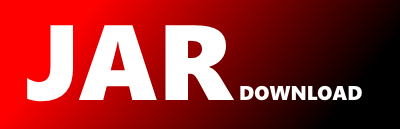
com.google.api.services.run.v1.model.ObjectMeta Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v1.model;
/**
* google.cloud.run.meta.v1.ObjectMeta is metadata that all persisted resources must have, which
* includes all objects users must create.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ObjectMeta extends com.google.api.client.json.GenericJson {
/**
* Unstructured key value map stored with a resource that may be set by external tools to store
* and retrieve arbitrary metadata. They are not queryable and should be preserved when modifying
* objects. In Cloud Run, annotations with 'run.googleapis.com/' and 'autoscaling.knative.dev' are
* restricted, and the accepted annotations will be different depending on the resource type. *
* `autoscaling.knative.dev/maxScale`: Revision. * `autoscaling.knative.dev/minScale`: Revision. *
* `run.googleapis.com/base-images`: Revision, Service. * `run.googleapis.com/binary-
* authorization-breakglass`: Service, Job, * `run.googleapis.com/binary-authorization`: Service,
* Job, Execution. * `run.googleapis.com/build-environment-variables`: Service. *
* `run.googleapis.com/build-id`: Service. * `run.googleapis.com/build-name`: Service. *
* `run.googleapis.com/build-service-account`: Service. * `run.googleapis.com/build-worker-pool`:
* Service. * `run.googleapis.com/client-name`: All resources. * `run.googleapis.com/cloudsql-
* instances`: Revision, Execution. * `run.googleapis.com/container-dependencies`: Revision . *
* `run.googleapis.com/cpu-throttling`: Revision. * `run.googleapis.com/custom-audiences`:
* Service. * `run.googleapis.com/default-url-disabled`: Service. *
* `run.googleapis.com/description`: Service. * `run.googleapis.com/encryption-key-shutdown-
* hours`: Revision * `run.googleapis.com/encryption-key`: Revision, Execution. *
* `run.googleapis.com/execution-environment`: Revision, Execution. *
* `run.googleapis.com/function-target`: Service. * `run.googleapis.com/gc-traffic-tags`: Service.
* * `run.googleapis.com/image-uri`: Service. * `run.googleapis.com/ingress`: Service. *
* `run.googleapis.com/launch-stage`: Service, Job. * `run.googleapis.com/minScale`: Service
* (ALPHA) * `run.googleapis.com/network-interfaces`: Revision, Execution. *
* `run.googleapis.com/post-key-revocation-action-type`: Revision. * `run.googleapis.com/runtime`:
* Service. * `run.googleapis.com/secrets`: Revision, Execution. * `run.googleapis.com/secure-
* session-agent`: Revision. * `run.googleapis.com/sessionAffinity`: Revision. *
* `run.googleapis.com/source-location`: Service. * `run.googleapis.com/startup-cpu-boost`:
* Revision. * `run.googleapis.com/vpc-access-connector`: Revision, Execution. *
* `run.googleapis.com/vpc-access-egress`: Revision, Execution.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map annotations;
/**
* Not supported by Cloud Run
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clusterName;
/**
* UTC timestamp representing the server time when this object was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String creationTimestamp;
/**
* Not supported by Cloud Run
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer deletionGracePeriodSeconds;
/**
* The read-only soft deletion timestamp for this resource. In Cloud Run, users are not able to
* set this field. Instead, they must call the corresponding Delete API.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String deletionTimestamp;
/**
* Not supported by Cloud Run
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List finalizers;
/**
* Not supported by Cloud Run
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String generateName;
/**
* A system-provided sequence number representing a specific generation of the desired state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer generation;
/**
* Map of string keys and values that can be used to organize and categorize (scope and select)
* objects. May match selectors of replication controllers and routes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Required. The name of the resource. Name is required when creating top-level resources
* (Service, Job), must be unique within a Cloud Run project/region, and cannot be changed once
* created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Required. Defines the space within each name must be unique within a Cloud Run region. In Cloud
* Run, it must be project ID or number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String namespace;
/**
* Not supported by Cloud Run
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List ownerReferences;
/**
* Opaque, system-generated value that represents the internal version of this object that can be
* used by clients to determine when objects have changed. May be used for optimistic concurrency,
* change detection, and the watch operation on a resource or set of resources. Clients must treat
* these values as opaque and passed unmodified back to the server or omit the value to disable
* conflict-detection.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/**
* URL representing this object.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* Unique, system-generated identifier for this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* Unstructured key value map stored with a resource that may be set by external tools to store
* and retrieve arbitrary metadata. They are not queryable and should be preserved when modifying
* objects. In Cloud Run, annotations with 'run.googleapis.com/' and 'autoscaling.knative.dev' are
* restricted, and the accepted annotations will be different depending on the resource type. *
* `autoscaling.knative.dev/maxScale`: Revision. * `autoscaling.knative.dev/minScale`: Revision. *
* `run.googleapis.com/base-images`: Revision, Service. * `run.googleapis.com/binary-
* authorization-breakglass`: Service, Job, * `run.googleapis.com/binary-authorization`: Service,
* Job, Execution. * `run.googleapis.com/build-environment-variables`: Service. *
* `run.googleapis.com/build-id`: Service. * `run.googleapis.com/build-name`: Service. *
* `run.googleapis.com/build-service-account`: Service. * `run.googleapis.com/build-worker-pool`:
* Service. * `run.googleapis.com/client-name`: All resources. * `run.googleapis.com/cloudsql-
* instances`: Revision, Execution. * `run.googleapis.com/container-dependencies`: Revision . *
* `run.googleapis.com/cpu-throttling`: Revision. * `run.googleapis.com/custom-audiences`:
* Service. * `run.googleapis.com/default-url-disabled`: Service. *
* `run.googleapis.com/description`: Service. * `run.googleapis.com/encryption-key-shutdown-
* hours`: Revision * `run.googleapis.com/encryption-key`: Revision, Execution. *
* `run.googleapis.com/execution-environment`: Revision, Execution. *
* `run.googleapis.com/function-target`: Service. * `run.googleapis.com/gc-traffic-tags`: Service.
* * `run.googleapis.com/image-uri`: Service. * `run.googleapis.com/ingress`: Service. *
* `run.googleapis.com/launch-stage`: Service, Job. * `run.googleapis.com/minScale`: Service
* (ALPHA) * `run.googleapis.com/network-interfaces`: Revision, Execution. *
* `run.googleapis.com/post-key-revocation-action-type`: Revision. * `run.googleapis.com/runtime`:
* Service. * `run.googleapis.com/secrets`: Revision, Execution. * `run.googleapis.com/secure-
* session-agent`: Revision. * `run.googleapis.com/sessionAffinity`: Revision. *
* `run.googleapis.com/source-location`: Service. * `run.googleapis.com/startup-cpu-boost`:
* Revision. * `run.googleapis.com/vpc-access-connector`: Revision, Execution. *
* `run.googleapis.com/vpc-access-egress`: Revision, Execution.
* @return value or {@code null} for none
*/
public java.util.Map getAnnotations() {
return annotations;
}
/**
* Unstructured key value map stored with a resource that may be set by external tools to store
* and retrieve arbitrary metadata. They are not queryable and should be preserved when modifying
* objects. In Cloud Run, annotations with 'run.googleapis.com/' and 'autoscaling.knative.dev' are
* restricted, and the accepted annotations will be different depending on the resource type. *
* `autoscaling.knative.dev/maxScale`: Revision. * `autoscaling.knative.dev/minScale`: Revision. *
* `run.googleapis.com/base-images`: Revision, Service. * `run.googleapis.com/binary-
* authorization-breakglass`: Service, Job, * `run.googleapis.com/binary-authorization`: Service,
* Job, Execution. * `run.googleapis.com/build-environment-variables`: Service. *
* `run.googleapis.com/build-id`: Service. * `run.googleapis.com/build-name`: Service. *
* `run.googleapis.com/build-service-account`: Service. * `run.googleapis.com/build-worker-pool`:
* Service. * `run.googleapis.com/client-name`: All resources. * `run.googleapis.com/cloudsql-
* instances`: Revision, Execution. * `run.googleapis.com/container-dependencies`: Revision . *
* `run.googleapis.com/cpu-throttling`: Revision. * `run.googleapis.com/custom-audiences`:
* Service. * `run.googleapis.com/default-url-disabled`: Service. *
* `run.googleapis.com/description`: Service. * `run.googleapis.com/encryption-key-shutdown-
* hours`: Revision * `run.googleapis.com/encryption-key`: Revision, Execution. *
* `run.googleapis.com/execution-environment`: Revision, Execution. *
* `run.googleapis.com/function-target`: Service. * `run.googleapis.com/gc-traffic-tags`: Service.
* * `run.googleapis.com/image-uri`: Service. * `run.googleapis.com/ingress`: Service. *
* `run.googleapis.com/launch-stage`: Service, Job. * `run.googleapis.com/minScale`: Service
* (ALPHA) * `run.googleapis.com/network-interfaces`: Revision, Execution. *
* `run.googleapis.com/post-key-revocation-action-type`: Revision. * `run.googleapis.com/runtime`:
* Service. * `run.googleapis.com/secrets`: Revision, Execution. * `run.googleapis.com/secure-
* session-agent`: Revision. * `run.googleapis.com/sessionAffinity`: Revision. *
* `run.googleapis.com/source-location`: Service. * `run.googleapis.com/startup-cpu-boost`:
* Revision. * `run.googleapis.com/vpc-access-connector`: Revision, Execution. *
* `run.googleapis.com/vpc-access-egress`: Revision, Execution.
* @param annotations annotations or {@code null} for none
*/
public ObjectMeta setAnnotations(java.util.Map annotations) {
this.annotations = annotations;
return this;
}
/**
* Not supported by Cloud Run
* @return value or {@code null} for none
*/
public java.lang.String getClusterName() {
return clusterName;
}
/**
* Not supported by Cloud Run
* @param clusterName clusterName or {@code null} for none
*/
public ObjectMeta setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* UTC timestamp representing the server time when this object was created.
* @return value or {@code null} for none
*/
public String getCreationTimestamp() {
return creationTimestamp;
}
/**
* UTC timestamp representing the server time when this object was created.
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public ObjectMeta setCreationTimestamp(String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* Not supported by Cloud Run
* @return value or {@code null} for none
*/
public java.lang.Integer getDeletionGracePeriodSeconds() {
return deletionGracePeriodSeconds;
}
/**
* Not supported by Cloud Run
* @param deletionGracePeriodSeconds deletionGracePeriodSeconds or {@code null} for none
*/
public ObjectMeta setDeletionGracePeriodSeconds(java.lang.Integer deletionGracePeriodSeconds) {
this.deletionGracePeriodSeconds = deletionGracePeriodSeconds;
return this;
}
/**
* The read-only soft deletion timestamp for this resource. In Cloud Run, users are not able to
* set this field. Instead, they must call the corresponding Delete API.
* @return value or {@code null} for none
*/
public String getDeletionTimestamp() {
return deletionTimestamp;
}
/**
* The read-only soft deletion timestamp for this resource. In Cloud Run, users are not able to
* set this field. Instead, they must call the corresponding Delete API.
* @param deletionTimestamp deletionTimestamp or {@code null} for none
*/
public ObjectMeta setDeletionTimestamp(String deletionTimestamp) {
this.deletionTimestamp = deletionTimestamp;
return this;
}
/**
* Not supported by Cloud Run
* @return value or {@code null} for none
*/
public java.util.List getFinalizers() {
return finalizers;
}
/**
* Not supported by Cloud Run
* @param finalizers finalizers or {@code null} for none
*/
public ObjectMeta setFinalizers(java.util.List finalizers) {
this.finalizers = finalizers;
return this;
}
/**
* Not supported by Cloud Run
* @return value or {@code null} for none
*/
public java.lang.String getGenerateName() {
return generateName;
}
/**
* Not supported by Cloud Run
* @param generateName generateName or {@code null} for none
*/
public ObjectMeta setGenerateName(java.lang.String generateName) {
this.generateName = generateName;
return this;
}
/**
* A system-provided sequence number representing a specific generation of the desired state.
* @return value or {@code null} for none
*/
public java.lang.Integer getGeneration() {
return generation;
}
/**
* A system-provided sequence number representing a specific generation of the desired state.
* @param generation generation or {@code null} for none
*/
public ObjectMeta setGeneration(java.lang.Integer generation) {
this.generation = generation;
return this;
}
/**
* Map of string keys and values that can be used to organize and categorize (scope and select)
* objects. May match selectors of replication controllers and routes.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Map of string keys and values that can be used to organize and categorize (scope and select)
* objects. May match selectors of replication controllers and routes.
* @param labels labels or {@code null} for none
*/
public ObjectMeta setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Required. The name of the resource. Name is required when creating top-level resources
* (Service, Job), must be unique within a Cloud Run project/region, and cannot be changed once
* created.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource. Name is required when creating top-level resources
* (Service, Job), must be unique within a Cloud Run project/region, and cannot be changed once
* created.
* @param name name or {@code null} for none
*/
public ObjectMeta setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Required. Defines the space within each name must be unique within a Cloud Run region. In Cloud
* Run, it must be project ID or number.
* @return value or {@code null} for none
*/
public java.lang.String getNamespace() {
return namespace;
}
/**
* Required. Defines the space within each name must be unique within a Cloud Run region. In Cloud
* Run, it must be project ID or number.
* @param namespace namespace or {@code null} for none
*/
public ObjectMeta setNamespace(java.lang.String namespace) {
this.namespace = namespace;
return this;
}
/**
* Not supported by Cloud Run
* @return value or {@code null} for none
*/
public java.util.List getOwnerReferences() {
return ownerReferences;
}
/**
* Not supported by Cloud Run
* @param ownerReferences ownerReferences or {@code null} for none
*/
public ObjectMeta setOwnerReferences(java.util.List ownerReferences) {
this.ownerReferences = ownerReferences;
return this;
}
/**
* Opaque, system-generated value that represents the internal version of this object that can be
* used by clients to determine when objects have changed. May be used for optimistic concurrency,
* change detection, and the watch operation on a resource or set of resources. Clients must treat
* these values as opaque and passed unmodified back to the server or omit the value to disable
* conflict-detection.
* @return value or {@code null} for none
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* Opaque, system-generated value that represents the internal version of this object that can be
* used by clients to determine when objects have changed. May be used for optimistic concurrency,
* change detection, and the watch operation on a resource or set of resources. Clients must treat
* these values as opaque and passed unmodified back to the server or omit the value to disable
* conflict-detection.
* @param resourceVersion resourceVersion or {@code null} for none
*/
public ObjectMeta setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/**
* URL representing this object.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* URL representing this object.
* @param selfLink selfLink or {@code null} for none
*/
public ObjectMeta setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* Unique, system-generated identifier for this resource.
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* Unique, system-generated identifier for this resource.
* @param uid uid or {@code null} for none
*/
public ObjectMeta setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
@Override
public ObjectMeta set(String fieldName, Object value) {
return (ObjectMeta) super.set(fieldName, value);
}
@Override
public ObjectMeta clone() {
return (ObjectMeta) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy