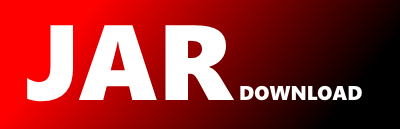
com.google.api.services.run.v1alpha1.CloudRun Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2019-11-08 at 00:46:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.run.v1alpha1;
/**
* Service definition for CloudRun (v1alpha1).
*
*
* Deploy and manage user provided container images that scale automatically based on HTTP traffic.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudRunRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudRun extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.18.0-rc of the Cloud Run API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://run.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudRun(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudRun(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Namespaces collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Namespaces.List request = run.namespaces().list(parameters ...)}
*
*
* @return the resource collection
*/
public Namespaces namespaces() {
return new Namespaces();
}
/**
* The "namespaces" collection of methods.
*/
public class Namespaces {
/**
* An accessor for creating requests from the Authorizeddomains collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Authorizeddomains.List request = run.authorizeddomains().list(parameters ...)}
*
*
* @return the resource collection
*/
public Authorizeddomains authorizeddomains() {
return new Authorizeddomains();
}
/**
* The "authorizeddomains" collection of methods.
*/
public class Authorizeddomains {
/**
* RPC to list authorized domains.
*
* Create a request for the method "authorizeddomains.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Name of the parent Application resource. Example: `apps/myapp`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/domains.cloudrun.com/v1alpha1/{+parent}/authorizeddomains";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* RPC to list authorized domains.
*
* Create a request for the method "authorizeddomains.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Name of the parent Application resource. Example: `apps/myapp`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListAuthorizedDomainsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Name of the parent Application resource. Example: `apps/myapp`. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Name of the parent Application resource. Example: `apps/myapp`.
*/
public java.lang.String getParent() {
return parent;
}
/** Name of the parent Application resource. Example: `apps/myapp`. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/** Continuation token for fetching the next page of results. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation token for fetching the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation token for fetching the next page of results. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum results to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum results to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum results to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Configurations collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Configurations.List request = run.configurations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Configurations configurations() {
return new Configurations();
}
/**
* The "configurations" collection of methods.
*/
public class Configurations {
/**
* Rpc to get information about a configuration.
*
* Create a request for the method "configurations.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the configuration being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/configurations/[^/]+$");
/**
* Rpc to get information about a configuration.
*
* Create a request for the method "configurations.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the configuration being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Configuration.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/configurations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the configuration being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the configuration being retrieved. If needed, replace {namespace_id} with the project
ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the configuration being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/configurations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list configurations.
*
* Create a request for the method "configurations.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the configurations should be
listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+parent}/configurations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list configurations.
*
* Create a request for the method "configurations.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the configurations should be
listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListConfigurationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the configurations should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the configurations should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the configurations should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Domainmappings collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Domainmappings.List request = run.domainmappings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Domainmappings domainmappings() {
return new Domainmappings();
}
/**
* The "domainmappings" collection of methods.
*/
public class Domainmappings {
/**
* Creates a new domain mapping.
*
* Create a request for the method "domainmappings.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this domain mapping should be
created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.DomainMapping}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.DomainMapping content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "apis/domains.cloudrun.com/v1alpha1/{+parent}/domainmappings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Creates a new domain mapping.
*
* Create a request for the method "domainmappings.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this domain mapping should be
created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.DomainMapping}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.DomainMapping content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.DomainMapping.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number in which this domain mapping should be created.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this domain mapping should be created.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number in which this domain mapping should be created.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a domain mapping.
*
* Create a request for the method "domainmappings.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the domain mapping being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "apis/domains.cloudrun.com/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/domainmappings/[^/]+$");
/**
* Rpc to delete a domain mapping.
*
* Create a request for the method "domainmappings.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the domain mapping being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/domainmappings/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the domain mapping being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the domain mapping being deleted. If needed, replace {namespace_id} with the project
ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the domain mapping being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/domainmappings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
@com.google.api.client.util.Key
private java.lang.Boolean orphanDependents;
/** Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading behavior,
so this must be false. This attribute is deprecated, and is now replaced with PropagationPolicy See
https://github.com/kubernetes/kubernetes/issues/46659 for more info.
*/
public java.lang.Boolean getOrphanDependents() {
return orphanDependents;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
public Delete setOrphanDependents(java.lang.Boolean orphanDependents) {
this.orphanDependents = orphanDependents;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a domain mapping.
*
* Create a request for the method "domainmappings.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the domain mapping being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/domains.cloudrun.com/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/domainmappings/[^/]+$");
/**
* Rpc to get information about a domain mapping.
*
* Create a request for the method "domainmappings.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the domain mapping being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.DomainMapping.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/domainmappings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the domain mapping being retrieved. If needed, replace {namespace_id} with
* the project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the domain mapping being retrieved. If needed, replace {namespace_id} with the project
ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the domain mapping being retrieved. If needed, replace {namespace_id} with
* the project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/domainmappings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list domain mappings.
*
* Create a request for the method "domainmappings.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the domain mappings should be
listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/domains.cloudrun.com/v1alpha1/{+parent}/domainmappings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list domain mappings.
*
* Create a request for the method "domainmappings.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the domain mappings should be
listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListDomainMappingsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the domain mappings should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the domain mappings should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the domain mappings should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Eventtypes collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Eventtypes.List request = run.eventtypes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Eventtypes eventtypes() {
return new Eventtypes();
}
/**
* The "eventtypes" collection of methods.
*/
public class Eventtypes {
/**
* Rpc to get information about an EventType.
*
* Create a request for the method "eventtypes.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/eventing.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/eventtypes/[^/]+$");
/**
* Rpc to get information about an EventType.
*
* Create a request for the method "eventtypes.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.EventType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/eventtypes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/eventtypes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list EventTypes.
*
* Create a request for the method "eventtypes.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the EventTypes should be
listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/eventing.knative.dev/v1alpha1/{+parent}/eventtypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list EventTypes.
*
* Create a request for the method "eventtypes.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the EventTypes should be
listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListEventTypesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the EventTypes should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the EventTypes should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the EventTypes should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Pubsubs collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Pubsubs.List request = run.pubsubs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Pubsubs pubsubs() {
return new Pubsubs();
}
/**
* The "pubsubs" collection of methods.
*/
public class Pubsubs {
/**
* Creates a new pubsub.
*
* Create a request for the method "pubsubs.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this pubsub should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.PubSub content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "apis/events.cloud.google.com/v1alpha1/{+parent}/pubsubs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Creates a new pubsub.
*
* Create a request for the method "pubsubs.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this pubsub should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.PubSub content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.PubSub.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number in which this pubsub should be created.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this pubsub should be created.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number in which this pubsub should be created.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a pubsub.
*
* Create a request for the method "pubsubs.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the pubsub being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "apis/events.cloud.google.com/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/pubsubs/[^/]+$");
/**
* Rpc to delete a pubsub.
*
* Create a request for the method "pubsubs.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the pubsub being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/pubsubs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the pubsub being deleted. If needed, replace {namespace_id} with the project
* ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the pubsub being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the pubsub being deleted. If needed, replace {namespace_id} with the project
* ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/pubsubs/[^/]+$");
}
this.name = name;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a pubsub.
*
* Create a request for the method "pubsubs.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/events.cloud.google.com/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/pubsubs/[^/]+$");
/**
* Rpc to get information about a pubsub.
*
* Create a request for the method "pubsubs.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.PubSub.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/pubsubs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the pubsub being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/pubsubs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list pubsubs.
*
* Create a request for the method "pubsubs.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the pubsubs should
be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/events.cloud.google.com/v1alpha1/{+parent}/pubsubs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list pubsubs.
*
* Create a request for the method "pubsubs.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the pubsubs should
be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListPubSubsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the pubsubs should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the pubsubs should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the pubsubs should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Rpc to replace a pubsub.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request, Cloud
* Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic concurrency
* control.
*
* Create a request for the method "pubsubs.replacePubSub".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ReplacePubSub#execute()} method to invoke the remote operation.
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @return the request
*/
public ReplacePubSub replacePubSub(java.lang.String name, com.google.api.services.run.v1alpha1.model.PubSub content) throws java.io.IOException {
ReplacePubSub result = new ReplacePubSub(name, content);
initialize(result);
return result;
}
public class ReplacePubSub extends CloudRunRequest {
private static final String REST_PATH = "apis/events.cloud.google.com/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/pubsubs/[^/]+$");
/**
* Rpc to replace a pubsub.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request,
* Cloud Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic
* concurrency control.
*
* Create a request for the method "pubsubs.replacePubSub".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ReplacePubSub#execute()} method to invoke the remote operation.
* {@link ReplacePubSub#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @since 1.13
*/
protected ReplacePubSub(java.lang.String name, com.google.api.services.run.v1alpha1.model.PubSub content) {
super(CloudRun.this, "PUT", REST_PATH, content, com.google.api.services.run.v1alpha1.model.PubSub.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/pubsubs/[^/]+$");
}
}
@Override
public ReplacePubSub set$Xgafv(java.lang.String $Xgafv) {
return (ReplacePubSub) super.set$Xgafv($Xgafv);
}
@Override
public ReplacePubSub setAccessToken(java.lang.String accessToken) {
return (ReplacePubSub) super.setAccessToken(accessToken);
}
@Override
public ReplacePubSub setAlt(java.lang.String alt) {
return (ReplacePubSub) super.setAlt(alt);
}
@Override
public ReplacePubSub setCallback(java.lang.String callback) {
return (ReplacePubSub) super.setCallback(callback);
}
@Override
public ReplacePubSub setFields(java.lang.String fields) {
return (ReplacePubSub) super.setFields(fields);
}
@Override
public ReplacePubSub setKey(java.lang.String key) {
return (ReplacePubSub) super.setKey(key);
}
@Override
public ReplacePubSub setOauthToken(java.lang.String oauthToken) {
return (ReplacePubSub) super.setOauthToken(oauthToken);
}
@Override
public ReplacePubSub setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplacePubSub) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplacePubSub setQuotaUser(java.lang.String quotaUser) {
return (ReplacePubSub) super.setQuotaUser(quotaUser);
}
@Override
public ReplacePubSub setUploadType(java.lang.String uploadType) {
return (ReplacePubSub) super.setUploadType(uploadType);
}
@Override
public ReplacePubSub setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplacePubSub) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the pubsub being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public ReplacePubSub setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/pubsubs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ReplacePubSub set(String parameterName, Object value) {
return (ReplacePubSub) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Revisions collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Revisions.List request = run.revisions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Revisions revisions() {
return new Revisions();
}
/**
* The "revisions" collection of methods.
*/
public class Revisions {
/**
* Rpc to delete a revision.
*
* Create a request for the method "revisions.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the revision being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/revisions/[^/]+$");
/**
* Rpc to delete a revision.
*
* Create a request for the method "revisions.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the revision being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/revisions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the revision being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the revision being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the revision being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/revisions/[^/]+$");
}
this.name = name;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
@com.google.api.client.util.Key
private java.lang.Boolean orphanDependents;
/** Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading behavior,
so this must be false. This attribute is deprecated, and is now replaced with PropagationPolicy See
https://github.com/kubernetes/kubernetes/issues/46659 for more info.
*/
public java.lang.Boolean getOrphanDependents() {
return orphanDependents;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
public Delete setOrphanDependents(java.lang.Boolean orphanDependents) {
this.orphanDependents = orphanDependents;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a revision.
*
* Create a request for the method "revisions.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the revision being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/revisions/[^/]+$");
/**
* Rpc to get information about a revision.
*
* Create a request for the method "revisions.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the revision being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Revision.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/revisions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the revision being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the revision being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the revision being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/revisions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list revisions.
*
* Create a request for the method "revisions.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the revisions should be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+parent}/revisions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list revisions.
*
* Create a request for the method "revisions.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the revisions should be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListRevisionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number from which the revisions should be listed. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the revisions should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number from which the revisions should be listed. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Routes collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Routes.List request = run.routes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Routes routes() {
return new Routes();
}
/**
* The "routes" collection of methods.
*/
public class Routes {
/**
* Rpc to get information about a route.
*
* Create a request for the method "routes.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the route being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/routes/[^/]+$");
/**
* Rpc to get information about a route.
*
* Create a request for the method "routes.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the route being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Route.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/routes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the route being retrieved. If needed, replace {namespace_id} with the project
* ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the route being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the route being retrieved. If needed, replace {namespace_id} with the project
* ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/routes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list routes.
*
* Create a request for the method "routes.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the routes should be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+parent}/routes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list routes.
*
* Create a request for the method "routes.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the routes should be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListRoutesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number from which the routes should be listed. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the routes should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number from which the routes should be listed. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Services collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Services.List request = run.services().list(parameters ...)}
*
*
* @return the resource collection
*/
public Services services() {
return new Services();
}
/**
* The "services" collection of methods.
*/
public class Services {
/**
* Rpc to create a service.
*
* Create a request for the method "services.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this service should be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Service content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+parent}/services";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to create a service.
*
* Create a request for the method "services.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this service should be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Service content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Service.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number in which this service should be created. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this service should be created.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number in which this service should be created. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a service. This will cause the Service to stop serving traffic and will delete the
* child entities like Routes, Configurations and Revisions.
*
* Create a request for the method "services.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the service being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/services/[^/]+$");
/**
* Rpc to delete a service. This will cause the Service to stop serving traffic and will delete
* the child entities like Routes, Configurations and Revisions.
*
* Create a request for the method "services.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the service being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/services/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the service being deleted. If needed, replace {namespace_id} with the project
* ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the service being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the service being deleted. If needed, replace {namespace_id} with the project
* ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
@com.google.api.client.util.Key
private java.lang.Boolean orphanDependents;
/** Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading behavior,
so this must be false. This attribute is deprecated, and is now replaced with PropagationPolicy See
https://github.com/kubernetes/kubernetes/issues/46659 for more info.
*/
public java.lang.Boolean getOrphanDependents() {
return orphanDependents;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
public Delete setOrphanDependents(java.lang.Boolean orphanDependents) {
this.orphanDependents = orphanDependents;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a service.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the service being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/services/[^/]+$");
/**
* Rpc to get information about a service.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the service being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Service.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/services/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the service being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the service being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the service being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list services.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the services should be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+parent}/services";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list services.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the services should be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListServicesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number from which the services should be listed. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the services should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number from which the services should be listed. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Rpc to replace a service.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request, Cloud
* Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic concurrency
* control.
*
* Create a request for the method "services.replaceService".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ReplaceService#execute()} method to invoke the remote operation.
*
* @param name The name of the service being replaced. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @return the request
*/
public ReplaceService replaceService(java.lang.String name, com.google.api.services.run.v1alpha1.model.Service content) throws java.io.IOException {
ReplaceService result = new ReplaceService(name, content);
initialize(result);
return result;
}
public class ReplaceService extends CloudRunRequest {
private static final String REST_PATH = "apis/serving.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/services/[^/]+$");
/**
* Rpc to replace a service.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request,
* Cloud Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic
* concurrency control.
*
* Create a request for the method "services.replaceService".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ReplaceService#execute()} method to invoke the remote operation.
* {@link ReplaceService#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name The name of the service being replaced. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @since 1.13
*/
protected ReplaceService(java.lang.String name, com.google.api.services.run.v1alpha1.model.Service content) {
super(CloudRun.this, "PUT", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Service.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/services/[^/]+$");
}
}
@Override
public ReplaceService set$Xgafv(java.lang.String $Xgafv) {
return (ReplaceService) super.set$Xgafv($Xgafv);
}
@Override
public ReplaceService setAccessToken(java.lang.String accessToken) {
return (ReplaceService) super.setAccessToken(accessToken);
}
@Override
public ReplaceService setAlt(java.lang.String alt) {
return (ReplaceService) super.setAlt(alt);
}
@Override
public ReplaceService setCallback(java.lang.String callback) {
return (ReplaceService) super.setCallback(callback);
}
@Override
public ReplaceService setFields(java.lang.String fields) {
return (ReplaceService) super.setFields(fields);
}
@Override
public ReplaceService setKey(java.lang.String key) {
return (ReplaceService) super.setKey(key);
}
@Override
public ReplaceService setOauthToken(java.lang.String oauthToken) {
return (ReplaceService) super.setOauthToken(oauthToken);
}
@Override
public ReplaceService setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplaceService) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplaceService setQuotaUser(java.lang.String quotaUser) {
return (ReplaceService) super.setQuotaUser(quotaUser);
}
@Override
public ReplaceService setUploadType(java.lang.String uploadType) {
return (ReplaceService) super.setUploadType(uploadType);
}
@Override
public ReplaceService setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplaceService) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the service being replaced. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the service being replaced. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the service being replaced. If needed, replace {namespace_id} with the
* project ID.
*/
public ReplaceService setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ReplaceService set(String parameterName, Object value) {
return (ReplaceService) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Triggers collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Triggers.List request = run.triggers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Triggers triggers() {
return new Triggers();
}
/**
* The "triggers" collection of methods.
*/
public class Triggers {
/**
* Creates a new trigger.
*
* Create a request for the method "triggers.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this trigger should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Trigger content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "apis/eventing.knative.dev/v1alpha1/{+parent}/triggers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Creates a new trigger.
*
* Create a request for the method "triggers.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this trigger should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Trigger content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Trigger.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number in which this trigger should be created.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this trigger should be created.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number in which this trigger should be created.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a trigger.
*
* Create a request for the method "triggers.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "apis/eventing.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/triggers/[^/]+$");
/**
* Rpc to delete a trigger.
*
* Create a request for the method "triggers.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the trigger being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/triggers/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being deleted. If needed, replace {namespace_id} with the project
* ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being deleted. If needed, replace {namespace_id} with the project
* ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/triggers/[^/]+$");
}
this.name = name;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and
* deletes in the background. Please see kubernetes.io/docs/concepts/workloads/controllers
* /garbage-collection/ for more information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a trigger.
*
* Create a request for the method "triggers.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "apis/eventing.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/triggers/[^/]+$");
/**
* Rpc to get information about a trigger.
*
* Create a request for the method "triggers.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Trigger.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/triggers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/triggers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list triggers.
*
* Create a request for the method "triggers.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the triggers should
be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "apis/eventing.knative.dev/v1alpha1/{+parent}/triggers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+$");
/**
* Rpc to list triggers.
*
* Create a request for the method "triggers.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the triggers should
be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListTriggersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the triggers should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the triggers should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the triggers should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^namespaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not currently
* used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists, in,
* and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Rpc to replace a trigger.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request, Cloud
* Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic concurrency
* control.
*
* Create a request for the method "triggers.replaceTrigger".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ReplaceTrigger#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @return the request
*/
public ReplaceTrigger replaceTrigger(java.lang.String name, com.google.api.services.run.v1alpha1.model.Trigger content) throws java.io.IOException {
ReplaceTrigger result = new ReplaceTrigger(name, content);
initialize(result);
return result;
}
public class ReplaceTrigger extends CloudRunRequest {
private static final String REST_PATH = "apis/eventing.knative.dev/v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^namespaces/[^/]+/triggers/[^/]+$");
/**
* Rpc to replace a trigger.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request,
* Cloud Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic
* concurrency control.
*
* Create a request for the method "triggers.replaceTrigger".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ReplaceTrigger#execute()} method to invoke the remote operation.
* {@link ReplaceTrigger#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @since 1.13
*/
protected ReplaceTrigger(java.lang.String name, com.google.api.services.run.v1alpha1.model.Trigger content) {
super(CloudRun.this, "PUT", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Trigger.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/triggers/[^/]+$");
}
}
@Override
public ReplaceTrigger set$Xgafv(java.lang.String $Xgafv) {
return (ReplaceTrigger) super.set$Xgafv($Xgafv);
}
@Override
public ReplaceTrigger setAccessToken(java.lang.String accessToken) {
return (ReplaceTrigger) super.setAccessToken(accessToken);
}
@Override
public ReplaceTrigger setAlt(java.lang.String alt) {
return (ReplaceTrigger) super.setAlt(alt);
}
@Override
public ReplaceTrigger setCallback(java.lang.String callback) {
return (ReplaceTrigger) super.setCallback(callback);
}
@Override
public ReplaceTrigger setFields(java.lang.String fields) {
return (ReplaceTrigger) super.setFields(fields);
}
@Override
public ReplaceTrigger setKey(java.lang.String key) {
return (ReplaceTrigger) super.setKey(key);
}
@Override
public ReplaceTrigger setOauthToken(java.lang.String oauthToken) {
return (ReplaceTrigger) super.setOauthToken(oauthToken);
}
@Override
public ReplaceTrigger setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplaceTrigger) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplaceTrigger setQuotaUser(java.lang.String quotaUser) {
return (ReplaceTrigger) super.setQuotaUser(quotaUser);
}
@Override
public ReplaceTrigger setUploadType(java.lang.String uploadType) {
return (ReplaceTrigger) super.setUploadType(uploadType);
}
@Override
public ReplaceTrigger setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplaceTrigger) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public ReplaceTrigger setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^namespaces/[^/]+/triggers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ReplaceTrigger set(String parameterName, Object value) {
return (ReplaceTrigger) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Projects.List request = run.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Locations.List request = run.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Authorizeddomains collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Authorizeddomains.List request = run.authorizeddomains().list(parameters ...)}
*
*
* @return the resource collection
*/
public Authorizeddomains authorizeddomains() {
return new Authorizeddomains();
}
/**
* The "authorizeddomains" collection of methods.
*/
public class Authorizeddomains {
/**
* RPC to list authorized domains.
*
* Create a request for the method "authorizeddomains.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Name of the parent Application resource. Example: `apps/myapp`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/authorizeddomains";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* RPC to list authorized domains.
*
* Create a request for the method "authorizeddomains.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Name of the parent Application resource. Example: `apps/myapp`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListAuthorizedDomainsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Name of the parent Application resource. Example: `apps/myapp`. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Name of the parent Application resource. Example: `apps/myapp`.
*/
public java.lang.String getParent() {
return parent;
}
/** Name of the parent Application resource. Example: `apps/myapp`. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Continuation token for fetching the next page of results. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation token for fetching the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation token for fetching the next page of results. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum results to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum results to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum results to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Configurations collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Configurations.List request = run.configurations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Configurations configurations() {
return new Configurations();
}
/**
* The "configurations" collection of methods.
*/
public class Configurations {
/**
* Rpc to get information about a configuration.
*
* Create a request for the method "configurations.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the configuration being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/configurations/[^/]+$");
/**
* Rpc to get information about a configuration.
*
* Create a request for the method "configurations.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the configuration being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Configuration.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/configurations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the configuration being retrieved. If needed, replace {namespace_id} with
* the project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the configuration being retrieved. If needed, replace {namespace_id} with the project
ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the configuration being retrieved. If needed, replace {namespace_id} with
* the project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/configurations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list configurations.
*
* Create a request for the method "configurations.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the configurations should be
listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/configurations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list configurations.
*
* Create a request for the method "configurations.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the configurations should be
listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListConfigurationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the configurations should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the configurations should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the configurations should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Domainmappings collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Domainmappings.List request = run.domainmappings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Domainmappings domainmappings() {
return new Domainmappings();
}
/**
* The "domainmappings" collection of methods.
*/
public class Domainmappings {
/**
* Creates a new domain mapping.
*
* Create a request for the method "domainmappings.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this domain mapping should be
created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.DomainMapping}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.DomainMapping content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/domainmappings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new domain mapping.
*
* Create a request for the method "domainmappings.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this domain mapping should be
created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.DomainMapping}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.DomainMapping content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.DomainMapping.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number in which this domain mapping should be created.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this domain mapping should be created.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number in which this domain mapping should be created.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a domain mapping.
*
* Create a request for the method "domainmappings.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the domain mapping being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/domainmappings/[^/]+$");
/**
* Rpc to delete a domain mapping.
*
* Create a request for the method "domainmappings.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the domain mapping being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/domainmappings/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the domain mapping being deleted. If needed, replace {namespace_id} with
* the project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the domain mapping being deleted. If needed, replace {namespace_id} with the project
ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the domain mapping being deleted. If needed, replace {namespace_id} with
* the project ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/domainmappings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
@com.google.api.client.util.Key
private java.lang.Boolean orphanDependents;
/** Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading behavior,
so this must be false. This attribute is deprecated, and is now replaced with PropagationPolicy See
https://github.com/kubernetes/kubernetes/issues/46659 for more info.
*/
public java.lang.Boolean getOrphanDependents() {
return orphanDependents;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
public Delete setOrphanDependents(java.lang.Boolean orphanDependents) {
this.orphanDependents = orphanDependents;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a domain mapping.
*
* Create a request for the method "domainmappings.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the domain mapping being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/domainmappings/[^/]+$");
/**
* Rpc to get information about a domain mapping.
*
* Create a request for the method "domainmappings.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the domain mapping being retrieved. If needed, replace
{namespace_id} with the project
* ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.DomainMapping.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/domainmappings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the domain mapping being retrieved. If needed, replace {namespace_id} with
* the project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the domain mapping being retrieved. If needed, replace {namespace_id} with the project
ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the domain mapping being retrieved. If needed, replace {namespace_id} with
* the project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/domainmappings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list domain mappings.
*
* Create a request for the method "domainmappings.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the domain mappings should be
listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/domainmappings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list domain mappings.
*
* Create a request for the method "domainmappings.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the domain mappings should be
listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListDomainMappingsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the domain mappings should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the domain mappings should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the domain mappings should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Eventtypes collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Eventtypes.List request = run.eventtypes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Eventtypes eventtypes() {
return new Eventtypes();
}
/**
* The "eventtypes" collection of methods.
*/
public class Eventtypes {
/**
* Rpc to get information about an EventType.
*
* Create a request for the method "eventtypes.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/eventtypes/[^/]+$");
/**
* Rpc to get information about an EventType.
*
* Create a request for the method "eventtypes.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.EventType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/eventtypes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/eventtypes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list EventTypes.
*
* Create a request for the method "eventtypes.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the EventTypes should be
listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/eventtypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list EventTypes.
*
* Create a request for the method "eventtypes.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the EventTypes should be
listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListEventTypesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the EventTypes should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the EventTypes should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the EventTypes should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Pubsubs collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Pubsubs.List request = run.pubsubs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Pubsubs pubsubs() {
return new Pubsubs();
}
/**
* The "pubsubs" collection of methods.
*/
public class Pubsubs {
/**
* Creates a new pubsub.
*
* Create a request for the method "pubsubs.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this pubsub should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.PubSub content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/pubsubs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new pubsub.
*
* Create a request for the method "pubsubs.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this pubsub should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.PubSub content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.PubSub.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number in which this pubsub should be created.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this pubsub should be created.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number in which this pubsub should be created.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a pubsub.
*
* Create a request for the method "pubsubs.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the pubsub being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
/**
* Rpc to delete a pubsub.
*
* Create a request for the method "pubsubs.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the pubsub being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the pubsub being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the pubsub being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the pubsub being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
}
this.name = name;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a pubsub.
*
* Create a request for the method "pubsubs.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
/**
* Rpc to get information about a pubsub.
*
* Create a request for the method "pubsubs.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.PubSub.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the pubsub being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list pubsubs.
*
* Create a request for the method "pubsubs.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the pubsubs should
be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/pubsubs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list pubsubs.
*
* Create a request for the method "pubsubs.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the pubsubs should
be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListPubSubsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the pubsubs should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the pubsubs should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the pubsubs should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Rpc to replace a pubsub.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request, Cloud
* Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic concurrency
* control.
*
* Create a request for the method "pubsubs.replacePubSub".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ReplacePubSub#execute()} method to invoke the remote operation.
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @return the request
*/
public ReplacePubSub replacePubSub(java.lang.String name, com.google.api.services.run.v1alpha1.model.PubSub content) throws java.io.IOException {
ReplacePubSub result = new ReplacePubSub(name, content);
initialize(result);
return result;
}
public class ReplacePubSub extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
/**
* Rpc to replace a pubsub.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request,
* Cloud Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic
* concurrency control.
*
* Create a request for the method "pubsubs.replacePubSub".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ReplacePubSub#execute()} method to invoke the remote operation.
* {@link ReplacePubSub#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param name The name of the pubsub being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.PubSub}
* @since 1.13
*/
protected ReplacePubSub(java.lang.String name, com.google.api.services.run.v1alpha1.model.PubSub content) {
super(CloudRun.this, "PUT", REST_PATH, content, com.google.api.services.run.v1alpha1.model.PubSub.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
}
}
@Override
public ReplacePubSub set$Xgafv(java.lang.String $Xgafv) {
return (ReplacePubSub) super.set$Xgafv($Xgafv);
}
@Override
public ReplacePubSub setAccessToken(java.lang.String accessToken) {
return (ReplacePubSub) super.setAccessToken(accessToken);
}
@Override
public ReplacePubSub setAlt(java.lang.String alt) {
return (ReplacePubSub) super.setAlt(alt);
}
@Override
public ReplacePubSub setCallback(java.lang.String callback) {
return (ReplacePubSub) super.setCallback(callback);
}
@Override
public ReplacePubSub setFields(java.lang.String fields) {
return (ReplacePubSub) super.setFields(fields);
}
@Override
public ReplacePubSub setKey(java.lang.String key) {
return (ReplacePubSub) super.setKey(key);
}
@Override
public ReplacePubSub setOauthToken(java.lang.String oauthToken) {
return (ReplacePubSub) super.setOauthToken(oauthToken);
}
@Override
public ReplacePubSub setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplacePubSub) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplacePubSub setQuotaUser(java.lang.String quotaUser) {
return (ReplacePubSub) super.setQuotaUser(quotaUser);
}
@Override
public ReplacePubSub setUploadType(java.lang.String uploadType) {
return (ReplacePubSub) super.setUploadType(uploadType);
}
@Override
public ReplacePubSub setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplacePubSub) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the pubsub being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the pubsub being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public ReplacePubSub setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/pubsubs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ReplacePubSub set(String parameterName, Object value) {
return (ReplacePubSub) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Revisions collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Revisions.List request = run.revisions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Revisions revisions() {
return new Revisions();
}
/**
* The "revisions" collection of methods.
*/
public class Revisions {
/**
* Rpc to delete a revision.
*
* Create a request for the method "revisions.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the revision being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/revisions/[^/]+$");
/**
* Rpc to delete a revision.
*
* Create a request for the method "revisions.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the revision being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/revisions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the revision being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the revision being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the revision being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/revisions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
@com.google.api.client.util.Key
private java.lang.Boolean orphanDependents;
/** Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading behavior,
so this must be false. This attribute is deprecated, and is now replaced with PropagationPolicy See
https://github.com/kubernetes/kubernetes/issues/46659 for more info.
*/
public java.lang.Boolean getOrphanDependents() {
return orphanDependents;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
public Delete setOrphanDependents(java.lang.Boolean orphanDependents) {
this.orphanDependents = orphanDependents;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a revision.
*
* Create a request for the method "revisions.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the revision being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/revisions/[^/]+$");
/**
* Rpc to get information about a revision.
*
* Create a request for the method "revisions.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the revision being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Revision.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/revisions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the revision being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the revision being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the revision being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/revisions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list revisions.
*
* Create a request for the method "revisions.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the revisions should be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/revisions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list revisions.
*
* Create a request for the method "revisions.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the revisions should be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListRevisionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number from which the revisions should be listed. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the revisions should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number from which the revisions should be listed. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Routes collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Routes.List request = run.routes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Routes routes() {
return new Routes();
}
/**
* The "routes" collection of methods.
*/
public class Routes {
/**
* Rpc to get information about a route.
*
* Create a request for the method "routes.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the route being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/routes/[^/]+$");
/**
* Rpc to get information about a route.
*
* Create a request for the method "routes.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the route being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Route.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/routes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the route being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the route being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the route being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/routes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list routes.
*
* Create a request for the method "routes.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the routes should be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/routes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list routes.
*
* Create a request for the method "routes.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the routes should be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListRoutesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number from which the routes should be listed. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the routes should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number from which the routes should be listed. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Services collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Services.List request = run.services().list(parameters ...)}
*
*
* @return the resource collection
*/
public Services services() {
return new Services();
}
/**
* The "services" collection of methods.
*/
public class Services {
/**
* Rpc to create a service.
*
* Create a request for the method "services.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this service should be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Service content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/services";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to create a service.
*
* Create a request for the method "services.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this service should be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Service content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Service.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number in which this service should be created. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this service should be created.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number in which this service should be created. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a service. This will cause the Service to stop serving traffic and will delete the
* child entities like Routes, Configurations and Revisions.
*
* Create a request for the method "services.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the service being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Rpc to delete a service. This will cause the Service to stop serving traffic and will delete
* the child entities like Routes, Configurations and Revisions.
*
* Create a request for the method "services.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the service being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the service being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the service being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the service being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
@com.google.api.client.util.Key
private java.lang.Boolean orphanDependents;
/** Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading behavior,
so this must be false. This attribute is deprecated, and is now replaced with PropagationPolicy See
https://github.com/kubernetes/kubernetes/issues/46659 for more info.
*/
public java.lang.Boolean getOrphanDependents() {
return orphanDependents;
}
/**
* Deprecated. Specifies the cascade behavior on delete. Cloud Run only supports cascading
* behavior, so this must be false. This attribute is deprecated, and is now replaced with
* PropagationPolicy See https://github.com/kubernetes/kubernetes/issues/46659 for more
* info.
*/
public Delete setOrphanDependents(java.lang.Boolean orphanDependents) {
this.orphanDependents = orphanDependents;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a service.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the service being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Rpc to get information about a service.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the service being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Service.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the service being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the service being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the service being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Get the IAM Access Control policy currently in effect for the given Cloud Run service. This
* result does not include any inherited policies.
*
* Create a request for the method "services.getIamPolicy".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested.
See the operation documentation for
* the appropriate value for this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Get the IAM Access Control policy currently in effect for the given Cloud Run service. This
* result does not include any inherited policies.
*
* Create a request for the method "services.getIamPolicy".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested.
See the operation documentation for
* the appropriate value for this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The policy format version to be returned.
*
* Valid values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
*
* Requests for policies with any conditional bindings must specify version 3. Policies
* without any conditional bindings may specify any valid value or leave the field unset.
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The policy format version to be returned.
Valid values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
Requests for policies with any conditional bindings must specify version 3. Policies without any
conditional bindings may specify any valid value or leave the field unset.
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The policy format version to be returned.
*
* Valid values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
*
* Requests for policies with any conditional bindings must specify version 3. Policies
* without any conditional bindings may specify any valid value or leave the field unset.
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Rpc to list services.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the services should be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/services";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list services.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the services should be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListServicesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID or project number from which the services should be listed. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the services should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/** The project ID or project number from which the services should be listed. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Rpc to replace a service.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request, Cloud
* Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic concurrency
* control.
*
* Create a request for the method "services.replaceService".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ReplaceService#execute()} method to invoke the remote operation.
*
* @param name The name of the service being replaced. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @return the request
*/
public ReplaceService replaceService(java.lang.String name, com.google.api.services.run.v1alpha1.model.Service content) throws java.io.IOException {
ReplaceService result = new ReplaceService(name, content);
initialize(result);
return result;
}
public class ReplaceService extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Rpc to replace a service.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request,
* Cloud Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic
* concurrency control.
*
* Create a request for the method "services.replaceService".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ReplaceService#execute()} method to invoke the remote operation.
* {@link ReplaceService#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name The name of the service being replaced. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Service}
* @since 1.13
*/
protected ReplaceService(java.lang.String name, com.google.api.services.run.v1alpha1.model.Service content) {
super(CloudRun.this, "PUT", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Service.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public ReplaceService set$Xgafv(java.lang.String $Xgafv) {
return (ReplaceService) super.set$Xgafv($Xgafv);
}
@Override
public ReplaceService setAccessToken(java.lang.String accessToken) {
return (ReplaceService) super.setAccessToken(accessToken);
}
@Override
public ReplaceService setAlt(java.lang.String alt) {
return (ReplaceService) super.setAlt(alt);
}
@Override
public ReplaceService setCallback(java.lang.String callback) {
return (ReplaceService) super.setCallback(callback);
}
@Override
public ReplaceService setFields(java.lang.String fields) {
return (ReplaceService) super.setFields(fields);
}
@Override
public ReplaceService setKey(java.lang.String key) {
return (ReplaceService) super.setKey(key);
}
@Override
public ReplaceService setOauthToken(java.lang.String oauthToken) {
return (ReplaceService) super.setOauthToken(oauthToken);
}
@Override
public ReplaceService setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplaceService) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplaceService setQuotaUser(java.lang.String quotaUser) {
return (ReplaceService) super.setQuotaUser(quotaUser);
}
@Override
public ReplaceService setUploadType(java.lang.String uploadType) {
return (ReplaceService) super.setUploadType(uploadType);
}
@Override
public ReplaceService setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplaceService) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the service being replaced. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the service being replaced. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the service being replaced. If needed, replace {namespace_id} with the
* project ID.
*/
public ReplaceService setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ReplaceService set(String parameterName, Object value) {
return (ReplaceService) super.set(parameterName, value);
}
}
/**
* Sets the IAM Access control policy for the specified Service. Overwrites any existing policy.
*
* Create a request for the method "services.setIamPolicy".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.run.v1alpha1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.run.v1alpha1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Sets the IAM Access control policy for the specified Service. Overwrites any existing policy.
*
* Create a request for the method "services.setIamPolicy".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified.
See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.run.v1alpha1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.run.v1alpha1.model.SetIamPolicyRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified Project.
*
* There are no permissions required for making this API call.
*
* Create a request for the method "services.testIamPermissions".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested.
See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.run.v1alpha1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.run.v1alpha1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Returns permissions that a caller has on the specified Project.
*
* There are no permissions required for making this API call.
*
* Create a request for the method "services.testIamPermissions".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested.
See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.run.v1alpha1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.run.v1alpha1.model.TestIamPermissionsRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Triggers collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Triggers.List request = run.triggers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Triggers triggers() {
return new Triggers();
}
/**
* The "triggers" collection of methods.
*/
public class Triggers {
/**
* Creates a new trigger.
*
* Create a request for the method "triggers.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number in which this trigger should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Trigger content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/triggers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new trigger.
*
* Create a request for the method "triggers.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number in which this trigger should
be created.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v1alpha1.model.Trigger content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Trigger.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number in which this trigger should be created.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number in which this trigger should be created.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number in which this trigger should be created.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Rpc to delete a trigger.
*
* Create a request for the method "triggers.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being deleted. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
/**
* Rpc to delete a trigger.
*
* Create a request for the method "triggers.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the trigger being deleted. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being deleted. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being deleted. If needed, replace {namespace_id} with the
* project ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
}
this.name = name;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/** Cloud Run currently ignores this parameter. */
public Delete setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
@com.google.api.client.util.Key
private java.lang.String propagationPolicy;
/** Specifies the propagation policy of delete. Cloud Run currently ignores this setting, and deletes
in the background. Please see kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/
for more information.
*/
public java.lang.String getPropagationPolicy() {
return propagationPolicy;
}
/**
* Specifies the propagation policy of delete. Cloud Run currently ignores this setting,
* and deletes in the background. Please see
* kubernetes.io/docs/concepts/workloads/controllers/garbage-collection/ for more
* information.
*/
public Delete setPropagationPolicy(java.lang.String propagationPolicy) {
this.propagationPolicy = propagationPolicy;
return this;
}
/** Cloud Run currently ignores this parameter. */
@com.google.api.client.util.Key
private java.lang.String kind;
/** Cloud Run currently ignores this parameter.
*/
public java.lang.String getKind() {
return kind;
}
/** Cloud Run currently ignores this parameter. */
public Delete setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Rpc to get information about a trigger.
*
* Create a request for the method "triggers.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
/**
* Rpc to get information about a trigger.
*
* Create a request for the method "triggers.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.Trigger.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Rpc to list triggers.
*
* Create a request for the method "triggers.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The project ID or project number from which the triggers should
be listed.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+parent}/triggers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Rpc to list triggers.
*
* Create a request for the method "triggers.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The project ID or project number from which the triggers should
be listed.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v1alpha1.model.ListTriggersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The project ID or project number from which the triggers should be listed.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The project ID or project number from which the triggers should be listed.
*/
public java.lang.String getParent() {
return parent;
}
/**
* The project ID or project number from which the triggers should be listed.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.Boolean watch;
/** Flag that indicates that the client expects to watch this resource as well. Not currently used by
Cloud Run.
*/
public java.lang.Boolean getWatch() {
return watch;
}
/**
* Flag that indicates that the client expects to watch this resource as well. Not
* currently used by Cloud Run.
*/
public List setWatch(java.lang.Boolean watch) {
this.watch = watch;
return this;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
@com.google.api.client.util.Key
private java.lang.String labelSelector;
/** Allows to filter resources based on a label. Supported operations are =, !=, exists, in, and notIn.
*/
public java.lang.String getLabelSelector() {
return labelSelector;
}
/**
* Allows to filter resources based on a label. Supported operations are =, !=, exists,
* in, and notIn.
*/
public List setLabelSelector(java.lang.String labelSelector) {
this.labelSelector = labelSelector;
return this;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/** The baseline resource version from which the list or watch operation should start. Not currently
used by Cloud Run.
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* The baseline resource version from which the list or watch operation should start. Not
* currently used by Cloud Run.
*/
public List setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/** Not currently used by Cloud Run. */
@com.google.api.client.util.Key
private java.lang.Boolean includeUninitialized;
/** Not currently used by Cloud Run.
*/
public java.lang.Boolean getIncludeUninitialized() {
return includeUninitialized;
}
/** Not currently used by Cloud Run. */
public List setIncludeUninitialized(java.lang.Boolean includeUninitialized) {
this.includeUninitialized = includeUninitialized;
return this;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
@com.google.api.client.util.Key
private java.lang.String fieldSelector;
/** Allows to filter resources based on a specific value for a field name. Send this in a query string
format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public java.lang.String getFieldSelector() {
return fieldSelector;
}
/**
* Allows to filter resources based on a specific value for a field name. Send this in a
* query string format. i.e. 'metadata.name%3Dlorem'. Not currently used by Cloud Run.
*/
public List setFieldSelector(java.lang.String fieldSelector) {
this.fieldSelector = fieldSelector;
return this;
}
/** Optional encoded string to continue paging. */
@com.google.api.client.util.Key("continue")
private java.lang.String continue__;
/** Optional encoded string to continue paging.
*/
public java.lang.String getContinue() {
return continue__;
}
/** Optional encoded string to continue paging. */
public List setContinue(java.lang.String continue__) {
this.continue__ = continue__;
return this;
}
/** The maximum number of records that should be returned. */
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of records that should be returned.
*/
public java.lang.Integer getLimit() {
return limit;
}
/** The maximum number of records that should be returned. */
public List setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Rpc to replace a trigger.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request, Cloud
* Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic concurrency
* control.
*
* Create a request for the method "triggers.replaceTrigger".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ReplaceTrigger#execute()} method to invoke the remote operation.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @return the request
*/
public ReplaceTrigger replaceTrigger(java.lang.String name, com.google.api.services.run.v1alpha1.model.Trigger content) throws java.io.IOException {
ReplaceTrigger result = new ReplaceTrigger(name, content);
initialize(result);
return result;
}
public class ReplaceTrigger extends CloudRunRequest {
private static final String REST_PATH = "v1alpha1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
/**
* Rpc to replace a trigger.
*
* Only the spec and metadata labels and annotations are modifiable. After the Update request,
* Cloud Run will work to make the 'status' match the requested 'spec'.
*
* May provide metadata.resourceVersion to enforce update from last read for optimistic
* concurrency control.
*
* Create a request for the method "triggers.replaceTrigger".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ReplaceTrigger#execute()} method to invoke the remote operation.
* {@link ReplaceTrigger#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name The name of the trigger being retrieved. If needed, replace
{namespace_id} with the project ID.
* @param content the {@link com.google.api.services.run.v1alpha1.model.Trigger}
* @since 1.13
*/
protected ReplaceTrigger(java.lang.String name, com.google.api.services.run.v1alpha1.model.Trigger content) {
super(CloudRun.this, "PUT", REST_PATH, content, com.google.api.services.run.v1alpha1.model.Trigger.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
}
}
@Override
public ReplaceTrigger set$Xgafv(java.lang.String $Xgafv) {
return (ReplaceTrigger) super.set$Xgafv($Xgafv);
}
@Override
public ReplaceTrigger setAccessToken(java.lang.String accessToken) {
return (ReplaceTrigger) super.setAccessToken(accessToken);
}
@Override
public ReplaceTrigger setAlt(java.lang.String alt) {
return (ReplaceTrigger) super.setAlt(alt);
}
@Override
public ReplaceTrigger setCallback(java.lang.String callback) {
return (ReplaceTrigger) super.setCallback(callback);
}
@Override
public ReplaceTrigger setFields(java.lang.String fields) {
return (ReplaceTrigger) super.setFields(fields);
}
@Override
public ReplaceTrigger setKey(java.lang.String key) {
return (ReplaceTrigger) super.setKey(key);
}
@Override
public ReplaceTrigger setOauthToken(java.lang.String oauthToken) {
return (ReplaceTrigger) super.setOauthToken(oauthToken);
}
@Override
public ReplaceTrigger setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplaceTrigger) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplaceTrigger setQuotaUser(java.lang.String quotaUser) {
return (ReplaceTrigger) super.setQuotaUser(quotaUser);
}
@Override
public ReplaceTrigger setUploadType(java.lang.String uploadType) {
return (ReplaceTrigger) super.setUploadType(uploadType);
}
@Override
public ReplaceTrigger setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplaceTrigger) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the trigger being retrieved. If needed, replace {namespace_id} with the project ID.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the trigger being retrieved. If needed, replace {namespace_id} with the
* project ID.
*/
public ReplaceTrigger setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/triggers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ReplaceTrigger set(String parameterName, Object value) {
return (ReplaceTrigger) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link CloudRun}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link CloudRun}. */
@Override
public CloudRun build() {
return new CloudRun(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudRunRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudRunRequestInitializer(
CloudRunRequestInitializer cloudrunRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudrunRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}