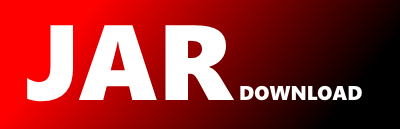
com.google.api.services.retail.v2.model.GoogleCloudRetailV2Rule Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.retail.v2.model;
/**
* A rule is a condition-action pair * A condition defines when a rule is to be triggered. * An
* action specifies what occurs on that trigger. Currently rules only work for controls with
* SOLUTION_TYPE_SEARCH.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Vertex AI Search for Retail API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRetailV2Rule extends com.google.api.client.json.GenericJson {
/**
* A boost action.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleBoostAction boostAction;
/**
* Required. The condition that triggers the rule. If the condition is empty, the rule will always
* apply.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2Condition condition;
/**
* Prevents term from being associated with other terms.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleDoNotAssociateAction doNotAssociateAction;
/**
* Filters results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleFilterAction filterAction;
/**
* Force returns an attribute as a facet in the request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleForceReturnFacetAction forceReturnFacetAction;
/**
* Ignores specific terms from query during search.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleIgnoreAction ignoreAction;
/**
* Treats specific term as a synonym with a group of terms. Group of terms will not be treated as
* synonyms with the specific term.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleOnewaySynonymsAction onewaySynonymsAction;
/**
* Redirects a shopper to a specific page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleRedirectAction redirectAction;
/**
* Remove an attribute as a facet in the request (if present).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleRemoveFacetAction removeFacetAction;
/**
* Replaces specific terms in the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleReplacementAction replacementAction;
/**
* Treats a set of terms as synonyms of one another.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2RuleTwowaySynonymsAction twowaySynonymsAction;
/**
* A boost action.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleBoostAction getBoostAction() {
return boostAction;
}
/**
* A boost action.
* @param boostAction boostAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setBoostAction(GoogleCloudRetailV2RuleBoostAction boostAction) {
this.boostAction = boostAction;
return this;
}
/**
* Required. The condition that triggers the rule. If the condition is empty, the rule will always
* apply.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2Condition getCondition() {
return condition;
}
/**
* Required. The condition that triggers the rule. If the condition is empty, the rule will always
* apply.
* @param condition condition or {@code null} for none
*/
public GoogleCloudRetailV2Rule setCondition(GoogleCloudRetailV2Condition condition) {
this.condition = condition;
return this;
}
/**
* Prevents term from being associated with other terms.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleDoNotAssociateAction getDoNotAssociateAction() {
return doNotAssociateAction;
}
/**
* Prevents term from being associated with other terms.
* @param doNotAssociateAction doNotAssociateAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setDoNotAssociateAction(GoogleCloudRetailV2RuleDoNotAssociateAction doNotAssociateAction) {
this.doNotAssociateAction = doNotAssociateAction;
return this;
}
/**
* Filters results.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleFilterAction getFilterAction() {
return filterAction;
}
/**
* Filters results.
* @param filterAction filterAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setFilterAction(GoogleCloudRetailV2RuleFilterAction filterAction) {
this.filterAction = filterAction;
return this;
}
/**
* Force returns an attribute as a facet in the request.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleForceReturnFacetAction getForceReturnFacetAction() {
return forceReturnFacetAction;
}
/**
* Force returns an attribute as a facet in the request.
* @param forceReturnFacetAction forceReturnFacetAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setForceReturnFacetAction(GoogleCloudRetailV2RuleForceReturnFacetAction forceReturnFacetAction) {
this.forceReturnFacetAction = forceReturnFacetAction;
return this;
}
/**
* Ignores specific terms from query during search.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleIgnoreAction getIgnoreAction() {
return ignoreAction;
}
/**
* Ignores specific terms from query during search.
* @param ignoreAction ignoreAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setIgnoreAction(GoogleCloudRetailV2RuleIgnoreAction ignoreAction) {
this.ignoreAction = ignoreAction;
return this;
}
/**
* Treats specific term as a synonym with a group of terms. Group of terms will not be treated as
* synonyms with the specific term.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleOnewaySynonymsAction getOnewaySynonymsAction() {
return onewaySynonymsAction;
}
/**
* Treats specific term as a synonym with a group of terms. Group of terms will not be treated as
* synonyms with the specific term.
* @param onewaySynonymsAction onewaySynonymsAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setOnewaySynonymsAction(GoogleCloudRetailV2RuleOnewaySynonymsAction onewaySynonymsAction) {
this.onewaySynonymsAction = onewaySynonymsAction;
return this;
}
/**
* Redirects a shopper to a specific page.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleRedirectAction getRedirectAction() {
return redirectAction;
}
/**
* Redirects a shopper to a specific page.
* @param redirectAction redirectAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setRedirectAction(GoogleCloudRetailV2RuleRedirectAction redirectAction) {
this.redirectAction = redirectAction;
return this;
}
/**
* Remove an attribute as a facet in the request (if present).
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleRemoveFacetAction getRemoveFacetAction() {
return removeFacetAction;
}
/**
* Remove an attribute as a facet in the request (if present).
* @param removeFacetAction removeFacetAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setRemoveFacetAction(GoogleCloudRetailV2RuleRemoveFacetAction removeFacetAction) {
this.removeFacetAction = removeFacetAction;
return this;
}
/**
* Replaces specific terms in the query.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleReplacementAction getReplacementAction() {
return replacementAction;
}
/**
* Replaces specific terms in the query.
* @param replacementAction replacementAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setReplacementAction(GoogleCloudRetailV2RuleReplacementAction replacementAction) {
this.replacementAction = replacementAction;
return this;
}
/**
* Treats a set of terms as synonyms of one another.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2RuleTwowaySynonymsAction getTwowaySynonymsAction() {
return twowaySynonymsAction;
}
/**
* Treats a set of terms as synonyms of one another.
* @param twowaySynonymsAction twowaySynonymsAction or {@code null} for none
*/
public GoogleCloudRetailV2Rule setTwowaySynonymsAction(GoogleCloudRetailV2RuleTwowaySynonymsAction twowaySynonymsAction) {
this.twowaySynonymsAction = twowaySynonymsAction;
return this;
}
@Override
public GoogleCloudRetailV2Rule set(String fieldName, Object value) {
return (GoogleCloudRetailV2Rule) super.set(fieldName, value);
}
@Override
public GoogleCloudRetailV2Rule clone() {
return (GoogleCloudRetailV2Rule) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy