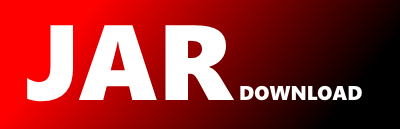
com.google.api.services.run.v2.model.GoogleCloudRunV2Container Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.run.v2.model;
/**
* A single application container. This specifies both the container to run, the command to run in
* the container and the arguments to supply to it. Note that additional arguments can be supplied
* by the system to the container at runtime.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Run Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudRunV2Container extends com.google.api.client.json.GenericJson {
/**
* Arguments to the entrypoint. The docker image's CMD is used if this is not provided.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List args;
/**
* Entrypoint array. Not executed within a shell. The docker image's ENTRYPOINT is used if this is
* not provided.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List command;
/**
* Names of the containers that must start before this container.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List dependsOn;
/**
* List of environment variables to set in the container.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List env;
/**
* Required. Name of the container image in Dockerhub, Google Artifact Registry, or Google
* Container Registry. If the host is not provided, Dockerhub is assumed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String image;
/**
* Periodic probe of container liveness. Container will be restarted if the probe fails.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2Probe livenessProbe;
/**
* Name of the container specified as a DNS_LABEL (RFC 1123).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* List of ports to expose from the container. Only a single port can be specified. The specified
* ports must be listening on all interfaces (0.0.0.0) within the container to be accessible. If
* omitted, a port number will be chosen and passed to the container through the PORT environment
* variable for the container to listen on.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List ports;
/**
* Compute Resource requirements by this container.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2ResourceRequirements resources;
/**
* Startup probe of application within the container. All other probes are disabled if a startup
* probe is provided, until it succeeds. Container will not be added to service endpoints if the
* probe fails.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudRunV2Probe startupProbe;
/**
* Volume to mount into the container's filesystem.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List volumeMounts;
/**
* Container's working directory. If not specified, the container runtime's default will be used,
* which might be configured in the container image.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String workingDir;
/**
* Arguments to the entrypoint. The docker image's CMD is used if this is not provided.
* @return value or {@code null} for none
*/
public java.util.List getArgs() {
return args;
}
/**
* Arguments to the entrypoint. The docker image's CMD is used if this is not provided.
* @param args args or {@code null} for none
*/
public GoogleCloudRunV2Container setArgs(java.util.List args) {
this.args = args;
return this;
}
/**
* Entrypoint array. Not executed within a shell. The docker image's ENTRYPOINT is used if this is
* not provided.
* @return value or {@code null} for none
*/
public java.util.List getCommand() {
return command;
}
/**
* Entrypoint array. Not executed within a shell. The docker image's ENTRYPOINT is used if this is
* not provided.
* @param command command or {@code null} for none
*/
public GoogleCloudRunV2Container setCommand(java.util.List command) {
this.command = command;
return this;
}
/**
* Names of the containers that must start before this container.
* @return value or {@code null} for none
*/
public java.util.List getDependsOn() {
return dependsOn;
}
/**
* Names of the containers that must start before this container.
* @param dependsOn dependsOn or {@code null} for none
*/
public GoogleCloudRunV2Container setDependsOn(java.util.List dependsOn) {
this.dependsOn = dependsOn;
return this;
}
/**
* List of environment variables to set in the container.
* @return value or {@code null} for none
*/
public java.util.List getEnv() {
return env;
}
/**
* List of environment variables to set in the container.
* @param env env or {@code null} for none
*/
public GoogleCloudRunV2Container setEnv(java.util.List env) {
this.env = env;
return this;
}
/**
* Required. Name of the container image in Dockerhub, Google Artifact Registry, or Google
* Container Registry. If the host is not provided, Dockerhub is assumed.
* @return value or {@code null} for none
*/
public java.lang.String getImage() {
return image;
}
/**
* Required. Name of the container image in Dockerhub, Google Artifact Registry, or Google
* Container Registry. If the host is not provided, Dockerhub is assumed.
* @param image image or {@code null} for none
*/
public GoogleCloudRunV2Container setImage(java.lang.String image) {
this.image = image;
return this;
}
/**
* Periodic probe of container liveness. Container will be restarted if the probe fails.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2Probe getLivenessProbe() {
return livenessProbe;
}
/**
* Periodic probe of container liveness. Container will be restarted if the probe fails.
* @param livenessProbe livenessProbe or {@code null} for none
*/
public GoogleCloudRunV2Container setLivenessProbe(GoogleCloudRunV2Probe livenessProbe) {
this.livenessProbe = livenessProbe;
return this;
}
/**
* Name of the container specified as a DNS_LABEL (RFC 1123).
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the container specified as a DNS_LABEL (RFC 1123).
* @param name name or {@code null} for none
*/
public GoogleCloudRunV2Container setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* List of ports to expose from the container. Only a single port can be specified. The specified
* ports must be listening on all interfaces (0.0.0.0) within the container to be accessible. If
* omitted, a port number will be chosen and passed to the container through the PORT environment
* variable for the container to listen on.
* @return value or {@code null} for none
*/
public java.util.List getPorts() {
return ports;
}
/**
* List of ports to expose from the container. Only a single port can be specified. The specified
* ports must be listening on all interfaces (0.0.0.0) within the container to be accessible. If
* omitted, a port number will be chosen and passed to the container through the PORT environment
* variable for the container to listen on.
* @param ports ports or {@code null} for none
*/
public GoogleCloudRunV2Container setPorts(java.util.List ports) {
this.ports = ports;
return this;
}
/**
* Compute Resource requirements by this container.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2ResourceRequirements getResources() {
return resources;
}
/**
* Compute Resource requirements by this container.
* @param resources resources or {@code null} for none
*/
public GoogleCloudRunV2Container setResources(GoogleCloudRunV2ResourceRequirements resources) {
this.resources = resources;
return this;
}
/**
* Startup probe of application within the container. All other probes are disabled if a startup
* probe is provided, until it succeeds. Container will not be added to service endpoints if the
* probe fails.
* @return value or {@code null} for none
*/
public GoogleCloudRunV2Probe getStartupProbe() {
return startupProbe;
}
/**
* Startup probe of application within the container. All other probes are disabled if a startup
* probe is provided, until it succeeds. Container will not be added to service endpoints if the
* probe fails.
* @param startupProbe startupProbe or {@code null} for none
*/
public GoogleCloudRunV2Container setStartupProbe(GoogleCloudRunV2Probe startupProbe) {
this.startupProbe = startupProbe;
return this;
}
/**
* Volume to mount into the container's filesystem.
* @return value or {@code null} for none
*/
public java.util.List getVolumeMounts() {
return volumeMounts;
}
/**
* Volume to mount into the container's filesystem.
* @param volumeMounts volumeMounts or {@code null} for none
*/
public GoogleCloudRunV2Container setVolumeMounts(java.util.List volumeMounts) {
this.volumeMounts = volumeMounts;
return this;
}
/**
* Container's working directory. If not specified, the container runtime's default will be used,
* which might be configured in the container image.
* @return value or {@code null} for none
*/
public java.lang.String getWorkingDir() {
return workingDir;
}
/**
* Container's working directory. If not specified, the container runtime's default will be used,
* which might be configured in the container image.
* @param workingDir workingDir or {@code null} for none
*/
public GoogleCloudRunV2Container setWorkingDir(java.lang.String workingDir) {
this.workingDir = workingDir;
return this;
}
@Override
public GoogleCloudRunV2Container set(String fieldName, Object value) {
return (GoogleCloudRunV2Container) super.set(fieldName, value);
}
@Override
public GoogleCloudRunV2Container clone() {
return (GoogleCloudRunV2Container) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy