesConfig set(String parameterName, Object value) {
return (GetAttributesConfig) super.set(parameterName, value);
}
}
/**
* Gets a CompletionConfig.
*
* Create a request for the method "catalogs.getCompletionConfig".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link GetCompletionConfig#execute()} method to invoke the remote operation.
*
* @param name Required. Full CompletionConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/completionConfig`
* @return the request
*/
public GetCompletionConfig getCompletionConfig(java.lang.String name) throws java.io.IOException {
GetCompletionConfig result = new GetCompletionConfig(name);
initialize(result);
return result;
}
public class GetCompletionConfig extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/completionConfig$");
/**
* Gets a CompletionConfig.
*
* Create a request for the method "catalogs.getCompletionConfig".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link GetCompletionConfig#execute()} method to invoke the remote
* operation. {@link GetCompletionConfig#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. Full CompletionConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/completionConfig`
* @since 1.13
*/
protected GetCompletionConfig(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2CompletionConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/completionConfig$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetCompletionConfig set$Xgafv(java.lang.String $Xgafv) {
return (GetCompletionConfig) super.set$Xgafv($Xgafv);
}
@Override
public GetCompletionConfig setAccessToken(java.lang.String accessToken) {
return (GetCompletionConfig) super.setAccessToken(accessToken);
}
@Override
public GetCompletionConfig setAlt(java.lang.String alt) {
return (GetCompletionConfig) super.setAlt(alt);
}
@Override
public GetCompletionConfig setCallback(java.lang.String callback) {
return (GetCompletionConfig) super.setCallback(callback);
}
@Override
public GetCompletionConfig setFields(java.lang.String fields) {
return (GetCompletionConfig) super.setFields(fields);
}
@Override
public GetCompletionConfig setKey(java.lang.String key) {
return (GetCompletionConfig) super.setKey(key);
}
@Override
public GetCompletionConfig setOauthToken(java.lang.String oauthToken) {
return (GetCompletionConfig) super.setOauthToken(oauthToken);
}
@Override
public GetCompletionConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetCompletionConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public GetCompletionConfig setQuotaUser(java.lang.String quotaUser) {
return (GetCompletionConfig) super.setQuotaUser(quotaUser);
}
@Override
public GetCompletionConfig setUploadType(java.lang.String uploadType) {
return (GetCompletionConfig) super.setUploadType(uploadType);
}
@Override
public GetCompletionConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (GetCompletionConfig) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full CompletionConfig resource name. Format: `projects/{project_number}/locat
* ions/{location_id}/catalogs/{catalog_id}/completionConfig`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Full CompletionConfig resource name. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/completionConfig`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Full CompletionConfig resource name. Format: `projects/{project_number}/locat
* ions/{location_id}/catalogs/{catalog_id}/completionConfig`
*/
public GetCompletionConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/completionConfig$");
}
this.name = name;
return this;
}
@Override
public GetCompletionConfig set(String parameterName, Object value) {
return (GetCompletionConfig) super.set(parameterName, value);
}
}
/**
* Get which branch is currently default branch set by CatalogService.SetDefaultBranch method under
* a specified parent catalog.
*
* Create a request for the method "catalogs.getDefaultBranch".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link GetDefaultBranch#execute()} method to invoke the remote operation.
*
* @param catalog The parent catalog resource name, such as `projects/locations/global/catalogs/default_catalog`.
* @return the request
*/
public GetDefaultBranch getDefaultBranch(java.lang.String catalog) throws java.io.IOException {
GetDefaultBranch result = new GetDefaultBranch(catalog);
initialize(result);
return result;
}
public class GetDefaultBranch extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+catalog}:getDefaultBranch";
private final java.util.regex.Pattern CATALOG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Get which branch is currently default branch set by CatalogService.SetDefaultBranch method
* under a specified parent catalog.
*
* Create a request for the method "catalogs.getDefaultBranch".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link GetDefaultBranch#execute()} method to invoke the remote operation.
* {@link GetDefaultBranch#initialize(com.google.api.client.googleapis.services.AbstractGoogle
* ClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param catalog The parent catalog resource name, such as `projects/locations/global/catalogs/default_catalog`.
* @since 1.13
*/
protected GetDefaultBranch(java.lang.String catalog) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GetDefaultBranchResponse.class);
this.catalog = com.google.api.client.util.Preconditions.checkNotNull(catalog, "Required parameter catalog must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetDefaultBranch set$Xgafv(java.lang.String $Xgafv) {
return (GetDefaultBranch) super.set$Xgafv($Xgafv);
}
@Override
public GetDefaultBranch setAccessToken(java.lang.String accessToken) {
return (GetDefaultBranch) super.setAccessToken(accessToken);
}
@Override
public GetDefaultBranch setAlt(java.lang.String alt) {
return (GetDefaultBranch) super.setAlt(alt);
}
@Override
public GetDefaultBranch setCallback(java.lang.String callback) {
return (GetDefaultBranch) super.setCallback(callback);
}
@Override
public GetDefaultBranch setFields(java.lang.String fields) {
return (GetDefaultBranch) super.setFields(fields);
}
@Override
public GetDefaultBranch setKey(java.lang.String key) {
return (GetDefaultBranch) super.setKey(key);
}
@Override
public GetDefaultBranch setOauthToken(java.lang.String oauthToken) {
return (GetDefaultBranch) super.setOauthToken(oauthToken);
}
@Override
public GetDefaultBranch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetDefaultBranch) super.setPrettyPrint(prettyPrint);
}
@Override
public GetDefaultBranch setQuotaUser(java.lang.String quotaUser) {
return (GetDefaultBranch) super.setQuotaUser(quotaUser);
}
@Override
public GetDefaultBranch setUploadType(java.lang.String uploadType) {
return (GetDefaultBranch) super.setUploadType(uploadType);
}
@Override
public GetDefaultBranch setUploadProtocol(java.lang.String uploadProtocol) {
return (GetDefaultBranch) super.setUploadProtocol(uploadProtocol);
}
/**
* The parent catalog resource name, such as
* `projects/locations/global/catalogs/default_catalog`.
*/
@com.google.api.client.util.Key
private java.lang.String catalog;
/** The parent catalog resource name, such as `projects/locations/global/catalogs/default_catalog`.
*/
public java.lang.String getCatalog() {
return catalog;
}
/**
* The parent catalog resource name, such as
* `projects/locations/global/catalogs/default_catalog`.
*/
public GetDefaultBranch setCatalog(java.lang.String catalog) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.catalog = catalog;
return this;
}
@Override
public GetDefaultBranch set(String parameterName, Object value) {
return (GetDefaultBranch) super.set(parameterName, value);
}
}
/**
* Manages overal generative question feature state -- enables toggling feature on and off.
*
* Create a request for the method "catalogs.getGenerativeQuestionFeature".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link GetGenerativeQuestionFeature#execute()} method to invoke the remote
* operation.
*
* @param catalog Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @return the request
*/
public GetGenerativeQuestionFeature getGenerativeQuestionFeature(java.lang.String catalog) throws java.io.IOException {
GetGenerativeQuestionFeature result = new GetGenerativeQuestionFeature(catalog);
initialize(result);
return result;
}
public class GetGenerativeQuestionFeature extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+catalog}/generativeQuestionFeature";
private final java.util.regex.Pattern CATALOG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Manages overal generative question feature state -- enables toggling feature on and off.
*
* Create a request for the method "catalogs.getGenerativeQuestionFeature".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link GetGenerativeQuestionFeature#execute()} method to invoke the remote
* operation. {@link GetGenerativeQuestionFeature#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param catalog Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @since 1.13
*/
protected GetGenerativeQuestionFeature(java.lang.String catalog) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionsFeatureConfig.class);
this.catalog = com.google.api.client.util.Preconditions.checkNotNull(catalog, "Required parameter catalog must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetGenerativeQuestionFeature set$Xgafv(java.lang.String $Xgafv) {
return (GetGenerativeQuestionFeature) super.set$Xgafv($Xgafv);
}
@Override
public GetGenerativeQuestionFeature setAccessToken(java.lang.String accessToken) {
return (GetGenerativeQuestionFeature) super.setAccessToken(accessToken);
}
@Override
public GetGenerativeQuestionFeature setAlt(java.lang.String alt) {
return (GetGenerativeQuestionFeature) super.setAlt(alt);
}
@Override
public GetGenerativeQuestionFeature setCallback(java.lang.String callback) {
return (GetGenerativeQuestionFeature) super.setCallback(callback);
}
@Override
public GetGenerativeQuestionFeature setFields(java.lang.String fields) {
return (GetGenerativeQuestionFeature) super.setFields(fields);
}
@Override
public GetGenerativeQuestionFeature setKey(java.lang.String key) {
return (GetGenerativeQuestionFeature) super.setKey(key);
}
@Override
public GetGenerativeQuestionFeature setOauthToken(java.lang.String oauthToken) {
return (GetGenerativeQuestionFeature) super.setOauthToken(oauthToken);
}
@Override
public GetGenerativeQuestionFeature setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetGenerativeQuestionFeature) super.setPrettyPrint(prettyPrint);
}
@Override
public GetGenerativeQuestionFeature setQuotaUser(java.lang.String quotaUser) {
return (GetGenerativeQuestionFeature) super.setQuotaUser(quotaUser);
}
@Override
public GetGenerativeQuestionFeature setUploadType(java.lang.String uploadType) {
return (GetGenerativeQuestionFeature) super.setUploadType(uploadType);
}
@Override
public GetGenerativeQuestionFeature setUploadProtocol(java.lang.String uploadProtocol) {
return (GetGenerativeQuestionFeature) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
@com.google.api.client.util.Key
private java.lang.String catalog;
/** Required. Resource name of the parent catalog. Format:
projects/{project}/locations/{location}/catalogs/{catalog}
*/
public java.lang.String getCatalog() {
return catalog;
}
/**
* Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
public GetGenerativeQuestionFeature setCatalog(java.lang.String catalog) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.catalog = catalog;
return this;
}
@Override
public GetGenerativeQuestionFeature set(String parameterName, Object value) {
return (GetGenerativeQuestionFeature) super.set(parameterName, value);
}
}
/**
* Lists all the Catalogs associated with the project.
*
* Create a request for the method "catalogs.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The account resource name with an associated location. If the caller does not have
* permission to list Catalogs under this location, regardless of whether or not this
* location exists, a PERMISSION_DENIED error is returned.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/catalogs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists all the Catalogs associated with the project.
*
* Create a request for the method "catalogs.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The account resource name with an associated location. If the caller does not have
* permission to list Catalogs under this location, regardless of whether or not this
* location exists, a PERMISSION_DENIED error is returned.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ListCatalogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account resource name with an associated location. If the caller does not
* have permission to list Catalogs under this location, regardless of whether or not this
* location exists, a PERMISSION_DENIED error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The account resource name with an associated location. If the caller does not have
permission to list Catalogs under this location, regardless of whether or not this location exists,
a PERMISSION_DENIED error is returned.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The account resource name with an associated location. If the caller does not
* have permission to list Catalogs under this location, regardless of whether or not this
* location exists, a PERMISSION_DENIED error is returned.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Maximum number of Catalogs to return. If unspecified, defaults to 50. The maximum
* allowed value is 1000. Values above 1000 will be coerced to 1000. If this field is
* negative, an INVALID_ARGUMENT is returned.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of Catalogs to return. If unspecified, defaults to 50. The maximum allowed value is
1000. Values above 1000 will be coerced to 1000. If this field is negative, an INVALID_ARGUMENT is
returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Maximum number of Catalogs to return. If unspecified, defaults to 50. The maximum
* allowed value is 1000. Values above 1000 will be coerced to 1000. If this field is
* negative, an INVALID_ARGUMENT is returned.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token ListCatalogsResponse.next_page_token, received from a previous
* CatalogService.ListCatalogs call. Provide this to retrieve the subsequent page. When
* paginating, all other parameters provided to CatalogService.ListCatalogs must match the
* call that provided the page token. Otherwise, an INVALID_ARGUMENT error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token ListCatalogsResponse.next_page_token, received from a previous
CatalogService.ListCatalogs call. Provide this to retrieve the subsequent page. When paginating,
all other parameters provided to CatalogService.ListCatalogs must match the call that provided the
page token. Otherwise, an INVALID_ARGUMENT error is returned.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token ListCatalogsResponse.next_page_token, received from a previous
* CatalogService.ListCatalogs call. Provide this to retrieve the subsequent page. When
* paginating, all other parameters provided to CatalogService.ListCatalogs must match the
* call that provided the page token. Otherwise, an INVALID_ARGUMENT error is returned.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the Catalogs.
*
* Create a request for the method "catalogs.patch".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. Immutable. The fully qualified resource name of the catalog.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Catalog}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Catalog content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Updates the Catalogs.
*
* Create a request for the method "catalogs.patch".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Immutable. The fully qualified resource name of the catalog.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Catalog}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Catalog content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Catalog.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Required. Immutable. The fully qualified resource name of the catalog. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Immutable. The fully qualified resource name of the catalog.
*/
public java.lang.String getName() {
return name;
}
/** Required. Immutable. The fully qualified resource name of the catalog. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.name = name;
return this;
}
/**
* Indicates which fields in the provided Catalog to update. If an unsupported or unknown
* field is provided, an INVALID_ARGUMENT error is returned.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Indicates which fields in the provided Catalog to update. If an unsupported or unknown field is
provided, an INVALID_ARGUMENT error is returned.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Indicates which fields in the provided Catalog to update. If an unsupported or unknown
* field is provided, an INVALID_ARGUMENT error is returned.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Set a specified branch id as default branch. API methods such as SearchService.Search,
* ProductService.GetProduct, ProductService.ListProducts will treat requests using "default_branch"
* to the actual branch id set as default. For example, if `projects/locations/catalogs/branches/1`
* is set as default, setting SearchRequest.branch to
* `projects/locations/catalogs/branches/default_branch` is equivalent to setting
* SearchRequest.branch to `projects/locations/catalogs/branches/1`. Using multiple branches can be
* useful when developers would like to have a staging branch to test and verify for future usage.
* When it becomes ready, developers switch on the staging branch using this API while keeping using
* `projects/locations/catalogs/branches/default_branch` as SearchRequest.branch to route the
* traffic to this staging branch. CAUTION: If you have live predict/search traffic, switching the
* default branch could potentially cause outages if the ID space of the new branch is very
* different from the old one. More specifically: * PredictionService will only return product IDs
* from branch {newBranch}. * SearchService will only return product IDs from branch {newBranch} (if
* branch is not explicitly set). * UserEventService will only join events with products from branch
* {newBranch}.
*
* Create a request for the method "catalogs.setDefaultBranch".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link SetDefaultBranch#execute()} method to invoke the remote operation.
*
* @param catalog Full resource name of the catalog, such as `projects/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetDefaultBranchRequest}
* @return the request
*/
public SetDefaultBranch setDefaultBranch(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetDefaultBranchRequest content) throws java.io.IOException {
SetDefaultBranch result = new SetDefaultBranch(catalog, content);
initialize(result);
return result;
}
public class SetDefaultBranch extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+catalog}:setDefaultBranch";
private final java.util.regex.Pattern CATALOG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Set a specified branch id as default branch. API methods such as SearchService.Search,
* ProductService.GetProduct, ProductService.ListProducts will treat requests using
* "default_branch" to the actual branch id set as default. For example, if
* `projects/locations/catalogs/branches/1` is set as default, setting SearchRequest.branch to
* `projects/locations/catalogs/branches/default_branch` is equivalent to setting
* SearchRequest.branch to `projects/locations/catalogs/branches/1`. Using multiple branches can
* be useful when developers would like to have a staging branch to test and verify for future
* usage. When it becomes ready, developers switch on the staging branch using this API while
* keeping using `projects/locations/catalogs/branches/default_branch` as SearchRequest.branch to
* route the traffic to this staging branch. CAUTION: If you have live predict/search traffic,
* switching the default branch could potentially cause outages if the ID space of the new branch
* is very different from the old one. More specifically: * PredictionService will only return
* product IDs from branch {newBranch}. * SearchService will only return product IDs from branch
* {newBranch} (if branch is not explicitly set). * UserEventService will only join events with
* products from branch {newBranch}.
*
* Create a request for the method "catalogs.setDefaultBranch".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link SetDefaultBranch#execute()} method to invoke the remote operation.
* {@link SetDefaultBranch#initialize(com.google.api.client.googleapis.services.AbstractGoogle
* ClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param catalog Full resource name of the catalog, such as `projects/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetDefaultBranchRequest}
* @since 1.13
*/
protected SetDefaultBranch(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetDefaultBranchRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleProtobufEmpty.class);
this.catalog = com.google.api.client.util.Preconditions.checkNotNull(catalog, "Required parameter catalog must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public SetDefaultBranch set$Xgafv(java.lang.String $Xgafv) {
return (SetDefaultBranch) super.set$Xgafv($Xgafv);
}
@Override
public SetDefaultBranch setAccessToken(java.lang.String accessToken) {
return (SetDefaultBranch) super.setAccessToken(accessToken);
}
@Override
public SetDefaultBranch setAlt(java.lang.String alt) {
return (SetDefaultBranch) super.setAlt(alt);
}
@Override
public SetDefaultBranch setCallback(java.lang.String callback) {
return (SetDefaultBranch) super.setCallback(callback);
}
@Override
public SetDefaultBranch setFields(java.lang.String fields) {
return (SetDefaultBranch) super.setFields(fields);
}
@Override
public SetDefaultBranch setKey(java.lang.String key) {
return (SetDefaultBranch) super.setKey(key);
}
@Override
public SetDefaultBranch setOauthToken(java.lang.String oauthToken) {
return (SetDefaultBranch) super.setOauthToken(oauthToken);
}
@Override
public SetDefaultBranch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetDefaultBranch) super.setPrettyPrint(prettyPrint);
}
@Override
public SetDefaultBranch setQuotaUser(java.lang.String quotaUser) {
return (SetDefaultBranch) super.setQuotaUser(quotaUser);
}
@Override
public SetDefaultBranch setUploadType(java.lang.String uploadType) {
return (SetDefaultBranch) super.setUploadType(uploadType);
}
@Override
public SetDefaultBranch setUploadProtocol(java.lang.String uploadProtocol) {
return (SetDefaultBranch) super.setUploadProtocol(uploadProtocol);
}
/**
* Full resource name of the catalog, such as
* `projects/locations/global/catalogs/default_catalog`.
*/
@com.google.api.client.util.Key
private java.lang.String catalog;
/** Full resource name of the catalog, such as `projects/locations/global/catalogs/default_catalog`.
*/
public java.lang.String getCatalog() {
return catalog;
}
/**
* Full resource name of the catalog, such as
* `projects/locations/global/catalogs/default_catalog`.
*/
public SetDefaultBranch setCatalog(java.lang.String catalog) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.catalog = catalog;
return this;
}
@Override
public SetDefaultBranch set(String parameterName, Object value) {
return (SetDefaultBranch) super.set(parameterName, value);
}
}
/**
* Updates the AttributesConfig. The catalog attributes in the request will be updated in the
* catalog, or inserted if they do not exist. Existing catalog attributes not included in the
* request will remain unchanged. Attributes that are assigned to products, but do not exist at the
* catalog level, are always included in the response. The product attribute is assigned default
* values for missing catalog attribute fields, e.g., searchable and dynamic facetable options.
*
* Create a request for the method "catalogs.updateAttributesConfig".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link UpdateAttributesConfig#execute()} method to invoke the remote
* operation.
*
* @param name Required. Immutable. The fully qualified resource name of the attribute config. Format:
* `projects/locations/catalogs/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig}
* @return the request
*/
public UpdateAttributesConfig updateAttributesConfig(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig content) throws java.io.IOException {
UpdateAttributesConfig result = new UpdateAttributesConfig(name, content);
initialize(result);
return result;
}
public class UpdateAttributesConfig extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
/**
* Updates the AttributesConfig. The catalog attributes in the request will be updated in the
* catalog, or inserted if they do not exist. Existing catalog attributes not included in the
* request will remain unchanged. Attributes that are assigned to products, but do not exist at
* the catalog level, are always included in the response. The product attribute is assigned
* default values for missing catalog attribute fields, e.g., searchable and dynamic facetable
* options.
*
* Create a request for the method "catalogs.updateAttributesConfig".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link UpdateAttributesConfig#execute()} method to invoke the remote
* operation. {@link UpdateAttributesConfig#initialize(com.google.api.client.googleapis.servic
* es.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. Immutable. The fully qualified resource name of the attribute config. Format:
* `projects/locations/catalogs/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig}
* @since 1.13
*/
protected UpdateAttributesConfig(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
}
@Override
public UpdateAttributesConfig set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAttributesConfig) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAttributesConfig setAccessToken(java.lang.String accessToken) {
return (UpdateAttributesConfig) super.setAccessToken(accessToken);
}
@Override
public UpdateAttributesConfig setAlt(java.lang.String alt) {
return (UpdateAttributesConfig) super.setAlt(alt);
}
@Override
public UpdateAttributesConfig setCallback(java.lang.String callback) {
return (UpdateAttributesConfig) super.setCallback(callback);
}
@Override
public UpdateAttributesConfig setFields(java.lang.String fields) {
return (UpdateAttributesConfig) super.setFields(fields);
}
@Override
public UpdateAttributesConfig setKey(java.lang.String key) {
return (UpdateAttributesConfig) super.setKey(key);
}
@Override
public UpdateAttributesConfig setOauthToken(java.lang.String oauthToken) {
return (UpdateAttributesConfig) super.setOauthToken(oauthToken);
}
@Override
public UpdateAttributesConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAttributesConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAttributesConfig setQuotaUser(java.lang.String quotaUser) {
return (UpdateAttributesConfig) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAttributesConfig setUploadType(java.lang.String uploadType) {
return (UpdateAttributesConfig) super.setUploadType(uploadType);
}
@Override
public UpdateAttributesConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAttributesConfig) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Immutable. The fully qualified resource name of the attribute config. Format:
* `projects/locations/catalogs/attributesConfig`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Immutable. The fully qualified resource name of the attribute config. Format:
`projects/locations/catalogs/attributesConfig`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Immutable. The fully qualified resource name of the attribute config. Format:
* `projects/locations/catalogs/attributesConfig`
*/
public UpdateAttributesConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
this.name = name;
return this;
}
/**
* Indicates which fields in the provided AttributesConfig to update. The following is the
* only supported field: * AttributesConfig.catalog_attributes If not set, all supported
* fields are updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Indicates which fields in the provided AttributesConfig to update. The following is the only
supported field: * AttributesConfig.catalog_attributes If not set, all supported fields are
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Indicates which fields in the provided AttributesConfig to update. The following is the
* only supported field: * AttributesConfig.catalog_attributes If not set, all supported
* fields are updated.
*/
public UpdateAttributesConfig setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAttributesConfig set(String parameterName, Object value) {
return (UpdateAttributesConfig) super.set(parameterName, value);
}
}
/**
* Updates the CompletionConfigs.
*
* Create a request for the method "catalogs.updateCompletionConfig".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link UpdateCompletionConfig#execute()} method to invoke the remote
* operation.
*
* @param name Required. Immutable. Fully qualified name `projects/locations/catalogs/completionConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2CompletionConfig}
* @return the request
*/
public UpdateCompletionConfig updateCompletionConfig(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2CompletionConfig content) throws java.io.IOException {
UpdateCompletionConfig result = new UpdateCompletionConfig(name, content);
initialize(result);
return result;
}
public class UpdateCompletionConfig extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/completionConfig$");
/**
* Updates the CompletionConfigs.
*
* Create a request for the method "catalogs.updateCompletionConfig".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link UpdateCompletionConfig#execute()} method to invoke the remote
* operation. {@link UpdateCompletionConfig#initialize(com.google.api.client.googleapis.servic
* es.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. Immutable. Fully qualified name `projects/locations/catalogs/completionConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2CompletionConfig}
* @since 1.13
*/
protected UpdateCompletionConfig(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2CompletionConfig content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2CompletionConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/completionConfig$");
}
}
@Override
public UpdateCompletionConfig set$Xgafv(java.lang.String $Xgafv) {
return (UpdateCompletionConfig) super.set$Xgafv($Xgafv);
}
@Override
public UpdateCompletionConfig setAccessToken(java.lang.String accessToken) {
return (UpdateCompletionConfig) super.setAccessToken(accessToken);
}
@Override
public UpdateCompletionConfig setAlt(java.lang.String alt) {
return (UpdateCompletionConfig) super.setAlt(alt);
}
@Override
public UpdateCompletionConfig setCallback(java.lang.String callback) {
return (UpdateCompletionConfig) super.setCallback(callback);
}
@Override
public UpdateCompletionConfig setFields(java.lang.String fields) {
return (UpdateCompletionConfig) super.setFields(fields);
}
@Override
public UpdateCompletionConfig setKey(java.lang.String key) {
return (UpdateCompletionConfig) super.setKey(key);
}
@Override
public UpdateCompletionConfig setOauthToken(java.lang.String oauthToken) {
return (UpdateCompletionConfig) super.setOauthToken(oauthToken);
}
@Override
public UpdateCompletionConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateCompletionConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateCompletionConfig setQuotaUser(java.lang.String quotaUser) {
return (UpdateCompletionConfig) super.setQuotaUser(quotaUser);
}
@Override
public UpdateCompletionConfig setUploadType(java.lang.String uploadType) {
return (UpdateCompletionConfig) super.setUploadType(uploadType);
}
@Override
public UpdateCompletionConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateCompletionConfig) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Immutable. Fully qualified name
* `projects/locations/catalogs/completionConfig`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Immutable. Fully qualified name `projects/locations/catalogs/completionConfig`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Immutable. Fully qualified name
* `projects/locations/catalogs/completionConfig`
*/
public UpdateCompletionConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/completionConfig$");
}
this.name = name;
return this;
}
/**
* Indicates which fields in the provided CompletionConfig to update. The following are
* the only supported fields: * CompletionConfig.matching_order *
* CompletionConfig.max_suggestions * CompletionConfig.min_prefix_length *
* CompletionConfig.auto_learning If not set, all supported fields are updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Indicates which fields in the provided CompletionConfig to update. The following are the only
supported fields: * CompletionConfig.matching_order * CompletionConfig.max_suggestions *
CompletionConfig.min_prefix_length * CompletionConfig.auto_learning If not set, all supported
fields are updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Indicates which fields in the provided CompletionConfig to update. The following are
* the only supported fields: * CompletionConfig.matching_order *
* CompletionConfig.max_suggestions * CompletionConfig.min_prefix_length *
* CompletionConfig.auto_learning If not set, all supported fields are updated.
*/
public UpdateCompletionConfig setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateCompletionConfig set(String parameterName, Object value) {
return (UpdateCompletionConfig) super.set(parameterName, value);
}
}
/**
* Allows management of individual questions.
*
* Create a request for the method "catalogs.updateGenerativeQuestion".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link UpdateGenerativeQuestion#execute()} method to invoke the remote
* operation.
*
* @param catalog Required. Resource name of the catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionConfig}
* @return the request
*/
public UpdateGenerativeQuestion updateGenerativeQuestion(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionConfig content) throws java.io.IOException {
UpdateGenerativeQuestion result = new UpdateGenerativeQuestion(catalog, content);
initialize(result);
return result;
}
public class UpdateGenerativeQuestion extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+catalog}/generativeQuestion";
private final java.util.regex.Pattern CATALOG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Allows management of individual questions.
*
* Create a request for the method "catalogs.updateGenerativeQuestion".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link UpdateGenerativeQuestion#execute()} method to invoke the remote
* operation. {@link UpdateGenerativeQuestion#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param catalog Required. Resource name of the catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionConfig}
* @since 1.13
*/
protected UpdateGenerativeQuestion(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionConfig content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionConfig.class);
this.catalog = com.google.api.client.util.Preconditions.checkNotNull(catalog, "Required parameter catalog must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public UpdateGenerativeQuestion set$Xgafv(java.lang.String $Xgafv) {
return (UpdateGenerativeQuestion) super.set$Xgafv($Xgafv);
}
@Override
public UpdateGenerativeQuestion setAccessToken(java.lang.String accessToken) {
return (UpdateGenerativeQuestion) super.setAccessToken(accessToken);
}
@Override
public UpdateGenerativeQuestion setAlt(java.lang.String alt) {
return (UpdateGenerativeQuestion) super.setAlt(alt);
}
@Override
public UpdateGenerativeQuestion setCallback(java.lang.String callback) {
return (UpdateGenerativeQuestion) super.setCallback(callback);
}
@Override
public UpdateGenerativeQuestion setFields(java.lang.String fields) {
return (UpdateGenerativeQuestion) super.setFields(fields);
}
@Override
public UpdateGenerativeQuestion setKey(java.lang.String key) {
return (UpdateGenerativeQuestion) super.setKey(key);
}
@Override
public UpdateGenerativeQuestion setOauthToken(java.lang.String oauthToken) {
return (UpdateGenerativeQuestion) super.setOauthToken(oauthToken);
}
@Override
public UpdateGenerativeQuestion setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateGenerativeQuestion) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateGenerativeQuestion setQuotaUser(java.lang.String quotaUser) {
return (UpdateGenerativeQuestion) super.setQuotaUser(quotaUser);
}
@Override
public UpdateGenerativeQuestion setUploadType(java.lang.String uploadType) {
return (UpdateGenerativeQuestion) super.setUploadType(uploadType);
}
@Override
public UpdateGenerativeQuestion setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateGenerativeQuestion) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
@com.google.api.client.util.Key
private java.lang.String catalog;
/** Required. Resource name of the catalog. Format:
projects/{project}/locations/{location}/catalogs/{catalog}
*/
public java.lang.String getCatalog() {
return catalog;
}
/**
* Required. Resource name of the catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
public UpdateGenerativeQuestion setCatalog(java.lang.String catalog) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.catalog = catalog;
return this;
}
/**
* Optional. Indicates which fields in the provided GenerativeQuestionConfig to update.
* The following are NOT supported: * GenerativeQuestionConfig.frequency If not set or
* empty, all supported fields are updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Indicates which fields in the provided GenerativeQuestionConfig to update. The following
are NOT supported: * GenerativeQuestionConfig.frequency If not set or empty, all supported fields
are updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Indicates which fields in the provided GenerativeQuestionConfig to update.
* The following are NOT supported: * GenerativeQuestionConfig.frequency If not set or
* empty, all supported fields are updated.
*/
public UpdateGenerativeQuestion setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateGenerativeQuestion set(String parameterName, Object value) {
return (UpdateGenerativeQuestion) super.set(parameterName, value);
}
}
/**
* Manages overal generative question feature state -- enables toggling feature on and off.
*
* Create a request for the method "catalogs.updateGenerativeQuestionFeature".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link UpdateGenerativeQuestionFeature#execute()} method to invoke the
* remote operation.
*
* @param catalog Required. Resource name of the affected catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionsFeatureConfig}
* @return the request
*/
public UpdateGenerativeQuestionFeature updateGenerativeQuestionFeature(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionsFeatureConfig content) throws java.io.IOException {
UpdateGenerativeQuestionFeature result = new UpdateGenerativeQuestionFeature(catalog, content);
initialize(result);
return result;
}
public class UpdateGenerativeQuestionFeature extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+catalog}/generativeQuestionFeature";
private final java.util.regex.Pattern CATALOG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Manages overal generative question feature state -- enables toggling feature on and off.
*
* Create a request for the method "catalogs.updateGenerativeQuestionFeature".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link UpdateGenerativeQuestionFeature#execute()} method to invoke the
* remote operation. {@link UpdateGenerativeQuestionFeature#initialize(com.google.api.client.g
* oogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param catalog Required. Resource name of the affected catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionsFeatureConfig}
* @since 1.13
*/
protected UpdateGenerativeQuestionFeature(java.lang.String catalog, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionsFeatureConfig content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2GenerativeQuestionsFeatureConfig.class);
this.catalog = com.google.api.client.util.Preconditions.checkNotNull(catalog, "Required parameter catalog must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public UpdateGenerativeQuestionFeature set$Xgafv(java.lang.String $Xgafv) {
return (UpdateGenerativeQuestionFeature) super.set$Xgafv($Xgafv);
}
@Override
public UpdateGenerativeQuestionFeature setAccessToken(java.lang.String accessToken) {
return (UpdateGenerativeQuestionFeature) super.setAccessToken(accessToken);
}
@Override
public UpdateGenerativeQuestionFeature setAlt(java.lang.String alt) {
return (UpdateGenerativeQuestionFeature) super.setAlt(alt);
}
@Override
public UpdateGenerativeQuestionFeature setCallback(java.lang.String callback) {
return (UpdateGenerativeQuestionFeature) super.setCallback(callback);
}
@Override
public UpdateGenerativeQuestionFeature setFields(java.lang.String fields) {
return (UpdateGenerativeQuestionFeature) super.setFields(fields);
}
@Override
public UpdateGenerativeQuestionFeature setKey(java.lang.String key) {
return (UpdateGenerativeQuestionFeature) super.setKey(key);
}
@Override
public UpdateGenerativeQuestionFeature setOauthToken(java.lang.String oauthToken) {
return (UpdateGenerativeQuestionFeature) super.setOauthToken(oauthToken);
}
@Override
public UpdateGenerativeQuestionFeature setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateGenerativeQuestionFeature) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateGenerativeQuestionFeature setQuotaUser(java.lang.String quotaUser) {
return (UpdateGenerativeQuestionFeature) super.setQuotaUser(quotaUser);
}
@Override
public UpdateGenerativeQuestionFeature setUploadType(java.lang.String uploadType) {
return (UpdateGenerativeQuestionFeature) super.setUploadType(uploadType);
}
@Override
public UpdateGenerativeQuestionFeature setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateGenerativeQuestionFeature) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the affected catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
@com.google.api.client.util.Key
private java.lang.String catalog;
/** Required. Resource name of the affected catalog. Format:
projects/{project}/locations/{location}/catalogs/{catalog}
*/
public java.lang.String getCatalog() {
return catalog;
}
/**
* Required. Resource name of the affected catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
public UpdateGenerativeQuestionFeature setCatalog(java.lang.String catalog) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CATALOG_PATTERN.matcher(catalog).matches(),
"Parameter catalog must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.catalog = catalog;
return this;
}
/**
* Optional. Indicates which fields in the provided GenerativeQuestionsFeatureConfig to
* update. If not set or empty, all supported fields are updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Indicates which fields in the provided GenerativeQuestionsFeatureConfig to update. If not
set or empty, all supported fields are updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Indicates which fields in the provided GenerativeQuestionsFeatureConfig to
* update. If not set or empty, all supported fields are updated.
*/
public UpdateGenerativeQuestionFeature setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateGenerativeQuestionFeature set(String parameterName, Object value) {
return (UpdateGenerativeQuestionFeature) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the AttributesConfig collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.AttributesConfig.List request = retail.attributesConfig().list(parameters ...)}
*
*
* @return the resource collection
*/
public AttributesConfig attributesConfig() {
return new AttributesConfig();
}
/**
* The "attributesConfig" collection of methods.
*/
public class AttributesConfig {
/**
* Adds the specified CatalogAttribute to the AttributesConfig. If the CatalogAttribute to add
* already exists, an ALREADY_EXISTS error is returned.
*
* Create a request for the method "attributesConfig.addCatalogAttribute".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link AddCatalogAttribute#execute()} method to invoke the remote operation.
*
* @param attributesConfig Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddCatalogAttributeRequest}
* @return the request
*/
public AddCatalogAttribute addCatalogAttribute(java.lang.String attributesConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddCatalogAttributeRequest content) throws java.io.IOException {
AddCatalogAttribute result = new AddCatalogAttribute(attributesConfig, content);
initialize(result);
return result;
}
public class AddCatalogAttribute extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+attributesConfig}:addCatalogAttribute";
private final java.util.regex.Pattern ATTRIBUTES_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
/**
* Adds the specified CatalogAttribute to the AttributesConfig. If the CatalogAttribute to add
* already exists, an ALREADY_EXISTS error is returned.
*
* Create a request for the method "attributesConfig.addCatalogAttribute".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link AddCatalogAttribute#execute()} method to invoke the remote
* operation. {@link AddCatalogAttribute#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param attributesConfig Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddCatalogAttributeRequest}
* @since 1.13
*/
protected AddCatalogAttribute(java.lang.String attributesConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddCatalogAttributeRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig.class);
this.attributesConfig = com.google.api.client.util.Preconditions.checkNotNull(attributesConfig, "Required parameter attributesConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ATTRIBUTES_CONFIG_PATTERN.matcher(attributesConfig).matches(),
"Parameter attributesConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
}
@Override
public AddCatalogAttribute set$Xgafv(java.lang.String $Xgafv) {
return (AddCatalogAttribute) super.set$Xgafv($Xgafv);
}
@Override
public AddCatalogAttribute setAccessToken(java.lang.String accessToken) {
return (AddCatalogAttribute) super.setAccessToken(accessToken);
}
@Override
public AddCatalogAttribute setAlt(java.lang.String alt) {
return (AddCatalogAttribute) super.setAlt(alt);
}
@Override
public AddCatalogAttribute setCallback(java.lang.String callback) {
return (AddCatalogAttribute) super.setCallback(callback);
}
@Override
public AddCatalogAttribute setFields(java.lang.String fields) {
return (AddCatalogAttribute) super.setFields(fields);
}
@Override
public AddCatalogAttribute setKey(java.lang.String key) {
return (AddCatalogAttribute) super.setKey(key);
}
@Override
public AddCatalogAttribute setOauthToken(java.lang.String oauthToken) {
return (AddCatalogAttribute) super.setOauthToken(oauthToken);
}
@Override
public AddCatalogAttribute setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddCatalogAttribute) super.setPrettyPrint(prettyPrint);
}
@Override
public AddCatalogAttribute setQuotaUser(java.lang.String quotaUser) {
return (AddCatalogAttribute) super.setQuotaUser(quotaUser);
}
@Override
public AddCatalogAttribute setUploadType(java.lang.String uploadType) {
return (AddCatalogAttribute) super.setUploadType(uploadType);
}
@Override
public AddCatalogAttribute setUploadProtocol(java.lang.String uploadProtocol) {
return (AddCatalogAttribute) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/loc
* ations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
@com.google.api.client.util.Key
private java.lang.String attributesConfig;
/** Required. Full AttributesConfig resource name. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public java.lang.String getAttributesConfig() {
return attributesConfig;
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/loc
* ations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public AddCatalogAttribute setAttributesConfig(java.lang.String attributesConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ATTRIBUTES_CONFIG_PATTERN.matcher(attributesConfig).matches(),
"Parameter attributesConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
this.attributesConfig = attributesConfig;
return this;
}
@Override
public AddCatalogAttribute set(String parameterName, Object value) {
return (AddCatalogAttribute) super.set(parameterName, value);
}
}
/**
* Removes the specified CatalogAttribute from the AttributesConfig. If the CatalogAttribute to
* remove does not exist, a NOT_FOUND error is returned.
*
* Create a request for the method "attributesConfig.removeCatalogAttribute".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link RemoveCatalogAttribute#execute()} method to invoke the remote
* operation.
*
* @param attributesConfig Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveCatalogAttributeRequest}
* @return the request
*/
public RemoveCatalogAttribute removeCatalogAttribute(java.lang.String attributesConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveCatalogAttributeRequest content) throws java.io.IOException {
RemoveCatalogAttribute result = new RemoveCatalogAttribute(attributesConfig, content);
initialize(result);
return result;
}
public class RemoveCatalogAttribute extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+attributesConfig}:removeCatalogAttribute";
private final java.util.regex.Pattern ATTRIBUTES_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
/**
* Removes the specified CatalogAttribute from the AttributesConfig. If the CatalogAttribute to
* remove does not exist, a NOT_FOUND error is returned.
*
* Create a request for the method "attributesConfig.removeCatalogAttribute".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link RemoveCatalogAttribute#execute()} method to invoke the remote
* operation. {@link RemoveCatalogAttribute#initialize(com.google.api.client.googleapis.servic
* es.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param attributesConfig Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveCatalogAttributeRequest}
* @since 1.13
*/
protected RemoveCatalogAttribute(java.lang.String attributesConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveCatalogAttributeRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig.class);
this.attributesConfig = com.google.api.client.util.Preconditions.checkNotNull(attributesConfig, "Required parameter attributesConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ATTRIBUTES_CONFIG_PATTERN.matcher(attributesConfig).matches(),
"Parameter attributesConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
}
@Override
public RemoveCatalogAttribute set$Xgafv(java.lang.String $Xgafv) {
return (RemoveCatalogAttribute) super.set$Xgafv($Xgafv);
}
@Override
public RemoveCatalogAttribute setAccessToken(java.lang.String accessToken) {
return (RemoveCatalogAttribute) super.setAccessToken(accessToken);
}
@Override
public RemoveCatalogAttribute setAlt(java.lang.String alt) {
return (RemoveCatalogAttribute) super.setAlt(alt);
}
@Override
public RemoveCatalogAttribute setCallback(java.lang.String callback) {
return (RemoveCatalogAttribute) super.setCallback(callback);
}
@Override
public RemoveCatalogAttribute setFields(java.lang.String fields) {
return (RemoveCatalogAttribute) super.setFields(fields);
}
@Override
public RemoveCatalogAttribute setKey(java.lang.String key) {
return (RemoveCatalogAttribute) super.setKey(key);
}
@Override
public RemoveCatalogAttribute setOauthToken(java.lang.String oauthToken) {
return (RemoveCatalogAttribute) super.setOauthToken(oauthToken);
}
@Override
public RemoveCatalogAttribute setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveCatalogAttribute) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveCatalogAttribute setQuotaUser(java.lang.String quotaUser) {
return (RemoveCatalogAttribute) super.setQuotaUser(quotaUser);
}
@Override
public RemoveCatalogAttribute setUploadType(java.lang.String uploadType) {
return (RemoveCatalogAttribute) super.setUploadType(uploadType);
}
@Override
public RemoveCatalogAttribute setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveCatalogAttribute) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/loc
* ations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
@com.google.api.client.util.Key
private java.lang.String attributesConfig;
/** Required. Full AttributesConfig resource name. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public java.lang.String getAttributesConfig() {
return attributesConfig;
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/loc
* ations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public RemoveCatalogAttribute setAttributesConfig(java.lang.String attributesConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ATTRIBUTES_CONFIG_PATTERN.matcher(attributesConfig).matches(),
"Parameter attributesConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
this.attributesConfig = attributesConfig;
return this;
}
@Override
public RemoveCatalogAttribute set(String parameterName, Object value) {
return (RemoveCatalogAttribute) super.set(parameterName, value);
}
}
/**
* Replaces the specified CatalogAttribute in the AttributesConfig by updating the catalog attribute
* with the same CatalogAttribute.key. If the CatalogAttribute to replace does not exist, a
* NOT_FOUND error is returned.
*
* Create a request for the method "attributesConfig.replaceCatalogAttribute".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link ReplaceCatalogAttribute#execute()} method to invoke the remote
* operation.
*
* @param attributesConfig Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ReplaceCatalogAttributeRequest}
* @return the request
*/
public ReplaceCatalogAttribute replaceCatalogAttribute(java.lang.String attributesConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ReplaceCatalogAttributeRequest content) throws java.io.IOException {
ReplaceCatalogAttribute result = new ReplaceCatalogAttribute(attributesConfig, content);
initialize(result);
return result;
}
public class ReplaceCatalogAttribute extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+attributesConfig}:replaceCatalogAttribute";
private final java.util.regex.Pattern ATTRIBUTES_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
/**
* Replaces the specified CatalogAttribute in the AttributesConfig by updating the catalog
* attribute with the same CatalogAttribute.key. If the CatalogAttribute to replace does not
* exist, a NOT_FOUND error is returned.
*
* Create a request for the method "attributesConfig.replaceCatalogAttribute".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link ReplaceCatalogAttribute#execute()} method to invoke the remote
* operation. {@link ReplaceCatalogAttribute#initialize(com.google.api.client.googleapis.servi
* ces.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param attributesConfig Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ReplaceCatalogAttributeRequest}
* @since 1.13
*/
protected ReplaceCatalogAttribute(java.lang.String attributesConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ReplaceCatalogAttributeRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AttributesConfig.class);
this.attributesConfig = com.google.api.client.util.Preconditions.checkNotNull(attributesConfig, "Required parameter attributesConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ATTRIBUTES_CONFIG_PATTERN.matcher(attributesConfig).matches(),
"Parameter attributesConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
}
@Override
public ReplaceCatalogAttribute set$Xgafv(java.lang.String $Xgafv) {
return (ReplaceCatalogAttribute) super.set$Xgafv($Xgafv);
}
@Override
public ReplaceCatalogAttribute setAccessToken(java.lang.String accessToken) {
return (ReplaceCatalogAttribute) super.setAccessToken(accessToken);
}
@Override
public ReplaceCatalogAttribute setAlt(java.lang.String alt) {
return (ReplaceCatalogAttribute) super.setAlt(alt);
}
@Override
public ReplaceCatalogAttribute setCallback(java.lang.String callback) {
return (ReplaceCatalogAttribute) super.setCallback(callback);
}
@Override
public ReplaceCatalogAttribute setFields(java.lang.String fields) {
return (ReplaceCatalogAttribute) super.setFields(fields);
}
@Override
public ReplaceCatalogAttribute setKey(java.lang.String key) {
return (ReplaceCatalogAttribute) super.setKey(key);
}
@Override
public ReplaceCatalogAttribute setOauthToken(java.lang.String oauthToken) {
return (ReplaceCatalogAttribute) super.setOauthToken(oauthToken);
}
@Override
public ReplaceCatalogAttribute setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplaceCatalogAttribute) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplaceCatalogAttribute setQuotaUser(java.lang.String quotaUser) {
return (ReplaceCatalogAttribute) super.setQuotaUser(quotaUser);
}
@Override
public ReplaceCatalogAttribute setUploadType(java.lang.String uploadType) {
return (ReplaceCatalogAttribute) super.setUploadType(uploadType);
}
@Override
public ReplaceCatalogAttribute setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplaceCatalogAttribute) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/loc
* ations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
@com.google.api.client.util.Key
private java.lang.String attributesConfig;
/** Required. Full AttributesConfig resource name. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public java.lang.String getAttributesConfig() {
return attributesConfig;
}
/**
* Required. Full AttributesConfig resource name. Format: `projects/{project_number}/loc
* ations/{location_id}/catalogs/{catalog_id}/attributesConfig`
*/
public ReplaceCatalogAttribute setAttributesConfig(java.lang.String attributesConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ATTRIBUTES_CONFIG_PATTERN.matcher(attributesConfig).matches(),
"Parameter attributesConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/attributesConfig$");
}
this.attributesConfig = attributesConfig;
return this;
}
@Override
public ReplaceCatalogAttribute set(String parameterName, Object value) {
return (ReplaceCatalogAttribute) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Branches collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Branches.List request = retail.branches().list(parameters ...)}
*
*
* @return the resource collection
*/
public Branches branches() {
return new Branches();
}
/**
* The "branches" collection of methods.
*/
public class Branches {
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Operations.List request = retail.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Products collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Products.List request = retail.products().list(parameters ...)}
*
*
* @return the resource collection
*/
public Products products() {
return new Products();
}
/**
* The "products" collection of methods.
*/
public class Products {
/**
* We recommend that you use the ProductService.AddLocalInventories method instead of the
* ProductService.AddFulfillmentPlaces method. ProductService.AddLocalInventories achieves the same
* results but provides more fine-grained control over ingesting local inventory data. Incrementally
* adds place IDs to Product.fulfillment_info.place_ids. This process is asynchronous and does not
* require the Product to exist before updating fulfillment information. If the request is valid,
* the update will be enqueued and processed downstream. As a consequence, when a response is
* returned, the added place IDs are not immediately manifested in the Product queried by
* ProductService.GetProduct or ProductService.ListProducts. The returned Operations will be
* obsolete after 1 day, and GetOperation API will return NOT_FOUND afterwards. If conflicting
* updates are issued, the Operations associated with the stale updates will not be marked as done
* until being obsolete.
*
* Create a request for the method "products.addFulfillmentPlaces".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link AddFulfillmentPlaces#execute()} method to invoke the remote
* operation.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddFulfillmentPlacesRequest}
* @return the request
*/
public AddFulfillmentPlaces addFulfillmentPlaces(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddFulfillmentPlacesRequest content) throws java.io.IOException {
AddFulfillmentPlaces result = new AddFulfillmentPlaces(product, content);
initialize(result);
return result;
}
public class AddFulfillmentPlaces extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+product}:addFulfillmentPlaces";
private final java.util.regex.Pattern PRODUCT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* We recommend that you use the ProductService.AddLocalInventories method instead of the
* ProductService.AddFulfillmentPlaces method. ProductService.AddLocalInventories achieves the
* same results but provides more fine-grained control over ingesting local inventory data.
* Incrementally adds place IDs to Product.fulfillment_info.place_ids. This process is
* asynchronous and does not require the Product to exist before updating fulfillment information.
* If the request is valid, the update will be enqueued and processed downstream. As a
* consequence, when a response is returned, the added place IDs are not immediately manifested in
* the Product queried by ProductService.GetProduct or ProductService.ListProducts. The returned
* Operations will be obsolete after 1 day, and GetOperation API will return NOT_FOUND afterwards.
* If conflicting updates are issued, the Operations associated with the stale updates will not be
* marked as done until being obsolete.
*
* Create a request for the method "products.addFulfillmentPlaces".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link AddFulfillmentPlaces#execute()} method to invoke the remote
* operation. {@link AddFulfillmentPlaces#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddFulfillmentPlacesRequest}
* @since 1.13
*/
protected AddFulfillmentPlaces(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddFulfillmentPlacesRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.product = com.google.api.client.util.Preconditions.checkNotNull(product, "Required parameter product must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public AddFulfillmentPlaces set$Xgafv(java.lang.String $Xgafv) {
return (AddFulfillmentPlaces) super.set$Xgafv($Xgafv);
}
@Override
public AddFulfillmentPlaces setAccessToken(java.lang.String accessToken) {
return (AddFulfillmentPlaces) super.setAccessToken(accessToken);
}
@Override
public AddFulfillmentPlaces setAlt(java.lang.String alt) {
return (AddFulfillmentPlaces) super.setAlt(alt);
}
@Override
public AddFulfillmentPlaces setCallback(java.lang.String callback) {
return (AddFulfillmentPlaces) super.setCallback(callback);
}
@Override
public AddFulfillmentPlaces setFields(java.lang.String fields) {
return (AddFulfillmentPlaces) super.setFields(fields);
}
@Override
public AddFulfillmentPlaces setKey(java.lang.String key) {
return (AddFulfillmentPlaces) super.setKey(key);
}
@Override
public AddFulfillmentPlaces setOauthToken(java.lang.String oauthToken) {
return (AddFulfillmentPlaces) super.setOauthToken(oauthToken);
}
@Override
public AddFulfillmentPlaces setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddFulfillmentPlaces) super.setPrettyPrint(prettyPrint);
}
@Override
public AddFulfillmentPlaces setQuotaUser(java.lang.String quotaUser) {
return (AddFulfillmentPlaces) super.setQuotaUser(quotaUser);
}
@Override
public AddFulfillmentPlaces setUploadType(java.lang.String uploadType) {
return (AddFulfillmentPlaces) super.setUploadType(uploadType);
}
@Override
public AddFulfillmentPlaces setUploadProtocol(java.lang.String uploadProtocol) {
return (AddFulfillmentPlaces) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String product;
/** Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalo
g/branches/default_branch/products/some_product_id`. If the caller does not have permission to
access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error is returned.
*/
public java.lang.String getProduct() {
return product;
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
public AddFulfillmentPlaces setProduct(java.lang.String product) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.product = product;
return this;
}
@Override
public AddFulfillmentPlaces set(String parameterName, Object value) {
return (AddFulfillmentPlaces) super.set(parameterName, value);
}
}
/**
* Updates local inventory information for a Product at a list of places, while respecting the last
* update timestamps of each inventory field. This process is asynchronous and does not require the
* Product to exist before updating inventory information. If the request is valid, the update will
* be enqueued and processed downstream. As a consequence, when a response is returned, updates are
* not immediately manifested in the Product queried by ProductService.GetProduct or
* ProductService.ListProducts. Local inventory information can only be modified using this method.
* ProductService.CreateProduct and ProductService.UpdateProduct has no effect on local inventories.
* The returned Operations will be obsolete after 1 day, and GetOperation API will return NOT_FOUND
* afterwards. If conflicting updates are issued, the Operations associated with the stale updates
* will not be marked as done until being obsolete.
*
* Create a request for the method "products.addLocalInventories".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link AddLocalInventories#execute()} method to invoke the remote operation.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddLocalInventoriesRequest}
* @return the request
*/
public AddLocalInventories addLocalInventories(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddLocalInventoriesRequest content) throws java.io.IOException {
AddLocalInventories result = new AddLocalInventories(product, content);
initialize(result);
return result;
}
public class AddLocalInventories extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+product}:addLocalInventories";
private final java.util.regex.Pattern PRODUCT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* Updates local inventory information for a Product at a list of places, while respecting the
* last update timestamps of each inventory field. This process is asynchronous and does not
* require the Product to exist before updating inventory information. If the request is valid,
* the update will be enqueued and processed downstream. As a consequence, when a response is
* returned, updates are not immediately manifested in the Product queried by
* ProductService.GetProduct or ProductService.ListProducts. Local inventory information can only
* be modified using this method. ProductService.CreateProduct and ProductService.UpdateProduct
* has no effect on local inventories. The returned Operations will be obsolete after 1 day, and
* GetOperation API will return NOT_FOUND afterwards. If conflicting updates are issued, the
* Operations associated with the stale updates will not be marked as done until being obsolete.
*
* Create a request for the method "products.addLocalInventories".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link AddLocalInventories#execute()} method to invoke the remote
* operation. {@link AddLocalInventories#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddLocalInventoriesRequest}
* @since 1.13
*/
protected AddLocalInventories(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddLocalInventoriesRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.product = com.google.api.client.util.Preconditions.checkNotNull(product, "Required parameter product must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public AddLocalInventories set$Xgafv(java.lang.String $Xgafv) {
return (AddLocalInventories) super.set$Xgafv($Xgafv);
}
@Override
public AddLocalInventories setAccessToken(java.lang.String accessToken) {
return (AddLocalInventories) super.setAccessToken(accessToken);
}
@Override
public AddLocalInventories setAlt(java.lang.String alt) {
return (AddLocalInventories) super.setAlt(alt);
}
@Override
public AddLocalInventories setCallback(java.lang.String callback) {
return (AddLocalInventories) super.setCallback(callback);
}
@Override
public AddLocalInventories setFields(java.lang.String fields) {
return (AddLocalInventories) super.setFields(fields);
}
@Override
public AddLocalInventories setKey(java.lang.String key) {
return (AddLocalInventories) super.setKey(key);
}
@Override
public AddLocalInventories setOauthToken(java.lang.String oauthToken) {
return (AddLocalInventories) super.setOauthToken(oauthToken);
}
@Override
public AddLocalInventories setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddLocalInventories) super.setPrettyPrint(prettyPrint);
}
@Override
public AddLocalInventories setQuotaUser(java.lang.String quotaUser) {
return (AddLocalInventories) super.setQuotaUser(quotaUser);
}
@Override
public AddLocalInventories setUploadType(java.lang.String uploadType) {
return (AddLocalInventories) super.setUploadType(uploadType);
}
@Override
public AddLocalInventories setUploadProtocol(java.lang.String uploadProtocol) {
return (AddLocalInventories) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String product;
/** Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalo
g/branches/default_branch/products/some_product_id`. If the caller does not have permission to
access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error is returned.
*/
public java.lang.String getProduct() {
return product;
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
public AddLocalInventories setProduct(java.lang.String product) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.product = product;
return this;
}
@Override
public AddLocalInventories set(String parameterName, Object value) {
return (AddLocalInventories) super.set(parameterName, value);
}
}
/**
* Creates a Product.
*
* Create a request for the method "products.create".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent catalog resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/products";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
/**
* Creates a Product.
*
* Create a request for the method "products.create".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent catalog resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent catalog resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent catalog resource name, such as
`projects/locations/global/catalogs/default_catalog/branches/default_branch`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent catalog resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the Product, which will become the final component of
* the Product.name. If the caller does not have permission to create the Product,
* regardless of whether or not it exists, a PERMISSION_DENIED error is returned. This
* field must be unique among all Products with the same parent. Otherwise, an
* ALREADY_EXISTS error is returned. This field must be a UTF-8 encoded string with a
* length limit of 128 characters. Otherwise, an INVALID_ARGUMENT error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String productId;
/** Required. The ID to use for the Product, which will become the final component of the Product.name.
If the caller does not have permission to create the Product, regardless of whether or not it
exists, a PERMISSION_DENIED error is returned. This field must be unique among all Products with
the same parent. Otherwise, an ALREADY_EXISTS error is returned. This field must be a UTF-8 encoded
string with a length limit of 128 characters. Otherwise, an INVALID_ARGUMENT error is returned.
*/
public java.lang.String getProductId() {
return productId;
}
/**
* Required. The ID to use for the Product, which will become the final component of
* the Product.name. If the caller does not have permission to create the Product,
* regardless of whether or not it exists, a PERMISSION_DENIED error is returned. This
* field must be unique among all Products with the same parent. Otherwise, an
* ALREADY_EXISTS error is returned. This field must be a UTF-8 encoded string with a
* length limit of 128 characters. Otherwise, an INVALID_ARGUMENT error is returned.
*/
public Create setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a Product.
*
* Create a request for the method "products.delete".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to delete the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned. If the Product to delete does not exist, a NOT_FOUND error is returned. The
* Product to delete can neither be a Product.Type.COLLECTION Product member nor a
* Product.Type.PRIMARY Product with more than one variants. Otherwise, an INVALID_ARGUMENT
* error is returned. All inventory information for the named Product will be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* Deletes a Product.
*
* Create a request for the method "products.delete".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to delete the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned. If the Product to delete does not exist, a NOT_FOUND error is returned. The
* Product to delete can neither be a Product.Type.COLLECTION Product member nor a
* Product.Type.PRIMARY Product with more than one variants. Otherwise, an INVALID_ARGUMENT
* error is returned. All inventory information for the named Product will be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRetail.this, "DELETE", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to delete the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned. If the Product to delete does not
* exist, a NOT_FOUND error is returned. The Product to delete can neither be a
* Product.Type.COLLECTION Product member nor a Product.Type.PRIMARY Product with more
* than one variants. Otherwise, an INVALID_ARGUMENT error is returned. All inventory
* information for the named Product will be deleted.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalo
g/branches/default_branch/products/some_product_id`. If the caller does not have permission to
delete the Product, regardless of whether or not it exists, a PERMISSION_DENIED error is returned.
If the Product to delete does not exist, a NOT_FOUND error is returned. The Product to delete can
neither be a Product.Type.COLLECTION Product member nor a Product.Type.PRIMARY Product with more
than one variants. Otherwise, an INVALID_ARGUMENT error is returned. All inventory information for
the named Product will be deleted.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to delete the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned. If the Product to delete does not
* exist, a NOT_FOUND error is returned. The Product to delete can neither be a
* Product.Type.COLLECTION Product member nor a Product.Type.PRIMARY Product with more
* than one variants. Otherwise, an INVALID_ARGUMENT error is returned. All inventory
* information for the named Product will be deleted.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a Product.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned. If the requested Product does not exist, a NOT_FOUND error is returned.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* Gets a Product.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned. If the requested Product does not exist, a NOT_FOUND error is returned.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned. If the requested Product does not
* exist, a NOT_FOUND error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalo
g/branches/default_branch/products/some_product_id`. If the caller does not have permission to
access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error is returned.
If the requested Product does not exist, a NOT_FOUND error is returned.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned. If the requested Product does not
* exist, a NOT_FOUND error is returned.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Bulk import of multiple Products. Request processing may be synchronous. Non-existing items are
* created. Note that it is possible for a subset of the Products to be successfully updated.
*
* Create a request for the method "products.import".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link CloudRetailImport#execute()} method to invoke the remote operation.
*
* @param parent Required. `projects/1234/locations/global/catalogs/default_catalog/branches/default_branch` If no
* updateMask is specified, requires products.create permission. If updateMask is specified,
* requires products.update permission.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportProductsRequest}
* @return the request
*/
public CloudRetailImport retailImport(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportProductsRequest content) throws java.io.IOException {
CloudRetailImport result = new CloudRetailImport(parent, content);
initialize(result);
return result;
}
public class CloudRetailImport extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/products:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
/**
* Bulk import of multiple Products. Request processing may be synchronous. Non-existing items are
* created. Note that it is possible for a subset of the Products to be successfully updated.
*
* Create a request for the method "products.import".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link CloudRetailImport#execute()} method to invoke the remote operation.
* {@link CloudRetailImport#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param parent Required. `projects/1234/locations/global/catalogs/default_catalog/branches/default_branch` If no
* updateMask is specified, requires products.create permission. If updateMask is specified,
* requires products.update permission.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportProductsRequest}
* @since 1.13
*/
protected CloudRetailImport(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportProductsRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
}
@Override
public CloudRetailImport set$Xgafv(java.lang.String $Xgafv) {
return (CloudRetailImport) super.set$Xgafv($Xgafv);
}
@Override
public CloudRetailImport setAccessToken(java.lang.String accessToken) {
return (CloudRetailImport) super.setAccessToken(accessToken);
}
@Override
public CloudRetailImport setAlt(java.lang.String alt) {
return (CloudRetailImport) super.setAlt(alt);
}
@Override
public CloudRetailImport setCallback(java.lang.String callback) {
return (CloudRetailImport) super.setCallback(callback);
}
@Override
public CloudRetailImport setFields(java.lang.String fields) {
return (CloudRetailImport) super.setFields(fields);
}
@Override
public CloudRetailImport setKey(java.lang.String key) {
return (CloudRetailImport) super.setKey(key);
}
@Override
public CloudRetailImport setOauthToken(java.lang.String oauthToken) {
return (CloudRetailImport) super.setOauthToken(oauthToken);
}
@Override
public CloudRetailImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CloudRetailImport) super.setPrettyPrint(prettyPrint);
}
@Override
public CloudRetailImport setQuotaUser(java.lang.String quotaUser) {
return (CloudRetailImport) super.setQuotaUser(quotaUser);
}
@Override
public CloudRetailImport setUploadType(java.lang.String uploadType) {
return (CloudRetailImport) super.setUploadType(uploadType);
}
@Override
public CloudRetailImport setUploadProtocol(java.lang.String uploadProtocol) {
return (CloudRetailImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required.
* `projects/1234/locations/global/catalogs/default_catalog/branches/default_branch`
* If no updateMask is specified, requires products.create permission. If updateMask
* is specified, requires products.update permission.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. `projects/1234/locations/global/catalogs/default_catalog/branches/default_branch` If no
updateMask is specified, requires products.create permission. If updateMask is specified, requires
products.update permission.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required.
* `projects/1234/locations/global/catalogs/default_catalog/branches/default_branch`
* If no updateMask is specified, requires products.create permission. If updateMask
* is specified, requires products.update permission.
*/
public CloudRetailImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public CloudRetailImport set(String parameterName, Object value) {
return (CloudRetailImport) super.set(parameterName, value);
}
}
/**
* Gets a list of Products.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent branch resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/0`. Use `default_branch` as
* the branch ID, to list products under the default branch. If the caller does not have
* permission to list Products under this branch, regardless of whether or not this branch
* exists, a PERMISSION_DENIED error is returned.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/products";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
/**
* Gets a list of Products.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent branch resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/0`. Use `default_branch` as
* the branch ID, to list products under the default branch. If the caller does not have
* permission to list Products under this branch, regardless of whether or not this branch
* exists, a PERMISSION_DENIED error is returned.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ListProductsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent branch resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/0`. Use
* `default_branch` as the branch ID, to list products under the default branch. If
* the caller does not have permission to list Products under this branch, regardless
* of whether or not this branch exists, a PERMISSION_DENIED error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent branch resource name, such as
`projects/locations/global/catalogs/default_catalog/branches/0`. Use `default_branch` as the branch
ID, to list products under the default branch. If the caller does not have permission to list
Products under this branch, regardless of whether or not this branch exists, a PERMISSION_DENIED
error is returned.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent branch resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/0`. Use
* `default_branch` as the branch ID, to list products under the default branch. If
* the caller does not have permission to list Products under this branch, regardless
* of whether or not this branch exists, a PERMISSION_DENIED error is returned.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter to apply on the list results. Supported features: * List all the products
* under the parent branch if filter is unset. * List Product.Type.VARIANT Products
* sharing the same Product.Type.PRIMARY Product. For example: `primary_product_id =
* "some_product_id"` * List Products bundled in a Product.Type.COLLECTION Product.
* For example: `collection_product_id = "some_product_id"` * List Products with a
* partibular type. For example: `type = "PRIMARY"` `type = "VARIANT"` `type =
* "COLLECTION"` If the field is unrecognizable, an INVALID_ARGUMENT error is
* returned. If the specified Product.Type.PRIMARY Product or Product.Type.COLLECTION
* Product does not exist, a NOT_FOUND error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to apply on the list results. Supported features: * List all the products under the parent
branch if filter is unset. * List Product.Type.VARIANT Products sharing the same
Product.Type.PRIMARY Product. For example: `primary_product_id = "some_product_id"` * List Products
bundled in a Product.Type.COLLECTION Product. For example: `collection_product_id =
"some_product_id"` * List Products with a partibular type. For example: `type = "PRIMARY"` `type =
"VARIANT"` `type = "COLLECTION"` If the field is unrecognizable, an INVALID_ARGUMENT error is
returned. If the specified Product.Type.PRIMARY Product or Product.Type.COLLECTION Product does not
exist, a NOT_FOUND error is returned.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to apply on the list results. Supported features: * List all the products
* under the parent branch if filter is unset. * List Product.Type.VARIANT Products
* sharing the same Product.Type.PRIMARY Product. For example: `primary_product_id =
* "some_product_id"` * List Products bundled in a Product.Type.COLLECTION Product.
* For example: `collection_product_id = "some_product_id"` * List Products with a
* partibular type. For example: `type = "PRIMARY"` `type = "VARIANT"` `type =
* "COLLECTION"` If the field is unrecognizable, an INVALID_ARGUMENT error is
* returned. If the specified Product.Type.PRIMARY Product or Product.Type.COLLECTION
* Product does not exist, a NOT_FOUND error is returned.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Maximum number of Products to return. If unspecified, defaults to 100. The maximum
* allowed value is 1000. Values above 1000 will be coerced to 1000. If this field is
* negative, an INVALID_ARGUMENT error is returned.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of Products to return. If unspecified, defaults to 100. The maximum allowed value is
1000. Values above 1000 will be coerced to 1000. If this field is negative, an INVALID_ARGUMENT
error is returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Maximum number of Products to return. If unspecified, defaults to 100. The maximum
* allowed value is 1000. Values above 1000 will be coerced to 1000. If this field is
* negative, an INVALID_ARGUMENT error is returned.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token ListProductsResponse.next_page_token, received from a previous
* ProductService.ListProducts call. Provide this to retrieve the subsequent page.
* When paginating, all other parameters provided to ProductService.ListProducts must
* match the call that provided the page token. Otherwise, an INVALID_ARGUMENT error
* is returned.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token ListProductsResponse.next_page_token, received from a previous
ProductService.ListProducts call. Provide this to retrieve the subsequent page. When paginating,
all other parameters provided to ProductService.ListProducts must match the call that provided the
page token. Otherwise, an INVALID_ARGUMENT error is returned.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token ListProductsResponse.next_page_token, received from a previous
* ProductService.ListProducts call. Provide this to retrieve the subsequent page.
* When paginating, all other parameters provided to ProductService.ListProducts must
* match the call that provided the page token. Otherwise, an INVALID_ARGUMENT error
* is returned.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The fields of Product to return in the responses. If not set or empty, the
* following fields are returned: * Product.name * Product.id * Product.title *
* Product.uri * Product.images * Product.price_info * Product.brands If "*" is
* provided, all fields are returned. Product.name is always returned no matter what
* mask is set. If an unsupported or unknown field is provided, an INVALID_ARGUMENT
* error is returned.
*/
@com.google.api.client.util.Key
private String readMask;
/** The fields of Product to return in the responses. If not set or empty, the following fields are
returned: * Product.name * Product.id * Product.title * Product.uri * Product.images *
Product.price_info * Product.brands If "*" is provided, all fields are returned. Product.name is
always returned no matter what mask is set. If an unsupported or unknown field is provided, an
INVALID_ARGUMENT error is returned.
*/
public String getReadMask() {
return readMask;
}
/**
* The fields of Product to return in the responses. If not set or empty, the
* following fields are returned: * Product.name * Product.id * Product.title *
* Product.uri * Product.images * Product.price_info * Product.brands If "*" is
* provided, all fields are returned. Product.name is always returned no matter what
* mask is set. If an unsupported or unknown field is provided, an INVALID_ARGUMENT
* error is returned.
*/
public List setReadMask(String readMask) {
this.readMask = readMask;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a Product.
*
* Create a request for the method "products.patch".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Immutable. Full resource name of the product, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch/products/produ
* ct_id`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* Updates a Product.
*
* Create a request for the method "products.patch".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Immutable. Full resource name of the product, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch/products/produ
* ct_id`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Product.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Immutable. Full resource name of the product, such as `projects/locations/global/ca
* talogs/default_catalog/branches/default_branch/products/product_id`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Immutable. Full resource name of the product, such as
`projects/locations/global/catalogs/default_catalog/branches/default_branch/products/product_id`.
*/
public java.lang.String getName() {
return name;
}
/**
* Immutable. Full resource name of the product, such as `projects/locations/global/ca
* talogs/default_catalog/branches/default_branch/products/product_id`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.name = name;
return this;
}
/**
* If set to true, and the Product is not found, a new Product will be created. In
* this situation, `update_mask` is ignored.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowMissing;
/** If set to true, and the Product is not found, a new Product will be created. In this situation,
`update_mask` is ignored.
*/
public java.lang.Boolean getAllowMissing() {
return allowMissing;
}
/**
* If set to true, and the Product is not found, a new Product will be created. In
* this situation, `update_mask` is ignored.
*/
public Patch setAllowMissing(java.lang.Boolean allowMissing) {
this.allowMissing = allowMissing;
return this;
}
/**
* Indicates which fields in the provided Product to update. The immutable and output
* only fields are NOT supported. If not set, all supported fields (the fields that
* are neither immutable nor output only) are updated. If an unsupported or unknown
* field is provided, an INVALID_ARGUMENT error is returned. The attribute key can be
* updated by setting the mask path as "attributes.${key_name}". If a key name is
* present in the mask but not in the patching product from the request, this key will
* be deleted after the update.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Indicates which fields in the provided Product to update. The immutable and output only fields are
NOT supported. If not set, all supported fields (the fields that are neither immutable nor output
only) are updated. If an unsupported or unknown field is provided, an INVALID_ARGUMENT error is
returned. The attribute key can be updated by setting the mask path as "attributes.${key_name}". If
a key name is present in the mask but not in the patching product from the request, this key will
be deleted after the update.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Indicates which fields in the provided Product to update. The immutable and output
* only fields are NOT supported. If not set, all supported fields (the fields that
* are neither immutable nor output only) are updated. If an unsupported or unknown
* field is provided, an INVALID_ARGUMENT error is returned. The attribute key can be
* updated by setting the mask path as "attributes.${key_name}". If a key name is
* present in the mask but not in the patching product from the request, this key will
* be deleted after the update.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Permanently deletes all selected Products under a branch. This process is asynchronous. If the
* request is valid, the removal will be enqueued and processed offline. Depending on the number of
* Products, this operation could take hours to complete. Before the operation completes, some
* Products may still be returned by ProductService.GetProduct or ProductService.ListProducts.
* Depending on the number of Products, this operation could take hours to complete. To get a sample
* of Products that would be deleted, set PurgeProductsRequest.force to false.
*
* Create a request for the method "products.purge".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Purge#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the branch under which the products are created. The format is
* `projects/${projectId}/locations/global/catalogs/${catalogId}/branches/${branchId}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeProductsRequest}
* @return the request
*/
public Purge purge(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeProductsRequest content) throws java.io.IOException {
Purge result = new Purge(parent, content);
initialize(result);
return result;
}
public class Purge extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/products:purge";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
/**
* Permanently deletes all selected Products under a branch. This process is asynchronous. If the
* request is valid, the removal will be enqueued and processed offline. Depending on the number
* of Products, this operation could take hours to complete. Before the operation completes, some
* Products may still be returned by ProductService.GetProduct or ProductService.ListProducts.
* Depending on the number of Products, this operation could take hours to complete. To get a
* sample of Products that would be deleted, set PurgeProductsRequest.force to false.
*
* Create a request for the method "products.purge".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Purge#execute()} method to invoke the remote operation. {@link
* Purge#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the branch under which the products are created. The format is
* `projects/${projectId}/locations/global/catalogs/${catalogId}/branches/${branchId}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeProductsRequest}
* @since 1.13
*/
protected Purge(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeProductsRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
}
@Override
public Purge set$Xgafv(java.lang.String $Xgafv) {
return (Purge) super.set$Xgafv($Xgafv);
}
@Override
public Purge setAccessToken(java.lang.String accessToken) {
return (Purge) super.setAccessToken(accessToken);
}
@Override
public Purge setAlt(java.lang.String alt) {
return (Purge) super.setAlt(alt);
}
@Override
public Purge setCallback(java.lang.String callback) {
return (Purge) super.setCallback(callback);
}
@Override
public Purge setFields(java.lang.String fields) {
return (Purge) super.setFields(fields);
}
@Override
public Purge setKey(java.lang.String key) {
return (Purge) super.setKey(key);
}
@Override
public Purge setOauthToken(java.lang.String oauthToken) {
return (Purge) super.setOauthToken(oauthToken);
}
@Override
public Purge setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Purge) super.setPrettyPrint(prettyPrint);
}
@Override
public Purge setQuotaUser(java.lang.String quotaUser) {
return (Purge) super.setQuotaUser(quotaUser);
}
@Override
public Purge setUploadType(java.lang.String uploadType) {
return (Purge) super.setUploadType(uploadType);
}
@Override
public Purge setUploadProtocol(java.lang.String uploadProtocol) {
return (Purge) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the branch under which the products are created. The
* format is
* `projects/${projectId}/locations/global/catalogs/${catalogId}/branches/${branchId}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the branch under which the products are created. The format is
`projects/${projectId}/locations/global/catalogs/${catalogId}/branches/${branchId}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the branch under which the products are created. The
* format is
* `projects/${projectId}/locations/global/catalogs/${catalogId}/branches/${branchId}`
*/
public Purge setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Purge set(String parameterName, Object value) {
return (Purge) super.set(parameterName, value);
}
}
/**
* We recommend that you use the ProductService.RemoveLocalInventories method instead of the
* ProductService.RemoveFulfillmentPlaces method. ProductService.RemoveLocalInventories achieves the
* same results but provides more fine-grained control over ingesting local inventory data.
* Incrementally removes place IDs from a Product.fulfillment_info.place_ids. This process is
* asynchronous and does not require the Product to exist before updating fulfillment information.
* If the request is valid, the update will be enqueued and processed downstream. As a consequence,
* when a response is returned, the removed place IDs are not immediately manifested in the Product
* queried by ProductService.GetProduct or ProductService.ListProducts. The returned Operations will
* be obsolete after 1 day, and GetOperation API will return NOT_FOUND afterwards. If conflicting
* updates are issued, the Operations associated with the stale updates will not be marked as done
* until being obsolete.
*
* Create a request for the method "products.removeFulfillmentPlaces".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link RemoveFulfillmentPlaces#execute()} method to invoke the remote
* operation.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveFulfillmentPlacesRequest}
* @return the request
*/
public RemoveFulfillmentPlaces removeFulfillmentPlaces(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveFulfillmentPlacesRequest content) throws java.io.IOException {
RemoveFulfillmentPlaces result = new RemoveFulfillmentPlaces(product, content);
initialize(result);
return result;
}
public class RemoveFulfillmentPlaces extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+product}:removeFulfillmentPlaces";
private final java.util.regex.Pattern PRODUCT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* We recommend that you use the ProductService.RemoveLocalInventories method instead of the
* ProductService.RemoveFulfillmentPlaces method. ProductService.RemoveLocalInventories achieves
* the same results but provides more fine-grained control over ingesting local inventory data.
* Incrementally removes place IDs from a Product.fulfillment_info.place_ids. This process is
* asynchronous and does not require the Product to exist before updating fulfillment information.
* If the request is valid, the update will be enqueued and processed downstream. As a
* consequence, when a response is returned, the removed place IDs are not immediately manifested
* in the Product queried by ProductService.GetProduct or ProductService.ListProducts. The
* returned Operations will be obsolete after 1 day, and GetOperation API will return NOT_FOUND
* afterwards. If conflicting updates are issued, the Operations associated with the stale updates
* will not be marked as done until being obsolete.
*
* Create a request for the method "products.removeFulfillmentPlaces".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link RemoveFulfillmentPlaces#execute()} method to invoke the remote
* operation. {@link RemoveFulfillmentPlaces#initialize(com.google.api.client.googleapis.servi
* ces.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveFulfillmentPlacesRequest}
* @since 1.13
*/
protected RemoveFulfillmentPlaces(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveFulfillmentPlacesRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.product = com.google.api.client.util.Preconditions.checkNotNull(product, "Required parameter product must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public RemoveFulfillmentPlaces set$Xgafv(java.lang.String $Xgafv) {
return (RemoveFulfillmentPlaces) super.set$Xgafv($Xgafv);
}
@Override
public RemoveFulfillmentPlaces setAccessToken(java.lang.String accessToken) {
return (RemoveFulfillmentPlaces) super.setAccessToken(accessToken);
}
@Override
public RemoveFulfillmentPlaces setAlt(java.lang.String alt) {
return (RemoveFulfillmentPlaces) super.setAlt(alt);
}
@Override
public RemoveFulfillmentPlaces setCallback(java.lang.String callback) {
return (RemoveFulfillmentPlaces) super.setCallback(callback);
}
@Override
public RemoveFulfillmentPlaces setFields(java.lang.String fields) {
return (RemoveFulfillmentPlaces) super.setFields(fields);
}
@Override
public RemoveFulfillmentPlaces setKey(java.lang.String key) {
return (RemoveFulfillmentPlaces) super.setKey(key);
}
@Override
public RemoveFulfillmentPlaces setOauthToken(java.lang.String oauthToken) {
return (RemoveFulfillmentPlaces) super.setOauthToken(oauthToken);
}
@Override
public RemoveFulfillmentPlaces setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveFulfillmentPlaces) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveFulfillmentPlaces setQuotaUser(java.lang.String quotaUser) {
return (RemoveFulfillmentPlaces) super.setQuotaUser(quotaUser);
}
@Override
public RemoveFulfillmentPlaces setUploadType(java.lang.String uploadType) {
return (RemoveFulfillmentPlaces) super.setUploadType(uploadType);
}
@Override
public RemoveFulfillmentPlaces setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveFulfillmentPlaces) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String product;
/** Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalo
g/branches/default_branch/products/some_product_id`. If the caller does not have permission to
access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error is returned.
*/
public java.lang.String getProduct() {
return product;
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
public RemoveFulfillmentPlaces setProduct(java.lang.String product) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.product = product;
return this;
}
@Override
public RemoveFulfillmentPlaces set(String parameterName, Object value) {
return (RemoveFulfillmentPlaces) super.set(parameterName, value);
}
}
/**
* Remove local inventory information for a Product at a list of places at a removal timestamp. This
* process is asynchronous. If the request is valid, the removal will be enqueued and processed
* downstream. As a consequence, when a response is returned, removals are not immediately
* manifested in the Product queried by ProductService.GetProduct or ProductService.ListProducts.
* Local inventory information can only be removed using this method. ProductService.CreateProduct
* and ProductService.UpdateProduct has no effect on local inventories. The returned Operations will
* be obsolete after 1 day, and GetOperation API will return NOT_FOUND afterwards. If conflicting
* updates are issued, the Operations associated with the stale updates will not be marked as done
* until being obsolete.
*
* Create a request for the method "products.removeLocalInventories".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link RemoveLocalInventories#execute()} method to invoke the remote
* operation.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveLocalInventoriesRequest}
* @return the request
*/
public RemoveLocalInventories removeLocalInventories(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveLocalInventoriesRequest content) throws java.io.IOException {
RemoveLocalInventories result = new RemoveLocalInventories(product, content);
initialize(result);
return result;
}
public class RemoveLocalInventories extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+product}:removeLocalInventories";
private final java.util.regex.Pattern PRODUCT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* Remove local inventory information for a Product at a list of places at a removal timestamp.
* This process is asynchronous. If the request is valid, the removal will be enqueued and
* processed downstream. As a consequence, when a response is returned, removals are not
* immediately manifested in the Product queried by ProductService.GetProduct or
* ProductService.ListProducts. Local inventory information can only be removed using this method.
* ProductService.CreateProduct and ProductService.UpdateProduct has no effect on local
* inventories. The returned Operations will be obsolete after 1 day, and GetOperation API will
* return NOT_FOUND afterwards. If conflicting updates are issued, the Operations associated with
* the stale updates will not be marked as done until being obsolete.
*
* Create a request for the method "products.removeLocalInventories".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link RemoveLocalInventories#execute()} method to invoke the remote
* operation. {@link RemoveLocalInventories#initialize(com.google.api.client.googleapis.servic
* es.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param product Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalog
* /branches/default_branch/products/some_product_id`. If the caller does not have permission
* to access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error
* is returned.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveLocalInventoriesRequest}
* @since 1.13
*/
protected RemoveLocalInventories(java.lang.String product, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveLocalInventoriesRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.product = com.google.api.client.util.Preconditions.checkNotNull(product, "Required parameter product must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public RemoveLocalInventories set$Xgafv(java.lang.String $Xgafv) {
return (RemoveLocalInventories) super.set$Xgafv($Xgafv);
}
@Override
public RemoveLocalInventories setAccessToken(java.lang.String accessToken) {
return (RemoveLocalInventories) super.setAccessToken(accessToken);
}
@Override
public RemoveLocalInventories setAlt(java.lang.String alt) {
return (RemoveLocalInventories) super.setAlt(alt);
}
@Override
public RemoveLocalInventories setCallback(java.lang.String callback) {
return (RemoveLocalInventories) super.setCallback(callback);
}
@Override
public RemoveLocalInventories setFields(java.lang.String fields) {
return (RemoveLocalInventories) super.setFields(fields);
}
@Override
public RemoveLocalInventories setKey(java.lang.String key) {
return (RemoveLocalInventories) super.setKey(key);
}
@Override
public RemoveLocalInventories setOauthToken(java.lang.String oauthToken) {
return (RemoveLocalInventories) super.setOauthToken(oauthToken);
}
@Override
public RemoveLocalInventories setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveLocalInventories) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveLocalInventories setQuotaUser(java.lang.String quotaUser) {
return (RemoveLocalInventories) super.setQuotaUser(quotaUser);
}
@Override
public RemoveLocalInventories setUploadType(java.lang.String uploadType) {
return (RemoveLocalInventories) super.setUploadType(uploadType);
}
@Override
public RemoveLocalInventories setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveLocalInventories) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
@com.google.api.client.util.Key
private java.lang.String product;
/** Required. Full resource name of Product, such as `projects/locations/global/catalogs/default_catalo
g/branches/default_branch/products/some_product_id`. If the caller does not have permission to
access the Product, regardless of whether or not it exists, a PERMISSION_DENIED error is returned.
*/
public java.lang.String getProduct() {
return product;
}
/**
* Required. Full resource name of Product, such as `projects/locations/global/catalog
* s/default_catalog/branches/default_branch/products/some_product_id`. If the caller
* does not have permission to access the Product, regardless of whether or not it
* exists, a PERMISSION_DENIED error is returned.
*/
public RemoveLocalInventories setProduct(java.lang.String product) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRODUCT_PATTERN.matcher(product).matches(),
"Parameter product must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.product = product;
return this;
}
@Override
public RemoveLocalInventories set(String parameterName, Object value) {
return (RemoveLocalInventories) super.set(parameterName, value);
}
}
/**
* Updates inventory information for a Product while respecting the last update timestamps of each
* inventory field. This process is asynchronous and does not require the Product to exist before
* updating fulfillment information. If the request is valid, the update is enqueued and processed
* downstream. As a consequence, when a response is returned, updates are not immediately manifested
* in the Product queried by ProductService.GetProduct or ProductService.ListProducts. When
* inventory is updated with ProductService.CreateProduct and ProductService.UpdateProduct, the
* specified inventory field value(s) overwrite any existing value(s) while ignoring the last update
* time for this field. Furthermore, the last update times for the specified inventory fields are
* overwritten by the times of the ProductService.CreateProduct or ProductService.UpdateProduct
* request. If no inventory fields are set in CreateProductRequest.product, then any pre-existing
* inventory information for this product is used. If no inventory fields are set in
* SetInventoryRequest.set_mask, then any existing inventory information is preserved. Pre-existing
* inventory information can only be updated with ProductService.SetInventory,
* ProductService.AddFulfillmentPlaces, and ProductService.RemoveFulfillmentPlaces. The returned
* Operations is obsolete after one day, and the GetOperation API returns `NOT_FOUND` afterwards. If
* conflicting updates are issued, the Operations associated with the stale updates are not marked
* as done until they are obsolete.
*
* Create a request for the method "products.setInventory".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link SetInventory#execute()} method to invoke the remote operation.
*
* @param name Immutable. Full resource name of the product, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch/products/produ
* ct_id`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetInventoryRequest}
* @return the request
*/
public SetInventory setInventory(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetInventoryRequest content) throws java.io.IOException {
SetInventory result = new SetInventory(name, content);
initialize(result);
return result;
}
public class SetInventory extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}:setInventory";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
/**
* Updates inventory information for a Product while respecting the last update timestamps of each
* inventory field. This process is asynchronous and does not require the Product to exist before
* updating fulfillment information. If the request is valid, the update is enqueued and processed
* downstream. As a consequence, when a response is returned, updates are not immediately
* manifested in the Product queried by ProductService.GetProduct or ProductService.ListProducts.
* When inventory is updated with ProductService.CreateProduct and ProductService.UpdateProduct,
* the specified inventory field value(s) overwrite any existing value(s) while ignoring the last
* update time for this field. Furthermore, the last update times for the specified inventory
* fields are overwritten by the times of the ProductService.CreateProduct or
* ProductService.UpdateProduct request. If no inventory fields are set in
* CreateProductRequest.product, then any pre-existing inventory information for this product is
* used. If no inventory fields are set in SetInventoryRequest.set_mask, then any existing
* inventory information is preserved. Pre-existing inventory information can only be updated with
* ProductService.SetInventory, ProductService.AddFulfillmentPlaces, and
* ProductService.RemoveFulfillmentPlaces. The returned Operations is obsolete after one day, and
* the GetOperation API returns `NOT_FOUND` afterwards. If conflicting updates are issued, the
* Operations associated with the stale updates are not marked as done until they are obsolete.
*
* Create a request for the method "products.setInventory".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link SetInventory#execute()} method to invoke the remote operation.
* {@link
* SetInventory#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Immutable. Full resource name of the product, such as
* `projects/locations/global/catalogs/default_catalog/branches/default_branch/products/produ
* ct_id`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetInventoryRequest}
* @since 1.13
*/
protected SetInventory(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SetInventoryRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
}
@Override
public SetInventory set$Xgafv(java.lang.String $Xgafv) {
return (SetInventory) super.set$Xgafv($Xgafv);
}
@Override
public SetInventory setAccessToken(java.lang.String accessToken) {
return (SetInventory) super.setAccessToken(accessToken);
}
@Override
public SetInventory setAlt(java.lang.String alt) {
return (SetInventory) super.setAlt(alt);
}
@Override
public SetInventory setCallback(java.lang.String callback) {
return (SetInventory) super.setCallback(callback);
}
@Override
public SetInventory setFields(java.lang.String fields) {
return (SetInventory) super.setFields(fields);
}
@Override
public SetInventory setKey(java.lang.String key) {
return (SetInventory) super.setKey(key);
}
@Override
public SetInventory setOauthToken(java.lang.String oauthToken) {
return (SetInventory) super.setOauthToken(oauthToken);
}
@Override
public SetInventory setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetInventory) super.setPrettyPrint(prettyPrint);
}
@Override
public SetInventory setQuotaUser(java.lang.String quotaUser) {
return (SetInventory) super.setQuotaUser(quotaUser);
}
@Override
public SetInventory setUploadType(java.lang.String uploadType) {
return (SetInventory) super.setUploadType(uploadType);
}
@Override
public SetInventory setUploadProtocol(java.lang.String uploadProtocol) {
return (SetInventory) super.setUploadProtocol(uploadProtocol);
}
/**
* Immutable. Full resource name of the product, such as `projects/locations/global/ca
* talogs/default_catalog/branches/default_branch/products/product_id`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Immutable. Full resource name of the product, such as
`projects/locations/global/catalogs/default_catalog/branches/default_branch/products/product_id`.
*/
public java.lang.String getName() {
return name;
}
/**
* Immutable. Full resource name of the product, such as `projects/locations/global/ca
* talogs/default_catalog/branches/default_branch/products/product_id`.
*/
public SetInventory setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/branches/[^/]+/products/.*$");
}
this.name = name;
return this;
}
@Override
public SetInventory set(String parameterName, Object value) {
return (SetInventory) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the CompletionData collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.CompletionData.List request = retail.completionData().list(parameters ...)}
*
*
* @return the resource collection
*/
public CompletionData completionData() {
return new CompletionData();
}
/**
* The "completionData" collection of methods.
*/
public class CompletionData {
/**
* Bulk import of processed completion dataset. Request processing is asynchronous. Partial updating
* is not supported. The operation is successfully finished only after the imported suggestions are
* indexed successfully and ready for serving. The process takes hours. This feature is only
* available for users who have Retail Search enabled. Enable Retail Search on Cloud Console before
* using this feature.
*
* Create a request for the method "completionData.import".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link CloudRetailImport#execute()} method to invoke the remote operation.
*
* @param parent Required. The catalog which the suggestions dataset belongs to. Format:
* `projects/1234/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportCompletionDataRequest}
* @return the request
*/
public CloudRetailImport retailImport(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportCompletionDataRequest content) throws java.io.IOException {
CloudRetailImport result = new CloudRetailImport(parent, content);
initialize(result);
return result;
}
public class CloudRetailImport extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/completionData:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Bulk import of processed completion dataset. Request processing is asynchronous. Partial
* updating is not supported. The operation is successfully finished only after the imported
* suggestions are indexed successfully and ready for serving. The process takes hours. This
* feature is only available for users who have Retail Search enabled. Enable Retail Search on
* Cloud Console before using this feature.
*
* Create a request for the method "completionData.import".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link CloudRetailImport#execute()} method to invoke the remote operation.
* {@link CloudRetailImport#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param parent Required. The catalog which the suggestions dataset belongs to. Format:
* `projects/1234/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportCompletionDataRequest}
* @since 1.13
*/
protected CloudRetailImport(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportCompletionDataRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public CloudRetailImport set$Xgafv(java.lang.String $Xgafv) {
return (CloudRetailImport) super.set$Xgafv($Xgafv);
}
@Override
public CloudRetailImport setAccessToken(java.lang.String accessToken) {
return (CloudRetailImport) super.setAccessToken(accessToken);
}
@Override
public CloudRetailImport setAlt(java.lang.String alt) {
return (CloudRetailImport) super.setAlt(alt);
}
@Override
public CloudRetailImport setCallback(java.lang.String callback) {
return (CloudRetailImport) super.setCallback(callback);
}
@Override
public CloudRetailImport setFields(java.lang.String fields) {
return (CloudRetailImport) super.setFields(fields);
}
@Override
public CloudRetailImport setKey(java.lang.String key) {
return (CloudRetailImport) super.setKey(key);
}
@Override
public CloudRetailImport setOauthToken(java.lang.String oauthToken) {
return (CloudRetailImport) super.setOauthToken(oauthToken);
}
@Override
public CloudRetailImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CloudRetailImport) super.setPrettyPrint(prettyPrint);
}
@Override
public CloudRetailImport setQuotaUser(java.lang.String quotaUser) {
return (CloudRetailImport) super.setQuotaUser(quotaUser);
}
@Override
public CloudRetailImport setUploadType(java.lang.String uploadType) {
return (CloudRetailImport) super.setUploadType(uploadType);
}
@Override
public CloudRetailImport setUploadProtocol(java.lang.String uploadProtocol) {
return (CloudRetailImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The catalog which the suggestions dataset belongs to. Format:
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The catalog which the suggestions dataset belongs to. Format:
`projects/1234/locations/global/catalogs/default_catalog`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The catalog which the suggestions dataset belongs to. Format:
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
public CloudRetailImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public CloudRetailImport set(String parameterName, Object value) {
return (CloudRetailImport) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Controls collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Controls.List request = retail.controls().list(parameters ...)}
*
*
* @return the resource collection
*/
public Controls controls() {
return new Controls();
}
/**
* The "controls" collection of methods.
*/
public class Controls {
/**
* Creates a Control. If the Control to create already exists, an ALREADY_EXISTS error is returned.
*
* Create a request for the method "controls.create".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Full resource name of parent catalog. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/controls";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Creates a Control. If the Control to create already exists, an ALREADY_EXISTS error is
* returned.
*
* Create a request for the method "controls.create".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Full resource name of parent catalog. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of parent catalog. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Full resource name of parent catalog. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Full resource name of parent catalog. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the Control, which will become the final component of the
* Control's resource name. This value should be 4-63 characters, and valid characters
* are /a-z-_/.
*/
@com.google.api.client.util.Key
private java.lang.String controlId;
/** Required. The ID to use for the Control, which will become the final component of the Control's
resource name. This value should be 4-63 characters, and valid characters are /a-z-_/.
*/
public java.lang.String getControlId() {
return controlId;
}
/**
* Required. The ID to use for the Control, which will become the final component of the
* Control's resource name. This value should be 4-63 characters, and valid characters
* are /a-z-_/.
*/
public Create setControlId(java.lang.String controlId) {
this.controlId = controlId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a Control. If the Control to delete does not exist, a NOT_FOUND error is returned.
*
* Create a request for the method "controls.delete".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the Control to delete. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/controls/{control
* _id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
/**
* Deletes a Control. If the Control to delete does not exist, a NOT_FOUND error is returned.
*
* Create a request for the method "controls.delete".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the Control to delete. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/controls/{control
* _id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRetail.this, "DELETE", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Control to delete. Format: `projects/{project_numb
* er}/locations/{location_id}/catalogs/{catalog_id}/controls/{control_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the Control to delete. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/controls/{control_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the Control to delete. Format: `projects/{project_numb
* er}/locations/{location_id}/catalogs/{catalog_id}/controls/{control_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a Control.
*
* Create a request for the method "controls.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the Control to get. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/controls/{control
* _id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
/**
* Gets a Control.
*
* Create a request for the method "controls.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the Control to get. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/controls/{control
* _id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Control to get. Format: `projects/{project_number}
* /locations/{location_id}/catalogs/{catalog_id}/controls/{control_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the Control to get. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/controls/{control_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the Control to get. Format: `projects/{project_number}
* /locations/{location_id}/catalogs/{catalog_id}/controls/{control_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all Controls by their parent Catalog.
*
* Create a request for the method "controls.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/controls";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Lists all Controls by their parent Catalog.
*
* Create a request for the method "controls.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ListControlsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The catalog resource name. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A filter to apply on the list results. Supported features: * List all the
* products under the parent branch if filter is unset. * List controls that are used in
* a single ServingConfig: 'serving_config = "boosted_home_page_cvr"'
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter to apply on the list results. Supported features: * List all the products under
the parent branch if filter is unset. * List controls that are used in a single ServingConfig:
'serving_config = "boosted_home_page_cvr"'
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter to apply on the list results. Supported features: * List all the
* products under the parent branch if filter is unset. * List controls that are used in
* a single ServingConfig: 'serving_config = "boosted_home_page_cvr"'
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Maximum number of results to return. If unspecified, defaults to 50. Max
* allowed value is 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Maximum number of results to return. If unspecified, defaults to 50. Max allowed value is
1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Maximum number of results to return. If unspecified, defaults to 50. Max
* allowed value is 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListControls` call. Provide this to
* retrieve the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListControls` call. Provide this to retrieve the
subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListControls` call. Provide this to
* retrieve the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a Control. Control cannot be set to a different oneof field, if so an INVALID_ARGUMENT is
* returned. If the Control to update does not exist, a NOT_FOUND error is returned.
*
* Create a request for the method "controls.patch".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Immutable. Fully qualified name `projects/locations/global/catalogs/controls`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
/**
* Updates a Control. Control cannot be set to a different oneof field, if so an INVALID_ARGUMENT
* is returned. If the Control to update does not exist, a NOT_FOUND error is returned.
*
* Create a request for the method "controls.patch".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Immutable. Fully qualified name `projects/locations/global/catalogs/controls`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Control.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Immutable. Fully qualified name `projects/locations/global/catalogs/controls` */
@com.google.api.client.util.Key
private java.lang.String name;
/** Immutable. Fully qualified name `projects/locations/global/catalogs/controls`
*/
public java.lang.String getName() {
return name;
}
/** Immutable. Fully qualified name `projects/locations/global/catalogs/controls` */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/controls/[^/]+$");
}
this.name = name;
return this;
}
/**
* Indicates which fields in the provided Control to update. The following are NOT
* supported: * Control.name If not set or empty, all supported fields are updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Indicates which fields in the provided Control to update. The following are NOT supported: *
Control.name If not set or empty, all supported fields are updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Indicates which fields in the provided Control to update. The following are NOT
* supported: * Control.name If not set or empty, all supported fields are updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the GenerativeQuestion collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.GenerativeQuestion.List request = retail.generativeQuestion().list(parameters ...)}
*
*
* @return the resource collection
*/
public GenerativeQuestion generativeQuestion() {
return new GenerativeQuestion();
}
/**
* The "generativeQuestion" collection of methods.
*/
public class GenerativeQuestion {
/**
* Allows management of multiple questions.
*
* Create a request for the method "generativeQuestion.batchUpdate".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
*
* @param parent Optional. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2BatchUpdateGenerativeQuestionConfigsRequest}
* @return the request
*/
public BatchUpdate batchUpdate(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2BatchUpdateGenerativeQuestionConfigsRequest content) throws java.io.IOException {
BatchUpdate result = new BatchUpdate(parent, content);
initialize(result);
return result;
}
public class BatchUpdate extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/generativeQuestion:batchUpdate";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Allows management of multiple questions.
*
* Create a request for the method "generativeQuestion.batchUpdate".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link BatchUpdate#execute()} method to invoke the remote operation.
* {@link
* BatchUpdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Optional. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2BatchUpdateGenerativeQuestionConfigsRequest}
* @since 1.13
*/
protected BatchUpdate(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2BatchUpdateGenerativeQuestionConfigsRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2BatchUpdateGenerativeQuestionConfigsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public BatchUpdate set$Xgafv(java.lang.String $Xgafv) {
return (BatchUpdate) super.set$Xgafv($Xgafv);
}
@Override
public BatchUpdate setAccessToken(java.lang.String accessToken) {
return (BatchUpdate) super.setAccessToken(accessToken);
}
@Override
public BatchUpdate setAlt(java.lang.String alt) {
return (BatchUpdate) super.setAlt(alt);
}
@Override
public BatchUpdate setCallback(java.lang.String callback) {
return (BatchUpdate) super.setCallback(callback);
}
@Override
public BatchUpdate setFields(java.lang.String fields) {
return (BatchUpdate) super.setFields(fields);
}
@Override
public BatchUpdate setKey(java.lang.String key) {
return (BatchUpdate) super.setKey(key);
}
@Override
public BatchUpdate setOauthToken(java.lang.String oauthToken) {
return (BatchUpdate) super.setOauthToken(oauthToken);
}
@Override
public BatchUpdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchUpdate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchUpdate setQuotaUser(java.lang.String quotaUser) {
return (BatchUpdate) super.setQuotaUser(quotaUser);
}
@Override
public BatchUpdate setUploadType(java.lang.String uploadType) {
return (BatchUpdate) super.setUploadType(uploadType);
}
@Override
public BatchUpdate setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchUpdate) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Optional. Resource name of the parent catalog. Format:
projects/{project}/locations/{location}/catalogs/{catalog}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Optional. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
public BatchUpdate setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchUpdate set(String parameterName, Object value) {
return (BatchUpdate) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the GenerativeQuestions collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.GenerativeQuestions.List request = retail.generativeQuestions().list(parameters ...)}
*
*
* @return the resource collection
*/
public GenerativeQuestions generativeQuestions() {
return new GenerativeQuestions();
}
/**
* The "generativeQuestions" collection of methods.
*/
public class GenerativeQuestions {
/**
* Returns all questions for a given catalog.
*
* Create a request for the method "generativeQuestions.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/generativeQuestions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Returns all questions for a given catalog.
*
* Create a request for the method "generativeQuestions.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ListGenerativeQuestionConfigsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the parent catalog. Format:
projects/{project}/locations/{location}/catalogs/{catalog}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name of the parent catalog. Format:
* projects/{project}/locations/{location}/catalogs/{catalog}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Models collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Models.List request = retail.models().list(parameters ...)}
*
*
* @return the resource collection
*/
public Models models() {
return new Models();
}
/**
* The "models" collection of methods.
*/
public class Models {
/**
* Creates a new model.
*
* Create a request for the method "models.create".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource under which to create the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/models";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Creates a new model.
*
* Create a request for the method "models.create".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource under which to create the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource under which to create the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource under which to create the model. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource under which to create the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Whether to run a dry run to validate the request (without actually creating
* the model).
*/
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/** Optional. Whether to run a dry run to validate the request (without actually creating the model).
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/**
* Optional. Whether to run a dry run to validate the request (without actually creating
* the model).
*/
public Create setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an existing model.
*
* Create a request for the method "models.delete".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the Model to delete. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
/**
* Deletes an existing model.
*
* Create a request for the method "models.delete".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the Model to delete. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRetail.this, "DELETE", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Model to delete. Format: `projects/{project_number
* }/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the Model to delete. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the Model to delete. Format: `projects/{project_number
* }/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a model.
*
* Create a request for the method "models.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the Model to get. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog}/models/{model_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
/**
* Gets a model.
*
* Create a request for the method "models.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the Model to get. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog}/models/{model_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Model to get. Format: `projects/{project_number}/l
* ocations/{location_id}/catalogs/{catalog}/models/{model_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the Model to get. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog}/models/{model_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the Model to get. Format: `projects/{project_number}/l
* ocations/{location_id}/catalogs/{catalog}/models/{model_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the models linked to this event store.
*
* Create a request for the method "models.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent for which to list models. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/models";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Lists all the models linked to this event store.
*
* Create a request for the method "models.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent for which to list models. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ListModelsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent for which to list models. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent for which to list models. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent for which to list models. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Maximum number of results to return. If unspecified, defaults to 50. Max
* allowed value is 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Maximum number of results to return. If unspecified, defaults to 50. Max allowed value is
1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Maximum number of results to return. If unspecified, defaults to 50. Max
* allowed value is 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListModels` call. Provide this to
* retrieve the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListModels` call. Provide this to retrieve the
subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListModels` call. Provide this to
* retrieve the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Update of model metadata. Only fields that currently can be updated are: `filtering_option` and
* `periodic_tuning_state`. If other values are provided, this API method ignores them.
*
* Create a request for the method "models.patch".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The fully qualified resource name of the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* ` catalog_id has char limit of 50. recommendation_model_id has char limit of 40.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
/**
* Update of model metadata. Only fields that currently can be updated are: `filtering_option` and
* `periodic_tuning_state`. If other values are provided, this API method ignores them.
*
* Create a request for the method "models.patch".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The fully qualified resource name of the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* ` catalog_id has char limit of 50. recommendation_model_id has char limit of 40.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The fully qualified resource name of the model. Format: `projects/{project_
* number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}` catalog_id
* has char limit of 50. recommendation_model_id has char limit of 40.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The fully qualified resource name of the model. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
catalog_id has char limit of 50. recommendation_model_id has char limit of 40.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The fully qualified resource name of the model. Format: `projects/{project_
* number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}` catalog_id
* has char limit of 50. recommendation_model_id has char limit of 40.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Indicates which fields in the provided 'model' to update. If not set, by
* default updates all fields.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Indicates which fields in the provided 'model' to update. If not set, by default updates
all fields.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Indicates which fields in the provided 'model' to update. If not set, by
* default updates all fields.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Pauses the training of an existing model.
*
* Create a request for the method "models.pause".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Pause#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the model to pause. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PauseModelRequest}
* @return the request
*/
public Pause pause(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PauseModelRequest content) throws java.io.IOException {
Pause result = new Pause(name, content);
initialize(result);
return result;
}
public class Pause extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}:pause";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
/**
* Pauses the training of an existing model.
*
* Create a request for the method "models.pause".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Pause#execute()} method to invoke the remote operation. {@link
* Pause#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the model to pause. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PauseModelRequest}
* @since 1.13
*/
protected Pause(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PauseModelRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
}
@Override
public Pause set$Xgafv(java.lang.String $Xgafv) {
return (Pause) super.set$Xgafv($Xgafv);
}
@Override
public Pause setAccessToken(java.lang.String accessToken) {
return (Pause) super.setAccessToken(accessToken);
}
@Override
public Pause setAlt(java.lang.String alt) {
return (Pause) super.setAlt(alt);
}
@Override
public Pause setCallback(java.lang.String callback) {
return (Pause) super.setCallback(callback);
}
@Override
public Pause setFields(java.lang.String fields) {
return (Pause) super.setFields(fields);
}
@Override
public Pause setKey(java.lang.String key) {
return (Pause) super.setKey(key);
}
@Override
public Pause setOauthToken(java.lang.String oauthToken) {
return (Pause) super.setOauthToken(oauthToken);
}
@Override
public Pause setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Pause) super.setPrettyPrint(prettyPrint);
}
@Override
public Pause setQuotaUser(java.lang.String quotaUser) {
return (Pause) super.setQuotaUser(quotaUser);
}
@Override
public Pause setUploadType(java.lang.String uploadType) {
return (Pause) super.setUploadType(uploadType);
}
@Override
public Pause setUploadProtocol(java.lang.String uploadProtocol) {
return (Pause) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the model to pause. Format: `projects/{project_number}/location
* s/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the model to pause. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the model to pause. Format: `projects/{project_number}/location
* s/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public Pause setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Pause set(String parameterName, Object value) {
return (Pause) super.set(parameterName, value);
}
}
/**
* Resumes the training of an existing model.
*
* Create a request for the method "models.resume".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Resume#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the model to resume. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ResumeModelRequest}
* @return the request
*/
public Resume resume(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ResumeModelRequest content) throws java.io.IOException {
Resume result = new Resume(name, content);
initialize(result);
return result;
}
public class Resume extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}:resume";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
/**
* Resumes the training of an existing model.
*
* Create a request for the method "models.resume".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Resume#execute()} method to invoke the remote operation. {@link
* Resume#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the model to resume. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ResumeModelRequest}
* @since 1.13
*/
protected Resume(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ResumeModelRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2Model.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
}
@Override
public Resume set$Xgafv(java.lang.String $Xgafv) {
return (Resume) super.set$Xgafv($Xgafv);
}
@Override
public Resume setAccessToken(java.lang.String accessToken) {
return (Resume) super.setAccessToken(accessToken);
}
@Override
public Resume setAlt(java.lang.String alt) {
return (Resume) super.setAlt(alt);
}
@Override
public Resume setCallback(java.lang.String callback) {
return (Resume) super.setCallback(callback);
}
@Override
public Resume setFields(java.lang.String fields) {
return (Resume) super.setFields(fields);
}
@Override
public Resume setKey(java.lang.String key) {
return (Resume) super.setKey(key);
}
@Override
public Resume setOauthToken(java.lang.String oauthToken) {
return (Resume) super.setOauthToken(oauthToken);
}
@Override
public Resume setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Resume) super.setPrettyPrint(prettyPrint);
}
@Override
public Resume setQuotaUser(java.lang.String quotaUser) {
return (Resume) super.setQuotaUser(quotaUser);
}
@Override
public Resume setUploadType(java.lang.String uploadType) {
return (Resume) super.setUploadType(uploadType);
}
@Override
public Resume setUploadProtocol(java.lang.String uploadProtocol) {
return (Resume) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the model to resume. Format: `projects/{project_number}/locatio
* ns/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the model to resume. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the model to resume. Format: `projects/{project_number}/locatio
* ns/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public Resume setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Resume set(String parameterName, Object value) {
return (Resume) super.set(parameterName, value);
}
}
/**
* Tunes an existing model.
*
* Create a request for the method "models.tune".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Tune#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the model to tune. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2TuneModelRequest}
* @return the request
*/
public Tune tune(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2TuneModelRequest content) throws java.io.IOException {
Tune result = new Tune(name, content);
initialize(result);
return result;
}
public class Tune extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}:tune";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
/**
* Tunes an existing model.
*
* Create a request for the method "models.tune".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Tune#execute()} method to invoke the remote operation. {@link
* Tune#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the model to tune. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}
* `
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2TuneModelRequest}
* @since 1.13
*/
protected Tune(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2TuneModelRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
}
@Override
public Tune set$Xgafv(java.lang.String $Xgafv) {
return (Tune) super.set$Xgafv($Xgafv);
}
@Override
public Tune setAccessToken(java.lang.String accessToken) {
return (Tune) super.setAccessToken(accessToken);
}
@Override
public Tune setAlt(java.lang.String alt) {
return (Tune) super.setAlt(alt);
}
@Override
public Tune setCallback(java.lang.String callback) {
return (Tune) super.setCallback(callback);
}
@Override
public Tune setFields(java.lang.String fields) {
return (Tune) super.setFields(fields);
}
@Override
public Tune setKey(java.lang.String key) {
return (Tune) super.setKey(key);
}
@Override
public Tune setOauthToken(java.lang.String oauthToken) {
return (Tune) super.setOauthToken(oauthToken);
}
@Override
public Tune setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Tune) super.setPrettyPrint(prettyPrint);
}
@Override
public Tune setQuotaUser(java.lang.String quotaUser) {
return (Tune) super.setQuotaUser(quotaUser);
}
@Override
public Tune setUploadType(java.lang.String uploadType) {
return (Tune) super.setUploadType(uploadType);
}
@Override
public Tune setUploadProtocol(java.lang.String uploadProtocol) {
return (Tune) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the model to tune. Format: `projects/{project_number}/
* locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the model to tune. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the model to tune. Format: `projects/{project_number}/
* locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
*/
public Tune setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/models/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Tune set(String parameterName, Object value) {
return (Tune) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Operations.List request = retail.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleLongrunningListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Placements collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Placements.List request = retail.placements().list(parameters ...)}
*
*
* @return the resource collection
*/
public Placements placements() {
return new Placements();
}
/**
* The "placements" collection of methods.
*/
public class Placements {
/**
* Makes a recommendation prediction.
*
* Create a request for the method "placements.predict".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Predict#execute()} method to invoke the remote operation.
*
* @param placement Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We recommend
* using the `servingConfigs` resource. `placements` is a legacy resource. The ID of the
* Recommendations AI serving config or placement. Before you can request predictions from
* your model, you must create at least one serving config or placement for it. For more
* information, see [Manage serving configs] (https://cloud.google.com/retail/docs/manage-
* configs). The full list of available serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest}
* @return the request
*/
public Predict predict(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest content) throws java.io.IOException {
Predict result = new Predict(placement, content);
initialize(result);
return result;
}
public class Predict extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+placement}:predict";
private final java.util.regex.Pattern PLACEMENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/placements/[^/]+$");
/**
* Makes a recommendation prediction.
*
* Create a request for the method "placements.predict".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Predict#execute()} method to invoke the remote operation.
* {@link
* Predict#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param placement Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We recommend
* using the `servingConfigs` resource. `placements` is a legacy resource. The ID of the
* Recommendations AI serving config or placement. Before you can request predictions from
* your model, you must create at least one serving config or placement for it. For more
* information, see [Manage serving configs] (https://cloud.google.com/retail/docs/manage-
* configs). The full list of available serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest}
* @since 1.13
*/
protected Predict(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictResponse.class);
this.placement = com.google.api.client.util.Preconditions.checkNotNull(placement, "Required parameter placement must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/placements/[^/]+$");
}
}
@Override
public Predict set$Xgafv(java.lang.String $Xgafv) {
return (Predict) super.set$Xgafv($Xgafv);
}
@Override
public Predict setAccessToken(java.lang.String accessToken) {
return (Predict) super.setAccessToken(accessToken);
}
@Override
public Predict setAlt(java.lang.String alt) {
return (Predict) super.setAlt(alt);
}
@Override
public Predict setCallback(java.lang.String callback) {
return (Predict) super.setCallback(callback);
}
@Override
public Predict setFields(java.lang.String fields) {
return (Predict) super.setFields(fields);
}
@Override
public Predict setKey(java.lang.String key) {
return (Predict) super.setKey(key);
}
@Override
public Predict setOauthToken(java.lang.String oauthToken) {
return (Predict) super.setOauthToken(oauthToken);
}
@Override
public Predict setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Predict) super.setPrettyPrint(prettyPrint);
}
@Override
public Predict setQuotaUser(java.lang.String quotaUser) {
return (Predict) super.setQuotaUser(quotaUser);
}
@Override
public Predict setUploadType(java.lang.String uploadType) {
return (Predict) super.setUploadType(uploadType);
}
@Override
public Predict setUploadProtocol(java.lang.String uploadProtocol) {
return (Predict) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We
* recommend using the `servingConfigs` resource. `placements` is a legacy resource. The
* ID of the Recommendations AI serving config or placement. Before you can request
* predictions from your model, you must create at least one serving config or placement
* for it. For more information, see [Manage serving configs]
* (https://cloud.google.com/retail/docs/manage-configs). The full list of available
* serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
*/
@com.google.api.client.util.Key
private java.lang.String placement;
/** Required. Full resource name of the format:
`{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
`{placement=projects/locations/global/catalogs/default_catalog/placements}`. We recommend using the
`servingConfigs` resource. `placements` is a legacy resource. The ID of the Recommendations AI
serving config or placement. Before you can request predictions from your model, you must create at
least one serving config or placement for it. For more information, see [Manage serving configs]
(https://cloud.google.com/retail/docs/manage-configs). The full list of available serving configs
can be seen at https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
*/
public java.lang.String getPlacement() {
return placement;
}
/**
* Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We
* recommend using the `servingConfigs` resource. `placements` is a legacy resource. The
* ID of the Recommendations AI serving config or placement. Before you can request
* predictions from your model, you must create at least one serving config or placement
* for it. For more information, see [Manage serving configs]
* (https://cloud.google.com/retail/docs/manage-configs). The full list of available
* serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
*/
public Predict setPlacement(java.lang.String placement) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/placements/[^/]+$");
}
this.placement = placement;
return this;
}
@Override
public Predict set(String parameterName, Object value) {
return (Predict) super.set(parameterName, value);
}
}
/**
* Performs a search. This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
* Create a request for the method "placements.search".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param placement Required. The resource name of the Retail Search serving config, such as
* `projects/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config`
* or the name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This field
* is used to identify the serving config name and the set of models that are used to make
* the search.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest}
* @return the request
*/
public Search search(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest content) throws java.io.IOException {
Search result = new Search(placement, content);
initialize(result);
return result;
}
public class Search extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+placement}:search";
private final java.util.regex.Pattern PLACEMENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/placements/[^/]+$");
/**
* Performs a search. This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
* Create a request for the method "placements.search".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation. {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param placement Required. The resource name of the Retail Search serving config, such as
* `projects/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config`
* or the name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This field
* is used to identify the serving config name and the set of models that are used to make
* the search.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest}
* @since 1.13
*/
protected Search(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchResponse.class);
this.placement = com.google.api.client.util.Preconditions.checkNotNull(placement, "Required parameter placement must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/placements/[^/]+$");
}
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Retail Search serving config, such as `projects/lo
* cations/global/catalogs/default_catalog/servingConfigs/default_serving_config` or the
* name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This
* field is used to identify the serving config name and the set of models that are used
* to make the search.
*/
@com.google.api.client.util.Key
private java.lang.String placement;
/** Required. The resource name of the Retail Search serving config, such as
`projects/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config` or the
name of the legacy placement resource, such as
`projects/locations/global/catalogs/default_catalog/placements/default_search`. This field is used
to identify the serving config name and the set of models that are used to make the search.
*/
public java.lang.String getPlacement() {
return placement;
}
/**
* Required. The resource name of the Retail Search serving config, such as `projects/lo
* cations/global/catalogs/default_catalog/servingConfigs/default_serving_config` or the
* name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This
* field is used to identify the serving config name and the set of models that are used
* to make the search.
*/
public Search setPlacement(java.lang.String placement) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/placements/[^/]+$");
}
this.placement = placement;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ServingConfigs collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.ServingConfigs.List request = retail.servingConfigs().list(parameters ...)}
*
*
* @return the resource collection
*/
public ServingConfigs servingConfigs() {
return new ServingConfigs();
}
/**
* The "servingConfigs" collection of methods.
*/
public class ServingConfigs {
/**
* Enables a Control on the specified ServingConfig. The control is added in the last position of
* the list of controls it belongs to (e.g. if it's a facet spec control it will be applied in the
* last position of servingConfig.facetSpecIds) Returns a ALREADY_EXISTS error if the control has
* already been applied. Returns a FAILED_PRECONDITION error if the addition could exceed maximum
* number of control allowed for that type of control.
*
* Create a request for the method "servingConfigs.addControl".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link AddControl#execute()} method to invoke the remote operation.
*
* @param servingConfig Required. The source ServingConfig resource name . Format: `projects/{project_number}/locations/{loc
* ation_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddControlRequest}
* @return the request
*/
public AddControl addControl(java.lang.String servingConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddControlRequest content) throws java.io.IOException {
AddControl result = new AddControl(servingConfig, content);
initialize(result);
return result;
}
public class AddControl extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+servingConfig}:addControl";
private final java.util.regex.Pattern SERVING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
/**
* Enables a Control on the specified ServingConfig. The control is added in the last position of
* the list of controls it belongs to (e.g. if it's a facet spec control it will be applied in the
* last position of servingConfig.facetSpecIds) Returns a ALREADY_EXISTS error if the control has
* already been applied. Returns a FAILED_PRECONDITION error if the addition could exceed maximum
* number of control allowed for that type of control.
*
* Create a request for the method "servingConfigs.addControl".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link AddControl#execute()} method to invoke the remote operation.
* {@link
* AddControl#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param servingConfig Required. The source ServingConfig resource name . Format: `projects/{project_number}/locations/{loc
* ation_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddControlRequest}
* @since 1.13
*/
protected AddControl(java.lang.String servingConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2AddControlRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig.class);
this.servingConfig = com.google.api.client.util.Preconditions.checkNotNull(servingConfig, "Required parameter servingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SERVING_CONFIG_PATTERN.matcher(servingConfig).matches(),
"Parameter servingConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
}
@Override
public AddControl set$Xgafv(java.lang.String $Xgafv) {
return (AddControl) super.set$Xgafv($Xgafv);
}
@Override
public AddControl setAccessToken(java.lang.String accessToken) {
return (AddControl) super.setAccessToken(accessToken);
}
@Override
public AddControl setAlt(java.lang.String alt) {
return (AddControl) super.setAlt(alt);
}
@Override
public AddControl setCallback(java.lang.String callback) {
return (AddControl) super.setCallback(callback);
}
@Override
public AddControl setFields(java.lang.String fields) {
return (AddControl) super.setFields(fields);
}
@Override
public AddControl setKey(java.lang.String key) {
return (AddControl) super.setKey(key);
}
@Override
public AddControl setOauthToken(java.lang.String oauthToken) {
return (AddControl) super.setOauthToken(oauthToken);
}
@Override
public AddControl setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddControl) super.setPrettyPrint(prettyPrint);
}
@Override
public AddControl setQuotaUser(java.lang.String quotaUser) {
return (AddControl) super.setQuotaUser(quotaUser);
}
@Override
public AddControl setUploadType(java.lang.String uploadType) {
return (AddControl) super.setUploadType(uploadType);
}
@Override
public AddControl setUploadProtocol(java.lang.String uploadProtocol) {
return (AddControl) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The source ServingConfig resource name . Format: `projects/{project_number}
* /locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
@com.google.api.client.util.Key
private java.lang.String servingConfig;
/** Required. The source ServingConfig resource name . Format: `projects/{project_number}/locations/{lo
cation_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
public java.lang.String getServingConfig() {
return servingConfig;
}
/**
* Required. The source ServingConfig resource name . Format: `projects/{project_number}
* /locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
public AddControl setServingConfig(java.lang.String servingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SERVING_CONFIG_PATTERN.matcher(servingConfig).matches(),
"Parameter servingConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
this.servingConfig = servingConfig;
return this;
}
@Override
public AddControl set(String parameterName, Object value) {
return (AddControl) super.set(parameterName, value);
}
}
/**
* Creates a ServingConfig. A maximum of 100 ServingConfigs are allowed in a Catalog, otherwise a
* FAILED_PRECONDITION error is returned.
*
* Create a request for the method "servingConfigs.create".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Full resource name of parent. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/servingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Creates a ServingConfig. A maximum of 100 ServingConfigs are allowed in a Catalog, otherwise a
* FAILED_PRECONDITION error is returned.
*
* Create a request for the method "servingConfigs.create".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Full resource name of parent. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of parent. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Full resource name of parent. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Full resource name of parent. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the ServingConfig, which will become the final component
* of the ServingConfig's resource name. This value should be 4-63 characters, and valid
* characters are /a-z-_/.
*/
@com.google.api.client.util.Key
private java.lang.String servingConfigId;
/** Required. The ID to use for the ServingConfig, which will become the final component of the
ServingConfig's resource name. This value should be 4-63 characters, and valid characters are
/a-z-_/.
*/
public java.lang.String getServingConfigId() {
return servingConfigId;
}
/**
* Required. The ID to use for the ServingConfig, which will become the final component
* of the ServingConfig's resource name. This value should be 4-63 characters, and valid
* characters are /a-z-_/.
*/
public Create setServingConfigId(java.lang.String servingConfigId) {
this.servingConfigId = servingConfigId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a ServingConfig. Returns a NotFound error if the ServingConfig does not exist.
*
* Create a request for the method "servingConfigs.delete".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the ServingConfig to delete. Format: `projects/{project_number}/locat
* ions/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
/**
* Deletes a ServingConfig. Returns a NotFound error if the ServingConfig does not exist.
*
* Create a request for the method "servingConfigs.delete".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the ServingConfig to delete. Format: `projects/{project_number}/locat
* ions/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRetail.this, "DELETE", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the ServingConfig to delete. Format: `projects/{projec
* t_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_confi
* g_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the ServingConfig to delete. Format: `projects/{project_number}/loca
tions/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the ServingConfig to delete. Format: `projects/{projec
* t_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_confi
* g_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a ServingConfig. Returns a NotFound error if the ServingConfig does not exist.
*
* Create a request for the method "servingConfigs.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the ServingConfig to get. Format: `projects/{project_number}/location
* s/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
/**
* Gets a ServingConfig. Returns a NotFound error if the ServingConfig does not exist.
*
* Create a request for the method "servingConfigs.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the ServingConfig to get. Format: `projects/{project_number}/location
* s/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the ServingConfig to get. Format: `projects/{project_n
* umber}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_i
* d}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the ServingConfig to get. Format: `projects/{project_number}/locatio
ns/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the ServingConfig to get. Format: `projects/{project_n
* umber}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_i
* d}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all ServingConfigs linked to this catalog.
*
* Create a request for the method "servingConfigs.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/servingConfigs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Lists all ServingConfigs linked to this catalog.
*
* Create a request for the method "servingConfigs.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ListServingConfigsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The catalog resource name. Format:
`projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Maximum number of results to return. If unspecified, defaults to 100. If a
* value greater than 100 is provided, at most 100 results are returned.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Maximum number of results to return. If unspecified, defaults to 100. If a value greater
than 100 is provided, at most 100 results are returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Maximum number of results to return. If unspecified, defaults to 100. If a
* value greater than 100 is provided, at most 100 results are returned.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListServingConfigs` call. Provide
* this to retrieve the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListServingConfigs` call. Provide this to
retrieve the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListServingConfigs` call. Provide
* this to retrieve the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a ServingConfig.
*
* Create a request for the method "servingConfigs.patch".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Immutable. Fully qualified name `projects/locations/global/catalogs/servingConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
/**
* Updates a ServingConfig.
*
* Create a request for the method "servingConfigs.patch".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Immutable. Fully qualified name `projects/locations/global/catalogs/servingConfig`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig content) {
super(CloudRetail.this, "PATCH", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Immutable. Fully qualified name `projects/locations/global/catalogs/servingConfig`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Immutable. Fully qualified name `projects/locations/global/catalogs/servingConfig`
*/
public java.lang.String getName() {
return name;
}
/**
* Immutable. Fully qualified name `projects/locations/global/catalogs/servingConfig`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
this.name = name;
return this;
}
/**
* Indicates which fields in the provided ServingConfig to update. The following are NOT
* supported: * ServingConfig.name If not set, all supported fields are updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Indicates which fields in the provided ServingConfig to update. The following are NOT supported: *
ServingConfig.name If not set, all supported fields are updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Indicates which fields in the provided ServingConfig to update. The following are NOT
* supported: * ServingConfig.name If not set, all supported fields are updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Makes a recommendation prediction.
*
* Create a request for the method "servingConfigs.predict".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Predict#execute()} method to invoke the remote operation.
*
* @param placement Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We recommend
* using the `servingConfigs` resource. `placements` is a legacy resource. The ID of the
* Recommendations AI serving config or placement. Before you can request predictions from
* your model, you must create at least one serving config or placement for it. For more
* information, see [Manage serving configs] (https://cloud.google.com/retail/docs/manage-
* configs). The full list of available serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest}
* @return the request
*/
public Predict predict(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest content) throws java.io.IOException {
Predict result = new Predict(placement, content);
initialize(result);
return result;
}
public class Predict extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+placement}:predict";
private final java.util.regex.Pattern PLACEMENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
/**
* Makes a recommendation prediction.
*
* Create a request for the method "servingConfigs.predict".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Predict#execute()} method to invoke the remote operation.
* {@link
* Predict#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param placement Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We recommend
* using the `servingConfigs` resource. `placements` is a legacy resource. The ID of the
* Recommendations AI serving config or placement. Before you can request predictions from
* your model, you must create at least one serving config or placement for it. For more
* information, see [Manage serving configs] (https://cloud.google.com/retail/docs/manage-
* configs). The full list of available serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest}
* @since 1.13
*/
protected Predict(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PredictResponse.class);
this.placement = com.google.api.client.util.Preconditions.checkNotNull(placement, "Required parameter placement must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
}
@Override
public Predict set$Xgafv(java.lang.String $Xgafv) {
return (Predict) super.set$Xgafv($Xgafv);
}
@Override
public Predict setAccessToken(java.lang.String accessToken) {
return (Predict) super.setAccessToken(accessToken);
}
@Override
public Predict setAlt(java.lang.String alt) {
return (Predict) super.setAlt(alt);
}
@Override
public Predict setCallback(java.lang.String callback) {
return (Predict) super.setCallback(callback);
}
@Override
public Predict setFields(java.lang.String fields) {
return (Predict) super.setFields(fields);
}
@Override
public Predict setKey(java.lang.String key) {
return (Predict) super.setKey(key);
}
@Override
public Predict setOauthToken(java.lang.String oauthToken) {
return (Predict) super.setOauthToken(oauthToken);
}
@Override
public Predict setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Predict) super.setPrettyPrint(prettyPrint);
}
@Override
public Predict setQuotaUser(java.lang.String quotaUser) {
return (Predict) super.setQuotaUser(quotaUser);
}
@Override
public Predict setUploadType(java.lang.String uploadType) {
return (Predict) super.setUploadType(uploadType);
}
@Override
public Predict setUploadProtocol(java.lang.String uploadProtocol) {
return (Predict) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We
* recommend using the `servingConfigs` resource. `placements` is a legacy resource. The
* ID of the Recommendations AI serving config or placement. Before you can request
* predictions from your model, you must create at least one serving config or placement
* for it. For more information, see [Manage serving configs]
* (https://cloud.google.com/retail/docs/manage-configs). The full list of available
* serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
*/
@com.google.api.client.util.Key
private java.lang.String placement;
/** Required. Full resource name of the format:
`{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
`{placement=projects/locations/global/catalogs/default_catalog/placements}`. We recommend using the
`servingConfigs` resource. `placements` is a legacy resource. The ID of the Recommendations AI
serving config or placement. Before you can request predictions from your model, you must create at
least one serving config or placement for it. For more information, see [Manage serving configs]
(https://cloud.google.com/retail/docs/manage-configs). The full list of available serving configs
can be seen at https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
*/
public java.lang.String getPlacement() {
return placement;
}
/**
* Required. Full resource name of the format:
* `{placement=projects/locations/global/catalogs/default_catalog/servingConfigs}` or
* `{placement=projects/locations/global/catalogs/default_catalog/placements}`. We
* recommend using the `servingConfigs` resource. `placements` is a legacy resource. The
* ID of the Recommendations AI serving config or placement. Before you can request
* predictions from your model, you must create at least one serving config or placement
* for it. For more information, see [Manage serving configs]
* (https://cloud.google.com/retail/docs/manage-configs). The full list of available
* serving configs can be seen at
* https://console.cloud.google.com/ai/retail/catalogs/default_catalog/configs
*/
public Predict setPlacement(java.lang.String placement) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
this.placement = placement;
return this;
}
@Override
public Predict set(String parameterName, Object value) {
return (Predict) super.set(parameterName, value);
}
}
/**
* Disables a Control on the specified ServingConfig. The control is removed from the ServingConfig.
* Returns a NOT_FOUND error if the Control is not enabled for the ServingConfig.
*
* Create a request for the method "servingConfigs.removeControl".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link RemoveControl#execute()} method to invoke the remote operation.
*
* @param servingConfig Required. The source ServingConfig resource name . Format: `projects/{project_number}/locations/{loc
* ation_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveControlRequest}
* @return the request
*/
public RemoveControl removeControl(java.lang.String servingConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveControlRequest content) throws java.io.IOException {
RemoveControl result = new RemoveControl(servingConfig, content);
initialize(result);
return result;
}
public class RemoveControl extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+servingConfig}:removeControl";
private final java.util.regex.Pattern SERVING_CONFIG_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
/**
* Disables a Control on the specified ServingConfig. The control is removed from the
* ServingConfig. Returns a NOT_FOUND error if the Control is not enabled for the ServingConfig.
*
* Create a request for the method "servingConfigs.removeControl".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link RemoveControl#execute()} method to invoke the remote operation.
* {@link RemoveControl#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param servingConfig Required. The source ServingConfig resource name . Format: `projects/{project_number}/locations/{loc
* ation_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveControlRequest}
* @since 1.13
*/
protected RemoveControl(java.lang.String servingConfig, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RemoveControlRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ServingConfig.class);
this.servingConfig = com.google.api.client.util.Preconditions.checkNotNull(servingConfig, "Required parameter servingConfig must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SERVING_CONFIG_PATTERN.matcher(servingConfig).matches(),
"Parameter servingConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
}
@Override
public RemoveControl set$Xgafv(java.lang.String $Xgafv) {
return (RemoveControl) super.set$Xgafv($Xgafv);
}
@Override
public RemoveControl setAccessToken(java.lang.String accessToken) {
return (RemoveControl) super.setAccessToken(accessToken);
}
@Override
public RemoveControl setAlt(java.lang.String alt) {
return (RemoveControl) super.setAlt(alt);
}
@Override
public RemoveControl setCallback(java.lang.String callback) {
return (RemoveControl) super.setCallback(callback);
}
@Override
public RemoveControl setFields(java.lang.String fields) {
return (RemoveControl) super.setFields(fields);
}
@Override
public RemoveControl setKey(java.lang.String key) {
return (RemoveControl) super.setKey(key);
}
@Override
public RemoveControl setOauthToken(java.lang.String oauthToken) {
return (RemoveControl) super.setOauthToken(oauthToken);
}
@Override
public RemoveControl setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveControl) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveControl setQuotaUser(java.lang.String quotaUser) {
return (RemoveControl) super.setQuotaUser(quotaUser);
}
@Override
public RemoveControl setUploadType(java.lang.String uploadType) {
return (RemoveControl) super.setUploadType(uploadType);
}
@Override
public RemoveControl setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveControl) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The source ServingConfig resource name . Format: `projects/{project_number}
* /locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
@com.google.api.client.util.Key
private java.lang.String servingConfig;
/** Required. The source ServingConfig resource name . Format: `projects/{project_number}/locations/{lo
cation_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
public java.lang.String getServingConfig() {
return servingConfig;
}
/**
* Required. The source ServingConfig resource name . Format: `projects/{project_number}
* /locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
*/
public RemoveControl setServingConfig(java.lang.String servingConfig) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SERVING_CONFIG_PATTERN.matcher(servingConfig).matches(),
"Parameter servingConfig must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
this.servingConfig = servingConfig;
return this;
}
@Override
public RemoveControl set(String parameterName, Object value) {
return (RemoveControl) super.set(parameterName, value);
}
}
/**
* Performs a search. This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
* Create a request for the method "servingConfigs.search".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param placement Required. The resource name of the Retail Search serving config, such as
* `projects/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config`
* or the name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This field
* is used to identify the serving config name and the set of models that are used to make
* the search.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest}
* @return the request
*/
public Search search(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest content) throws java.io.IOException {
Search result = new Search(placement, content);
initialize(result);
return result;
}
public class Search extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+placement}:search";
private final java.util.regex.Pattern PLACEMENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
/**
* Performs a search. This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
* Create a request for the method "servingConfigs.search".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation. {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param placement Required. The resource name of the Retail Search serving config, such as
* `projects/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config`
* or the name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This field
* is used to identify the serving config name and the set of models that are used to make
* the search.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest}
* @since 1.13
*/
protected Search(java.lang.String placement, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchResponse.class);
this.placement = com.google.api.client.util.Preconditions.checkNotNull(placement, "Required parameter placement must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Retail Search serving config, such as `projects/lo
* cations/global/catalogs/default_catalog/servingConfigs/default_serving_config` or the
* name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This
* field is used to identify the serving config name and the set of models that are used
* to make the search.
*/
@com.google.api.client.util.Key
private java.lang.String placement;
/** Required. The resource name of the Retail Search serving config, such as
`projects/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config` or the
name of the legacy placement resource, such as
`projects/locations/global/catalogs/default_catalog/placements/default_search`. This field is used
to identify the serving config name and the set of models that are used to make the search.
*/
public java.lang.String getPlacement() {
return placement;
}
/**
* Required. The resource name of the Retail Search serving config, such as `projects/lo
* cations/global/catalogs/default_catalog/servingConfigs/default_serving_config` or the
* name of the legacy placement resource, such as
* `projects/locations/global/catalogs/default_catalog/placements/default_search`. This
* field is used to identify the serving config name and the set of models that are used
* to make the search.
*/
public Search setPlacement(java.lang.String placement) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PLACEMENT_PATTERN.matcher(placement).matches(),
"Parameter placement must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+/servingConfigs/[^/]+$");
}
this.placement = placement;
return this;
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the UserEvents collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.UserEvents.List request = retail.userEvents().list(parameters ...)}
*
*
* @return the resource collection
*/
public UserEvents userEvents() {
return new UserEvents();
}
/**
* The "userEvents" collection of methods.
*/
public class UserEvents {
/**
* Writes a single user event from the browser. This uses a GET request to due to browser
* restriction of POST-ing to a 3rd party domain. This method is used only by the Retail API
* JavaScript pixel and Google Tag Manager. Users should not call this method directly.
*
* Create a request for the method "userEvents.collect".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Collect#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent catalog name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
* @return the request
*/
public Collect collect(java.lang.String parent) throws java.io.IOException {
Collect result = new Collect(parent);
initialize(result);
return result;
}
public class Collect extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/userEvents:collect";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Writes a single user event from the browser. This uses a GET request to due to browser
* restriction of POST-ing to a 3rd party domain. This method is used only by the Retail API
* JavaScript pixel and Google Tag Manager. Users should not call this method directly.
*
* Create a request for the method "userEvents.collect".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Collect#execute()} method to invoke the remote operation.
* {@link
* Collect#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent catalog name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
* @since 1.13
*/
protected Collect(java.lang.String parent) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleApiHttpBody.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Collect set$Xgafv(java.lang.String $Xgafv) {
return (Collect) super.set$Xgafv($Xgafv);
}
@Override
public Collect setAccessToken(java.lang.String accessToken) {
return (Collect) super.setAccessToken(accessToken);
}
@Override
public Collect setAlt(java.lang.String alt) {
return (Collect) super.setAlt(alt);
}
@Override
public Collect setCallback(java.lang.String callback) {
return (Collect) super.setCallback(callback);
}
@Override
public Collect setFields(java.lang.String fields) {
return (Collect) super.setFields(fields);
}
@Override
public Collect setKey(java.lang.String key) {
return (Collect) super.setKey(key);
}
@Override
public Collect setOauthToken(java.lang.String oauthToken) {
return (Collect) super.setOauthToken(oauthToken);
}
@Override
public Collect setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Collect) super.setPrettyPrint(prettyPrint);
}
@Override
public Collect setQuotaUser(java.lang.String quotaUser) {
return (Collect) super.setQuotaUser(quotaUser);
}
@Override
public Collect setUploadType(java.lang.String uploadType) {
return (Collect) super.setUploadType(uploadType);
}
@Override
public Collect setUploadProtocol(java.lang.String uploadProtocol) {
return (Collect) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent catalog name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent catalog name, such as
`projects/1234/locations/global/catalogs/default_catalog`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent catalog name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
public Collect setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The event timestamp in milliseconds. This prevents browser caching of otherwise
* identical get requests. The name is abbreviated to reduce the payload bytes.
*/
@com.google.api.client.util.Key
private java.lang.Long ets;
/** The event timestamp in milliseconds. This prevents browser caching of otherwise identical get
requests. The name is abbreviated to reduce the payload bytes.
*/
public java.lang.Long getEts() {
return ets;
}
/**
* The event timestamp in milliseconds. This prevents browser caching of otherwise
* identical get requests. The name is abbreviated to reduce the payload bytes.
*/
public Collect setEts(java.lang.Long ets) {
this.ets = ets;
return this;
}
/**
* The prebuilt rule name that can convert a specific type of raw_json. For example:
* "ga4_bq" rule for the GA4 user event schema.
*/
@com.google.api.client.util.Key
private java.lang.String prebuiltRule;
/** The prebuilt rule name that can convert a specific type of raw_json. For example: "ga4_bq" rule for
the GA4 user event schema.
*/
public java.lang.String getPrebuiltRule() {
return prebuiltRule;
}
/**
* The prebuilt rule name that can convert a specific type of raw_json. For example:
* "ga4_bq" rule for the GA4 user event schema.
*/
public Collect setPrebuiltRule(java.lang.String prebuiltRule) {
this.prebuiltRule = prebuiltRule;
return this;
}
/**
* An arbitrary serialized JSON string that contains necessary information that can
* comprise a user event. When this field is specified, the user_event field will be
* ignored. Note: line-delimited JSON is not supported, a single JSON only.
*/
@com.google.api.client.util.Key
private java.lang.String rawJson;
/** An arbitrary serialized JSON string that contains necessary information that can comprise a user
event. When this field is specified, the user_event field will be ignored. Note: line-delimited
JSON is not supported, a single JSON only.
*/
public java.lang.String getRawJson() {
return rawJson;
}
/**
* An arbitrary serialized JSON string that contains necessary information that can
* comprise a user event. When this field is specified, the user_event field will be
* ignored. Note: line-delimited JSON is not supported, a single JSON only.
*/
public Collect setRawJson(java.lang.String rawJson) {
this.rawJson = rawJson;
return this;
}
/**
* The URL including cgi-parameters but excluding the hash fragment with a length limit
* of 5,000 characters. This is often more useful than the referer URL, because many
* browsers only send the domain for 3rd party requests.
*/
@com.google.api.client.util.Key
private java.lang.String uri;
/** The URL including cgi-parameters but excluding the hash fragment with a length limit of 5,000
characters. This is often more useful than the referer URL, because many browsers only send the
domain for 3rd party requests.
*/
public java.lang.String getUri() {
return uri;
}
/**
* The URL including cgi-parameters but excluding the hash fragment with a length limit
* of 5,000 characters. This is often more useful than the referer URL, because many
* browsers only send the domain for 3rd party requests.
*/
public Collect setUri(java.lang.String uri) {
this.uri = uri;
return this;
}
/**
* Required. URL encoded UserEvent proto with a length limit of 2,000,000 characters.
*/
@com.google.api.client.util.Key
private java.lang.String userEvent;
/** Required. URL encoded UserEvent proto with a length limit of 2,000,000 characters.
*/
public java.lang.String getUserEvent() {
return userEvent;
}
/**
* Required. URL encoded UserEvent proto with a length limit of 2,000,000 characters.
*/
public Collect setUserEvent(java.lang.String userEvent) {
this.userEvent = userEvent;
return this;
}
@Override
public Collect set(String parameterName, Object value) {
return (Collect) super.set(parameterName, value);
}
}
/**
* Bulk import of User events. Request processing might be synchronous. Events that already exist
* are skipped. Use this method for backfilling historical user events. `Operation.response` is of
* type `ImportResponse`. Note that it is possible for a subset of the items to be successfully
* inserted. `Operation.metadata` is of type `ImportMetadata`.
*
* Create a request for the method "userEvents.import".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link CloudRetailImport#execute()} method to invoke the remote operation.
*
* @param parent Required. `projects/1234/locations/global/catalogs/default_catalog`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportUserEventsRequest}
* @return the request
*/
public CloudRetailImport retailImport(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportUserEventsRequest content) throws java.io.IOException {
CloudRetailImport result = new CloudRetailImport(parent, content);
initialize(result);
return result;
}
public class CloudRetailImport extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/userEvents:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Bulk import of User events. Request processing might be synchronous. Events that already exist
* are skipped. Use this method for backfilling historical user events. `Operation.response` is of
* type `ImportResponse`. Note that it is possible for a subset of the items to be successfully
* inserted. `Operation.metadata` is of type `ImportMetadata`.
*
* Create a request for the method "userEvents.import".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link CloudRetailImport#execute()} method to invoke the remote operation.
* {@link CloudRetailImport#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param parent Required. `projects/1234/locations/global/catalogs/default_catalog`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportUserEventsRequest}
* @since 1.13
*/
protected CloudRetailImport(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2ImportUserEventsRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public CloudRetailImport set$Xgafv(java.lang.String $Xgafv) {
return (CloudRetailImport) super.set$Xgafv($Xgafv);
}
@Override
public CloudRetailImport setAccessToken(java.lang.String accessToken) {
return (CloudRetailImport) super.setAccessToken(accessToken);
}
@Override
public CloudRetailImport setAlt(java.lang.String alt) {
return (CloudRetailImport) super.setAlt(alt);
}
@Override
public CloudRetailImport setCallback(java.lang.String callback) {
return (CloudRetailImport) super.setCallback(callback);
}
@Override
public CloudRetailImport setFields(java.lang.String fields) {
return (CloudRetailImport) super.setFields(fields);
}
@Override
public CloudRetailImport setKey(java.lang.String key) {
return (CloudRetailImport) super.setKey(key);
}
@Override
public CloudRetailImport setOauthToken(java.lang.String oauthToken) {
return (CloudRetailImport) super.setOauthToken(oauthToken);
}
@Override
public CloudRetailImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CloudRetailImport) super.setPrettyPrint(prettyPrint);
}
@Override
public CloudRetailImport setQuotaUser(java.lang.String quotaUser) {
return (CloudRetailImport) super.setQuotaUser(quotaUser);
}
@Override
public CloudRetailImport setUploadType(java.lang.String uploadType) {
return (CloudRetailImport) super.setUploadType(uploadType);
}
@Override
public CloudRetailImport setUploadProtocol(java.lang.String uploadProtocol) {
return (CloudRetailImport) super.setUploadProtocol(uploadProtocol);
}
/** Required. `projects/1234/locations/global/catalogs/default_catalog` */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. `projects/1234/locations/global/catalogs/default_catalog`
*/
public java.lang.String getParent() {
return parent;
}
/** Required. `projects/1234/locations/global/catalogs/default_catalog` */
public CloudRetailImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public CloudRetailImport set(String parameterName, Object value) {
return (CloudRetailImport) super.set(parameterName, value);
}
}
/**
* Deletes permanently all user events specified by the filter provided. Depending on the number of
* events specified by the filter, this operation could take hours or days to complete. To test a
* filter, use the list command first.
*
* Create a request for the method "userEvents.purge".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Purge#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the catalog under which the events are created. The format is
* `projects/${projectId}/locations/global/catalogs/${catalogId}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeUserEventsRequest}
* @return the request
*/
public Purge purge(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeUserEventsRequest content) throws java.io.IOException {
Purge result = new Purge(parent, content);
initialize(result);
return result;
}
public class Purge extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/userEvents:purge";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Deletes permanently all user events specified by the filter provided. Depending on the number
* of events specified by the filter, this operation could take hours or days to complete. To test
* a filter, use the list command first.
*
* Create a request for the method "userEvents.purge".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Purge#execute()} method to invoke the remote operation. {@link
* Purge#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the catalog under which the events are created. The format is
* `projects/${projectId}/locations/global/catalogs/${catalogId}`
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeUserEventsRequest}
* @since 1.13
*/
protected Purge(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2PurgeUserEventsRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public Purge set$Xgafv(java.lang.String $Xgafv) {
return (Purge) super.set$Xgafv($Xgafv);
}
@Override
public Purge setAccessToken(java.lang.String accessToken) {
return (Purge) super.setAccessToken(accessToken);
}
@Override
public Purge setAlt(java.lang.String alt) {
return (Purge) super.setAlt(alt);
}
@Override
public Purge setCallback(java.lang.String callback) {
return (Purge) super.setCallback(callback);
}
@Override
public Purge setFields(java.lang.String fields) {
return (Purge) super.setFields(fields);
}
@Override
public Purge setKey(java.lang.String key) {
return (Purge) super.setKey(key);
}
@Override
public Purge setOauthToken(java.lang.String oauthToken) {
return (Purge) super.setOauthToken(oauthToken);
}
@Override
public Purge setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Purge) super.setPrettyPrint(prettyPrint);
}
@Override
public Purge setQuotaUser(java.lang.String quotaUser) {
return (Purge) super.setQuotaUser(quotaUser);
}
@Override
public Purge setUploadType(java.lang.String uploadType) {
return (Purge) super.setUploadType(uploadType);
}
@Override
public Purge setUploadProtocol(java.lang.String uploadProtocol) {
return (Purge) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the catalog under which the events are created. The
* format is `projects/${projectId}/locations/global/catalogs/${catalogId}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the catalog under which the events are created. The format is
`projects/${projectId}/locations/global/catalogs/${catalogId}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the catalog under which the events are created. The
* format is `projects/${projectId}/locations/global/catalogs/${catalogId}`
*/
public Purge setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Purge set(String parameterName, Object value) {
return (Purge) super.set(parameterName, value);
}
}
/**
* Starts a user-event rejoin operation with latest product catalog. Events are not annotated with
* detailed product information for products that are missing from the catalog when the user event
* is ingested. These events are stored as unjoined events with limited usage on training and
* serving. You can use this method to start a join operation on specified events with the latest
* version of product catalog. You can also use this method to correct events joined with the wrong
* product catalog. A rejoin operation can take hours or days to complete.
*
* Create a request for the method "userEvents.rejoin".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Rejoin#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RejoinUserEventsRequest}
* @return the request
*/
public Rejoin rejoin(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RejoinUserEventsRequest content) throws java.io.IOException {
Rejoin result = new Rejoin(parent, content);
initialize(result);
return result;
}
public class Rejoin extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/userEvents:rejoin";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Starts a user-event rejoin operation with latest product catalog. Events are not annotated with
* detailed product information for products that are missing from the catalog when the user event
* is ingested. These events are stored as unjoined events with limited usage on training and
* serving. You can use this method to start a join operation on specified events with the latest
* version of product catalog. You can also use this method to correct events joined with the
* wrong product catalog. A rejoin operation can take hours or days to complete.
*
* Create a request for the method "userEvents.rejoin".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Rejoin#execute()} method to invoke the remote operation. {@link
* Rejoin#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2RejoinUserEventsRequest}
* @since 1.13
*/
protected Rejoin(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2RejoinUserEventsRequest content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public Rejoin set$Xgafv(java.lang.String $Xgafv) {
return (Rejoin) super.set$Xgafv($Xgafv);
}
@Override
public Rejoin setAccessToken(java.lang.String accessToken) {
return (Rejoin) super.setAccessToken(accessToken);
}
@Override
public Rejoin setAlt(java.lang.String alt) {
return (Rejoin) super.setAlt(alt);
}
@Override
public Rejoin setCallback(java.lang.String callback) {
return (Rejoin) super.setCallback(callback);
}
@Override
public Rejoin setFields(java.lang.String fields) {
return (Rejoin) super.setFields(fields);
}
@Override
public Rejoin setKey(java.lang.String key) {
return (Rejoin) super.setKey(key);
}
@Override
public Rejoin setOauthToken(java.lang.String oauthToken) {
return (Rejoin) super.setOauthToken(oauthToken);
}
@Override
public Rejoin setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rejoin) super.setPrettyPrint(prettyPrint);
}
@Override
public Rejoin setQuotaUser(java.lang.String quotaUser) {
return (Rejoin) super.setQuotaUser(quotaUser);
}
@Override
public Rejoin setUploadType(java.lang.String uploadType) {
return (Rejoin) super.setUploadType(uploadType);
}
@Override
public Rejoin setUploadProtocol(java.lang.String uploadProtocol) {
return (Rejoin) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent catalog resource name, such as
`projects/1234/locations/global/catalogs/default_catalog`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
public Rejoin setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Rejoin set(String parameterName, Object value) {
return (Rejoin) super.set(parameterName, value);
}
}
/**
* Writes a single user event.
*
* Create a request for the method "userEvents.write".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Write#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2UserEvent}
* @return the request
*/
public Write write(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2UserEvent content) throws java.io.IOException {
Write result = new Write(parent, content);
initialize(result);
return result;
}
public class Write extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+parent}/userEvents:write";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
/**
* Writes a single user event.
*
* Create a request for the method "userEvents.write".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Write#execute()} method to invoke the remote operation. {@link
* Write#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
* @param content the {@link com.google.api.services.retail.v2.model.GoogleCloudRetailV2UserEvent}
* @since 1.13
*/
protected Write(java.lang.String parent, com.google.api.services.retail.v2.model.GoogleCloudRetailV2UserEvent content) {
super(CloudRetail.this, "POST", REST_PATH, content, com.google.api.services.retail.v2.model.GoogleCloudRetailV2UserEvent.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
}
@Override
public Write set$Xgafv(java.lang.String $Xgafv) {
return (Write) super.set$Xgafv($Xgafv);
}
@Override
public Write setAccessToken(java.lang.String accessToken) {
return (Write) super.setAccessToken(accessToken);
}
@Override
public Write setAlt(java.lang.String alt) {
return (Write) super.setAlt(alt);
}
@Override
public Write setCallback(java.lang.String callback) {
return (Write) super.setCallback(callback);
}
@Override
public Write setFields(java.lang.String fields) {
return (Write) super.setFields(fields);
}
@Override
public Write setKey(java.lang.String key) {
return (Write) super.setKey(key);
}
@Override
public Write setOauthToken(java.lang.String oauthToken) {
return (Write) super.setOauthToken(oauthToken);
}
@Override
public Write setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Write) super.setPrettyPrint(prettyPrint);
}
@Override
public Write setQuotaUser(java.lang.String quotaUser) {
return (Write) super.setQuotaUser(quotaUser);
}
@Override
public Write setUploadType(java.lang.String uploadType) {
return (Write) super.setUploadType(uploadType);
}
@Override
public Write setUploadProtocol(java.lang.String uploadProtocol) {
return (Write) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent catalog resource name, such as
`projects/1234/locations/global/catalogs/default_catalog`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent catalog resource name, such as
* `projects/1234/locations/global/catalogs/default_catalog`.
*/
public Write setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/catalogs/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* If set to true, the user event will be written asynchronously after validation, and
* the API will respond without waiting for the write. Therefore, silent failures can
* occur even if the API returns success. In case of silent failures, error messages can
* be found in Stackdriver logs.
*/
@com.google.api.client.util.Key
private java.lang.Boolean writeAsync;
/** If set to true, the user event will be written asynchronously after validation, and the API will
respond without waiting for the write. Therefore, silent failures can occur even if the API returns
success. In case of silent failures, error messages can be found in Stackdriver logs.
*/
public java.lang.Boolean getWriteAsync() {
return writeAsync;
}
/**
* If set to true, the user event will be written asynchronously after validation, and
* the API will respond without waiting for the write. Therefore, silent failures can
* occur even if the API returns success. In case of silent failures, error messages can
* be found in Stackdriver logs.
*/
public Write setWriteAsync(java.lang.Boolean writeAsync) {
this.writeAsync = writeAsync;
return this;
}
@Override
public Write set(String parameterName, Object value) {
return (Write) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Operations.List request = retail.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleLongrunningListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudRetail retail = new CloudRetail(...);}
* {@code CloudRetail.Operations.List request = retail.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends CloudRetailRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the retail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(CloudRetail.this, "GET", REST_PATH, null, com.google.api.services.retail.v2.model.GoogleLongrunningListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link CloudRetail}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudRetail}. */
@Override
public CloudRetail build() {
return new CloudRetail(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudRetailRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudRetailRequestInitializer(
CloudRetailRequestInitializer cloudretailRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudretailRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}