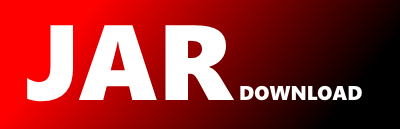
com.google.api.services.run.v1.model.TaskSpec Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v1.model;
/**
* TaskSpec is a description of a task.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class TaskSpec extends com.google.api.client.json.GenericJson {
/**
* Optional. List of containers belonging to the task. We disallow a number of fields on this
* Container.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List containers;
static {
// hack to force ProGuard to consider Container used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Container.class);
}
/**
* Optional. Number of retries allowed per task, before marking this job failed. Defaults to 3.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxRetries;
/**
* Optional. Email address of the IAM service account associated with the task of a job execution.
* The service account represents the identity of the running task, and determines what
* permissions the task has. If not provided, the task will use the project's default service
* account.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceAccountName;
/**
* Optional. Duration in seconds the task may be active before the system will actively try to
* mark it failed and kill associated containers. This applies per attempt of a task, meaning each
* retry can run for the full timeout. Defaults to 600 seconds.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long timeoutSeconds;
/**
* Optional. List of volumes that can be mounted by containers belonging to the task.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List volumes;
/**
* Optional. List of containers belonging to the task. We disallow a number of fields on this
* Container.
* @return value or {@code null} for none
*/
public java.util.List getContainers() {
return containers;
}
/**
* Optional. List of containers belonging to the task. We disallow a number of fields on this
* Container.
* @param containers containers or {@code null} for none
*/
public TaskSpec setContainers(java.util.List containers) {
this.containers = containers;
return this;
}
/**
* Optional. Number of retries allowed per task, before marking this job failed. Defaults to 3.
* @return value or {@code null} for none
*/
public java.lang.Integer getMaxRetries() {
return maxRetries;
}
/**
* Optional. Number of retries allowed per task, before marking this job failed. Defaults to 3.
* @param maxRetries maxRetries or {@code null} for none
*/
public TaskSpec setMaxRetries(java.lang.Integer maxRetries) {
this.maxRetries = maxRetries;
return this;
}
/**
* Optional. Email address of the IAM service account associated with the task of a job execution.
* The service account represents the identity of the running task, and determines what
* permissions the task has. If not provided, the task will use the project's default service
* account.
* @return value or {@code null} for none
*/
public java.lang.String getServiceAccountName() {
return serviceAccountName;
}
/**
* Optional. Email address of the IAM service account associated with the task of a job execution.
* The service account represents the identity of the running task, and determines what
* permissions the task has. If not provided, the task will use the project's default service
* account.
* @param serviceAccountName serviceAccountName or {@code null} for none
*/
public TaskSpec setServiceAccountName(java.lang.String serviceAccountName) {
this.serviceAccountName = serviceAccountName;
return this;
}
/**
* Optional. Duration in seconds the task may be active before the system will actively try to
* mark it failed and kill associated containers. This applies per attempt of a task, meaning each
* retry can run for the full timeout. Defaults to 600 seconds.
* @return value or {@code null} for none
*/
public java.lang.Long getTimeoutSeconds() {
return timeoutSeconds;
}
/**
* Optional. Duration in seconds the task may be active before the system will actively try to
* mark it failed and kill associated containers. This applies per attempt of a task, meaning each
* retry can run for the full timeout. Defaults to 600 seconds.
* @param timeoutSeconds timeoutSeconds or {@code null} for none
*/
public TaskSpec setTimeoutSeconds(java.lang.Long timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
return this;
}
/**
* Optional. List of volumes that can be mounted by containers belonging to the task.
* @return value or {@code null} for none
*/
public java.util.List getVolumes() {
return volumes;
}
/**
* Optional. List of volumes that can be mounted by containers belonging to the task.
* @param volumes volumes or {@code null} for none
*/
public TaskSpec setVolumes(java.util.List volumes) {
this.volumes = volumes;
return this;
}
@Override
public TaskSpec set(String fieldName, Object value) {
return (TaskSpec) super.set(fieldName, value);
}
@Override
public TaskSpec clone() {
return (TaskSpec) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy