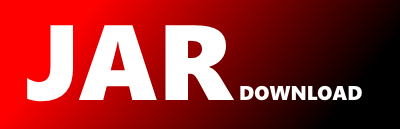
com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.retail.v2.model;
/**
* Request message for SearchService.Search method.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Vertex AI Search for Retail API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRetailV2SearchRequest extends com.google.api.client.json.GenericJson {
/**
* Boost specification to boost certain products. For more information, see [Boost
* results](https://cloud.google.com/retail/docs/boosting). Notice that if both
* ServingConfig.boost_control_ids and SearchRequest.boost_spec are set, the boost conditions from
* both places are evaluated. If a search request matches multiple boost conditions, the final
* boost score is equal to the sum of the boost scores from all matched boost conditions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestBoostSpec boostSpec;
/**
* The branch resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/0`. Use "default_branch" as the
* branch ID or leave this field empty, to search products under the default branch.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String branch;
/**
* The default filter that is applied when a user performs a search without checking any filters
* on the search page. The filter applied to every search request when quality improvement such as
* query expansion is needed. In the case a query does not have a sufficient amount of results
* this filter will be used to determine whether or not to enable the query expansion flow. The
* original filter will still be used for the query expanded search. This field is strongly
* recommended to achieve high search quality. For more information about filter syntax, see
* SearchRequest.filter.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String canonicalFilter;
/**
* Optional. This field specifies all conversational related parameters addition to traditional
* retail search.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestConversationalSearchSpec conversationalSearchSpec;
/**
* Deprecated. Refer to https://cloud.google.com/retail/docs/configs#dynamic to enable dynamic
* facets. Do not set this field. The specification for dynamically generated facets. Notice that
* only textual facets can be dynamically generated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestDynamicFacetSpec dynamicFacetSpec;
/**
* The entity for customers that may run multiple different entities, domains, sites or regions,
* for example, `Google US`, `Google Ads`, `Waymo`, `google.com`, `youtube.com`, etc. If this is
* set, it should be exactly matched with UserEvent.entity to get search results boosted by
* entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String entity;
/**
* Facet specifications for faceted search. If empty, no facets are returned. A maximum of 200
* values are allowed. Otherwise, an INVALID_ARGUMENT error is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List facetSpecs;
/**
* The filter syntax consists of an expression language for constructing a predicate from one or
* more fields of the products being filtered. Filter expression is case-sensitive. For more
* information, see [Filter](https://cloud.google.com/retail/docs/filter-and-order#filter). If
* this field is unrecognizable, an INVALID_ARGUMENT is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**
* The labels applied to a resource must meet the following requirements: * Each resource can have
* multiple labels, up to a maximum of 64. * Each label must be a key-value pair. * Keys have a
* minimum length of 1 character and a maximum length of 63 characters and cannot be empty. Values
* can be empty and have a maximum length of 63 characters. * Keys and values can contain only
* lowercase letters, numeric characters, underscores, and dashes. All characters must use UTF-8
* encoding, and international characters are allowed. * The key portion of a label must be
* unique. However, you can use the same key with multiple resources. * Keys must start with a
* lowercase letter or international character. For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* A 0-indexed integer that specifies the current offset (that is, starting result location,
* amongst the Products deemed by the API as relevant) in search results. This field is only
* considered if page_token is unset. If this field is negative, an INVALID_ARGUMENT is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer offset;
/**
* The order in which products are returned. Products can be ordered by a field in an Product
* object. Leave it unset if ordered by relevance. OrderBy expression is case-sensitive. For more
* information, see [Order](https://cloud.google.com/retail/docs/filter-and-order#order). If this
* field is unrecognizable, an INVALID_ARGUMENT is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/**
* The categories associated with a category page. Must be set for category navigation queries to
* achieve good search quality. The format should be the same as UserEvent.page_categories; To
* represent full path of category, use '>' sign to separate different hierarchies. If '>' is part
* of the category name, replace it with other character(s). Category pages include special pages
* such as sales or promotions. For instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List pageCategories;
/**
* Maximum number of Products to return. If unspecified, defaults to a reasonable value. The
* maximum allowed value is 120. Values above 120 will be coerced to 120. If this field is
* negative, an INVALID_ARGUMENT is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/**
* A page token SearchResponse.next_page_token, received from a previous SearchService.Search
* call. Provide this to retrieve the subsequent page. When paginating, all other parameters
* provided to SearchService.Search must match the call that provided the page token. Otherwise,
* an INVALID_ARGUMENT error is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/**
* The specification for personalization. Notice that if both ServingConfig.personalization_spec
* and SearchRequest.personalization_spec are set. SearchRequest.personalization_spec will
* override ServingConfig.personalization_spec.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestPersonalizationSpec personalizationSpec;
/**
* Raw search query. If this field is empty, the request is considered a category browsing request
* and returned results are based on filter and page_categories.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String query;
/**
* The query expansion specification that specifies the conditions under which query expansion
* occurs. For more information, see [Query
* expansion](https://cloud.google.com/retail/docs/result-size#query_expansion).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestQueryExpansionSpec queryExpansionSpec;
/**
* The search mode of the search request. If not specified, a single search request triggers both
* product search and faceted search.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String searchMode;
/**
* The spell correction specification that specifies the mode under which spell correction will
* take effect.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestSpellCorrectionSpec spellCorrectionSpec;
/**
* Optional. This field specifies tile navigation related parameters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2SearchRequestTileNavigationSpec tileNavigationSpec;
/**
* User information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRetailV2UserInfo userInfo;
/**
* The keys to fetch and rollup the matching variant Products attributes, FulfillmentInfo or
* LocalInventorys attributes. The attributes from all the matching variant Products or
* LocalInventorys are merged and de-duplicated. Notice that rollup attributes will lead to extra
* query latency. Maximum number of keys is 30. For FulfillmentInfo, a fulfillment type and a
* fulfillment ID must be provided in the format of "fulfillmentType.fulfillmentId". E.g., in
* "pickupInStore.store123", "pickupInStore" is fulfillment type and "store123" is the store ID.
* Supported keys are: * colorFamilies * price * originalPrice * discount * variantId *
* inventory(place_id,price) * inventory(place_id,original_price) *
* inventory(place_id,attributes.key), where key is any key in the
* Product.local_inventories.attributes map. * attributes.key, where key is any key in the
* Product.attributes map. * pickupInStore.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "pickup-in-store". * shipToStore.id, where id is any
* FulfillmentInfo.place_ids for FulfillmentInfo.type "ship-to-store". * sameDayDelivery.id, where
* id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "same-day-delivery". *
* nextDayDelivery.id, where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "next-
* day-delivery". * customFulfillment1.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "custom-type-1". * customFulfillment2.id, where id is any
* FulfillmentInfo.place_ids for FulfillmentInfo.type "custom-type-2". * customFulfillment3.id,
* where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "custom-type-3". *
* customFulfillment4.id, where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type
* "custom-type-4". * customFulfillment5.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "custom-type-5". If this field is set to an invalid value other than
* these, an INVALID_ARGUMENT error is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List variantRollupKeys;
/**
* Required. A unique identifier for tracking visitors. For example, this could be implemented
* with an HTTP cookie, which should be able to uniquely identify a visitor on a single device.
* This unique identifier should not change if the visitor logs in or out of the website. This
* should be the same identifier as UserEvent.visitor_id. The field must be a UTF-8 encoded string
* with a length limit of 128 characters. Otherwise, an INVALID_ARGUMENT error is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String visitorId;
/**
* Boost specification to boost certain products. For more information, see [Boost
* results](https://cloud.google.com/retail/docs/boosting). Notice that if both
* ServingConfig.boost_control_ids and SearchRequest.boost_spec are set, the boost conditions from
* both places are evaluated. If a search request matches multiple boost conditions, the final
* boost score is equal to the sum of the boost scores from all matched boost conditions.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestBoostSpec getBoostSpec() {
return boostSpec;
}
/**
* Boost specification to boost certain products. For more information, see [Boost
* results](https://cloud.google.com/retail/docs/boosting). Notice that if both
* ServingConfig.boost_control_ids and SearchRequest.boost_spec are set, the boost conditions from
* both places are evaluated. If a search request matches multiple boost conditions, the final
* boost score is equal to the sum of the boost scores from all matched boost conditions.
* @param boostSpec boostSpec or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setBoostSpec(GoogleCloudRetailV2SearchRequestBoostSpec boostSpec) {
this.boostSpec = boostSpec;
return this;
}
/**
* The branch resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/0`. Use "default_branch" as the
* branch ID or leave this field empty, to search products under the default branch.
* @return value or {@code null} for none
*/
public java.lang.String getBranch() {
return branch;
}
/**
* The branch resource name, such as
* `projects/locations/global/catalogs/default_catalog/branches/0`. Use "default_branch" as the
* branch ID or leave this field empty, to search products under the default branch.
* @param branch branch or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setBranch(java.lang.String branch) {
this.branch = branch;
return this;
}
/**
* The default filter that is applied when a user performs a search without checking any filters
* on the search page. The filter applied to every search request when quality improvement such as
* query expansion is needed. In the case a query does not have a sufficient amount of results
* this filter will be used to determine whether or not to enable the query expansion flow. The
* original filter will still be used for the query expanded search. This field is strongly
* recommended to achieve high search quality. For more information about filter syntax, see
* SearchRequest.filter.
* @return value or {@code null} for none
*/
public java.lang.String getCanonicalFilter() {
return canonicalFilter;
}
/**
* The default filter that is applied when a user performs a search without checking any filters
* on the search page. The filter applied to every search request when quality improvement such as
* query expansion is needed. In the case a query does not have a sufficient amount of results
* this filter will be used to determine whether or not to enable the query expansion flow. The
* original filter will still be used for the query expanded search. This field is strongly
* recommended to achieve high search quality. For more information about filter syntax, see
* SearchRequest.filter.
* @param canonicalFilter canonicalFilter or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setCanonicalFilter(java.lang.String canonicalFilter) {
this.canonicalFilter = canonicalFilter;
return this;
}
/**
* Optional. This field specifies all conversational related parameters addition to traditional
* retail search.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestConversationalSearchSpec getConversationalSearchSpec() {
return conversationalSearchSpec;
}
/**
* Optional. This field specifies all conversational related parameters addition to traditional
* retail search.
* @param conversationalSearchSpec conversationalSearchSpec or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setConversationalSearchSpec(GoogleCloudRetailV2SearchRequestConversationalSearchSpec conversationalSearchSpec) {
this.conversationalSearchSpec = conversationalSearchSpec;
return this;
}
/**
* Deprecated. Refer to https://cloud.google.com/retail/docs/configs#dynamic to enable dynamic
* facets. Do not set this field. The specification for dynamically generated facets. Notice that
* only textual facets can be dynamically generated.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestDynamicFacetSpec getDynamicFacetSpec() {
return dynamicFacetSpec;
}
/**
* Deprecated. Refer to https://cloud.google.com/retail/docs/configs#dynamic to enable dynamic
* facets. Do not set this field. The specification for dynamically generated facets. Notice that
* only textual facets can be dynamically generated.
* @param dynamicFacetSpec dynamicFacetSpec or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setDynamicFacetSpec(GoogleCloudRetailV2SearchRequestDynamicFacetSpec dynamicFacetSpec) {
this.dynamicFacetSpec = dynamicFacetSpec;
return this;
}
/**
* The entity for customers that may run multiple different entities, domains, sites or regions,
* for example, `Google US`, `Google Ads`, `Waymo`, `google.com`, `youtube.com`, etc. If this is
* set, it should be exactly matched with UserEvent.entity to get search results boosted by
* entity.
* @return value or {@code null} for none
*/
public java.lang.String getEntity() {
return entity;
}
/**
* The entity for customers that may run multiple different entities, domains, sites or regions,
* for example, `Google US`, `Google Ads`, `Waymo`, `google.com`, `youtube.com`, etc. If this is
* set, it should be exactly matched with UserEvent.entity to get search results boosted by
* entity.
* @param entity entity or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setEntity(java.lang.String entity) {
this.entity = entity;
return this;
}
/**
* Facet specifications for faceted search. If empty, no facets are returned. A maximum of 200
* values are allowed. Otherwise, an INVALID_ARGUMENT error is returned.
* @return value or {@code null} for none
*/
public java.util.List getFacetSpecs() {
return facetSpecs;
}
/**
* Facet specifications for faceted search. If empty, no facets are returned. A maximum of 200
* values are allowed. Otherwise, an INVALID_ARGUMENT error is returned.
* @param facetSpecs facetSpecs or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setFacetSpecs(java.util.List facetSpecs) {
this.facetSpecs = facetSpecs;
return this;
}
/**
* The filter syntax consists of an expression language for constructing a predicate from one or
* more fields of the products being filtered. Filter expression is case-sensitive. For more
* information, see [Filter](https://cloud.google.com/retail/docs/filter-and-order#filter). If
* this field is unrecognizable, an INVALID_ARGUMENT is returned.
* @return value or {@code null} for none
*/
public java.lang.String getFilter() {
return filter;
}
/**
* The filter syntax consists of an expression language for constructing a predicate from one or
* more fields of the products being filtered. Filter expression is case-sensitive. For more
* information, see [Filter](https://cloud.google.com/retail/docs/filter-and-order#filter). If
* this field is unrecognizable, an INVALID_ARGUMENT is returned.
* @param filter filter or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The labels applied to a resource must meet the following requirements: * Each resource can have
* multiple labels, up to a maximum of 64. * Each label must be a key-value pair. * Keys have a
* minimum length of 1 character and a maximum length of 63 characters and cannot be empty. Values
* can be empty and have a maximum length of 63 characters. * Keys and values can contain only
* lowercase letters, numeric characters, underscores, and dashes. All characters must use UTF-8
* encoding, and international characters are allowed. * The key portion of a label must be
* unique. However, you can use the same key with multiple resources. * Keys must start with a
* lowercase letter or international character. For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* The labels applied to a resource must meet the following requirements: * Each resource can have
* multiple labels, up to a maximum of 64. * Each label must be a key-value pair. * Keys have a
* minimum length of 1 character and a maximum length of 63 characters and cannot be empty. Values
* can be empty and have a maximum length of 63 characters. * Keys and values can contain only
* lowercase letters, numeric characters, underscores, and dashes. All characters must use UTF-8
* encoding, and international characters are allowed. * The key portion of a label must be
* unique. However, you can use the same key with multiple resources. * Keys must start with a
* lowercase letter or international character. For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
* @param labels labels or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* A 0-indexed integer that specifies the current offset (that is, starting result location,
* amongst the Products deemed by the API as relevant) in search results. This field is only
* considered if page_token is unset. If this field is negative, an INVALID_ARGUMENT is returned.
* @return value or {@code null} for none
*/
public java.lang.Integer getOffset() {
return offset;
}
/**
* A 0-indexed integer that specifies the current offset (that is, starting result location,
* amongst the Products deemed by the API as relevant) in search results. This field is only
* considered if page_token is unset. If this field is negative, an INVALID_ARGUMENT is returned.
* @param offset offset or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setOffset(java.lang.Integer offset) {
this.offset = offset;
return this;
}
/**
* The order in which products are returned. Products can be ordered by a field in an Product
* object. Leave it unset if ordered by relevance. OrderBy expression is case-sensitive. For more
* information, see [Order](https://cloud.google.com/retail/docs/filter-and-order#order). If this
* field is unrecognizable, an INVALID_ARGUMENT is returned.
* @return value or {@code null} for none
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* The order in which products are returned. Products can be ordered by a field in an Product
* object. Leave it unset if ordered by relevance. OrderBy expression is case-sensitive. For more
* information, see [Order](https://cloud.google.com/retail/docs/filter-and-order#order). If this
* field is unrecognizable, an INVALID_ARGUMENT is returned.
* @param orderBy orderBy or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The categories associated with a category page. Must be set for category navigation queries to
* achieve good search quality. The format should be the same as UserEvent.page_categories; To
* represent full path of category, use '>' sign to separate different hierarchies. If '>' is part
* of the category name, replace it with other character(s). Category pages include special pages
* such as sales or promotions. For instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
* @return value or {@code null} for none
*/
public java.util.List getPageCategories() {
return pageCategories;
}
/**
* The categories associated with a category page. Must be set for category navigation queries to
* achieve good search quality. The format should be the same as UserEvent.page_categories; To
* represent full path of category, use '>' sign to separate different hierarchies. If '>' is part
* of the category name, replace it with other character(s). Category pages include special pages
* such as sales or promotions. For instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
* @param pageCategories pageCategories or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setPageCategories(java.util.List pageCategories) {
this.pageCategories = pageCategories;
return this;
}
/**
* Maximum number of Products to return. If unspecified, defaults to a reasonable value. The
* maximum allowed value is 120. Values above 120 will be coerced to 120. If this field is
* negative, an INVALID_ARGUMENT is returned.
* @return value or {@code null} for none
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Maximum number of Products to return. If unspecified, defaults to a reasonable value. The
* maximum allowed value is 120. Values above 120 will be coerced to 120. If this field is
* negative, an INVALID_ARGUMENT is returned.
* @param pageSize pageSize or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token SearchResponse.next_page_token, received from a previous SearchService.Search
* call. Provide this to retrieve the subsequent page. When paginating, all other parameters
* provided to SearchService.Search must match the call that provided the page token. Otherwise,
* an INVALID_ARGUMENT error is returned.
* @return value or {@code null} for none
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token SearchResponse.next_page_token, received from a previous SearchService.Search
* call. Provide this to retrieve the subsequent page. When paginating, all other parameters
* provided to SearchService.Search must match the call that provided the page token. Otherwise,
* an INVALID_ARGUMENT error is returned.
* @param pageToken pageToken or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The specification for personalization. Notice that if both ServingConfig.personalization_spec
* and SearchRequest.personalization_spec are set. SearchRequest.personalization_spec will
* override ServingConfig.personalization_spec.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestPersonalizationSpec getPersonalizationSpec() {
return personalizationSpec;
}
/**
* The specification for personalization. Notice that if both ServingConfig.personalization_spec
* and SearchRequest.personalization_spec are set. SearchRequest.personalization_spec will
* override ServingConfig.personalization_spec.
* @param personalizationSpec personalizationSpec or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setPersonalizationSpec(GoogleCloudRetailV2SearchRequestPersonalizationSpec personalizationSpec) {
this.personalizationSpec = personalizationSpec;
return this;
}
/**
* Raw search query. If this field is empty, the request is considered a category browsing request
* and returned results are based on filter and page_categories.
* @return value or {@code null} for none
*/
public java.lang.String getQuery() {
return query;
}
/**
* Raw search query. If this field is empty, the request is considered a category browsing request
* and returned results are based on filter and page_categories.
* @param query query or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* The query expansion specification that specifies the conditions under which query expansion
* occurs. For more information, see [Query
* expansion](https://cloud.google.com/retail/docs/result-size#query_expansion).
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestQueryExpansionSpec getQueryExpansionSpec() {
return queryExpansionSpec;
}
/**
* The query expansion specification that specifies the conditions under which query expansion
* occurs. For more information, see [Query
* expansion](https://cloud.google.com/retail/docs/result-size#query_expansion).
* @param queryExpansionSpec queryExpansionSpec or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setQueryExpansionSpec(GoogleCloudRetailV2SearchRequestQueryExpansionSpec queryExpansionSpec) {
this.queryExpansionSpec = queryExpansionSpec;
return this;
}
/**
* The search mode of the search request. If not specified, a single search request triggers both
* product search and faceted search.
* @return value or {@code null} for none
*/
public java.lang.String getSearchMode() {
return searchMode;
}
/**
* The search mode of the search request. If not specified, a single search request triggers both
* product search and faceted search.
* @param searchMode searchMode or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setSearchMode(java.lang.String searchMode) {
this.searchMode = searchMode;
return this;
}
/**
* The spell correction specification that specifies the mode under which spell correction will
* take effect.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestSpellCorrectionSpec getSpellCorrectionSpec() {
return spellCorrectionSpec;
}
/**
* The spell correction specification that specifies the mode under which spell correction will
* take effect.
* @param spellCorrectionSpec spellCorrectionSpec or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setSpellCorrectionSpec(GoogleCloudRetailV2SearchRequestSpellCorrectionSpec spellCorrectionSpec) {
this.spellCorrectionSpec = spellCorrectionSpec;
return this;
}
/**
* Optional. This field specifies tile navigation related parameters.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestTileNavigationSpec getTileNavigationSpec() {
return tileNavigationSpec;
}
/**
* Optional. This field specifies tile navigation related parameters.
* @param tileNavigationSpec tileNavigationSpec or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setTileNavigationSpec(GoogleCloudRetailV2SearchRequestTileNavigationSpec tileNavigationSpec) {
this.tileNavigationSpec = tileNavigationSpec;
return this;
}
/**
* User information.
* @return value or {@code null} for none
*/
public GoogleCloudRetailV2UserInfo getUserInfo() {
return userInfo;
}
/**
* User information.
* @param userInfo userInfo or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setUserInfo(GoogleCloudRetailV2UserInfo userInfo) {
this.userInfo = userInfo;
return this;
}
/**
* The keys to fetch and rollup the matching variant Products attributes, FulfillmentInfo or
* LocalInventorys attributes. The attributes from all the matching variant Products or
* LocalInventorys are merged and de-duplicated. Notice that rollup attributes will lead to extra
* query latency. Maximum number of keys is 30. For FulfillmentInfo, a fulfillment type and a
* fulfillment ID must be provided in the format of "fulfillmentType.fulfillmentId". E.g., in
* "pickupInStore.store123", "pickupInStore" is fulfillment type and "store123" is the store ID.
* Supported keys are: * colorFamilies * price * originalPrice * discount * variantId *
* inventory(place_id,price) * inventory(place_id,original_price) *
* inventory(place_id,attributes.key), where key is any key in the
* Product.local_inventories.attributes map. * attributes.key, where key is any key in the
* Product.attributes map. * pickupInStore.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "pickup-in-store". * shipToStore.id, where id is any
* FulfillmentInfo.place_ids for FulfillmentInfo.type "ship-to-store". * sameDayDelivery.id, where
* id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "same-day-delivery". *
* nextDayDelivery.id, where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "next-
* day-delivery". * customFulfillment1.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "custom-type-1". * customFulfillment2.id, where id is any
* FulfillmentInfo.place_ids for FulfillmentInfo.type "custom-type-2". * customFulfillment3.id,
* where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "custom-type-3". *
* customFulfillment4.id, where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type
* "custom-type-4". * customFulfillment5.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "custom-type-5". If this field is set to an invalid value other than
* these, an INVALID_ARGUMENT error is returned.
* @return value or {@code null} for none
*/
public java.util.List getVariantRollupKeys() {
return variantRollupKeys;
}
/**
* The keys to fetch and rollup the matching variant Products attributes, FulfillmentInfo or
* LocalInventorys attributes. The attributes from all the matching variant Products or
* LocalInventorys are merged and de-duplicated. Notice that rollup attributes will lead to extra
* query latency. Maximum number of keys is 30. For FulfillmentInfo, a fulfillment type and a
* fulfillment ID must be provided in the format of "fulfillmentType.fulfillmentId". E.g., in
* "pickupInStore.store123", "pickupInStore" is fulfillment type and "store123" is the store ID.
* Supported keys are: * colorFamilies * price * originalPrice * discount * variantId *
* inventory(place_id,price) * inventory(place_id,original_price) *
* inventory(place_id,attributes.key), where key is any key in the
* Product.local_inventories.attributes map. * attributes.key, where key is any key in the
* Product.attributes map. * pickupInStore.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "pickup-in-store". * shipToStore.id, where id is any
* FulfillmentInfo.place_ids for FulfillmentInfo.type "ship-to-store". * sameDayDelivery.id, where
* id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "same-day-delivery". *
* nextDayDelivery.id, where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "next-
* day-delivery". * customFulfillment1.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "custom-type-1". * customFulfillment2.id, where id is any
* FulfillmentInfo.place_ids for FulfillmentInfo.type "custom-type-2". * customFulfillment3.id,
* where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type "custom-type-3". *
* customFulfillment4.id, where id is any FulfillmentInfo.place_ids for FulfillmentInfo.type
* "custom-type-4". * customFulfillment5.id, where id is any FulfillmentInfo.place_ids for
* FulfillmentInfo.type "custom-type-5". If this field is set to an invalid value other than
* these, an INVALID_ARGUMENT error is returned.
* @param variantRollupKeys variantRollupKeys or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setVariantRollupKeys(java.util.List variantRollupKeys) {
this.variantRollupKeys = variantRollupKeys;
return this;
}
/**
* Required. A unique identifier for tracking visitors. For example, this could be implemented
* with an HTTP cookie, which should be able to uniquely identify a visitor on a single device.
* This unique identifier should not change if the visitor logs in or out of the website. This
* should be the same identifier as UserEvent.visitor_id. The field must be a UTF-8 encoded string
* with a length limit of 128 characters. Otherwise, an INVALID_ARGUMENT error is returned.
* @return value or {@code null} for none
*/
public java.lang.String getVisitorId() {
return visitorId;
}
/**
* Required. A unique identifier for tracking visitors. For example, this could be implemented
* with an HTTP cookie, which should be able to uniquely identify a visitor on a single device.
* This unique identifier should not change if the visitor logs in or out of the website. This
* should be the same identifier as UserEvent.visitor_id. The field must be a UTF-8 encoded string
* with a length limit of 128 characters. Otherwise, an INVALID_ARGUMENT error is returned.
* @param visitorId visitorId or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequest setVisitorId(java.lang.String visitorId) {
this.visitorId = visitorId;
return this;
}
@Override
public GoogleCloudRetailV2SearchRequest set(String fieldName, Object value) {
return (GoogleCloudRetailV2SearchRequest) super.set(fieldName, value);
}
@Override
public GoogleCloudRetailV2SearchRequest clone() {
return (GoogleCloudRetailV2SearchRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy