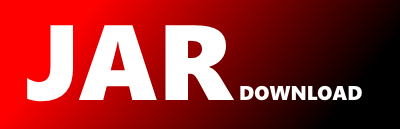
com.google.api.services.run.v2.CloudRun Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2;
/**
* Service definition for CloudRun (v2).
*
*
* Deploy and manage user provided container images that scale automatically based on incoming requests. The Cloud Run Admin API v1 follows the Knative Serving API specification, while v2 is aligned with Google Cloud AIP-based API standards, as described in https://google.aip.dev/.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudRunRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudRun extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Run Admin API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://run.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://run.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudRun(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudRun(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Projects.List request = run.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Locations.List request = run.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Export image for a given resource.
*
* Create a request for the method "locations.exportImage".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ExportImage#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the resource of which image metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2ExportImageRequest}
* @return the request
*/
public ExportImage exportImage(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2ExportImageRequest content) throws java.io.IOException {
ExportImage result = new ExportImage(name, content);
initialize(result);
return result;
}
public class ExportImage extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}:exportImage";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/.*$");
/**
* Export image for a given resource.
*
* Create a request for the method "locations.exportImage".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ExportImage#execute()} method to invoke the remote operation.
* {@link
* ExportImage#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the resource of which image metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2ExportImageRequest}
* @since 1.13
*/
protected ExportImage(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2ExportImageRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleCloudRunV2ExportImageResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/.*$");
}
}
@Override
public ExportImage set$Xgafv(java.lang.String $Xgafv) {
return (ExportImage) super.set$Xgafv($Xgafv);
}
@Override
public ExportImage setAccessToken(java.lang.String accessToken) {
return (ExportImage) super.setAccessToken(accessToken);
}
@Override
public ExportImage setAlt(java.lang.String alt) {
return (ExportImage) super.setAlt(alt);
}
@Override
public ExportImage setCallback(java.lang.String callback) {
return (ExportImage) super.setCallback(callback);
}
@Override
public ExportImage setFields(java.lang.String fields) {
return (ExportImage) super.setFields(fields);
}
@Override
public ExportImage setKey(java.lang.String key) {
return (ExportImage) super.setKey(key);
}
@Override
public ExportImage setOauthToken(java.lang.String oauthToken) {
return (ExportImage) super.setOauthToken(oauthToken);
}
@Override
public ExportImage setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportImage) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportImage setQuotaUser(java.lang.String quotaUser) {
return (ExportImage) super.setQuotaUser(quotaUser);
}
@Override
public ExportImage setUploadType(java.lang.String uploadType) {
return (ExportImage) super.setUploadType(uploadType);
}
@Override
public ExportImage setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportImage) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource of which image metadata should be exported. Format: `p
* rojects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisio
* n}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource of which image metadata should be exported. Format:
`projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision}` for
Revision `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
for Execution
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource of which image metadata should be exported. Format: `p
* rojects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisio
* n}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
*/
public ExportImage setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/.*$");
}
this.name = name;
return this;
}
@Override
public ExportImage set(String parameterName, Object value) {
return (ExportImage) super.set(parameterName, value);
}
}
/**
* Export image metadata for a given resource.
*
* Create a request for the method "locations.exportImageMetadata".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ExportImageMetadata#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the resource of which image metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @return the request
*/
public ExportImageMetadata exportImageMetadata(java.lang.String name) throws java.io.IOException {
ExportImageMetadata result = new ExportImageMetadata(name);
initialize(result);
return result;
}
public class ExportImageMetadata extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}:exportImageMetadata";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/.*$");
/**
* Export image metadata for a given resource.
*
* Create a request for the method "locations.exportImageMetadata".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ExportImageMetadata#execute()} method to invoke the remote
* operation. {@link ExportImageMetadata#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The name of the resource of which image metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @since 1.13
*/
protected ExportImageMetadata(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Metadata.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ExportImageMetadata set$Xgafv(java.lang.String $Xgafv) {
return (ExportImageMetadata) super.set$Xgafv($Xgafv);
}
@Override
public ExportImageMetadata setAccessToken(java.lang.String accessToken) {
return (ExportImageMetadata) super.setAccessToken(accessToken);
}
@Override
public ExportImageMetadata setAlt(java.lang.String alt) {
return (ExportImageMetadata) super.setAlt(alt);
}
@Override
public ExportImageMetadata setCallback(java.lang.String callback) {
return (ExportImageMetadata) super.setCallback(callback);
}
@Override
public ExportImageMetadata setFields(java.lang.String fields) {
return (ExportImageMetadata) super.setFields(fields);
}
@Override
public ExportImageMetadata setKey(java.lang.String key) {
return (ExportImageMetadata) super.setKey(key);
}
@Override
public ExportImageMetadata setOauthToken(java.lang.String oauthToken) {
return (ExportImageMetadata) super.setOauthToken(oauthToken);
}
@Override
public ExportImageMetadata setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportImageMetadata) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportImageMetadata setQuotaUser(java.lang.String quotaUser) {
return (ExportImageMetadata) super.setQuotaUser(quotaUser);
}
@Override
public ExportImageMetadata setUploadType(java.lang.String uploadType) {
return (ExportImageMetadata) super.setUploadType(uploadType);
}
@Override
public ExportImageMetadata setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportImageMetadata) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource of which image metadata should be exported. Format: `p
* rojects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisio
* n}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource of which image metadata should be exported. Format:
`projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision}` for
Revision `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
for Execution
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource of which image metadata should be exported. Format: `p
* rojects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisio
* n}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
*/
public ExportImageMetadata setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/.*$");
}
this.name = name;
return this;
}
@Override
public ExportImageMetadata set(String parameterName, Object value) {
return (ExportImageMetadata) super.set(parameterName, value);
}
}
/**
* Export generated customer metadata for a given resource.
*
* Create a request for the method "locations.exportMetadata".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ExportMetadata#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the resource of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}` for Service `pro
* jects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision}`
* for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @return the request
*/
public ExportMetadata exportMetadata(java.lang.String name) throws java.io.IOException {
ExportMetadata result = new ExportMetadata(name);
initialize(result);
return result;
}
public class ExportMetadata extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}:exportMetadata";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/.*$");
/**
* Export generated customer metadata for a given resource.
*
* Create a request for the method "locations.exportMetadata".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ExportMetadata#execute()} method to invoke the remote operation.
* {@link ExportMetadata#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The name of the resource of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}` for Service `pro
* jects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision}`
* for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @since 1.13
*/
protected ExportMetadata(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Metadata.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ExportMetadata set$Xgafv(java.lang.String $Xgafv) {
return (ExportMetadata) super.set$Xgafv($Xgafv);
}
@Override
public ExportMetadata setAccessToken(java.lang.String accessToken) {
return (ExportMetadata) super.setAccessToken(accessToken);
}
@Override
public ExportMetadata setAlt(java.lang.String alt) {
return (ExportMetadata) super.setAlt(alt);
}
@Override
public ExportMetadata setCallback(java.lang.String callback) {
return (ExportMetadata) super.setCallback(callback);
}
@Override
public ExportMetadata setFields(java.lang.String fields) {
return (ExportMetadata) super.setFields(fields);
}
@Override
public ExportMetadata setKey(java.lang.String key) {
return (ExportMetadata) super.setKey(key);
}
@Override
public ExportMetadata setOauthToken(java.lang.String oauthToken) {
return (ExportMetadata) super.setOauthToken(oauthToken);
}
@Override
public ExportMetadata setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportMetadata) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportMetadata setQuotaUser(java.lang.String quotaUser) {
return (ExportMetadata) super.setQuotaUser(quotaUser);
}
@Override
public ExportMetadata setUploadType(java.lang.String uploadType) {
return (ExportMetadata) super.setUploadType(uploadType);
}
@Override
public ExportMetadata setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportMetadata) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}` for Service `pr
* ojects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision
* }` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource of which metadata should be exported. Format:
`projects/{project_id_or_number}/locations/{location}/services/{service}` for Service
`projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision}` for
Revision `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
for Execution
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}` for Service `pr
* ojects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision
* }` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
*/
public ExportMetadata setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/.*$");
}
this.name = name;
return this;
}
@Override
public ExportMetadata set(String parameterName, Object value) {
return (ExportMetadata) super.set(parameterName, value);
}
}
/**
* Export generated customer metadata for a given project.
*
* Create a request for the method "locations.exportProjectMetadata".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ExportProjectMetadata#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the project of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}` for Project in a given location.
* @return the request
*/
public ExportProjectMetadata exportProjectMetadata(java.lang.String name) throws java.io.IOException {
ExportProjectMetadata result = new ExportProjectMetadata(name);
initialize(result);
return result;
}
public class ExportProjectMetadata extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}:exportProjectMetadata";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Export generated customer metadata for a given project.
*
* Create a request for the method "locations.exportProjectMetadata".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ExportProjectMetadata#execute()} method to invoke the remote
* operation. {@link ExportProjectMetadata#initialize(com.google.api.client.googleapis.service
* s.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The name of the project of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}` for Project in a given location.
* @since 1.13
*/
protected ExportProjectMetadata(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Metadata.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ExportProjectMetadata set$Xgafv(java.lang.String $Xgafv) {
return (ExportProjectMetadata) super.set$Xgafv($Xgafv);
}
@Override
public ExportProjectMetadata setAccessToken(java.lang.String accessToken) {
return (ExportProjectMetadata) super.setAccessToken(accessToken);
}
@Override
public ExportProjectMetadata setAlt(java.lang.String alt) {
return (ExportProjectMetadata) super.setAlt(alt);
}
@Override
public ExportProjectMetadata setCallback(java.lang.String callback) {
return (ExportProjectMetadata) super.setCallback(callback);
}
@Override
public ExportProjectMetadata setFields(java.lang.String fields) {
return (ExportProjectMetadata) super.setFields(fields);
}
@Override
public ExportProjectMetadata setKey(java.lang.String key) {
return (ExportProjectMetadata) super.setKey(key);
}
@Override
public ExportProjectMetadata setOauthToken(java.lang.String oauthToken) {
return (ExportProjectMetadata) super.setOauthToken(oauthToken);
}
@Override
public ExportProjectMetadata setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportProjectMetadata) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportProjectMetadata setQuotaUser(java.lang.String quotaUser) {
return (ExportProjectMetadata) super.setQuotaUser(quotaUser);
}
@Override
public ExportProjectMetadata setUploadType(java.lang.String uploadType) {
return (ExportProjectMetadata) super.setUploadType(uploadType);
}
@Override
public ExportProjectMetadata setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportProjectMetadata) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}` for Project in a given location.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the project of which metadata should be exported. Format:
`projects/{project_id_or_number}/locations/{location}` for Project in a given location.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the project of which metadata should be exported. Format:
* `projects/{project_id_or_number}/locations/{location}` for Project in a given location.
*/
public ExportProjectMetadata setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ExportProjectMetadata set(String parameterName, Object value) {
return (ExportProjectMetadata) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Builds collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Builds.List request = run.builds().list(parameters ...)}
*
*
* @return the resource collection
*/
public Builds builds() {
return new Builds();
}
/**
* The "builds" collection of methods.
*/
public class Builds {
/**
* Submits a build in a given project.
*
* Create a request for the method "builds.submit".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Submit#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location to build in. Location must be a region, e.g., 'us-central1' or
* 'global' if the global builder is to be used. Format:
* `projects/{project}/locations/{location}`
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2SubmitBuildRequest}
* @return the request
*/
public Submit submit(java.lang.String parent, com.google.api.services.run.v2.model.GoogleCloudRunV2SubmitBuildRequest content) throws java.io.IOException {
Submit result = new Submit(parent, content);
initialize(result);
return result;
}
public class Submit extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/builds:submit";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Submits a build in a given project.
*
* Create a request for the method "builds.submit".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Submit#execute()} method to invoke the remote operation. {@link
* Submit#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location to build in. Location must be a region, e.g., 'us-central1' or
* 'global' if the global builder is to be used. Format:
* `projects/{project}/locations/{location}`
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2SubmitBuildRequest}
* @since 1.13
*/
protected Submit(java.lang.String parent, com.google.api.services.run.v2.model.GoogleCloudRunV2SubmitBuildRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleCloudRunV2SubmitBuildResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Submit set$Xgafv(java.lang.String $Xgafv) {
return (Submit) super.set$Xgafv($Xgafv);
}
@Override
public Submit setAccessToken(java.lang.String accessToken) {
return (Submit) super.setAccessToken(accessToken);
}
@Override
public Submit setAlt(java.lang.String alt) {
return (Submit) super.setAlt(alt);
}
@Override
public Submit setCallback(java.lang.String callback) {
return (Submit) super.setCallback(callback);
}
@Override
public Submit setFields(java.lang.String fields) {
return (Submit) super.setFields(fields);
}
@Override
public Submit setKey(java.lang.String key) {
return (Submit) super.setKey(key);
}
@Override
public Submit setOauthToken(java.lang.String oauthToken) {
return (Submit) super.setOauthToken(oauthToken);
}
@Override
public Submit setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Submit) super.setPrettyPrint(prettyPrint);
}
@Override
public Submit setQuotaUser(java.lang.String quotaUser) {
return (Submit) super.setQuotaUser(quotaUser);
}
@Override
public Submit setUploadType(java.lang.String uploadType) {
return (Submit) super.setUploadType(uploadType);
}
@Override
public Submit setUploadProtocol(java.lang.String uploadProtocol) {
return (Submit) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location to build in. Location must be a region, e.g., 'us-
* central1' or 'global' if the global builder is to be used. Format:
* `projects/{project}/locations/{location}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location to build in. Location must be a region, e.g., 'us-central1' or
'global' if the global builder is to be used. Format: `projects/{project}/locations/{location}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location to build in. Location must be a region, e.g., 'us-
* central1' or 'global' if the global builder is to be used. Format:
* `projects/{project}/locations/{location}`
*/
public Submit setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Submit set(String parameterName, Object value) {
return (Submit) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Jobs collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Jobs.List request = run.jobs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Jobs jobs() {
return new Jobs();
}
/**
* The "jobs" collection of methods.
*/
public class Jobs {
/**
* Creates a Job.
*
* Create a request for the method "jobs.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The location and project in which this Job should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Job}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v2.model.GoogleCloudRunV2Job content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/jobs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a Job.
*
* Create a request for the method "jobs.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location and project in which this Job should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Job}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v2.model.GoogleCloudRunV2Job content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The location and project in which this Job should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location and project in which this Job should be created. Format:
projects/{project}/locations/{location}, where {project} can be project id or number.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The location and project in which this Job should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The unique identifier for the Job. The name of the job becomes
* {parent}/jobs/{job_id}.
*/
@com.google.api.client.util.Key
private java.lang.String jobId;
/** Required. The unique identifier for the Job. The name of the job becomes {parent}/jobs/{job_id}.
*/
public java.lang.String getJobId() {
return jobId;
}
/**
* Required. The unique identifier for the Job. The name of the job becomes
* {parent}/jobs/{job_id}.
*/
public Create setJobId(java.lang.String jobId) {
this.jobId = jobId;
return this;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or creating any resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated and default values populated, without persisting the
request or creating any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or creating any resources.
*/
public Create setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a Job.
*
* Create a request for the method "jobs.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
* where {project} can be project id or number.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Deletes a Job.
*
* Create a request for the method "jobs.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
* where {project} can be project id or number.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Job. Format:
* projects/{project}/locations/{location}/jobs/{job}, where {project} can be project id
* or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
where {project} can be project id or number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Job. Format:
* projects/{project}/locations/{location}/jobs/{job}, where {project} can be project id
* or number.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.name = name;
return this;
}
/**
* A system-generated fingerprint for this version of the resource. May be used to detect
* modification conflict during updates.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** A system-generated fingerprint for this version of the resource. May be used to detect modification
conflict during updates.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* A system-generated fingerprint for this version of the resource. May be used to detect
* modification conflict during updates.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Indicates that the request should be validated without actually deleting any resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated without actually deleting any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated without actually deleting any resources.
*/
public Delete setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about a Job.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
* where {project} can be project id or number.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Gets information about a Job.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
* where {project} can be project id or number.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Job.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Job. Format:
* projects/{project}/locations/{location}/jobs/{job}, where {project} can be project id
* or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
where {project} can be project id or number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Job. Format:
* projects/{project}/locations/{location}/jobs/{job}, where {project} can be project id
* or number.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the IAM Access Control policy currently in effect for the given Job. This result does not
* include any inherited policies.
*
* Create a request for the method "jobs.getIamPolicy".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends CloudRunRequest {
private static final String REST_PATH = "v2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Gets the IAM Access Control policy currently in effect for the given Job. This result does not
* include any inherited policies.
*
* Create a request for the method "jobs.getIamPolicy".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleIamV1Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists Jobs. Results are sorted by creation time, descending.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The location and project to list resources on. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/jobs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists Jobs. Results are sorted by creation time, descending.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location and project to list resources on. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2ListJobsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The location and project to list resources on. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location and project to list resources on. Format:
projects/{project}/locations/{location}, where {project} can be project id or number.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The location and project to list resources on. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Maximum number of Jobs to return in this call. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of Jobs to return in this call.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum number of Jobs to return in this call. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from a previous call to ListJobs. All other parameters must
* match.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from a previous call to ListJobs. All other parameters must match.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from a previous call to ListJobs. All other parameters must
* match.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** If true, returns deleted (but unexpired) resources along with active ones.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a Job.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The fully qualified name of this Job. Format: projects/{project}/locations/{location}/jobs/{job}
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Job}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2Job content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Updates a Job.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The fully qualified name of this Job. Format: projects/{project}/locations/{location}/jobs/{job}
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Job}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2Job content) {
super(CloudRun.this, "PATCH", REST_PATH, content, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The fully qualified name of this Job. Format:
* projects/{project}/locations/{location}/jobs/{job}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The fully qualified name of this Job. Format: projects/{project}/locations/{location}/jobs/{job}
*/
public java.lang.String getName() {
return name;
}
/**
* The fully qualified name of this Job. Format:
* projects/{project}/locations/{location}/jobs/{job}
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. If set to true, and if the Job does not exist, it will create a new one.
* Caller must have both create and update permissions for this call if this is set to
* true.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowMissing;
/** Optional. If set to true, and if the Job does not exist, it will create a new one. Caller must have
both create and update permissions for this call if this is set to true.
*/
public java.lang.Boolean getAllowMissing() {
return allowMissing;
}
/**
* Optional. If set to true, and if the Job does not exist, it will create a new one.
* Caller must have both create and update permissions for this call if this is set to
* true.
*/
public Patch setAllowMissing(java.lang.Boolean allowMissing) {
this.allowMissing = allowMissing;
return this;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or updating any resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated and default values populated, without persisting the
request or updating any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or updating any resources.
*/
public Patch setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Triggers creation of a new Execution of this Job.
*
* Create a request for the method "jobs.run".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Run#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
* where {project} can be project id or number.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2RunJobRequest}
* @return the request
*/
public Run run(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2RunJobRequest content) throws java.io.IOException {
Run result = new Run(name, content);
initialize(result);
return result;
}
public class Run extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}:run";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Triggers creation of a new Execution of this Job.
*
* Create a request for the method "jobs.run".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Run#execute()} method to invoke the remote operation. {@link
* Run#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
* where {project} can be project id or number.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2RunJobRequest}
* @since 1.13
*/
protected Run(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2RunJobRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public Run set$Xgafv(java.lang.String $Xgafv) {
return (Run) super.set$Xgafv($Xgafv);
}
@Override
public Run setAccessToken(java.lang.String accessToken) {
return (Run) super.setAccessToken(accessToken);
}
@Override
public Run setAlt(java.lang.String alt) {
return (Run) super.setAlt(alt);
}
@Override
public Run setCallback(java.lang.String callback) {
return (Run) super.setCallback(callback);
}
@Override
public Run setFields(java.lang.String fields) {
return (Run) super.setFields(fields);
}
@Override
public Run setKey(java.lang.String key) {
return (Run) super.setKey(key);
}
@Override
public Run setOauthToken(java.lang.String oauthToken) {
return (Run) super.setOauthToken(oauthToken);
}
@Override
public Run setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Run) super.setPrettyPrint(prettyPrint);
}
@Override
public Run setQuotaUser(java.lang.String quotaUser) {
return (Run) super.setQuotaUser(quotaUser);
}
@Override
public Run setUploadType(java.lang.String uploadType) {
return (Run) super.setUploadType(uploadType);
}
@Override
public Run setUploadProtocol(java.lang.String uploadProtocol) {
return (Run) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Job. Format:
* projects/{project}/locations/{location}/jobs/{job}, where {project} can be project id
* or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Job. Format: projects/{project}/locations/{location}/jobs/{job},
where {project} can be project id or number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Job. Format:
* projects/{project}/locations/{location}/jobs/{job}, where {project} can be project id
* or number.
*/
public Run setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Run set(String parameterName, Object value) {
return (Run) super.set(parameterName, value);
}
}
/**
* Sets the IAM Access control policy for the specified Job. Overwrites any existing policy.
*
* Create a request for the method "jobs.setIamPolicy".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends CloudRunRequest {
private static final String REST_PATH = "v2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Sets the IAM Access control policy for the specified Job. Overwrites any existing policy.
*
* Create a request for the method "jobs.setIamPolicy".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleIamV1Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified Project. There are no permissions required
* for making this API call.
*
* Create a request for the method "jobs.testIamPermissions".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends CloudRunRequest {
private static final String REST_PATH = "v2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Returns permissions that a caller has on the specified Project. There are no permissions
* required for making this API call.
*
* Create a request for the method "jobs.testIamPermissions".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Executions collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Executions.List request = run.executions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Executions executions() {
return new Executions();
}
/**
* The "executions" collection of methods.
*/
public class Executions {
/**
* Cancels an Execution.
*
* Create a request for the method "executions.cancel".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Execution to cancel. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2CancelExecutionRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2CancelExecutionRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
/**
* Cancels an Execution.
*
* Create a request for the method "executions.cancel".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Execution to cancel. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2CancelExecutionRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2CancelExecutionRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Execution to cancel. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Execution to cancel. Format:
`projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where `{project}` can
be project id or number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Execution to cancel. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
*/
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes an Execution.
*
* Create a request for the method "executions.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Execution to delete. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
/**
* Deletes an Execution.
*
* Create a request for the method "executions.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Execution to delete. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Execution to delete. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Execution to delete. Format:
`projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where `{project}` can
be project id or number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Execution to delete. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
this.name = name;
return this;
}
/**
* A system-generated fingerprint for this version of the resource. This may be used to
* detect modification conflict during updates.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** A system-generated fingerprint for this version of the resource. This may be used to detect
modification conflict during updates.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* A system-generated fingerprint for this version of the resource. This may be used to
* detect modification conflict during updates.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Indicates that the request should be validated without actually deleting any
* resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated without actually deleting any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated without actually deleting any
* resources.
*/
public Delete setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Read the status of an image export operation.
*
* Create a request for the method "executions.exportStatus".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ExportStatus#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the resource of which image export operation status has to be fetched. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @param operationId Required. The operation id returned from ExportImage.
* @return the request
*/
public ExportStatus exportStatus(java.lang.String name, java.lang.String operationId) throws java.io.IOException {
ExportStatus result = new ExportStatus(name, operationId);
initialize(result);
return result;
}
public class ExportStatus extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}/{+operationId}:exportStatus";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
private final java.util.regex.Pattern OPERATION_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Read the status of an image export operation.
*
* Create a request for the method "executions.exportStatus".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ExportStatus#execute()} method to invoke the remote operation.
* {@link
* ExportStatus#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the resource of which image export operation status has to be fetched. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @param operationId Required. The operation id returned from ExportImage.
* @since 1.13
*/
protected ExportStatus(java.lang.String name, java.lang.String operationId) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2ExportStatusResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
this.operationId = com.google.api.client.util.Preconditions.checkNotNull(operationId, "Required parameter operationId must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_ID_PATTERN.matcher(operationId).matches(),
"Parameter operationId must conform to the pattern " +
"^[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ExportStatus set$Xgafv(java.lang.String $Xgafv) {
return (ExportStatus) super.set$Xgafv($Xgafv);
}
@Override
public ExportStatus setAccessToken(java.lang.String accessToken) {
return (ExportStatus) super.setAccessToken(accessToken);
}
@Override
public ExportStatus setAlt(java.lang.String alt) {
return (ExportStatus) super.setAlt(alt);
}
@Override
public ExportStatus setCallback(java.lang.String callback) {
return (ExportStatus) super.setCallback(callback);
}
@Override
public ExportStatus setFields(java.lang.String fields) {
return (ExportStatus) super.setFields(fields);
}
@Override
public ExportStatus setKey(java.lang.String key) {
return (ExportStatus) super.setKey(key);
}
@Override
public ExportStatus setOauthToken(java.lang.String oauthToken) {
return (ExportStatus) super.setOauthToken(oauthToken);
}
@Override
public ExportStatus setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportStatus) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportStatus setQuotaUser(java.lang.String quotaUser) {
return (ExportStatus) super.setQuotaUser(quotaUser);
}
@Override
public ExportStatus setUploadType(java.lang.String uploadType) {
return (ExportStatus) super.setUploadType(uploadType);
}
@Override
public ExportStatus setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportStatus) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource of which image export operation status has to be
* fetched. Format: `projects/{project_id_or_number}/locations/{location}/services/{serv
* ice}/revisions/{revision}` for Revision `projects/{project_id_or_number}/locations/{l
* ocation}/jobs/{job}/executions/{execution}` for Execution
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource of which image export operation status has to be fetched.
Format:
`projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision}` for
Revision `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
for Execution
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource of which image export operation status has to be
* fetched. Format: `projects/{project_id_or_number}/locations/{location}/services/{serv
* ice}/revisions/{revision}` for Revision `projects/{project_id_or_number}/locations/{l
* ocation}/jobs/{job}/executions/{execution}` for Execution
*/
public ExportStatus setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
this.name = name;
return this;
}
/** Required. The operation id returned from ExportImage. */
@com.google.api.client.util.Key
private java.lang.String operationId;
/** Required. The operation id returned from ExportImage.
*/
public java.lang.String getOperationId() {
return operationId;
}
/** Required. The operation id returned from ExportImage. */
public ExportStatus setOperationId(java.lang.String operationId) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_ID_PATTERN.matcher(operationId).matches(),
"Parameter operationId must conform to the pattern " +
"^[^/]+$");
}
this.operationId = operationId;
return this;
}
@Override
public ExportStatus set(String parameterName, Object value) {
return (ExportStatus) super.set(parameterName, value);
}
}
/**
* Gets information about an Execution.
*
* Create a request for the method "executions.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Execution. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
/**
* Gets information about an Execution.
*
* Create a request for the method "executions.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Execution. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Execution.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Execution. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Execution. Format:
`projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where `{project}` can
be project id or number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Execution. Format:
* `projects/{project}/locations/{location}/jobs/{job}/executions/{execution}`, where
* `{project}` can be project id or number.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Executions from a Job. Results are sorted by creation time, descending.
*
* Create a request for the method "executions.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The Execution from which the Executions should be listed. To list all Executions across
* Jobs, use "-" instead of Job name. Format:
* `projects/{project}/locations/{location}/jobs/{job}`, where `{project}` can be project id
* or number.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/executions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
/**
* Lists Executions from a Job. Results are sorted by creation time, descending.
*
* Create a request for the method "executions.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Execution from which the Executions should be listed. To list all Executions across
* Jobs, use "-" instead of Job name. Format:
* `projects/{project}/locations/{location}/jobs/{job}`, where `{project}` can be project id
* or number.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2ListExecutionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Execution from which the Executions should be listed. To list all
* Executions across Jobs, use "-" instead of Job name. Format:
* `projects/{project}/locations/{location}/jobs/{job}`, where `{project}` can be
* project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Execution from which the Executions should be listed. To list all Executions across
Jobs, use "-" instead of Job name. Format: `projects/{project}/locations/{location}/jobs/{job}`,
where `{project}` can be project id or number.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Execution from which the Executions should be listed. To list all
* Executions across Jobs, use "-" instead of Job name. Format:
* `projects/{project}/locations/{location}/jobs/{job}`, where `{project}` can be
* project id or number.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+$");
}
this.parent = parent;
return this;
}
/** Maximum number of Executions to return in this call. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of Executions to return in this call.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum number of Executions to return in this call. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from a previous call to ListExecutions. All other parameters
* must match.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from a previous call to ListExecutions. All other parameters must match.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from a previous call to ListExecutions. All other parameters
* must match.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** If true, returns deleted (but unexpired) resources along with active ones.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Tasks collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Tasks.List request = run.tasks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tasks tasks() {
return new Tasks();
}
/**
* The "tasks" collection of methods.
*/
public class Tasks {
/**
* Gets information about a Task.
*
* Create a request for the method "tasks.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Task. Format:
* projects/{project}/locations/{location}/jobs/{job}/executions/{execution}/tasks/{task}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+/tasks/[^/]+$");
/**
* Gets information about a Task.
*
* Create a request for the method "tasks.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Task. Format:
* projects/{project}/locations/{location}/jobs/{job}/executions/{execution}/tasks/{task}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Task.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+/tasks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Task. Format: projects/{project}/locations/{location
* }/jobs/{job}/executions/{execution}/tasks/{task}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Task. Format:
projects/{project}/locations/{location}/jobs/{job}/executions/{execution}/tasks/{task}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Task. Format: projects/{project}/locations/{location
* }/jobs/{job}/executions/{execution}/tasks/{task}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+/tasks/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Tasks from an Execution of a Job.
*
* Create a request for the method "tasks.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The Execution from which the Tasks should be listed. To list all Tasks across Executions
* of a Job, use "-" instead of Execution name. To list all Tasks across Jobs, use "-"
* instead of Job name. Format:
* projects/{project}/locations/{location}/jobs/{job}/executions/{execution}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/tasks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
/**
* Lists Tasks from an Execution of a Job.
*
* Create a request for the method "tasks.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Execution from which the Tasks should be listed. To list all Tasks across Executions
* of a Job, use "-" instead of Execution name. To list all Tasks across Jobs, use "-"
* instead of Job name. Format:
* projects/{project}/locations/{location}/jobs/{job}/executions/{execution}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2ListTasksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Execution from which the Tasks should be listed. To list all Tasks
* across Executions of a Job, use "-" instead of Execution name. To list all Tasks
* across Jobs, use "-" instead of Job name. Format:
* projects/{project}/locations/{location}/jobs/{job}/executions/{execution}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Execution from which the Tasks should be listed. To list all Tasks across Executions
of a Job, use "-" instead of Execution name. To list all Tasks across Jobs, use "-" instead of Job
name. Format: projects/{project}/locations/{location}/jobs/{job}/executions/{execution}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Execution from which the Tasks should be listed. To list all Tasks
* across Executions of a Job, use "-" instead of Execution name. To list all Tasks
* across Jobs, use "-" instead of Job name. Format:
* projects/{project}/locations/{location}/jobs/{job}/executions/{execution}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/jobs/[^/]+/executions/[^/]+$");
}
this.parent = parent;
return this;
}
/** Maximum number of Tasks to return in this call. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of Tasks to return in this call.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum number of Tasks to return in this call. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from a previous call to ListTasks. All other parameters must
* match.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from a previous call to ListTasks. All other parameters must match.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from a previous call to ListTasks. All other parameters must
* match.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** If true, returns deleted (but unexpired) resources along with active ones.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Operations.List request = run.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v2.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name Required. To query for all of the operations for a project.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. To query for all of the operations for a project.
* @since 1.13
*/
protected List(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleLongrunningListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. To query for all of the operations for a project. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. To query for all of the operations for a project.
*/
public java.lang.String getName() {
return name;
}
/** Required. To query for all of the operations for a project. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A filter for matching the completed or in-progress operations. The supported
* formats of *filter* are: To query for only completed operations: done:true To query for
* only ongoing operations: done:false Must be empty to query for all of the latest
* operations for the given parent project.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter for matching the completed or in-progress operations. The supported formats of
*filter* are: To query for only completed operations: done:true To query for only ongoing
operations: done:false Must be empty to query for all of the latest operations for the given parent
project.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter for matching the completed or in-progress operations. The supported
* formats of *filter* are: To query for only completed operations: done:true To query for
* only ongoing operations: done:false Must be empty to query for all of the latest
* operations for the given parent project.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of records that should be returned. Requested page size cannot
* exceed 100. If not set or set to less than or equal to 0, the default page size is 100.
* .
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of records that should be returned. Requested page size cannot exceed 100. If
not set or set to less than or equal to 0, the default page size is 100. .
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of records that should be returned. Requested page size cannot
* exceed 100. If not set or set to less than or equal to 0, the default page size is 100.
* .
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Token identifying which result to start with, which is returned by a previous list
* call.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Token identifying which result to start with, which is returned by a previous list call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Token identifying which result to start with, which is returned by a previous list
* call.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Waits until the specified long-running operation is done or reaches at most a specified timeout,
* returning the latest state. If the operation is already done, the latest state is immediately
* returned. If the timeout specified is greater than the default HTTP/RPC timeout, the HTTP/RPC
* timeout is used. If the server does not support this method, it returns
* `google.rpc.Code.UNIMPLEMENTED`. Note that this method is on a best-effort basis. It may return
* the latest state before the specified timeout (including immediately), meaning even an immediate
* response is no guarantee that the operation is done.
*
* Create a request for the method "operations.wait".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Wait#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to wait on.
* @param content the {@link com.google.api.services.run.v2.model.GoogleLongrunningWaitOperationRequest}
* @return the request
*/
public Wait wait(java.lang.String name, com.google.api.services.run.v2.model.GoogleLongrunningWaitOperationRequest content) throws java.io.IOException {
Wait result = new Wait(name, content);
initialize(result);
return result;
}
public class Wait extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}:wait";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Waits until the specified long-running operation is done or reaches at most a specified
* timeout, returning the latest state. If the operation is already done, the latest state is
* immediately returned. If the timeout specified is greater than the default HTTP/RPC timeout,
* the HTTP/RPC timeout is used. If the server does not support this method, it returns
* `google.rpc.Code.UNIMPLEMENTED`. Note that this method is on a best-effort basis. It may return
* the latest state before the specified timeout (including immediately), meaning even an
* immediate response is no guarantee that the operation is done.
*
* Create a request for the method "operations.wait".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Wait#execute()} method to invoke the remote operation. {@link
* Wait#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to wait on.
* @param content the {@link com.google.api.services.run.v2.model.GoogleLongrunningWaitOperationRequest}
* @since 1.13
*/
protected Wait(java.lang.String name, com.google.api.services.run.v2.model.GoogleLongrunningWaitOperationRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Wait set$Xgafv(java.lang.String $Xgafv) {
return (Wait) super.set$Xgafv($Xgafv);
}
@Override
public Wait setAccessToken(java.lang.String accessToken) {
return (Wait) super.setAccessToken(accessToken);
}
@Override
public Wait setAlt(java.lang.String alt) {
return (Wait) super.setAlt(alt);
}
@Override
public Wait setCallback(java.lang.String callback) {
return (Wait) super.setCallback(callback);
}
@Override
public Wait setFields(java.lang.String fields) {
return (Wait) super.setFields(fields);
}
@Override
public Wait setKey(java.lang.String key) {
return (Wait) super.setKey(key);
}
@Override
public Wait setOauthToken(java.lang.String oauthToken) {
return (Wait) super.setOauthToken(oauthToken);
}
@Override
public Wait setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Wait) super.setPrettyPrint(prettyPrint);
}
@Override
public Wait setQuotaUser(java.lang.String quotaUser) {
return (Wait) super.setQuotaUser(quotaUser);
}
@Override
public Wait setUploadType(java.lang.String uploadType) {
return (Wait) super.setUploadType(uploadType);
}
@Override
public Wait setUploadProtocol(java.lang.String uploadProtocol) {
return (Wait) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to wait on. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to wait on.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to wait on. */
public Wait setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Wait set(String parameterName, Object value) {
return (Wait) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Services collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Services.List request = run.services().list(parameters ...)}
*
*
* @return the resource collection
*/
public Services services() {
return new Services();
}
/**
* The "services" collection of methods.
*/
public class Services {
/**
* Creates a new Service in a given project and location.
*
* Create a request for the method "services.create".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The location and project in which this service should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number. Only
* lowercase characters, digits, and hyphens.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Service}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.run.v2.model.GoogleCloudRunV2Service content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/services";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new Service in a given project and location.
*
* Create a request for the method "services.create".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location and project in which this service should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number. Only
* lowercase characters, digits, and hyphens.
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Service}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.run.v2.model.GoogleCloudRunV2Service content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The location and project in which this service should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
* Only lowercase characters, digits, and hyphens.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location and project in which this service should be created. Format:
projects/{project}/locations/{location}, where {project} can be project id or number. Only
lowercase characters, digits, and hyphens.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The location and project in which this service should be created. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
* Only lowercase characters, digits, and hyphens.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The unique identifier for the Service. It must begin with letter, and cannot
* end with hyphen; must contain fewer than 50 characters. The name of the service becomes
* {parent}/services/{service_id}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceId;
/** Required. The unique identifier for the Service. It must begin with letter, and cannot end with
hyphen; must contain fewer than 50 characters. The name of the service becomes
{parent}/services/{service_id}.
*/
public java.lang.String getServiceId() {
return serviceId;
}
/**
* Required. The unique identifier for the Service. It must begin with letter, and cannot
* end with hyphen; must contain fewer than 50 characters. The name of the service becomes
* {parent}/services/{service_id}.
*/
public Create setServiceId(java.lang.String serviceId) {
this.serviceId = serviceId;
return this;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or creating any resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated and default values populated, without persisting the
request or creating any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or creating any resources.
*/
public Create setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a Service. This will cause the Service to stop serving traffic and will delete all
* revisions.
*
* Create a request for the method "services.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be project
* id or number.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Deletes a Service. This will cause the Service to stop serving traffic and will delete all
* revisions.
*
* Create a request for the method "services.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be project
* id or number.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be
* project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Service. Format:
projects/{project}/locations/{location}/services/{service}, where {project} can be project id or
number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be
* project id or number.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
/**
* A system-generated fingerprint for this version of the resource. May be used to detect
* modification conflict during updates.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** A system-generated fingerprint for this version of the resource. May be used to detect modification
conflict during updates.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* A system-generated fingerprint for this version of the resource. May be used to detect
* modification conflict during updates.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Indicates that the request should be validated without actually deleting any resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated without actually deleting any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated without actually deleting any resources.
*/
public Delete setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about a Service.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be project
* id or number.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Gets information about a Service.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be project
* id or number.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Service.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be
* project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Service. Format:
projects/{project}/locations/{location}/services/{service}, where {project} can be project id or
number.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Service. Format:
* projects/{project}/locations/{location}/services/{service}, where {project} can be
* project id or number.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the IAM Access Control policy currently in effect for the given Cloud Run Service. This
* result does not include any inherited policies.
*
* Create a request for the method "services.getIamPolicy".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends CloudRunRequest {
private static final String REST_PATH = "v2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Gets the IAM Access Control policy currently in effect for the given Cloud Run Service. This
* result does not include any inherited policies.
*
* Create a request for the method "services.getIamPolicy".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleIamV1Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists Services. Results are sorted by creation time, descending.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The location and project to list resources on. Location must be a valid Google Cloud
* region, and cannot be the "-" wildcard. Format: projects/{project}/locations/{location},
* where {project} can be project id or number.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/services";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists Services. Results are sorted by creation time, descending.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location and project to list resources on. Location must be a valid Google Cloud
* region, and cannot be the "-" wildcard. Format: projects/{project}/locations/{location},
* where {project} can be project id or number.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2ListServicesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The location and project to list resources on. Location must be a valid
* Google Cloud region, and cannot be the "-" wildcard. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location and project to list resources on. Location must be a valid Google Cloud
region, and cannot be the "-" wildcard. Format: projects/{project}/locations/{location}, where
{project} can be project id or number.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The location and project to list resources on. Location must be a valid
* Google Cloud region, and cannot be the "-" wildcard. Format:
* projects/{project}/locations/{location}, where {project} can be project id or number.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Maximum number of Services to return in this call. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of Services to return in this call.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum number of Services to return in this call. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from a previous call to ListServices. All other parameters must
* match.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from a previous call to ListServices. All other parameters must match.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from a previous call to ListServices. All other parameters must
* match.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** If true, returns deleted (but unexpired) resources along with active ones.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a Service.
*
* Create a request for the method "services.patch".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The fully qualified name of this Service. In CreateServiceRequest, this field is ignored, and
* instead composed from CreateServiceRequest.parent and CreateServiceRequest.service_id.
* Format: projects/{project}/locations/{location}/services/{service_id}
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Service}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2Service content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Updates a Service.
*
* Create a request for the method "services.patch".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The fully qualified name of this Service. In CreateServiceRequest, this field is ignored, and
* instead composed from CreateServiceRequest.parent and CreateServiceRequest.service_id.
* Format: projects/{project}/locations/{location}/services/{service_id}
* @param content the {@link com.google.api.services.run.v2.model.GoogleCloudRunV2Service}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.run.v2.model.GoogleCloudRunV2Service content) {
super(CloudRun.this, "PATCH", REST_PATH, content, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The fully qualified name of this Service. In CreateServiceRequest, this field is
* ignored, and instead composed from CreateServiceRequest.parent and
* CreateServiceRequest.service_id. Format:
* projects/{project}/locations/{location}/services/{service_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The fully qualified name of this Service. In CreateServiceRequest, this field is ignored, and
instead composed from CreateServiceRequest.parent and CreateServiceRequest.service_id. Format:
projects/{project}/locations/{location}/services/{service_id}
*/
public java.lang.String getName() {
return name;
}
/**
* The fully qualified name of this Service. In CreateServiceRequest, this field is
* ignored, and instead composed from CreateServiceRequest.parent and
* CreateServiceRequest.service_id. Format:
* projects/{project}/locations/{location}/services/{service_id}
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. If set to true, and if the Service does not exist, it will create a new one.
* The caller must have 'run.services.create' permissions if this is set to true and the
* Service does not exist.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowMissing;
/** Optional. If set to true, and if the Service does not exist, it will create a new one. The caller
must have 'run.services.create' permissions if this is set to true and the Service does not exist.
*/
public java.lang.Boolean getAllowMissing() {
return allowMissing;
}
/**
* Optional. If set to true, and if the Service does not exist, it will create a new one.
* The caller must have 'run.services.create' permissions if this is set to true and the
* Service does not exist.
*/
public Patch setAllowMissing(java.lang.Boolean allowMissing) {
this.allowMissing = allowMissing;
return this;
}
/** Optional. The list of fields to be updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Optional. The list of fields to be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Optional. The list of fields to be updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or updating any resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated and default values populated, without persisting the
request or updating any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated and default values populated, without
* persisting the request or updating any resources.
*/
public Patch setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the IAM Access control policy for the specified Service. Overwrites any existing policy.
*
* Create a request for the method "services.setIamPolicy".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends CloudRunRequest {
private static final String REST_PATH = "v2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Sets the IAM Access control policy for the specified Service. Overwrites any existing policy.
*
* Create a request for the method "services.setIamPolicy".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1SetIamPolicyRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleIamV1Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified Project. There are no permissions required
* for making this API call.
*
* Create a request for the method "services.testIamPermissions".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends CloudRunRequest {
private static final String REST_PATH = "v2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Returns permissions that a caller has on the specified Project. There are no permissions
* required for making this API call.
*
* Create a request for the method "services.testIamPermissions".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsRequest content) {
super(CloudRun.this, "POST", REST_PATH, content, com.google.api.services.run.v2.model.GoogleIamV1TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Revisions collection.
*
* The typical use is:
*
* {@code CloudRun run = new CloudRun(...);}
* {@code CloudRun.Revisions.List request = run.revisions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Revisions revisions() {
return new Revisions();
}
/**
* The "revisions" collection of methods.
*/
public class Revisions {
/**
* Deletes a Revision.
*
* Create a request for the method "revisions.delete".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Revision to delete. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
/**
* Deletes a Revision.
*
* Create a request for the method "revisions.delete".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Revision to delete. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudRun.this, "DELETE", REST_PATH, null, com.google.api.services.run.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Revision to delete. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Revision to delete. Format:
projects/{project}/locations/{location}/services/{service}/revisions/{revision}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Revision to delete. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
}
this.name = name;
return this;
}
/**
* A system-generated fingerprint for this version of the resource. This may be used to
* detect modification conflict during updates.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** A system-generated fingerprint for this version of the resource. This may be used to detect
modification conflict during updates.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* A system-generated fingerprint for this version of the resource. This may be used to
* detect modification conflict during updates.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Indicates that the request should be validated without actually deleting any
* resources.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Indicates that the request should be validated without actually deleting any resources.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Indicates that the request should be validated without actually deleting any
* resources.
*/
public Delete setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Read the status of an image export operation.
*
* Create a request for the method "revisions.exportStatus".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link ExportStatus#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the resource of which image export operation status has to be fetched. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @param operationId Required. The operation id returned from ExportImage.
* @return the request
*/
public ExportStatus exportStatus(java.lang.String name, java.lang.String operationId) throws java.io.IOException {
ExportStatus result = new ExportStatus(name, operationId);
initialize(result);
return result;
}
public class ExportStatus extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}/{+operationId}:exportStatus";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
private final java.util.regex.Pattern OPERATION_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Read the status of an image export operation.
*
* Create a request for the method "revisions.exportStatus".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link ExportStatus#execute()} method to invoke the remote operation.
* {@link
* ExportStatus#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the resource of which image export operation status has to be fetched. Format:
* `projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revisi
* on}` for Revision
* `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
* for Execution
* @param operationId Required. The operation id returned from ExportImage.
* @since 1.13
*/
protected ExportStatus(java.lang.String name, java.lang.String operationId) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2ExportStatusResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
}
this.operationId = com.google.api.client.util.Preconditions.checkNotNull(operationId, "Required parameter operationId must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_ID_PATTERN.matcher(operationId).matches(),
"Parameter operationId must conform to the pattern " +
"^[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ExportStatus set$Xgafv(java.lang.String $Xgafv) {
return (ExportStatus) super.set$Xgafv($Xgafv);
}
@Override
public ExportStatus setAccessToken(java.lang.String accessToken) {
return (ExportStatus) super.setAccessToken(accessToken);
}
@Override
public ExportStatus setAlt(java.lang.String alt) {
return (ExportStatus) super.setAlt(alt);
}
@Override
public ExportStatus setCallback(java.lang.String callback) {
return (ExportStatus) super.setCallback(callback);
}
@Override
public ExportStatus setFields(java.lang.String fields) {
return (ExportStatus) super.setFields(fields);
}
@Override
public ExportStatus setKey(java.lang.String key) {
return (ExportStatus) super.setKey(key);
}
@Override
public ExportStatus setOauthToken(java.lang.String oauthToken) {
return (ExportStatus) super.setOauthToken(oauthToken);
}
@Override
public ExportStatus setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ExportStatus) super.setPrettyPrint(prettyPrint);
}
@Override
public ExportStatus setQuotaUser(java.lang.String quotaUser) {
return (ExportStatus) super.setQuotaUser(quotaUser);
}
@Override
public ExportStatus setUploadType(java.lang.String uploadType) {
return (ExportStatus) super.setUploadType(uploadType);
}
@Override
public ExportStatus setUploadProtocol(java.lang.String uploadProtocol) {
return (ExportStatus) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource of which image export operation status has to be
* fetched. Format: `projects/{project_id_or_number}/locations/{location}/services/{serv
* ice}/revisions/{revision}` for Revision `projects/{project_id_or_number}/locations/{l
* ocation}/jobs/{job}/executions/{execution}` for Execution
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource of which image export operation status has to be fetched.
Format:
`projects/{project_id_or_number}/locations/{location}/services/{service}/revisions/{revision}` for
Revision `projects/{project_id_or_number}/locations/{location}/jobs/{job}/executions/{execution}`
for Execution
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource of which image export operation status has to be
* fetched. Format: `projects/{project_id_or_number}/locations/{location}/services/{serv
* ice}/revisions/{revision}` for Revision `projects/{project_id_or_number}/locations/{l
* ocation}/jobs/{job}/executions/{execution}` for Execution
*/
public ExportStatus setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
}
this.name = name;
return this;
}
/** Required. The operation id returned from ExportImage. */
@com.google.api.client.util.Key
private java.lang.String operationId;
/** Required. The operation id returned from ExportImage.
*/
public java.lang.String getOperationId() {
return operationId;
}
/** Required. The operation id returned from ExportImage. */
public ExportStatus setOperationId(java.lang.String operationId) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OPERATION_ID_PATTERN.matcher(operationId).matches(),
"Parameter operationId must conform to the pattern " +
"^[^/]+$");
}
this.operationId = operationId;
return this;
}
@Override
public ExportStatus set(String parameterName, Object value) {
return (ExportStatus) super.set(parameterName, value);
}
}
/**
* Gets information about a Revision.
*
* Create a request for the method "revisions.get".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The full name of the Revision. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudRunRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
/**
* Gets information about a Revision.
*
* Create a request for the method "revisions.get".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The full name of the Revision. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2Revision.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The full name of the Revision. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full name of the Revision. Format:
projects/{project}/locations/{location}/services/{service}/revisions/{revision}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The full name of the Revision. Format:
* projects/{project}/locations/{location}/services/{service}/revisions/{revision}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+/revisions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Revisions from a given Service, or from a given location. Results are sorted by creation
* time, descending.
*
* Create a request for the method "revisions.list".
*
* This request holds the parameters needed by the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The Service from which the Revisions should be listed. To list all Revisions across
* Services, use "-" instead of Service name. Format:
* projects/{project}/locations/{location}/services/{service}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudRunRequest {
private static final String REST_PATH = "v2/{+parent}/revisions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/services/[^/]+$");
/**
* Lists Revisions from a given Service, or from a given location. Results are sorted by creation
* time, descending.
*
* Create a request for the method "revisions.list".
*
* This request holds the parameters needed by the the run server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Service from which the Revisions should be listed. To list all Revisions across
* Services, use "-" instead of Service name. Format:
* projects/{project}/locations/{location}/services/{service}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudRun.this, "GET", REST_PATH, null, com.google.api.services.run.v2.model.GoogleCloudRunV2ListRevisionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Service from which the Revisions should be listed. To list all
* Revisions across Services, use "-" instead of Service name. Format:
* projects/{project}/locations/{location}/services/{service}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Service from which the Revisions should be listed. To list all Revisions across
Services, use "-" instead of Service name. Format:
projects/{project}/locations/{location}/services/{service}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Service from which the Revisions should be listed. To list all
* Revisions across Services, use "-" instead of Service name. Format:
* projects/{project}/locations/{location}/services/{service}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/services/[^/]+$");
}
this.parent = parent;
return this;
}
/** Maximum number of revisions to return in this call. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of revisions to return in this call.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum number of revisions to return in this call. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from a previous call to ListRevisions. All other parameters
* must match.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from a previous call to ListRevisions. All other parameters must match.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from a previous call to ListRevisions. All other parameters
* must match.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
@com.google.api.client.util.Key
private java.lang.Boolean showDeleted;
/** If true, returns deleted (but unexpired) resources along with active ones.
*/
public java.lang.Boolean getShowDeleted() {
return showDeleted;
}
/** If true, returns deleted (but unexpired) resources along with active ones. */
public List setShowDeleted(java.lang.Boolean showDeleted) {
this.showDeleted = showDeleted;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
}
/**
* Builder for {@link CloudRun}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudRun}. */
@Override
public CloudRun build() {
return new CloudRun(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudRunRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudRunRequestInitializer(
CloudRunRequestInitializer cloudrunRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudrunRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}