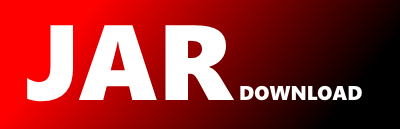
com.google.api.services.run.v1alpha1.model.ObjectReference Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2019-11-08 at 00:46:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.run.v1alpha1.model;
/**
* ObjectReference contains enough information to let you inspect or modify the referred object.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ObjectReference extends com.google.api.client.json.GenericJson {
/**
* API version of the referent. +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/**
* If referring to a piece of an object instead of an entire object, this string should contain a
* valid JSON/Go field access statement, such as desiredState.manifest.containers[2]. For example,
* if the object reference is to a container within a pod, this would take on a value like:
* "spec.containers{name}" (where "name" refers to the name of the container that triggered the
* event) or if no container name is specified "spec.containers[2]" (container with index 2 in
* this pod). This syntax is chosen only to have some well-defined way of referencing a part of an
* object.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fieldPath;
/**
* Kind of the referent. More info: https://git.k8s.io/community/contributors/devel/api-
* conventions.md#types-kinds +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Name of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-with-
* objects/names/#names +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Namespace of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-
* with-objects/namespaces/ +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String namespace;
/**
* Specific resourceVersion to which this reference is made, if any. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#concurrency-control-and-
* consistency +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resourceVersion;
/**
* UID of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-with-
* objects/names/#uids +optional
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* API version of the referent. +optional
* @return value or {@code null} for none
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/**
* API version of the referent. +optional
* @param apiVersion apiVersion or {@code null} for none
*/
public ObjectReference setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* If referring to a piece of an object instead of an entire object, this string should contain a
* valid JSON/Go field access statement, such as desiredState.manifest.containers[2]. For example,
* if the object reference is to a container within a pod, this would take on a value like:
* "spec.containers{name}" (where "name" refers to the name of the container that triggered the
* event) or if no container name is specified "spec.containers[2]" (container with index 2 in
* this pod). This syntax is chosen only to have some well-defined way of referencing a part of an
* object.
* @return value or {@code null} for none
*/
public java.lang.String getFieldPath() {
return fieldPath;
}
/**
* If referring to a piece of an object instead of an entire object, this string should contain a
* valid JSON/Go field access statement, such as desiredState.manifest.containers[2]. For example,
* if the object reference is to a container within a pod, this would take on a value like:
* "spec.containers{name}" (where "name" refers to the name of the container that triggered the
* event) or if no container name is specified "spec.containers[2]" (container with index 2 in
* this pod). This syntax is chosen only to have some well-defined way of referencing a part of an
* object.
* @param fieldPath fieldPath or {@code null} for none
*/
public ObjectReference setFieldPath(java.lang.String fieldPath) {
this.fieldPath = fieldPath;
return this;
}
/**
* Kind of the referent. More info: https://git.k8s.io/community/contributors/devel/api-
* conventions.md#types-kinds +optional
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Kind of the referent. More info: https://git.k8s.io/community/contributors/devel/api-
* conventions.md#types-kinds +optional
* @param kind kind or {@code null} for none
*/
public ObjectReference setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Name of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-with-
* objects/names/#names +optional
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-with-
* objects/names/#names +optional
* @param name name or {@code null} for none
*/
public ObjectReference setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Namespace of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-
* with-objects/namespaces/ +optional
* @return value or {@code null} for none
*/
public java.lang.String getNamespace() {
return namespace;
}
/**
* Namespace of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-
* with-objects/namespaces/ +optional
* @param namespace namespace or {@code null} for none
*/
public ObjectReference setNamespace(java.lang.String namespace) {
this.namespace = namespace;
return this;
}
/**
* Specific resourceVersion to which this reference is made, if any. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#concurrency-control-and-
* consistency +optional
* @return value or {@code null} for none
*/
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* Specific resourceVersion to which this reference is made, if any. More info:
* https://git.k8s.io/community/contributors/devel/api-conventions.md#concurrency-control-and-
* consistency +optional
* @param resourceVersion resourceVersion or {@code null} for none
*/
public ObjectReference setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
return this;
}
/**
* UID of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-with-
* objects/names/#uids +optional
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* UID of the referent. More info: https://kubernetes.io/docs/concepts/overview/working-with-
* objects/names/#uids +optional
* @param uid uid or {@code null} for none
*/
public ObjectReference setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
@Override
public ObjectReference set(String fieldName, Object value) {
return (ObjectReference) super.set(fieldName, value);
}
@Override
public ObjectReference clone() {
return (ObjectReference) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy