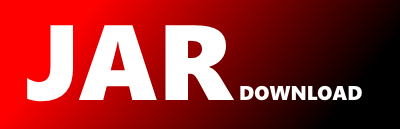
com.google.api.services.run.v1alpha1.model.ObjectMeta Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2019-11-08 at 00:46:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.run.v1alpha1.model;
/**
* ObjectMeta is metadata that all persisted resources must have, which includes all objects users
* must create.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ObjectMeta extends com.google.api.client.json.GenericJson {
/**
* Annotations is an unstructured key value map stored with a resource that may be set by external
* tools to store and retrieve arbitrary metadata. They are not queryable and should be preserved
* when modifying objects. More info: http://kubernetes.io/docs/user-guide/annotations +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map annotations;
/**
* Not currently supported by Cloud Run.
*
* The name of the cluster which the object belongs to. This is used to distinguish resources with
* same name and namespace in different clusters. This field is not set anywhere right now and
* apiserver is going to ignore it if set in create or update request. +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clusterName;
/**
* CreationTimestamp is a timestamp representing the server time when this object was created. It
* is not guaranteed to be set in happens-before order across separate operations. Clients may not
* set this value. It is represented in RFC3339 form and is in UTC.
*
* Populated by the system. Read-only. Null for lists. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#metadata +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String creationTimestamp;
/**
* Not currently supported by Cloud Run.
*
* Number of seconds allowed for this object to gracefully terminate before it will be removed
* from the system. Only set when deletionTimestamp is also set. May only be shortened. Read-only.
* +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer deletionGracePeriodSeconds;
/**
* DeletionTimestamp is RFC 3339 date and time at which this resource will be deleted. This field
* is set by the server when a graceful deletion is requested by the user, and is not directly
* settable by a client. The resource is expected to be deleted (no longer visible from resource
* lists, and not reachable by name) after the time in this field, once the finalizers list is
* empty. As long as the finalizers list contains items, deletion is blocked. Once the
* deletionTimestamp is set, this value may not be unset or be set further into the future,
* although it may be shortened or the resource may be deleted prior to this time. For example, a
* user may request that a pod is deleted in 30 seconds. The Kubelet will react by sending a
* graceful termination signal to the containers in the pod. After that 30 seconds, the Kubelet
* will send a hard termination signal (SIGKILL) to the container and after cleanup, remove the
* pod from the API. In the presence of network partitions, this object may still exist after this
* timestamp, until an administrator or automated process can determine the resource is fully
* terminated. If not set, graceful deletion of the object has not been requested.
*
* Populated by the system when a graceful deletion is requested. Read-only. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#metadata +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String deletionTimestamp;
/**
* Not currently supported by Cloud Run.
*
* Must be empty before the object is deleted from the registry. Each entry is an identifier for
* the responsible component that will remove the entry from the list. If the deletionTimestamp of
* the object is non-nil, entries in this list can only be removed. +optional +patchStrategy=merge
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List finalizers;
/**
* Not currently supported by Cloud Run.
*
* GenerateName is an optional prefix, used by the server, to generate a unique name ONLY IF the
* Name field has not been provided. If this field is used, the name returned to the client will
* be different than the name passed. This value will also be combined with a unique suffix. The
* provided value has the same validation rules as the Name field, and may be truncated by the
* length of the suffix required to make the value unique on the server.
*
* If this field is specified and the generated name exists, the server will NOT return a 409 -
* instead, it will either return 201 Created or 500 with Reason ServerTimeout indicating a unique
* name could not be found in the time allotted, and the client should retry (optionally after the
* time indicated in the Retry-After header).
*
* Applied only if Name is not specified. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#idempotency +optional
* string generateName = 2;
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String generateName;
/**
* A sequence number representing a specific generation of the desired state. Populated by the
* system. Read-only. +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer generation;
/**
* Not currently supported by Cloud Run.
*
* An initializer is a controller which enforces some system invariant at object creation time.
* This field is a list of initializers that have not yet acted on this object. If nil or empty,
* this object has been completely initialized. Otherwise, the object is considered uninitialized
* and is hidden (in list/watch and get calls) from clients that haven't explicitly asked to
* observe uninitialized objects.
*
* When an object is created, the system will populate this list with the current set of
* initializers. Only privileged users may set or modify this list. Once it is empty, it may not
* be modified further by any user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Initializers initializers;
/**
* Map of string keys and values that can be used to organize and categorize (scope and select)
* objects. May match selectors of replication controllers and routes. More info:
* http://kubernetes.io/docs/user-guide/labels +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Name must be unique within a namespace, within a Cloud Run region. Is required when creating
* resources, although some resources may allow a client to request the generation of an
* appropriate name automatically. Name is primarily intended for creation idempotence and
* configuration definition. Cannot be updated. More info: http://kubernetes.io/docs/user-
* guide/identifiers#names +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Namespace defines the space within each name must be unique, within a Cloud Run region. In
* Cloud Run the namespace must be equal to either the project ID or project number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String namespace;
/**
* List of objects that own this object. If ALL objects in the list have been deleted, this object
* will be garbage collected. +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List ownerReferences;
/**
* An opaque value that represents the internal version of this object that can be used by clients
* to determine when objects have changed. May be used for optimistic concurrency, change
* detection, and the watch operation on a resource or set of resources. Clients must treat these
* values as opaque and passed unmodified back to the server. They may only be valid for a
* particular resource or set of resources.
*
* Populated by the system. Read-only. Value must be treated as opaque by clients and . More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#concurrency-control-and-
* consistency +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/**
* SelfLink is a URL representing this object. Populated by the system. Read-only. +optional
* string selfLink = 4;
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* UID is the unique in time and space value for this object. It is typically generated by the
* server on successful creation of a resource and is not allowed to change on PUT operations.
*
* Populated by the system. Read-only. More info: http://kubernetes.io/docs/user-
* guide/identifiers#uids +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* Annotations is an unstructured key value map stored with a resource that may be set by external
* tools to store and retrieve arbitrary metadata. They are not queryable and should be preserved
* when modifying objects. More info: http://kubernetes.io/docs/user-guide/annotations +optional
* @return value or {@code null} for none
*/
public java.util.Map getAnnotations() {
return annotations;
}
/**
* Annotations is an unstructured key value map stored with a resource that may be set by external
* tools to store and retrieve arbitrary metadata. They are not queryable and should be preserved
* when modifying objects. More info: http://kubernetes.io/docs/user-guide/annotations +optional
* @param annotations annotations or {@code null} for none
*/
public ObjectMeta setAnnotations(java.util.Map annotations) {
this.annotations = annotations;
return this;
}
/**
* Not currently supported by Cloud Run.
*
* The name of the cluster which the object belongs to. This is used to distinguish resources with
* same name and namespace in different clusters. This field is not set anywhere right now and
* apiserver is going to ignore it if set in create or update request. +optional
* @return value or {@code null} for none
*/
public java.lang.String getClusterName() {
return clusterName;
}
/**
* Not currently supported by Cloud Run.
*
* The name of the cluster which the object belongs to. This is used to distinguish resources with
* same name and namespace in different clusters. This field is not set anywhere right now and
* apiserver is going to ignore it if set in create or update request. +optional
* @param clusterName clusterName or {@code null} for none
*/
public ObjectMeta setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* CreationTimestamp is a timestamp representing the server time when this object was created. It
* is not guaranteed to be set in happens-before order across separate operations. Clients may not
* set this value. It is represented in RFC3339 form and is in UTC.
*
* Populated by the system. Read-only. Null for lists. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#metadata +optional
* @return value or {@code null} for none
*/
public String getCreationTimestamp() {
return creationTimestamp;
}
/**
* CreationTimestamp is a timestamp representing the server time when this object was created. It
* is not guaranteed to be set in happens-before order across separate operations. Clients may not
* set this value. It is represented in RFC3339 form and is in UTC.
*
* Populated by the system. Read-only. Null for lists. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#metadata +optional
* @param creationTimestamp creationTimestamp or {@code null} for none
*/
public ObjectMeta setCreationTimestamp(String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
return this;
}
/**
* Not currently supported by Cloud Run.
*
* Number of seconds allowed for this object to gracefully terminate before it will be removed
* from the system. Only set when deletionTimestamp is also set. May only be shortened. Read-only.
* +optional
* @return value or {@code null} for none
*/
public java.lang.Integer getDeletionGracePeriodSeconds() {
return deletionGracePeriodSeconds;
}
/**
* Not currently supported by Cloud Run.
*
* Number of seconds allowed for this object to gracefully terminate before it will be removed
* from the system. Only set when deletionTimestamp is also set. May only be shortened. Read-only.
* +optional
* @param deletionGracePeriodSeconds deletionGracePeriodSeconds or {@code null} for none
*/
public ObjectMeta setDeletionGracePeriodSeconds(java.lang.Integer deletionGracePeriodSeconds) {
this.deletionGracePeriodSeconds = deletionGracePeriodSeconds;
return this;
}
/**
* DeletionTimestamp is RFC 3339 date and time at which this resource will be deleted. This field
* is set by the server when a graceful deletion is requested by the user, and is not directly
* settable by a client. The resource is expected to be deleted (no longer visible from resource
* lists, and not reachable by name) after the time in this field, once the finalizers list is
* empty. As long as the finalizers list contains items, deletion is blocked. Once the
* deletionTimestamp is set, this value may not be unset or be set further into the future,
* although it may be shortened or the resource may be deleted prior to this time. For example, a
* user may request that a pod is deleted in 30 seconds. The Kubelet will react by sending a
* graceful termination signal to the containers in the pod. After that 30 seconds, the Kubelet
* will send a hard termination signal (SIGKILL) to the container and after cleanup, remove the
* pod from the API. In the presence of network partitions, this object may still exist after this
* timestamp, until an administrator or automated process can determine the resource is fully
* terminated. If not set, graceful deletion of the object has not been requested.
*
* Populated by the system when a graceful deletion is requested. Read-only. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#metadata +optional
* @return value or {@code null} for none
*/
public String getDeletionTimestamp() {
return deletionTimestamp;
}
/**
* DeletionTimestamp is RFC 3339 date and time at which this resource will be deleted. This field
* is set by the server when a graceful deletion is requested by the user, and is not directly
* settable by a client. The resource is expected to be deleted (no longer visible from resource
* lists, and not reachable by name) after the time in this field, once the finalizers list is
* empty. As long as the finalizers list contains items, deletion is blocked. Once the
* deletionTimestamp is set, this value may not be unset or be set further into the future,
* although it may be shortened or the resource may be deleted prior to this time. For example, a
* user may request that a pod is deleted in 30 seconds. The Kubelet will react by sending a
* graceful termination signal to the containers in the pod. After that 30 seconds, the Kubelet
* will send a hard termination signal (SIGKILL) to the container and after cleanup, remove the
* pod from the API. In the presence of network partitions, this object may still exist after this
* timestamp, until an administrator or automated process can determine the resource is fully
* terminated. If not set, graceful deletion of the object has not been requested.
*
* Populated by the system when a graceful deletion is requested. Read-only. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#metadata +optional
* @param deletionTimestamp deletionTimestamp or {@code null} for none
*/
public ObjectMeta setDeletionTimestamp(String deletionTimestamp) {
this.deletionTimestamp = deletionTimestamp;
return this;
}
/**
* Not currently supported by Cloud Run.
*
* Must be empty before the object is deleted from the registry. Each entry is an identifier for
* the responsible component that will remove the entry from the list. If the deletionTimestamp of
* the object is non-nil, entries in this list can only be removed. +optional +patchStrategy=merge
* @return value or {@code null} for none
*/
public java.util.List getFinalizers() {
return finalizers;
}
/**
* Not currently supported by Cloud Run.
*
* Must be empty before the object is deleted from the registry. Each entry is an identifier for
* the responsible component that will remove the entry from the list. If the deletionTimestamp of
* the object is non-nil, entries in this list can only be removed. +optional +patchStrategy=merge
* @param finalizers finalizers or {@code null} for none
*/
public ObjectMeta setFinalizers(java.util.List finalizers) {
this.finalizers = finalizers;
return this;
}
/**
* Not currently supported by Cloud Run.
*
* GenerateName is an optional prefix, used by the server, to generate a unique name ONLY IF the
* Name field has not been provided. If this field is used, the name returned to the client will
* be different than the name passed. This value will also be combined with a unique suffix. The
* provided value has the same validation rules as the Name field, and may be truncated by the
* length of the suffix required to make the value unique on the server.
*
* If this field is specified and the generated name exists, the server will NOT return a 409 -
* instead, it will either return 201 Created or 500 with Reason ServerTimeout indicating a unique
* name could not be found in the time allotted, and the client should retry (optionally after the
* time indicated in the Retry-After header).
*
* Applied only if Name is not specified. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#idempotency +optional
* string generateName = 2;
* @return value or {@code null} for none
*/
public java.lang.String getGenerateName() {
return generateName;
}
/**
* Not currently supported by Cloud Run.
*
* GenerateName is an optional prefix, used by the server, to generate a unique name ONLY IF the
* Name field has not been provided. If this field is used, the name returned to the client will
* be different than the name passed. This value will also be combined with a unique suffix. The
* provided value has the same validation rules as the Name field, and may be truncated by the
* length of the suffix required to make the value unique on the server.
*
* If this field is specified and the generated name exists, the server will NOT return a 409 -
* instead, it will either return 201 Created or 500 with Reason ServerTimeout indicating a unique
* name could not be found in the time allotted, and the client should retry (optionally after the
* time indicated in the Retry-After header).
*
* Applied only if Name is not specified. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#idempotency +optional
* string generateName = 2;
* @param generateName generateName or {@code null} for none
*/
public ObjectMeta setGenerateName(java.lang.String generateName) {
this.generateName = generateName;
return this;
}
/**
* A sequence number representing a specific generation of the desired state. Populated by the
* system. Read-only. +optional
* @return value or {@code null} for none
*/
public java.lang.Integer getGeneration() {
return generation;
}
/**
* A sequence number representing a specific generation of the desired state. Populated by the
* system. Read-only. +optional
* @param generation generation or {@code null} for none
*/
public ObjectMeta setGeneration(java.lang.Integer generation) {
this.generation = generation;
return this;
}
/**
* Not currently supported by Cloud Run.
*
* An initializer is a controller which enforces some system invariant at object creation time.
* This field is a list of initializers that have not yet acted on this object. If nil or empty,
* this object has been completely initialized. Otherwise, the object is considered uninitialized
* and is hidden (in list/watch and get calls) from clients that haven't explicitly asked to
* observe uninitialized objects.
*
* When an object is created, the system will populate this list with the current set of
* initializers. Only privileged users may set or modify this list. Once it is empty, it may not
* be modified further by any user.
* @return value or {@code null} for none
*/
public Initializers getInitializers() {
return initializers;
}
/**
* Not currently supported by Cloud Run.
*
* An initializer is a controller which enforces some system invariant at object creation time.
* This field is a list of initializers that have not yet acted on this object. If nil or empty,
* this object has been completely initialized. Otherwise, the object is considered uninitialized
* and is hidden (in list/watch and get calls) from clients that haven't explicitly asked to
* observe uninitialized objects.
*
* When an object is created, the system will populate this list with the current set of
* initializers. Only privileged users may set or modify this list. Once it is empty, it may not
* be modified further by any user.
* @param initializers initializers or {@code null} for none
*/
public ObjectMeta setInitializers(Initializers initializers) {
this.initializers = initializers;
return this;
}
/**
* Map of string keys and values that can be used to organize and categorize (scope and select)
* objects. May match selectors of replication controllers and routes. More info:
* http://kubernetes.io/docs/user-guide/labels +optional
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Map of string keys and values that can be used to organize and categorize (scope and select)
* objects. May match selectors of replication controllers and routes. More info:
* http://kubernetes.io/docs/user-guide/labels +optional
* @param labels labels or {@code null} for none
*/
public ObjectMeta setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Name must be unique within a namespace, within a Cloud Run region. Is required when creating
* resources, although some resources may allow a client to request the generation of an
* appropriate name automatically. Name is primarily intended for creation idempotence and
* configuration definition. Cannot be updated. More info: http://kubernetes.io/docs/user-
* guide/identifiers#names +optional
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name must be unique within a namespace, within a Cloud Run region. Is required when creating
* resources, although some resources may allow a client to request the generation of an
* appropriate name automatically. Name is primarily intended for creation idempotence and
* configuration definition. Cannot be updated. More info: http://kubernetes.io/docs/user-
* guide/identifiers#names +optional
* @param name name or {@code null} for none
*/
public ObjectMeta setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Namespace defines the space within each name must be unique, within a Cloud Run region. In
* Cloud Run the namespace must be equal to either the project ID or project number.
* @return value or {@code null} for none
*/
public java.lang.String getNamespace() {
return namespace;
}
/**
* Namespace defines the space within each name must be unique, within a Cloud Run region. In
* Cloud Run the namespace must be equal to either the project ID or project number.
* @param namespace namespace or {@code null} for none
*/
public ObjectMeta setNamespace(java.lang.String namespace) {
this.namespace = namespace;
return this;
}
/**
* List of objects that own this object. If ALL objects in the list have been deleted, this object
* will be garbage collected. +optional
* @return value or {@code null} for none
*/
public java.util.List getOwnerReferences() {
return ownerReferences;
}
/**
* List of objects that own this object. If ALL objects in the list have been deleted, this object
* will be garbage collected. +optional
* @param ownerReferences ownerReferences or {@code null} for none
*/
public ObjectMeta setOwnerReferences(java.util.List ownerReferences) {
this.ownerReferences = ownerReferences;
return this;
}
/**
* An opaque value that represents the internal version of this object that can be used by clients
* to determine when objects have changed. May be used for optimistic concurrency, change
* detection, and the watch operation on a resource or set of resources. Clients must treat these
* values as opaque and passed unmodified back to the server. They may only be valid for a
* particular resource or set of resources.
*
* Populated by the system. Read-only. Value must be treated as opaque by clients and . More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#concurrency-control-and-
* consistency +optional
* @return value or {@code null} for none
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* An opaque value that represents the internal version of this object that can be used by clients
* to determine when objects have changed. May be used for optimistic concurrency, change
* detection, and the watch operation on a resource or set of resources. Clients must treat these
* values as opaque and passed unmodified back to the server. They may only be valid for a
* particular resource or set of resources.
*
* Populated by the system. Read-only. Value must be treated as opaque by clients and . More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#concurrency-control-and-
* consistency +optional
* @param resourceVersion resourceVersion or {@code null} for none
*/
public ObjectMeta setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/**
* SelfLink is a URL representing this object. Populated by the system. Read-only. +optional
* string selfLink = 4;
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* SelfLink is a URL representing this object. Populated by the system. Read-only. +optional
* string selfLink = 4;
* @param selfLink selfLink or {@code null} for none
*/
public ObjectMeta setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
/**
* UID is the unique in time and space value for this object. It is typically generated by the
* server on successful creation of a resource and is not allowed to change on PUT operations.
*
* Populated by the system. Read-only. More info: http://kubernetes.io/docs/user-
* guide/identifiers#uids +optional
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* UID is the unique in time and space value for this object. It is typically generated by the
* server on successful creation of a resource and is not allowed to change on PUT operations.
*
* Populated by the system. Read-only. More info: http://kubernetes.io/docs/user-
* guide/identifiers#uids +optional
* @param uid uid or {@code null} for none
*/
public ObjectMeta setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
@Override
public ObjectMeta set(String fieldName, Object value) {
return (ObjectMeta) super.set(fieldName, value);
}
@Override
public ObjectMeta clone() {
return (ObjectMeta) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy