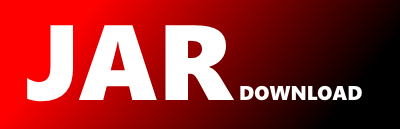
com.google.api.services.run.v1.model.TrafficTarget Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v1.model;
/**
* TrafficTarget holds a single entry of the routing table for a Route.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class TrafficTarget extends com.google.api.client.json.GenericJson {
/**
* [Deprecated] Not supported in Cloud Run. It must be empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String configurationName;
/**
* Uses the "status.latestReadyRevisionName" of the Service to determine the traffic target. When
* it changes, traffic will automatically migrate from the prior "latest ready" revision to the
* new one. This field must be false if RevisionName is set. This field defaults to true
* otherwise. If the field is set to true on Status, this means that the Revision was resolved
* from the Service's latest ready revision.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean latestRevision;
/**
* Percent specifies percent of the traffic to this Revision or Configuration. This defaults to
* zero if unspecified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer percent;
/**
* Points this traffic target to a specific Revision. This field is mutually exclusive with
* latest_revision.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String revisionName;
/**
* Tag is used to expose a dedicated url for referencing this target exclusively.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String tag;
/**
* Output only. URL displays the URL for accessing tagged traffic targets. URL is displayed in
* status, and is disallowed on spec. URL must contain a scheme (e.g. https://) and a hostname,
* but may not contain anything else (e.g. basic auth, url path, etc.)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String url;
/**
* [Deprecated] Not supported in Cloud Run. It must be empty.
* @return value or {@code null} for none
*/
public java.lang.String getConfigurationName() {
return configurationName;
}
/**
* [Deprecated] Not supported in Cloud Run. It must be empty.
* @param configurationName configurationName or {@code null} for none
*/
public TrafficTarget setConfigurationName(java.lang.String configurationName) {
this.configurationName = configurationName;
return this;
}
/**
* Uses the "status.latestReadyRevisionName" of the Service to determine the traffic target. When
* it changes, traffic will automatically migrate from the prior "latest ready" revision to the
* new one. This field must be false if RevisionName is set. This field defaults to true
* otherwise. If the field is set to true on Status, this means that the Revision was resolved
* from the Service's latest ready revision.
* @return value or {@code null} for none
*/
public java.lang.Boolean getLatestRevision() {
return latestRevision;
}
/**
* Uses the "status.latestReadyRevisionName" of the Service to determine the traffic target. When
* it changes, traffic will automatically migrate from the prior "latest ready" revision to the
* new one. This field must be false if RevisionName is set. This field defaults to true
* otherwise. If the field is set to true on Status, this means that the Revision was resolved
* from the Service's latest ready revision.
* @param latestRevision latestRevision or {@code null} for none
*/
public TrafficTarget setLatestRevision(java.lang.Boolean latestRevision) {
this.latestRevision = latestRevision;
return this;
}
/**
* Percent specifies percent of the traffic to this Revision or Configuration. This defaults to
* zero if unspecified.
* @return value or {@code null} for none
*/
public java.lang.Integer getPercent() {
return percent;
}
/**
* Percent specifies percent of the traffic to this Revision or Configuration. This defaults to
* zero if unspecified.
* @param percent percent or {@code null} for none
*/
public TrafficTarget setPercent(java.lang.Integer percent) {
this.percent = percent;
return this;
}
/**
* Points this traffic target to a specific Revision. This field is mutually exclusive with
* latest_revision.
* @return value or {@code null} for none
*/
public java.lang.String getRevisionName() {
return revisionName;
}
/**
* Points this traffic target to a specific Revision. This field is mutually exclusive with
* latest_revision.
* @param revisionName revisionName or {@code null} for none
*/
public TrafficTarget setRevisionName(java.lang.String revisionName) {
this.revisionName = revisionName;
return this;
}
/**
* Tag is used to expose a dedicated url for referencing this target exclusively.
* @return value or {@code null} for none
*/
public java.lang.String getTag() {
return tag;
}
/**
* Tag is used to expose a dedicated url for referencing this target exclusively.
* @param tag tag or {@code null} for none
*/
public TrafficTarget setTag(java.lang.String tag) {
this.tag = tag;
return this;
}
/**
* Output only. URL displays the URL for accessing tagged traffic targets. URL is displayed in
* status, and is disallowed on spec. URL must contain a scheme (e.g. https://) and a hostname,
* but may not contain anything else (e.g. basic auth, url path, etc.)
* @return value or {@code null} for none
*/
public java.lang.String getUrl() {
return url;
}
/**
* Output only. URL displays the URL for accessing tagged traffic targets. URL is displayed in
* status, and is disallowed on spec. URL must contain a scheme (e.g. https://) and a hostname,
* but may not contain anything else (e.g. basic auth, url path, etc.)
* @param url url or {@code null} for none
*/
public TrafficTarget setUrl(java.lang.String url) {
this.url = url;
return this;
}
@Override
public TrafficTarget set(String fieldName, Object value) {
return (TrafficTarget) super.set(fieldName, value);
}
@Override
public TrafficTarget clone() {
return (TrafficTarget) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy