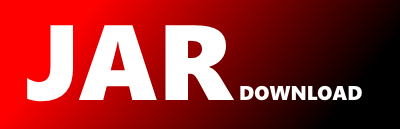
com.google.api.services.run.v2.model.GoogleCloudRunV2BuildpacksBuild Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* Build the source using Buildpacks.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRunV2BuildpacksBuild extends com.google.api.client.json.GenericJson {
/**
* Optional. The base image used to opt into automatic base image updates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String baseImage;
/**
* Optional. cache_image_uri is the GCR/AR URL where the cache image will be stored.
* cache_image_uri is optional and omitting it will disable caching. This URL must be stable
* across builds. It is used to derive a build-specific temporary URL by substituting the tag with
* the build ID. The build will clean up the temporary image on a best-effort basis.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cacheImageUri;
/**
* Optional. Whether or not the application container will be enrolled in automatic base image
* updates. When true, the application will be built on a scratch base image, so the base layers
* can be appended at run time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableAutomaticUpdates;
/**
* Optional. User-provided build-time environment variables.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map environmentVariables;
/**
* Optional. Name of the function target if the source is a function source. Required for function
* builds.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String functionTarget;
/**
* The runtime name, e.g. 'go113'. Leave blank for generic builds.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String runtime;
/**
* Optional. The base image used to opt into automatic base image updates.
* @return value or {@code null} for none
*/
public java.lang.String getBaseImage() {
return baseImage;
}
/**
* Optional. The base image used to opt into automatic base image updates.
* @param baseImage baseImage or {@code null} for none
*/
public GoogleCloudRunV2BuildpacksBuild setBaseImage(java.lang.String baseImage) {
this.baseImage = baseImage;
return this;
}
/**
* Optional. cache_image_uri is the GCR/AR URL where the cache image will be stored.
* cache_image_uri is optional and omitting it will disable caching. This URL must be stable
* across builds. It is used to derive a build-specific temporary URL by substituting the tag with
* the build ID. The build will clean up the temporary image on a best-effort basis.
* @return value or {@code null} for none
*/
public java.lang.String getCacheImageUri() {
return cacheImageUri;
}
/**
* Optional. cache_image_uri is the GCR/AR URL where the cache image will be stored.
* cache_image_uri is optional and omitting it will disable caching. This URL must be stable
* across builds. It is used to derive a build-specific temporary URL by substituting the tag with
* the build ID. The build will clean up the temporary image on a best-effort basis.
* @param cacheImageUri cacheImageUri or {@code null} for none
*/
public GoogleCloudRunV2BuildpacksBuild setCacheImageUri(java.lang.String cacheImageUri) {
this.cacheImageUri = cacheImageUri;
return this;
}
/**
* Optional. Whether or not the application container will be enrolled in automatic base image
* updates. When true, the application will be built on a scratch base image, so the base layers
* can be appended at run time.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableAutomaticUpdates() {
return enableAutomaticUpdates;
}
/**
* Optional. Whether or not the application container will be enrolled in automatic base image
* updates. When true, the application will be built on a scratch base image, so the base layers
* can be appended at run time.
* @param enableAutomaticUpdates enableAutomaticUpdates or {@code null} for none
*/
public GoogleCloudRunV2BuildpacksBuild setEnableAutomaticUpdates(java.lang.Boolean enableAutomaticUpdates) {
this.enableAutomaticUpdates = enableAutomaticUpdates;
return this;
}
/**
* Optional. User-provided build-time environment variables.
* @return value or {@code null} for none
*/
public java.util.Map getEnvironmentVariables() {
return environmentVariables;
}
/**
* Optional. User-provided build-time environment variables.
* @param environmentVariables environmentVariables or {@code null} for none
*/
public GoogleCloudRunV2BuildpacksBuild setEnvironmentVariables(java.util.Map environmentVariables) {
this.environmentVariables = environmentVariables;
return this;
}
/**
* Optional. Name of the function target if the source is a function source. Required for function
* builds.
* @return value or {@code null} for none
*/
public java.lang.String getFunctionTarget() {
return functionTarget;
}
/**
* Optional. Name of the function target if the source is a function source. Required for function
* builds.
* @param functionTarget functionTarget or {@code null} for none
*/
public GoogleCloudRunV2BuildpacksBuild setFunctionTarget(java.lang.String functionTarget) {
this.functionTarget = functionTarget;
return this;
}
/**
* The runtime name, e.g. 'go113'. Leave blank for generic builds.
* @return value or {@code null} for none
*/
public java.lang.String getRuntime() {
return runtime;
}
/**
* The runtime name, e.g. 'go113'. Leave blank for generic builds.
* @param runtime runtime or {@code null} for none
*/
public GoogleCloudRunV2BuildpacksBuild setRuntime(java.lang.String runtime) {
this.runtime = runtime;
return this;
}
@Override
public GoogleCloudRunV2BuildpacksBuild set(String fieldName, Object value) {
return (GoogleCloudRunV2BuildpacksBuild) super.set(fieldName, value);
}
@Override
public GoogleCloudRunV2BuildpacksBuild clone() {
return (GoogleCloudRunV2BuildpacksBuild) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy