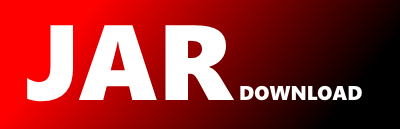
com.google.api.services.retail.v2.model.GoogleCloudRetailV2alphaMerchantCenterAccountLink Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.retail.v2.model;
/**
* Represents a link between a Merchant Center account and a branch. After a link is established,
* products from the linked Merchant Center account are streamed to the linked branch.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Vertex AI Search for Retail API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRetailV2alphaMerchantCenterAccountLink extends com.google.api.client.json.GenericJson {
/**
* Required. The branch ID (e.g. 0/1/2) within the catalog that products from
* merchant_center_account_id are streamed to. When updating this field, an empty value will use
* the currently configured default branch. However, changing the default branch later on won't
* change the linked branch here. A single branch ID can only have one linked Merchant Center
* account ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String branchId;
/**
* Criteria for the Merchant Center feeds to be ingested via the link. All offers will be ingested
* if the list is empty. Otherwise the offers will be ingested from selected feeds.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List feedFilters;
/**
* The FeedLabel used to perform filtering. Note: this replaces
* [region_id](https://developers.google.com/shopping-
* content/reference/rest/v2.1/products#Product.FIELDS.feed_label). Example value: `US`. Example
* value: `FeedLabel1`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String feedLabel;
/**
* Output only. Immutable. MerchantCenterAccountLink identifier, which is the final component of
* name. This field is auto generated and follows the convention:
* `BranchId_MerchantCenterAccountId`.
* `projects/locations/global/catalogs/default_catalog/merchantCenterAccountLinks/id_1`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* Language of the title/description and other string attributes. Use language tags defined by
* [BCP 47](https://www.rfc-editor.org/rfc/bcp/bcp47.txt). ISO 639-1. This specifies the language
* of offers in Merchant Center that will be accepted. If empty, no language filtering will be
* performed. Example value: `en`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/**
* Required. The linked [Merchant center account id](https://developers.google.com/shopping-
* content/guides/accountstatuses). The account must be a standalone account or a sub-account of a
* MCA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long merchantCenterAccountId;
/**
* Output only. Immutable. Full resource name of the Merchant Center Account Link, such as `projec
* ts/locations/global/catalogs/default_catalog/merchantCenterAccountLinks/merchant_center_account
* _link`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. Google Cloud project ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/**
* Optional. An optional arbitrary string that could be used as a tag for tracking link source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String source;
/**
* Output only. Represents the state of the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Required. The branch ID (e.g. 0/1/2) within the catalog that products from
* merchant_center_account_id are streamed to. When updating this field, an empty value will use
* the currently configured default branch. However, changing the default branch later on won't
* change the linked branch here. A single branch ID can only have one linked Merchant Center
* account ID.
* @return value or {@code null} for none
*/
public java.lang.String getBranchId() {
return branchId;
}
/**
* Required. The branch ID (e.g. 0/1/2) within the catalog that products from
* merchant_center_account_id are streamed to. When updating this field, an empty value will use
* the currently configured default branch. However, changing the default branch later on won't
* change the linked branch here. A single branch ID can only have one linked Merchant Center
* account ID.
* @param branchId branchId or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setBranchId(java.lang.String branchId) {
this.branchId = branchId;
return this;
}
/**
* Criteria for the Merchant Center feeds to be ingested via the link. All offers will be ingested
* if the list is empty. Otherwise the offers will be ingested from selected feeds.
* @return value or {@code null} for none
*/
public java.util.List getFeedFilters() {
return feedFilters;
}
/**
* Criteria for the Merchant Center feeds to be ingested via the link. All offers will be ingested
* if the list is empty. Otherwise the offers will be ingested from selected feeds.
* @param feedFilters feedFilters or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setFeedFilters(java.util.List feedFilters) {
this.feedFilters = feedFilters;
return this;
}
/**
* The FeedLabel used to perform filtering. Note: this replaces
* [region_id](https://developers.google.com/shopping-
* content/reference/rest/v2.1/products#Product.FIELDS.feed_label). Example value: `US`. Example
* value: `FeedLabel1`.
* @return value or {@code null} for none
*/
public java.lang.String getFeedLabel() {
return feedLabel;
}
/**
* The FeedLabel used to perform filtering. Note: this replaces
* [region_id](https://developers.google.com/shopping-
* content/reference/rest/v2.1/products#Product.FIELDS.feed_label). Example value: `US`. Example
* value: `FeedLabel1`.
* @param feedLabel feedLabel or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setFeedLabel(java.lang.String feedLabel) {
this.feedLabel = feedLabel;
return this;
}
/**
* Output only. Immutable. MerchantCenterAccountLink identifier, which is the final component of
* name. This field is auto generated and follows the convention:
* `BranchId_MerchantCenterAccountId`.
* `projects/locations/global/catalogs/default_catalog/merchantCenterAccountLinks/id_1`.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* Output only. Immutable. MerchantCenterAccountLink identifier, which is the final component of
* name. This field is auto generated and follows the convention:
* `BranchId_MerchantCenterAccountId`.
* `projects/locations/global/catalogs/default_catalog/merchantCenterAccountLinks/id_1`.
* @param id id or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Language of the title/description and other string attributes. Use language tags defined by
* [BCP 47](https://www.rfc-editor.org/rfc/bcp/bcp47.txt). ISO 639-1. This specifies the language
* of offers in Merchant Center that will be accepted. If empty, no language filtering will be
* performed. Example value: `en`.
* @return value or {@code null} for none
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Language of the title/description and other string attributes. Use language tags defined by
* [BCP 47](https://www.rfc-editor.org/rfc/bcp/bcp47.txt). ISO 639-1. This specifies the language
* of offers in Merchant Center that will be accepted. If empty, no language filtering will be
* performed. Example value: `en`.
* @param languageCode languageCode or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Required. The linked [Merchant center account id](https://developers.google.com/shopping-
* content/guides/accountstatuses). The account must be a standalone account or a sub-account of a
* MCA.
* @return value or {@code null} for none
*/
public java.lang.Long getMerchantCenterAccountId() {
return merchantCenterAccountId;
}
/**
* Required. The linked [Merchant center account id](https://developers.google.com/shopping-
* content/guides/accountstatuses). The account must be a standalone account or a sub-account of a
* MCA.
* @param merchantCenterAccountId merchantCenterAccountId or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setMerchantCenterAccountId(java.lang.Long merchantCenterAccountId) {
this.merchantCenterAccountId = merchantCenterAccountId;
return this;
}
/**
* Output only. Immutable. Full resource name of the Merchant Center Account Link, such as `projec
* ts/locations/global/catalogs/default_catalog/merchantCenterAccountLinks/merchant_center_account
* _link`.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Immutable. Full resource name of the Merchant Center Account Link, such as `projec
* ts/locations/global/catalogs/default_catalog/merchantCenterAccountLinks/merchant_center_account
* _link`.
* @param name name or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. Google Cloud project ID.
* @return value or {@code null} for none
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* Output only. Google Cloud project ID.
* @param projectId projectId or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Optional. An optional arbitrary string that could be used as a tag for tracking link source.
* @return value or {@code null} for none
*/
public java.lang.String getSource() {
return source;
}
/**
* Optional. An optional arbitrary string that could be used as a tag for tracking link source.
* @param source source or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setSource(java.lang.String source) {
this.source = source;
return this;
}
/**
* Output only. Represents the state of the link.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. Represents the state of the link.
* @param state state or {@code null} for none
*/
public GoogleCloudRetailV2alphaMerchantCenterAccountLink setState(java.lang.String state) {
this.state = state;
return this;
}
@Override
public GoogleCloudRetailV2alphaMerchantCenterAccountLink set(String fieldName, Object value) {
return (GoogleCloudRetailV2alphaMerchantCenterAccountLink) super.set(fieldName, value);
}
@Override
public GoogleCloudRetailV2alphaMerchantCenterAccountLink clone() {
return (GoogleCloudRetailV2alphaMerchantCenterAccountLink) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy