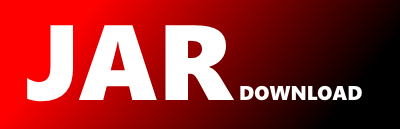
com.google.api.services.retail.v2.model.GoogleCloudRetailV2SearchRequestFacetSpecFacetKey Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.retail.v2.model;
/**
* Specifies how a facet is computed.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Vertex AI Search for Retail API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRetailV2SearchRequestFacetSpecFacetKey extends com.google.api.client.json.GenericJson {
/**
* True to make facet keys case insensitive when getting faceting values with prefixes or
* contains; false otherwise.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean caseInsensitive;
/**
* Only get facet values that contains the given strings. For example, suppose "categories" has
* three values "Women > Shoe", "Women > Dress" and "Men > Shoe". If set "contains" to "Shoe", the
* "categories" facet gives only "Women > Shoe" and "Men > Shoe". Only supported on textual
* fields. Maximum is 10.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List contains;
/**
* Set only if values should be bucketized into intervals. Must be set for facets with numerical
* values. Must not be set for facet with text values. Maximum number of intervals is 40. For all
* numerical facet keys that appear in the list of products from the catalog, the percentiles 0,
* 10, 30, 50, 70, 90, and 100 are computed from their distribution weekly. If the model assigns a
* high score to a numerical facet key and its intervals are not specified in the search request,
* these percentiles become the bounds for its intervals and are returned in the response. If the
* facet key intervals are specified in the request, then the specified intervals are returned
* instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List intervals;
static {
// hack to force ProGuard to consider GoogleCloudRetailV2Interval used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudRetailV2Interval.class);
}
/**
* Required. Supported textual and numerical facet keys in Product object, over which the facet
* values are computed. Facet key is case-sensitive. Allowed facet keys when FacetKey.query is not
* specified: * textual_field = * "brands" * "categories" * "genders" * "ageGroups" *
* "availability" * "colorFamilies" * "colors" * "sizes" * "materials" * "patterns" * "conditions"
* * "attributes.key" * "pickupInStore" * "shipToStore" * "sameDayDelivery" * "nextDayDelivery" *
* "customFulfillment1" * "customFulfillment2" * "customFulfillment3" * "customFulfillment4" *
* "customFulfillment5" * "inventory(place_id,attributes.key)" * numerical_field = * "price" *
* "discount" * "rating" * "ratingCount" * "attributes.key" * "inventory(place_id,price)" *
* "inventory(place_id,original_price)" * "inventory(place_id,attributes.key)"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String key;
/**
* The order in which SearchResponse.Facet.values are returned. Allowed values are: * "count
* desc", which means order by SearchResponse.Facet.values.count descending. * "value desc", which
* means order by SearchResponse.Facet.values.value descending. Only applies to textual facets. If
* not set, textual values are sorted in [natural
* order](https://en.wikipedia.org/wiki/Natural_sort_order); numerical intervals are sorted in the
* order given by FacetSpec.FacetKey.intervals; FulfillmentInfo.place_ids are sorted in the order
* given by FacetSpec.FacetKey.restricted_values.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/**
* Only get facet values that start with the given string prefix. For example, suppose
* "categories" has three values "Women > Shoe", "Women > Dress" and "Men > Shoe". If set
* "prefixes" to "Women", the "categories" facet gives only "Women > Shoe" and "Women > Dress".
* Only supported on textual fields. Maximum is 10.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List prefixes;
/**
* The query that is used to compute facet for the given facet key. When provided, it overrides
* the default behavior of facet computation. The query syntax is the same as a filter expression.
* See SearchRequest.filter for detail syntax and limitations. Notice that there is no limitation
* on FacetKey.key when query is specified. In the response, SearchResponse.Facet.values.value is
* always "1" and SearchResponse.Facet.values.count is the number of results that match the query.
* For example, you can set a customized facet for "shipToStore", where FacetKey.key is
* "customizedShipToStore", and FacetKey.query is "availability: ANY(\"IN_STOCK\") AND
* shipToStore: ANY(\"123\")". Then the facet counts the products that are both in stock and ship
* to store "123".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String query;
/**
* Only get facet for the given restricted values. For example, when using "pickupInStore" as key
* and set restricted values to ["store123", "store456"], only facets for "store123" and
* "store456" are returned. Only supported on predefined textual fields, custom textual attributes
* and fulfillments. Maximum is 20. Must be set for the fulfillment facet keys: * pickupInStore *
* shipToStore * sameDayDelivery * nextDayDelivery * customFulfillment1 * customFulfillment2 *
* customFulfillment3 * customFulfillment4 * customFulfillment5
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List restrictedValues;
/**
* Returns the min and max value for each numerical facet intervals. Ignored for textual facets.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean returnMinMax;
/**
* True to make facet keys case insensitive when getting faceting values with prefixes or
* contains; false otherwise.
* @return value or {@code null} for none
*/
public java.lang.Boolean getCaseInsensitive() {
return caseInsensitive;
}
/**
* True to make facet keys case insensitive when getting faceting values with prefixes or
* contains; false otherwise.
* @param caseInsensitive caseInsensitive or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setCaseInsensitive(java.lang.Boolean caseInsensitive) {
this.caseInsensitive = caseInsensitive;
return this;
}
/**
* Only get facet values that contains the given strings. For example, suppose "categories" has
* three values "Women > Shoe", "Women > Dress" and "Men > Shoe". If set "contains" to "Shoe", the
* "categories" facet gives only "Women > Shoe" and "Men > Shoe". Only supported on textual
* fields. Maximum is 10.
* @return value or {@code null} for none
*/
public java.util.List getContains() {
return contains;
}
/**
* Only get facet values that contains the given strings. For example, suppose "categories" has
* three values "Women > Shoe", "Women > Dress" and "Men > Shoe". If set "contains" to "Shoe", the
* "categories" facet gives only "Women > Shoe" and "Men > Shoe". Only supported on textual
* fields. Maximum is 10.
* @param contains contains or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setContains(java.util.List contains) {
this.contains = contains;
return this;
}
/**
* Set only if values should be bucketized into intervals. Must be set for facets with numerical
* values. Must not be set for facet with text values. Maximum number of intervals is 40. For all
* numerical facet keys that appear in the list of products from the catalog, the percentiles 0,
* 10, 30, 50, 70, 90, and 100 are computed from their distribution weekly. If the model assigns a
* high score to a numerical facet key and its intervals are not specified in the search request,
* these percentiles become the bounds for its intervals and are returned in the response. If the
* facet key intervals are specified in the request, then the specified intervals are returned
* instead.
* @return value or {@code null} for none
*/
public java.util.List getIntervals() {
return intervals;
}
/**
* Set only if values should be bucketized into intervals. Must be set for facets with numerical
* values. Must not be set for facet with text values. Maximum number of intervals is 40. For all
* numerical facet keys that appear in the list of products from the catalog, the percentiles 0,
* 10, 30, 50, 70, 90, and 100 are computed from their distribution weekly. If the model assigns a
* high score to a numerical facet key and its intervals are not specified in the search request,
* these percentiles become the bounds for its intervals and are returned in the response. If the
* facet key intervals are specified in the request, then the specified intervals are returned
* instead.
* @param intervals intervals or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setIntervals(java.util.List intervals) {
this.intervals = intervals;
return this;
}
/**
* Required. Supported textual and numerical facet keys in Product object, over which the facet
* values are computed. Facet key is case-sensitive. Allowed facet keys when FacetKey.query is not
* specified: * textual_field = * "brands" * "categories" * "genders" * "ageGroups" *
* "availability" * "colorFamilies" * "colors" * "sizes" * "materials" * "patterns" * "conditions"
* * "attributes.key" * "pickupInStore" * "shipToStore" * "sameDayDelivery" * "nextDayDelivery" *
* "customFulfillment1" * "customFulfillment2" * "customFulfillment3" * "customFulfillment4" *
* "customFulfillment5" * "inventory(place_id,attributes.key)" * numerical_field = * "price" *
* "discount" * "rating" * "ratingCount" * "attributes.key" * "inventory(place_id,price)" *
* "inventory(place_id,original_price)" * "inventory(place_id,attributes.key)"
* @return value or {@code null} for none
*/
public java.lang.String getKey() {
return key;
}
/**
* Required. Supported textual and numerical facet keys in Product object, over which the facet
* values are computed. Facet key is case-sensitive. Allowed facet keys when FacetKey.query is not
* specified: * textual_field = * "brands" * "categories" * "genders" * "ageGroups" *
* "availability" * "colorFamilies" * "colors" * "sizes" * "materials" * "patterns" * "conditions"
* * "attributes.key" * "pickupInStore" * "shipToStore" * "sameDayDelivery" * "nextDayDelivery" *
* "customFulfillment1" * "customFulfillment2" * "customFulfillment3" * "customFulfillment4" *
* "customFulfillment5" * "inventory(place_id,attributes.key)" * numerical_field = * "price" *
* "discount" * "rating" * "ratingCount" * "attributes.key" * "inventory(place_id,price)" *
* "inventory(place_id,original_price)" * "inventory(place_id,attributes.key)"
* @param key key or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setKey(java.lang.String key) {
this.key = key;
return this;
}
/**
* The order in which SearchResponse.Facet.values are returned. Allowed values are: * "count
* desc", which means order by SearchResponse.Facet.values.count descending. * "value desc", which
* means order by SearchResponse.Facet.values.value descending. Only applies to textual facets. If
* not set, textual values are sorted in [natural
* order](https://en.wikipedia.org/wiki/Natural_sort_order); numerical intervals are sorted in the
* order given by FacetSpec.FacetKey.intervals; FulfillmentInfo.place_ids are sorted in the order
* given by FacetSpec.FacetKey.restricted_values.
* @return value or {@code null} for none
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* The order in which SearchResponse.Facet.values are returned. Allowed values are: * "count
* desc", which means order by SearchResponse.Facet.values.count descending. * "value desc", which
* means order by SearchResponse.Facet.values.value descending. Only applies to textual facets. If
* not set, textual values are sorted in [natural
* order](https://en.wikipedia.org/wiki/Natural_sort_order); numerical intervals are sorted in the
* order given by FacetSpec.FacetKey.intervals; FulfillmentInfo.place_ids are sorted in the order
* given by FacetSpec.FacetKey.restricted_values.
* @param orderBy orderBy or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Only get facet values that start with the given string prefix. For example, suppose
* "categories" has three values "Women > Shoe", "Women > Dress" and "Men > Shoe". If set
* "prefixes" to "Women", the "categories" facet gives only "Women > Shoe" and "Women > Dress".
* Only supported on textual fields. Maximum is 10.
* @return value or {@code null} for none
*/
public java.util.List getPrefixes() {
return prefixes;
}
/**
* Only get facet values that start with the given string prefix. For example, suppose
* "categories" has three values "Women > Shoe", "Women > Dress" and "Men > Shoe". If set
* "prefixes" to "Women", the "categories" facet gives only "Women > Shoe" and "Women > Dress".
* Only supported on textual fields. Maximum is 10.
* @param prefixes prefixes or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setPrefixes(java.util.List prefixes) {
this.prefixes = prefixes;
return this;
}
/**
* The query that is used to compute facet for the given facet key. When provided, it overrides
* the default behavior of facet computation. The query syntax is the same as a filter expression.
* See SearchRequest.filter for detail syntax and limitations. Notice that there is no limitation
* on FacetKey.key when query is specified. In the response, SearchResponse.Facet.values.value is
* always "1" and SearchResponse.Facet.values.count is the number of results that match the query.
* For example, you can set a customized facet for "shipToStore", where FacetKey.key is
* "customizedShipToStore", and FacetKey.query is "availability: ANY(\"IN_STOCK\") AND
* shipToStore: ANY(\"123\")". Then the facet counts the products that are both in stock and ship
* to store "123".
* @return value or {@code null} for none
*/
public java.lang.String getQuery() {
return query;
}
/**
* The query that is used to compute facet for the given facet key. When provided, it overrides
* the default behavior of facet computation. The query syntax is the same as a filter expression.
* See SearchRequest.filter for detail syntax and limitations. Notice that there is no limitation
* on FacetKey.key when query is specified. In the response, SearchResponse.Facet.values.value is
* always "1" and SearchResponse.Facet.values.count is the number of results that match the query.
* For example, you can set a customized facet for "shipToStore", where FacetKey.key is
* "customizedShipToStore", and FacetKey.query is "availability: ANY(\"IN_STOCK\") AND
* shipToStore: ANY(\"123\")". Then the facet counts the products that are both in stock and ship
* to store "123".
* @param query query or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* Only get facet for the given restricted values. For example, when using "pickupInStore" as key
* and set restricted values to ["store123", "store456"], only facets for "store123" and
* "store456" are returned. Only supported on predefined textual fields, custom textual attributes
* and fulfillments. Maximum is 20. Must be set for the fulfillment facet keys: * pickupInStore *
* shipToStore * sameDayDelivery * nextDayDelivery * customFulfillment1 * customFulfillment2 *
* customFulfillment3 * customFulfillment4 * customFulfillment5
* @return value or {@code null} for none
*/
public java.util.List getRestrictedValues() {
return restrictedValues;
}
/**
* Only get facet for the given restricted values. For example, when using "pickupInStore" as key
* and set restricted values to ["store123", "store456"], only facets for "store123" and
* "store456" are returned. Only supported on predefined textual fields, custom textual attributes
* and fulfillments. Maximum is 20. Must be set for the fulfillment facet keys: * pickupInStore *
* shipToStore * sameDayDelivery * nextDayDelivery * customFulfillment1 * customFulfillment2 *
* customFulfillment3 * customFulfillment4 * customFulfillment5
* @param restrictedValues restrictedValues or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setRestrictedValues(java.util.List restrictedValues) {
this.restrictedValues = restrictedValues;
return this;
}
/**
* Returns the min and max value for each numerical facet intervals. Ignored for textual facets.
* @return value or {@code null} for none
*/
public java.lang.Boolean getReturnMinMax() {
return returnMinMax;
}
/**
* Returns the min and max value for each numerical facet intervals. Ignored for textual facets.
* @param returnMinMax returnMinMax or {@code null} for none
*/
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey setReturnMinMax(java.lang.Boolean returnMinMax) {
this.returnMinMax = returnMinMax;
return this;
}
@Override
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey set(String fieldName, Object value) {
return (GoogleCloudRetailV2SearchRequestFacetSpecFacetKey) super.set(fieldName, value);
}
@Override
public GoogleCloudRetailV2SearchRequestFacetSpecFacetKey clone() {
return (GoogleCloudRetailV2SearchRequestFacetSpecFacetKey) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy