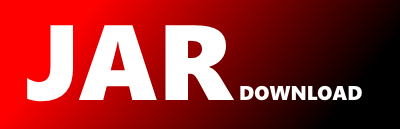
com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequestFacetSpec Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.retail.v2.model;
/**
* A facet specification to perform faceted search.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Vertex AI Search for Retail API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRetailV2SearchRequestFacetSpec extends com.google.api.client.json.GenericJson {
/**
* Enables dynamic position for this facet. If set to true, the position of this facet among all
* facets in the response is determined by Google Retail Search. It is ordered together with
* dynamic facets if dynamic facets is enabled. If set to false, the position of this facet in the
* response is the same as in the request, and it is ranked before the facets with dynamic
* position enable and all dynamic facets. For example, you may always want to have rating facet
* returned in the response, but it's not necessarily to always display the rating facet at the
* top. In that case, you can set enable_dynamic_position to true so that the position of rating
* facet in response is determined by Google Retail Search. Another example, assuming you have the
* following facets in the request: * "rating", enable_dynamic_position = true * "price",
* enable_dynamic_position = false * "brands", enable_dynamic_position = false And also you have a
* dynamic facets enable, which generates a facet "gender". Then, the final order of the facets in
* the response can be ("price", "brands", "rating", "gender") or ("price", "brands", "gender",
* "rating") depends on how Google Retail Search orders "gender" and "rating" facets. However,
* notice that "price" and "brands" are always ranked at first and second position because their
* enable_dynamic_position values are false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableDynamicPosition;
/**
* List of keys to exclude when faceting. By default, FacetKey.key is not excluded from the filter
* unless it is listed in this field. Listing a facet key in this field allows its values to
* appear as facet results, even when they are filtered out of search results. Using this field
* does not affect what search results are returned. For example, suppose there are 100 products
* with the color facet "Red" and 200 products with the color facet "Blue". A query containing the
* filter "colorFamilies:ANY("Red")" and having "colorFamilies" as FacetKey.key would by default
* return only "Red" products in the search results, and also return "Red" with count 100 as the
* only color facet. Although there are also blue products available, "Blue" would not be shown as
* an available facet value. If "colorFamilies" is listed in "excludedFilterKeys", then the query
* returns the facet values "Red" with count 100 and "Blue" with count 200, because the
* "colorFamilies" key is now excluded from the filter. Because this field doesn't affect search
* results, the search results are still correctly filtered to return only "Red" products. A
* maximum of 100 values are allowed. Otherwise, an INVALID_ARGUMENT error is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List excludedFilterKeys;
/**
* Required. The facet key specification.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestFacetSpecFacetKey facetKey;
/**
* Maximum of facet values that should be returned for this facet. If unspecified, defaults to 50.
* The maximum allowed value is 300. Values above 300 will be coerced to 300. If this field is
* negative, an INVALID_ARGUMENT is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer limit;
/**
* Enables dynamic position for this facet. If set to true, the position of this facet among all
* facets in the response is determined by Google Retail Search. It is ordered together with
* dynamic facets if dynamic facets is enabled. If set to false, the position of this facet in the
* response is the same as in the request, and it is ranked before the facets with dynamic
* position enable and all dynamic facets. For example, you may always want to have rating facet
* returned in the response, but it's not necessarily to always display the rating facet at the
* top. In that case, you can set enable_dynamic_position to true so that the position of rating
* facet in response is determined by Google Retail Search. Another example, assuming you have the
* following facets in the request: * "rating", enable_dynamic_position = true * "price",
* enable_dynamic_position = false * "brands", enable_dynamic_position = false And also you have a
* dynamic facets enable, which generates a facet "gender". Then, the final order of the facets in
* the response can be ("price", "brands", "rating", "gender") or ("price", "brands", "gender",
* "rating") depends on how Google Retail Search orders "gender" and "rating" facets. However,
* notice that "price" and "brands" are always ranked at first and second position because their
* enable_dynamic_position values are false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableDynamicPosition() {
return enableDynamicPosition;
}
/**
* Enables dynamic position for this facet. If set to true, the position of this facet among all
* facets in the response is determined by Google Retail Search. It is ordered together with
* dynamic facets if dynamic facets is enabled. If set to false, the position of this facet in the
* response is the same as in the request, and it is ranked before the facets with dynamic
* position enable and all dynamic facets. For example, you may always want to have rating facet
* returned in the response, but it's not necessarily to always display the rating facet at the
* top. In that case, you can set enable_dynamic_position to true so that the position of rating
* facet in response is determined by Google Retail Search. Another example, assuming you have the
* following facets in the request: * "rating", enable_dynamic_position = true * "price",
* enable_dynamic_position = false * "brands", enable_dynamic_position = false And also you have a
* dynamic facets enable, which generates a facet "gender". Then, the final order of the facets in
* the response can be ("price", "brands", "rating", "gender") or ("price", "brands", "gender",
* "rating") depends on how Google Retail Search orders "gender" and "rating" facets. However,
* notice that "price" and "brands" are always ranked at first and second position because their
* enable_dynamic_position values are false.
* @param enableDynamicPosition enableDynamicPosition or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpec setEnableDynamicPosition(java.lang.Boolean enableDynamicPosition) {
this.enableDynamicPosition = enableDynamicPosition;
return this;
}
/**
* List of keys to exclude when faceting. By default, FacetKey.key is not excluded from the filter
* unless it is listed in this field. Listing a facet key in this field allows its values to
* appear as facet results, even when they are filtered out of search results. Using this field
* does not affect what search results are returned. For example, suppose there are 100 products
* with the color facet "Red" and 200 products with the color facet "Blue". A query containing the
* filter "colorFamilies:ANY("Red")" and having "colorFamilies" as FacetKey.key would by default
* return only "Red" products in the search results, and also return "Red" with count 100 as the
* only color facet. Although there are also blue products available, "Blue" would not be shown as
* an available facet value. If "colorFamilies" is listed in "excludedFilterKeys", then the query
* returns the facet values "Red" with count 100 and "Blue" with count 200, because the
* "colorFamilies" key is now excluded from the filter. Because this field doesn't affect search
* results, the search results are still correctly filtered to return only "Red" products. A
* maximum of 100 values are allowed. Otherwise, an INVALID_ARGUMENT error is returned.
* @return value or {@code null} for none
*/
public java.util.List getExcludedFilterKeys() {
return excludedFilterKeys;
}
/**
* List of keys to exclude when faceting. By default, FacetKey.key is not excluded from the filter
* unless it is listed in this field. Listing a facet key in this field allows its values to
* appear as facet results, even when they are filtered out of search results. Using this field
* does not affect what search results are returned. For example, suppose there are 100 products
* with the color facet "Red" and 200 products with the color facet "Blue". A query containing the
* filter "colorFamilies:ANY("Red")" and having "colorFamilies" as FacetKey.key would by default
* return only "Red" products in the search results, and also return "Red" with count 100 as the
* only color facet. Although there are also blue products available, "Blue" would not be shown as
* an available facet value. If "colorFamilies" is listed in "excludedFilterKeys", then the query
* returns the facet values "Red" with count 100 and "Blue" with count 200, because the
* "colorFamilies" key is now excluded from the filter. Because this field doesn't affect search
* results, the search results are still correctly filtered to return only "Red" products. A
* maximum of 100 values are allowed. Otherwise, an INVALID_ARGUMENT error is returned.
* @param excludedFilterKeys excludedFilterKeys or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpec setExcludedFilterKeys(java.util.List excludedFilterKeys) {
this.excludedFilterKeys = excludedFilterKeys;
return this;
}
/**
* Required. The facet key specification.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey getFacetKey() {
return facetKey;
}
/**
* Required. The facet key specification.
* @param facetKey facetKey or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpec setFacetKey(GoogleCloudRetailV2SearchRequestFacetSpecFacetKey facetKey) {
this.facetKey = facetKey;
return this;
}
/**
* Maximum of facet values that should be returned for this facet. If unspecified, defaults to 50.
* The maximum allowed value is 300. Values above 300 will be coerced to 300. If this field is
* negative, an INVALID_ARGUMENT is returned.
* @return value or {@code null} for none
*/
public java.lang.Integer getLimit() {
return limit;
}
/**
* Maximum of facet values that should be returned for this facet. If unspecified, defaults to 50.
* The maximum allowed value is 300. Values above 300 will be coerced to 300. If this field is
* negative, an INVALID_ARGUMENT is returned.
* @param limit limit or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpec setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
@Override
public GoogleCloudRetailV2SearchRequestFacetSpec set(String fieldName, Object value) {
return (GoogleCloudRetailV2SearchRequestFacetSpec) super.set(fieldName, value);
}
@Override
public GoogleCloudRetailV2SearchRequestFacetSpec clone() {
return (GoogleCloudRetailV2SearchRequestFacetSpec) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy