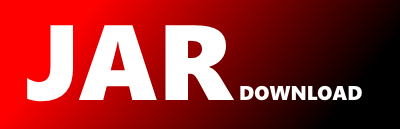
com.google.api.services.run.v2.model.GoogleCloudRunV2BinaryAuthorization Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Settings for Binary Authorization feature.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRunV2BinaryAuthorization extends com.google.api.client.json.GenericJson {
/**
* Optional. If present, indicates to use Breakglass using this justification. If use_default is
* False, then it must be empty. For more information on breakglass, see
* https://cloud.google.com/binary-authorization/docs/using-breakglass
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String breakglassJustification;
/**
* Optional. The path to a binary authorization policy. Format:
* `projects/{project}/platforms/cloudRun/{policy-name}`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String policy;
/**
* Optional. If True, indicates to use the default project's binary authorization policy. If
* False, binary authorization will be disabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useDefault;
/**
* Optional. If present, indicates to use Breakglass using this justification. If use_default is
* False, then it must be empty. For more information on breakglass, see
* https://cloud.google.com/binary-authorization/docs/using-breakglass
* @return value or {@code null} for none
*/
public java.lang.String getBreakglassJustification() {
return breakglassJustification;
}
/**
* Optional. If present, indicates to use Breakglass using this justification. If use_default is
* False, then it must be empty. For more information on breakglass, see
* https://cloud.google.com/binary-authorization/docs/using-breakglass
* @param breakglassJustification breakglassJustification or {@code null} for none
*/
public GoogleCloudRunV2BinaryAuthorization setBreakglassJustification(java.lang.String breakglassJustification) {
this.breakglassJustification = breakglassJustification;
return this;
}
/**
* Optional. The path to a binary authorization policy. Format:
* `projects/{project}/platforms/cloudRun/{policy-name}`
* @return value or {@code null} for none
*/
public java.lang.String getPolicy() {
return policy;
}
/**
* Optional. The path to a binary authorization policy. Format:
* `projects/{project}/platforms/cloudRun/{policy-name}`
* @param policy policy or {@code null} for none
*/
public GoogleCloudRunV2BinaryAuthorization setPolicy(java.lang.String policy) {
this.policy = policy;
return this;
}
/**
* Optional. If True, indicates to use the default project's binary authorization policy. If
* False, binary authorization will be disabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getUseDefault() {
return useDefault;
}
/**
* Optional. If True, indicates to use the default project's binary authorization policy. If
* False, binary authorization will be disabled.
* @param useDefault useDefault or {@code null} for none
*/
public GoogleCloudRunV2BinaryAuthorization setUseDefault(java.lang.Boolean useDefault) {
this.useDefault = useDefault;
return this;
}
@Override
public GoogleCloudRunV2BinaryAuthorization set(String fieldName, Object value) {
return (GoogleCloudRunV2BinaryAuthorization) super.set(fieldName, value);
}
@Override
public GoogleCloudRunV2BinaryAuthorization clone() {
return (GoogleCloudRunV2BinaryAuthorization) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy