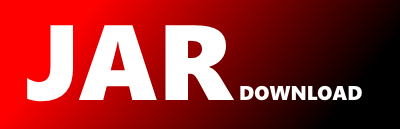
com.google.api.services.run.v1.model.TaskStatus Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v1.model;
/**
* TaskStatus represents the status of a task.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class TaskStatus extends com.google.api.client.json.GenericJson {
/**
* Optional. Represents time when the task was completed. It is not guaranteed to be set in
* happens-before order across separate operations. It is represented in RFC3339 form and is in
* UTC.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String completionTime;
/**
* Optional. Conditions communicate information about ongoing/complete reconciliation processes
* that bring the "spec" inline with the observed state of the world. Task-specific conditions
* include: * `Started`: `True` when the task has started to execute. * `Completed`: `True` when
* the task has succeeded. `False` when the task has failed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List conditions;
static {
// hack to force ProGuard to consider GoogleCloudRunV1Condition used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudRunV1Condition.class);
}
/**
* Required. Index of the task, unique per execution, and beginning at 0.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer index;
/**
* Optional. Result of the last attempt of this task.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TaskAttemptResult lastAttemptResult;
/**
* Optional. URI where logs for this task can be found in Cloud Console.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String logUri;
/**
* Optional. The 'generation' of the task that was last processed by the controller.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer observedGeneration;
/**
* Optional. The number of times this task was retried. Instances are retried when they fail up to
* the maxRetries limit.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer retried;
/**
* Optional. Represents time when the task started to run. It is not guaranteed to be set in
* happens-before order across separate operations. It is represented in RFC3339 form and is in
* UTC.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* Optional. Represents time when the task was completed. It is not guaranteed to be set in
* happens-before order across separate operations. It is represented in RFC3339 form and is in
* UTC.
* @return value or {@code null} for none
*/
public String getCompletionTime() {
return completionTime;
}
/**
* Optional. Represents time when the task was completed. It is not guaranteed to be set in
* happens-before order across separate operations. It is represented in RFC3339 form and is in
* UTC.
* @param completionTime completionTime or {@code null} for none
*/
public TaskStatus setCompletionTime(String completionTime) {
this.completionTime = completionTime;
return this;
}
/**
* Optional. Conditions communicate information about ongoing/complete reconciliation processes
* that bring the "spec" inline with the observed state of the world. Task-specific conditions
* include: * `Started`: `True` when the task has started to execute. * `Completed`: `True` when
* the task has succeeded. `False` when the task has failed.
* @return value or {@code null} for none
*/
public java.util.List getConditions() {
return conditions;
}
/**
* Optional. Conditions communicate information about ongoing/complete reconciliation processes
* that bring the "spec" inline with the observed state of the world. Task-specific conditions
* include: * `Started`: `True` when the task has started to execute. * `Completed`: `True` when
* the task has succeeded. `False` when the task has failed.
* @param conditions conditions or {@code null} for none
*/
public TaskStatus setConditions(java.util.List conditions) {
this.conditions = conditions;
return this;
}
/**
* Required. Index of the task, unique per execution, and beginning at 0.
* @return value or {@code null} for none
*/
public java.lang.Integer getIndex() {
return index;
}
/**
* Required. Index of the task, unique per execution, and beginning at 0.
* @param index index or {@code null} for none
*/
public TaskStatus setIndex(java.lang.Integer index) {
this.index = index;
return this;
}
/**
* Optional. Result of the last attempt of this task.
* @return value or {@code null} for none
*/
public TaskAttemptResult getLastAttemptResult() {
return lastAttemptResult;
}
/**
* Optional. Result of the last attempt of this task.
* @param lastAttemptResult lastAttemptResult or {@code null} for none
*/
public TaskStatus setLastAttemptResult(TaskAttemptResult lastAttemptResult) {
this.lastAttemptResult = lastAttemptResult;
return this;
}
/**
* Optional. URI where logs for this task can be found in Cloud Console.
* @return value or {@code null} for none
*/
public java.lang.String getLogUri() {
return logUri;
}
/**
* Optional. URI where logs for this task can be found in Cloud Console.
* @param logUri logUri or {@code null} for none
*/
public TaskStatus setLogUri(java.lang.String logUri) {
this.logUri = logUri;
return this;
}
/**
* Optional. The 'generation' of the task that was last processed by the controller.
* @return value or {@code null} for none
*/
public java.lang.Integer getObservedGeneration() {
return observedGeneration;
}
/**
* Optional. The 'generation' of the task that was last processed by the controller.
* @param observedGeneration observedGeneration or {@code null} for none
*/
public TaskStatus setObservedGeneration(java.lang.Integer observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
/**
* Optional. The number of times this task was retried. Instances are retried when they fail up to
* the maxRetries limit.
* @return value or {@code null} for none
*/
public java.lang.Integer getRetried() {
return retried;
}
/**
* Optional. The number of times this task was retried. Instances are retried when they fail up to
* the maxRetries limit.
* @param retried retried or {@code null} for none
*/
public TaskStatus setRetried(java.lang.Integer retried) {
this.retried = retried;
return this;
}
/**
* Optional. Represents time when the task started to run. It is not guaranteed to be set in
* happens-before order across separate operations. It is represented in RFC3339 form and is in
* UTC.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* Optional. Represents time when the task started to run. It is not guaranteed to be set in
* happens-before order across separate operations. It is represented in RFC3339 form and is in
* UTC.
* @param startTime startTime or {@code null} for none
*/
public TaskStatus setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
@Override
public TaskStatus set(String fieldName, Object value) {
return (TaskStatus) super.set(fieldName, value);
}
@Override
public TaskStatus clone() {
return (TaskStatus) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy